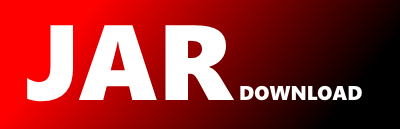
com.amazonaws.services.eventbridge.model.PutPermissionRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-eventbridge Show documentation
/*
* Copyright 2018-2023 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.eventbridge.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class PutPermissionRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The name of the event bus associated with the rule. If you omit this, the default event bus is used.
*
*/
private String eventBusName;
/**
*
* The action that you are enabling the other account to perform.
*
*/
private String action;
/**
*
* The 12-digit Amazon Web Services account ID that you are permitting to put events to your default event bus.
* Specify "*" to permit any account to put events to your default event bus.
*
*
* If you specify "*" without specifying Condition
, avoid creating rules that may match undesirable
* events. To create more secure rules, make sure that the event pattern for each rule contains an
* account
field with a specific account ID from which to receive events. Rules with an account field
* do not match any events sent from other accounts.
*
*/
private String principal;
/**
*
* An identifier string for the external account that you are granting permissions to. If you later want to revoke
* the permission for this external account, specify this StatementId
when you run RemovePermission.
*
*
*
* Each StatementId
must be unique.
*
*
*/
private String statementId;
/**
*
* This parameter enables you to limit the permission to accounts that fulfill a certain condition, such as being a
* member of a certain Amazon Web Services organization. For more information about Amazon Web Services
* Organizations, see What Is Amazon Web
* Services Organizations in the Amazon Web Services Organizations User Guide.
*
*
* If you specify Condition
with an Amazon Web Services organization ID, and specify "*" as the value
* for Principal
, you grant permission to all the accounts in the named organization.
*
*
* The Condition
is a JSON string which must contain Type
, Key
, and
* Value
fields.
*
*/
private Condition condition;
/**
*
* A JSON string that describes the permission policy statement. You can include a Policy
parameter in
* the request instead of using the StatementId
, Action
, Principal
, or
* Condition
parameters.
*
*/
private String policy;
/**
*
* The name of the event bus associated with the rule. If you omit this, the default event bus is used.
*
*
* @param eventBusName
* The name of the event bus associated with the rule. If you omit this, the default event bus is used.
*/
public void setEventBusName(String eventBusName) {
this.eventBusName = eventBusName;
}
/**
*
* The name of the event bus associated with the rule. If you omit this, the default event bus is used.
*
*
* @return The name of the event bus associated with the rule. If you omit this, the default event bus is used.
*/
public String getEventBusName() {
return this.eventBusName;
}
/**
*
* The name of the event bus associated with the rule. If you omit this, the default event bus is used.
*
*
* @param eventBusName
* The name of the event bus associated with the rule. If you omit this, the default event bus is used.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PutPermissionRequest withEventBusName(String eventBusName) {
setEventBusName(eventBusName);
return this;
}
/**
*
* The action that you are enabling the other account to perform.
*
*
* @param action
* The action that you are enabling the other account to perform.
*/
public void setAction(String action) {
this.action = action;
}
/**
*
* The action that you are enabling the other account to perform.
*
*
* @return The action that you are enabling the other account to perform.
*/
public String getAction() {
return this.action;
}
/**
*
* The action that you are enabling the other account to perform.
*
*
* @param action
* The action that you are enabling the other account to perform.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PutPermissionRequest withAction(String action) {
setAction(action);
return this;
}
/**
*
* The 12-digit Amazon Web Services account ID that you are permitting to put events to your default event bus.
* Specify "*" to permit any account to put events to your default event bus.
*
*
* If you specify "*" without specifying Condition
, avoid creating rules that may match undesirable
* events. To create more secure rules, make sure that the event pattern for each rule contains an
* account
field with a specific account ID from which to receive events. Rules with an account field
* do not match any events sent from other accounts.
*
*
* @param principal
* The 12-digit Amazon Web Services account ID that you are permitting to put events to your default event
* bus. Specify "*" to permit any account to put events to your default event bus.
*
* If you specify "*" without specifying Condition
, avoid creating rules that may match
* undesirable events. To create more secure rules, make sure that the event pattern for each rule contains
* an account
field with a specific account ID from which to receive events. Rules with an
* account field do not match any events sent from other accounts.
*/
public void setPrincipal(String principal) {
this.principal = principal;
}
/**
*
* The 12-digit Amazon Web Services account ID that you are permitting to put events to your default event bus.
* Specify "*" to permit any account to put events to your default event bus.
*
*
* If you specify "*" without specifying Condition
, avoid creating rules that may match undesirable
* events. To create more secure rules, make sure that the event pattern for each rule contains an
* account
field with a specific account ID from which to receive events. Rules with an account field
* do not match any events sent from other accounts.
*
*
* @return The 12-digit Amazon Web Services account ID that you are permitting to put events to your default event
* bus. Specify "*" to permit any account to put events to your default event bus.
*
* If you specify "*" without specifying Condition
, avoid creating rules that may match
* undesirable events. To create more secure rules, make sure that the event pattern for each rule contains
* an account
field with a specific account ID from which to receive events. Rules with an
* account field do not match any events sent from other accounts.
*/
public String getPrincipal() {
return this.principal;
}
/**
*
* The 12-digit Amazon Web Services account ID that you are permitting to put events to your default event bus.
* Specify "*" to permit any account to put events to your default event bus.
*
*
* If you specify "*" without specifying Condition
, avoid creating rules that may match undesirable
* events. To create more secure rules, make sure that the event pattern for each rule contains an
* account
field with a specific account ID from which to receive events. Rules with an account field
* do not match any events sent from other accounts.
*
*
* @param principal
* The 12-digit Amazon Web Services account ID that you are permitting to put events to your default event
* bus. Specify "*" to permit any account to put events to your default event bus.
*
* If you specify "*" without specifying Condition
, avoid creating rules that may match
* undesirable events. To create more secure rules, make sure that the event pattern for each rule contains
* an account
field with a specific account ID from which to receive events. Rules with an
* account field do not match any events sent from other accounts.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PutPermissionRequest withPrincipal(String principal) {
setPrincipal(principal);
return this;
}
/**
*
* An identifier string for the external account that you are granting permissions to. If you later want to revoke
* the permission for this external account, specify this StatementId
when you run RemovePermission.
*
*
*
* Each StatementId
must be unique.
*
*
*
* @param statementId
* An identifier string for the external account that you are granting permissions to. If you later want to
* revoke the permission for this external account, specify this StatementId
when you run RemovePermission
* .
*
* Each StatementId
must be unique.
*
*/
public void setStatementId(String statementId) {
this.statementId = statementId;
}
/**
*
* An identifier string for the external account that you are granting permissions to. If you later want to revoke
* the permission for this external account, specify this StatementId
when you run RemovePermission.
*
*
*
* Each StatementId
must be unique.
*
*
*
* @return An identifier string for the external account that you are granting permissions to. If you later want to
* revoke the permission for this external account, specify this StatementId
when you run
* RemovePermission.
*
* Each StatementId
must be unique.
*
*/
public String getStatementId() {
return this.statementId;
}
/**
*
* An identifier string for the external account that you are granting permissions to. If you later want to revoke
* the permission for this external account, specify this StatementId
when you run RemovePermission.
*
*
*
* Each StatementId
must be unique.
*
*
*
* @param statementId
* An identifier string for the external account that you are granting permissions to. If you later want to
* revoke the permission for this external account, specify this StatementId
when you run RemovePermission
* .
*
* Each StatementId
must be unique.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PutPermissionRequest withStatementId(String statementId) {
setStatementId(statementId);
return this;
}
/**
*
* This parameter enables you to limit the permission to accounts that fulfill a certain condition, such as being a
* member of a certain Amazon Web Services organization. For more information about Amazon Web Services
* Organizations, see What Is Amazon Web
* Services Organizations in the Amazon Web Services Organizations User Guide.
*
*
* If you specify Condition
with an Amazon Web Services organization ID, and specify "*" as the value
* for Principal
, you grant permission to all the accounts in the named organization.
*
*
* The Condition
is a JSON string which must contain Type
, Key
, and
* Value
fields.
*
*
* @param condition
* This parameter enables you to limit the permission to accounts that fulfill a certain condition, such as
* being a member of a certain Amazon Web Services organization. For more information about Amazon Web
* Services Organizations, see What Is Amazon
* Web Services Organizations in the Amazon Web Services Organizations User Guide.
*
* If you specify Condition
with an Amazon Web Services organization ID, and specify "*" as the
* value for Principal
, you grant permission to all the accounts in the named organization.
*
*
* The Condition
is a JSON string which must contain Type
, Key
, and
* Value
fields.
*/
public void setCondition(Condition condition) {
this.condition = condition;
}
/**
*
* This parameter enables you to limit the permission to accounts that fulfill a certain condition, such as being a
* member of a certain Amazon Web Services organization. For more information about Amazon Web Services
* Organizations, see What Is Amazon Web
* Services Organizations in the Amazon Web Services Organizations User Guide.
*
*
* If you specify Condition
with an Amazon Web Services organization ID, and specify "*" as the value
* for Principal
, you grant permission to all the accounts in the named organization.
*
*
* The Condition
is a JSON string which must contain Type
, Key
, and
* Value
fields.
*
*
* @return This parameter enables you to limit the permission to accounts that fulfill a certain condition, such as
* being a member of a certain Amazon Web Services organization. For more information about Amazon Web
* Services Organizations, see What Is Amazon
* Web Services Organizations in the Amazon Web Services Organizations User Guide.
*
* If you specify Condition
with an Amazon Web Services organization ID, and specify "*" as the
* value for Principal
, you grant permission to all the accounts in the named organization.
*
*
* The Condition
is a JSON string which must contain Type
, Key
, and
* Value
fields.
*/
public Condition getCondition() {
return this.condition;
}
/**
*
* This parameter enables you to limit the permission to accounts that fulfill a certain condition, such as being a
* member of a certain Amazon Web Services organization. For more information about Amazon Web Services
* Organizations, see What Is Amazon Web
* Services Organizations in the Amazon Web Services Organizations User Guide.
*
*
* If you specify Condition
with an Amazon Web Services organization ID, and specify "*" as the value
* for Principal
, you grant permission to all the accounts in the named organization.
*
*
* The Condition
is a JSON string which must contain Type
, Key
, and
* Value
fields.
*
*
* @param condition
* This parameter enables you to limit the permission to accounts that fulfill a certain condition, such as
* being a member of a certain Amazon Web Services organization. For more information about Amazon Web
* Services Organizations, see What Is Amazon
* Web Services Organizations in the Amazon Web Services Organizations User Guide.
*
* If you specify Condition
with an Amazon Web Services organization ID, and specify "*" as the
* value for Principal
, you grant permission to all the accounts in the named organization.
*
*
* The Condition
is a JSON string which must contain Type
, Key
, and
* Value
fields.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PutPermissionRequest withCondition(Condition condition) {
setCondition(condition);
return this;
}
/**
*
* A JSON string that describes the permission policy statement. You can include a Policy
parameter in
* the request instead of using the StatementId
, Action
, Principal
, or
* Condition
parameters.
*
*
* @param policy
* A JSON string that describes the permission policy statement. You can include a Policy
* parameter in the request instead of using the StatementId
, Action
,
* Principal
, or Condition
parameters.
*/
public void setPolicy(String policy) {
this.policy = policy;
}
/**
*
* A JSON string that describes the permission policy statement. You can include a Policy
parameter in
* the request instead of using the StatementId
, Action
, Principal
, or
* Condition
parameters.
*
*
* @return A JSON string that describes the permission policy statement. You can include a Policy
* parameter in the request instead of using the StatementId
, Action
,
* Principal
, or Condition
parameters.
*/
public String getPolicy() {
return this.policy;
}
/**
*
* A JSON string that describes the permission policy statement. You can include a Policy
parameter in
* the request instead of using the StatementId
, Action
, Principal
, or
* Condition
parameters.
*
*
* @param policy
* A JSON string that describes the permission policy statement. You can include a Policy
* parameter in the request instead of using the StatementId
, Action
,
* Principal
, or Condition
parameters.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PutPermissionRequest withPolicy(String policy) {
setPolicy(policy);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getEventBusName() != null)
sb.append("EventBusName: ").append(getEventBusName()).append(",");
if (getAction() != null)
sb.append("Action: ").append(getAction()).append(",");
if (getPrincipal() != null)
sb.append("Principal: ").append(getPrincipal()).append(",");
if (getStatementId() != null)
sb.append("StatementId: ").append(getStatementId()).append(",");
if (getCondition() != null)
sb.append("Condition: ").append(getCondition()).append(",");
if (getPolicy() != null)
sb.append("Policy: ").append(getPolicy());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof PutPermissionRequest == false)
return false;
PutPermissionRequest other = (PutPermissionRequest) obj;
if (other.getEventBusName() == null ^ this.getEventBusName() == null)
return false;
if (other.getEventBusName() != null && other.getEventBusName().equals(this.getEventBusName()) == false)
return false;
if (other.getAction() == null ^ this.getAction() == null)
return false;
if (other.getAction() != null && other.getAction().equals(this.getAction()) == false)
return false;
if (other.getPrincipal() == null ^ this.getPrincipal() == null)
return false;
if (other.getPrincipal() != null && other.getPrincipal().equals(this.getPrincipal()) == false)
return false;
if (other.getStatementId() == null ^ this.getStatementId() == null)
return false;
if (other.getStatementId() != null && other.getStatementId().equals(this.getStatementId()) == false)
return false;
if (other.getCondition() == null ^ this.getCondition() == null)
return false;
if (other.getCondition() != null && other.getCondition().equals(this.getCondition()) == false)
return false;
if (other.getPolicy() == null ^ this.getPolicy() == null)
return false;
if (other.getPolicy() != null && other.getPolicy().equals(this.getPolicy()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getEventBusName() == null) ? 0 : getEventBusName().hashCode());
hashCode = prime * hashCode + ((getAction() == null) ? 0 : getAction().hashCode());
hashCode = prime * hashCode + ((getPrincipal() == null) ? 0 : getPrincipal().hashCode());
hashCode = prime * hashCode + ((getStatementId() == null) ? 0 : getStatementId().hashCode());
hashCode = prime * hashCode + ((getCondition() == null) ? 0 : getCondition().hashCode());
hashCode = prime * hashCode + ((getPolicy() == null) ? 0 : getPolicy().hashCode());
return hashCode;
}
@Override
public PutPermissionRequest clone() {
return (PutPermissionRequest) super.clone();
}
}