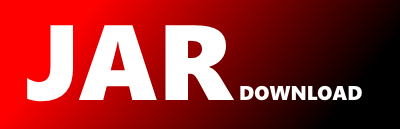
com.amazonaws.services.entityresolution.model.NamespaceRuleBasedProperties Maven / Gradle / Ivy
Show all versions of aws-java-sdk-entityresolution Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.entityresolution.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* The rule-based properties of an ID namespace. These properties define how the ID namespace can be used in an ID
* mapping workflow.
*
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class NamespaceRuleBasedProperties implements Serializable, Cloneable, StructuredPojo {
/**
*
* The comparison type. You can either choose ONE_TO_ONE
or MANY_TO_MANY
as the
* attributeMatchingModel
.
*
*
* If you choose MANY_TO_MANY
, the system can match attributes across the sub-types of an attribute
* type. For example, if the value of the Email
field of Profile A matches the value of
* BusinessEmail
field of Profile B, the two profiles are matched on the Email
attribute
* type.
*
*
* If you choose ONE_TO_ONE
, the system can only match attributes if the sub-types are an exact match.
* For example, for the Email
attribute type, the system will only consider it a match if the value of
* the Email
field of Profile A matches the value of the Email
field of Profile B.
*
*/
private String attributeMatchingModel;
/**
*
* The type of matching record that is allowed to be used in an ID mapping workflow.
*
*
* If the value is set to ONE_SOURCE_TO_ONE_TARGET
, only one record in the source is matched to one
* record in the target.
*
*
* If the value is set to MANY_SOURCE_TO_ONE_TARGET
, all matching records in the source are matched to
* one record in the target.
*
*/
private java.util.List recordMatchingModels;
/**
*
* The sets of rules you can use in an ID mapping workflow. The limitations specified for the source and target must
* be compatible.
*
*/
private java.util.List ruleDefinitionTypes;
/**
*
* The rules for the ID namespace.
*
*/
private java.util.List rules;
/**
*
* The comparison type. You can either choose ONE_TO_ONE
or MANY_TO_MANY
as the
* attributeMatchingModel
.
*
*
* If you choose MANY_TO_MANY
, the system can match attributes across the sub-types of an attribute
* type. For example, if the value of the Email
field of Profile A matches the value of
* BusinessEmail
field of Profile B, the two profiles are matched on the Email
attribute
* type.
*
*
* If you choose ONE_TO_ONE
, the system can only match attributes if the sub-types are an exact match.
* For example, for the Email
attribute type, the system will only consider it a match if the value of
* the Email
field of Profile A matches the value of the Email
field of Profile B.
*
*
* @param attributeMatchingModel
* The comparison type. You can either choose ONE_TO_ONE
or MANY_TO_MANY
as the
* attributeMatchingModel
.
*
* If you choose MANY_TO_MANY
, the system can match attributes across the sub-types of an
* attribute type. For example, if the value of the Email
field of Profile A matches the value
* of BusinessEmail
field of Profile B, the two profiles are matched on the Email
* attribute type.
*
*
* If you choose ONE_TO_ONE
, the system can only match attributes if the sub-types are an exact
* match. For example, for the Email
attribute type, the system will only consider it a match if
* the value of the Email
field of Profile A matches the value of the Email
field
* of Profile B.
* @see AttributeMatchingModel
*/
public void setAttributeMatchingModel(String attributeMatchingModel) {
this.attributeMatchingModel = attributeMatchingModel;
}
/**
*
* The comparison type. You can either choose ONE_TO_ONE
or MANY_TO_MANY
as the
* attributeMatchingModel
.
*
*
* If you choose MANY_TO_MANY
, the system can match attributes across the sub-types of an attribute
* type. For example, if the value of the Email
field of Profile A matches the value of
* BusinessEmail
field of Profile B, the two profiles are matched on the Email
attribute
* type.
*
*
* If you choose ONE_TO_ONE
, the system can only match attributes if the sub-types are an exact match.
* For example, for the Email
attribute type, the system will only consider it a match if the value of
* the Email
field of Profile A matches the value of the Email
field of Profile B.
*
*
* @return The comparison type. You can either choose ONE_TO_ONE
or MANY_TO_MANY
as the
* attributeMatchingModel
.
*
* If you choose MANY_TO_MANY
, the system can match attributes across the sub-types of an
* attribute type. For example, if the value of the Email
field of Profile A matches the value
* of BusinessEmail
field of Profile B, the two profiles are matched on the Email
* attribute type.
*
*
* If you choose ONE_TO_ONE
, the system can only match attributes if the sub-types are an exact
* match. For example, for the Email
attribute type, the system will only consider it a match
* if the value of the Email
field of Profile A matches the value of the Email
* field of Profile B.
* @see AttributeMatchingModel
*/
public String getAttributeMatchingModel() {
return this.attributeMatchingModel;
}
/**
*
* The comparison type. You can either choose ONE_TO_ONE
or MANY_TO_MANY
as the
* attributeMatchingModel
.
*
*
* If you choose MANY_TO_MANY
, the system can match attributes across the sub-types of an attribute
* type. For example, if the value of the Email
field of Profile A matches the value of
* BusinessEmail
field of Profile B, the two profiles are matched on the Email
attribute
* type.
*
*
* If you choose ONE_TO_ONE
, the system can only match attributes if the sub-types are an exact match.
* For example, for the Email
attribute type, the system will only consider it a match if the value of
* the Email
field of Profile A matches the value of the Email
field of Profile B.
*
*
* @param attributeMatchingModel
* The comparison type. You can either choose ONE_TO_ONE
or MANY_TO_MANY
as the
* attributeMatchingModel
.
*
* If you choose MANY_TO_MANY
, the system can match attributes across the sub-types of an
* attribute type. For example, if the value of the Email
field of Profile A matches the value
* of BusinessEmail
field of Profile B, the two profiles are matched on the Email
* attribute type.
*
*
* If you choose ONE_TO_ONE
, the system can only match attributes if the sub-types are an exact
* match. For example, for the Email
attribute type, the system will only consider it a match if
* the value of the Email
field of Profile A matches the value of the Email
field
* of Profile B.
* @return Returns a reference to this object so that method calls can be chained together.
* @see AttributeMatchingModel
*/
public NamespaceRuleBasedProperties withAttributeMatchingModel(String attributeMatchingModel) {
setAttributeMatchingModel(attributeMatchingModel);
return this;
}
/**
*
* The comparison type. You can either choose ONE_TO_ONE
or MANY_TO_MANY
as the
* attributeMatchingModel
.
*
*
* If you choose MANY_TO_MANY
, the system can match attributes across the sub-types of an attribute
* type. For example, if the value of the Email
field of Profile A matches the value of
* BusinessEmail
field of Profile B, the two profiles are matched on the Email
attribute
* type.
*
*
* If you choose ONE_TO_ONE
, the system can only match attributes if the sub-types are an exact match.
* For example, for the Email
attribute type, the system will only consider it a match if the value of
* the Email
field of Profile A matches the value of the Email
field of Profile B.
*
*
* @param attributeMatchingModel
* The comparison type. You can either choose ONE_TO_ONE
or MANY_TO_MANY
as the
* attributeMatchingModel
.
*
* If you choose MANY_TO_MANY
, the system can match attributes across the sub-types of an
* attribute type. For example, if the value of the Email
field of Profile A matches the value
* of BusinessEmail
field of Profile B, the two profiles are matched on the Email
* attribute type.
*
*
* If you choose ONE_TO_ONE
, the system can only match attributes if the sub-types are an exact
* match. For example, for the Email
attribute type, the system will only consider it a match if
* the value of the Email
field of Profile A matches the value of the Email
field
* of Profile B.
* @return Returns a reference to this object so that method calls can be chained together.
* @see AttributeMatchingModel
*/
public NamespaceRuleBasedProperties withAttributeMatchingModel(AttributeMatchingModel attributeMatchingModel) {
this.attributeMatchingModel = attributeMatchingModel.toString();
return this;
}
/**
*
* The type of matching record that is allowed to be used in an ID mapping workflow.
*
*
* If the value is set to ONE_SOURCE_TO_ONE_TARGET
, only one record in the source is matched to one
* record in the target.
*
*
* If the value is set to MANY_SOURCE_TO_ONE_TARGET
, all matching records in the source are matched to
* one record in the target.
*
*
* @return The type of matching record that is allowed to be used in an ID mapping workflow.
*
* If the value is set to ONE_SOURCE_TO_ONE_TARGET
, only one record in the source is matched to
* one record in the target.
*
*
* If the value is set to MANY_SOURCE_TO_ONE_TARGET
, all matching records in the source are
* matched to one record in the target.
* @see RecordMatchingModel
*/
public java.util.List getRecordMatchingModels() {
return recordMatchingModels;
}
/**
*
* The type of matching record that is allowed to be used in an ID mapping workflow.
*
*
* If the value is set to ONE_SOURCE_TO_ONE_TARGET
, only one record in the source is matched to one
* record in the target.
*
*
* If the value is set to MANY_SOURCE_TO_ONE_TARGET
, all matching records in the source are matched to
* one record in the target.
*
*
* @param recordMatchingModels
* The type of matching record that is allowed to be used in an ID mapping workflow.
*
* If the value is set to ONE_SOURCE_TO_ONE_TARGET
, only one record in the source is matched to
* one record in the target.
*
*
* If the value is set to MANY_SOURCE_TO_ONE_TARGET
, all matching records in the source are
* matched to one record in the target.
* @see RecordMatchingModel
*/
public void setRecordMatchingModels(java.util.Collection recordMatchingModels) {
if (recordMatchingModels == null) {
this.recordMatchingModels = null;
return;
}
this.recordMatchingModels = new java.util.ArrayList(recordMatchingModels);
}
/**
*
* The type of matching record that is allowed to be used in an ID mapping workflow.
*
*
* If the value is set to ONE_SOURCE_TO_ONE_TARGET
, only one record in the source is matched to one
* record in the target.
*
*
* If the value is set to MANY_SOURCE_TO_ONE_TARGET
, all matching records in the source are matched to
* one record in the target.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setRecordMatchingModels(java.util.Collection)} or {@link #withRecordMatchingModels(java.util.Collection)}
* if you want to override the existing values.
*
*
* @param recordMatchingModels
* The type of matching record that is allowed to be used in an ID mapping workflow.
*
* If the value is set to ONE_SOURCE_TO_ONE_TARGET
, only one record in the source is matched to
* one record in the target.
*
*
* If the value is set to MANY_SOURCE_TO_ONE_TARGET
, all matching records in the source are
* matched to one record in the target.
* @return Returns a reference to this object so that method calls can be chained together.
* @see RecordMatchingModel
*/
public NamespaceRuleBasedProperties withRecordMatchingModels(String... recordMatchingModels) {
if (this.recordMatchingModels == null) {
setRecordMatchingModels(new java.util.ArrayList(recordMatchingModels.length));
}
for (String ele : recordMatchingModels) {
this.recordMatchingModels.add(ele);
}
return this;
}
/**
*
* The type of matching record that is allowed to be used in an ID mapping workflow.
*
*
* If the value is set to ONE_SOURCE_TO_ONE_TARGET
, only one record in the source is matched to one
* record in the target.
*
*
* If the value is set to MANY_SOURCE_TO_ONE_TARGET
, all matching records in the source are matched to
* one record in the target.
*
*
* @param recordMatchingModels
* The type of matching record that is allowed to be used in an ID mapping workflow.
*
* If the value is set to ONE_SOURCE_TO_ONE_TARGET
, only one record in the source is matched to
* one record in the target.
*
*
* If the value is set to MANY_SOURCE_TO_ONE_TARGET
, all matching records in the source are
* matched to one record in the target.
* @return Returns a reference to this object so that method calls can be chained together.
* @see RecordMatchingModel
*/
public NamespaceRuleBasedProperties withRecordMatchingModels(java.util.Collection recordMatchingModels) {
setRecordMatchingModels(recordMatchingModels);
return this;
}
/**
*
* The type of matching record that is allowed to be used in an ID mapping workflow.
*
*
* If the value is set to ONE_SOURCE_TO_ONE_TARGET
, only one record in the source is matched to one
* record in the target.
*
*
* If the value is set to MANY_SOURCE_TO_ONE_TARGET
, all matching records in the source are matched to
* one record in the target.
*
*
* @param recordMatchingModels
* The type of matching record that is allowed to be used in an ID mapping workflow.
*
* If the value is set to ONE_SOURCE_TO_ONE_TARGET
, only one record in the source is matched to
* one record in the target.
*
*
* If the value is set to MANY_SOURCE_TO_ONE_TARGET
, all matching records in the source are
* matched to one record in the target.
* @return Returns a reference to this object so that method calls can be chained together.
* @see RecordMatchingModel
*/
public NamespaceRuleBasedProperties withRecordMatchingModels(RecordMatchingModel... recordMatchingModels) {
java.util.ArrayList recordMatchingModelsCopy = new java.util.ArrayList(recordMatchingModels.length);
for (RecordMatchingModel value : recordMatchingModels) {
recordMatchingModelsCopy.add(value.toString());
}
if (getRecordMatchingModels() == null) {
setRecordMatchingModels(recordMatchingModelsCopy);
} else {
getRecordMatchingModels().addAll(recordMatchingModelsCopy);
}
return this;
}
/**
*
* The sets of rules you can use in an ID mapping workflow. The limitations specified for the source and target must
* be compatible.
*
*
* @return The sets of rules you can use in an ID mapping workflow. The limitations specified for the source and
* target must be compatible.
* @see IdMappingWorkflowRuleDefinitionType
*/
public java.util.List getRuleDefinitionTypes() {
return ruleDefinitionTypes;
}
/**
*
* The sets of rules you can use in an ID mapping workflow. The limitations specified for the source and target must
* be compatible.
*
*
* @param ruleDefinitionTypes
* The sets of rules you can use in an ID mapping workflow. The limitations specified for the source and
* target must be compatible.
* @see IdMappingWorkflowRuleDefinitionType
*/
public void setRuleDefinitionTypes(java.util.Collection ruleDefinitionTypes) {
if (ruleDefinitionTypes == null) {
this.ruleDefinitionTypes = null;
return;
}
this.ruleDefinitionTypes = new java.util.ArrayList(ruleDefinitionTypes);
}
/**
*
* The sets of rules you can use in an ID mapping workflow. The limitations specified for the source and target must
* be compatible.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setRuleDefinitionTypes(java.util.Collection)} or {@link #withRuleDefinitionTypes(java.util.Collection)}
* if you want to override the existing values.
*
*
* @param ruleDefinitionTypes
* The sets of rules you can use in an ID mapping workflow. The limitations specified for the source and
* target must be compatible.
* @return Returns a reference to this object so that method calls can be chained together.
* @see IdMappingWorkflowRuleDefinitionType
*/
public NamespaceRuleBasedProperties withRuleDefinitionTypes(String... ruleDefinitionTypes) {
if (this.ruleDefinitionTypes == null) {
setRuleDefinitionTypes(new java.util.ArrayList(ruleDefinitionTypes.length));
}
for (String ele : ruleDefinitionTypes) {
this.ruleDefinitionTypes.add(ele);
}
return this;
}
/**
*
* The sets of rules you can use in an ID mapping workflow. The limitations specified for the source and target must
* be compatible.
*
*
* @param ruleDefinitionTypes
* The sets of rules you can use in an ID mapping workflow. The limitations specified for the source and
* target must be compatible.
* @return Returns a reference to this object so that method calls can be chained together.
* @see IdMappingWorkflowRuleDefinitionType
*/
public NamespaceRuleBasedProperties withRuleDefinitionTypes(java.util.Collection ruleDefinitionTypes) {
setRuleDefinitionTypes(ruleDefinitionTypes);
return this;
}
/**
*
* The sets of rules you can use in an ID mapping workflow. The limitations specified for the source and target must
* be compatible.
*
*
* @param ruleDefinitionTypes
* The sets of rules you can use in an ID mapping workflow. The limitations specified for the source and
* target must be compatible.
* @return Returns a reference to this object so that method calls can be chained together.
* @see IdMappingWorkflowRuleDefinitionType
*/
public NamespaceRuleBasedProperties withRuleDefinitionTypes(IdMappingWorkflowRuleDefinitionType... ruleDefinitionTypes) {
java.util.ArrayList ruleDefinitionTypesCopy = new java.util.ArrayList(ruleDefinitionTypes.length);
for (IdMappingWorkflowRuleDefinitionType value : ruleDefinitionTypes) {
ruleDefinitionTypesCopy.add(value.toString());
}
if (getRuleDefinitionTypes() == null) {
setRuleDefinitionTypes(ruleDefinitionTypesCopy);
} else {
getRuleDefinitionTypes().addAll(ruleDefinitionTypesCopy);
}
return this;
}
/**
*
* The rules for the ID namespace.
*
*
* @return The rules for the ID namespace.
*/
public java.util.List getRules() {
return rules;
}
/**
*
* The rules for the ID namespace.
*
*
* @param rules
* The rules for the ID namespace.
*/
public void setRules(java.util.Collection rules) {
if (rules == null) {
this.rules = null;
return;
}
this.rules = new java.util.ArrayList(rules);
}
/**
*
* The rules for the ID namespace.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setRules(java.util.Collection)} or {@link #withRules(java.util.Collection)} if you want to override the
* existing values.
*
*
* @param rules
* The rules for the ID namespace.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public NamespaceRuleBasedProperties withRules(Rule... rules) {
if (this.rules == null) {
setRules(new java.util.ArrayList(rules.length));
}
for (Rule ele : rules) {
this.rules.add(ele);
}
return this;
}
/**
*
* The rules for the ID namespace.
*
*
* @param rules
* The rules for the ID namespace.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public NamespaceRuleBasedProperties withRules(java.util.Collection rules) {
setRules(rules);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getAttributeMatchingModel() != null)
sb.append("AttributeMatchingModel: ").append(getAttributeMatchingModel()).append(",");
if (getRecordMatchingModels() != null)
sb.append("RecordMatchingModels: ").append(getRecordMatchingModels()).append(",");
if (getRuleDefinitionTypes() != null)
sb.append("RuleDefinitionTypes: ").append(getRuleDefinitionTypes()).append(",");
if (getRules() != null)
sb.append("Rules: ").append(getRules());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof NamespaceRuleBasedProperties == false)
return false;
NamespaceRuleBasedProperties other = (NamespaceRuleBasedProperties) obj;
if (other.getAttributeMatchingModel() == null ^ this.getAttributeMatchingModel() == null)
return false;
if (other.getAttributeMatchingModel() != null && other.getAttributeMatchingModel().equals(this.getAttributeMatchingModel()) == false)
return false;
if (other.getRecordMatchingModels() == null ^ this.getRecordMatchingModels() == null)
return false;
if (other.getRecordMatchingModels() != null && other.getRecordMatchingModels().equals(this.getRecordMatchingModels()) == false)
return false;
if (other.getRuleDefinitionTypes() == null ^ this.getRuleDefinitionTypes() == null)
return false;
if (other.getRuleDefinitionTypes() != null && other.getRuleDefinitionTypes().equals(this.getRuleDefinitionTypes()) == false)
return false;
if (other.getRules() == null ^ this.getRules() == null)
return false;
if (other.getRules() != null && other.getRules().equals(this.getRules()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getAttributeMatchingModel() == null) ? 0 : getAttributeMatchingModel().hashCode());
hashCode = prime * hashCode + ((getRecordMatchingModels() == null) ? 0 : getRecordMatchingModels().hashCode());
hashCode = prime * hashCode + ((getRuleDefinitionTypes() == null) ? 0 : getRuleDefinitionTypes().hashCode());
hashCode = prime * hashCode + ((getRules() == null) ? 0 : getRules().hashCode());
return hashCode;
}
@Override
public NamespaceRuleBasedProperties clone() {
try {
return (NamespaceRuleBasedProperties) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.entityresolution.model.transform.NamespaceRuleBasedPropertiesMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}