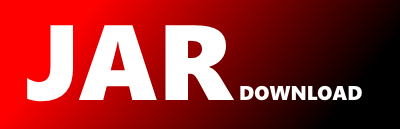
com.amazonaws.services.cloudwatchevents.AmazonCloudWatchEventsAsync Maven / Gradle / Ivy
Show all versions of aws-java-sdk-events Show documentation
/*
* Copyright 2011-2016 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not
* use this file except in compliance with the License. A copy of the License is
* located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on
* an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package com.amazonaws.services.cloudwatchevents;
import com.amazonaws.services.cloudwatchevents.model.*;
/**
* Interface for accessing Amazon CloudWatch Events asynchronously. Each
* asynchronous method will return a Java Future object representing the
* asynchronous operation; overloads which accept an {@code AsyncHandler} can be
* used to receive notification when an asynchronous operation completes.
*
*
* Amazon CloudWatch Events helps you to respond to state changes in your AWS
* resources. When your resources change state they automatically send events
* into an event stream. You can create rules that match selected events in the
* stream and route them to targets to take action. You can also use rules to
* take action on a pre-determined schedule. For example, you can configure
* rules to:
*
*
* - Automatically invoke an AWS Lambda function to update DNS entries when an
* event notifies you that Amazon EC2 instance enters the running state.
* - Direct specific API records from CloudTrail to an Amazon Kinesis stream
* for detailed analysis of potential security or availability risks.
* - Periodically invoke a built-in target to create a snapshot of an Amazon
* EBS volume.
*
*
* For more information about Amazon CloudWatch Events features, see the Amazon
* CloudWatch Developer Guide.
*
*/
public interface AmazonCloudWatchEventsAsync extends AmazonCloudWatchEvents {
/**
*
* Deletes a rule. You must remove all targets from a rule using
* RemoveTargets before you can delete the rule.
*
*
* Note: When you delete a rule, incoming events might still continue
* to match to the deleted rule. Please allow a short period of time for
* changes to take effect.
*
*
* @param deleteRuleRequest
* Container for the parameters to the DeleteRule operation.
* @return A Java Future containing the result of the DeleteRule operation
* returned by the service.
* @sample AmazonCloudWatchEventsAsync.DeleteRule
*/
java.util.concurrent.Future deleteRuleAsync(
DeleteRuleRequest deleteRuleRequest);
/**
*
* Deletes a rule. You must remove all targets from a rule using
* RemoveTargets before you can delete the rule.
*
*
* Note: When you delete a rule, incoming events might still continue
* to match to the deleted rule. Please allow a short period of time for
* changes to take effect.
*
*
* @param deleteRuleRequest
* Container for the parameters to the DeleteRule operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteRule operation
* returned by the service.
* @sample AmazonCloudWatchEventsAsyncHandler.DeleteRule
*/
java.util.concurrent.Future deleteRuleAsync(
DeleteRuleRequest deleteRuleRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes the details of the specified rule.
*
*
* @param describeRuleRequest
* Container for the parameters to the DescribeRule operation.
* @return A Java Future containing the result of the DescribeRule operation
* returned by the service.
* @sample AmazonCloudWatchEventsAsync.DescribeRule
*/
java.util.concurrent.Future describeRuleAsync(
DescribeRuleRequest describeRuleRequest);
/**
*
* Describes the details of the specified rule.
*
*
* @param describeRuleRequest
* Container for the parameters to the DescribeRule operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeRule operation
* returned by the service.
* @sample AmazonCloudWatchEventsAsyncHandler.DescribeRule
*/
java.util.concurrent.Future describeRuleAsync(
DescribeRuleRequest describeRuleRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Disables a rule. A disabled rule won't match any events, and won't
* self-trigger if it has a schedule expression.
*
*
* Note: When you disable a rule, incoming events might still
* continue to match to the disabled rule. Please allow a short period of
* time for changes to take effect.
*
*
* @param disableRuleRequest
* Container for the parameters to the DisableRule operation.
* @return A Java Future containing the result of the DisableRule operation
* returned by the service.
* @sample AmazonCloudWatchEventsAsync.DisableRule
*/
java.util.concurrent.Future disableRuleAsync(
DisableRuleRequest disableRuleRequest);
/**
*
* Disables a rule. A disabled rule won't match any events, and won't
* self-trigger if it has a schedule expression.
*
*
* Note: When you disable a rule, incoming events might still
* continue to match to the disabled rule. Please allow a short period of
* time for changes to take effect.
*
*
* @param disableRuleRequest
* Container for the parameters to the DisableRule operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DisableRule operation
* returned by the service.
* @sample AmazonCloudWatchEventsAsyncHandler.DisableRule
*/
java.util.concurrent.Future disableRuleAsync(
DisableRuleRequest disableRuleRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Enables a rule. If the rule does not exist, the operation fails.
*
*
* Note: When you enable a rule, incoming events might not
* immediately start matching to a newly enabled rule. Please allow a short
* period of time for changes to take effect.
*
*
* @param enableRuleRequest
* Container for the parameters to the EnableRule operation.
* @return A Java Future containing the result of the EnableRule operation
* returned by the service.
* @sample AmazonCloudWatchEventsAsync.EnableRule
*/
java.util.concurrent.Future enableRuleAsync(
EnableRuleRequest enableRuleRequest);
/**
*
* Enables a rule. If the rule does not exist, the operation fails.
*
*
* Note: When you enable a rule, incoming events might not
* immediately start matching to a newly enabled rule. Please allow a short
* period of time for changes to take effect.
*
*
* @param enableRuleRequest
* Container for the parameters to the EnableRule operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the EnableRule operation
* returned by the service.
* @sample AmazonCloudWatchEventsAsyncHandler.EnableRule
*/
java.util.concurrent.Future enableRuleAsync(
EnableRuleRequest enableRuleRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the names of the rules that the given target is put to. You can see
* which of the rules in Amazon CloudWatch Events can invoke a specific
* target in your account. If you have more rules in your account than the
* given limit, the results will be paginated. In that case, use the next
* token returned in the response and repeat ListRulesByTarget until the
* NextToken in the response is returned as null.
*
*
* @param listRuleNamesByTargetRequest
* Container for the parameters to the ListRuleNamesByTarget
* operation.
* @return A Java Future containing the result of the ListRuleNamesByTarget
* operation returned by the service.
* @sample AmazonCloudWatchEventsAsync.ListRuleNamesByTarget
*/
java.util.concurrent.Future listRuleNamesByTargetAsync(
ListRuleNamesByTargetRequest listRuleNamesByTargetRequest);
/**
*
* Lists the names of the rules that the given target is put to. You can see
* which of the rules in Amazon CloudWatch Events can invoke a specific
* target in your account. If you have more rules in your account than the
* given limit, the results will be paginated. In that case, use the next
* token returned in the response and repeat ListRulesByTarget until the
* NextToken in the response is returned as null.
*
*
* @param listRuleNamesByTargetRequest
* Container for the parameters to the ListRuleNamesByTarget
* operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListRuleNamesByTarget
* operation returned by the service.
* @sample AmazonCloudWatchEventsAsyncHandler.ListRuleNamesByTarget
*/
java.util.concurrent.Future listRuleNamesByTargetAsync(
ListRuleNamesByTargetRequest listRuleNamesByTargetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the Amazon CloudWatch Events rules in your account. You can either
* list all the rules or you can provide a prefix to match to the rule
* names. If you have more rules in your account than the given limit, the
* results will be paginated. In that case, use the next token returned in
* the response and repeat ListRules until the NextToken in the response is
* returned as null.
*
*
* @param listRulesRequest
* Container for the parameters to the ListRules operation.
* @return A Java Future containing the result of the ListRules operation
* returned by the service.
* @sample AmazonCloudWatchEventsAsync.ListRules
*/
java.util.concurrent.Future listRulesAsync(
ListRulesRequest listRulesRequest);
/**
*
* Lists the Amazon CloudWatch Events rules in your account. You can either
* list all the rules or you can provide a prefix to match to the rule
* names. If you have more rules in your account than the given limit, the
* results will be paginated. In that case, use the next token returned in
* the response and repeat ListRules until the NextToken in the response is
* returned as null.
*
*
* @param listRulesRequest
* Container for the parameters to the ListRules operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListRules operation
* returned by the service.
* @sample AmazonCloudWatchEventsAsyncHandler.ListRules
*/
java.util.concurrent.Future listRulesAsync(
ListRulesRequest listRulesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists of targets assigned to the rule.
*
*
* @param listTargetsByRuleRequest
* Container for the parameters to the ListTargetsByRule
* operation.
* @return A Java Future containing the result of the ListTargetsByRule
* operation returned by the service.
* @sample AmazonCloudWatchEventsAsync.ListTargetsByRule
*/
java.util.concurrent.Future listTargetsByRuleAsync(
ListTargetsByRuleRequest listTargetsByRuleRequest);
/**
*
* Lists of targets assigned to the rule.
*
*
* @param listTargetsByRuleRequest
* Container for the parameters to the ListTargetsByRule
* operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListTargetsByRule
* operation returned by the service.
* @sample AmazonCloudWatchEventsAsyncHandler.ListTargetsByRule
*/
java.util.concurrent.Future listTargetsByRuleAsync(
ListTargetsByRuleRequest listTargetsByRuleRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Sends custom events to Amazon CloudWatch Events so that they can be
* matched to rules.
*
*
* @param putEventsRequest
* Container for the parameters to the PutEvents operation.
* @return A Java Future containing the result of the PutEvents operation
* returned by the service.
* @sample AmazonCloudWatchEventsAsync.PutEvents
*/
java.util.concurrent.Future putEventsAsync(
PutEventsRequest putEventsRequest);
/**
*
* Sends custom events to Amazon CloudWatch Events so that they can be
* matched to rules.
*
*
* @param putEventsRequest
* Container for the parameters to the PutEvents operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the PutEvents operation
* returned by the service.
* @sample AmazonCloudWatchEventsAsyncHandler.PutEvents
*/
java.util.concurrent.Future putEventsAsync(
PutEventsRequest putEventsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates or updates a rule. Rules are enabled by default, or based on
* value of the State parameter. You can disable a rule using
* DisableRule.
*
*
* Note: When you create or update a rule, incoming events might not
* immediately start matching to new or updated rules. Please allow a short
* period of time for changes to take effect.
*
*
* A rule must contain at least an EventPattern or ScheduleExpression. Rules
* with EventPatterns are triggered when a matching event is observed. Rules
* with ScheduleExpressions self-trigger based on the given schedule. A rule
* can have both an EventPattern and a ScheduleExpression, in which case the
* rule will trigger on matching events as well as on a schedule.
*
*
* Note: Most services in AWS treat : or / as the same character in
* Amazon Resource Names (ARNs). However, CloudWatch Events uses an exact
* match in event patterns and rules. Be sure to use the correct ARN
* characters when creating event patterns so that they match the ARN syntax
* in the event you want to match.
*
*
* @param putRuleRequest
* Container for the parameters to the PutRule operation.
* @return A Java Future containing the result of the PutRule operation
* returned by the service.
* @sample AmazonCloudWatchEventsAsync.PutRule
*/
java.util.concurrent.Future putRuleAsync(
PutRuleRequest putRuleRequest);
/**
*
* Creates or updates a rule. Rules are enabled by default, or based on
* value of the State parameter. You can disable a rule using
* DisableRule.
*
*
* Note: When you create or update a rule, incoming events might not
* immediately start matching to new or updated rules. Please allow a short
* period of time for changes to take effect.
*
*
* A rule must contain at least an EventPattern or ScheduleExpression. Rules
* with EventPatterns are triggered when a matching event is observed. Rules
* with ScheduleExpressions self-trigger based on the given schedule. A rule
* can have both an EventPattern and a ScheduleExpression, in which case the
* rule will trigger on matching events as well as on a schedule.
*
*
* Note: Most services in AWS treat : or / as the same character in
* Amazon Resource Names (ARNs). However, CloudWatch Events uses an exact
* match in event patterns and rules. Be sure to use the correct ARN
* characters when creating event patterns so that they match the ARN syntax
* in the event you want to match.
*
*
* @param putRuleRequest
* Container for the parameters to the PutRule operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the PutRule operation
* returned by the service.
* @sample AmazonCloudWatchEventsAsyncHandler.PutRule
*/
java.util.concurrent.Future putRuleAsync(
PutRuleRequest putRuleRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Adds target(s) to a rule. Targets are the resources that can be invoked
* when a rule is triggered. For example, AWS Lambda functions, Amazon
* Kinesis streams, and built-in targets. Updates the target(s) if they are
* already associated with the role. In other words, if there is already a
* target with the given target ID, then the target associated with that ID
* is updated.
*
*
* In order to be able to make API calls against the resources you own,
* Amazon CloudWatch Events needs the appropriate permissions. For AWS
* Lambda and Amazon SNS resources, CloudWatch Events relies on
* resource-based policies. For Amazon Kinesis streams, CloudWatch Events
* relies on IAM roles. For more information, see Permissions for Sending Events to Targets in the Amazon
* CloudWatch Developer Guide.
*
*
* Input and InputPath are mutually-exclusive and optional
* parameters of a target. When a rule is triggered due to a matched event,
* if for a target:
*
*
* - Neither Input nor InputPath is specified, then the
* entire event is passed to the target in JSON form.
* - InputPath is specified in the form of JSONPath (e.g.
* $.detail), then only the part of the event specified in the path
* is passed to the target (e.g. only the detail part of the event is
* passed).
* - Input is specified in the form of a valid JSON, then the
* matched event is overridden with this constant.
*
*
* Note: When you add targets to a rule, when the associated rule
* triggers, new or updated targets might not be immediately invoked. Please
* allow a short period of time for changes to take effect.
*
*
* @param putTargetsRequest
* Container for the parameters to the PutTargets operation.
* @return A Java Future containing the result of the PutTargets operation
* returned by the service.
* @sample AmazonCloudWatchEventsAsync.PutTargets
*/
java.util.concurrent.Future putTargetsAsync(
PutTargetsRequest putTargetsRequest);
/**
*
* Adds target(s) to a rule. Targets are the resources that can be invoked
* when a rule is triggered. For example, AWS Lambda functions, Amazon
* Kinesis streams, and built-in targets. Updates the target(s) if they are
* already associated with the role. In other words, if there is already a
* target with the given target ID, then the target associated with that ID
* is updated.
*
*
* In order to be able to make API calls against the resources you own,
* Amazon CloudWatch Events needs the appropriate permissions. For AWS
* Lambda and Amazon SNS resources, CloudWatch Events relies on
* resource-based policies. For Amazon Kinesis streams, CloudWatch Events
* relies on IAM roles. For more information, see Permissions for Sending Events to Targets in the Amazon
* CloudWatch Developer Guide.
*
*
* Input and InputPath are mutually-exclusive and optional
* parameters of a target. When a rule is triggered due to a matched event,
* if for a target:
*
*
* - Neither Input nor InputPath is specified, then the
* entire event is passed to the target in JSON form.
* - InputPath is specified in the form of JSONPath (e.g.
* $.detail), then only the part of the event specified in the path
* is passed to the target (e.g. only the detail part of the event is
* passed).
* - Input is specified in the form of a valid JSON, then the
* matched event is overridden with this constant.
*
*
* Note: When you add targets to a rule, when the associated rule
* triggers, new or updated targets might not be immediately invoked. Please
* allow a short period of time for changes to take effect.
*
*
* @param putTargetsRequest
* Container for the parameters to the PutTargets operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the PutTargets operation
* returned by the service.
* @sample AmazonCloudWatchEventsAsyncHandler.PutTargets
*/
java.util.concurrent.Future putTargetsAsync(
PutTargetsRequest putTargetsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Removes target(s) from a rule so that when the rule is triggered, those
* targets will no longer be invoked.
*
*
* Note: When you remove a target, when the associated rule triggers,
* removed targets might still continue to be invoked. Please allow a short
* period of time for changes to take effect.
*
*
* @param removeTargetsRequest
* Container for the parameters to the RemoveTargets
* operation.
* @return A Java Future containing the result of the RemoveTargets
* operation returned by the service.
* @sample AmazonCloudWatchEventsAsync.RemoveTargets
*/
java.util.concurrent.Future removeTargetsAsync(
RemoveTargetsRequest removeTargetsRequest);
/**
*
* Removes target(s) from a rule so that when the rule is triggered, those
* targets will no longer be invoked.
*
*
* Note: When you remove a target, when the associated rule triggers,
* removed targets might still continue to be invoked. Please allow a short
* period of time for changes to take effect.
*
*
* @param removeTargetsRequest
* Container for the parameters to the RemoveTargets
* operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the RemoveTargets
* operation returned by the service.
* @sample AmazonCloudWatchEventsAsyncHandler.RemoveTargets
*/
java.util.concurrent.Future removeTargetsAsync(
RemoveTargetsRequest removeTargetsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Tests whether an event pattern matches the provided event.
*
*
* Note: Most services in AWS treat : or / as the same character in
* Amazon Resource Names (ARNs). However, CloudWatch Events uses an exact
* match in event patterns and rules. Be sure to use the correct ARN
* characters when creating event patterns so that they match the ARN syntax
* in the event you want to match.
*
*
* @param testEventPatternRequest
* Container for the parameters to the TestEventPattern
* operation.
* @return A Java Future containing the result of the TestEventPattern
* operation returned by the service.
* @sample AmazonCloudWatchEventsAsync.TestEventPattern
*/
java.util.concurrent.Future testEventPatternAsync(
TestEventPatternRequest testEventPatternRequest);
/**
*
* Tests whether an event pattern matches the provided event.
*
*
* Note: Most services in AWS treat : or / as the same character in
* Amazon Resource Names (ARNs). However, CloudWatch Events uses an exact
* match in event patterns and rules. Be sure to use the correct ARN
* characters when creating event patterns so that they match the ARN syntax
* in the event you want to match.
*
*
* @param testEventPatternRequest
* Container for the parameters to the TestEventPattern
* operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the TestEventPattern
* operation returned by the service.
* @sample AmazonCloudWatchEventsAsyncHandler.TestEventPattern
*/
java.util.concurrent.Future testEventPatternAsync(
TestEventPatternRequest testEventPatternRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
}