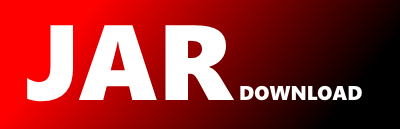
com.amazonaws.services.eventbridge.model.EcsParameters Maven / Gradle / Ivy
Show all versions of aws-java-sdk-eventbridge Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.eventbridge.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* The custom parameters to be used when the target is an Amazon ECS task.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class EcsParameters implements Serializable, Cloneable, StructuredPojo {
/**
*
* The ARN of the task definition to use if the event target is an Amazon ECS task.
*
*/
private String taskDefinitionArn;
/**
*
* The number of tasks to create based on TaskDefinition
. The default is 1.
*
*/
private Integer taskCount;
/**
*
* Specifies the launch type on which your task is running. The launch type that you specify here must match one of
* the launch type (compatibilities) of the target task. The FARGATE
value is supported only in the
* Regions where Fargate with Amazon ECS is supported. For more information, see Fargate on Amazon ECS in
* the Amazon Elastic Container Service Developer Guide.
*
*/
private String launchType;
/**
*
* Use this structure if the Amazon ECS task uses the awsvpc
network mode. This structure specifies the
* VPC subnets and security groups associated with the task, and whether a public IP address is to be used. This
* structure is required if LaunchType
is FARGATE
because the awsvpc
mode is
* required for Fargate tasks.
*
*
* If you specify NetworkConfiguration
when the target ECS task does not use the awsvpc
* network mode, the task fails.
*
*/
private NetworkConfiguration networkConfiguration;
/**
*
* Specifies the platform version for the task. Specify only the numeric portion of the platform version, such as
* 1.1.0
.
*
*
* This structure is used only if LaunchType
is FARGATE
. For more information about valid
* platform versions, see Fargate Platform
* Versions in the Amazon Elastic Container Service Developer Guide.
*
*/
private String platformVersion;
/**
*
* Specifies an ECS task group for the task. The maximum length is 255 characters.
*
*/
private String group;
/**
*
* The capacity provider strategy to use for the task.
*
*
* If a capacityProviderStrategy
is specified, the launchType
parameter must be omitted.
* If no capacityProviderStrategy
or launchType is specified, the
* defaultCapacityProviderStrategy
for the cluster is used.
*
*/
private java.util.List capacityProviderStrategy;
/**
*
* Specifies whether to enable Amazon ECS managed tags for the task. For more information, see Tagging Your Amazon ECS
* Resources in the Amazon Elastic Container Service Developer Guide.
*
*/
private Boolean enableECSManagedTags;
/**
*
* Whether or not to enable the execute command functionality for the containers in this task. If true, this enables
* execute command functionality on all containers in the task.
*
*/
private Boolean enableExecuteCommand;
/**
*
* An array of placement constraint objects to use for the task. You can specify up to 10 constraints per task
* (including constraints in the task definition and those specified at runtime).
*
*/
private java.util.List placementConstraints;
/**
*
* The placement strategy objects to use for the task. You can specify a maximum of five strategy rules per task.
*
*/
private java.util.List placementStrategy;
/**
*
* Specifies whether to propagate the tags from the task definition to the task. If no value is specified, the tags
* are not propagated. Tags can only be propagated to the task during task creation. To add tags to a task after
* task creation, use the TagResource API action.
*
*/
private String propagateTags;
/**
*
* The reference ID to use for the task.
*
*/
private String referenceId;
/**
*
* The metadata that you apply to the task to help you categorize and organize them. Each tag consists of a key and
* an optional value, both of which you define. To learn more, see RunTask in the Amazon ECS API Reference.
*
*/
private java.util.List tags;
/**
*
* The ARN of the task definition to use if the event target is an Amazon ECS task.
*
*
* @param taskDefinitionArn
* The ARN of the task definition to use if the event target is an Amazon ECS task.
*/
public void setTaskDefinitionArn(String taskDefinitionArn) {
this.taskDefinitionArn = taskDefinitionArn;
}
/**
*
* The ARN of the task definition to use if the event target is an Amazon ECS task.
*
*
* @return The ARN of the task definition to use if the event target is an Amazon ECS task.
*/
public String getTaskDefinitionArn() {
return this.taskDefinitionArn;
}
/**
*
* The ARN of the task definition to use if the event target is an Amazon ECS task.
*
*
* @param taskDefinitionArn
* The ARN of the task definition to use if the event target is an Amazon ECS task.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public EcsParameters withTaskDefinitionArn(String taskDefinitionArn) {
setTaskDefinitionArn(taskDefinitionArn);
return this;
}
/**
*
* The number of tasks to create based on TaskDefinition
. The default is 1.
*
*
* @param taskCount
* The number of tasks to create based on TaskDefinition
. The default is 1.
*/
public void setTaskCount(Integer taskCount) {
this.taskCount = taskCount;
}
/**
*
* The number of tasks to create based on TaskDefinition
. The default is 1.
*
*
* @return The number of tasks to create based on TaskDefinition
. The default is 1.
*/
public Integer getTaskCount() {
return this.taskCount;
}
/**
*
* The number of tasks to create based on TaskDefinition
. The default is 1.
*
*
* @param taskCount
* The number of tasks to create based on TaskDefinition
. The default is 1.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public EcsParameters withTaskCount(Integer taskCount) {
setTaskCount(taskCount);
return this;
}
/**
*
* Specifies the launch type on which your task is running. The launch type that you specify here must match one of
* the launch type (compatibilities) of the target task. The FARGATE
value is supported only in the
* Regions where Fargate with Amazon ECS is supported. For more information, see Fargate on Amazon ECS in
* the Amazon Elastic Container Service Developer Guide.
*
*
* @param launchType
* Specifies the launch type on which your task is running. The launch type that you specify here must match
* one of the launch type (compatibilities) of the target task. The FARGATE
value is supported
* only in the Regions where Fargate with Amazon ECS is supported. For more information, see Fargate on Amazon
* ECS in the Amazon Elastic Container Service Developer Guide.
* @see LaunchType
*/
public void setLaunchType(String launchType) {
this.launchType = launchType;
}
/**
*
* Specifies the launch type on which your task is running. The launch type that you specify here must match one of
* the launch type (compatibilities) of the target task. The FARGATE
value is supported only in the
* Regions where Fargate with Amazon ECS is supported. For more information, see Fargate on Amazon ECS in
* the Amazon Elastic Container Service Developer Guide.
*
*
* @return Specifies the launch type on which your task is running. The launch type that you specify here must match
* one of the launch type (compatibilities) of the target task. The FARGATE
value is supported
* only in the Regions where Fargate with Amazon ECS is supported. For more information, see Fargate on Amazon
* ECS in the Amazon Elastic Container Service Developer Guide.
* @see LaunchType
*/
public String getLaunchType() {
return this.launchType;
}
/**
*
* Specifies the launch type on which your task is running. The launch type that you specify here must match one of
* the launch type (compatibilities) of the target task. The FARGATE
value is supported only in the
* Regions where Fargate with Amazon ECS is supported. For more information, see Fargate on Amazon ECS in
* the Amazon Elastic Container Service Developer Guide.
*
*
* @param launchType
* Specifies the launch type on which your task is running. The launch type that you specify here must match
* one of the launch type (compatibilities) of the target task. The FARGATE
value is supported
* only in the Regions where Fargate with Amazon ECS is supported. For more information, see Fargate on Amazon
* ECS in the Amazon Elastic Container Service Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
* @see LaunchType
*/
public EcsParameters withLaunchType(String launchType) {
setLaunchType(launchType);
return this;
}
/**
*
* Specifies the launch type on which your task is running. The launch type that you specify here must match one of
* the launch type (compatibilities) of the target task. The FARGATE
value is supported only in the
* Regions where Fargate with Amazon ECS is supported. For more information, see Fargate on Amazon ECS in
* the Amazon Elastic Container Service Developer Guide.
*
*
* @param launchType
* Specifies the launch type on which your task is running. The launch type that you specify here must match
* one of the launch type (compatibilities) of the target task. The FARGATE
value is supported
* only in the Regions where Fargate with Amazon ECS is supported. For more information, see Fargate on Amazon
* ECS in the Amazon Elastic Container Service Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
* @see LaunchType
*/
public EcsParameters withLaunchType(LaunchType launchType) {
this.launchType = launchType.toString();
return this;
}
/**
*
* Use this structure if the Amazon ECS task uses the awsvpc
network mode. This structure specifies the
* VPC subnets and security groups associated with the task, and whether a public IP address is to be used. This
* structure is required if LaunchType
is FARGATE
because the awsvpc
mode is
* required for Fargate tasks.
*
*
* If you specify NetworkConfiguration
when the target ECS task does not use the awsvpc
* network mode, the task fails.
*
*
* @param networkConfiguration
* Use this structure if the Amazon ECS task uses the awsvpc
network mode. This structure
* specifies the VPC subnets and security groups associated with the task, and whether a public IP address is
* to be used. This structure is required if LaunchType
is FARGATE
because the
* awsvpc
mode is required for Fargate tasks.
*
* If you specify NetworkConfiguration
when the target ECS task does not use the
* awsvpc
network mode, the task fails.
*/
public void setNetworkConfiguration(NetworkConfiguration networkConfiguration) {
this.networkConfiguration = networkConfiguration;
}
/**
*
* Use this structure if the Amazon ECS task uses the awsvpc
network mode. This structure specifies the
* VPC subnets and security groups associated with the task, and whether a public IP address is to be used. This
* structure is required if LaunchType
is FARGATE
because the awsvpc
mode is
* required for Fargate tasks.
*
*
* If you specify NetworkConfiguration
when the target ECS task does not use the awsvpc
* network mode, the task fails.
*
*
* @return Use this structure if the Amazon ECS task uses the awsvpc
network mode. This structure
* specifies the VPC subnets and security groups associated with the task, and whether a public IP address
* is to be used. This structure is required if LaunchType
is FARGATE
because the
* awsvpc
mode is required for Fargate tasks.
*
* If you specify NetworkConfiguration
when the target ECS task does not use the
* awsvpc
network mode, the task fails.
*/
public NetworkConfiguration getNetworkConfiguration() {
return this.networkConfiguration;
}
/**
*
* Use this structure if the Amazon ECS task uses the awsvpc
network mode. This structure specifies the
* VPC subnets and security groups associated with the task, and whether a public IP address is to be used. This
* structure is required if LaunchType
is FARGATE
because the awsvpc
mode is
* required for Fargate tasks.
*
*
* If you specify NetworkConfiguration
when the target ECS task does not use the awsvpc
* network mode, the task fails.
*
*
* @param networkConfiguration
* Use this structure if the Amazon ECS task uses the awsvpc
network mode. This structure
* specifies the VPC subnets and security groups associated with the task, and whether a public IP address is
* to be used. This structure is required if LaunchType
is FARGATE
because the
* awsvpc
mode is required for Fargate tasks.
*
* If you specify NetworkConfiguration
when the target ECS task does not use the
* awsvpc
network mode, the task fails.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public EcsParameters withNetworkConfiguration(NetworkConfiguration networkConfiguration) {
setNetworkConfiguration(networkConfiguration);
return this;
}
/**
*
* Specifies the platform version for the task. Specify only the numeric portion of the platform version, such as
* 1.1.0
.
*
*
* This structure is used only if LaunchType
is FARGATE
. For more information about valid
* platform versions, see Fargate Platform
* Versions in the Amazon Elastic Container Service Developer Guide.
*
*
* @param platformVersion
* Specifies the platform version for the task. Specify only the numeric portion of the platform version,
* such as 1.1.0
.
*
* This structure is used only if LaunchType
is FARGATE
. For more information about
* valid platform versions, see Fargate Platform
* Versions in the Amazon Elastic Container Service Developer Guide.
*/
public void setPlatformVersion(String platformVersion) {
this.platformVersion = platformVersion;
}
/**
*
* Specifies the platform version for the task. Specify only the numeric portion of the platform version, such as
* 1.1.0
.
*
*
* This structure is used only if LaunchType
is FARGATE
. For more information about valid
* platform versions, see Fargate Platform
* Versions in the Amazon Elastic Container Service Developer Guide.
*
*
* @return Specifies the platform version for the task. Specify only the numeric portion of the platform version,
* such as 1.1.0
.
*
* This structure is used only if LaunchType
is FARGATE
. For more information
* about valid platform versions, see Fargate
* Platform Versions in the Amazon Elastic Container Service Developer Guide.
*/
public String getPlatformVersion() {
return this.platformVersion;
}
/**
*
* Specifies the platform version for the task. Specify only the numeric portion of the platform version, such as
* 1.1.0
.
*
*
* This structure is used only if LaunchType
is FARGATE
. For more information about valid
* platform versions, see Fargate Platform
* Versions in the Amazon Elastic Container Service Developer Guide.
*
*
* @param platformVersion
* Specifies the platform version for the task. Specify only the numeric portion of the platform version,
* such as 1.1.0
.
*
* This structure is used only if LaunchType
is FARGATE
. For more information about
* valid platform versions, see Fargate Platform
* Versions in the Amazon Elastic Container Service Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public EcsParameters withPlatformVersion(String platformVersion) {
setPlatformVersion(platformVersion);
return this;
}
/**
*
* Specifies an ECS task group for the task. The maximum length is 255 characters.
*
*
* @param group
* Specifies an ECS task group for the task. The maximum length is 255 characters.
*/
public void setGroup(String group) {
this.group = group;
}
/**
*
* Specifies an ECS task group for the task. The maximum length is 255 characters.
*
*
* @return Specifies an ECS task group for the task. The maximum length is 255 characters.
*/
public String getGroup() {
return this.group;
}
/**
*
* Specifies an ECS task group for the task. The maximum length is 255 characters.
*
*
* @param group
* Specifies an ECS task group for the task. The maximum length is 255 characters.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public EcsParameters withGroup(String group) {
setGroup(group);
return this;
}
/**
*
* The capacity provider strategy to use for the task.
*
*
* If a capacityProviderStrategy
is specified, the launchType
parameter must be omitted.
* If no capacityProviderStrategy
or launchType is specified, the
* defaultCapacityProviderStrategy
for the cluster is used.
*
*
* @return The capacity provider strategy to use for the task.
*
* If a capacityProviderStrategy
is specified, the launchType
parameter must be
* omitted. If no capacityProviderStrategy
or launchType is specified, the
* defaultCapacityProviderStrategy
for the cluster is used.
*/
public java.util.List getCapacityProviderStrategy() {
return capacityProviderStrategy;
}
/**
*
* The capacity provider strategy to use for the task.
*
*
* If a capacityProviderStrategy
is specified, the launchType
parameter must be omitted.
* If no capacityProviderStrategy
or launchType is specified, the
* defaultCapacityProviderStrategy
for the cluster is used.
*
*
* @param capacityProviderStrategy
* The capacity provider strategy to use for the task.
*
* If a capacityProviderStrategy
is specified, the launchType
parameter must be
* omitted. If no capacityProviderStrategy
or launchType is specified, the
* defaultCapacityProviderStrategy
for the cluster is used.
*/
public void setCapacityProviderStrategy(java.util.Collection capacityProviderStrategy) {
if (capacityProviderStrategy == null) {
this.capacityProviderStrategy = null;
return;
}
this.capacityProviderStrategy = new java.util.ArrayList(capacityProviderStrategy);
}
/**
*
* The capacity provider strategy to use for the task.
*
*
* If a capacityProviderStrategy
is specified, the launchType
parameter must be omitted.
* If no capacityProviderStrategy
or launchType is specified, the
* defaultCapacityProviderStrategy
for the cluster is used.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setCapacityProviderStrategy(java.util.Collection)} or
* {@link #withCapacityProviderStrategy(java.util.Collection)} if you want to override the existing values.
*
*
* @param capacityProviderStrategy
* The capacity provider strategy to use for the task.
*
* If a capacityProviderStrategy
is specified, the launchType
parameter must be
* omitted. If no capacityProviderStrategy
or launchType is specified, the
* defaultCapacityProviderStrategy
for the cluster is used.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public EcsParameters withCapacityProviderStrategy(CapacityProviderStrategyItem... capacityProviderStrategy) {
if (this.capacityProviderStrategy == null) {
setCapacityProviderStrategy(new java.util.ArrayList(capacityProviderStrategy.length));
}
for (CapacityProviderStrategyItem ele : capacityProviderStrategy) {
this.capacityProviderStrategy.add(ele);
}
return this;
}
/**
*
* The capacity provider strategy to use for the task.
*
*
* If a capacityProviderStrategy
is specified, the launchType
parameter must be omitted.
* If no capacityProviderStrategy
or launchType is specified, the
* defaultCapacityProviderStrategy
for the cluster is used.
*
*
* @param capacityProviderStrategy
* The capacity provider strategy to use for the task.
*
* If a capacityProviderStrategy
is specified, the launchType
parameter must be
* omitted. If no capacityProviderStrategy
or launchType is specified, the
* defaultCapacityProviderStrategy
for the cluster is used.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public EcsParameters withCapacityProviderStrategy(java.util.Collection capacityProviderStrategy) {
setCapacityProviderStrategy(capacityProviderStrategy);
return this;
}
/**
*
* Specifies whether to enable Amazon ECS managed tags for the task. For more information, see Tagging Your Amazon ECS
* Resources in the Amazon Elastic Container Service Developer Guide.
*
*
* @param enableECSManagedTags
* Specifies whether to enable Amazon ECS managed tags for the task. For more information, see Tagging Your Amazon
* ECS Resources in the Amazon Elastic Container Service Developer Guide.
*/
public void setEnableECSManagedTags(Boolean enableECSManagedTags) {
this.enableECSManagedTags = enableECSManagedTags;
}
/**
*
* Specifies whether to enable Amazon ECS managed tags for the task. For more information, see Tagging Your Amazon ECS
* Resources in the Amazon Elastic Container Service Developer Guide.
*
*
* @return Specifies whether to enable Amazon ECS managed tags for the task. For more information, see Tagging Your
* Amazon ECS Resources in the Amazon Elastic Container Service Developer Guide.
*/
public Boolean getEnableECSManagedTags() {
return this.enableECSManagedTags;
}
/**
*
* Specifies whether to enable Amazon ECS managed tags for the task. For more information, see Tagging Your Amazon ECS
* Resources in the Amazon Elastic Container Service Developer Guide.
*
*
* @param enableECSManagedTags
* Specifies whether to enable Amazon ECS managed tags for the task. For more information, see Tagging Your Amazon
* ECS Resources in the Amazon Elastic Container Service Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public EcsParameters withEnableECSManagedTags(Boolean enableECSManagedTags) {
setEnableECSManagedTags(enableECSManagedTags);
return this;
}
/**
*
* Specifies whether to enable Amazon ECS managed tags for the task. For more information, see Tagging Your Amazon ECS
* Resources in the Amazon Elastic Container Service Developer Guide.
*
*
* @return Specifies whether to enable Amazon ECS managed tags for the task. For more information, see Tagging Your
* Amazon ECS Resources in the Amazon Elastic Container Service Developer Guide.
*/
public Boolean isEnableECSManagedTags() {
return this.enableECSManagedTags;
}
/**
*
* Whether or not to enable the execute command functionality for the containers in this task. If true, this enables
* execute command functionality on all containers in the task.
*
*
* @param enableExecuteCommand
* Whether or not to enable the execute command functionality for the containers in this task. If true, this
* enables execute command functionality on all containers in the task.
*/
public void setEnableExecuteCommand(Boolean enableExecuteCommand) {
this.enableExecuteCommand = enableExecuteCommand;
}
/**
*
* Whether or not to enable the execute command functionality for the containers in this task. If true, this enables
* execute command functionality on all containers in the task.
*
*
* @return Whether or not to enable the execute command functionality for the containers in this task. If true, this
* enables execute command functionality on all containers in the task.
*/
public Boolean getEnableExecuteCommand() {
return this.enableExecuteCommand;
}
/**
*
* Whether or not to enable the execute command functionality for the containers in this task. If true, this enables
* execute command functionality on all containers in the task.
*
*
* @param enableExecuteCommand
* Whether or not to enable the execute command functionality for the containers in this task. If true, this
* enables execute command functionality on all containers in the task.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public EcsParameters withEnableExecuteCommand(Boolean enableExecuteCommand) {
setEnableExecuteCommand(enableExecuteCommand);
return this;
}
/**
*
* Whether or not to enable the execute command functionality for the containers in this task. If true, this enables
* execute command functionality on all containers in the task.
*
*
* @return Whether or not to enable the execute command functionality for the containers in this task. If true, this
* enables execute command functionality on all containers in the task.
*/
public Boolean isEnableExecuteCommand() {
return this.enableExecuteCommand;
}
/**
*
* An array of placement constraint objects to use for the task. You can specify up to 10 constraints per task
* (including constraints in the task definition and those specified at runtime).
*
*
* @return An array of placement constraint objects to use for the task. You can specify up to 10 constraints per
* task (including constraints in the task definition and those specified at runtime).
*/
public java.util.List getPlacementConstraints() {
return placementConstraints;
}
/**
*
* An array of placement constraint objects to use for the task. You can specify up to 10 constraints per task
* (including constraints in the task definition and those specified at runtime).
*
*
* @param placementConstraints
* An array of placement constraint objects to use for the task. You can specify up to 10 constraints per
* task (including constraints in the task definition and those specified at runtime).
*/
public void setPlacementConstraints(java.util.Collection placementConstraints) {
if (placementConstraints == null) {
this.placementConstraints = null;
return;
}
this.placementConstraints = new java.util.ArrayList(placementConstraints);
}
/**
*
* An array of placement constraint objects to use for the task. You can specify up to 10 constraints per task
* (including constraints in the task definition and those specified at runtime).
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setPlacementConstraints(java.util.Collection)} or {@link #withPlacementConstraints(java.util.Collection)}
* if you want to override the existing values.
*
*
* @param placementConstraints
* An array of placement constraint objects to use for the task. You can specify up to 10 constraints per
* task (including constraints in the task definition and those specified at runtime).
* @return Returns a reference to this object so that method calls can be chained together.
*/
public EcsParameters withPlacementConstraints(PlacementConstraint... placementConstraints) {
if (this.placementConstraints == null) {
setPlacementConstraints(new java.util.ArrayList(placementConstraints.length));
}
for (PlacementConstraint ele : placementConstraints) {
this.placementConstraints.add(ele);
}
return this;
}
/**
*
* An array of placement constraint objects to use for the task. You can specify up to 10 constraints per task
* (including constraints in the task definition and those specified at runtime).
*
*
* @param placementConstraints
* An array of placement constraint objects to use for the task. You can specify up to 10 constraints per
* task (including constraints in the task definition and those specified at runtime).
* @return Returns a reference to this object so that method calls can be chained together.
*/
public EcsParameters withPlacementConstraints(java.util.Collection placementConstraints) {
setPlacementConstraints(placementConstraints);
return this;
}
/**
*
* The placement strategy objects to use for the task. You can specify a maximum of five strategy rules per task.
*
*
* @return The placement strategy objects to use for the task. You can specify a maximum of five strategy rules per
* task.
*/
public java.util.List getPlacementStrategy() {
return placementStrategy;
}
/**
*
* The placement strategy objects to use for the task. You can specify a maximum of five strategy rules per task.
*
*
* @param placementStrategy
* The placement strategy objects to use for the task. You can specify a maximum of five strategy rules per
* task.
*/
public void setPlacementStrategy(java.util.Collection placementStrategy) {
if (placementStrategy == null) {
this.placementStrategy = null;
return;
}
this.placementStrategy = new java.util.ArrayList(placementStrategy);
}
/**
*
* The placement strategy objects to use for the task. You can specify a maximum of five strategy rules per task.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setPlacementStrategy(java.util.Collection)} or {@link #withPlacementStrategy(java.util.Collection)} if
* you want to override the existing values.
*
*
* @param placementStrategy
* The placement strategy objects to use for the task. You can specify a maximum of five strategy rules per
* task.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public EcsParameters withPlacementStrategy(PlacementStrategy... placementStrategy) {
if (this.placementStrategy == null) {
setPlacementStrategy(new java.util.ArrayList(placementStrategy.length));
}
for (PlacementStrategy ele : placementStrategy) {
this.placementStrategy.add(ele);
}
return this;
}
/**
*
* The placement strategy objects to use for the task. You can specify a maximum of five strategy rules per task.
*
*
* @param placementStrategy
* The placement strategy objects to use for the task. You can specify a maximum of five strategy rules per
* task.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public EcsParameters withPlacementStrategy(java.util.Collection placementStrategy) {
setPlacementStrategy(placementStrategy);
return this;
}
/**
*
* Specifies whether to propagate the tags from the task definition to the task. If no value is specified, the tags
* are not propagated. Tags can only be propagated to the task during task creation. To add tags to a task after
* task creation, use the TagResource API action.
*
*
* @param propagateTags
* Specifies whether to propagate the tags from the task definition to the task. If no value is specified,
* the tags are not propagated. Tags can only be propagated to the task during task creation. To add tags to
* a task after task creation, use the TagResource API action.
* @see PropagateTags
*/
public void setPropagateTags(String propagateTags) {
this.propagateTags = propagateTags;
}
/**
*
* Specifies whether to propagate the tags from the task definition to the task. If no value is specified, the tags
* are not propagated. Tags can only be propagated to the task during task creation. To add tags to a task after
* task creation, use the TagResource API action.
*
*
* @return Specifies whether to propagate the tags from the task definition to the task. If no value is specified,
* the tags are not propagated. Tags can only be propagated to the task during task creation. To add tags to
* a task after task creation, use the TagResource API action.
* @see PropagateTags
*/
public String getPropagateTags() {
return this.propagateTags;
}
/**
*
* Specifies whether to propagate the tags from the task definition to the task. If no value is specified, the tags
* are not propagated. Tags can only be propagated to the task during task creation. To add tags to a task after
* task creation, use the TagResource API action.
*
*
* @param propagateTags
* Specifies whether to propagate the tags from the task definition to the task. If no value is specified,
* the tags are not propagated. Tags can only be propagated to the task during task creation. To add tags to
* a task after task creation, use the TagResource API action.
* @return Returns a reference to this object so that method calls can be chained together.
* @see PropagateTags
*/
public EcsParameters withPropagateTags(String propagateTags) {
setPropagateTags(propagateTags);
return this;
}
/**
*
* Specifies whether to propagate the tags from the task definition to the task. If no value is specified, the tags
* are not propagated. Tags can only be propagated to the task during task creation. To add tags to a task after
* task creation, use the TagResource API action.
*
*
* @param propagateTags
* Specifies whether to propagate the tags from the task definition to the task. If no value is specified,
* the tags are not propagated. Tags can only be propagated to the task during task creation. To add tags to
* a task after task creation, use the TagResource API action.
* @return Returns a reference to this object so that method calls can be chained together.
* @see PropagateTags
*/
public EcsParameters withPropagateTags(PropagateTags propagateTags) {
this.propagateTags = propagateTags.toString();
return this;
}
/**
*
* The reference ID to use for the task.
*
*
* @param referenceId
* The reference ID to use for the task.
*/
public void setReferenceId(String referenceId) {
this.referenceId = referenceId;
}
/**
*
* The reference ID to use for the task.
*
*
* @return The reference ID to use for the task.
*/
public String getReferenceId() {
return this.referenceId;
}
/**
*
* The reference ID to use for the task.
*
*
* @param referenceId
* The reference ID to use for the task.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public EcsParameters withReferenceId(String referenceId) {
setReferenceId(referenceId);
return this;
}
/**
*
* The metadata that you apply to the task to help you categorize and organize them. Each tag consists of a key and
* an optional value, both of which you define. To learn more, see RunTask in the Amazon ECS API Reference.
*
*
* @return The metadata that you apply to the task to help you categorize and organize them. Each tag consists of a
* key and an optional value, both of which you define. To learn more, see RunTask in the Amazon ECS API Reference.
*/
public java.util.List getTags() {
return tags;
}
/**
*
* The metadata that you apply to the task to help you categorize and organize them. Each tag consists of a key and
* an optional value, both of which you define. To learn more, see RunTask in the Amazon ECS API Reference.
*
*
* @param tags
* The metadata that you apply to the task to help you categorize and organize them. Each tag consists of a
* key and an optional value, both of which you define. To learn more, see RunTask in the Amazon ECS API Reference.
*/
public void setTags(java.util.Collection tags) {
if (tags == null) {
this.tags = null;
return;
}
this.tags = new java.util.ArrayList(tags);
}
/**
*
* The metadata that you apply to the task to help you categorize and organize them. Each tag consists of a key and
* an optional value, both of which you define. To learn more, see RunTask in the Amazon ECS API Reference.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTags(java.util.Collection)} or {@link #withTags(java.util.Collection)} if you want to override the
* existing values.
*
*
* @param tags
* The metadata that you apply to the task to help you categorize and organize them. Each tag consists of a
* key and an optional value, both of which you define. To learn more, see RunTask in the Amazon ECS API Reference.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public EcsParameters withTags(Tag... tags) {
if (this.tags == null) {
setTags(new java.util.ArrayList(tags.length));
}
for (Tag ele : tags) {
this.tags.add(ele);
}
return this;
}
/**
*
* The metadata that you apply to the task to help you categorize and organize them. Each tag consists of a key and
* an optional value, both of which you define. To learn more, see RunTask in the Amazon ECS API Reference.
*
*
* @param tags
* The metadata that you apply to the task to help you categorize and organize them. Each tag consists of a
* key and an optional value, both of which you define. To learn more, see RunTask in the Amazon ECS API Reference.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public EcsParameters withTags(java.util.Collection tags) {
setTags(tags);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getTaskDefinitionArn() != null)
sb.append("TaskDefinitionArn: ").append(getTaskDefinitionArn()).append(",");
if (getTaskCount() != null)
sb.append("TaskCount: ").append(getTaskCount()).append(",");
if (getLaunchType() != null)
sb.append("LaunchType: ").append(getLaunchType()).append(",");
if (getNetworkConfiguration() != null)
sb.append("NetworkConfiguration: ").append(getNetworkConfiguration()).append(",");
if (getPlatformVersion() != null)
sb.append("PlatformVersion: ").append(getPlatformVersion()).append(",");
if (getGroup() != null)
sb.append("Group: ").append(getGroup()).append(",");
if (getCapacityProviderStrategy() != null)
sb.append("CapacityProviderStrategy: ").append(getCapacityProviderStrategy()).append(",");
if (getEnableECSManagedTags() != null)
sb.append("EnableECSManagedTags: ").append(getEnableECSManagedTags()).append(",");
if (getEnableExecuteCommand() != null)
sb.append("EnableExecuteCommand: ").append(getEnableExecuteCommand()).append(",");
if (getPlacementConstraints() != null)
sb.append("PlacementConstraints: ").append(getPlacementConstraints()).append(",");
if (getPlacementStrategy() != null)
sb.append("PlacementStrategy: ").append(getPlacementStrategy()).append(",");
if (getPropagateTags() != null)
sb.append("PropagateTags: ").append(getPropagateTags()).append(",");
if (getReferenceId() != null)
sb.append("ReferenceId: ").append(getReferenceId()).append(",");
if (getTags() != null)
sb.append("Tags: ").append(getTags());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof EcsParameters == false)
return false;
EcsParameters other = (EcsParameters) obj;
if (other.getTaskDefinitionArn() == null ^ this.getTaskDefinitionArn() == null)
return false;
if (other.getTaskDefinitionArn() != null && other.getTaskDefinitionArn().equals(this.getTaskDefinitionArn()) == false)
return false;
if (other.getTaskCount() == null ^ this.getTaskCount() == null)
return false;
if (other.getTaskCount() != null && other.getTaskCount().equals(this.getTaskCount()) == false)
return false;
if (other.getLaunchType() == null ^ this.getLaunchType() == null)
return false;
if (other.getLaunchType() != null && other.getLaunchType().equals(this.getLaunchType()) == false)
return false;
if (other.getNetworkConfiguration() == null ^ this.getNetworkConfiguration() == null)
return false;
if (other.getNetworkConfiguration() != null && other.getNetworkConfiguration().equals(this.getNetworkConfiguration()) == false)
return false;
if (other.getPlatformVersion() == null ^ this.getPlatformVersion() == null)
return false;
if (other.getPlatformVersion() != null && other.getPlatformVersion().equals(this.getPlatformVersion()) == false)
return false;
if (other.getGroup() == null ^ this.getGroup() == null)
return false;
if (other.getGroup() != null && other.getGroup().equals(this.getGroup()) == false)
return false;
if (other.getCapacityProviderStrategy() == null ^ this.getCapacityProviderStrategy() == null)
return false;
if (other.getCapacityProviderStrategy() != null && other.getCapacityProviderStrategy().equals(this.getCapacityProviderStrategy()) == false)
return false;
if (other.getEnableECSManagedTags() == null ^ this.getEnableECSManagedTags() == null)
return false;
if (other.getEnableECSManagedTags() != null && other.getEnableECSManagedTags().equals(this.getEnableECSManagedTags()) == false)
return false;
if (other.getEnableExecuteCommand() == null ^ this.getEnableExecuteCommand() == null)
return false;
if (other.getEnableExecuteCommand() != null && other.getEnableExecuteCommand().equals(this.getEnableExecuteCommand()) == false)
return false;
if (other.getPlacementConstraints() == null ^ this.getPlacementConstraints() == null)
return false;
if (other.getPlacementConstraints() != null && other.getPlacementConstraints().equals(this.getPlacementConstraints()) == false)
return false;
if (other.getPlacementStrategy() == null ^ this.getPlacementStrategy() == null)
return false;
if (other.getPlacementStrategy() != null && other.getPlacementStrategy().equals(this.getPlacementStrategy()) == false)
return false;
if (other.getPropagateTags() == null ^ this.getPropagateTags() == null)
return false;
if (other.getPropagateTags() != null && other.getPropagateTags().equals(this.getPropagateTags()) == false)
return false;
if (other.getReferenceId() == null ^ this.getReferenceId() == null)
return false;
if (other.getReferenceId() != null && other.getReferenceId().equals(this.getReferenceId()) == false)
return false;
if (other.getTags() == null ^ this.getTags() == null)
return false;
if (other.getTags() != null && other.getTags().equals(this.getTags()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getTaskDefinitionArn() == null) ? 0 : getTaskDefinitionArn().hashCode());
hashCode = prime * hashCode + ((getTaskCount() == null) ? 0 : getTaskCount().hashCode());
hashCode = prime * hashCode + ((getLaunchType() == null) ? 0 : getLaunchType().hashCode());
hashCode = prime * hashCode + ((getNetworkConfiguration() == null) ? 0 : getNetworkConfiguration().hashCode());
hashCode = prime * hashCode + ((getPlatformVersion() == null) ? 0 : getPlatformVersion().hashCode());
hashCode = prime * hashCode + ((getGroup() == null) ? 0 : getGroup().hashCode());
hashCode = prime * hashCode + ((getCapacityProviderStrategy() == null) ? 0 : getCapacityProviderStrategy().hashCode());
hashCode = prime * hashCode + ((getEnableECSManagedTags() == null) ? 0 : getEnableECSManagedTags().hashCode());
hashCode = prime * hashCode + ((getEnableExecuteCommand() == null) ? 0 : getEnableExecuteCommand().hashCode());
hashCode = prime * hashCode + ((getPlacementConstraints() == null) ? 0 : getPlacementConstraints().hashCode());
hashCode = prime * hashCode + ((getPlacementStrategy() == null) ? 0 : getPlacementStrategy().hashCode());
hashCode = prime * hashCode + ((getPropagateTags() == null) ? 0 : getPropagateTags().hashCode());
hashCode = prime * hashCode + ((getReferenceId() == null) ? 0 : getReferenceId().hashCode());
hashCode = prime * hashCode + ((getTags() == null) ? 0 : getTags().hashCode());
return hashCode;
}
@Override
public EcsParameters clone() {
try {
return (EcsParameters) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.eventbridge.model.transform.EcsParametersMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}