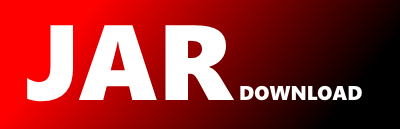
com.amazonaws.services.entityresolution.model.IdMappingJobMetrics Maven / Gradle / Ivy
Show all versions of aws-java-sdk-entityresolution Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.entityresolution.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* An object containing InputRecords
, RecordsNotProcessed
, TotalRecordsProcessed
,
* TotalMappedRecords
, TotalMappedSourceRecords
, and TotalMappedTargetRecords
.
*
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class IdMappingJobMetrics implements Serializable, Cloneable, StructuredPojo {
/**
*
* The total number of records that were input for processing.
*
*/
private Integer inputRecords;
/**
*
* The total number of records that did not get processed.
*
*/
private Integer recordsNotProcessed;
/**
*
* The total number of records that were mapped.
*
*/
private Integer totalMappedRecords;
/**
*
* The total number of mapped source records.
*
*/
private Integer totalMappedSourceRecords;
/**
*
* The total number of distinct mapped target records.
*
*/
private Integer totalMappedTargetRecords;
/**
*
* The total number of records that were processed.
*
*/
private Integer totalRecordsProcessed;
/**
*
* The total number of records that were input for processing.
*
*
* @param inputRecords
* The total number of records that were input for processing.
*/
public void setInputRecords(Integer inputRecords) {
this.inputRecords = inputRecords;
}
/**
*
* The total number of records that were input for processing.
*
*
* @return The total number of records that were input for processing.
*/
public Integer getInputRecords() {
return this.inputRecords;
}
/**
*
* The total number of records that were input for processing.
*
*
* @param inputRecords
* The total number of records that were input for processing.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public IdMappingJobMetrics withInputRecords(Integer inputRecords) {
setInputRecords(inputRecords);
return this;
}
/**
*
* The total number of records that did not get processed.
*
*
* @param recordsNotProcessed
* The total number of records that did not get processed.
*/
public void setRecordsNotProcessed(Integer recordsNotProcessed) {
this.recordsNotProcessed = recordsNotProcessed;
}
/**
*
* The total number of records that did not get processed.
*
*
* @return The total number of records that did not get processed.
*/
public Integer getRecordsNotProcessed() {
return this.recordsNotProcessed;
}
/**
*
* The total number of records that did not get processed.
*
*
* @param recordsNotProcessed
* The total number of records that did not get processed.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public IdMappingJobMetrics withRecordsNotProcessed(Integer recordsNotProcessed) {
setRecordsNotProcessed(recordsNotProcessed);
return this;
}
/**
*
* The total number of records that were mapped.
*
*
* @param totalMappedRecords
* The total number of records that were mapped.
*/
public void setTotalMappedRecords(Integer totalMappedRecords) {
this.totalMappedRecords = totalMappedRecords;
}
/**
*
* The total number of records that were mapped.
*
*
* @return The total number of records that were mapped.
*/
public Integer getTotalMappedRecords() {
return this.totalMappedRecords;
}
/**
*
* The total number of records that were mapped.
*
*
* @param totalMappedRecords
* The total number of records that were mapped.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public IdMappingJobMetrics withTotalMappedRecords(Integer totalMappedRecords) {
setTotalMappedRecords(totalMappedRecords);
return this;
}
/**
*
* The total number of mapped source records.
*
*
* @param totalMappedSourceRecords
* The total number of mapped source records.
*/
public void setTotalMappedSourceRecords(Integer totalMappedSourceRecords) {
this.totalMappedSourceRecords = totalMappedSourceRecords;
}
/**
*
* The total number of mapped source records.
*
*
* @return The total number of mapped source records.
*/
public Integer getTotalMappedSourceRecords() {
return this.totalMappedSourceRecords;
}
/**
*
* The total number of mapped source records.
*
*
* @param totalMappedSourceRecords
* The total number of mapped source records.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public IdMappingJobMetrics withTotalMappedSourceRecords(Integer totalMappedSourceRecords) {
setTotalMappedSourceRecords(totalMappedSourceRecords);
return this;
}
/**
*
* The total number of distinct mapped target records.
*
*
* @param totalMappedTargetRecords
* The total number of distinct mapped target records.
*/
public void setTotalMappedTargetRecords(Integer totalMappedTargetRecords) {
this.totalMappedTargetRecords = totalMappedTargetRecords;
}
/**
*
* The total number of distinct mapped target records.
*
*
* @return The total number of distinct mapped target records.
*/
public Integer getTotalMappedTargetRecords() {
return this.totalMappedTargetRecords;
}
/**
*
* The total number of distinct mapped target records.
*
*
* @param totalMappedTargetRecords
* The total number of distinct mapped target records.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public IdMappingJobMetrics withTotalMappedTargetRecords(Integer totalMappedTargetRecords) {
setTotalMappedTargetRecords(totalMappedTargetRecords);
return this;
}
/**
*
* The total number of records that were processed.
*
*
* @param totalRecordsProcessed
* The total number of records that were processed.
*/
public void setTotalRecordsProcessed(Integer totalRecordsProcessed) {
this.totalRecordsProcessed = totalRecordsProcessed;
}
/**
*
* The total number of records that were processed.
*
*
* @return The total number of records that were processed.
*/
public Integer getTotalRecordsProcessed() {
return this.totalRecordsProcessed;
}
/**
*
* The total number of records that were processed.
*
*
* @param totalRecordsProcessed
* The total number of records that were processed.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public IdMappingJobMetrics withTotalRecordsProcessed(Integer totalRecordsProcessed) {
setTotalRecordsProcessed(totalRecordsProcessed);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getInputRecords() != null)
sb.append("InputRecords: ").append(getInputRecords()).append(",");
if (getRecordsNotProcessed() != null)
sb.append("RecordsNotProcessed: ").append(getRecordsNotProcessed()).append(",");
if (getTotalMappedRecords() != null)
sb.append("TotalMappedRecords: ").append(getTotalMappedRecords()).append(",");
if (getTotalMappedSourceRecords() != null)
sb.append("TotalMappedSourceRecords: ").append(getTotalMappedSourceRecords()).append(",");
if (getTotalMappedTargetRecords() != null)
sb.append("TotalMappedTargetRecords: ").append(getTotalMappedTargetRecords()).append(",");
if (getTotalRecordsProcessed() != null)
sb.append("TotalRecordsProcessed: ").append(getTotalRecordsProcessed());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof IdMappingJobMetrics == false)
return false;
IdMappingJobMetrics other = (IdMappingJobMetrics) obj;
if (other.getInputRecords() == null ^ this.getInputRecords() == null)
return false;
if (other.getInputRecords() != null && other.getInputRecords().equals(this.getInputRecords()) == false)
return false;
if (other.getRecordsNotProcessed() == null ^ this.getRecordsNotProcessed() == null)
return false;
if (other.getRecordsNotProcessed() != null && other.getRecordsNotProcessed().equals(this.getRecordsNotProcessed()) == false)
return false;
if (other.getTotalMappedRecords() == null ^ this.getTotalMappedRecords() == null)
return false;
if (other.getTotalMappedRecords() != null && other.getTotalMappedRecords().equals(this.getTotalMappedRecords()) == false)
return false;
if (other.getTotalMappedSourceRecords() == null ^ this.getTotalMappedSourceRecords() == null)
return false;
if (other.getTotalMappedSourceRecords() != null && other.getTotalMappedSourceRecords().equals(this.getTotalMappedSourceRecords()) == false)
return false;
if (other.getTotalMappedTargetRecords() == null ^ this.getTotalMappedTargetRecords() == null)
return false;
if (other.getTotalMappedTargetRecords() != null && other.getTotalMappedTargetRecords().equals(this.getTotalMappedTargetRecords()) == false)
return false;
if (other.getTotalRecordsProcessed() == null ^ this.getTotalRecordsProcessed() == null)
return false;
if (other.getTotalRecordsProcessed() != null && other.getTotalRecordsProcessed().equals(this.getTotalRecordsProcessed()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getInputRecords() == null) ? 0 : getInputRecords().hashCode());
hashCode = prime * hashCode + ((getRecordsNotProcessed() == null) ? 0 : getRecordsNotProcessed().hashCode());
hashCode = prime * hashCode + ((getTotalMappedRecords() == null) ? 0 : getTotalMappedRecords().hashCode());
hashCode = prime * hashCode + ((getTotalMappedSourceRecords() == null) ? 0 : getTotalMappedSourceRecords().hashCode());
hashCode = prime * hashCode + ((getTotalMappedTargetRecords() == null) ? 0 : getTotalMappedTargetRecords().hashCode());
hashCode = prime * hashCode + ((getTotalRecordsProcessed() == null) ? 0 : getTotalRecordsProcessed().hashCode());
return hashCode;
}
@Override
public IdMappingJobMetrics clone() {
try {
return (IdMappingJobMetrics) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.entityresolution.model.transform.IdMappingJobMetricsMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}