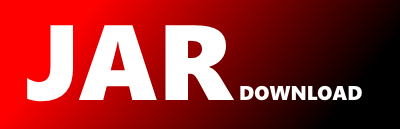
com.amazonaws.services.cloudwatchevents.AmazonCloudWatchEventsAsync Maven / Gradle / Ivy
/*
* Copyright 2012-2017 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.cloudwatchevents;
import javax.annotation.Generated;
import com.amazonaws.services.cloudwatchevents.model.*;
/**
* Interface for accessing Amazon CloudWatch Events asynchronously. Each asynchronous method will return a Java Future
* object representing the asynchronous operation; overloads which accept an {@code AsyncHandler} can be used to receive
* notification when an asynchronous operation completes.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.cloudwatchevents.AbstractAmazonCloudWatchEventsAsync} instead.
*
*
*
* Amazon CloudWatch Events helps you to respond to state changes in your AWS resources. When your resources change
* state, they automatically send events into an event stream. You can create rules that match selected events in the
* stream and route them to targets to take action. You can also use rules to take action on a pre-determined schedule.
* For example, you can configure rules to:
*
*
* -
*
* Automatically invoke an AWS Lambda function to update DNS entries when an event notifies you that Amazon EC2 instance
* enters the running state.
*
*
* -
*
* Direct specific API records from CloudTrail to an Amazon Kinesis stream for detailed analysis of potential security
* or availability risks.
*
*
* -
*
* Periodically invoke a built-in target to create a snapshot of an Amazon EBS volume.
*
*
*
*
* For more information about the features of Amazon CloudWatch Events, see the Amazon CloudWatch Events User Guide.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AmazonCloudWatchEventsAsync extends AmazonCloudWatchEvents {
/**
*
* Deletes the specified rule.
*
*
* You must remove all targets from a rule using RemoveTargets before you can delete the rule.
*
*
* When you delete a rule, incoming events might continue to match to the deleted rule. Please allow a short period
* of time for changes to take effect.
*
*
* @param deleteRuleRequest
* @return A Java Future containing the result of the DeleteRule operation returned by the service.
* @sample AmazonCloudWatchEventsAsync.DeleteRule
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteRuleAsync(DeleteRuleRequest deleteRuleRequest);
/**
*
* Deletes the specified rule.
*
*
* You must remove all targets from a rule using RemoveTargets before you can delete the rule.
*
*
* When you delete a rule, incoming events might continue to match to the deleted rule. Please allow a short period
* of time for changes to take effect.
*
*
* @param deleteRuleRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteRule operation returned by the service.
* @sample AmazonCloudWatchEventsAsyncHandler.DeleteRule
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteRuleAsync(DeleteRuleRequest deleteRuleRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes the specified rule.
*
*
* @param describeRuleRequest
* @return A Java Future containing the result of the DescribeRule operation returned by the service.
* @sample AmazonCloudWatchEventsAsync.DescribeRule
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeRuleAsync(DescribeRuleRequest describeRuleRequest);
/**
*
* Describes the specified rule.
*
*
* @param describeRuleRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeRule operation returned by the service.
* @sample AmazonCloudWatchEventsAsyncHandler.DescribeRule
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeRuleAsync(DescribeRuleRequest describeRuleRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Disables the specified rule. A disabled rule won't match any events, and won't self-trigger if it has a schedule
* expression.
*
*
* When you disable a rule, incoming events might continue to match to the disabled rule. Please allow a short
* period of time for changes to take effect.
*
*
* @param disableRuleRequest
* @return A Java Future containing the result of the DisableRule operation returned by the service.
* @sample AmazonCloudWatchEventsAsync.DisableRule
* @see AWS API
* Documentation
*/
java.util.concurrent.Future disableRuleAsync(DisableRuleRequest disableRuleRequest);
/**
*
* Disables the specified rule. A disabled rule won't match any events, and won't self-trigger if it has a schedule
* expression.
*
*
* When you disable a rule, incoming events might continue to match to the disabled rule. Please allow a short
* period of time for changes to take effect.
*
*
* @param disableRuleRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DisableRule operation returned by the service.
* @sample AmazonCloudWatchEventsAsyncHandler.DisableRule
* @see AWS API
* Documentation
*/
java.util.concurrent.Future disableRuleAsync(DisableRuleRequest disableRuleRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Enables the specified rule. If the rule does not exist, the operation fails.
*
*
* When you enable a rule, incoming events might not immediately start matching to a newly enabled rule. Please
* allow a short period of time for changes to take effect.
*
*
* @param enableRuleRequest
* @return A Java Future containing the result of the EnableRule operation returned by the service.
* @sample AmazonCloudWatchEventsAsync.EnableRule
* @see AWS API
* Documentation
*/
java.util.concurrent.Future enableRuleAsync(EnableRuleRequest enableRuleRequest);
/**
*
* Enables the specified rule. If the rule does not exist, the operation fails.
*
*
* When you enable a rule, incoming events might not immediately start matching to a newly enabled rule. Please
* allow a short period of time for changes to take effect.
*
*
* @param enableRuleRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the EnableRule operation returned by the service.
* @sample AmazonCloudWatchEventsAsyncHandler.EnableRule
* @see AWS API
* Documentation
*/
java.util.concurrent.Future enableRuleAsync(EnableRuleRequest enableRuleRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the rules for the specified target. You can see which of the rules in Amazon CloudWatch Events can invoke a
* specific target in your account.
*
*
* @param listRuleNamesByTargetRequest
* @return A Java Future containing the result of the ListRuleNamesByTarget operation returned by the service.
* @sample AmazonCloudWatchEventsAsync.ListRuleNamesByTarget
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listRuleNamesByTargetAsync(ListRuleNamesByTargetRequest listRuleNamesByTargetRequest);
/**
*
* Lists the rules for the specified target. You can see which of the rules in Amazon CloudWatch Events can invoke a
* specific target in your account.
*
*
* @param listRuleNamesByTargetRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListRuleNamesByTarget operation returned by the service.
* @sample AmazonCloudWatchEventsAsyncHandler.ListRuleNamesByTarget
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listRuleNamesByTargetAsync(ListRuleNamesByTargetRequest listRuleNamesByTargetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists your Amazon CloudWatch Events rules. You can either list all the rules or you can provide a prefix to match
* to the rule names.
*
*
* @param listRulesRequest
* @return A Java Future containing the result of the ListRules operation returned by the service.
* @sample AmazonCloudWatchEventsAsync.ListRules
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listRulesAsync(ListRulesRequest listRulesRequest);
/**
*
* Lists your Amazon CloudWatch Events rules. You can either list all the rules or you can provide a prefix to match
* to the rule names.
*
*
* @param listRulesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListRules operation returned by the service.
* @sample AmazonCloudWatchEventsAsyncHandler.ListRules
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listRulesAsync(ListRulesRequest listRulesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the targets assigned to the specified rule.
*
*
* @param listTargetsByRuleRequest
* @return A Java Future containing the result of the ListTargetsByRule operation returned by the service.
* @sample AmazonCloudWatchEventsAsync.ListTargetsByRule
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listTargetsByRuleAsync(ListTargetsByRuleRequest listTargetsByRuleRequest);
/**
*
* Lists the targets assigned to the specified rule.
*
*
* @param listTargetsByRuleRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListTargetsByRule operation returned by the service.
* @sample AmazonCloudWatchEventsAsyncHandler.ListTargetsByRule
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listTargetsByRuleAsync(ListTargetsByRuleRequest listTargetsByRuleRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Sends custom events to Amazon CloudWatch Events so that they can be matched to rules.
*
*
* @param putEventsRequest
* @return A Java Future containing the result of the PutEvents operation returned by the service.
* @sample AmazonCloudWatchEventsAsync.PutEvents
* @see AWS API
* Documentation
*/
java.util.concurrent.Future putEventsAsync(PutEventsRequest putEventsRequest);
/**
*
* Sends custom events to Amazon CloudWatch Events so that they can be matched to rules.
*
*
* @param putEventsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the PutEvents operation returned by the service.
* @sample AmazonCloudWatchEventsAsyncHandler.PutEvents
* @see AWS API
* Documentation
*/
java.util.concurrent.Future putEventsAsync(PutEventsRequest putEventsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates or updates the specified rule. Rules are enabled by default, or based on value of the state. You can
* disable a rule using DisableRule.
*
*
* When you create or update a rule, incoming events might not immediately start matching to new or updated rules.
* Please allow a short period of time for changes to take effect.
*
*
* A rule must contain at least an EventPattern or ScheduleExpression. Rules with EventPatterns are triggered when a
* matching event is observed. Rules with ScheduleExpressions self-trigger based on the given schedule. A rule can
* have both an EventPattern and a ScheduleExpression, in which case the rule triggers on matching events as well as
* on a schedule.
*
*
* Most services in AWS treat : or / as the same character in Amazon Resource Names (ARNs). However, CloudWatch
* Events uses an exact match in event patterns and rules. Be sure to use the correct ARN characters when creating
* event patterns so that they match the ARN syntax in the event you want to match.
*
*
* @param putRuleRequest
* @return A Java Future containing the result of the PutRule operation returned by the service.
* @sample AmazonCloudWatchEventsAsync.PutRule
* @see AWS API
* Documentation
*/
java.util.concurrent.Future putRuleAsync(PutRuleRequest putRuleRequest);
/**
*
* Creates or updates the specified rule. Rules are enabled by default, or based on value of the state. You can
* disable a rule using DisableRule.
*
*
* When you create or update a rule, incoming events might not immediately start matching to new or updated rules.
* Please allow a short period of time for changes to take effect.
*
*
* A rule must contain at least an EventPattern or ScheduleExpression. Rules with EventPatterns are triggered when a
* matching event is observed. Rules with ScheduleExpressions self-trigger based on the given schedule. A rule can
* have both an EventPattern and a ScheduleExpression, in which case the rule triggers on matching events as well as
* on a schedule.
*
*
* Most services in AWS treat : or / as the same character in Amazon Resource Names (ARNs). However, CloudWatch
* Events uses an exact match in event patterns and rules. Be sure to use the correct ARN characters when creating
* event patterns so that they match the ARN syntax in the event you want to match.
*
*
* @param putRuleRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the PutRule operation returned by the service.
* @sample AmazonCloudWatchEventsAsyncHandler.PutRule
* @see AWS API
* Documentation
*/
java.util.concurrent.Future putRuleAsync(PutRuleRequest putRuleRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Adds the specified targets to the specified rule, or updates the targets if they are already associated with the
* rule.
*
*
* Targets are the resources that are invoked when a rule is triggered. Example targets include EC2 instances, AWS
* Lambda functions, Amazon Kinesis streams, Amazon ECS tasks, AWS Step Functions state machines, and built-in
* targets. Note that creating rules with built-in targets is supported only in the AWS Management Console.
*
*
* For some target types, PutTargets
provides target-specific parameters. If the target is an Amazon
* Kinesis stream, you can optionally specify which shard the event goes to by using the
* KinesisParameters
argument. To invoke a command on multiple EC2 instances with one rule, you can use
* the RunCommandParameters
field.
*
*
* To be able to make API calls against the resources that you own, Amazon CloudWatch Events needs the appropriate
* permissions. For AWS Lambda and Amazon SNS resources, CloudWatch Events relies on resource-based policies. For
* EC2 instances, Amazon Kinesis streams, and AWS Step Functions state machines, CloudWatch Events relies on IAM
* roles that you specify in the RoleARN
argument in PutTarget
. For more information, see
*
* Authentication and Access Control in the Amazon CloudWatch Events User Guide.
*
*
* Input, InputPath and InputTransformer are mutually exclusive and optional parameters of a
* target. When a rule is triggered due to a matched event:
*
*
* -
*
* If none of the following arguments are specified for a target, then the entire event is passed to the target in
* JSON form (unless the target is Amazon EC2 Run Command or Amazon ECS task, in which case nothing from the event
* is passed to the target).
*
*
* -
*
* If Input is specified in the form of valid JSON, then the matched event is overridden with this constant.
*
*
* -
*
* If InputPath is specified in the form of JSONPath (for example, $.detail
), then only the part
* of the event specified in the path is passed to the target (for example, only the detail part of the event is
* passed).
*
*
* -
*
* If InputTransformer is specified, then one or more specified JSONPaths are extracted from the event and
* used as values in a template that you specify as the input to the target.
*
*
*
*
* When you add targets to a rule and the associated rule triggers soon after, new or updated targets might not be
* immediately invoked. Please allow a short period of time for changes to take effect.
*
*
* @param putTargetsRequest
* @return A Java Future containing the result of the PutTargets operation returned by the service.
* @sample AmazonCloudWatchEventsAsync.PutTargets
* @see AWS API
* Documentation
*/
java.util.concurrent.Future putTargetsAsync(PutTargetsRequest putTargetsRequest);
/**
*
* Adds the specified targets to the specified rule, or updates the targets if they are already associated with the
* rule.
*
*
* Targets are the resources that are invoked when a rule is triggered. Example targets include EC2 instances, AWS
* Lambda functions, Amazon Kinesis streams, Amazon ECS tasks, AWS Step Functions state machines, and built-in
* targets. Note that creating rules with built-in targets is supported only in the AWS Management Console.
*
*
* For some target types, PutTargets
provides target-specific parameters. If the target is an Amazon
* Kinesis stream, you can optionally specify which shard the event goes to by using the
* KinesisParameters
argument. To invoke a command on multiple EC2 instances with one rule, you can use
* the RunCommandParameters
field.
*
*
* To be able to make API calls against the resources that you own, Amazon CloudWatch Events needs the appropriate
* permissions. For AWS Lambda and Amazon SNS resources, CloudWatch Events relies on resource-based policies. For
* EC2 instances, Amazon Kinesis streams, and AWS Step Functions state machines, CloudWatch Events relies on IAM
* roles that you specify in the RoleARN
argument in PutTarget
. For more information, see
*
* Authentication and Access Control in the Amazon CloudWatch Events User Guide.
*
*
* Input, InputPath and InputTransformer are mutually exclusive and optional parameters of a
* target. When a rule is triggered due to a matched event:
*
*
* -
*
* If none of the following arguments are specified for a target, then the entire event is passed to the target in
* JSON form (unless the target is Amazon EC2 Run Command or Amazon ECS task, in which case nothing from the event
* is passed to the target).
*
*
* -
*
* If Input is specified in the form of valid JSON, then the matched event is overridden with this constant.
*
*
* -
*
* If InputPath is specified in the form of JSONPath (for example, $.detail
), then only the part
* of the event specified in the path is passed to the target (for example, only the detail part of the event is
* passed).
*
*
* -
*
* If InputTransformer is specified, then one or more specified JSONPaths are extracted from the event and
* used as values in a template that you specify as the input to the target.
*
*
*
*
* When you add targets to a rule and the associated rule triggers soon after, new or updated targets might not be
* immediately invoked. Please allow a short period of time for changes to take effect.
*
*
* @param putTargetsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the PutTargets operation returned by the service.
* @sample AmazonCloudWatchEventsAsyncHandler.PutTargets
* @see AWS API
* Documentation
*/
java.util.concurrent.Future putTargetsAsync(PutTargetsRequest putTargetsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Removes the specified targets from the specified rule. When the rule is triggered, those targets are no longer be
* invoked.
*
*
* When you remove a target, when the associated rule triggers, removed targets might continue to be invoked. Please
* allow a short period of time for changes to take effect.
*
*
* @param removeTargetsRequest
* @return A Java Future containing the result of the RemoveTargets operation returned by the service.
* @sample AmazonCloudWatchEventsAsync.RemoveTargets
* @see AWS API
* Documentation
*/
java.util.concurrent.Future removeTargetsAsync(RemoveTargetsRequest removeTargetsRequest);
/**
*
* Removes the specified targets from the specified rule. When the rule is triggered, those targets are no longer be
* invoked.
*
*
* When you remove a target, when the associated rule triggers, removed targets might continue to be invoked. Please
* allow a short period of time for changes to take effect.
*
*
* @param removeTargetsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the RemoveTargets operation returned by the service.
* @sample AmazonCloudWatchEventsAsyncHandler.RemoveTargets
* @see AWS API
* Documentation
*/
java.util.concurrent.Future removeTargetsAsync(RemoveTargetsRequest removeTargetsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Tests whether the specified event pattern matches the provided event.
*
*
* Most services in AWS treat : or / as the same character in Amazon Resource Names (ARNs). However, CloudWatch
* Events uses an exact match in event patterns and rules. Be sure to use the correct ARN characters when creating
* event patterns so that they match the ARN syntax in the event you want to match.
*
*
* @param testEventPatternRequest
* @return A Java Future containing the result of the TestEventPattern operation returned by the service.
* @sample AmazonCloudWatchEventsAsync.TestEventPattern
* @see AWS API
* Documentation
*/
java.util.concurrent.Future testEventPatternAsync(TestEventPatternRequest testEventPatternRequest);
/**
*
* Tests whether the specified event pattern matches the provided event.
*
*
* Most services in AWS treat : or / as the same character in Amazon Resource Names (ARNs). However, CloudWatch
* Events uses an exact match in event patterns and rules. Be sure to use the correct ARN characters when creating
* event patterns so that they match the ARN syntax in the event you want to match.
*
*
* @param testEventPatternRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the TestEventPattern operation returned by the service.
* @sample AmazonCloudWatchEventsAsyncHandler.TestEventPattern
* @see AWS API
* Documentation
*/
java.util.concurrent.Future testEventPatternAsync(TestEventPatternRequest testEventPatternRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
}