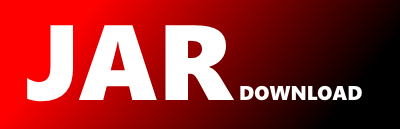
com.amazonaws.services.eventbridge.AmazonEventBridgeClient Maven / Gradle / Ivy
Show all versions of aws-java-sdk-eventbridge Show documentation
/*
* Copyright 2014-2019 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.eventbridge;
import org.w3c.dom.*;
import java.net.*;
import java.util.*;
import javax.annotation.Generated;
import org.apache.commons.logging.*;
import com.amazonaws.*;
import com.amazonaws.annotation.SdkInternalApi;
import com.amazonaws.auth.*;
import com.amazonaws.handlers.*;
import com.amazonaws.http.*;
import com.amazonaws.internal.*;
import com.amazonaws.internal.auth.*;
import com.amazonaws.metrics.*;
import com.amazonaws.regions.*;
import com.amazonaws.transform.*;
import com.amazonaws.util.*;
import com.amazonaws.protocol.json.*;
import com.amazonaws.util.AWSRequestMetrics.Field;
import com.amazonaws.annotation.ThreadSafe;
import com.amazonaws.client.AwsSyncClientParams;
import com.amazonaws.client.builder.AdvancedConfig;
import com.amazonaws.services.eventbridge.AmazonEventBridgeClientBuilder;
import com.amazonaws.AmazonServiceException;
import com.amazonaws.services.eventbridge.model.*;
import com.amazonaws.services.eventbridge.model.transform.*;
/**
* Client for accessing Amazon EventBridge. All service calls made using this client are blocking, and will not return
* until the service call completes.
*
*
* Amazon EventBridge helps you to respond to state changes in your AWS resources. When your resources change state,
* they automatically send events into an event stream. You can create rules that match selected events in the stream
* and route them to targets to take action. You can also use rules to take action on a predetermined schedule. For
* example, you can configure rules to:
*
*
* -
*
* Automatically invoke an AWS Lambda function to update DNS entries when an event notifies you that Amazon EC2 instance
* enters the running state
*
*
* -
*
* Direct specific API records from AWS CloudTrail to an Amazon Kinesis data stream for detailed analysis of potential
* security or availability risks
*
*
* -
*
* Periodically invoke a built-in target to create a snapshot of an Amazon EBS volume
*
*
*
*
* For more information about the features of Amazon EventBridge, see the Amazon EventBridge User Guide.
*
*/
@ThreadSafe
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class AmazonEventBridgeClient extends AmazonWebServiceClient implements AmazonEventBridge {
/** Provider for AWS credentials. */
private final AWSCredentialsProvider awsCredentialsProvider;
private static final Log log = LogFactory.getLog(AmazonEventBridge.class);
/** Default signing name for the service. */
private static final String DEFAULT_SIGNING_NAME = "events";
/** Client configuration factory providing ClientConfigurations tailored to this client */
protected static final ClientConfigurationFactory configFactory = new ClientConfigurationFactory();
private final AdvancedConfig advancedConfig;
private static final com.amazonaws.protocol.json.SdkJsonProtocolFactory protocolFactory = new com.amazonaws.protocol.json.SdkJsonProtocolFactory(
new JsonClientMetadata()
.withProtocolVersion("1.1")
.withSupportsCbor(false)
.withSupportsIon(false)
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ConcurrentModificationException").withExceptionUnmarshaller(
com.amazonaws.services.eventbridge.model.transform.ConcurrentModificationExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("PolicyLengthExceededException").withExceptionUnmarshaller(
com.amazonaws.services.eventbridge.model.transform.PolicyLengthExceededExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InvalidStateException").withExceptionUnmarshaller(
com.amazonaws.services.eventbridge.model.transform.InvalidStateExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InvalidEventPatternException").withExceptionUnmarshaller(
com.amazonaws.services.eventbridge.model.transform.InvalidEventPatternExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("LimitExceededException").withExceptionUnmarshaller(
com.amazonaws.services.eventbridge.model.transform.LimitExceededExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ResourceNotFoundException").withExceptionUnmarshaller(
com.amazonaws.services.eventbridge.model.transform.ResourceNotFoundExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ResourceAlreadyExistsException").withExceptionUnmarshaller(
com.amazonaws.services.eventbridge.model.transform.ResourceAlreadyExistsExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InternalException").withExceptionUnmarshaller(
com.amazonaws.services.eventbridge.model.transform.InternalExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ManagedRuleException").withExceptionUnmarshaller(
com.amazonaws.services.eventbridge.model.transform.ManagedRuleExceptionUnmarshaller.getInstance()))
.withBaseServiceExceptionClass(com.amazonaws.services.eventbridge.model.AmazonEventBridgeException.class));
public static AmazonEventBridgeClientBuilder builder() {
return AmazonEventBridgeClientBuilder.standard();
}
/**
* Constructs a new client to invoke service methods on Amazon EventBridge using the specified parameters.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param clientParams
* Object providing client parameters.
*/
AmazonEventBridgeClient(AwsSyncClientParams clientParams) {
this(clientParams, false);
}
/**
* Constructs a new client to invoke service methods on Amazon EventBridge using the specified parameters.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param clientParams
* Object providing client parameters.
*/
AmazonEventBridgeClient(AwsSyncClientParams clientParams, boolean endpointDiscoveryEnabled) {
super(clientParams);
this.awsCredentialsProvider = clientParams.getCredentialsProvider();
this.advancedConfig = clientParams.getAdvancedConfig();
init();
}
private void init() {
setServiceNameIntern(DEFAULT_SIGNING_NAME);
setEndpointPrefix(ENDPOINT_PREFIX);
// calling this.setEndPoint(...) will also modify the signer accordingly
setEndpoint("events.us-east-1.amazonaws.com");
HandlerChainFactory chainFactory = new HandlerChainFactory();
requestHandler2s.addAll(chainFactory.newRequestHandlerChain("/com/amazonaws/services/eventbridge/request.handlers"));
requestHandler2s.addAll(chainFactory.newRequestHandler2Chain("/com/amazonaws/services/eventbridge/request.handler2s"));
requestHandler2s.addAll(chainFactory.getGlobalHandlers());
}
/**
*
* Activates a partner event source that has been deactivated. Once activated, your matching event bus will start
* receiving events from the event source.
*
*
*
* This operation is performed by AWS customers, not by SaaS partners.
*
*
*
* @param activateEventSourceRequest
* @return Result of the ActivateEventSource operation returned by the service.
* @throws ResourceNotFoundException
* An entity that you specified doesn't exist.
* @throws InvalidStateException
* The specified state isn't a valid state for an event source.
* @throws InternalException
* This exception occurs due to unexpected causes.
* @sample AmazonEventBridge.ActivateEventSource
* @see AWS API Documentation
*/
@Override
public ActivateEventSourceResult activateEventSource(ActivateEventSourceRequest request) {
request = beforeClientExecution(request);
return executeActivateEventSource(request);
}
@SdkInternalApi
final ActivateEventSourceResult executeActivateEventSource(ActivateEventSourceRequest activateEventSourceRequest) {
ExecutionContext executionContext = createExecutionContext(activateEventSourceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ActivateEventSourceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(activateEventSourceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "EventBridge");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ActivateEventSource");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ActivateEventSourceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a new event bus within your account. This can be a custom event bus which you can use to receive events
* from your own custom applications and services, or it can be a partner event bus which can be matched to a
* partner event source.
*
*
*
* This operation is used by AWS customers, not by SaaS partners.
*
*
*
* @param createEventBusRequest
* @return Result of the CreateEventBus operation returned by the service.
* @throws ResourceAlreadyExistsException
* The resource that you're trying to create already exists.
* @throws ResourceNotFoundException
* An entity that you specified doesn't exist.
* @throws InvalidStateException
* The specified state isn't a valid state for an event source.
* @throws InternalException
* This exception occurs due to unexpected causes.
* @throws ConcurrentModificationException
* There is concurrent modification on a resource.
* @throws LimitExceededException
* You tried to create more resources than is allowed.
* @sample AmazonEventBridge.CreateEventBus
* @see AWS API
* Documentation
*/
@Override
public CreateEventBusResult createEventBus(CreateEventBusRequest request) {
request = beforeClientExecution(request);
return executeCreateEventBus(request);
}
@SdkInternalApi
final CreateEventBusResult executeCreateEventBus(CreateEventBusRequest createEventBusRequest) {
ExecutionContext executionContext = createExecutionContext(createEventBusRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateEventBusRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createEventBusRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "EventBridge");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateEventBus");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateEventBusResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Called by an SaaS partner to create a partner event source.
*
*
*
* This operation is not used by AWS customers.
*
*
*
* Each partner event source can be used by one AWS account to create a matching partner event bus in that AWS
* account. A SaaS partner must create one partner event source for each AWS account that wants to receive those
* event types.
*
*
* A partner event source creates events based on resources in the SaaS partner's service or application.
*
*
* An AWS account that creates a partner event bus that matches the partner event source can use that event bus to
* receive events from the partner, and then process them using AWS Events rules and targets.
*
*
* Partner event source names follow this format:
*
*
* aws.partner/partner_name/event_namespace/event_name
*
*
* -
*
* partner_name is determined during partner registration and identifies the partner to AWS customers.
*
*
* -
*
* For event_namespace, we recommend that partners use a string that identifies the AWS customer within the
* partner's system. This should not be the customer's AWS account ID.
*
*
* -
*
* event_name is determined by the partner, and should uniquely identify an event-generating resource within
* the partner system. This should help AWS customers decide whether to create an event bus to receive these events.
*
*
*
*
* @param createPartnerEventSourceRequest
* @return Result of the CreatePartnerEventSource operation returned by the service.
* @throws ResourceAlreadyExistsException
* The resource that you're trying to create already exists.
* @throws InternalException
* This exception occurs due to unexpected causes.
* @throws ConcurrentModificationException
* There is concurrent modification on a resource.
* @throws LimitExceededException
* You tried to create more resources than is allowed.
* @sample AmazonEventBridge.CreatePartnerEventSource
* @see AWS API Documentation
*/
@Override
public CreatePartnerEventSourceResult createPartnerEventSource(CreatePartnerEventSourceRequest request) {
request = beforeClientExecution(request);
return executeCreatePartnerEventSource(request);
}
@SdkInternalApi
final CreatePartnerEventSourceResult executeCreatePartnerEventSource(CreatePartnerEventSourceRequest createPartnerEventSourceRequest) {
ExecutionContext executionContext = createExecutionContext(createPartnerEventSourceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreatePartnerEventSourceRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(createPartnerEventSourceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "EventBridge");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreatePartnerEventSource");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new CreatePartnerEventSourceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* An AWS customer uses this operation to temporarily stop receiving events from the specified partner event source.
* The matching event bus isn't deleted.
*
*
* When you deactivate a partner event source, the source goes into PENDING
state. If it remains in
* PENDING
state for more than two weeks, it's deleted.
*
*
* To activate a deactivated partner event source, use ActivateEventSource.
*
*
* @param deactivateEventSourceRequest
* @return Result of the DeactivateEventSource operation returned by the service.
* @throws ResourceNotFoundException
* An entity that you specified doesn't exist.
* @throws InvalidStateException
* The specified state isn't a valid state for an event source.
* @throws InternalException
* This exception occurs due to unexpected causes.
* @sample AmazonEventBridge.DeactivateEventSource
* @see AWS API Documentation
*/
@Override
public DeactivateEventSourceResult deactivateEventSource(DeactivateEventSourceRequest request) {
request = beforeClientExecution(request);
return executeDeactivateEventSource(request);
}
@SdkInternalApi
final DeactivateEventSourceResult executeDeactivateEventSource(DeactivateEventSourceRequest deactivateEventSourceRequest) {
ExecutionContext executionContext = createExecutionContext(deactivateEventSourceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeactivateEventSourceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deactivateEventSourceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "EventBridge");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeactivateEventSource");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DeactivateEventSourceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the specified custom event bus or partner event bus. All rules associated with this event bus are also
* deleted. You can't delete your account's default event bus.
*
*
*
* This operation is performed by AWS customers, not by SaaS partners.
*
*
*
* @param deleteEventBusRequest
* @return Result of the DeleteEventBus operation returned by the service.
* @throws InternalException
* This exception occurs due to unexpected causes.
* @sample AmazonEventBridge.DeleteEventBus
* @see AWS API
* Documentation
*/
@Override
public DeleteEventBusResult deleteEventBus(DeleteEventBusRequest request) {
request = beforeClientExecution(request);
return executeDeleteEventBus(request);
}
@SdkInternalApi
final DeleteEventBusResult executeDeleteEventBus(DeleteEventBusRequest deleteEventBusRequest) {
ExecutionContext executionContext = createExecutionContext(deleteEventBusRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteEventBusRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteEventBusRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "EventBridge");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteEventBus");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteEventBusResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* This operation is used by SaaS partners to delete a partner event source. AWS customers don't use this operation.
*
*
* When you delete an event source, the status of the corresponding partner event bus in the AWS customer account
* becomes DELETED
.
*
*
* @param deletePartnerEventSourceRequest
* @return Result of the DeletePartnerEventSource operation returned by the service.
* @throws InternalException
* This exception occurs due to unexpected causes.
* @sample AmazonEventBridge.DeletePartnerEventSource
* @see AWS API Documentation
*/
@Override
public DeletePartnerEventSourceResult deletePartnerEventSource(DeletePartnerEventSourceRequest request) {
request = beforeClientExecution(request);
return executeDeletePartnerEventSource(request);
}
@SdkInternalApi
final DeletePartnerEventSourceResult executeDeletePartnerEventSource(DeletePartnerEventSourceRequest deletePartnerEventSourceRequest) {
ExecutionContext executionContext = createExecutionContext(deletePartnerEventSourceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeletePartnerEventSourceRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(deletePartnerEventSourceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "EventBridge");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeletePartnerEventSource");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DeletePartnerEventSourceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the specified rule.
*
*
* Before you can delete the rule, you must remove all targets, using RemoveTargets.
*
*
* When you delete a rule, incoming events might continue to match to the deleted rule. Allow a short period of time
* for changes to take effect.
*
*
* Managed rules are rules created and managed by another AWS service on your behalf. These rules are created by
* those other AWS services to support functionality in those services. You can delete these rules using the
* Force
option, but you should do so only if you're sure that the other service isn't still using that
* rule.
*
*
* @param deleteRuleRequest
* @return Result of the DeleteRule operation returned by the service.
* @throws ConcurrentModificationException
* There is concurrent modification on a resource.
* @throws ManagedRuleException
* An AWS service created this rule on behalf of your account. That service manages it. If you see this
* error in response to DeleteRule
or RemoveTargets
, you can use the
* Force
parameter in those calls to delete the rule or remove targets from the rule. You can't
* modify these managed rules by using DisableRule
, EnableRule
,
* PutTargets
, PutRule
, TagResource
, or UntagResource
.
* @throws InternalException
* This exception occurs due to unexpected causes.
* @throws ResourceNotFoundException
* An entity that you specified doesn't exist.
* @sample AmazonEventBridge.DeleteRule
* @see AWS API
* Documentation
*/
@Override
public DeleteRuleResult deleteRule(DeleteRuleRequest request) {
request = beforeClientExecution(request);
return executeDeleteRule(request);
}
@SdkInternalApi
final DeleteRuleResult executeDeleteRule(DeleteRuleRequest deleteRuleRequest) {
ExecutionContext executionContext = createExecutionContext(deleteRuleRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteRuleRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteRuleRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "EventBridge");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteRule");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteRuleResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Displays details about an event bus in your account. This can include the external AWS accounts that are
* permitted to write events to your default event bus, and the associated policy. For custom event buses and
* partner event buses, it displays the name, ARN, policy, state, and creation time.
*
*
* To enable your account to receive events from other accounts on its default event bus, use PutPermission.
*
*
* For more information about partner event buses, see CreateEventBus.
*
*
* @param describeEventBusRequest
* @return Result of the DescribeEventBus operation returned by the service.
* @throws ResourceNotFoundException
* An entity that you specified doesn't exist.
* @throws InternalException
* This exception occurs due to unexpected causes.
* @sample AmazonEventBridge.DescribeEventBus
* @see AWS
* API Documentation
*/
@Override
public DescribeEventBusResult describeEventBus(DescribeEventBusRequest request) {
request = beforeClientExecution(request);
return executeDescribeEventBus(request);
}
@SdkInternalApi
final DescribeEventBusResult executeDescribeEventBus(DescribeEventBusRequest describeEventBusRequest) {
ExecutionContext executionContext = createExecutionContext(describeEventBusRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeEventBusRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(describeEventBusRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "EventBridge");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeEventBus");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DescribeEventBusResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* This operation lists details about a partner event source that is shared with your account.
*
*
*
* This operation is run by AWS customers, not by SaaS partners.
*
*
*
* @param describeEventSourceRequest
* @return Result of the DescribeEventSource operation returned by the service.
* @throws ResourceNotFoundException
* An entity that you specified doesn't exist.
* @throws InternalException
* This exception occurs due to unexpected causes.
* @sample AmazonEventBridge.DescribeEventSource
* @see AWS API Documentation
*/
@Override
public DescribeEventSourceResult describeEventSource(DescribeEventSourceRequest request) {
request = beforeClientExecution(request);
return executeDescribeEventSource(request);
}
@SdkInternalApi
final DescribeEventSourceResult executeDescribeEventSource(DescribeEventSourceRequest describeEventSourceRequest) {
ExecutionContext executionContext = createExecutionContext(describeEventSourceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeEventSourceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(describeEventSourceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "EventBridge");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeEventSource");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DescribeEventSourceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* An SaaS partner can use this operation to list details about a partner event source that they have created.
*
*
*
* AWS customers do not use this operation. Instead, AWS customers can use DescribeEventSource to see details
* about a partner event source that is shared with them.
*
*
*
* @param describePartnerEventSourceRequest
* @return Result of the DescribePartnerEventSource operation returned by the service.
* @throws ResourceNotFoundException
* An entity that you specified doesn't exist.
* @throws InternalException
* This exception occurs due to unexpected causes.
* @sample AmazonEventBridge.DescribePartnerEventSource
* @see AWS API Documentation
*/
@Override
public DescribePartnerEventSourceResult describePartnerEventSource(DescribePartnerEventSourceRequest request) {
request = beforeClientExecution(request);
return executeDescribePartnerEventSource(request);
}
@SdkInternalApi
final DescribePartnerEventSourceResult executeDescribePartnerEventSource(DescribePartnerEventSourceRequest describePartnerEventSourceRequest) {
ExecutionContext executionContext = createExecutionContext(describePartnerEventSourceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribePartnerEventSourceRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(describePartnerEventSourceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "EventBridge");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribePartnerEventSource");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DescribePartnerEventSourceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Describes the specified rule.
*
*
* DescribeRule
doesn't list the targets of a rule. To see the targets associated with a rule, use
* ListTargetsByRule.
*
*
* @param describeRuleRequest
* @return Result of the DescribeRule operation returned by the service.
* @throws ResourceNotFoundException
* An entity that you specified doesn't exist.
* @throws InternalException
* This exception occurs due to unexpected causes.
* @sample AmazonEventBridge.DescribeRule
* @see AWS API
* Documentation
*/
@Override
public DescribeRuleResult describeRule(DescribeRuleRequest request) {
request = beforeClientExecution(request);
return executeDescribeRule(request);
}
@SdkInternalApi
final DescribeRuleResult executeDescribeRule(DescribeRuleRequest describeRuleRequest) {
ExecutionContext executionContext = createExecutionContext(describeRuleRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeRuleRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(describeRuleRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "EventBridge");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeRule");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DescribeRuleResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Disables the specified rule. A disabled rule won't match any events and won't self-trigger if it has a schedule
* expression.
*
*
* When you disable a rule, incoming events might continue to match to the disabled rule. Allow a short period of
* time for changes to take effect.
*
*
* @param disableRuleRequest
* @return Result of the DisableRule operation returned by the service.
* @throws ResourceNotFoundException
* An entity that you specified doesn't exist.
* @throws ConcurrentModificationException
* There is concurrent modification on a resource.
* @throws ManagedRuleException
* An AWS service created this rule on behalf of your account. That service manages it. If you see this
* error in response to DeleteRule
or RemoveTargets
, you can use the
* Force
parameter in those calls to delete the rule or remove targets from the rule. You can't
* modify these managed rules by using DisableRule
, EnableRule
,
* PutTargets
, PutRule
, TagResource
, or UntagResource
.
* @throws InternalException
* This exception occurs due to unexpected causes.
* @sample AmazonEventBridge.DisableRule
* @see AWS API
* Documentation
*/
@Override
public DisableRuleResult disableRule(DisableRuleRequest request) {
request = beforeClientExecution(request);
return executeDisableRule(request);
}
@SdkInternalApi
final DisableRuleResult executeDisableRule(DisableRuleRequest disableRuleRequest) {
ExecutionContext executionContext = createExecutionContext(disableRuleRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DisableRuleRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(disableRuleRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "EventBridge");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DisableRule");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DisableRuleResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Enables the specified rule. If the rule doesn't exist, the operation fails.
*
*
* When you enable a rule, incoming events might not immediately start matching to a newly enabled rule. Allow a
* short period of time for changes to take effect.
*
*
* @param enableRuleRequest
* @return Result of the EnableRule operation returned by the service.
* @throws ResourceNotFoundException
* An entity that you specified doesn't exist.
* @throws ConcurrentModificationException
* There is concurrent modification on a resource.
* @throws ManagedRuleException
* An AWS service created this rule on behalf of your account. That service manages it. If you see this
* error in response to DeleteRule
or RemoveTargets
, you can use the
* Force
parameter in those calls to delete the rule or remove targets from the rule. You can't
* modify these managed rules by using DisableRule
, EnableRule
,
* PutTargets
, PutRule
, TagResource
, or UntagResource
.
* @throws InternalException
* This exception occurs due to unexpected causes.
* @sample AmazonEventBridge.EnableRule
* @see AWS API
* Documentation
*/
@Override
public EnableRuleResult enableRule(EnableRuleRequest request) {
request = beforeClientExecution(request);
return executeEnableRule(request);
}
@SdkInternalApi
final EnableRuleResult executeEnableRule(EnableRuleRequest enableRuleRequest) {
ExecutionContext executionContext = createExecutionContext(enableRuleRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new EnableRuleRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(enableRuleRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "EventBridge");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "EnableRule");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true).withHasStreamingSuccessResponse(false), new EnableRuleResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists all the event buses in your account, including the default event bus, custom event buses, and partner event
* buses.
*
*
*
* This operation is run by AWS customers, not by SaaS partners.
*
*
*
* @param listEventBusesRequest
* @return Result of the ListEventBuses operation returned by the service.
* @throws InternalException
* This exception occurs due to unexpected causes.
* @sample AmazonEventBridge.ListEventBuses
* @see AWS API
* Documentation
*/
@Override
public ListEventBusesResult listEventBuses(ListEventBusesRequest request) {
request = beforeClientExecution(request);
return executeListEventBuses(request);
}
@SdkInternalApi
final ListEventBusesResult executeListEventBuses(ListEventBusesRequest listEventBusesRequest) {
ExecutionContext executionContext = createExecutionContext(listEventBusesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListEventBusesRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listEventBusesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "EventBridge");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListEventBuses");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListEventBusesResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* You can use this to see all the partner event sources that have been shared with your AWS account. For more
* information about partner event sources, see CreateEventBus.
*
*
*
* This operation is run by AWS customers, not by SaaS partners.
*
*
*
* @param listEventSourcesRequest
* @return Result of the ListEventSources operation returned by the service.
* @throws InternalException
* This exception occurs due to unexpected causes.
* @sample AmazonEventBridge.ListEventSources
* @see AWS
* API Documentation
*/
@Override
public ListEventSourcesResult listEventSources(ListEventSourcesRequest request) {
request = beforeClientExecution(request);
return executeListEventSources(request);
}
@SdkInternalApi
final ListEventSourcesResult executeListEventSources(ListEventSourcesRequest listEventSourcesRequest) {
ExecutionContext executionContext = createExecutionContext(listEventSourcesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListEventSourcesRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listEventSourcesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "EventBridge");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListEventSources");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListEventSourcesResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* An SaaS partner can use this operation to display the AWS account ID that a particular partner event source name
* is associated with.
*
*
*
* This operation is used by SaaS partners, not by AWS customers.
*
*
*
* @param listPartnerEventSourceAccountsRequest
* @return Result of the ListPartnerEventSourceAccounts operation returned by the service.
* @throws ResourceNotFoundException
* An entity that you specified doesn't exist.
* @throws InternalException
* This exception occurs due to unexpected causes.
* @sample AmazonEventBridge.ListPartnerEventSourceAccounts
* @see AWS API Documentation
*/
@Override
public ListPartnerEventSourceAccountsResult listPartnerEventSourceAccounts(ListPartnerEventSourceAccountsRequest request) {
request = beforeClientExecution(request);
return executeListPartnerEventSourceAccounts(request);
}
@SdkInternalApi
final ListPartnerEventSourceAccountsResult executeListPartnerEventSourceAccounts(ListPartnerEventSourceAccountsRequest listPartnerEventSourceAccountsRequest) {
ExecutionContext executionContext = createExecutionContext(listPartnerEventSourceAccountsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListPartnerEventSourceAccountsRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(listPartnerEventSourceAccountsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "EventBridge");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListPartnerEventSourceAccounts");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ListPartnerEventSourceAccountsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* An SaaS partner can use this operation to list all the partner event source names that they have created.
*
*
*
* This operation is not used by AWS customers.
*
*
*
* @param listPartnerEventSourcesRequest
* @return Result of the ListPartnerEventSources operation returned by the service.
* @throws InternalException
* This exception occurs due to unexpected causes.
* @sample AmazonEventBridge.ListPartnerEventSources
* @see AWS API Documentation
*/
@Override
public ListPartnerEventSourcesResult listPartnerEventSources(ListPartnerEventSourcesRequest request) {
request = beforeClientExecution(request);
return executeListPartnerEventSources(request);
}
@SdkInternalApi
final ListPartnerEventSourcesResult executeListPartnerEventSources(ListPartnerEventSourcesRequest listPartnerEventSourcesRequest) {
ExecutionContext executionContext = createExecutionContext(listPartnerEventSourcesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListPartnerEventSourcesRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(listPartnerEventSourcesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "EventBridge");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListPartnerEventSources");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ListPartnerEventSourcesResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists the rules for the specified target. You can see which rules can invoke a specific target in your account.
*
*
* @param listRuleNamesByTargetRequest
* @return Result of the ListRuleNamesByTarget operation returned by the service.
* @throws InternalException
* This exception occurs due to unexpected causes.
* @throws ResourceNotFoundException
* An entity that you specified doesn't exist.
* @sample AmazonEventBridge.ListRuleNamesByTarget
* @see AWS API Documentation
*/
@Override
public ListRuleNamesByTargetResult listRuleNamesByTarget(ListRuleNamesByTargetRequest request) {
request = beforeClientExecution(request);
return executeListRuleNamesByTarget(request);
}
@SdkInternalApi
final ListRuleNamesByTargetResult executeListRuleNamesByTarget(ListRuleNamesByTargetRequest listRuleNamesByTargetRequest) {
ExecutionContext executionContext = createExecutionContext(listRuleNamesByTargetRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListRuleNamesByTargetRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listRuleNamesByTargetRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "EventBridge");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListRuleNamesByTarget");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ListRuleNamesByTargetResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists your EventBridge rules. You can either list all the rules or provide a prefix to match to the rule names.
*
*
* ListRules
doesn't list the targets of a rule. To see the targets associated with a rule, use
* ListTargetsByRule.
*
*
* @param listRulesRequest
* @return Result of the ListRules operation returned by the service.
* @throws InternalException
* This exception occurs due to unexpected causes.
* @throws ResourceNotFoundException
* An entity that you specified doesn't exist.
* @sample AmazonEventBridge.ListRules
* @see AWS API
* Documentation
*/
@Override
public ListRulesResult listRules(ListRulesRequest request) {
request = beforeClientExecution(request);
return executeListRules(request);
}
@SdkInternalApi
final ListRulesResult executeListRules(ListRulesRequest listRulesRequest) {
ExecutionContext executionContext = createExecutionContext(listRulesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListRulesRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listRulesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "EventBridge");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListRules");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListRulesResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Displays the tags associated with an EventBridge resource. In EventBridge, rules can be tagged.
*
*
* @param listTagsForResourceRequest
* @return Result of the ListTagsForResource operation returned by the service.
* @throws ResourceNotFoundException
* An entity that you specified doesn't exist.
* @throws InternalException
* This exception occurs due to unexpected causes.
* @sample AmazonEventBridge.ListTagsForResource
* @see AWS API Documentation
*/
@Override
public ListTagsForResourceResult listTagsForResource(ListTagsForResourceRequest request) {
request = beforeClientExecution(request);
return executeListTagsForResource(request);
}
@SdkInternalApi
final ListTagsForResourceResult executeListTagsForResource(ListTagsForResourceRequest listTagsForResourceRequest) {
ExecutionContext executionContext = createExecutionContext(listTagsForResourceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListTagsForResourceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listTagsForResourceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "EventBridge");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListTagsForResource");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListTagsForResourceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists the targets assigned to the specified rule.
*
*
* @param listTargetsByRuleRequest
* @return Result of the ListTargetsByRule operation returned by the service.
* @throws ResourceNotFoundException
* An entity that you specified doesn't exist.
* @throws InternalException
* This exception occurs due to unexpected causes.
* @sample AmazonEventBridge.ListTargetsByRule
* @see AWS
* API Documentation
*/
@Override
public ListTargetsByRuleResult listTargetsByRule(ListTargetsByRuleRequest request) {
request = beforeClientExecution(request);
return executeListTargetsByRule(request);
}
@SdkInternalApi
final ListTargetsByRuleResult executeListTargetsByRule(ListTargetsByRuleRequest listTargetsByRuleRequest) {
ExecutionContext executionContext = createExecutionContext(listTargetsByRuleRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListTargetsByRuleRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listTargetsByRuleRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "EventBridge");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListTargetsByRule");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListTargetsByRuleResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Sends custom events to EventBridge so that they can be matched to rules. These events can be from your custom
* applications and services.
*
*
* @param putEventsRequest
* @return Result of the PutEvents operation returned by the service.
* @throws InternalException
* This exception occurs due to unexpected causes.
* @sample AmazonEventBridge.PutEvents
* @see AWS API
* Documentation
*/
@Override
public PutEventsResult putEvents(PutEventsRequest request) {
request = beforeClientExecution(request);
return executePutEvents(request);
}
@SdkInternalApi
final PutEventsResult executePutEvents(PutEventsRequest putEventsRequest) {
ExecutionContext executionContext = createExecutionContext(putEventsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new PutEventsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(putEventsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "EventBridge");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "PutEvents");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true).withHasStreamingSuccessResponse(false), new PutEventsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* This is used by SaaS partners to write events to a customer's partner event bus.
*
*
*
* AWS customers do not use this operation. Instead, AWS customers can use PutEvents to write custom events
* from their own applications to an event bus.
*
*
*
* @param putPartnerEventsRequest
* @return Result of the PutPartnerEvents operation returned by the service.
* @throws InternalException
* This exception occurs due to unexpected causes.
* @sample AmazonEventBridge.PutPartnerEvents
* @see AWS
* API Documentation
*/
@Override
public PutPartnerEventsResult putPartnerEvents(PutPartnerEventsRequest request) {
request = beforeClientExecution(request);
return executePutPartnerEvents(request);
}
@SdkInternalApi
final PutPartnerEventsResult executePutPartnerEvents(PutPartnerEventsRequest putPartnerEventsRequest) {
ExecutionContext executionContext = createExecutionContext(putPartnerEventsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new PutPartnerEventsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(putPartnerEventsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "EventBridge");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "PutPartnerEvents");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new PutPartnerEventsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Running PutPermission
permits the specified AWS account or AWS organization to put events to the
* specified event bus. Rules in your account are triggered by these events arriving to an event bus in your
* account.
*
*
* For another account to send events to your account, that external account must have a rule with your account's
* event bus as a target.
*
*
* To enable multiple AWS accounts to put events to an event bus, run PutPermission
once for each of
* these accounts. Or, if all the accounts are members of the same AWS organization, you can run
* PutPermission
once specifying Principal
as "*" and specifying the AWS organization ID
* in Condition
, to grant permissions to all accounts in that organization.
*
*
* If you grant permissions using an organization, then accounts in that organization must specify a
* RoleArn
with proper permissions when they use PutTarget
to add your account's event bus
* as a target. For more information, see Sending and Receiving Events Between AWS Accounts in the Amazon EventBridge User Guide.
*
*
* The permission policy on an event bus can't exceed 10 KB in size.
*
*
* @param putPermissionRequest
* @return Result of the PutPermission operation returned by the service.
* @throws ResourceNotFoundException
* An entity that you specified doesn't exist.
* @throws PolicyLengthExceededException
* The event bus policy is too long. For more information, see the limits.
* @throws InternalException
* This exception occurs due to unexpected causes.
* @throws ConcurrentModificationException
* There is concurrent modification on a resource.
* @sample AmazonEventBridge.PutPermission
* @see AWS API
* Documentation
*/
@Override
public PutPermissionResult putPermission(PutPermissionRequest request) {
request = beforeClientExecution(request);
return executePutPermission(request);
}
@SdkInternalApi
final PutPermissionResult executePutPermission(PutPermissionRequest putPermissionRequest) {
ExecutionContext executionContext = createExecutionContext(putPermissionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new PutPermissionRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(putPermissionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "EventBridge");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "PutPermission");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new PutPermissionResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates or updates the specified rule. Rules are enabled by default or based on value of the state. You can
* disable a rule using DisableRule.
*
*
* A single rule watches for events from a single event bus. Events generated by AWS services go to your account's
* default event bus. Events generated by SaaS partner services or applications go to the matching partner event
* bus. If you have custom applications or services, you can specify whether their events go to your default event
* bus or a custom event bus that you have created. For more information, see CreateEventBus.
*
*
* If you're updating an existing rule, the rule is replaced with what you specify in this PutRule
* command. If you omit arguments in PutRule
, the old values for those arguments aren't kept. Instead,
* they're replaced with null values.
*
*
* When you create or update a rule, incoming events might not immediately start matching to new or updated rules.
* Allow a short period of time for changes to take effect.
*
*
* A rule must contain at least an EventPattern
or ScheduleExpression
. Rules with
* EventPatterns
are triggered when a matching event is observed. Rules with
* ScheduleExpressions
self-trigger based on the given schedule. A rule can have both an
* EventPattern
and a ScheduleExpression
, in which case the rule triggers on matching
* events as well as on a schedule.
*
*
* When you initially create a rule, you can optionally assign one or more tags to the rule. Tags can help you
* organize and categorize your resources. You can also use them to scope user permissions, by granting a user
* permission to access or change only rules with certain tag values. To use the PutRule
operation and
* assign tags, you must have both the events:PutRule
and events:TagResource
permissions.
*
*
* If you are updating an existing rule, any tags you specify in the PutRule
operation are ignored. To
* update the tags of an existing rule, use TagResource and UntagResource.
*
*
* Most services in AWS treat :
or /
as the same character in Amazon Resource Names
* (ARNs). However, EventBridge uses an exact match in event patterns and rules. Be sure to use the correct ARN
* characters when creating event patterns so that they match the ARN syntax in the event that you want to match.
*
*
* In EventBridge, you could create rules that lead to infinite loops, where a rule is fired repeatedly. For
* example, a rule might detect that ACLs have changed on an S3 bucket, and trigger software to change them to the
* desired state. If you don't write the rule carefully, the subsequent change to the ACLs fires the rule again,
* creating an infinite loop.
*
*
* To prevent this, write the rules so that the triggered actions don't refire the same rule. For example, your rule
* could fire only if ACLs are found to be in a bad state, instead of after any change.
*
*
* An infinite loop can quickly cause higher than expected charges. We recommend that you use budgeting, which
* alerts you when charges exceed your specified limit. For more information, see Managing Your
* Costs with Budgets.
*
*
* @param putRuleRequest
* @return Result of the PutRule operation returned by the service.
* @throws InvalidEventPatternException
* The event pattern isn't valid.
* @throws LimitExceededException
* You tried to create more resources than is allowed.
* @throws ConcurrentModificationException
* There is concurrent modification on a resource.
* @throws ManagedRuleException
* An AWS service created this rule on behalf of your account. That service manages it. If you see this
* error in response to DeleteRule
or RemoveTargets
, you can use the
* Force
parameter in those calls to delete the rule or remove targets from the rule. You can't
* modify these managed rules by using DisableRule
, EnableRule
,
* PutTargets
, PutRule
, TagResource
, or UntagResource
.
* @throws InternalException
* This exception occurs due to unexpected causes.
* @throws ResourceNotFoundException
* An entity that you specified doesn't exist.
* @sample AmazonEventBridge.PutRule
* @see AWS API
* Documentation
*/
@Override
public PutRuleResult putRule(PutRuleRequest request) {
request = beforeClientExecution(request);
return executePutRule(request);
}
@SdkInternalApi
final PutRuleResult executePutRule(PutRuleRequest putRuleRequest) {
ExecutionContext executionContext = createExecutionContext(putRuleRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new PutRuleRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(putRuleRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "EventBridge");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "PutRule");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true).withHasStreamingSuccessResponse(false), new PutRuleResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Adds the specified targets to the specified rule, or updates the targets if they're already associated with the
* rule.
*
*
* Targets are the resources that are invoked when a rule is triggered.
*
*
* You can configure the following as targets in EventBridge:
*
*
* -
*
* EC2 instances
*
*
* -
*
* SSM Run Command
*
*
* -
*
* SSM Automation
*
*
* -
*
* AWS Lambda functions
*
*
* -
*
* Data streams in Amazon Kinesis Data Streams
*
*
* -
*
* Data delivery streams in Amazon Kinesis Data Firehose
*
*
* -
*
* Amazon ECS tasks
*
*
* -
*
* AWS Step Functions state machines
*
*
* -
*
* AWS Batch jobs
*
*
* -
*
* AWS CodeBuild projects
*
*
* -
*
* Pipelines in AWS CodePipeline
*
*
* -
*
* Amazon Inspector assessment templates
*
*
* -
*
* Amazon SNS topics
*
*
* -
*
* Amazon SQS queues, including FIFO queues
*
*
* -
*
* The default event bus of another AWS account
*
*
*
*
* Creating rules with built-in targets is supported only on the AWS Management Console. The built-in targets are
* EC2 CreateSnapshot API call
, EC2 RebootInstances API call
,
* EC2 StopInstances API call
, and EC2 TerminateInstances API call
.
*
*
* For some target types, PutTargets
provides target-specific parameters. If the target is a Kinesis
* data stream, you can optionally specify which shard the event goes to by using the KinesisParameters
* argument. To invoke a command on multiple EC2 instances with one rule, you can use the
* RunCommandParameters
field.
*
*
* To be able to make API calls against the resources that you own, Amazon EventBridge needs the appropriate
* permissions. For AWS Lambda and Amazon SNS resources, EventBridge relies on resource-based policies. For EC2
* instances, Kinesis data streams, and AWS Step Functions state machines, EventBridge relies on IAM roles that you
* specify in the RoleARN
argument in PutTargets
. For more information, see Authentication and Access Control in the Amazon EventBridge User Guide.
*
*
* If another AWS account is in the same Region and has granted you permission (using PutPermission
),
* you can send events to that account. Set that account's event bus as a target of the rules in your account. To
* send the matched events to the other account, specify that account's event bus as the Arn
value when
* you run PutTargets
. If your account sends events to another account, your account is charged for
* each sent event. Each event sent to another account is charged as a custom event. The account receiving the event
* isn't charged. For more information, see Amazon EventBridge
* Pricing.
*
*
* If you're setting an event bus in another account as the target and that account granted permission to your
* account through an organization instead of directly by the account ID, you must specify a RoleArn
* with proper permissions in the Target
structure. For more information, see Sending and Receiving Events Between AWS Accounts in the Amazon EventBridge User Guide.
*
*
* For more information about enabling cross-account events, see PutPermission.
*
*
* Input
, InputPath
, and InputTransformer
are mutually exclusive and optional
* parameters of a target. When a rule is triggered due to a matched event:
*
*
* -
*
* If none of the following arguments are specified for a target, the entire event is passed to the target in JSON
* format (unless the target is Amazon EC2 Run Command or Amazon ECS task, in which case nothing from the event is
* passed to the target).
*
*
* -
*
* If Input
is specified in the form of valid JSON, then the matched event is overridden with this
* constant.
*
*
* -
*
* If InputPath
is specified in the form of JSONPath (for example, $.detail
), only the
* part of the event specified in the path is passed to the target (for example, only the detail part of the event
* is passed).
*
*
* -
*
* If InputTransformer
is specified, one or more specified JSONPaths are extracted from the event and
* used as values in a template that you specify as the input to the target.
*
*
*
*
* When you specify InputPath
or InputTransformer
, you must use JSON dot notation, not
* bracket notation.
*
*
* When you add targets to a rule and the associated rule triggers soon after, new or updated targets might not be
* immediately invoked. Allow a short period of time for changes to take effect.
*
*
* This action can partially fail if too many requests are made at the same time. If that happens,
* FailedEntryCount
is nonzero in the response, and each entry in FailedEntries
provides
* the ID of the failed target and the error code.
*
*
* @param putTargetsRequest
* @return Result of the PutTargets operation returned by the service.
* @throws ResourceNotFoundException
* An entity that you specified doesn't exist.
* @throws ConcurrentModificationException
* There is concurrent modification on a resource.
* @throws LimitExceededException
* You tried to create more resources than is allowed.
* @throws ManagedRuleException
* An AWS service created this rule on behalf of your account. That service manages it. If you see this
* error in response to DeleteRule
or RemoveTargets
, you can use the
* Force
parameter in those calls to delete the rule or remove targets from the rule. You can't
* modify these managed rules by using DisableRule
, EnableRule
,
* PutTargets
, PutRule
, TagResource
, or UntagResource
.
* @throws InternalException
* This exception occurs due to unexpected causes.
* @sample AmazonEventBridge.PutTargets
* @see AWS API
* Documentation
*/
@Override
public PutTargetsResult putTargets(PutTargetsRequest request) {
request = beforeClientExecution(request);
return executePutTargets(request);
}
@SdkInternalApi
final PutTargetsResult executePutTargets(PutTargetsRequest putTargetsRequest) {
ExecutionContext executionContext = createExecutionContext(putTargetsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new PutTargetsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(putTargetsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "EventBridge");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "PutTargets");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true).withHasStreamingSuccessResponse(false), new PutTargetsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Revokes the permission of another AWS account to be able to put events to the specified event bus. Specify the
* account to revoke by the StatementId
value that you associated with the account when you granted it
* permission with PutPermission
. You can find the StatementId
by using
* DescribeEventBus.
*
*
* @param removePermissionRequest
* @return Result of the RemovePermission operation returned by the service.
* @throws ResourceNotFoundException
* An entity that you specified doesn't exist.
* @throws InternalException
* This exception occurs due to unexpected causes.
* @throws ConcurrentModificationException
* There is concurrent modification on a resource.
* @sample AmazonEventBridge.RemovePermission
* @see AWS
* API Documentation
*/
@Override
public RemovePermissionResult removePermission(RemovePermissionRequest request) {
request = beforeClientExecution(request);
return executeRemovePermission(request);
}
@SdkInternalApi
final RemovePermissionResult executeRemovePermission(RemovePermissionRequest removePermissionRequest) {
ExecutionContext executionContext = createExecutionContext(removePermissionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new RemovePermissionRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(removePermissionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "EventBridge");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "RemovePermission");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new RemovePermissionResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Removes the specified targets from the specified rule. When the rule is triggered, those targets are no longer be
* invoked.
*
*
* When you remove a target, when the associated rule triggers, removed targets might continue to be invoked. Allow
* a short period of time for changes to take effect.
*
*
* This action can partially fail if too many requests are made at the same time. If that happens,
* FailedEntryCount
is non-zero in the response and each entry in FailedEntries
provides
* the ID of the failed target and the error code.
*
*
* @param removeTargetsRequest
* @return Result of the RemoveTargets operation returned by the service.
* @throws ResourceNotFoundException
* An entity that you specified doesn't exist.
* @throws ConcurrentModificationException
* There is concurrent modification on a resource.
* @throws ManagedRuleException
* An AWS service created this rule on behalf of your account. That service manages it. If you see this
* error in response to DeleteRule
or RemoveTargets
, you can use the
* Force
parameter in those calls to delete the rule or remove targets from the rule. You can't
* modify these managed rules by using DisableRule
, EnableRule
,
* PutTargets
, PutRule
, TagResource
, or UntagResource
.
* @throws InternalException
* This exception occurs due to unexpected causes.
* @sample AmazonEventBridge.RemoveTargets
* @see AWS API
* Documentation
*/
@Override
public RemoveTargetsResult removeTargets(RemoveTargetsRequest request) {
request = beforeClientExecution(request);
return executeRemoveTargets(request);
}
@SdkInternalApi
final RemoveTargetsResult executeRemoveTargets(RemoveTargetsRequest removeTargetsRequest) {
ExecutionContext executionContext = createExecutionContext(removeTargetsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new RemoveTargetsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(removeTargetsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "EventBridge");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "RemoveTargets");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new RemoveTargetsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Assigns one or more tags (key-value pairs) to the specified EventBridge resource. Tags can help you organize and
* categorize your resources. You can also use them to scope user permissions by granting a user permission to
* access or change only resources with certain tag values. In EventBridge, rules can be tagged.
*
*
* Tags don't have any semantic meaning to AWS and are interpreted strictly as strings of characters.
*
*
* You can use the TagResource
action with a rule that already has tags. If you specify a new tag key
* for the rule, this tag is appended to the list of tags associated with the rule. If you specify a tag key that is
* already associated with the rule, the new tag value that you specify replaces the previous value for that tag.
*
*
* You can associate as many as 50 tags with a resource.
*
*
* @param tagResourceRequest
* @return Result of the TagResource operation returned by the service.
* @throws ResourceNotFoundException
* An entity that you specified doesn't exist.
* @throws ConcurrentModificationException
* There is concurrent modification on a resource.
* @throws InternalException
* This exception occurs due to unexpected causes.
* @throws ManagedRuleException
* An AWS service created this rule on behalf of your account. That service manages it. If you see this
* error in response to DeleteRule
or RemoveTargets
, you can use the
* Force
parameter in those calls to delete the rule or remove targets from the rule. You can't
* modify these managed rules by using DisableRule
, EnableRule
,
* PutTargets
, PutRule
, TagResource
, or UntagResource
.
* @sample AmazonEventBridge.TagResource
* @see AWS API
* Documentation
*/
@Override
public TagResourceResult tagResource(TagResourceRequest request) {
request = beforeClientExecution(request);
return executeTagResource(request);
}
@SdkInternalApi
final TagResourceResult executeTagResource(TagResourceRequest tagResourceRequest) {
ExecutionContext executionContext = createExecutionContext(tagResourceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new TagResourceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(tagResourceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "EventBridge");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "TagResource");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new TagResourceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Tests whether the specified event pattern matches the provided event.
*
*
* Most services in AWS treat :
or /
as the same character in Amazon Resource Names
* (ARNs). However, EventBridge uses an exact match in event patterns and rules. Be sure to use the correct ARN
* characters when creating event patterns so that they match the ARN syntax in the event that you want to match.
*
*
* @param testEventPatternRequest
* @return Result of the TestEventPattern operation returned by the service.
* @throws InvalidEventPatternException
* The event pattern isn't valid.
* @throws InternalException
* This exception occurs due to unexpected causes.
* @sample AmazonEventBridge.TestEventPattern
* @see AWS
* API Documentation
*/
@Override
public TestEventPatternResult testEventPattern(TestEventPatternRequest request) {
request = beforeClientExecution(request);
return executeTestEventPattern(request);
}
@SdkInternalApi
final TestEventPatternResult executeTestEventPattern(TestEventPatternRequest testEventPatternRequest) {
ExecutionContext executionContext = createExecutionContext(testEventPatternRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new TestEventPatternRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(testEventPatternRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "EventBridge");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "TestEventPattern");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new TestEventPatternResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Removes one or more tags from the specified EventBridge resource. In EventBridge, rules can be tagged.
*
*
* @param untagResourceRequest
* @return Result of the UntagResource operation returned by the service.
* @throws ResourceNotFoundException
* An entity that you specified doesn't exist.
* @throws InternalException
* This exception occurs due to unexpected causes.
* @throws ConcurrentModificationException
* There is concurrent modification on a resource.
* @throws ManagedRuleException
* An AWS service created this rule on behalf of your account. That service manages it. If you see this
* error in response to DeleteRule
or RemoveTargets
, you can use the
* Force
parameter in those calls to delete the rule or remove targets from the rule. You can't
* modify these managed rules by using DisableRule
, EnableRule
,
* PutTargets
, PutRule
, TagResource
, or UntagResource
.
* @sample AmazonEventBridge.UntagResource
* @see AWS API
* Documentation
*/
@Override
public UntagResourceResult untagResource(UntagResourceRequest request) {
request = beforeClientExecution(request);
return executeUntagResource(request);
}
@SdkInternalApi
final UntagResourceResult executeUntagResource(UntagResourceRequest untagResourceRequest) {
ExecutionContext executionContext = createExecutionContext(untagResourceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UntagResourceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(untagResourceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "EventBridge");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UntagResource");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new UntagResourceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
* Returns additional metadata for a previously executed successful, request, typically used for debugging issues
* where a service isn't acting as expected. This data isn't considered part of the result data returned by an
* operation, so it's available through this separate, diagnostic interface.
*
* Response metadata is only cached for a limited period of time, so if you need to access this extra diagnostic
* information for an executed request, you should use this method to retrieve it as soon as possible after
* executing the request.
*
* @param request
* The originally executed request
*
* @return The response metadata for the specified request, or null if none is available.
*/
public ResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request) {
return client.getResponseMetadataForRequest(request);
}
/**
* Normal invoke with authentication. Credentials are required and may be overriden at the request level.
**/
private Response invoke(Request request, HttpResponseHandler> responseHandler,
ExecutionContext executionContext) {
return invoke(request, responseHandler, executionContext, null, null);
}
/**
* Normal invoke with authentication. Credentials are required and may be overriden at the request level.
**/
private Response invoke(Request request, HttpResponseHandler> responseHandler,
ExecutionContext executionContext, URI cachedEndpoint, URI uriFromEndpointTrait) {
executionContext.setCredentialsProvider(CredentialUtils.getCredentialsProvider(request.getOriginalRequest(), awsCredentialsProvider));
return doInvoke(request, responseHandler, executionContext, cachedEndpoint, uriFromEndpointTrait);
}
/**
* Invoke with no authentication. Credentials are not required and any credentials set on the client or request will
* be ignored for this operation.
**/
private Response anonymousInvoke(Request request,
HttpResponseHandler> responseHandler, ExecutionContext executionContext) {
return doInvoke(request, responseHandler, executionContext, null, null);
}
/**
* Invoke the request using the http client. Assumes credentials (or lack thereof) have been configured in the
* ExecutionContext beforehand.
**/
private Response doInvoke(Request request, HttpResponseHandler> responseHandler,
ExecutionContext executionContext, URI discoveredEndpoint, URI uriFromEndpointTrait) {
if (discoveredEndpoint != null) {
request.setEndpoint(discoveredEndpoint);
request.getOriginalRequest().getRequestClientOptions().appendUserAgent("endpoint-discovery");
} else if (uriFromEndpointTrait != null) {
request.setEndpoint(uriFromEndpointTrait);
} else {
request.setEndpoint(endpoint);
}
request.setTimeOffset(timeOffset);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler(new JsonErrorResponseMetadata());
return client.execute(request, responseHandler, errorResponseHandler, executionContext);
}
@com.amazonaws.annotation.SdkInternalApi
static com.amazonaws.protocol.json.SdkJsonProtocolFactory getProtocolFactory() {
return protocolFactory;
}
}