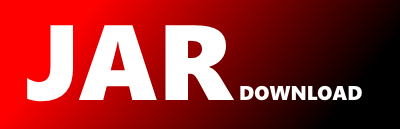
com.amazonaws.services.finspace.AWSfinspace Maven / Gradle / Ivy
Show all versions of aws-java-sdk-finspace Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.finspace;
import javax.annotation.Generated;
import com.amazonaws.*;
import com.amazonaws.regions.*;
import com.amazonaws.services.finspace.model.*;
/**
* Interface for accessing finspace.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.finspace.AbstractAWSfinspace} instead.
*
*
*
* The FinSpace management service provides the APIs for managing FinSpace environments.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AWSfinspace {
/**
* The region metadata service name for computing region endpoints. You can use this value to retrieve metadata
* (such as supported regions) of the service.
*
* @see RegionUtils#getRegionsForService(String)
*/
String ENDPOINT_PREFIX = "finspace";
/**
*
* Create a new FinSpace environment.
*
*
* @param createEnvironmentRequest
* @return Result of the CreateEnvironment operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ServiceQuotaExceededException
* You have exceeded your service quota. To perform the requested action, remove some of the relevant
* resources, or use Service Quotas to request a service quota increase.
* @throws LimitExceededException
* A service limit or quota is exceeded.
* @sample AWSfinspace.CreateEnvironment
* @see AWS API
* Documentation
*/
@Deprecated
CreateEnvironmentResult createEnvironment(CreateEnvironmentRequest createEnvironmentRequest);
/**
*
* Creates a changeset for a kdb database. A changeset allows you to add and delete existing files by using an
* ordered list of change requests.
*
*
* @param createKxChangesetRequest
* @return Result of the CreateKxChangeset operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws ConflictException
* There was a conflict with this action, and it could not be completed.
* @throws LimitExceededException
* A service limit or quota is exceeded.
* @sample AWSfinspace.CreateKxChangeset
* @see AWS API
* Documentation
*/
CreateKxChangesetResult createKxChangeset(CreateKxChangesetRequest createKxChangesetRequest);
/**
*
* Creates a new kdb cluster.
*
*
* @param createKxClusterRequest
* @return Result of the CreateKxCluster operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws LimitExceededException
* A service limit or quota is exceeded.
* @throws ConflictException
* There was a conflict with this action, and it could not be completed.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @sample AWSfinspace.CreateKxCluster
* @see AWS API
* Documentation
*/
CreateKxClusterResult createKxCluster(CreateKxClusterRequest createKxClusterRequest);
/**
*
* Creates a new kdb database in the environment.
*
*
* @param createKxDatabaseRequest
* @return Result of the CreateKxDatabase operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ConflictException
* There was a conflict with this action, and it could not be completed.
* @throws ResourceAlreadyExistsException
* The specified resource group already exists.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws LimitExceededException
* A service limit or quota is exceeded.
* @sample AWSfinspace.CreateKxDatabase
* @see AWS API
* Documentation
*/
CreateKxDatabaseResult createKxDatabase(CreateKxDatabaseRequest createKxDatabaseRequest);
/**
*
* Creates a snapshot of kdb database with tiered storage capabilities and a pre-warmed cache, ready for mounting on
* kdb clusters. Dataviews are only available for clusters running on a scaling group. They are not supported on
* dedicated clusters.
*
*
* @param createKxDataviewRequest
* @return Result of the CreateKxDataview operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws ConflictException
* There was a conflict with this action, and it could not be completed.
* @throws LimitExceededException
* A service limit or quota is exceeded.
* @throws ResourceAlreadyExistsException
* The specified resource group already exists.
* @sample AWSfinspace.CreateKxDataview
* @see AWS API
* Documentation
*/
CreateKxDataviewResult createKxDataview(CreateKxDataviewRequest createKxDataviewRequest);
/**
*
* Creates a managed kdb environment for the account.
*
*
* @param createKxEnvironmentRequest
* @return Result of the CreateKxEnvironment operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ServiceQuotaExceededException
* You have exceeded your service quota. To perform the requested action, remove some of the relevant
* resources, or use Service Quotas to request a service quota increase.
* @throws LimitExceededException
* A service limit or quota is exceeded.
* @throws ConflictException
* There was a conflict with this action, and it could not be completed.
* @sample AWSfinspace.CreateKxEnvironment
* @see AWS
* API Documentation
*/
CreateKxEnvironmentResult createKxEnvironment(CreateKxEnvironmentRequest createKxEnvironmentRequest);
/**
*
* Creates a new scaling group.
*
*
* @param createKxScalingGroupRequest
* @return Result of the CreateKxScalingGroup operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws LimitExceededException
* A service limit or quota is exceeded.
* @throws ConflictException
* There was a conflict with this action, and it could not be completed.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @sample AWSfinspace.CreateKxScalingGroup
* @see AWS
* API Documentation
*/
CreateKxScalingGroupResult createKxScalingGroup(CreateKxScalingGroupRequest createKxScalingGroupRequest);
/**
*
* Creates a user in FinSpace kdb environment with an associated IAM role.
*
*
* @param createKxUserRequest
* @return Result of the CreateKxUser operation returned by the service.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws ResourceAlreadyExistsException
* The specified resource group already exists.
* @throws LimitExceededException
* A service limit or quota is exceeded.
* @throws ConflictException
* There was a conflict with this action, and it could not be completed.
* @sample AWSfinspace.CreateKxUser
* @see AWS API
* Documentation
*/
CreateKxUserResult createKxUser(CreateKxUserRequest createKxUserRequest);
/**
*
* Creates a new volume with a specific amount of throughput and storage capacity.
*
*
* @param createKxVolumeRequest
* @return Result of the CreateKxVolume operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws LimitExceededException
* A service limit or quota is exceeded.
* @throws ConflictException
* There was a conflict with this action, and it could not be completed.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws ResourceAlreadyExistsException
* The specified resource group already exists.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @sample AWSfinspace.CreateKxVolume
* @see AWS API
* Documentation
*/
CreateKxVolumeResult createKxVolume(CreateKxVolumeRequest createKxVolumeRequest);
/**
*
* Delete an FinSpace environment.
*
*
* @param deleteEnvironmentRequest
* @return Result of the DeleteEnvironment operation returned by the service.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @sample AWSfinspace.DeleteEnvironment
* @see AWS API
* Documentation
*/
@Deprecated
DeleteEnvironmentResult deleteEnvironment(DeleteEnvironmentRequest deleteEnvironmentRequest);
/**
*
* Deletes a kdb cluster.
*
*
* @param deleteKxClusterRequest
* @return Result of the DeleteKxCluster operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws LimitExceededException
* A service limit or quota is exceeded.
* @throws ConflictException
* There was a conflict with this action, and it could not be completed.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @sample AWSfinspace.DeleteKxCluster
* @see AWS API
* Documentation
*/
DeleteKxClusterResult deleteKxCluster(DeleteKxClusterRequest deleteKxClusterRequest);
/**
*
* Deletes the specified nodes from a cluster.
*
*
* @param deleteKxClusterNodeRequest
* @return Result of the DeleteKxClusterNode operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @sample AWSfinspace.DeleteKxClusterNode
* @see AWS
* API Documentation
*/
DeleteKxClusterNodeResult deleteKxClusterNode(DeleteKxClusterNodeRequest deleteKxClusterNodeRequest);
/**
*
* Deletes the specified database and all of its associated data. This action is irreversible. You must copy any
* data out of the database before deleting it if the data is to be retained.
*
*
* @param deleteKxDatabaseRequest
* @return Result of the DeleteKxDatabase operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws ConflictException
* There was a conflict with this action, and it could not be completed.
* @sample AWSfinspace.DeleteKxDatabase
* @see AWS API
* Documentation
*/
DeleteKxDatabaseResult deleteKxDatabase(DeleteKxDatabaseRequest deleteKxDatabaseRequest);
/**
*
* Deletes the specified dataview. Before deleting a dataview, make sure that it is not in use by any cluster.
*
*
* @param deleteKxDataviewRequest
* @return Result of the DeleteKxDataview operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws ConflictException
* There was a conflict with this action, and it could not be completed.
* @sample AWSfinspace.DeleteKxDataview
* @see AWS API
* Documentation
*/
DeleteKxDataviewResult deleteKxDataview(DeleteKxDataviewRequest deleteKxDataviewRequest);
/**
*
* Deletes the kdb environment. This action is irreversible. Deleting a kdb environment will remove all the
* associated data and any services running in it.
*
*
* @param deleteKxEnvironmentRequest
* @return Result of the DeleteKxEnvironment operation returned by the service.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws ConflictException
* There was a conflict with this action, and it could not be completed.
* @sample AWSfinspace.DeleteKxEnvironment
* @see AWS
* API Documentation
*/
DeleteKxEnvironmentResult deleteKxEnvironment(DeleteKxEnvironmentRequest deleteKxEnvironmentRequest);
/**
*
* Deletes the specified scaling group. This action is irreversible. You cannot delete a scaling group until all the
* clusters running on it have been deleted.
*
*
* @param deleteKxScalingGroupRequest
* @return Result of the DeleteKxScalingGroup operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws LimitExceededException
* A service limit or quota is exceeded.
* @throws ConflictException
* There was a conflict with this action, and it could not be completed.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @sample AWSfinspace.DeleteKxScalingGroup
* @see AWS
* API Documentation
*/
DeleteKxScalingGroupResult deleteKxScalingGroup(DeleteKxScalingGroupRequest deleteKxScalingGroupRequest);
/**
*
* Deletes a user in the specified kdb environment.
*
*
* @param deleteKxUserRequest
* @return Result of the DeleteKxUser operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws ConflictException
* There was a conflict with this action, and it could not be completed.
* @sample AWSfinspace.DeleteKxUser
* @see AWS API
* Documentation
*/
DeleteKxUserResult deleteKxUser(DeleteKxUserRequest deleteKxUserRequest);
/**
*
* Deletes a volume. You can only delete a volume if it's not attached to a cluster or a dataview. When a volume is
* deleted, any data on the volume is lost. This action is irreversible.
*
*
* @param deleteKxVolumeRequest
* @return Result of the DeleteKxVolume operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws LimitExceededException
* A service limit or quota is exceeded.
* @throws ConflictException
* There was a conflict with this action, and it could not be completed.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @sample AWSfinspace.DeleteKxVolume
* @see AWS API
* Documentation
*/
DeleteKxVolumeResult deleteKxVolume(DeleteKxVolumeRequest deleteKxVolumeRequest);
/**
*
* Returns the FinSpace environment object.
*
*
* @param getEnvironmentRequest
* @return Result of the GetEnvironment operation returned by the service.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @sample AWSfinspace.GetEnvironment
* @see AWS API
* Documentation
*/
@Deprecated
GetEnvironmentResult getEnvironment(GetEnvironmentRequest getEnvironmentRequest);
/**
*
* Returns information about a kdb changeset.
*
*
* @param getKxChangesetRequest
* @return Result of the GetKxChangeset operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @sample AWSfinspace.GetKxChangeset
* @see AWS API
* Documentation
*/
GetKxChangesetResult getKxChangeset(GetKxChangesetRequest getKxChangesetRequest);
/**
*
* Retrieves information about a kdb cluster.
*
*
* @param getKxClusterRequest
* @return Result of the GetKxCluster operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws LimitExceededException
* A service limit or quota is exceeded.
* @throws ConflictException
* There was a conflict with this action, and it could not be completed.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @sample AWSfinspace.GetKxCluster
* @see AWS API
* Documentation
*/
GetKxClusterResult getKxCluster(GetKxClusterRequest getKxClusterRequest);
/**
*
* Retrieves a connection string for a user to connect to a kdb cluster. You must call this API using the same role
* that you have defined while creating a user.
*
*
* @param getKxConnectionStringRequest
* @return Result of the GetKxConnectionString operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @sample AWSfinspace.GetKxConnectionString
* @see AWS
* API Documentation
*/
GetKxConnectionStringResult getKxConnectionString(GetKxConnectionStringRequest getKxConnectionStringRequest);
/**
*
* Returns database information for the specified environment ID.
*
*
* @param getKxDatabaseRequest
* @return Result of the GetKxDatabase operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @sample AWSfinspace.GetKxDatabase
* @see AWS API
* Documentation
*/
GetKxDatabaseResult getKxDatabase(GetKxDatabaseRequest getKxDatabaseRequest);
/**
*
* Retrieves details of the dataview.
*
*
* @param getKxDataviewRequest
* @return Result of the GetKxDataview operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @sample AWSfinspace.GetKxDataview
* @see AWS API
* Documentation
*/
GetKxDataviewResult getKxDataview(GetKxDataviewRequest getKxDataviewRequest);
/**
*
* Retrieves all the information for the specified kdb environment.
*
*
* @param getKxEnvironmentRequest
* @return Result of the GetKxEnvironment operation returned by the service.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ConflictException
* There was a conflict with this action, and it could not be completed.
* @sample AWSfinspace.GetKxEnvironment
* @see AWS API
* Documentation
*/
GetKxEnvironmentResult getKxEnvironment(GetKxEnvironmentRequest getKxEnvironmentRequest);
/**
*
* Retrieves details of a scaling group.
*
*
* @param getKxScalingGroupRequest
* @return Result of the GetKxScalingGroup operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws LimitExceededException
* A service limit or quota is exceeded.
* @throws ConflictException
* There was a conflict with this action, and it could not be completed.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @sample AWSfinspace.GetKxScalingGroup
* @see AWS API
* Documentation
*/
GetKxScalingGroupResult getKxScalingGroup(GetKxScalingGroupRequest getKxScalingGroupRequest);
/**
*
* Retrieves information about the specified kdb user.
*
*
* @param getKxUserRequest
* @return Result of the GetKxUser operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @sample AWSfinspace.GetKxUser
* @see AWS API
* Documentation
*/
GetKxUserResult getKxUser(GetKxUserRequest getKxUserRequest);
/**
*
* Retrieves the information about the volume.
*
*
* @param getKxVolumeRequest
* @return Result of the GetKxVolume operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws LimitExceededException
* A service limit or quota is exceeded.
* @throws ConflictException
* There was a conflict with this action, and it could not be completed.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @sample AWSfinspace.GetKxVolume
* @see AWS API
* Documentation
*/
GetKxVolumeResult getKxVolume(GetKxVolumeRequest getKxVolumeRequest);
/**
*
* A list of all of your FinSpace environments.
*
*
* @param listEnvironmentsRequest
* @return Result of the ListEnvironments operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @sample AWSfinspace.ListEnvironments
* @see AWS API
* Documentation
*/
@Deprecated
ListEnvironmentsResult listEnvironments(ListEnvironmentsRequest listEnvironmentsRequest);
/**
*
* Returns a list of all the changesets for a database.
*
*
* @param listKxChangesetsRequest
* @return Result of the ListKxChangesets operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @sample AWSfinspace.ListKxChangesets
* @see AWS API
* Documentation
*/
ListKxChangesetsResult listKxChangesets(ListKxChangesetsRequest listKxChangesetsRequest);
/**
*
* Lists all the nodes in a kdb cluster.
*
*
* @param listKxClusterNodesRequest
* @return Result of the ListKxClusterNodes operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws LimitExceededException
* A service limit or quota is exceeded.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @sample AWSfinspace.ListKxClusterNodes
* @see AWS
* API Documentation
*/
ListKxClusterNodesResult listKxClusterNodes(ListKxClusterNodesRequest listKxClusterNodesRequest);
/**
*
* Returns a list of clusters.
*
*
* @param listKxClustersRequest
* @return Result of the ListKxClusters operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws LimitExceededException
* A service limit or quota is exceeded.
* @throws ConflictException
* There was a conflict with this action, and it could not be completed.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @sample AWSfinspace.ListKxClusters
* @see AWS API
* Documentation
*/
ListKxClustersResult listKxClusters(ListKxClustersRequest listKxClustersRequest);
/**
*
* Returns a list of all the databases in the kdb environment.
*
*
* @param listKxDatabasesRequest
* @return Result of the ListKxDatabases operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @sample AWSfinspace.ListKxDatabases
* @see AWS API
* Documentation
*/
ListKxDatabasesResult listKxDatabases(ListKxDatabasesRequest listKxDatabasesRequest);
/**
*
* Returns a list of all the dataviews in the database.
*
*
* @param listKxDataviewsRequest
* @return Result of the ListKxDataviews operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @sample AWSfinspace.ListKxDataviews
* @see AWS API
* Documentation
*/
ListKxDataviewsResult listKxDataviews(ListKxDataviewsRequest listKxDataviewsRequest);
/**
*
* Returns a list of kdb environments created in an account.
*
*
* @param listKxEnvironmentsRequest
* @return Result of the ListKxEnvironments operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @sample AWSfinspace.ListKxEnvironments
* @see AWS
* API Documentation
*/
ListKxEnvironmentsResult listKxEnvironments(ListKxEnvironmentsRequest listKxEnvironmentsRequest);
/**
*
* Returns a list of scaling groups in a kdb environment.
*
*
* @param listKxScalingGroupsRequest
* @return Result of the ListKxScalingGroups operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws LimitExceededException
* A service limit or quota is exceeded.
* @throws ConflictException
* There was a conflict with this action, and it could not be completed.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @sample AWSfinspace.ListKxScalingGroups
* @see AWS
* API Documentation
*/
ListKxScalingGroupsResult listKxScalingGroups(ListKxScalingGroupsRequest listKxScalingGroupsRequest);
/**
*
* Lists all the users in a kdb environment.
*
*
* @param listKxUsersRequest
* @return Result of the ListKxUsers operation returned by the service.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @sample AWSfinspace.ListKxUsers
* @see AWS API
* Documentation
*/
ListKxUsersResult listKxUsers(ListKxUsersRequest listKxUsersRequest);
/**
*
* Lists all the volumes in a kdb environment.
*
*
* @param listKxVolumesRequest
* @return Result of the ListKxVolumes operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws LimitExceededException
* A service limit or quota is exceeded.
* @throws ConflictException
* There was a conflict with this action, and it could not be completed.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @sample AWSfinspace.ListKxVolumes
* @see AWS API
* Documentation
*/
ListKxVolumesResult listKxVolumes(ListKxVolumesRequest listKxVolumesRequest);
/**
*
* A list of all tags for a resource.
*
*
* @param listTagsForResourceRequest
* @return Result of the ListTagsForResource operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws InvalidRequestException
* The request is invalid. Something is wrong with the input to the request.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @sample AWSfinspace.ListTagsForResource
* @see AWS
* API Documentation
*/
ListTagsForResourceResult listTagsForResource(ListTagsForResourceRequest listTagsForResourceRequest);
/**
*
* Adds metadata tags to a FinSpace resource.
*
*
* @param tagResourceRequest
* @return Result of the TagResource operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws InvalidRequestException
* The request is invalid. Something is wrong with the input to the request.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @sample AWSfinspace.TagResource
* @see AWS API
* Documentation
*/
TagResourceResult tagResource(TagResourceRequest tagResourceRequest);
/**
*
* Removes metadata tags from a FinSpace resource.
*
*
* @param untagResourceRequest
* @return Result of the UntagResource operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws InvalidRequestException
* The request is invalid. Something is wrong with the input to the request.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @sample AWSfinspace.UntagResource
* @see AWS API
* Documentation
*/
UntagResourceResult untagResource(UntagResourceRequest untagResourceRequest);
/**
*
* Update your FinSpace environment.
*
*
* @param updateEnvironmentRequest
* @return Result of the UpdateEnvironment operation returned by the service.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @sample AWSfinspace.UpdateEnvironment
* @see AWS API
* Documentation
*/
@Deprecated
UpdateEnvironmentResult updateEnvironment(UpdateEnvironmentRequest updateEnvironmentRequest);
/**
*
* Allows you to update code configuration on a running cluster. By using this API you can update the code, the
* initialization script path, and the command line arguments for a specific cluster. The configuration that you
* want to update will override any existing configurations on the cluster.
*
*
* @param updateKxClusterCodeConfigurationRequest
* @return Result of the UpdateKxClusterCodeConfiguration operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws LimitExceededException
* A service limit or quota is exceeded.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws ConflictException
* There was a conflict with this action, and it could not be completed.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @sample AWSfinspace.UpdateKxClusterCodeConfiguration
* @see AWS API Documentation
*/
UpdateKxClusterCodeConfigurationResult updateKxClusterCodeConfiguration(UpdateKxClusterCodeConfigurationRequest updateKxClusterCodeConfigurationRequest);
/**
*
* Updates the databases mounted on a kdb cluster, which includes the changesetId
and all the dbPaths
* to be cached. This API does not allow you to change a database name or add a database if you created a cluster
* without one.
*
*
* Using this API you can point a cluster to a different changeset and modify a list of partitions being cached.
*
*
* @param updateKxClusterDatabasesRequest
* @return Result of the UpdateKxClusterDatabases operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws LimitExceededException
* A service limit or quota is exceeded.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws ConflictException
* There was a conflict with this action, and it could not be completed.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @sample AWSfinspace.UpdateKxClusterDatabases
* @see AWS API Documentation
*/
UpdateKxClusterDatabasesResult updateKxClusterDatabases(UpdateKxClusterDatabasesRequest updateKxClusterDatabasesRequest);
/**
*
* Updates information for the given kdb database.
*
*
* @param updateKxDatabaseRequest
* @return Result of the UpdateKxDatabase operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws ConflictException
* There was a conflict with this action, and it could not be completed.
* @sample AWSfinspace.UpdateKxDatabase
* @see AWS API
* Documentation
*/
UpdateKxDatabaseResult updateKxDatabase(UpdateKxDatabaseRequest updateKxDatabaseRequest);
/**
*
* Updates the specified dataview. The dataviews get automatically updated when any new changesets are ingested.
* Each update of the dataview creates a new version, including changeset details and cache configurations
*
*
* @param updateKxDataviewRequest
* @return Result of the UpdateKxDataview operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws ConflictException
* There was a conflict with this action, and it could not be completed.
* @throws ResourceAlreadyExistsException
* The specified resource group already exists.
* @sample AWSfinspace.UpdateKxDataview
* @see AWS API
* Documentation
*/
UpdateKxDataviewResult updateKxDataview(UpdateKxDataviewRequest updateKxDataviewRequest);
/**
*
* Updates information for the given kdb environment.
*
*
* @param updateKxEnvironmentRequest
* @return Result of the UpdateKxEnvironment operation returned by the service.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws ConflictException
* There was a conflict with this action, and it could not be completed.
* @sample AWSfinspace.UpdateKxEnvironment
* @see AWS
* API Documentation
*/
UpdateKxEnvironmentResult updateKxEnvironment(UpdateKxEnvironmentRequest updateKxEnvironmentRequest);
/**
*
* Updates environment network to connect to your internal network by using a transit gateway. This API supports
* request to create a transit gateway attachment from FinSpace VPC to your transit gateway ID and create a custom
* Route-53 outbound resolvers.
*
*
* Once you send a request to update a network, you cannot change it again. Network update might require termination
* of any clusters that are running in the existing network.
*
*
* @param updateKxEnvironmentNetworkRequest
* @return Result of the UpdateKxEnvironmentNetwork operation returned by the service.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws ConflictException
* There was a conflict with this action, and it could not be completed.
* @sample AWSfinspace.UpdateKxEnvironmentNetwork
* @see AWS API Documentation
*/
UpdateKxEnvironmentNetworkResult updateKxEnvironmentNetwork(UpdateKxEnvironmentNetworkRequest updateKxEnvironmentNetworkRequest);
/**
*
* Updates the user details. You can only update the IAM role associated with a user.
*
*
* @param updateKxUserRequest
* @return Result of the UpdateKxUser operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws LimitExceededException
* A service limit or quota is exceeded.
* @throws ConflictException
* There was a conflict with this action, and it could not be completed.
* @sample AWSfinspace.UpdateKxUser
* @see AWS API
* Documentation
*/
UpdateKxUserResult updateKxUser(UpdateKxUserRequest updateKxUserRequest);
/**
*
* Updates the throughput or capacity of a volume. During the update process, the filesystem might be unavailable
* for a few minutes. You can retry any operations after the update is complete.
*
*
* @param updateKxVolumeRequest
* @return Result of the UpdateKxVolume operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws LimitExceededException
* A service limit or quota is exceeded.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws ConflictException
* There was a conflict with this action, and it could not be completed.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @sample AWSfinspace.UpdateKxVolume
* @see AWS API
* Documentation
*/
UpdateKxVolumeResult updateKxVolume(UpdateKxVolumeRequest updateKxVolumeRequest);
/**
* Shuts down this client object, releasing any resources that might be held open. This is an optional method, and
* callers are not expected to call it, but can if they want to explicitly release any open resources. Once a client
* has been shutdown, it should not be used to make any more requests.
*/
void shutdown();
/**
* Returns additional metadata for a previously executed successful request, typically used for debugging issues
* where a service isn't acting as expected. This data isn't considered part of the result data returned by an
* operation, so it's available through this separate, diagnostic interface.
*
* Response metadata is only cached for a limited period of time, so if you need to access this extra diagnostic
* information for an executed request, you should use this method to retrieve it as soon as possible after
* executing a request.
*
* @param request
* The originally executed request.
*
* @return The response metadata for the specified request, or null if none is available.
*/
ResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request);
}