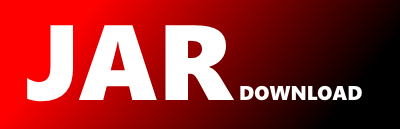
com.amazonaws.services.finspace.AWSfinspaceClient Maven / Gradle / Ivy
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.finspace;
import org.w3c.dom.*;
import java.net.*;
import java.util.*;
import javax.annotation.Generated;
import org.apache.commons.logging.*;
import com.amazonaws.*;
import com.amazonaws.annotation.SdkInternalApi;
import com.amazonaws.auth.*;
import com.amazonaws.handlers.*;
import com.amazonaws.http.*;
import com.amazonaws.internal.*;
import com.amazonaws.internal.auth.*;
import com.amazonaws.metrics.*;
import com.amazonaws.regions.*;
import com.amazonaws.transform.*;
import com.amazonaws.util.*;
import com.amazonaws.protocol.json.*;
import com.amazonaws.util.AWSRequestMetrics.Field;
import com.amazonaws.annotation.ThreadSafe;
import com.amazonaws.client.AwsSyncClientParams;
import com.amazonaws.client.builder.AdvancedConfig;
import com.amazonaws.services.finspace.AWSfinspaceClientBuilder;
import com.amazonaws.AmazonServiceException;
import com.amazonaws.services.finspace.model.*;
import com.amazonaws.services.finspace.model.transform.*;
/**
* Client for accessing finspace. All service calls made using this client are blocking, and will not return until the
* service call completes.
*
*
* The FinSpace management service provides the APIs for managing FinSpace environments.
*
*/
@ThreadSafe
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class AWSfinspaceClient extends AmazonWebServiceClient implements AWSfinspace {
/** Provider for AWS credentials. */
private final AWSCredentialsProvider awsCredentialsProvider;
private static final Log log = LogFactory.getLog(AWSfinspace.class);
/** Default signing name for the service. */
private static final String DEFAULT_SIGNING_NAME = "finspace";
/** Client configuration factory providing ClientConfigurations tailored to this client */
protected static final ClientConfigurationFactory configFactory = new ClientConfigurationFactory();
private final AdvancedConfig advancedConfig;
private static final com.amazonaws.protocol.json.SdkJsonProtocolFactory protocolFactory = new com.amazonaws.protocol.json.SdkJsonProtocolFactory(
new JsonClientMetadata()
.withProtocolVersion("1.1")
.withSupportsCbor(false)
.withSupportsIon(false)
.withContentTypeOverride("application/json")
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InvalidRequestException").withExceptionUnmarshaller(
com.amazonaws.services.finspace.model.transform.InvalidRequestExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ThrottlingException").withExceptionUnmarshaller(
com.amazonaws.services.finspace.model.transform.ThrottlingExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ServiceQuotaExceededException").withExceptionUnmarshaller(
com.amazonaws.services.finspace.model.transform.ServiceQuotaExceededExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InternalServerException").withExceptionUnmarshaller(
com.amazonaws.services.finspace.model.transform.InternalServerExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("LimitExceededException").withExceptionUnmarshaller(
com.amazonaws.services.finspace.model.transform.LimitExceededExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("AccessDeniedException").withExceptionUnmarshaller(
com.amazonaws.services.finspace.model.transform.AccessDeniedExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ConflictException").withExceptionUnmarshaller(
com.amazonaws.services.finspace.model.transform.ConflictExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ResourceNotFoundException").withExceptionUnmarshaller(
com.amazonaws.services.finspace.model.transform.ResourceNotFoundExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ValidationException").withExceptionUnmarshaller(
com.amazonaws.services.finspace.model.transform.ValidationExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ResourceAlreadyExistsException").withExceptionUnmarshaller(
com.amazonaws.services.finspace.model.transform.ResourceAlreadyExistsExceptionUnmarshaller.getInstance()))
.withBaseServiceExceptionClass(com.amazonaws.services.finspace.model.AWSfinspaceException.class));
public static AWSfinspaceClientBuilder builder() {
return AWSfinspaceClientBuilder.standard();
}
/**
* Constructs a new client to invoke service methods on finspace using the specified parameters.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param clientParams
* Object providing client parameters.
*/
AWSfinspaceClient(AwsSyncClientParams clientParams) {
this(clientParams, false);
}
/**
* Constructs a new client to invoke service methods on finspace using the specified parameters.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param clientParams
* Object providing client parameters.
*/
AWSfinspaceClient(AwsSyncClientParams clientParams, boolean endpointDiscoveryEnabled) {
super(clientParams);
this.awsCredentialsProvider = clientParams.getCredentialsProvider();
this.advancedConfig = clientParams.getAdvancedConfig();
init();
}
private void init() {
setServiceNameIntern(DEFAULT_SIGNING_NAME);
setEndpointPrefix(ENDPOINT_PREFIX);
// calling this.setEndPoint(...) will also modify the signer accordingly
setEndpoint("finspace.us-east-1.amazonaws.com");
HandlerChainFactory chainFactory = new HandlerChainFactory();
requestHandler2s.addAll(chainFactory.newRequestHandlerChain("/com/amazonaws/services/finspace/request.handlers"));
requestHandler2s.addAll(chainFactory.newRequestHandler2Chain("/com/amazonaws/services/finspace/request.handler2s"));
requestHandler2s.addAll(chainFactory.getGlobalHandlers());
}
/**
*
* Create a new FinSpace environment.
*
*
* @param createEnvironmentRequest
* @return Result of the CreateEnvironment operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ServiceQuotaExceededException
* You have exceeded your service quota. To perform the requested action, remove some of the relevant
* resources, or use Service Quotas to request a service quota increase.
* @throws LimitExceededException
* A service limit or quota is exceeded.
* @sample AWSfinspace.CreateEnvironment
* @see AWS API
* Documentation
*/
@Override
@Deprecated
public CreateEnvironmentResult createEnvironment(CreateEnvironmentRequest request) {
request = beforeClientExecution(request);
return executeCreateEnvironment(request);
}
@SdkInternalApi
final CreateEnvironmentResult executeCreateEnvironment(CreateEnvironmentRequest createEnvironmentRequest) {
ExecutionContext executionContext = createExecutionContext(createEnvironmentRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateEnvironmentRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createEnvironmentRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "finspace");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateEnvironment");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateEnvironmentResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a changeset for a kdb database. A changeset allows you to add and delete existing files by using an
* ordered list of change requests.
*
*
* @param createKxChangesetRequest
* @return Result of the CreateKxChangeset operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws ConflictException
* There was a conflict with this action, and it could not be completed.
* @throws LimitExceededException
* A service limit or quota is exceeded.
* @sample AWSfinspace.CreateKxChangeset
* @see AWS API
* Documentation
*/
@Override
public CreateKxChangesetResult createKxChangeset(CreateKxChangesetRequest request) {
request = beforeClientExecution(request);
return executeCreateKxChangeset(request);
}
@SdkInternalApi
final CreateKxChangesetResult executeCreateKxChangeset(CreateKxChangesetRequest createKxChangesetRequest) {
ExecutionContext executionContext = createExecutionContext(createKxChangesetRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateKxChangesetRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createKxChangesetRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "finspace");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateKxChangeset");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateKxChangesetResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a new kdb cluster.
*
*
* @param createKxClusterRequest
* @return Result of the CreateKxCluster operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws LimitExceededException
* A service limit or quota is exceeded.
* @throws ConflictException
* There was a conflict with this action, and it could not be completed.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @sample AWSfinspace.CreateKxCluster
* @see AWS API
* Documentation
*/
@Override
public CreateKxClusterResult createKxCluster(CreateKxClusterRequest request) {
request = beforeClientExecution(request);
return executeCreateKxCluster(request);
}
@SdkInternalApi
final CreateKxClusterResult executeCreateKxCluster(CreateKxClusterRequest createKxClusterRequest) {
ExecutionContext executionContext = createExecutionContext(createKxClusterRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateKxClusterRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createKxClusterRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "finspace");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateKxCluster");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateKxClusterResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a new kdb database in the environment.
*
*
* @param createKxDatabaseRequest
* @return Result of the CreateKxDatabase operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ConflictException
* There was a conflict with this action, and it could not be completed.
* @throws ResourceAlreadyExistsException
* The specified resource group already exists.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws LimitExceededException
* A service limit or quota is exceeded.
* @sample AWSfinspace.CreateKxDatabase
* @see AWS API
* Documentation
*/
@Override
public CreateKxDatabaseResult createKxDatabase(CreateKxDatabaseRequest request) {
request = beforeClientExecution(request);
return executeCreateKxDatabase(request);
}
@SdkInternalApi
final CreateKxDatabaseResult executeCreateKxDatabase(CreateKxDatabaseRequest createKxDatabaseRequest) {
ExecutionContext executionContext = createExecutionContext(createKxDatabaseRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateKxDatabaseRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createKxDatabaseRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "finspace");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateKxDatabase");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateKxDatabaseResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a snapshot of kdb database with tiered storage capabilities and a pre-warmed cache, ready for mounting on
* kdb clusters. Dataviews are only available for clusters running on a scaling group. They are not supported on
* dedicated clusters.
*
*
* @param createKxDataviewRequest
* @return Result of the CreateKxDataview operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws ConflictException
* There was a conflict with this action, and it could not be completed.
* @throws LimitExceededException
* A service limit or quota is exceeded.
* @throws ResourceAlreadyExistsException
* The specified resource group already exists.
* @sample AWSfinspace.CreateKxDataview
* @see AWS API
* Documentation
*/
@Override
public CreateKxDataviewResult createKxDataview(CreateKxDataviewRequest request) {
request = beforeClientExecution(request);
return executeCreateKxDataview(request);
}
@SdkInternalApi
final CreateKxDataviewResult executeCreateKxDataview(CreateKxDataviewRequest createKxDataviewRequest) {
ExecutionContext executionContext = createExecutionContext(createKxDataviewRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateKxDataviewRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createKxDataviewRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "finspace");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateKxDataview");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateKxDataviewResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a managed kdb environment for the account.
*
*
* @param createKxEnvironmentRequest
* @return Result of the CreateKxEnvironment operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ServiceQuotaExceededException
* You have exceeded your service quota. To perform the requested action, remove some of the relevant
* resources, or use Service Quotas to request a service quota increase.
* @throws LimitExceededException
* A service limit or quota is exceeded.
* @throws ConflictException
* There was a conflict with this action, and it could not be completed.
* @sample AWSfinspace.CreateKxEnvironment
* @see AWS
* API Documentation
*/
@Override
public CreateKxEnvironmentResult createKxEnvironment(CreateKxEnvironmentRequest request) {
request = beforeClientExecution(request);
return executeCreateKxEnvironment(request);
}
@SdkInternalApi
final CreateKxEnvironmentResult executeCreateKxEnvironment(CreateKxEnvironmentRequest createKxEnvironmentRequest) {
ExecutionContext executionContext = createExecutionContext(createKxEnvironmentRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateKxEnvironmentRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createKxEnvironmentRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "finspace");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateKxEnvironment");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateKxEnvironmentResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a new scaling group.
*
*
* @param createKxScalingGroupRequest
* @return Result of the CreateKxScalingGroup operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws LimitExceededException
* A service limit or quota is exceeded.
* @throws ConflictException
* There was a conflict with this action, and it could not be completed.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @sample AWSfinspace.CreateKxScalingGroup
* @see AWS
* API Documentation
*/
@Override
public CreateKxScalingGroupResult createKxScalingGroup(CreateKxScalingGroupRequest request) {
request = beforeClientExecution(request);
return executeCreateKxScalingGroup(request);
}
@SdkInternalApi
final CreateKxScalingGroupResult executeCreateKxScalingGroup(CreateKxScalingGroupRequest createKxScalingGroupRequest) {
ExecutionContext executionContext = createExecutionContext(createKxScalingGroupRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateKxScalingGroupRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createKxScalingGroupRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "finspace");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateKxScalingGroup");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateKxScalingGroupResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a user in FinSpace kdb environment with an associated IAM role.
*
*
* @param createKxUserRequest
* @return Result of the CreateKxUser operation returned by the service.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws ResourceAlreadyExistsException
* The specified resource group already exists.
* @throws LimitExceededException
* A service limit or quota is exceeded.
* @throws ConflictException
* There was a conflict with this action, and it could not be completed.
* @sample AWSfinspace.CreateKxUser
* @see AWS API
* Documentation
*/
@Override
public CreateKxUserResult createKxUser(CreateKxUserRequest request) {
request = beforeClientExecution(request);
return executeCreateKxUser(request);
}
@SdkInternalApi
final CreateKxUserResult executeCreateKxUser(CreateKxUserRequest createKxUserRequest) {
ExecutionContext executionContext = createExecutionContext(createKxUserRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateKxUserRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createKxUserRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "finspace");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateKxUser");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateKxUserResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a new volume with a specific amount of throughput and storage capacity.
*
*
* @param createKxVolumeRequest
* @return Result of the CreateKxVolume operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws LimitExceededException
* A service limit or quota is exceeded.
* @throws ConflictException
* There was a conflict with this action, and it could not be completed.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws ResourceAlreadyExistsException
* The specified resource group already exists.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @sample AWSfinspace.CreateKxVolume
* @see AWS API
* Documentation
*/
@Override
public CreateKxVolumeResult createKxVolume(CreateKxVolumeRequest request) {
request = beforeClientExecution(request);
return executeCreateKxVolume(request);
}
@SdkInternalApi
final CreateKxVolumeResult executeCreateKxVolume(CreateKxVolumeRequest createKxVolumeRequest) {
ExecutionContext executionContext = createExecutionContext(createKxVolumeRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateKxVolumeRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createKxVolumeRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "finspace");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateKxVolume");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateKxVolumeResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Delete an FinSpace environment.
*
*
* @param deleteEnvironmentRequest
* @return Result of the DeleteEnvironment operation returned by the service.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @sample AWSfinspace.DeleteEnvironment
* @see AWS API
* Documentation
*/
@Override
@Deprecated
public DeleteEnvironmentResult deleteEnvironment(DeleteEnvironmentRequest request) {
request = beforeClientExecution(request);
return executeDeleteEnvironment(request);
}
@SdkInternalApi
final DeleteEnvironmentResult executeDeleteEnvironment(DeleteEnvironmentRequest deleteEnvironmentRequest) {
ExecutionContext executionContext = createExecutionContext(deleteEnvironmentRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteEnvironmentRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteEnvironmentRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "finspace");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteEnvironment");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteEnvironmentResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes a kdb cluster.
*
*
* @param deleteKxClusterRequest
* @return Result of the DeleteKxCluster operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws LimitExceededException
* A service limit or quota is exceeded.
* @throws ConflictException
* There was a conflict with this action, and it could not be completed.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @sample AWSfinspace.DeleteKxCluster
* @see AWS API
* Documentation
*/
@Override
public DeleteKxClusterResult deleteKxCluster(DeleteKxClusterRequest request) {
request = beforeClientExecution(request);
return executeDeleteKxCluster(request);
}
@SdkInternalApi
final DeleteKxClusterResult executeDeleteKxCluster(DeleteKxClusterRequest deleteKxClusterRequest) {
ExecutionContext executionContext = createExecutionContext(deleteKxClusterRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteKxClusterRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteKxClusterRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "finspace");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteKxCluster");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteKxClusterResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the specified nodes from a cluster.
*
*
* @param deleteKxClusterNodeRequest
* @return Result of the DeleteKxClusterNode operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @sample AWSfinspace.DeleteKxClusterNode
* @see AWS
* API Documentation
*/
@Override
public DeleteKxClusterNodeResult deleteKxClusterNode(DeleteKxClusterNodeRequest request) {
request = beforeClientExecution(request);
return executeDeleteKxClusterNode(request);
}
@SdkInternalApi
final DeleteKxClusterNodeResult executeDeleteKxClusterNode(DeleteKxClusterNodeRequest deleteKxClusterNodeRequest) {
ExecutionContext executionContext = createExecutionContext(deleteKxClusterNodeRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteKxClusterNodeRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteKxClusterNodeRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "finspace");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteKxClusterNode");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteKxClusterNodeResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the specified database and all of its associated data. This action is irreversible. You must copy any
* data out of the database before deleting it if the data is to be retained.
*
*
* @param deleteKxDatabaseRequest
* @return Result of the DeleteKxDatabase operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws ConflictException
* There was a conflict with this action, and it could not be completed.
* @sample AWSfinspace.DeleteKxDatabase
* @see AWS API
* Documentation
*/
@Override
public DeleteKxDatabaseResult deleteKxDatabase(DeleteKxDatabaseRequest request) {
request = beforeClientExecution(request);
return executeDeleteKxDatabase(request);
}
@SdkInternalApi
final DeleteKxDatabaseResult executeDeleteKxDatabase(DeleteKxDatabaseRequest deleteKxDatabaseRequest) {
ExecutionContext executionContext = createExecutionContext(deleteKxDatabaseRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteKxDatabaseRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteKxDatabaseRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "finspace");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteKxDatabase");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteKxDatabaseResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the specified dataview. Before deleting a dataview, make sure that it is not in use by any cluster.
*
*
* @param deleteKxDataviewRequest
* @return Result of the DeleteKxDataview operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws ConflictException
* There was a conflict with this action, and it could not be completed.
* @sample AWSfinspace.DeleteKxDataview
* @see AWS API
* Documentation
*/
@Override
public DeleteKxDataviewResult deleteKxDataview(DeleteKxDataviewRequest request) {
request = beforeClientExecution(request);
return executeDeleteKxDataview(request);
}
@SdkInternalApi
final DeleteKxDataviewResult executeDeleteKxDataview(DeleteKxDataviewRequest deleteKxDataviewRequest) {
ExecutionContext executionContext = createExecutionContext(deleteKxDataviewRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteKxDataviewRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteKxDataviewRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "finspace");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteKxDataview");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteKxDataviewResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the kdb environment. This action is irreversible. Deleting a kdb environment will remove all the
* associated data and any services running in it.
*
*
* @param deleteKxEnvironmentRequest
* @return Result of the DeleteKxEnvironment operation returned by the service.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws ConflictException
* There was a conflict with this action, and it could not be completed.
* @sample AWSfinspace.DeleteKxEnvironment
* @see AWS
* API Documentation
*/
@Override
public DeleteKxEnvironmentResult deleteKxEnvironment(DeleteKxEnvironmentRequest request) {
request = beforeClientExecution(request);
return executeDeleteKxEnvironment(request);
}
@SdkInternalApi
final DeleteKxEnvironmentResult executeDeleteKxEnvironment(DeleteKxEnvironmentRequest deleteKxEnvironmentRequest) {
ExecutionContext executionContext = createExecutionContext(deleteKxEnvironmentRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteKxEnvironmentRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteKxEnvironmentRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "finspace");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteKxEnvironment");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteKxEnvironmentResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the specified scaling group. This action is irreversible. You cannot delete a scaling group until all the
* clusters running on it have been deleted.
*
*
* @param deleteKxScalingGroupRequest
* @return Result of the DeleteKxScalingGroup operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws LimitExceededException
* A service limit or quota is exceeded.
* @throws ConflictException
* There was a conflict with this action, and it could not be completed.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @sample AWSfinspace.DeleteKxScalingGroup
* @see AWS
* API Documentation
*/
@Override
public DeleteKxScalingGroupResult deleteKxScalingGroup(DeleteKxScalingGroupRequest request) {
request = beforeClientExecution(request);
return executeDeleteKxScalingGroup(request);
}
@SdkInternalApi
final DeleteKxScalingGroupResult executeDeleteKxScalingGroup(DeleteKxScalingGroupRequest deleteKxScalingGroupRequest) {
ExecutionContext executionContext = createExecutionContext(deleteKxScalingGroupRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteKxScalingGroupRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteKxScalingGroupRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "finspace");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteKxScalingGroup");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteKxScalingGroupResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes a user in the specified kdb environment.
*
*
* @param deleteKxUserRequest
* @return Result of the DeleteKxUser operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws ConflictException
* There was a conflict with this action, and it could not be completed.
* @sample AWSfinspace.DeleteKxUser
* @see AWS API
* Documentation
*/
@Override
public DeleteKxUserResult deleteKxUser(DeleteKxUserRequest request) {
request = beforeClientExecution(request);
return executeDeleteKxUser(request);
}
@SdkInternalApi
final DeleteKxUserResult executeDeleteKxUser(DeleteKxUserRequest deleteKxUserRequest) {
ExecutionContext executionContext = createExecutionContext(deleteKxUserRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteKxUserRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteKxUserRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "finspace");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteKxUser");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteKxUserResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes a volume. You can only delete a volume if it's not attached to a cluster or a dataview. When a volume is
* deleted, any data on the volume is lost. This action is irreversible.
*
*
* @param deleteKxVolumeRequest
* @return Result of the DeleteKxVolume operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws LimitExceededException
* A service limit or quota is exceeded.
* @throws ConflictException
* There was a conflict with this action, and it could not be completed.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @sample AWSfinspace.DeleteKxVolume
* @see AWS API
* Documentation
*/
@Override
public DeleteKxVolumeResult deleteKxVolume(DeleteKxVolumeRequest request) {
request = beforeClientExecution(request);
return executeDeleteKxVolume(request);
}
@SdkInternalApi
final DeleteKxVolumeResult executeDeleteKxVolume(DeleteKxVolumeRequest deleteKxVolumeRequest) {
ExecutionContext executionContext = createExecutionContext(deleteKxVolumeRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteKxVolumeRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteKxVolumeRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "finspace");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteKxVolume");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteKxVolumeResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns the FinSpace environment object.
*
*
* @param getEnvironmentRequest
* @return Result of the GetEnvironment operation returned by the service.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @sample AWSfinspace.GetEnvironment
* @see AWS API
* Documentation
*/
@Override
@Deprecated
public GetEnvironmentResult getEnvironment(GetEnvironmentRequest request) {
request = beforeClientExecution(request);
return executeGetEnvironment(request);
}
@SdkInternalApi
final GetEnvironmentResult executeGetEnvironment(GetEnvironmentRequest getEnvironmentRequest) {
ExecutionContext executionContext = createExecutionContext(getEnvironmentRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetEnvironmentRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getEnvironmentRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "finspace");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetEnvironment");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetEnvironmentResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns information about a kdb changeset.
*
*
* @param getKxChangesetRequest
* @return Result of the GetKxChangeset operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @sample AWSfinspace.GetKxChangeset
* @see AWS API
* Documentation
*/
@Override
public GetKxChangesetResult getKxChangeset(GetKxChangesetRequest request) {
request = beforeClientExecution(request);
return executeGetKxChangeset(request);
}
@SdkInternalApi
final GetKxChangesetResult executeGetKxChangeset(GetKxChangesetRequest getKxChangesetRequest) {
ExecutionContext executionContext = createExecutionContext(getKxChangesetRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetKxChangesetRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getKxChangesetRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "finspace");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetKxChangeset");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetKxChangesetResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves information about a kdb cluster.
*
*
* @param getKxClusterRequest
* @return Result of the GetKxCluster operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws LimitExceededException
* A service limit or quota is exceeded.
* @throws ConflictException
* There was a conflict with this action, and it could not be completed.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @sample AWSfinspace.GetKxCluster
* @see AWS API
* Documentation
*/
@Override
public GetKxClusterResult getKxCluster(GetKxClusterRequest request) {
request = beforeClientExecution(request);
return executeGetKxCluster(request);
}
@SdkInternalApi
final GetKxClusterResult executeGetKxCluster(GetKxClusterRequest getKxClusterRequest) {
ExecutionContext executionContext = createExecutionContext(getKxClusterRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetKxClusterRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getKxClusterRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "finspace");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetKxCluster");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetKxClusterResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves a connection string for a user to connect to a kdb cluster. You must call this API using the same role
* that you have defined while creating a user.
*
*
* @param getKxConnectionStringRequest
* @return Result of the GetKxConnectionString operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @sample AWSfinspace.GetKxConnectionString
* @see AWS
* API Documentation
*/
@Override
public GetKxConnectionStringResult getKxConnectionString(GetKxConnectionStringRequest request) {
request = beforeClientExecution(request);
return executeGetKxConnectionString(request);
}
@SdkInternalApi
final GetKxConnectionStringResult executeGetKxConnectionString(GetKxConnectionStringRequest getKxConnectionStringRequest) {
ExecutionContext executionContext = createExecutionContext(getKxConnectionStringRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetKxConnectionStringRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getKxConnectionStringRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "finspace");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetKxConnectionString");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new GetKxConnectionStringResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns database information for the specified environment ID.
*
*
* @param getKxDatabaseRequest
* @return Result of the GetKxDatabase operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @sample AWSfinspace.GetKxDatabase
* @see AWS API
* Documentation
*/
@Override
public GetKxDatabaseResult getKxDatabase(GetKxDatabaseRequest request) {
request = beforeClientExecution(request);
return executeGetKxDatabase(request);
}
@SdkInternalApi
final GetKxDatabaseResult executeGetKxDatabase(GetKxDatabaseRequest getKxDatabaseRequest) {
ExecutionContext executionContext = createExecutionContext(getKxDatabaseRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetKxDatabaseRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getKxDatabaseRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "finspace");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetKxDatabase");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetKxDatabaseResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves details of the dataview.
*
*
* @param getKxDataviewRequest
* @return Result of the GetKxDataview operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @sample AWSfinspace.GetKxDataview
* @see AWS API
* Documentation
*/
@Override
public GetKxDataviewResult getKxDataview(GetKxDataviewRequest request) {
request = beforeClientExecution(request);
return executeGetKxDataview(request);
}
@SdkInternalApi
final GetKxDataviewResult executeGetKxDataview(GetKxDataviewRequest getKxDataviewRequest) {
ExecutionContext executionContext = createExecutionContext(getKxDataviewRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetKxDataviewRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getKxDataviewRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "finspace");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetKxDataview");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetKxDataviewResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves all the information for the specified kdb environment.
*
*
* @param getKxEnvironmentRequest
* @return Result of the GetKxEnvironment operation returned by the service.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ConflictException
* There was a conflict with this action, and it could not be completed.
* @sample AWSfinspace.GetKxEnvironment
* @see AWS API
* Documentation
*/
@Override
public GetKxEnvironmentResult getKxEnvironment(GetKxEnvironmentRequest request) {
request = beforeClientExecution(request);
return executeGetKxEnvironment(request);
}
@SdkInternalApi
final GetKxEnvironmentResult executeGetKxEnvironment(GetKxEnvironmentRequest getKxEnvironmentRequest) {
ExecutionContext executionContext = createExecutionContext(getKxEnvironmentRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetKxEnvironmentRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getKxEnvironmentRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "finspace");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetKxEnvironment");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetKxEnvironmentResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves details of a scaling group.
*
*
* @param getKxScalingGroupRequest
* @return Result of the GetKxScalingGroup operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws LimitExceededException
* A service limit or quota is exceeded.
* @throws ConflictException
* There was a conflict with this action, and it could not be completed.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @sample AWSfinspace.GetKxScalingGroup
* @see AWS API
* Documentation
*/
@Override
public GetKxScalingGroupResult getKxScalingGroup(GetKxScalingGroupRequest request) {
request = beforeClientExecution(request);
return executeGetKxScalingGroup(request);
}
@SdkInternalApi
final GetKxScalingGroupResult executeGetKxScalingGroup(GetKxScalingGroupRequest getKxScalingGroupRequest) {
ExecutionContext executionContext = createExecutionContext(getKxScalingGroupRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetKxScalingGroupRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getKxScalingGroupRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "finspace");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetKxScalingGroup");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetKxScalingGroupResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves information about the specified kdb user.
*
*
* @param getKxUserRequest
* @return Result of the GetKxUser operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @sample AWSfinspace.GetKxUser
* @see AWS API
* Documentation
*/
@Override
public GetKxUserResult getKxUser(GetKxUserRequest request) {
request = beforeClientExecution(request);
return executeGetKxUser(request);
}
@SdkInternalApi
final GetKxUserResult executeGetKxUser(GetKxUserRequest getKxUserRequest) {
ExecutionContext executionContext = createExecutionContext(getKxUserRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetKxUserRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getKxUserRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "finspace");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetKxUser");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetKxUserResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves the information about the volume.
*
*
* @param getKxVolumeRequest
* @return Result of the GetKxVolume operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws LimitExceededException
* A service limit or quota is exceeded.
* @throws ConflictException
* There was a conflict with this action, and it could not be completed.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @sample AWSfinspace.GetKxVolume
* @see AWS API
* Documentation
*/
@Override
public GetKxVolumeResult getKxVolume(GetKxVolumeRequest request) {
request = beforeClientExecution(request);
return executeGetKxVolume(request);
}
@SdkInternalApi
final GetKxVolumeResult executeGetKxVolume(GetKxVolumeRequest getKxVolumeRequest) {
ExecutionContext executionContext = createExecutionContext(getKxVolumeRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetKxVolumeRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getKxVolumeRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "finspace");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetKxVolume");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetKxVolumeResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* A list of all of your FinSpace environments.
*
*
* @param listEnvironmentsRequest
* @return Result of the ListEnvironments operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @sample AWSfinspace.ListEnvironments
* @see AWS API
* Documentation
*/
@Override
@Deprecated
public ListEnvironmentsResult listEnvironments(ListEnvironmentsRequest request) {
request = beforeClientExecution(request);
return executeListEnvironments(request);
}
@SdkInternalApi
final ListEnvironmentsResult executeListEnvironments(ListEnvironmentsRequest listEnvironmentsRequest) {
ExecutionContext executionContext = createExecutionContext(listEnvironmentsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListEnvironmentsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listEnvironmentsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "finspace");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListEnvironments");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListEnvironmentsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns a list of all the changesets for a database.
*
*
* @param listKxChangesetsRequest
* @return Result of the ListKxChangesets operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @sample AWSfinspace.ListKxChangesets
* @see AWS API
* Documentation
*/
@Override
public ListKxChangesetsResult listKxChangesets(ListKxChangesetsRequest request) {
request = beforeClientExecution(request);
return executeListKxChangesets(request);
}
@SdkInternalApi
final ListKxChangesetsResult executeListKxChangesets(ListKxChangesetsRequest listKxChangesetsRequest) {
ExecutionContext executionContext = createExecutionContext(listKxChangesetsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListKxChangesetsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listKxChangesetsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "finspace");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListKxChangesets");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListKxChangesetsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists all the nodes in a kdb cluster.
*
*
* @param listKxClusterNodesRequest
* @return Result of the ListKxClusterNodes operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws LimitExceededException
* A service limit or quota is exceeded.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @sample AWSfinspace.ListKxClusterNodes
* @see AWS
* API Documentation
*/
@Override
public ListKxClusterNodesResult listKxClusterNodes(ListKxClusterNodesRequest request) {
request = beforeClientExecution(request);
return executeListKxClusterNodes(request);
}
@SdkInternalApi
final ListKxClusterNodesResult executeListKxClusterNodes(ListKxClusterNodesRequest listKxClusterNodesRequest) {
ExecutionContext executionContext = createExecutionContext(listKxClusterNodesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListKxClusterNodesRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listKxClusterNodesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "finspace");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListKxClusterNodes");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListKxClusterNodesResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns a list of clusters.
*
*
* @param listKxClustersRequest
* @return Result of the ListKxClusters operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws LimitExceededException
* A service limit or quota is exceeded.
* @throws ConflictException
* There was a conflict with this action, and it could not be completed.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @sample AWSfinspace.ListKxClusters
* @see AWS API
* Documentation
*/
@Override
public ListKxClustersResult listKxClusters(ListKxClustersRequest request) {
request = beforeClientExecution(request);
return executeListKxClusters(request);
}
@SdkInternalApi
final ListKxClustersResult executeListKxClusters(ListKxClustersRequest listKxClustersRequest) {
ExecutionContext executionContext = createExecutionContext(listKxClustersRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListKxClustersRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listKxClustersRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "finspace");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListKxClusters");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListKxClustersResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns a list of all the databases in the kdb environment.
*
*
* @param listKxDatabasesRequest
* @return Result of the ListKxDatabases operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @sample AWSfinspace.ListKxDatabases
* @see AWS API
* Documentation
*/
@Override
public ListKxDatabasesResult listKxDatabases(ListKxDatabasesRequest request) {
request = beforeClientExecution(request);
return executeListKxDatabases(request);
}
@SdkInternalApi
final ListKxDatabasesResult executeListKxDatabases(ListKxDatabasesRequest listKxDatabasesRequest) {
ExecutionContext executionContext = createExecutionContext(listKxDatabasesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListKxDatabasesRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listKxDatabasesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "finspace");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListKxDatabases");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListKxDatabasesResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns a list of all the dataviews in the database.
*
*
* @param listKxDataviewsRequest
* @return Result of the ListKxDataviews operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @sample AWSfinspace.ListKxDataviews
* @see AWS API
* Documentation
*/
@Override
public ListKxDataviewsResult listKxDataviews(ListKxDataviewsRequest request) {
request = beforeClientExecution(request);
return executeListKxDataviews(request);
}
@SdkInternalApi
final ListKxDataviewsResult executeListKxDataviews(ListKxDataviewsRequest listKxDataviewsRequest) {
ExecutionContext executionContext = createExecutionContext(listKxDataviewsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListKxDataviewsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listKxDataviewsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "finspace");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListKxDataviews");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListKxDataviewsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns a list of kdb environments created in an account.
*
*
* @param listKxEnvironmentsRequest
* @return Result of the ListKxEnvironments operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @sample AWSfinspace.ListKxEnvironments
* @see AWS
* API Documentation
*/
@Override
public ListKxEnvironmentsResult listKxEnvironments(ListKxEnvironmentsRequest request) {
request = beforeClientExecution(request);
return executeListKxEnvironments(request);
}
@SdkInternalApi
final ListKxEnvironmentsResult executeListKxEnvironments(ListKxEnvironmentsRequest listKxEnvironmentsRequest) {
ExecutionContext executionContext = createExecutionContext(listKxEnvironmentsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListKxEnvironmentsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listKxEnvironmentsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "finspace");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListKxEnvironments");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListKxEnvironmentsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns a list of scaling groups in a kdb environment.
*
*
* @param listKxScalingGroupsRequest
* @return Result of the ListKxScalingGroups operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws LimitExceededException
* A service limit or quota is exceeded.
* @throws ConflictException
* There was a conflict with this action, and it could not be completed.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @sample AWSfinspace.ListKxScalingGroups
* @see AWS
* API Documentation
*/
@Override
public ListKxScalingGroupsResult listKxScalingGroups(ListKxScalingGroupsRequest request) {
request = beforeClientExecution(request);
return executeListKxScalingGroups(request);
}
@SdkInternalApi
final ListKxScalingGroupsResult executeListKxScalingGroups(ListKxScalingGroupsRequest listKxScalingGroupsRequest) {
ExecutionContext executionContext = createExecutionContext(listKxScalingGroupsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListKxScalingGroupsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listKxScalingGroupsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "finspace");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListKxScalingGroups");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListKxScalingGroupsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists all the users in a kdb environment.
*
*
* @param listKxUsersRequest
* @return Result of the ListKxUsers operation returned by the service.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @sample AWSfinspace.ListKxUsers
* @see AWS API
* Documentation
*/
@Override
public ListKxUsersResult listKxUsers(ListKxUsersRequest request) {
request = beforeClientExecution(request);
return executeListKxUsers(request);
}
@SdkInternalApi
final ListKxUsersResult executeListKxUsers(ListKxUsersRequest listKxUsersRequest) {
ExecutionContext executionContext = createExecutionContext(listKxUsersRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListKxUsersRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listKxUsersRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "finspace");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListKxUsers");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListKxUsersResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists all the volumes in a kdb environment.
*
*
* @param listKxVolumesRequest
* @return Result of the ListKxVolumes operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws LimitExceededException
* A service limit or quota is exceeded.
* @throws ConflictException
* There was a conflict with this action, and it could not be completed.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @sample AWSfinspace.ListKxVolumes
* @see AWS API
* Documentation
*/
@Override
public ListKxVolumesResult listKxVolumes(ListKxVolumesRequest request) {
request = beforeClientExecution(request);
return executeListKxVolumes(request);
}
@SdkInternalApi
final ListKxVolumesResult executeListKxVolumes(ListKxVolumesRequest listKxVolumesRequest) {
ExecutionContext executionContext = createExecutionContext(listKxVolumesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListKxVolumesRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listKxVolumesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "finspace");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListKxVolumes");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListKxVolumesResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* A list of all tags for a resource.
*
*
* @param listTagsForResourceRequest
* @return Result of the ListTagsForResource operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws InvalidRequestException
* The request is invalid. Something is wrong with the input to the request.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @sample AWSfinspace.ListTagsForResource
* @see AWS
* API Documentation
*/
@Override
public ListTagsForResourceResult listTagsForResource(ListTagsForResourceRequest request) {
request = beforeClientExecution(request);
return executeListTagsForResource(request);
}
@SdkInternalApi
final ListTagsForResourceResult executeListTagsForResource(ListTagsForResourceRequest listTagsForResourceRequest) {
ExecutionContext executionContext = createExecutionContext(listTagsForResourceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListTagsForResourceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listTagsForResourceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "finspace");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListTagsForResource");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListTagsForResourceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Adds metadata tags to a FinSpace resource.
*
*
* @param tagResourceRequest
* @return Result of the TagResource operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws InvalidRequestException
* The request is invalid. Something is wrong with the input to the request.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @sample AWSfinspace.TagResource
* @see AWS API
* Documentation
*/
@Override
public TagResourceResult tagResource(TagResourceRequest request) {
request = beforeClientExecution(request);
return executeTagResource(request);
}
@SdkInternalApi
final TagResourceResult executeTagResource(TagResourceRequest tagResourceRequest) {
ExecutionContext executionContext = createExecutionContext(tagResourceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new TagResourceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(tagResourceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "finspace");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "TagResource");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new TagResourceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Removes metadata tags from a FinSpace resource.
*
*
* @param untagResourceRequest
* @return Result of the UntagResource operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws InvalidRequestException
* The request is invalid. Something is wrong with the input to the request.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @sample AWSfinspace.UntagResource
* @see AWS API
* Documentation
*/
@Override
public UntagResourceResult untagResource(UntagResourceRequest request) {
request = beforeClientExecution(request);
return executeUntagResource(request);
}
@SdkInternalApi
final UntagResourceResult executeUntagResource(UntagResourceRequest untagResourceRequest) {
ExecutionContext executionContext = createExecutionContext(untagResourceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UntagResourceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(untagResourceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "finspace");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UntagResource");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new UntagResourceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Update your FinSpace environment.
*
*
* @param updateEnvironmentRequest
* @return Result of the UpdateEnvironment operation returned by the service.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @sample AWSfinspace.UpdateEnvironment
* @see AWS API
* Documentation
*/
@Override
@Deprecated
public UpdateEnvironmentResult updateEnvironment(UpdateEnvironmentRequest request) {
request = beforeClientExecution(request);
return executeUpdateEnvironment(request);
}
@SdkInternalApi
final UpdateEnvironmentResult executeUpdateEnvironment(UpdateEnvironmentRequest updateEnvironmentRequest) {
ExecutionContext executionContext = createExecutionContext(updateEnvironmentRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateEnvironmentRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(updateEnvironmentRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "finspace");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateEnvironment");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new UpdateEnvironmentResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Allows you to update code configuration on a running cluster. By using this API you can update the code, the
* initialization script path, and the command line arguments for a specific cluster. The configuration that you
* want to update will override any existing configurations on the cluster.
*
*
* @param updateKxClusterCodeConfigurationRequest
* @return Result of the UpdateKxClusterCodeConfiguration operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws LimitExceededException
* A service limit or quota is exceeded.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws ConflictException
* There was a conflict with this action, and it could not be completed.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @sample AWSfinspace.UpdateKxClusterCodeConfiguration
* @see AWS API Documentation
*/
@Override
public UpdateKxClusterCodeConfigurationResult updateKxClusterCodeConfiguration(UpdateKxClusterCodeConfigurationRequest request) {
request = beforeClientExecution(request);
return executeUpdateKxClusterCodeConfiguration(request);
}
@SdkInternalApi
final UpdateKxClusterCodeConfigurationResult executeUpdateKxClusterCodeConfiguration(
UpdateKxClusterCodeConfigurationRequest updateKxClusterCodeConfigurationRequest) {
ExecutionContext executionContext = createExecutionContext(updateKxClusterCodeConfigurationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateKxClusterCodeConfigurationRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(updateKxClusterCodeConfigurationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "finspace");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateKxClusterCodeConfiguration");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new UpdateKxClusterCodeConfigurationResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates the databases mounted on a kdb cluster, which includes the changesetId
and all the dbPaths
* to be cached. This API does not allow you to change a database name or add a database if you created a cluster
* without one.
*
*
* Using this API you can point a cluster to a different changeset and modify a list of partitions being cached.
*
*
* @param updateKxClusterDatabasesRequest
* @return Result of the UpdateKxClusterDatabases operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws LimitExceededException
* A service limit or quota is exceeded.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws ConflictException
* There was a conflict with this action, and it could not be completed.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @sample AWSfinspace.UpdateKxClusterDatabases
* @see AWS API Documentation
*/
@Override
public UpdateKxClusterDatabasesResult updateKxClusterDatabases(UpdateKxClusterDatabasesRequest request) {
request = beforeClientExecution(request);
return executeUpdateKxClusterDatabases(request);
}
@SdkInternalApi
final UpdateKxClusterDatabasesResult executeUpdateKxClusterDatabases(UpdateKxClusterDatabasesRequest updateKxClusterDatabasesRequest) {
ExecutionContext executionContext = createExecutionContext(updateKxClusterDatabasesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateKxClusterDatabasesRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(updateKxClusterDatabasesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "finspace");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateKxClusterDatabases");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new UpdateKxClusterDatabasesResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates information for the given kdb database.
*
*
* @param updateKxDatabaseRequest
* @return Result of the UpdateKxDatabase operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws ConflictException
* There was a conflict with this action, and it could not be completed.
* @sample AWSfinspace.UpdateKxDatabase
* @see AWS API
* Documentation
*/
@Override
public UpdateKxDatabaseResult updateKxDatabase(UpdateKxDatabaseRequest request) {
request = beforeClientExecution(request);
return executeUpdateKxDatabase(request);
}
@SdkInternalApi
final UpdateKxDatabaseResult executeUpdateKxDatabase(UpdateKxDatabaseRequest updateKxDatabaseRequest) {
ExecutionContext executionContext = createExecutionContext(updateKxDatabaseRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateKxDatabaseRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(updateKxDatabaseRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "finspace");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateKxDatabase");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new UpdateKxDatabaseResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates the specified dataview. The dataviews get automatically updated when any new changesets are ingested.
* Each update of the dataview creates a new version, including changeset details and cache configurations
*
*
* @param updateKxDataviewRequest
* @return Result of the UpdateKxDataview operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws ConflictException
* There was a conflict with this action, and it could not be completed.
* @throws ResourceAlreadyExistsException
* The specified resource group already exists.
* @sample AWSfinspace.UpdateKxDataview
* @see AWS API
* Documentation
*/
@Override
public UpdateKxDataviewResult updateKxDataview(UpdateKxDataviewRequest request) {
request = beforeClientExecution(request);
return executeUpdateKxDataview(request);
}
@SdkInternalApi
final UpdateKxDataviewResult executeUpdateKxDataview(UpdateKxDataviewRequest updateKxDataviewRequest) {
ExecutionContext executionContext = createExecutionContext(updateKxDataviewRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateKxDataviewRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(updateKxDataviewRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "finspace");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateKxDataview");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new UpdateKxDataviewResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates information for the given kdb environment.
*
*
* @param updateKxEnvironmentRequest
* @return Result of the UpdateKxEnvironment operation returned by the service.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws ConflictException
* There was a conflict with this action, and it could not be completed.
* @sample AWSfinspace.UpdateKxEnvironment
* @see AWS
* API Documentation
*/
@Override
public UpdateKxEnvironmentResult updateKxEnvironment(UpdateKxEnvironmentRequest request) {
request = beforeClientExecution(request);
return executeUpdateKxEnvironment(request);
}
@SdkInternalApi
final UpdateKxEnvironmentResult executeUpdateKxEnvironment(UpdateKxEnvironmentRequest updateKxEnvironmentRequest) {
ExecutionContext executionContext = createExecutionContext(updateKxEnvironmentRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateKxEnvironmentRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(updateKxEnvironmentRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "finspace");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateKxEnvironment");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new UpdateKxEnvironmentResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates environment network to connect to your internal network by using a transit gateway. This API supports
* request to create a transit gateway attachment from FinSpace VPC to your transit gateway ID and create a custom
* Route-53 outbound resolvers.
*
*
* Once you send a request to update a network, you cannot change it again. Network update might require termination
* of any clusters that are running in the existing network.
*
*
* @param updateKxEnvironmentNetworkRequest
* @return Result of the UpdateKxEnvironmentNetwork operation returned by the service.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws ConflictException
* There was a conflict with this action, and it could not be completed.
* @sample AWSfinspace.UpdateKxEnvironmentNetwork
* @see AWS API Documentation
*/
@Override
public UpdateKxEnvironmentNetworkResult updateKxEnvironmentNetwork(UpdateKxEnvironmentNetworkRequest request) {
request = beforeClientExecution(request);
return executeUpdateKxEnvironmentNetwork(request);
}
@SdkInternalApi
final UpdateKxEnvironmentNetworkResult executeUpdateKxEnvironmentNetwork(UpdateKxEnvironmentNetworkRequest updateKxEnvironmentNetworkRequest) {
ExecutionContext executionContext = createExecutionContext(updateKxEnvironmentNetworkRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateKxEnvironmentNetworkRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(updateKxEnvironmentNetworkRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "finspace");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateKxEnvironmentNetwork");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new UpdateKxEnvironmentNetworkResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates the user details. You can only update the IAM role associated with a user.
*
*
* @param updateKxUserRequest
* @return Result of the UpdateKxUser operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws LimitExceededException
* A service limit or quota is exceeded.
* @throws ConflictException
* There was a conflict with this action, and it could not be completed.
* @sample AWSfinspace.UpdateKxUser
* @see AWS API
* Documentation
*/
@Override
public UpdateKxUserResult updateKxUser(UpdateKxUserRequest request) {
request = beforeClientExecution(request);
return executeUpdateKxUser(request);
}
@SdkInternalApi
final UpdateKxUserResult executeUpdateKxUser(UpdateKxUserRequest updateKxUserRequest) {
ExecutionContext executionContext = createExecutionContext(updateKxUserRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateKxUserRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(updateKxUserRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "finspace");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateKxUser");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new UpdateKxUserResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates the throughput or capacity of a volume. During the update process, the filesystem might be unavailable
* for a few minutes. You can retry any operations after the update is complete.
*
*
* @param updateKxVolumeRequest
* @return Result of the UpdateKxVolume operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws LimitExceededException
* A service limit or quota is exceeded.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws ConflictException
* There was a conflict with this action, and it could not be completed.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @sample AWSfinspace.UpdateKxVolume
* @see AWS API
* Documentation
*/
@Override
public UpdateKxVolumeResult updateKxVolume(UpdateKxVolumeRequest request) {
request = beforeClientExecution(request);
return executeUpdateKxVolume(request);
}
@SdkInternalApi
final UpdateKxVolumeResult executeUpdateKxVolume(UpdateKxVolumeRequest updateKxVolumeRequest) {
ExecutionContext executionContext = createExecutionContext(updateKxVolumeRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateKxVolumeRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(updateKxVolumeRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "finspace");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateKxVolume");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new UpdateKxVolumeResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
* Returns additional metadata for a previously executed successful, request, typically used for debugging issues
* where a service isn't acting as expected. This data isn't considered part of the result data returned by an
* operation, so it's available through this separate, diagnostic interface.
*
* Response metadata is only cached for a limited period of time, so if you need to access this extra diagnostic
* information for an executed request, you should use this method to retrieve it as soon as possible after
* executing the request.
*
* @param request
* The originally executed request
*
* @return The response metadata for the specified request, or null if none is available.
*/
public ResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request) {
return client.getResponseMetadataForRequest(request);
}
/**
* Normal invoke with authentication. Credentials are required and may be overriden at the request level.
**/
private Response invoke(Request request, HttpResponseHandler> responseHandler,
ExecutionContext executionContext) {
return invoke(request, responseHandler, executionContext, null, null);
}
/**
* Normal invoke with authentication. Credentials are required and may be overriden at the request level.
**/
private Response invoke(Request request, HttpResponseHandler> responseHandler,
ExecutionContext executionContext, URI cachedEndpoint, URI uriFromEndpointTrait) {
executionContext.setCredentialsProvider(CredentialUtils.getCredentialsProvider(request.getOriginalRequest(), awsCredentialsProvider));
return doInvoke(request, responseHandler, executionContext, cachedEndpoint, uriFromEndpointTrait);
}
/**
* Invoke with no authentication. Credentials are not required and any credentials set on the client or request will
* be ignored for this operation.
**/
private Response anonymousInvoke(Request request,
HttpResponseHandler> responseHandler, ExecutionContext executionContext) {
return doInvoke(request, responseHandler, executionContext, null, null);
}
/**
* Invoke the request using the http client. Assumes credentials (or lack thereof) have been configured in the
* ExecutionContext beforehand.
**/
private Response doInvoke(Request request, HttpResponseHandler> responseHandler,
ExecutionContext executionContext, URI discoveredEndpoint, URI uriFromEndpointTrait) {
if (discoveredEndpoint != null) {
request.setEndpoint(discoveredEndpoint);
request.getOriginalRequest().getRequestClientOptions().appendUserAgent("endpoint-discovery");
} else if (uriFromEndpointTrait != null) {
request.setEndpoint(uriFromEndpointTrait);
} else {
request.setEndpoint(endpoint);
}
request.setTimeOffset(timeOffset);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler(new JsonErrorResponseMetadata());
return client.execute(request, responseHandler, errorResponseHandler, executionContext);
}
@com.amazonaws.annotation.SdkInternalApi
static com.amazonaws.protocol.json.SdkJsonProtocolFactory getProtocolFactory() {
return protocolFactory;
}
@Override
public void shutdown() {
super.shutdown();
}
}