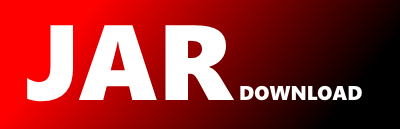
com.amazonaws.services.eventbridge.model.Target Maven / Gradle / Ivy
Show all versions of aws-java-sdk-eventbridge Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.eventbridge.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Targets are the resources to be invoked when a rule is triggered. For a complete list of services and resources that
* can be set as a target, see PutTargets.
*
*
* If you are setting the event bus of another account as the target, and that account granted permission to your
* account through an organization instead of directly by the account ID, then you must specify a RoleArn
* with proper permissions in the Target
structure. For more information, see Sending
* and Receiving Events Between Amazon Web Services Accounts in the Amazon EventBridge User Guide.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class Target implements Serializable, Cloneable, StructuredPojo {
/**
*
* The ID of the target within the specified rule. Use this ID to reference the target when updating the rule. We
* recommend using a memorable and unique string.
*
*/
private String id;
/**
*
* The Amazon Resource Name (ARN) of the target.
*
*/
private String arn;
/**
*
* The Amazon Resource Name (ARN) of the IAM role to be used for this target when the rule is triggered. If one rule
* triggers multiple targets, you can use a different IAM role for each target.
*
*/
private String roleArn;
/**
*
* Valid JSON text passed to the target. In this case, nothing from the event itself is passed to the target. For
* more information, see The JavaScript Object Notation (JSON)
* Data Interchange Format.
*
*/
private String input;
/**
*
* The value of the JSONPath that is used for extracting part of the matched event when passing it to the target.
* You may use JSON dot notation or bracket notation. For more information about JSON paths, see JSONPath.
*
*/
private String inputPath;
/**
*
* Settings to enable you to provide custom input to a target based on certain event data. You can extract one or
* more key-value pairs from the event and then use that data to send customized input to the target.
*
*/
private InputTransformer inputTransformer;
/**
*
* The custom parameter you can use to control the shard assignment, when the target is a Kinesis data stream. If
* you do not include this parameter, the default is to use the eventId
as the partition key.
*
*/
private KinesisParameters kinesisParameters;
/**
*
* Parameters used when you are using the rule to invoke Amazon EC2 Run Command.
*
*/
private RunCommandParameters runCommandParameters;
/**
*
* Contains the Amazon ECS task definition and task count to be used, if the event target is an Amazon ECS task. For
* more information about Amazon ECS tasks, see Task Definitions in
* the Amazon EC2 Container Service Developer Guide.
*
*/
private EcsParameters ecsParameters;
/**
*
* If the event target is an Batch job, this contains the job definition, job name, and other parameters. For more
* information, see Jobs in the Batch
* User Guide.
*
*/
private BatchParameters batchParameters;
/**
*
* Contains the message group ID to use when the target is a FIFO queue.
*
*
* If you specify an SQS FIFO queue as a target, the queue must have content-based deduplication enabled.
*
*/
private SqsParameters sqsParameters;
/**
*
* Contains the HTTP parameters to use when the target is a API Gateway endpoint or EventBridge ApiDestination.
*
*
* If you specify an API Gateway API or EventBridge ApiDestination as a target, you can use this parameter to
* specify headers, path parameters, and query string keys/values as part of your target invoking request. If you're
* using ApiDestinations, the corresponding Connection can also have these values configured. In case of any
* conflicting keys, values from the Connection take precedence.
*
*/
private HttpParameters httpParameters;
/**
*
* Contains the Amazon Redshift Data API parameters to use when the target is a Amazon Redshift cluster.
*
*
* If you specify a Amazon Redshift Cluster as a Target, you can use this to specify parameters to invoke the Amazon
* Redshift Data API ExecuteStatement based on EventBridge events.
*
*/
private RedshiftDataParameters redshiftDataParameters;
/**
*
* Contains the SageMaker Model Building Pipeline parameters to start execution of a SageMaker Model Building
* Pipeline.
*
*
* If you specify a SageMaker Model Building Pipeline as a target, you can use this to specify parameters to start a
* pipeline execution based on EventBridge events.
*
*/
private SageMakerPipelineParameters sageMakerPipelineParameters;
/**
*
* The DeadLetterConfig
that defines the target queue to send dead-letter queue events to.
*
*/
private DeadLetterConfig deadLetterConfig;
/**
*
* The RetryPolicy
object that contains the retry policy configuration to use for the dead-letter
* queue.
*
*/
private RetryPolicy retryPolicy;
/**
*
* Contains the GraphQL operation to be parsed and executed, if the event target is an AppSync API.
*
*/
private AppSyncParameters appSyncParameters;
/**
*
* The ID of the target within the specified rule. Use this ID to reference the target when updating the rule. We
* recommend using a memorable and unique string.
*
*
* @param id
* The ID of the target within the specified rule. Use this ID to reference the target when updating the
* rule. We recommend using a memorable and unique string.
*/
public void setId(String id) {
this.id = id;
}
/**
*
* The ID of the target within the specified rule. Use this ID to reference the target when updating the rule. We
* recommend using a memorable and unique string.
*
*
* @return The ID of the target within the specified rule. Use this ID to reference the target when updating the
* rule. We recommend using a memorable and unique string.
*/
public String getId() {
return this.id;
}
/**
*
* The ID of the target within the specified rule. Use this ID to reference the target when updating the rule. We
* recommend using a memorable and unique string.
*
*
* @param id
* The ID of the target within the specified rule. Use this ID to reference the target when updating the
* rule. We recommend using a memorable and unique string.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Target withId(String id) {
setId(id);
return this;
}
/**
*
* The Amazon Resource Name (ARN) of the target.
*
*
* @param arn
* The Amazon Resource Name (ARN) of the target.
*/
public void setArn(String arn) {
this.arn = arn;
}
/**
*
* The Amazon Resource Name (ARN) of the target.
*
*
* @return The Amazon Resource Name (ARN) of the target.
*/
public String getArn() {
return this.arn;
}
/**
*
* The Amazon Resource Name (ARN) of the target.
*
*
* @param arn
* The Amazon Resource Name (ARN) of the target.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Target withArn(String arn) {
setArn(arn);
return this;
}
/**
*
* The Amazon Resource Name (ARN) of the IAM role to be used for this target when the rule is triggered. If one rule
* triggers multiple targets, you can use a different IAM role for each target.
*
*
* @param roleArn
* The Amazon Resource Name (ARN) of the IAM role to be used for this target when the rule is triggered. If
* one rule triggers multiple targets, you can use a different IAM role for each target.
*/
public void setRoleArn(String roleArn) {
this.roleArn = roleArn;
}
/**
*
* The Amazon Resource Name (ARN) of the IAM role to be used for this target when the rule is triggered. If one rule
* triggers multiple targets, you can use a different IAM role for each target.
*
*
* @return The Amazon Resource Name (ARN) of the IAM role to be used for this target when the rule is triggered. If
* one rule triggers multiple targets, you can use a different IAM role for each target.
*/
public String getRoleArn() {
return this.roleArn;
}
/**
*
* The Amazon Resource Name (ARN) of the IAM role to be used for this target when the rule is triggered. If one rule
* triggers multiple targets, you can use a different IAM role for each target.
*
*
* @param roleArn
* The Amazon Resource Name (ARN) of the IAM role to be used for this target when the rule is triggered. If
* one rule triggers multiple targets, you can use a different IAM role for each target.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Target withRoleArn(String roleArn) {
setRoleArn(roleArn);
return this;
}
/**
*
* Valid JSON text passed to the target. In this case, nothing from the event itself is passed to the target. For
* more information, see The JavaScript Object Notation (JSON)
* Data Interchange Format.
*
*
* @param input
* Valid JSON text passed to the target. In this case, nothing from the event itself is passed to the target.
* For more information, see The JavaScript Object
* Notation (JSON) Data Interchange Format.
*/
public void setInput(String input) {
this.input = input;
}
/**
*
* Valid JSON text passed to the target. In this case, nothing from the event itself is passed to the target. For
* more information, see The JavaScript Object Notation (JSON)
* Data Interchange Format.
*
*
* @return Valid JSON text passed to the target. In this case, nothing from the event itself is passed to the
* target. For more information, see The JavaScript
* Object Notation (JSON) Data Interchange Format.
*/
public String getInput() {
return this.input;
}
/**
*
* Valid JSON text passed to the target. In this case, nothing from the event itself is passed to the target. For
* more information, see The JavaScript Object Notation (JSON)
* Data Interchange Format.
*
*
* @param input
* Valid JSON text passed to the target. In this case, nothing from the event itself is passed to the target.
* For more information, see The JavaScript Object
* Notation (JSON) Data Interchange Format.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Target withInput(String input) {
setInput(input);
return this;
}
/**
*
* The value of the JSONPath that is used for extracting part of the matched event when passing it to the target.
* You may use JSON dot notation or bracket notation. For more information about JSON paths, see JSONPath.
*
*
* @param inputPath
* The value of the JSONPath that is used for extracting part of the matched event when passing it to the
* target. You may use JSON dot notation or bracket notation. For more information about JSON paths, see JSONPath.
*/
public void setInputPath(String inputPath) {
this.inputPath = inputPath;
}
/**
*
* The value of the JSONPath that is used for extracting part of the matched event when passing it to the target.
* You may use JSON dot notation or bracket notation. For more information about JSON paths, see JSONPath.
*
*
* @return The value of the JSONPath that is used for extracting part of the matched event when passing it to the
* target. You may use JSON dot notation or bracket notation. For more information about JSON paths, see JSONPath.
*/
public String getInputPath() {
return this.inputPath;
}
/**
*
* The value of the JSONPath that is used for extracting part of the matched event when passing it to the target.
* You may use JSON dot notation or bracket notation. For more information about JSON paths, see JSONPath.
*
*
* @param inputPath
* The value of the JSONPath that is used for extracting part of the matched event when passing it to the
* target. You may use JSON dot notation or bracket notation. For more information about JSON paths, see JSONPath.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Target withInputPath(String inputPath) {
setInputPath(inputPath);
return this;
}
/**
*
* Settings to enable you to provide custom input to a target based on certain event data. You can extract one or
* more key-value pairs from the event and then use that data to send customized input to the target.
*
*
* @param inputTransformer
* Settings to enable you to provide custom input to a target based on certain event data. You can extract
* one or more key-value pairs from the event and then use that data to send customized input to the target.
*/
public void setInputTransformer(InputTransformer inputTransformer) {
this.inputTransformer = inputTransformer;
}
/**
*
* Settings to enable you to provide custom input to a target based on certain event data. You can extract one or
* more key-value pairs from the event and then use that data to send customized input to the target.
*
*
* @return Settings to enable you to provide custom input to a target based on certain event data. You can extract
* one or more key-value pairs from the event and then use that data to send customized input to the target.
*/
public InputTransformer getInputTransformer() {
return this.inputTransformer;
}
/**
*
* Settings to enable you to provide custom input to a target based on certain event data. You can extract one or
* more key-value pairs from the event and then use that data to send customized input to the target.
*
*
* @param inputTransformer
* Settings to enable you to provide custom input to a target based on certain event data. You can extract
* one or more key-value pairs from the event and then use that data to send customized input to the target.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Target withInputTransformer(InputTransformer inputTransformer) {
setInputTransformer(inputTransformer);
return this;
}
/**
*
* The custom parameter you can use to control the shard assignment, when the target is a Kinesis data stream. If
* you do not include this parameter, the default is to use the eventId
as the partition key.
*
*
* @param kinesisParameters
* The custom parameter you can use to control the shard assignment, when the target is a Kinesis data
* stream. If you do not include this parameter, the default is to use the eventId
as the
* partition key.
*/
public void setKinesisParameters(KinesisParameters kinesisParameters) {
this.kinesisParameters = kinesisParameters;
}
/**
*
* The custom parameter you can use to control the shard assignment, when the target is a Kinesis data stream. If
* you do not include this parameter, the default is to use the eventId
as the partition key.
*
*
* @return The custom parameter you can use to control the shard assignment, when the target is a Kinesis data
* stream. If you do not include this parameter, the default is to use the eventId
as the
* partition key.
*/
public KinesisParameters getKinesisParameters() {
return this.kinesisParameters;
}
/**
*
* The custom parameter you can use to control the shard assignment, when the target is a Kinesis data stream. If
* you do not include this parameter, the default is to use the eventId
as the partition key.
*
*
* @param kinesisParameters
* The custom parameter you can use to control the shard assignment, when the target is a Kinesis data
* stream. If you do not include this parameter, the default is to use the eventId
as the
* partition key.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Target withKinesisParameters(KinesisParameters kinesisParameters) {
setKinesisParameters(kinesisParameters);
return this;
}
/**
*
* Parameters used when you are using the rule to invoke Amazon EC2 Run Command.
*
*
* @param runCommandParameters
* Parameters used when you are using the rule to invoke Amazon EC2 Run Command.
*/
public void setRunCommandParameters(RunCommandParameters runCommandParameters) {
this.runCommandParameters = runCommandParameters;
}
/**
*
* Parameters used when you are using the rule to invoke Amazon EC2 Run Command.
*
*
* @return Parameters used when you are using the rule to invoke Amazon EC2 Run Command.
*/
public RunCommandParameters getRunCommandParameters() {
return this.runCommandParameters;
}
/**
*
* Parameters used when you are using the rule to invoke Amazon EC2 Run Command.
*
*
* @param runCommandParameters
* Parameters used when you are using the rule to invoke Amazon EC2 Run Command.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Target withRunCommandParameters(RunCommandParameters runCommandParameters) {
setRunCommandParameters(runCommandParameters);
return this;
}
/**
*
* Contains the Amazon ECS task definition and task count to be used, if the event target is an Amazon ECS task. For
* more information about Amazon ECS tasks, see Task Definitions in
* the Amazon EC2 Container Service Developer Guide.
*
*
* @param ecsParameters
* Contains the Amazon ECS task definition and task count to be used, if the event target is an Amazon ECS
* task. For more information about Amazon ECS tasks, see Task Definitions
* in the Amazon EC2 Container Service Developer Guide.
*/
public void setEcsParameters(EcsParameters ecsParameters) {
this.ecsParameters = ecsParameters;
}
/**
*
* Contains the Amazon ECS task definition and task count to be used, if the event target is an Amazon ECS task. For
* more information about Amazon ECS tasks, see Task Definitions in
* the Amazon EC2 Container Service Developer Guide.
*
*
* @return Contains the Amazon ECS task definition and task count to be used, if the event target is an Amazon ECS
* task. For more information about Amazon ECS tasks, see Task Definitions
* in the Amazon EC2 Container Service Developer Guide.
*/
public EcsParameters getEcsParameters() {
return this.ecsParameters;
}
/**
*
* Contains the Amazon ECS task definition and task count to be used, if the event target is an Amazon ECS task. For
* more information about Amazon ECS tasks, see Task Definitions in
* the Amazon EC2 Container Service Developer Guide.
*
*
* @param ecsParameters
* Contains the Amazon ECS task definition and task count to be used, if the event target is an Amazon ECS
* task. For more information about Amazon ECS tasks, see Task Definitions
* in the Amazon EC2 Container Service Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Target withEcsParameters(EcsParameters ecsParameters) {
setEcsParameters(ecsParameters);
return this;
}
/**
*
* If the event target is an Batch job, this contains the job definition, job name, and other parameters. For more
* information, see Jobs in the Batch
* User Guide.
*
*
* @param batchParameters
* If the event target is an Batch job, this contains the job definition, job name, and other parameters. For
* more information, see Jobs in
* the Batch User Guide.
*/
public void setBatchParameters(BatchParameters batchParameters) {
this.batchParameters = batchParameters;
}
/**
*
* If the event target is an Batch job, this contains the job definition, job name, and other parameters. For more
* information, see Jobs in the Batch
* User Guide.
*
*
* @return If the event target is an Batch job, this contains the job definition, job name, and other parameters.
* For more information, see Jobs
* in the Batch User Guide.
*/
public BatchParameters getBatchParameters() {
return this.batchParameters;
}
/**
*
* If the event target is an Batch job, this contains the job definition, job name, and other parameters. For more
* information, see Jobs in the Batch
* User Guide.
*
*
* @param batchParameters
* If the event target is an Batch job, this contains the job definition, job name, and other parameters. For
* more information, see Jobs in
* the Batch User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Target withBatchParameters(BatchParameters batchParameters) {
setBatchParameters(batchParameters);
return this;
}
/**
*
* Contains the message group ID to use when the target is a FIFO queue.
*
*
* If you specify an SQS FIFO queue as a target, the queue must have content-based deduplication enabled.
*
*
* @param sqsParameters
* Contains the message group ID to use when the target is a FIFO queue.
*
* If you specify an SQS FIFO queue as a target, the queue must have content-based deduplication enabled.
*/
public void setSqsParameters(SqsParameters sqsParameters) {
this.sqsParameters = sqsParameters;
}
/**
*
* Contains the message group ID to use when the target is a FIFO queue.
*
*
* If you specify an SQS FIFO queue as a target, the queue must have content-based deduplication enabled.
*
*
* @return Contains the message group ID to use when the target is a FIFO queue.
*
* If you specify an SQS FIFO queue as a target, the queue must have content-based deduplication enabled.
*/
public SqsParameters getSqsParameters() {
return this.sqsParameters;
}
/**
*
* Contains the message group ID to use when the target is a FIFO queue.
*
*
* If you specify an SQS FIFO queue as a target, the queue must have content-based deduplication enabled.
*
*
* @param sqsParameters
* Contains the message group ID to use when the target is a FIFO queue.
*
* If you specify an SQS FIFO queue as a target, the queue must have content-based deduplication enabled.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Target withSqsParameters(SqsParameters sqsParameters) {
setSqsParameters(sqsParameters);
return this;
}
/**
*
* Contains the HTTP parameters to use when the target is a API Gateway endpoint or EventBridge ApiDestination.
*
*
* If you specify an API Gateway API or EventBridge ApiDestination as a target, you can use this parameter to
* specify headers, path parameters, and query string keys/values as part of your target invoking request. If you're
* using ApiDestinations, the corresponding Connection can also have these values configured. In case of any
* conflicting keys, values from the Connection take precedence.
*
*
* @param httpParameters
* Contains the HTTP parameters to use when the target is a API Gateway endpoint or EventBridge
* ApiDestination.
*
* If you specify an API Gateway API or EventBridge ApiDestination as a target, you can use this parameter to
* specify headers, path parameters, and query string keys/values as part of your target invoking request. If
* you're using ApiDestinations, the corresponding Connection can also have these values configured. In case
* of any conflicting keys, values from the Connection take precedence.
*/
public void setHttpParameters(HttpParameters httpParameters) {
this.httpParameters = httpParameters;
}
/**
*
* Contains the HTTP parameters to use when the target is a API Gateway endpoint or EventBridge ApiDestination.
*
*
* If you specify an API Gateway API or EventBridge ApiDestination as a target, you can use this parameter to
* specify headers, path parameters, and query string keys/values as part of your target invoking request. If you're
* using ApiDestinations, the corresponding Connection can also have these values configured. In case of any
* conflicting keys, values from the Connection take precedence.
*
*
* @return Contains the HTTP parameters to use when the target is a API Gateway endpoint or EventBridge
* ApiDestination.
*
* If you specify an API Gateway API or EventBridge ApiDestination as a target, you can use this parameter
* to specify headers, path parameters, and query string keys/values as part of your target invoking
* request. If you're using ApiDestinations, the corresponding Connection can also have these values
* configured. In case of any conflicting keys, values from the Connection take precedence.
*/
public HttpParameters getHttpParameters() {
return this.httpParameters;
}
/**
*
* Contains the HTTP parameters to use when the target is a API Gateway endpoint or EventBridge ApiDestination.
*
*
* If you specify an API Gateway API or EventBridge ApiDestination as a target, you can use this parameter to
* specify headers, path parameters, and query string keys/values as part of your target invoking request. If you're
* using ApiDestinations, the corresponding Connection can also have these values configured. In case of any
* conflicting keys, values from the Connection take precedence.
*
*
* @param httpParameters
* Contains the HTTP parameters to use when the target is a API Gateway endpoint or EventBridge
* ApiDestination.
*
* If you specify an API Gateway API or EventBridge ApiDestination as a target, you can use this parameter to
* specify headers, path parameters, and query string keys/values as part of your target invoking request. If
* you're using ApiDestinations, the corresponding Connection can also have these values configured. In case
* of any conflicting keys, values from the Connection take precedence.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Target withHttpParameters(HttpParameters httpParameters) {
setHttpParameters(httpParameters);
return this;
}
/**
*
* Contains the Amazon Redshift Data API parameters to use when the target is a Amazon Redshift cluster.
*
*
* If you specify a Amazon Redshift Cluster as a Target, you can use this to specify parameters to invoke the Amazon
* Redshift Data API ExecuteStatement based on EventBridge events.
*
*
* @param redshiftDataParameters
* Contains the Amazon Redshift Data API parameters to use when the target is a Amazon Redshift cluster.
*
* If you specify a Amazon Redshift Cluster as a Target, you can use this to specify parameters to invoke the
* Amazon Redshift Data API ExecuteStatement based on EventBridge events.
*/
public void setRedshiftDataParameters(RedshiftDataParameters redshiftDataParameters) {
this.redshiftDataParameters = redshiftDataParameters;
}
/**
*
* Contains the Amazon Redshift Data API parameters to use when the target is a Amazon Redshift cluster.
*
*
* If you specify a Amazon Redshift Cluster as a Target, you can use this to specify parameters to invoke the Amazon
* Redshift Data API ExecuteStatement based on EventBridge events.
*
*
* @return Contains the Amazon Redshift Data API parameters to use when the target is a Amazon Redshift cluster.
*
* If you specify a Amazon Redshift Cluster as a Target, you can use this to specify parameters to invoke
* the Amazon Redshift Data API ExecuteStatement based on EventBridge events.
*/
public RedshiftDataParameters getRedshiftDataParameters() {
return this.redshiftDataParameters;
}
/**
*
* Contains the Amazon Redshift Data API parameters to use when the target is a Amazon Redshift cluster.
*
*
* If you specify a Amazon Redshift Cluster as a Target, you can use this to specify parameters to invoke the Amazon
* Redshift Data API ExecuteStatement based on EventBridge events.
*
*
* @param redshiftDataParameters
* Contains the Amazon Redshift Data API parameters to use when the target is a Amazon Redshift cluster.
*
* If you specify a Amazon Redshift Cluster as a Target, you can use this to specify parameters to invoke the
* Amazon Redshift Data API ExecuteStatement based on EventBridge events.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Target withRedshiftDataParameters(RedshiftDataParameters redshiftDataParameters) {
setRedshiftDataParameters(redshiftDataParameters);
return this;
}
/**
*
* Contains the SageMaker Model Building Pipeline parameters to start execution of a SageMaker Model Building
* Pipeline.
*
*
* If you specify a SageMaker Model Building Pipeline as a target, you can use this to specify parameters to start a
* pipeline execution based on EventBridge events.
*
*
* @param sageMakerPipelineParameters
* Contains the SageMaker Model Building Pipeline parameters to start execution of a SageMaker Model Building
* Pipeline.
*
* If you specify a SageMaker Model Building Pipeline as a target, you can use this to specify parameters to
* start a pipeline execution based on EventBridge events.
*/
public void setSageMakerPipelineParameters(SageMakerPipelineParameters sageMakerPipelineParameters) {
this.sageMakerPipelineParameters = sageMakerPipelineParameters;
}
/**
*
* Contains the SageMaker Model Building Pipeline parameters to start execution of a SageMaker Model Building
* Pipeline.
*
*
* If you specify a SageMaker Model Building Pipeline as a target, you can use this to specify parameters to start a
* pipeline execution based on EventBridge events.
*
*
* @return Contains the SageMaker Model Building Pipeline parameters to start execution of a SageMaker Model
* Building Pipeline.
*
* If you specify a SageMaker Model Building Pipeline as a target, you can use this to specify parameters to
* start a pipeline execution based on EventBridge events.
*/
public SageMakerPipelineParameters getSageMakerPipelineParameters() {
return this.sageMakerPipelineParameters;
}
/**
*
* Contains the SageMaker Model Building Pipeline parameters to start execution of a SageMaker Model Building
* Pipeline.
*
*
* If you specify a SageMaker Model Building Pipeline as a target, you can use this to specify parameters to start a
* pipeline execution based on EventBridge events.
*
*
* @param sageMakerPipelineParameters
* Contains the SageMaker Model Building Pipeline parameters to start execution of a SageMaker Model Building
* Pipeline.
*
* If you specify a SageMaker Model Building Pipeline as a target, you can use this to specify parameters to
* start a pipeline execution based on EventBridge events.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Target withSageMakerPipelineParameters(SageMakerPipelineParameters sageMakerPipelineParameters) {
setSageMakerPipelineParameters(sageMakerPipelineParameters);
return this;
}
/**
*
* The DeadLetterConfig
that defines the target queue to send dead-letter queue events to.
*
*
* @param deadLetterConfig
* The DeadLetterConfig
that defines the target queue to send dead-letter queue events to.
*/
public void setDeadLetterConfig(DeadLetterConfig deadLetterConfig) {
this.deadLetterConfig = deadLetterConfig;
}
/**
*
* The DeadLetterConfig
that defines the target queue to send dead-letter queue events to.
*
*
* @return The DeadLetterConfig
that defines the target queue to send dead-letter queue events to.
*/
public DeadLetterConfig getDeadLetterConfig() {
return this.deadLetterConfig;
}
/**
*
* The DeadLetterConfig
that defines the target queue to send dead-letter queue events to.
*
*
* @param deadLetterConfig
* The DeadLetterConfig
that defines the target queue to send dead-letter queue events to.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Target withDeadLetterConfig(DeadLetterConfig deadLetterConfig) {
setDeadLetterConfig(deadLetterConfig);
return this;
}
/**
*
* The RetryPolicy
object that contains the retry policy configuration to use for the dead-letter
* queue.
*
*
* @param retryPolicy
* The RetryPolicy
object that contains the retry policy configuration to use for the
* dead-letter queue.
*/
public void setRetryPolicy(RetryPolicy retryPolicy) {
this.retryPolicy = retryPolicy;
}
/**
*
* The RetryPolicy
object that contains the retry policy configuration to use for the dead-letter
* queue.
*
*
* @return The RetryPolicy
object that contains the retry policy configuration to use for the
* dead-letter queue.
*/
public RetryPolicy getRetryPolicy() {
return this.retryPolicy;
}
/**
*
* The RetryPolicy
object that contains the retry policy configuration to use for the dead-letter
* queue.
*
*
* @param retryPolicy
* The RetryPolicy
object that contains the retry policy configuration to use for the
* dead-letter queue.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Target withRetryPolicy(RetryPolicy retryPolicy) {
setRetryPolicy(retryPolicy);
return this;
}
/**
*
* Contains the GraphQL operation to be parsed and executed, if the event target is an AppSync API.
*
*
* @param appSyncParameters
* Contains the GraphQL operation to be parsed and executed, if the event target is an AppSync API.
*/
public void setAppSyncParameters(AppSyncParameters appSyncParameters) {
this.appSyncParameters = appSyncParameters;
}
/**
*
* Contains the GraphQL operation to be parsed and executed, if the event target is an AppSync API.
*
*
* @return Contains the GraphQL operation to be parsed and executed, if the event target is an AppSync API.
*/
public AppSyncParameters getAppSyncParameters() {
return this.appSyncParameters;
}
/**
*
* Contains the GraphQL operation to be parsed and executed, if the event target is an AppSync API.
*
*
* @param appSyncParameters
* Contains the GraphQL operation to be parsed and executed, if the event target is an AppSync API.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Target withAppSyncParameters(AppSyncParameters appSyncParameters) {
setAppSyncParameters(appSyncParameters);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getId() != null)
sb.append("Id: ").append(getId()).append(",");
if (getArn() != null)
sb.append("Arn: ").append(getArn()).append(",");
if (getRoleArn() != null)
sb.append("RoleArn: ").append(getRoleArn()).append(",");
if (getInput() != null)
sb.append("Input: ").append(getInput()).append(",");
if (getInputPath() != null)
sb.append("InputPath: ").append(getInputPath()).append(",");
if (getInputTransformer() != null)
sb.append("InputTransformer: ").append(getInputTransformer()).append(",");
if (getKinesisParameters() != null)
sb.append("KinesisParameters: ").append(getKinesisParameters()).append(",");
if (getRunCommandParameters() != null)
sb.append("RunCommandParameters: ").append(getRunCommandParameters()).append(",");
if (getEcsParameters() != null)
sb.append("EcsParameters: ").append(getEcsParameters()).append(",");
if (getBatchParameters() != null)
sb.append("BatchParameters: ").append(getBatchParameters()).append(",");
if (getSqsParameters() != null)
sb.append("SqsParameters: ").append(getSqsParameters()).append(",");
if (getHttpParameters() != null)
sb.append("HttpParameters: ").append(getHttpParameters()).append(",");
if (getRedshiftDataParameters() != null)
sb.append("RedshiftDataParameters: ").append(getRedshiftDataParameters()).append(",");
if (getSageMakerPipelineParameters() != null)
sb.append("SageMakerPipelineParameters: ").append(getSageMakerPipelineParameters()).append(",");
if (getDeadLetterConfig() != null)
sb.append("DeadLetterConfig: ").append(getDeadLetterConfig()).append(",");
if (getRetryPolicy() != null)
sb.append("RetryPolicy: ").append(getRetryPolicy()).append(",");
if (getAppSyncParameters() != null)
sb.append("AppSyncParameters: ").append(getAppSyncParameters());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof Target == false)
return false;
Target other = (Target) obj;
if (other.getId() == null ^ this.getId() == null)
return false;
if (other.getId() != null && other.getId().equals(this.getId()) == false)
return false;
if (other.getArn() == null ^ this.getArn() == null)
return false;
if (other.getArn() != null && other.getArn().equals(this.getArn()) == false)
return false;
if (other.getRoleArn() == null ^ this.getRoleArn() == null)
return false;
if (other.getRoleArn() != null && other.getRoleArn().equals(this.getRoleArn()) == false)
return false;
if (other.getInput() == null ^ this.getInput() == null)
return false;
if (other.getInput() != null && other.getInput().equals(this.getInput()) == false)
return false;
if (other.getInputPath() == null ^ this.getInputPath() == null)
return false;
if (other.getInputPath() != null && other.getInputPath().equals(this.getInputPath()) == false)
return false;
if (other.getInputTransformer() == null ^ this.getInputTransformer() == null)
return false;
if (other.getInputTransformer() != null && other.getInputTransformer().equals(this.getInputTransformer()) == false)
return false;
if (other.getKinesisParameters() == null ^ this.getKinesisParameters() == null)
return false;
if (other.getKinesisParameters() != null && other.getKinesisParameters().equals(this.getKinesisParameters()) == false)
return false;
if (other.getRunCommandParameters() == null ^ this.getRunCommandParameters() == null)
return false;
if (other.getRunCommandParameters() != null && other.getRunCommandParameters().equals(this.getRunCommandParameters()) == false)
return false;
if (other.getEcsParameters() == null ^ this.getEcsParameters() == null)
return false;
if (other.getEcsParameters() != null && other.getEcsParameters().equals(this.getEcsParameters()) == false)
return false;
if (other.getBatchParameters() == null ^ this.getBatchParameters() == null)
return false;
if (other.getBatchParameters() != null && other.getBatchParameters().equals(this.getBatchParameters()) == false)
return false;
if (other.getSqsParameters() == null ^ this.getSqsParameters() == null)
return false;
if (other.getSqsParameters() != null && other.getSqsParameters().equals(this.getSqsParameters()) == false)
return false;
if (other.getHttpParameters() == null ^ this.getHttpParameters() == null)
return false;
if (other.getHttpParameters() != null && other.getHttpParameters().equals(this.getHttpParameters()) == false)
return false;
if (other.getRedshiftDataParameters() == null ^ this.getRedshiftDataParameters() == null)
return false;
if (other.getRedshiftDataParameters() != null && other.getRedshiftDataParameters().equals(this.getRedshiftDataParameters()) == false)
return false;
if (other.getSageMakerPipelineParameters() == null ^ this.getSageMakerPipelineParameters() == null)
return false;
if (other.getSageMakerPipelineParameters() != null && other.getSageMakerPipelineParameters().equals(this.getSageMakerPipelineParameters()) == false)
return false;
if (other.getDeadLetterConfig() == null ^ this.getDeadLetterConfig() == null)
return false;
if (other.getDeadLetterConfig() != null && other.getDeadLetterConfig().equals(this.getDeadLetterConfig()) == false)
return false;
if (other.getRetryPolicy() == null ^ this.getRetryPolicy() == null)
return false;
if (other.getRetryPolicy() != null && other.getRetryPolicy().equals(this.getRetryPolicy()) == false)
return false;
if (other.getAppSyncParameters() == null ^ this.getAppSyncParameters() == null)
return false;
if (other.getAppSyncParameters() != null && other.getAppSyncParameters().equals(this.getAppSyncParameters()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getId() == null) ? 0 : getId().hashCode());
hashCode = prime * hashCode + ((getArn() == null) ? 0 : getArn().hashCode());
hashCode = prime * hashCode + ((getRoleArn() == null) ? 0 : getRoleArn().hashCode());
hashCode = prime * hashCode + ((getInput() == null) ? 0 : getInput().hashCode());
hashCode = prime * hashCode + ((getInputPath() == null) ? 0 : getInputPath().hashCode());
hashCode = prime * hashCode + ((getInputTransformer() == null) ? 0 : getInputTransformer().hashCode());
hashCode = prime * hashCode + ((getKinesisParameters() == null) ? 0 : getKinesisParameters().hashCode());
hashCode = prime * hashCode + ((getRunCommandParameters() == null) ? 0 : getRunCommandParameters().hashCode());
hashCode = prime * hashCode + ((getEcsParameters() == null) ? 0 : getEcsParameters().hashCode());
hashCode = prime * hashCode + ((getBatchParameters() == null) ? 0 : getBatchParameters().hashCode());
hashCode = prime * hashCode + ((getSqsParameters() == null) ? 0 : getSqsParameters().hashCode());
hashCode = prime * hashCode + ((getHttpParameters() == null) ? 0 : getHttpParameters().hashCode());
hashCode = prime * hashCode + ((getRedshiftDataParameters() == null) ? 0 : getRedshiftDataParameters().hashCode());
hashCode = prime * hashCode + ((getSageMakerPipelineParameters() == null) ? 0 : getSageMakerPipelineParameters().hashCode());
hashCode = prime * hashCode + ((getDeadLetterConfig() == null) ? 0 : getDeadLetterConfig().hashCode());
hashCode = prime * hashCode + ((getRetryPolicy() == null) ? 0 : getRetryPolicy().hashCode());
hashCode = prime * hashCode + ((getAppSyncParameters() == null) ? 0 : getAppSyncParameters().hashCode());
return hashCode;
}
@Override
public Target clone() {
try {
return (Target) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.eventbridge.model.transform.TargetMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}