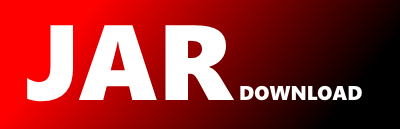
com.amazonaws.services.eventbridge.model.InputTransformer Maven / Gradle / Ivy
Show all versions of aws-java-sdk-eventbridge Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.eventbridge.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Contains the parameters needed for you to provide custom input to a target based on one or more pieces of data
* extracted from the event.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class InputTransformer implements Serializable, Cloneable, StructuredPojo {
/**
*
* Map of JSON paths to be extracted from the event. You can then insert these in the template in
* InputTemplate
to produce the output you want to be sent to the target.
*
*
* InputPathsMap
is an array key-value pairs, where each value is a valid JSON path. You can have as
* many as 100 key-value pairs. You must use JSON dot notation, not bracket notation.
*
*
* The keys cannot start with "Amazon Web Services."
*
*/
private java.util.Map inputPathsMap;
/**
*
* Input template where you specify placeholders that will be filled with the values of the keys from
* InputPathsMap
to customize the data sent to the target. Enclose each InputPathsMaps
* value in brackets: <value>
*
*
* If InputTemplate
is a JSON object (surrounded by curly braces), the following restrictions apply:
*
*
* -
*
* The placeholder cannot be used as an object key.
*
*
*
*
* The following example shows the syntax for using InputPathsMap
and InputTemplate
.
*
*
* "InputTransformer":
*
*
* {
*
*
* "InputPathsMap": {"instance": "$.detail.instance","status": "$.detail.status"},
*
*
* "InputTemplate": "<instance> is in state <status>"
*
*
* }
*
*
* To have the InputTemplate
include quote marks within a JSON string, escape each quote marks with a
* slash, as in the following example:
*
*
* "InputTransformer":
*
*
* {
*
*
* "InputPathsMap": {"instance": "$.detail.instance","status": "$.detail.status"},
*
*
* "InputTemplate": "<instance> is in state \"<status>\""
*
*
* }
*
*
* The InputTemplate
can also be valid JSON with varibles in quotes or out, as in the following
* example:
*
*
* "InputTransformer":
*
*
* {
*
*
* "InputPathsMap": {"instance": "$.detail.instance","status": "$.detail.status"},
*
*
* "InputTemplate": '{"myInstance": <instance>,"myStatus": "<instance> is in state \"<status>\""}'
*
*
* }
*
*/
private String inputTemplate;
/**
*
* Map of JSON paths to be extracted from the event. You can then insert these in the template in
* InputTemplate
to produce the output you want to be sent to the target.
*
*
* InputPathsMap
is an array key-value pairs, where each value is a valid JSON path. You can have as
* many as 100 key-value pairs. You must use JSON dot notation, not bracket notation.
*
*
* The keys cannot start with "Amazon Web Services."
*
*
* @return Map of JSON paths to be extracted from the event. You can then insert these in the template in
* InputTemplate
to produce the output you want to be sent to the target.
*
* InputPathsMap
is an array key-value pairs, where each value is a valid JSON path. You can
* have as many as 100 key-value pairs. You must use JSON dot notation, not bracket notation.
*
*
* The keys cannot start with "Amazon Web Services."
*/
public java.util.Map getInputPathsMap() {
return inputPathsMap;
}
/**
*
* Map of JSON paths to be extracted from the event. You can then insert these in the template in
* InputTemplate
to produce the output you want to be sent to the target.
*
*
* InputPathsMap
is an array key-value pairs, where each value is a valid JSON path. You can have as
* many as 100 key-value pairs. You must use JSON dot notation, not bracket notation.
*
*
* The keys cannot start with "Amazon Web Services."
*
*
* @param inputPathsMap
* Map of JSON paths to be extracted from the event. You can then insert these in the template in
* InputTemplate
to produce the output you want to be sent to the target.
*
* InputPathsMap
is an array key-value pairs, where each value is a valid JSON path. You can
* have as many as 100 key-value pairs. You must use JSON dot notation, not bracket notation.
*
*
* The keys cannot start with "Amazon Web Services."
*/
public void setInputPathsMap(java.util.Map inputPathsMap) {
this.inputPathsMap = inputPathsMap;
}
/**
*
* Map of JSON paths to be extracted from the event. You can then insert these in the template in
* InputTemplate
to produce the output you want to be sent to the target.
*
*
* InputPathsMap
is an array key-value pairs, where each value is a valid JSON path. You can have as
* many as 100 key-value pairs. You must use JSON dot notation, not bracket notation.
*
*
* The keys cannot start with "Amazon Web Services."
*
*
* @param inputPathsMap
* Map of JSON paths to be extracted from the event. You can then insert these in the template in
* InputTemplate
to produce the output you want to be sent to the target.
*
* InputPathsMap
is an array key-value pairs, where each value is a valid JSON path. You can
* have as many as 100 key-value pairs. You must use JSON dot notation, not bracket notation.
*
*
* The keys cannot start with "Amazon Web Services."
* @return Returns a reference to this object so that method calls can be chained together.
*/
public InputTransformer withInputPathsMap(java.util.Map inputPathsMap) {
setInputPathsMap(inputPathsMap);
return this;
}
/**
* Add a single InputPathsMap entry
*
* @see InputTransformer#withInputPathsMap
* @returns a reference to this object so that method calls can be chained together.
*/
public InputTransformer addInputPathsMapEntry(String key, String value) {
if (null == this.inputPathsMap) {
this.inputPathsMap = new java.util.HashMap();
}
if (this.inputPathsMap.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.inputPathsMap.put(key, value);
return this;
}
/**
* Removes all the entries added into InputPathsMap.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public InputTransformer clearInputPathsMapEntries() {
this.inputPathsMap = null;
return this;
}
/**
*
* Input template where you specify placeholders that will be filled with the values of the keys from
* InputPathsMap
to customize the data sent to the target. Enclose each InputPathsMaps
* value in brackets: <value>
*
*
* If InputTemplate
is a JSON object (surrounded by curly braces), the following restrictions apply:
*
*
* -
*
* The placeholder cannot be used as an object key.
*
*
*
*
* The following example shows the syntax for using InputPathsMap
and InputTemplate
.
*
*
* "InputTransformer":
*
*
* {
*
*
* "InputPathsMap": {"instance": "$.detail.instance","status": "$.detail.status"},
*
*
* "InputTemplate": "<instance> is in state <status>"
*
*
* }
*
*
* To have the InputTemplate
include quote marks within a JSON string, escape each quote marks with a
* slash, as in the following example:
*
*
* "InputTransformer":
*
*
* {
*
*
* "InputPathsMap": {"instance": "$.detail.instance","status": "$.detail.status"},
*
*
* "InputTemplate": "<instance> is in state \"<status>\""
*
*
* }
*
*
* The InputTemplate
can also be valid JSON with varibles in quotes or out, as in the following
* example:
*
*
* "InputTransformer":
*
*
* {
*
*
* "InputPathsMap": {"instance": "$.detail.instance","status": "$.detail.status"},
*
*
* "InputTemplate": '{"myInstance": <instance>,"myStatus": "<instance> is in state \"<status>\""}'
*
*
* }
*
*
* @param inputTemplate
* Input template where you specify placeholders that will be filled with the values of the keys from
* InputPathsMap
to customize the data sent to the target. Enclose each
* InputPathsMaps
value in brackets: <value>
*
* If InputTemplate
is a JSON object (surrounded by curly braces), the following restrictions
* apply:
*
*
* -
*
* The placeholder cannot be used as an object key.
*
*
*
*
* The following example shows the syntax for using InputPathsMap
and InputTemplate
* .
*
*
* "InputTransformer":
*
*
* {
*
*
* "InputPathsMap": {"instance": "$.detail.instance","status": "$.detail.status"},
*
*
* "InputTemplate": "<instance> is in state <status>"
*
*
* }
*
*
* To have the InputTemplate
include quote marks within a JSON string, escape each quote marks
* with a slash, as in the following example:
*
*
* "InputTransformer":
*
*
* {
*
*
* "InputPathsMap": {"instance": "$.detail.instance","status": "$.detail.status"},
*
*
* "InputTemplate": "<instance> is in state \"<status>\""
*
*
* }
*
*
* The InputTemplate
can also be valid JSON with varibles in quotes or out, as in the following
* example:
*
*
* "InputTransformer":
*
*
* {
*
*
* "InputPathsMap": {"instance": "$.detail.instance","status": "$.detail.status"},
*
*
* "InputTemplate": '{"myInstance": <instance>,"myStatus": "<instance> is in state \"<status>\""}'
*
*
* }
*/
public void setInputTemplate(String inputTemplate) {
this.inputTemplate = inputTemplate;
}
/**
*
* Input template where you specify placeholders that will be filled with the values of the keys from
* InputPathsMap
to customize the data sent to the target. Enclose each InputPathsMaps
* value in brackets: <value>
*
*
* If InputTemplate
is a JSON object (surrounded by curly braces), the following restrictions apply:
*
*
* -
*
* The placeholder cannot be used as an object key.
*
*
*
*
* The following example shows the syntax for using InputPathsMap
and InputTemplate
.
*
*
* "InputTransformer":
*
*
* {
*
*
* "InputPathsMap": {"instance": "$.detail.instance","status": "$.detail.status"},
*
*
* "InputTemplate": "<instance> is in state <status>"
*
*
* }
*
*
* To have the InputTemplate
include quote marks within a JSON string, escape each quote marks with a
* slash, as in the following example:
*
*
* "InputTransformer":
*
*
* {
*
*
* "InputPathsMap": {"instance": "$.detail.instance","status": "$.detail.status"},
*
*
* "InputTemplate": "<instance> is in state \"<status>\""
*
*
* }
*
*
* The InputTemplate
can also be valid JSON with varibles in quotes or out, as in the following
* example:
*
*
* "InputTransformer":
*
*
* {
*
*
* "InputPathsMap": {"instance": "$.detail.instance","status": "$.detail.status"},
*
*
* "InputTemplate": '{"myInstance": <instance>,"myStatus": "<instance> is in state \"<status>\""}'
*
*
* }
*
*
* @return Input template where you specify placeholders that will be filled with the values of the keys from
* InputPathsMap
to customize the data sent to the target. Enclose each
* InputPathsMaps
value in brackets: <value>
*
* If InputTemplate
is a JSON object (surrounded by curly braces), the following restrictions
* apply:
*
*
* -
*
* The placeholder cannot be used as an object key.
*
*
*
*
* The following example shows the syntax for using InputPathsMap
and
* InputTemplate
.
*
*
* "InputTransformer":
*
*
* {
*
*
* "InputPathsMap": {"instance": "$.detail.instance","status": "$.detail.status"},
*
*
* "InputTemplate": "<instance> is in state <status>"
*
*
* }
*
*
* To have the InputTemplate
include quote marks within a JSON string, escape each quote marks
* with a slash, as in the following example:
*
*
* "InputTransformer":
*
*
* {
*
*
* "InputPathsMap": {"instance": "$.detail.instance","status": "$.detail.status"},
*
*
* "InputTemplate": "<instance> is in state \"<status>\""
*
*
* }
*
*
* The InputTemplate
can also be valid JSON with varibles in quotes or out, as in the following
* example:
*
*
* "InputTransformer":
*
*
* {
*
*
* "InputPathsMap": {"instance": "$.detail.instance","status": "$.detail.status"},
*
*
* "InputTemplate": '{"myInstance": <instance>,"myStatus": "<instance> is in state \"<status>\""}'
*
*
* }
*/
public String getInputTemplate() {
return this.inputTemplate;
}
/**
*
* Input template where you specify placeholders that will be filled with the values of the keys from
* InputPathsMap
to customize the data sent to the target. Enclose each InputPathsMaps
* value in brackets: <value>
*
*
* If InputTemplate
is a JSON object (surrounded by curly braces), the following restrictions apply:
*
*
* -
*
* The placeholder cannot be used as an object key.
*
*
*
*
* The following example shows the syntax for using InputPathsMap
and InputTemplate
.
*
*
* "InputTransformer":
*
*
* {
*
*
* "InputPathsMap": {"instance": "$.detail.instance","status": "$.detail.status"},
*
*
* "InputTemplate": "<instance> is in state <status>"
*
*
* }
*
*
* To have the InputTemplate
include quote marks within a JSON string, escape each quote marks with a
* slash, as in the following example:
*
*
* "InputTransformer":
*
*
* {
*
*
* "InputPathsMap": {"instance": "$.detail.instance","status": "$.detail.status"},
*
*
* "InputTemplate": "<instance> is in state \"<status>\""
*
*
* }
*
*
* The InputTemplate
can also be valid JSON with varibles in quotes or out, as in the following
* example:
*
*
* "InputTransformer":
*
*
* {
*
*
* "InputPathsMap": {"instance": "$.detail.instance","status": "$.detail.status"},
*
*
* "InputTemplate": '{"myInstance": <instance>,"myStatus": "<instance> is in state \"<status>\""}'
*
*
* }
*
*
* @param inputTemplate
* Input template where you specify placeholders that will be filled with the values of the keys from
* InputPathsMap
to customize the data sent to the target. Enclose each
* InputPathsMaps
value in brackets: <value>
*
* If InputTemplate
is a JSON object (surrounded by curly braces), the following restrictions
* apply:
*
*
* -
*
* The placeholder cannot be used as an object key.
*
*
*
*
* The following example shows the syntax for using InputPathsMap
and InputTemplate
* .
*
*
* "InputTransformer":
*
*
* {
*
*
* "InputPathsMap": {"instance": "$.detail.instance","status": "$.detail.status"},
*
*
* "InputTemplate": "<instance> is in state <status>"
*
*
* }
*
*
* To have the InputTemplate
include quote marks within a JSON string, escape each quote marks
* with a slash, as in the following example:
*
*
* "InputTransformer":
*
*
* {
*
*
* "InputPathsMap": {"instance": "$.detail.instance","status": "$.detail.status"},
*
*
* "InputTemplate": "<instance> is in state \"<status>\""
*
*
* }
*
*
* The InputTemplate
can also be valid JSON with varibles in quotes or out, as in the following
* example:
*
*
* "InputTransformer":
*
*
* {
*
*
* "InputPathsMap": {"instance": "$.detail.instance","status": "$.detail.status"},
*
*
* "InputTemplate": '{"myInstance": <instance>,"myStatus": "<instance> is in state \"<status>\""}'
*
*
* }
* @return Returns a reference to this object so that method calls can be chained together.
*/
public InputTransformer withInputTemplate(String inputTemplate) {
setInputTemplate(inputTemplate);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getInputPathsMap() != null)
sb.append("InputPathsMap: ").append(getInputPathsMap()).append(",");
if (getInputTemplate() != null)
sb.append("InputTemplate: ").append(getInputTemplate());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof InputTransformer == false)
return false;
InputTransformer other = (InputTransformer) obj;
if (other.getInputPathsMap() == null ^ this.getInputPathsMap() == null)
return false;
if (other.getInputPathsMap() != null && other.getInputPathsMap().equals(this.getInputPathsMap()) == false)
return false;
if (other.getInputTemplate() == null ^ this.getInputTemplate() == null)
return false;
if (other.getInputTemplate() != null && other.getInputTemplate().equals(this.getInputTemplate()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getInputPathsMap() == null) ? 0 : getInputPathsMap().hashCode());
hashCode = prime * hashCode + ((getInputTemplate() == null) ? 0 : getInputTemplate().hashCode());
return hashCode;
}
@Override
public InputTransformer clone() {
try {
return (InputTransformer) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.eventbridge.model.transform.InputTransformerMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}