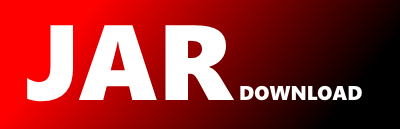
com.amazonaws.services.entityresolution.AWSEntityResolutionAsync Maven / Gradle / Ivy
Show all versions of aws-java-sdk-entityresolution Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.entityresolution;
import javax.annotation.Generated;
import com.amazonaws.services.entityresolution.model.*;
/**
* Interface for accessing AWSEntityResolution asynchronously. Each asynchronous method will return a Java Future object
* representing the asynchronous operation; overloads which accept an {@code AsyncHandler} can be used to receive
* notification when an asynchronous operation completes.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.entityresolution.AbstractAWSEntityResolutionAsync} instead.
*
*
*
* Welcome to the Entity Resolution API Reference.
*
*
* Entity Resolution is an Amazon Web Services service that provides pre-configured entity resolution capabilities that
* enable developers and analysts at advertising and marketing companies to build an accurate and complete view of their
* consumers.
*
*
* With Entity Resolution, you can match source records containing consumer identifiers, such as name, email address,
* and phone number. This is true even when these records have incomplete or conflicting identifiers. For example,
* Entity Resolution can effectively match a source record from a customer relationship management (CRM) system with a
* source record from a marketing system containing campaign information.
*
*
* To learn more about Entity Resolution concepts, procedures, and best practices, see the Entity Resolution User
* Guide.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AWSEntityResolutionAsync extends AWSEntityResolution {
/**
*
* Adds a policy statement object. To retrieve a list of existing policy statements, use the GetPolicy
* API.
*
*
* @param addPolicyStatementRequest
* @return A Java Future containing the result of the AddPolicyStatement operation returned by the service.
* @sample AWSEntityResolutionAsync.AddPolicyStatement
* @see AWS API Documentation
*/
java.util.concurrent.Future addPolicyStatementAsync(AddPolicyStatementRequest addPolicyStatementRequest);
/**
*
* Adds a policy statement object. To retrieve a list of existing policy statements, use the GetPolicy
* API.
*
*
* @param addPolicyStatementRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AddPolicyStatement operation returned by the service.
* @sample AWSEntityResolutionAsyncHandler.AddPolicyStatement
* @see AWS API Documentation
*/
java.util.concurrent.Future addPolicyStatementAsync(AddPolicyStatementRequest addPolicyStatementRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes multiple unique IDs in a matching workflow.
*
*
* @param batchDeleteUniqueIdRequest
* @return A Java Future containing the result of the BatchDeleteUniqueId operation returned by the service.
* @sample AWSEntityResolutionAsync.BatchDeleteUniqueId
* @see AWS API Documentation
*/
java.util.concurrent.Future batchDeleteUniqueIdAsync(BatchDeleteUniqueIdRequest batchDeleteUniqueIdRequest);
/**
*
* Deletes multiple unique IDs in a matching workflow.
*
*
* @param batchDeleteUniqueIdRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the BatchDeleteUniqueId operation returned by the service.
* @sample AWSEntityResolutionAsyncHandler.BatchDeleteUniqueId
* @see AWS API Documentation
*/
java.util.concurrent.Future batchDeleteUniqueIdAsync(BatchDeleteUniqueIdRequest batchDeleteUniqueIdRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates an IdMappingWorkflow
object which stores the configuration of the data processing job to be
* run. Each IdMappingWorkflow
must have a unique workflow name. To modify an existing workflow, use
* the UpdateIdMappingWorkflow
API.
*
*
* @param createIdMappingWorkflowRequest
* @return A Java Future containing the result of the CreateIdMappingWorkflow operation returned by the service.
* @sample AWSEntityResolutionAsync.CreateIdMappingWorkflow
* @see AWS API Documentation
*/
java.util.concurrent.Future createIdMappingWorkflowAsync(CreateIdMappingWorkflowRequest createIdMappingWorkflowRequest);
/**
*
* Creates an IdMappingWorkflow
object which stores the configuration of the data processing job to be
* run. Each IdMappingWorkflow
must have a unique workflow name. To modify an existing workflow, use
* the UpdateIdMappingWorkflow
API.
*
*
* @param createIdMappingWorkflowRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateIdMappingWorkflow operation returned by the service.
* @sample AWSEntityResolutionAsyncHandler.CreateIdMappingWorkflow
* @see AWS API Documentation
*/
java.util.concurrent.Future createIdMappingWorkflowAsync(CreateIdMappingWorkflowRequest createIdMappingWorkflowRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates an ID namespace object which will help customers provide metadata explaining their dataset and how to use
* it. Each ID namespace must have a unique name. To modify an existing ID namespace, use the
* UpdateIdNamespace
API.
*
*
* @param createIdNamespaceRequest
* @return A Java Future containing the result of the CreateIdNamespace operation returned by the service.
* @sample AWSEntityResolutionAsync.CreateIdNamespace
* @see AWS API Documentation
*/
java.util.concurrent.Future createIdNamespaceAsync(CreateIdNamespaceRequest createIdNamespaceRequest);
/**
*
* Creates an ID namespace object which will help customers provide metadata explaining their dataset and how to use
* it. Each ID namespace must have a unique name. To modify an existing ID namespace, use the
* UpdateIdNamespace
API.
*
*
* @param createIdNamespaceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateIdNamespace operation returned by the service.
* @sample AWSEntityResolutionAsyncHandler.CreateIdNamespace
* @see AWS API Documentation
*/
java.util.concurrent.Future createIdNamespaceAsync(CreateIdNamespaceRequest createIdNamespaceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a MatchingWorkflow
object which stores the configuration of the data processing job to be
* run. It is important to note that there should not be a pre-existing MatchingWorkflow
with the same
* name. To modify an existing workflow, utilize the UpdateMatchingWorkflow
API.
*
*
* @param createMatchingWorkflowRequest
* @return A Java Future containing the result of the CreateMatchingWorkflow operation returned by the service.
* @sample AWSEntityResolutionAsync.CreateMatchingWorkflow
* @see AWS API Documentation
*/
java.util.concurrent.Future createMatchingWorkflowAsync(CreateMatchingWorkflowRequest createMatchingWorkflowRequest);
/**
*
* Creates a MatchingWorkflow
object which stores the configuration of the data processing job to be
* run. It is important to note that there should not be a pre-existing MatchingWorkflow
with the same
* name. To modify an existing workflow, utilize the UpdateMatchingWorkflow
API.
*
*
* @param createMatchingWorkflowRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateMatchingWorkflow operation returned by the service.
* @sample AWSEntityResolutionAsyncHandler.CreateMatchingWorkflow
* @see AWS API Documentation
*/
java.util.concurrent.Future createMatchingWorkflowAsync(CreateMatchingWorkflowRequest createMatchingWorkflowRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a schema mapping, which defines the schema of the input customer records table. The
* SchemaMapping
also provides Entity Resolution with some metadata about the table, such as the
* attribute types of the columns and which columns to match on.
*
*
* @param createSchemaMappingRequest
* @return A Java Future containing the result of the CreateSchemaMapping operation returned by the service.
* @sample AWSEntityResolutionAsync.CreateSchemaMapping
* @see AWS API Documentation
*/
java.util.concurrent.Future createSchemaMappingAsync(CreateSchemaMappingRequest createSchemaMappingRequest);
/**
*
* Creates a schema mapping, which defines the schema of the input customer records table. The
* SchemaMapping
also provides Entity Resolution with some metadata about the table, such as the
* attribute types of the columns and which columns to match on.
*
*
* @param createSchemaMappingRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateSchemaMapping operation returned by the service.
* @sample AWSEntityResolutionAsyncHandler.CreateSchemaMapping
* @see AWS API Documentation
*/
java.util.concurrent.Future createSchemaMappingAsync(CreateSchemaMappingRequest createSchemaMappingRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the IdMappingWorkflow
with a given name. This operation will succeed even if a workflow with
* the given name does not exist.
*
*
* @param deleteIdMappingWorkflowRequest
* @return A Java Future containing the result of the DeleteIdMappingWorkflow operation returned by the service.
* @sample AWSEntityResolutionAsync.DeleteIdMappingWorkflow
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteIdMappingWorkflowAsync(DeleteIdMappingWorkflowRequest deleteIdMappingWorkflowRequest);
/**
*
* Deletes the IdMappingWorkflow
with a given name. This operation will succeed even if a workflow with
* the given name does not exist.
*
*
* @param deleteIdMappingWorkflowRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteIdMappingWorkflow operation returned by the service.
* @sample AWSEntityResolutionAsyncHandler.DeleteIdMappingWorkflow
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteIdMappingWorkflowAsync(DeleteIdMappingWorkflowRequest deleteIdMappingWorkflowRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the IdNamespace
with a given name.
*
*
* @param deleteIdNamespaceRequest
* @return A Java Future containing the result of the DeleteIdNamespace operation returned by the service.
* @sample AWSEntityResolutionAsync.DeleteIdNamespace
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteIdNamespaceAsync(DeleteIdNamespaceRequest deleteIdNamespaceRequest);
/**
*
* Deletes the IdNamespace
with a given name.
*
*
* @param deleteIdNamespaceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteIdNamespace operation returned by the service.
* @sample AWSEntityResolutionAsyncHandler.DeleteIdNamespace
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteIdNamespaceAsync(DeleteIdNamespaceRequest deleteIdNamespaceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the MatchingWorkflow
with a given name. This operation will succeed even if a workflow with
* the given name does not exist.
*
*
* @param deleteMatchingWorkflowRequest
* @return A Java Future containing the result of the DeleteMatchingWorkflow operation returned by the service.
* @sample AWSEntityResolutionAsync.DeleteMatchingWorkflow
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteMatchingWorkflowAsync(DeleteMatchingWorkflowRequest deleteMatchingWorkflowRequest);
/**
*
* Deletes the MatchingWorkflow
with a given name. This operation will succeed even if a workflow with
* the given name does not exist.
*
*
* @param deleteMatchingWorkflowRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteMatchingWorkflow operation returned by the service.
* @sample AWSEntityResolutionAsyncHandler.DeleteMatchingWorkflow
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteMatchingWorkflowAsync(DeleteMatchingWorkflowRequest deleteMatchingWorkflowRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the policy statement.
*
*
* @param deletePolicyStatementRequest
* @return A Java Future containing the result of the DeletePolicyStatement operation returned by the service.
* @sample AWSEntityResolutionAsync.DeletePolicyStatement
* @see AWS API Documentation
*/
java.util.concurrent.Future deletePolicyStatementAsync(DeletePolicyStatementRequest deletePolicyStatementRequest);
/**
*
* Deletes the policy statement.
*
*
* @param deletePolicyStatementRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeletePolicyStatement operation returned by the service.
* @sample AWSEntityResolutionAsyncHandler.DeletePolicyStatement
* @see AWS API Documentation
*/
java.util.concurrent.Future deletePolicyStatementAsync(DeletePolicyStatementRequest deletePolicyStatementRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the SchemaMapping
with a given name. This operation will succeed even if a schema with the
* given name does not exist. This operation will fail if there is a MatchingWorkflow
object that
* references the SchemaMapping
in the workflow's InputSourceConfig
.
*
*
* @param deleteSchemaMappingRequest
* @return A Java Future containing the result of the DeleteSchemaMapping operation returned by the service.
* @sample AWSEntityResolutionAsync.DeleteSchemaMapping
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteSchemaMappingAsync(DeleteSchemaMappingRequest deleteSchemaMappingRequest);
/**
*
* Deletes the SchemaMapping
with a given name. This operation will succeed even if a schema with the
* given name does not exist. This operation will fail if there is a MatchingWorkflow
object that
* references the SchemaMapping
in the workflow's InputSourceConfig
.
*
*
* @param deleteSchemaMappingRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteSchemaMapping operation returned by the service.
* @sample AWSEntityResolutionAsyncHandler.DeleteSchemaMapping
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteSchemaMappingAsync(DeleteSchemaMappingRequest deleteSchemaMappingRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets the status, metrics, and errors (if there are any) that are associated with a job.
*
*
* @param getIdMappingJobRequest
* @return A Java Future containing the result of the GetIdMappingJob operation returned by the service.
* @sample AWSEntityResolutionAsync.GetIdMappingJob
* @see AWS API Documentation
*/
java.util.concurrent.Future getIdMappingJobAsync(GetIdMappingJobRequest getIdMappingJobRequest);
/**
*
* Gets the status, metrics, and errors (if there are any) that are associated with a job.
*
*
* @param getIdMappingJobRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetIdMappingJob operation returned by the service.
* @sample AWSEntityResolutionAsyncHandler.GetIdMappingJob
* @see AWS API Documentation
*/
java.util.concurrent.Future getIdMappingJobAsync(GetIdMappingJobRequest getIdMappingJobRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns the IdMappingWorkflow
with a given name, if it exists.
*
*
* @param getIdMappingWorkflowRequest
* @return A Java Future containing the result of the GetIdMappingWorkflow operation returned by the service.
* @sample AWSEntityResolutionAsync.GetIdMappingWorkflow
* @see AWS API Documentation
*/
java.util.concurrent.Future getIdMappingWorkflowAsync(GetIdMappingWorkflowRequest getIdMappingWorkflowRequest);
/**
*
* Returns the IdMappingWorkflow
with a given name, if it exists.
*
*
* @param getIdMappingWorkflowRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetIdMappingWorkflow operation returned by the service.
* @sample AWSEntityResolutionAsyncHandler.GetIdMappingWorkflow
* @see AWS API Documentation
*/
java.util.concurrent.Future getIdMappingWorkflowAsync(GetIdMappingWorkflowRequest getIdMappingWorkflowRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns the IdNamespace
with a given name, if it exists.
*
*
* @param getIdNamespaceRequest
* @return A Java Future containing the result of the GetIdNamespace operation returned by the service.
* @sample AWSEntityResolutionAsync.GetIdNamespace
* @see AWS API Documentation
*/
java.util.concurrent.Future getIdNamespaceAsync(GetIdNamespaceRequest getIdNamespaceRequest);
/**
*
* Returns the IdNamespace
with a given name, if it exists.
*
*
* @param getIdNamespaceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetIdNamespace operation returned by the service.
* @sample AWSEntityResolutionAsyncHandler.GetIdNamespace
* @see AWS API Documentation
*/
java.util.concurrent.Future getIdNamespaceAsync(GetIdNamespaceRequest getIdNamespaceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns the corresponding Match ID of a customer record if the record has been processed.
*
*
* @param getMatchIdRequest
* @return A Java Future containing the result of the GetMatchId operation returned by the service.
* @sample AWSEntityResolutionAsync.GetMatchId
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getMatchIdAsync(GetMatchIdRequest getMatchIdRequest);
/**
*
* Returns the corresponding Match ID of a customer record if the record has been processed.
*
*
* @param getMatchIdRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetMatchId operation returned by the service.
* @sample AWSEntityResolutionAsyncHandler.GetMatchId
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getMatchIdAsync(GetMatchIdRequest getMatchIdRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets the status, metrics, and errors (if there are any) that are associated with a job.
*
*
* @param getMatchingJobRequest
* @return A Java Future containing the result of the GetMatchingJob operation returned by the service.
* @sample AWSEntityResolutionAsync.GetMatchingJob
* @see AWS API Documentation
*/
java.util.concurrent.Future getMatchingJobAsync(GetMatchingJobRequest getMatchingJobRequest);
/**
*
* Gets the status, metrics, and errors (if there are any) that are associated with a job.
*
*
* @param getMatchingJobRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetMatchingJob operation returned by the service.
* @sample AWSEntityResolutionAsyncHandler.GetMatchingJob
* @see AWS API Documentation
*/
java.util.concurrent.Future getMatchingJobAsync(GetMatchingJobRequest getMatchingJobRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns the MatchingWorkflow
with a given name, if it exists.
*
*
* @param getMatchingWorkflowRequest
* @return A Java Future containing the result of the GetMatchingWorkflow operation returned by the service.
* @sample AWSEntityResolutionAsync.GetMatchingWorkflow
* @see AWS API Documentation
*/
java.util.concurrent.Future getMatchingWorkflowAsync(GetMatchingWorkflowRequest getMatchingWorkflowRequest);
/**
*
* Returns the MatchingWorkflow
with a given name, if it exists.
*
*
* @param getMatchingWorkflowRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetMatchingWorkflow operation returned by the service.
* @sample AWSEntityResolutionAsyncHandler.GetMatchingWorkflow
* @see AWS API Documentation
*/
java.util.concurrent.Future getMatchingWorkflowAsync(GetMatchingWorkflowRequest getMatchingWorkflowRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns the resource-based policy.
*
*
* @param getPolicyRequest
* @return A Java Future containing the result of the GetPolicy operation returned by the service.
* @sample AWSEntityResolutionAsync.GetPolicy
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getPolicyAsync(GetPolicyRequest getPolicyRequest);
/**
*
* Returns the resource-based policy.
*
*
* @param getPolicyRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetPolicy operation returned by the service.
* @sample AWSEntityResolutionAsyncHandler.GetPolicy
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getPolicyAsync(GetPolicyRequest getPolicyRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns the SchemaMapping of a given name.
*
*
* @param getSchemaMappingRequest
* @return A Java Future containing the result of the GetSchemaMapping operation returned by the service.
* @sample AWSEntityResolutionAsync.GetSchemaMapping
* @see AWS API Documentation
*/
java.util.concurrent.Future getSchemaMappingAsync(GetSchemaMappingRequest getSchemaMappingRequest);
/**
*
* Returns the SchemaMapping of a given name.
*
*
* @param getSchemaMappingRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetSchemaMapping operation returned by the service.
* @sample AWSEntityResolutionAsyncHandler.GetSchemaMapping
* @see AWS API Documentation
*/
java.util.concurrent.Future getSchemaMappingAsync(GetSchemaMappingRequest getSchemaMappingRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists all ID mapping jobs for a given workflow.
*
*
* @param listIdMappingJobsRequest
* @return A Java Future containing the result of the ListIdMappingJobs operation returned by the service.
* @sample AWSEntityResolutionAsync.ListIdMappingJobs
* @see AWS API Documentation
*/
java.util.concurrent.Future listIdMappingJobsAsync(ListIdMappingJobsRequest listIdMappingJobsRequest);
/**
*
* Lists all ID mapping jobs for a given workflow.
*
*
* @param listIdMappingJobsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListIdMappingJobs operation returned by the service.
* @sample AWSEntityResolutionAsyncHandler.ListIdMappingJobs
* @see AWS API Documentation
*/
java.util.concurrent.Future listIdMappingJobsAsync(ListIdMappingJobsRequest listIdMappingJobsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of all the IdMappingWorkflows
that have been created for an Amazon Web Services
* account.
*
*
* @param listIdMappingWorkflowsRequest
* @return A Java Future containing the result of the ListIdMappingWorkflows operation returned by the service.
* @sample AWSEntityResolutionAsync.ListIdMappingWorkflows
* @see AWS API Documentation
*/
java.util.concurrent.Future listIdMappingWorkflowsAsync(ListIdMappingWorkflowsRequest listIdMappingWorkflowsRequest);
/**
*
* Returns a list of all the IdMappingWorkflows
that have been created for an Amazon Web Services
* account.
*
*
* @param listIdMappingWorkflowsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListIdMappingWorkflows operation returned by the service.
* @sample AWSEntityResolutionAsyncHandler.ListIdMappingWorkflows
* @see AWS API Documentation
*/
java.util.concurrent.Future listIdMappingWorkflowsAsync(ListIdMappingWorkflowsRequest listIdMappingWorkflowsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of all ID namespaces.
*
*
* @param listIdNamespacesRequest
* @return A Java Future containing the result of the ListIdNamespaces operation returned by the service.
* @sample AWSEntityResolutionAsync.ListIdNamespaces
* @see AWS API Documentation
*/
java.util.concurrent.Future listIdNamespacesAsync(ListIdNamespacesRequest listIdNamespacesRequest);
/**
*
* Returns a list of all ID namespaces.
*
*
* @param listIdNamespacesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListIdNamespaces operation returned by the service.
* @sample AWSEntityResolutionAsyncHandler.ListIdNamespaces
* @see AWS API Documentation
*/
java.util.concurrent.Future listIdNamespacesAsync(ListIdNamespacesRequest listIdNamespacesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists all jobs for a given workflow.
*
*
* @param listMatchingJobsRequest
* @return A Java Future containing the result of the ListMatchingJobs operation returned by the service.
* @sample AWSEntityResolutionAsync.ListMatchingJobs
* @see AWS API Documentation
*/
java.util.concurrent.Future listMatchingJobsAsync(ListMatchingJobsRequest listMatchingJobsRequest);
/**
*
* Lists all jobs for a given workflow.
*
*
* @param listMatchingJobsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListMatchingJobs operation returned by the service.
* @sample AWSEntityResolutionAsyncHandler.ListMatchingJobs
* @see AWS API Documentation
*/
java.util.concurrent.Future listMatchingJobsAsync(ListMatchingJobsRequest listMatchingJobsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of all the MatchingWorkflows
that have been created for an Amazon Web Services
* account.
*
*
* @param listMatchingWorkflowsRequest
* @return A Java Future containing the result of the ListMatchingWorkflows operation returned by the service.
* @sample AWSEntityResolutionAsync.ListMatchingWorkflows
* @see AWS API Documentation
*/
java.util.concurrent.Future listMatchingWorkflowsAsync(ListMatchingWorkflowsRequest listMatchingWorkflowsRequest);
/**
*
* Returns a list of all the MatchingWorkflows
that have been created for an Amazon Web Services
* account.
*
*
* @param listMatchingWorkflowsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListMatchingWorkflows operation returned by the service.
* @sample AWSEntityResolutionAsyncHandler.ListMatchingWorkflows
* @see AWS API Documentation
*/
java.util.concurrent.Future listMatchingWorkflowsAsync(ListMatchingWorkflowsRequest listMatchingWorkflowsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of all the ProviderServices
that are available in this Amazon Web Services Region.
*
*
* @param listProviderServicesRequest
* @return A Java Future containing the result of the ListProviderServices operation returned by the service.
* @sample AWSEntityResolutionAsync.ListProviderServices
* @see AWS API Documentation
*/
java.util.concurrent.Future listProviderServicesAsync(ListProviderServicesRequest listProviderServicesRequest);
/**
*
* Returns a list of all the ProviderServices
that are available in this Amazon Web Services Region.
*
*
* @param listProviderServicesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListProviderServices operation returned by the service.
* @sample AWSEntityResolutionAsyncHandler.ListProviderServices
* @see AWS API Documentation
*/
java.util.concurrent.Future listProviderServicesAsync(ListProviderServicesRequest listProviderServicesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of all the SchemaMappings
that have been created for an Amazon Web Services account.
*
*
* @param listSchemaMappingsRequest
* @return A Java Future containing the result of the ListSchemaMappings operation returned by the service.
* @sample AWSEntityResolutionAsync.ListSchemaMappings
* @see AWS API Documentation
*/
java.util.concurrent.Future listSchemaMappingsAsync(ListSchemaMappingsRequest listSchemaMappingsRequest);
/**
*
* Returns a list of all the SchemaMappings
that have been created for an Amazon Web Services account.
*
*
* @param listSchemaMappingsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListSchemaMappings operation returned by the service.
* @sample AWSEntityResolutionAsyncHandler.ListSchemaMappings
* @see AWS API Documentation
*/
java.util.concurrent.Future listSchemaMappingsAsync(ListSchemaMappingsRequest listSchemaMappingsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Displays the tags associated with an Entity Resolution resource. In Entity Resolution, SchemaMapping
* , and MatchingWorkflow
can be tagged.
*
*
* @param listTagsForResourceRequest
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AWSEntityResolutionAsync.ListTagsForResource
* @see AWS API Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest);
/**
*
* Displays the tags associated with an Entity Resolution resource. In Entity Resolution, SchemaMapping
* , and MatchingWorkflow
can be tagged.
*
*
* @param listTagsForResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AWSEntityResolutionAsyncHandler.ListTagsForResource
* @see AWS API Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates the resource-based policy.
*
*
* @param putPolicyRequest
* @return A Java Future containing the result of the PutPolicy operation returned by the service.
* @sample AWSEntityResolutionAsync.PutPolicy
* @see AWS API
* Documentation
*/
java.util.concurrent.Future putPolicyAsync(PutPolicyRequest putPolicyRequest);
/**
*
* Updates the resource-based policy.
*
*
* @param putPolicyRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the PutPolicy operation returned by the service.
* @sample AWSEntityResolutionAsyncHandler.PutPolicy
* @see AWS API
* Documentation
*/
java.util.concurrent.Future putPolicyAsync(PutPolicyRequest putPolicyRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Starts the IdMappingJob
of a workflow. The workflow must have previously been created using the
* CreateIdMappingWorkflow
endpoint.
*
*
* @param startIdMappingJobRequest
* @return A Java Future containing the result of the StartIdMappingJob operation returned by the service.
* @sample AWSEntityResolutionAsync.StartIdMappingJob
* @see AWS API Documentation
*/
java.util.concurrent.Future startIdMappingJobAsync(StartIdMappingJobRequest startIdMappingJobRequest);
/**
*
* Starts the IdMappingJob
of a workflow. The workflow must have previously been created using the
* CreateIdMappingWorkflow
endpoint.
*
*
* @param startIdMappingJobRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the StartIdMappingJob operation returned by the service.
* @sample AWSEntityResolutionAsyncHandler.StartIdMappingJob
* @see AWS API Documentation
*/
java.util.concurrent.Future startIdMappingJobAsync(StartIdMappingJobRequest startIdMappingJobRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Starts the MatchingJob
of a workflow. The workflow must have previously been created using the
* CreateMatchingWorkflow
endpoint.
*
*
* @param startMatchingJobRequest
* @return A Java Future containing the result of the StartMatchingJob operation returned by the service.
* @sample AWSEntityResolutionAsync.StartMatchingJob
* @see AWS API Documentation
*/
java.util.concurrent.Future startMatchingJobAsync(StartMatchingJobRequest startMatchingJobRequest);
/**
*
* Starts the MatchingJob
of a workflow. The workflow must have previously been created using the
* CreateMatchingWorkflow
endpoint.
*
*
* @param startMatchingJobRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the StartMatchingJob operation returned by the service.
* @sample AWSEntityResolutionAsyncHandler.StartMatchingJob
* @see AWS API Documentation
*/
java.util.concurrent.Future startMatchingJobAsync(StartMatchingJobRequest startMatchingJobRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Assigns one or more tags (key-value pairs) to the specified Entity Resolution resource. Tags can help you
* organize and categorize your resources. You can also use them to scope user permissions by granting a user
* permission to access or change only resources with certain tag values. In Entity Resolution,
* SchemaMapping
and MatchingWorkflow
can be tagged. Tags don't have any semantic meaning
* to Amazon Web Services and are interpreted strictly as strings of characters. You can use the
* TagResource
action with a resource that already has tags. If you specify a new tag key, this tag is
* appended to the list of tags associated with the resource. If you specify a tag key that is already associated
* with the resource, the new tag value that you specify replaces the previous value for that tag.
*
*
* @param tagResourceRequest
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AWSEntityResolutionAsync.TagResource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest);
/**
*
* Assigns one or more tags (key-value pairs) to the specified Entity Resolution resource. Tags can help you
* organize and categorize your resources. You can also use them to scope user permissions by granting a user
* permission to access or change only resources with certain tag values. In Entity Resolution,
* SchemaMapping
and MatchingWorkflow
can be tagged. Tags don't have any semantic meaning
* to Amazon Web Services and are interpreted strictly as strings of characters. You can use the
* TagResource
action with a resource that already has tags. If you specify a new tag key, this tag is
* appended to the list of tags associated with the resource. If you specify a tag key that is already associated
* with the resource, the new tag value that you specify replaces the previous value for that tag.
*
*
* @param tagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AWSEntityResolutionAsyncHandler.TagResource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Removes one or more tags from the specified Entity Resolution resource. In Entity Resolution,
* SchemaMapping
, and MatchingWorkflow
can be tagged.
*
*
* @param untagResourceRequest
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AWSEntityResolutionAsync.UntagResource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest);
/**
*
* Removes one or more tags from the specified Entity Resolution resource. In Entity Resolution,
* SchemaMapping
, and MatchingWorkflow
can be tagged.
*
*
* @param untagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AWSEntityResolutionAsyncHandler.UntagResource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates an existing IdMappingWorkflow
. This method is identical to
* CreateIdMappingWorkflow
, except it uses an HTTP PUT
request instead of a
* POST
request, and the IdMappingWorkflow
must already exist for the method to succeed.
*
*
* @param updateIdMappingWorkflowRequest
* @return A Java Future containing the result of the UpdateIdMappingWorkflow operation returned by the service.
* @sample AWSEntityResolutionAsync.UpdateIdMappingWorkflow
* @see AWS API Documentation
*/
java.util.concurrent.Future updateIdMappingWorkflowAsync(UpdateIdMappingWorkflowRequest updateIdMappingWorkflowRequest);
/**
*
* Updates an existing IdMappingWorkflow
. This method is identical to
* CreateIdMappingWorkflow
, except it uses an HTTP PUT
request instead of a
* POST
request, and the IdMappingWorkflow
must already exist for the method to succeed.
*
*
* @param updateIdMappingWorkflowRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateIdMappingWorkflow operation returned by the service.
* @sample AWSEntityResolutionAsyncHandler.UpdateIdMappingWorkflow
* @see AWS API Documentation
*/
java.util.concurrent.Future updateIdMappingWorkflowAsync(UpdateIdMappingWorkflowRequest updateIdMappingWorkflowRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates an existing ID namespace.
*
*
* @param updateIdNamespaceRequest
* @return A Java Future containing the result of the UpdateIdNamespace operation returned by the service.
* @sample AWSEntityResolutionAsync.UpdateIdNamespace
* @see AWS API Documentation
*/
java.util.concurrent.Future updateIdNamespaceAsync(UpdateIdNamespaceRequest updateIdNamespaceRequest);
/**
*
* Updates an existing ID namespace.
*
*
* @param updateIdNamespaceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateIdNamespace operation returned by the service.
* @sample AWSEntityResolutionAsyncHandler.UpdateIdNamespace
* @see AWS API Documentation
*/
java.util.concurrent.Future updateIdNamespaceAsync(UpdateIdNamespaceRequest updateIdNamespaceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates an existing MatchingWorkflow
. This method is identical to
* CreateMatchingWorkflow
, except it uses an HTTP PUT
request instead of a
* POST
request, and the MatchingWorkflow
must already exist for the method to succeed.
*
*
* @param updateMatchingWorkflowRequest
* @return A Java Future containing the result of the UpdateMatchingWorkflow operation returned by the service.
* @sample AWSEntityResolutionAsync.UpdateMatchingWorkflow
* @see AWS API Documentation
*/
java.util.concurrent.Future updateMatchingWorkflowAsync(UpdateMatchingWorkflowRequest updateMatchingWorkflowRequest);
/**
*
* Updates an existing MatchingWorkflow
. This method is identical to
* CreateMatchingWorkflow
, except it uses an HTTP PUT
request instead of a
* POST
request, and the MatchingWorkflow
must already exist for the method to succeed.
*
*
* @param updateMatchingWorkflowRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateMatchingWorkflow operation returned by the service.
* @sample AWSEntityResolutionAsyncHandler.UpdateMatchingWorkflow
* @see AWS API Documentation
*/
java.util.concurrent.Future updateMatchingWorkflowAsync(UpdateMatchingWorkflowRequest updateMatchingWorkflowRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates a schema mapping.
*
*
*
* A schema is immutable if it is being used by a workflow. Therefore, you can't update a schema mapping if it's
* associated with a workflow.
*
*
*
* @param updateSchemaMappingRequest
* @return A Java Future containing the result of the UpdateSchemaMapping operation returned by the service.
* @sample AWSEntityResolutionAsync.UpdateSchemaMapping
* @see AWS API Documentation
*/
java.util.concurrent.Future updateSchemaMappingAsync(UpdateSchemaMappingRequest updateSchemaMappingRequest);
/**
*
* Updates a schema mapping.
*
*
*
* A schema is immutable if it is being used by a workflow. Therefore, you can't update a schema mapping if it's
* associated with a workflow.
*
*
*
* @param updateSchemaMappingRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateSchemaMapping operation returned by the service.
* @sample AWSEntityResolutionAsyncHandler.UpdateSchemaMapping
* @see AWS API Documentation
*/
java.util.concurrent.Future updateSchemaMappingAsync(UpdateSchemaMappingRequest updateSchemaMappingRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
}