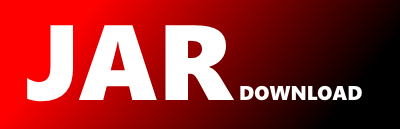
com.amazonaws.services.eventbridge.model.PutRuleRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-eventbridge Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.eventbridge.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class PutRuleRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The name of the rule that you are creating or updating.
*
*/
private String name;
/**
*
* The scheduling expression. For example, "cron(0 20 * * ? *)" or "rate(5 minutes)".
*
*/
private String scheduleExpression;
/**
*
* The event pattern. For more information, see Amazon EventBridge event
* patterns in the Amazon EventBridge User Guide .
*
*/
private String eventPattern;
/**
*
* The state of the rule.
*
*
* Valid values include:
*
*
* -
*
* DISABLED
: The rule is disabled. EventBridge does not match any events against the rule.
*
*
* -
*
* ENABLED
: The rule is enabled. EventBridge matches events against the rule, except for Amazon
* Web Services management events delivered through CloudTrail.
*
*
* -
*
* ENABLED_WITH_ALL_CLOUDTRAIL_MANAGEMENT_EVENTS
: The rule is enabled for all events, including Amazon
* Web Services management events delivered through CloudTrail.
*
*
* Management events provide visibility into management operations that are performed on resources in your Amazon
* Web Services account. These are also known as control plane operations. For more information, see Logging management events in the CloudTrail User Guide, and Filtering management events from Amazon Web Services services in the Amazon EventBridge User
* Guide .
*
*
* This value is only valid for rules on the default event bus or custom event buses.
* It does not apply to partner
* event buses.
*
*
*
*/
private String state;
/**
*
* A description of the rule.
*
*/
private String description;
/**
*
* The Amazon Resource Name (ARN) of the IAM role associated with the rule.
*
*
* If you're setting an event bus in another account as the target and that account granted permission to your
* account through an organization instead of directly by the account ID, you must specify a RoleArn
* with proper permissions in the Target
structure, instead of here in this parameter.
*
*/
private String roleArn;
/**
*
* The list of key-value pairs to associate with the rule.
*
*/
private java.util.List tags;
/**
*
* The name or ARN of the event bus to associate with this rule. If you omit this, the default event bus is used.
*
*/
private String eventBusName;
/**
*
* The name of the rule that you are creating or updating.
*
*
* @param name
* The name of the rule that you are creating or updating.
*/
public void setName(String name) {
this.name = name;
}
/**
*
* The name of the rule that you are creating or updating.
*
*
* @return The name of the rule that you are creating or updating.
*/
public String getName() {
return this.name;
}
/**
*
* The name of the rule that you are creating or updating.
*
*
* @param name
* The name of the rule that you are creating or updating.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PutRuleRequest withName(String name) {
setName(name);
return this;
}
/**
*
* The scheduling expression. For example, "cron(0 20 * * ? *)" or "rate(5 minutes)".
*
*
* @param scheduleExpression
* The scheduling expression. For example, "cron(0 20 * * ? *)" or "rate(5 minutes)".
*/
public void setScheduleExpression(String scheduleExpression) {
this.scheduleExpression = scheduleExpression;
}
/**
*
* The scheduling expression. For example, "cron(0 20 * * ? *)" or "rate(5 minutes)".
*
*
* @return The scheduling expression. For example, "cron(0 20 * * ? *)" or "rate(5 minutes)".
*/
public String getScheduleExpression() {
return this.scheduleExpression;
}
/**
*
* The scheduling expression. For example, "cron(0 20 * * ? *)" or "rate(5 minutes)".
*
*
* @param scheduleExpression
* The scheduling expression. For example, "cron(0 20 * * ? *)" or "rate(5 minutes)".
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PutRuleRequest withScheduleExpression(String scheduleExpression) {
setScheduleExpression(scheduleExpression);
return this;
}
/**
*
* The event pattern. For more information, see Amazon EventBridge event
* patterns in the Amazon EventBridge User Guide .
*
*
* @param eventPattern
* The event pattern. For more information, see Amazon EventBridge
* event patterns in the Amazon EventBridge User Guide .
*/
public void setEventPattern(String eventPattern) {
this.eventPattern = eventPattern;
}
/**
*
* The event pattern. For more information, see Amazon EventBridge event
* patterns in the Amazon EventBridge User Guide .
*
*
* @return The event pattern. For more information, see Amazon EventBridge
* event patterns in the Amazon EventBridge User Guide .
*/
public String getEventPattern() {
return this.eventPattern;
}
/**
*
* The event pattern. For more information, see Amazon EventBridge event
* patterns in the Amazon EventBridge User Guide .
*
*
* @param eventPattern
* The event pattern. For more information, see Amazon EventBridge
* event patterns in the Amazon EventBridge User Guide .
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PutRuleRequest withEventPattern(String eventPattern) {
setEventPattern(eventPattern);
return this;
}
/**
*
* The state of the rule.
*
*
* Valid values include:
*
*
* -
*
* DISABLED
: The rule is disabled. EventBridge does not match any events against the rule.
*
*
* -
*
* ENABLED
: The rule is enabled. EventBridge matches events against the rule, except for Amazon
* Web Services management events delivered through CloudTrail.
*
*
* -
*
* ENABLED_WITH_ALL_CLOUDTRAIL_MANAGEMENT_EVENTS
: The rule is enabled for all events, including Amazon
* Web Services management events delivered through CloudTrail.
*
*
* Management events provide visibility into management operations that are performed on resources in your Amazon
* Web Services account. These are also known as control plane operations. For more information, see Logging management events in the CloudTrail User Guide, and Filtering management events from Amazon Web Services services in the Amazon EventBridge User
* Guide .
*
*
* This value is only valid for rules on the default event bus or custom event buses.
* It does not apply to partner
* event buses.
*
*
*
*
* @param state
* The state of the rule.
*
* Valid values include:
*
*
* -
*
* DISABLED
: The rule is disabled. EventBridge does not match any events against the rule.
*
*
* -
*
* ENABLED
: The rule is enabled. EventBridge matches events against the rule, except for
* Amazon Web Services management events delivered through CloudTrail.
*
*
* -
*
* ENABLED_WITH_ALL_CLOUDTRAIL_MANAGEMENT_EVENTS
: The rule is enabled for all events, including
* Amazon Web Services management events delivered through CloudTrail.
*
*
* Management events provide visibility into management operations that are performed on resources in your
* Amazon Web Services account. These are also known as control plane operations. For more information, see
* Logging management events in the CloudTrail User Guide, and Filtering management events from Amazon Web Services services in the Amazon EventBridge User
* Guide .
*
*
* This value is only valid for rules on the default event bus or custom event
* buses. It does not apply to partner event buses.
*
*
* @see RuleState
*/
public void setState(String state) {
this.state = state;
}
/**
*
* The state of the rule.
*
*
* Valid values include:
*
*
* -
*
* DISABLED
: The rule is disabled. EventBridge does not match any events against the rule.
*
*
* -
*
* ENABLED
: The rule is enabled. EventBridge matches events against the rule, except for Amazon
* Web Services management events delivered through CloudTrail.
*
*
* -
*
* ENABLED_WITH_ALL_CLOUDTRAIL_MANAGEMENT_EVENTS
: The rule is enabled for all events, including Amazon
* Web Services management events delivered through CloudTrail.
*
*
* Management events provide visibility into management operations that are performed on resources in your Amazon
* Web Services account. These are also known as control plane operations. For more information, see Logging management events in the CloudTrail User Guide, and Filtering management events from Amazon Web Services services in the Amazon EventBridge User
* Guide .
*
*
* This value is only valid for rules on the default event bus or custom event buses.
* It does not apply to partner
* event buses.
*
*
*
*
* @return The state of the rule.
*
* Valid values include:
*
*
* -
*
* DISABLED
: The rule is disabled. EventBridge does not match any events against the rule.
*
*
* -
*
* ENABLED
: The rule is enabled. EventBridge matches events against the rule, except for
* Amazon Web Services management events delivered through CloudTrail.
*
*
* -
*
* ENABLED_WITH_ALL_CLOUDTRAIL_MANAGEMENT_EVENTS
: The rule is enabled for all events, including
* Amazon Web Services management events delivered through CloudTrail.
*
*
* Management events provide visibility into management operations that are performed on resources in your
* Amazon Web Services account. These are also known as control plane operations. For more information, see
* Logging management events in the CloudTrail User Guide, and Filtering management events from Amazon Web Services services in the Amazon EventBridge User
* Guide .
*
*
* This value is only valid for rules on the default event bus or custom event
* buses. It does not apply to partner event buses.
*
*
* @see RuleState
*/
public String getState() {
return this.state;
}
/**
*
* The state of the rule.
*
*
* Valid values include:
*
*
* -
*
* DISABLED
: The rule is disabled. EventBridge does not match any events against the rule.
*
*
* -
*
* ENABLED
: The rule is enabled. EventBridge matches events against the rule, except for Amazon
* Web Services management events delivered through CloudTrail.
*
*
* -
*
* ENABLED_WITH_ALL_CLOUDTRAIL_MANAGEMENT_EVENTS
: The rule is enabled for all events, including Amazon
* Web Services management events delivered through CloudTrail.
*
*
* Management events provide visibility into management operations that are performed on resources in your Amazon
* Web Services account. These are also known as control plane operations. For more information, see Logging management events in the CloudTrail User Guide, and Filtering management events from Amazon Web Services services in the Amazon EventBridge User
* Guide .
*
*
* This value is only valid for rules on the default event bus or custom event buses.
* It does not apply to partner
* event buses.
*
*
*
*
* @param state
* The state of the rule.
*
* Valid values include:
*
*
* -
*
* DISABLED
: The rule is disabled. EventBridge does not match any events against the rule.
*
*
* -
*
* ENABLED
: The rule is enabled. EventBridge matches events against the rule, except for
* Amazon Web Services management events delivered through CloudTrail.
*
*
* -
*
* ENABLED_WITH_ALL_CLOUDTRAIL_MANAGEMENT_EVENTS
: The rule is enabled for all events, including
* Amazon Web Services management events delivered through CloudTrail.
*
*
* Management events provide visibility into management operations that are performed on resources in your
* Amazon Web Services account. These are also known as control plane operations. For more information, see
* Logging management events in the CloudTrail User Guide, and Filtering management events from Amazon Web Services services in the Amazon EventBridge User
* Guide .
*
*
* This value is only valid for rules on the default event bus or custom event
* buses. It does not apply to partner event buses.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see RuleState
*/
public PutRuleRequest withState(String state) {
setState(state);
return this;
}
/**
*
* The state of the rule.
*
*
* Valid values include:
*
*
* -
*
* DISABLED
: The rule is disabled. EventBridge does not match any events against the rule.
*
*
* -
*
* ENABLED
: The rule is enabled. EventBridge matches events against the rule, except for Amazon
* Web Services management events delivered through CloudTrail.
*
*
* -
*
* ENABLED_WITH_ALL_CLOUDTRAIL_MANAGEMENT_EVENTS
: The rule is enabled for all events, including Amazon
* Web Services management events delivered through CloudTrail.
*
*
* Management events provide visibility into management operations that are performed on resources in your Amazon
* Web Services account. These are also known as control plane operations. For more information, see Logging management events in the CloudTrail User Guide, and Filtering management events from Amazon Web Services services in the Amazon EventBridge User
* Guide .
*
*
* This value is only valid for rules on the default event bus or custom event buses.
* It does not apply to partner
* event buses.
*
*
*
*
* @param state
* The state of the rule.
*
* Valid values include:
*
*
* -
*
* DISABLED
: The rule is disabled. EventBridge does not match any events against the rule.
*
*
* -
*
* ENABLED
: The rule is enabled. EventBridge matches events against the rule, except for
* Amazon Web Services management events delivered through CloudTrail.
*
*
* -
*
* ENABLED_WITH_ALL_CLOUDTRAIL_MANAGEMENT_EVENTS
: The rule is enabled for all events, including
* Amazon Web Services management events delivered through CloudTrail.
*
*
* Management events provide visibility into management operations that are performed on resources in your
* Amazon Web Services account. These are also known as control plane operations. For more information, see
* Logging management events in the CloudTrail User Guide, and Filtering management events from Amazon Web Services services in the Amazon EventBridge User
* Guide .
*
*
* This value is only valid for rules on the default event bus or custom event
* buses. It does not apply to partner event buses.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see RuleState
*/
public PutRuleRequest withState(RuleState state) {
this.state = state.toString();
return this;
}
/**
*
* A description of the rule.
*
*
* @param description
* A description of the rule.
*/
public void setDescription(String description) {
this.description = description;
}
/**
*
* A description of the rule.
*
*
* @return A description of the rule.
*/
public String getDescription() {
return this.description;
}
/**
*
* A description of the rule.
*
*
* @param description
* A description of the rule.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PutRuleRequest withDescription(String description) {
setDescription(description);
return this;
}
/**
*
* The Amazon Resource Name (ARN) of the IAM role associated with the rule.
*
*
* If you're setting an event bus in another account as the target and that account granted permission to your
* account through an organization instead of directly by the account ID, you must specify a RoleArn
* with proper permissions in the Target
structure, instead of here in this parameter.
*
*
* @param roleArn
* The Amazon Resource Name (ARN) of the IAM role associated with the rule.
*
* If you're setting an event bus in another account as the target and that account granted permission to
* your account through an organization instead of directly by the account ID, you must specify a
* RoleArn
with proper permissions in the Target
structure, instead of here in this
* parameter.
*/
public void setRoleArn(String roleArn) {
this.roleArn = roleArn;
}
/**
*
* The Amazon Resource Name (ARN) of the IAM role associated with the rule.
*
*
* If you're setting an event bus in another account as the target and that account granted permission to your
* account through an organization instead of directly by the account ID, you must specify a RoleArn
* with proper permissions in the Target
structure, instead of here in this parameter.
*
*
* @return The Amazon Resource Name (ARN) of the IAM role associated with the rule.
*
* If you're setting an event bus in another account as the target and that account granted permission to
* your account through an organization instead of directly by the account ID, you must specify a
* RoleArn
with proper permissions in the Target
structure, instead of here in
* this parameter.
*/
public String getRoleArn() {
return this.roleArn;
}
/**
*
* The Amazon Resource Name (ARN) of the IAM role associated with the rule.
*
*
* If you're setting an event bus in another account as the target and that account granted permission to your
* account through an organization instead of directly by the account ID, you must specify a RoleArn
* with proper permissions in the Target
structure, instead of here in this parameter.
*
*
* @param roleArn
* The Amazon Resource Name (ARN) of the IAM role associated with the rule.
*
* If you're setting an event bus in another account as the target and that account granted permission to
* your account through an organization instead of directly by the account ID, you must specify a
* RoleArn
with proper permissions in the Target
structure, instead of here in this
* parameter.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PutRuleRequest withRoleArn(String roleArn) {
setRoleArn(roleArn);
return this;
}
/**
*
* The list of key-value pairs to associate with the rule.
*
*
* @return The list of key-value pairs to associate with the rule.
*/
public java.util.List getTags() {
return tags;
}
/**
*
* The list of key-value pairs to associate with the rule.
*
*
* @param tags
* The list of key-value pairs to associate with the rule.
*/
public void setTags(java.util.Collection tags) {
if (tags == null) {
this.tags = null;
return;
}
this.tags = new java.util.ArrayList(tags);
}
/**
*
* The list of key-value pairs to associate with the rule.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTags(java.util.Collection)} or {@link #withTags(java.util.Collection)} if you want to override the
* existing values.
*
*
* @param tags
* The list of key-value pairs to associate with the rule.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PutRuleRequest withTags(Tag... tags) {
if (this.tags == null) {
setTags(new java.util.ArrayList(tags.length));
}
for (Tag ele : tags) {
this.tags.add(ele);
}
return this;
}
/**
*
* The list of key-value pairs to associate with the rule.
*
*
* @param tags
* The list of key-value pairs to associate with the rule.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PutRuleRequest withTags(java.util.Collection tags) {
setTags(tags);
return this;
}
/**
*
* The name or ARN of the event bus to associate with this rule. If you omit this, the default event bus is used.
*
*
* @param eventBusName
* The name or ARN of the event bus to associate with this rule. If you omit this, the default event bus is
* used.
*/
public void setEventBusName(String eventBusName) {
this.eventBusName = eventBusName;
}
/**
*
* The name or ARN of the event bus to associate with this rule. If you omit this, the default event bus is used.
*
*
* @return The name or ARN of the event bus to associate with this rule. If you omit this, the default event bus is
* used.
*/
public String getEventBusName() {
return this.eventBusName;
}
/**
*
* The name or ARN of the event bus to associate with this rule. If you omit this, the default event bus is used.
*
*
* @param eventBusName
* The name or ARN of the event bus to associate with this rule. If you omit this, the default event bus is
* used.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PutRuleRequest withEventBusName(String eventBusName) {
setEventBusName(eventBusName);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getName() != null)
sb.append("Name: ").append(getName()).append(",");
if (getScheduleExpression() != null)
sb.append("ScheduleExpression: ").append(getScheduleExpression()).append(",");
if (getEventPattern() != null)
sb.append("EventPattern: ").append(getEventPattern()).append(",");
if (getState() != null)
sb.append("State: ").append(getState()).append(",");
if (getDescription() != null)
sb.append("Description: ").append(getDescription()).append(",");
if (getRoleArn() != null)
sb.append("RoleArn: ").append(getRoleArn()).append(",");
if (getTags() != null)
sb.append("Tags: ").append(getTags()).append(",");
if (getEventBusName() != null)
sb.append("EventBusName: ").append(getEventBusName());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof PutRuleRequest == false)
return false;
PutRuleRequest other = (PutRuleRequest) obj;
if (other.getName() == null ^ this.getName() == null)
return false;
if (other.getName() != null && other.getName().equals(this.getName()) == false)
return false;
if (other.getScheduleExpression() == null ^ this.getScheduleExpression() == null)
return false;
if (other.getScheduleExpression() != null && other.getScheduleExpression().equals(this.getScheduleExpression()) == false)
return false;
if (other.getEventPattern() == null ^ this.getEventPattern() == null)
return false;
if (other.getEventPattern() != null && other.getEventPattern().equals(this.getEventPattern()) == false)
return false;
if (other.getState() == null ^ this.getState() == null)
return false;
if (other.getState() != null && other.getState().equals(this.getState()) == false)
return false;
if (other.getDescription() == null ^ this.getDescription() == null)
return false;
if (other.getDescription() != null && other.getDescription().equals(this.getDescription()) == false)
return false;
if (other.getRoleArn() == null ^ this.getRoleArn() == null)
return false;
if (other.getRoleArn() != null && other.getRoleArn().equals(this.getRoleArn()) == false)
return false;
if (other.getTags() == null ^ this.getTags() == null)
return false;
if (other.getTags() != null && other.getTags().equals(this.getTags()) == false)
return false;
if (other.getEventBusName() == null ^ this.getEventBusName() == null)
return false;
if (other.getEventBusName() != null && other.getEventBusName().equals(this.getEventBusName()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getName() == null) ? 0 : getName().hashCode());
hashCode = prime * hashCode + ((getScheduleExpression() == null) ? 0 : getScheduleExpression().hashCode());
hashCode = prime * hashCode + ((getEventPattern() == null) ? 0 : getEventPattern().hashCode());
hashCode = prime * hashCode + ((getState() == null) ? 0 : getState().hashCode());
hashCode = prime * hashCode + ((getDescription() == null) ? 0 : getDescription().hashCode());
hashCode = prime * hashCode + ((getRoleArn() == null) ? 0 : getRoleArn().hashCode());
hashCode = prime * hashCode + ((getTags() == null) ? 0 : getTags().hashCode());
hashCode = prime * hashCode + ((getEventBusName() == null) ? 0 : getEventBusName().hashCode());
return hashCode;
}
@Override
public PutRuleRequest clone() {
return (PutRuleRequest) super.clone();
}
}