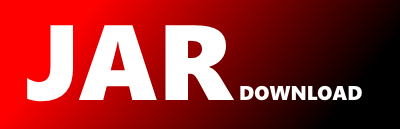
com.amazonaws.services.finspace.model.CreateKxChangesetRequest Maven / Gradle / Ivy
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.finspace.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class CreateKxChangesetRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* A unique identifier of the kdb environment.
*
*/
private String environmentId;
/**
*
* The name of the kdb database.
*
*/
private String databaseName;
/**
*
* A list of change request objects that are run in order. A change request object consists of
* changeType
, s3Path
, and dbPath
. A changeType can have the following
* values:
*
*
* -
*
* PUT – Adds or updates files in a database.
*
*
* -
*
* DELETE – Deletes files in a database.
*
*
*
*
* All the change requests require a mandatory dbPath
attribute that defines the path within the
* database directory. All database paths must start with a leading / and end with a trailing /. The
* s3Path
attribute defines the s3 source file path and is required for a PUT change type. The
* s3path
must end with a trailing / if it is a directory and must end without a trailing / if it is a
* file.
*
*
* Here are few examples of how you can use the change request object:
*
*
* -
*
* This request adds a single sym file at database root location.
*
*
* { "changeType": "PUT", "s3Path":"s3://bucket/db/sym", "dbPath":"/"}
*
*
* -
*
* This request adds files in the given s3Path
under the 2020.01.02 partition of the database.
*
*
* { "changeType": "PUT", "s3Path":"s3://bucket/db/2020.01.02/", "dbPath":"/2020.01.02/"}
*
*
* -
*
* This request adds files in the given s3Path
under the taq table partition of the database.
*
*
* [ { "changeType": "PUT", "s3Path":"s3://bucket/db/2020.01.02/taq/", "dbPath":"/2020.01.02/taq/"}]
*
*
* -
*
* This request deletes the 2020.01.02 partition of the database.
*
*
* [{ "changeType": "DELETE", "dbPath": "/2020.01.02/"} ]
*
*
* -
*
* The DELETE request allows you to delete the existing files under the 2020.01.02 partition of the database,
* and the PUT request adds a new taq table under it.
*
*
* [ {"changeType": "DELETE", "dbPath":"/2020.01.02/"}, {"changeType": "PUT", "s3Path":"s3://bucket/db/2020.01.02/taq/", "dbPath":"/2020.01.02/taq/"}]
*
*
*
*/
private java.util.List changeRequests;
/**
*
* A token that ensures idempotency. This token expires in 10 minutes.
*
*/
private String clientToken;
/**
*
* A unique identifier of the kdb environment.
*
*
* @param environmentId
* A unique identifier of the kdb environment.
*/
public void setEnvironmentId(String environmentId) {
this.environmentId = environmentId;
}
/**
*
* A unique identifier of the kdb environment.
*
*
* @return A unique identifier of the kdb environment.
*/
public String getEnvironmentId() {
return this.environmentId;
}
/**
*
* A unique identifier of the kdb environment.
*
*
* @param environmentId
* A unique identifier of the kdb environment.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateKxChangesetRequest withEnvironmentId(String environmentId) {
setEnvironmentId(environmentId);
return this;
}
/**
*
* The name of the kdb database.
*
*
* @param databaseName
* The name of the kdb database.
*/
public void setDatabaseName(String databaseName) {
this.databaseName = databaseName;
}
/**
*
* The name of the kdb database.
*
*
* @return The name of the kdb database.
*/
public String getDatabaseName() {
return this.databaseName;
}
/**
*
* The name of the kdb database.
*
*
* @param databaseName
* The name of the kdb database.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateKxChangesetRequest withDatabaseName(String databaseName) {
setDatabaseName(databaseName);
return this;
}
/**
*
* A list of change request objects that are run in order. A change request object consists of
* changeType
, s3Path
, and dbPath
. A changeType can have the following
* values:
*
*
* -
*
* PUT – Adds or updates files in a database.
*
*
* -
*
* DELETE – Deletes files in a database.
*
*
*
*
* All the change requests require a mandatory dbPath
attribute that defines the path within the
* database directory. All database paths must start with a leading / and end with a trailing /. The
* s3Path
attribute defines the s3 source file path and is required for a PUT change type. The
* s3path
must end with a trailing / if it is a directory and must end without a trailing / if it is a
* file.
*
*
* Here are few examples of how you can use the change request object:
*
*
* -
*
* This request adds a single sym file at database root location.
*
*
* { "changeType": "PUT", "s3Path":"s3://bucket/db/sym", "dbPath":"/"}
*
*
* -
*
* This request adds files in the given s3Path
under the 2020.01.02 partition of the database.
*
*
* { "changeType": "PUT", "s3Path":"s3://bucket/db/2020.01.02/", "dbPath":"/2020.01.02/"}
*
*
* -
*
* This request adds files in the given s3Path
under the taq table partition of the database.
*
*
* [ { "changeType": "PUT", "s3Path":"s3://bucket/db/2020.01.02/taq/", "dbPath":"/2020.01.02/taq/"}]
*
*
* -
*
* This request deletes the 2020.01.02 partition of the database.
*
*
* [{ "changeType": "DELETE", "dbPath": "/2020.01.02/"} ]
*
*
* -
*
* The DELETE request allows you to delete the existing files under the 2020.01.02 partition of the database,
* and the PUT request adds a new taq table under it.
*
*
* [ {"changeType": "DELETE", "dbPath":"/2020.01.02/"}, {"changeType": "PUT", "s3Path":"s3://bucket/db/2020.01.02/taq/", "dbPath":"/2020.01.02/taq/"}]
*
*
*
*
* @return A list of change request objects that are run in order. A change request object consists of
* changeType
, s3Path
, and dbPath
. A changeType can have the
* following values:
*
* -
*
* PUT – Adds or updates files in a database.
*
*
* -
*
* DELETE – Deletes files in a database.
*
*
*
*
* All the change requests require a mandatory dbPath
attribute that defines the path within
* the database directory. All database paths must start with a leading / and end with a trailing /. The
* s3Path
attribute defines the s3 source file path and is required for a PUT change type. The
* s3path
must end with a trailing / if it is a directory and must end without a trailing / if
* it is a file.
*
*
* Here are few examples of how you can use the change request object:
*
*
* -
*
* This request adds a single sym file at database root location.
*
*
* { "changeType": "PUT", "s3Path":"s3://bucket/db/sym", "dbPath":"/"}
*
*
* -
*
* This request adds files in the given s3Path
under the 2020.01.02 partition of the database.
*
*
* { "changeType": "PUT", "s3Path":"s3://bucket/db/2020.01.02/", "dbPath":"/2020.01.02/"}
*
*
* -
*
* This request adds files in the given s3Path
under the taq table partition of the
* database.
*
*
* [ { "changeType": "PUT", "s3Path":"s3://bucket/db/2020.01.02/taq/", "dbPath":"/2020.01.02/taq/"}]
*
*
* -
*
* This request deletes the 2020.01.02 partition of the database.
*
*
* [{ "changeType": "DELETE", "dbPath": "/2020.01.02/"} ]
*
*
* -
*
* The DELETE request allows you to delete the existing files under the 2020.01.02 partition of the
* database, and the PUT request adds a new taq table under it.
*
*
* [ {"changeType": "DELETE", "dbPath":"/2020.01.02/"}, {"changeType": "PUT", "s3Path":"s3://bucket/db/2020.01.02/taq/", "dbPath":"/2020.01.02/taq/"}]
*
*
*/
public java.util.List getChangeRequests() {
return changeRequests;
}
/**
*
* A list of change request objects that are run in order. A change request object consists of
* changeType
, s3Path
, and dbPath
. A changeType can have the following
* values:
*
*
* -
*
* PUT – Adds or updates files in a database.
*
*
* -
*
* DELETE – Deletes files in a database.
*
*
*
*
* All the change requests require a mandatory dbPath
attribute that defines the path within the
* database directory. All database paths must start with a leading / and end with a trailing /. The
* s3Path
attribute defines the s3 source file path and is required for a PUT change type. The
* s3path
must end with a trailing / if it is a directory and must end without a trailing / if it is a
* file.
*
*
* Here are few examples of how you can use the change request object:
*
*
* -
*
* This request adds a single sym file at database root location.
*
*
* { "changeType": "PUT", "s3Path":"s3://bucket/db/sym", "dbPath":"/"}
*
*
* -
*
* This request adds files in the given s3Path
under the 2020.01.02 partition of the database.
*
*
* { "changeType": "PUT", "s3Path":"s3://bucket/db/2020.01.02/", "dbPath":"/2020.01.02/"}
*
*
* -
*
* This request adds files in the given s3Path
under the taq table partition of the database.
*
*
* [ { "changeType": "PUT", "s3Path":"s3://bucket/db/2020.01.02/taq/", "dbPath":"/2020.01.02/taq/"}]
*
*
* -
*
* This request deletes the 2020.01.02 partition of the database.
*
*
* [{ "changeType": "DELETE", "dbPath": "/2020.01.02/"} ]
*
*
* -
*
* The DELETE request allows you to delete the existing files under the 2020.01.02 partition of the database,
* and the PUT request adds a new taq table under it.
*
*
* [ {"changeType": "DELETE", "dbPath":"/2020.01.02/"}, {"changeType": "PUT", "s3Path":"s3://bucket/db/2020.01.02/taq/", "dbPath":"/2020.01.02/taq/"}]
*
*
*
*
* @param changeRequests
* A list of change request objects that are run in order. A change request object consists of
* changeType
, s3Path
, and dbPath
. A changeType can have the
* following values:
*
* -
*
* PUT – Adds or updates files in a database.
*
*
* -
*
* DELETE – Deletes files in a database.
*
*
*
*
* All the change requests require a mandatory dbPath
attribute that defines the path within the
* database directory. All database paths must start with a leading / and end with a trailing /. The
* s3Path
attribute defines the s3 source file path and is required for a PUT change type. The
* s3path
must end with a trailing / if it is a directory and must end without a trailing / if
* it is a file.
*
*
* Here are few examples of how you can use the change request object:
*
*
* -
*
* This request adds a single sym file at database root location.
*
*
* { "changeType": "PUT", "s3Path":"s3://bucket/db/sym", "dbPath":"/"}
*
*
* -
*
* This request adds files in the given s3Path
under the 2020.01.02 partition of the database.
*
*
* { "changeType": "PUT", "s3Path":"s3://bucket/db/2020.01.02/", "dbPath":"/2020.01.02/"}
*
*
* -
*
* This request adds files in the given s3Path
under the taq table partition of the
* database.
*
*
* [ { "changeType": "PUT", "s3Path":"s3://bucket/db/2020.01.02/taq/", "dbPath":"/2020.01.02/taq/"}]
*
*
* -
*
* This request deletes the 2020.01.02 partition of the database.
*
*
* [{ "changeType": "DELETE", "dbPath": "/2020.01.02/"} ]
*
*
* -
*
* The DELETE request allows you to delete the existing files under the 2020.01.02 partition of the
* database, and the PUT request adds a new taq table under it.
*
*
* [ {"changeType": "DELETE", "dbPath":"/2020.01.02/"}, {"changeType": "PUT", "s3Path":"s3://bucket/db/2020.01.02/taq/", "dbPath":"/2020.01.02/taq/"}]
*
*
*/
public void setChangeRequests(java.util.Collection changeRequests) {
if (changeRequests == null) {
this.changeRequests = null;
return;
}
this.changeRequests = new java.util.ArrayList(changeRequests);
}
/**
*
* A list of change request objects that are run in order. A change request object consists of
* changeType
, s3Path
, and dbPath
. A changeType can have the following
* values:
*
*
* -
*
* PUT – Adds or updates files in a database.
*
*
* -
*
* DELETE – Deletes files in a database.
*
*
*
*
* All the change requests require a mandatory dbPath
attribute that defines the path within the
* database directory. All database paths must start with a leading / and end with a trailing /. The
* s3Path
attribute defines the s3 source file path and is required for a PUT change type. The
* s3path
must end with a trailing / if it is a directory and must end without a trailing / if it is a
* file.
*
*
* Here are few examples of how you can use the change request object:
*
*
* -
*
* This request adds a single sym file at database root location.
*
*
* { "changeType": "PUT", "s3Path":"s3://bucket/db/sym", "dbPath":"/"}
*
*
* -
*
* This request adds files in the given s3Path
under the 2020.01.02 partition of the database.
*
*
* { "changeType": "PUT", "s3Path":"s3://bucket/db/2020.01.02/", "dbPath":"/2020.01.02/"}
*
*
* -
*
* This request adds files in the given s3Path
under the taq table partition of the database.
*
*
* [ { "changeType": "PUT", "s3Path":"s3://bucket/db/2020.01.02/taq/", "dbPath":"/2020.01.02/taq/"}]
*
*
* -
*
* This request deletes the 2020.01.02 partition of the database.
*
*
* [{ "changeType": "DELETE", "dbPath": "/2020.01.02/"} ]
*
*
* -
*
* The DELETE request allows you to delete the existing files under the 2020.01.02 partition of the database,
* and the PUT request adds a new taq table under it.
*
*
* [ {"changeType": "DELETE", "dbPath":"/2020.01.02/"}, {"changeType": "PUT", "s3Path":"s3://bucket/db/2020.01.02/taq/", "dbPath":"/2020.01.02/taq/"}]
*
*
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setChangeRequests(java.util.Collection)} or {@link #withChangeRequests(java.util.Collection)} if you want
* to override the existing values.
*
*
* @param changeRequests
* A list of change request objects that are run in order. A change request object consists of
* changeType
, s3Path
, and dbPath
. A changeType can have the
* following values:
*
* -
*
* PUT – Adds or updates files in a database.
*
*
* -
*
* DELETE – Deletes files in a database.
*
*
*
*
* All the change requests require a mandatory dbPath
attribute that defines the path within the
* database directory. All database paths must start with a leading / and end with a trailing /. The
* s3Path
attribute defines the s3 source file path and is required for a PUT change type. The
* s3path
must end with a trailing / if it is a directory and must end without a trailing / if
* it is a file.
*
*
* Here are few examples of how you can use the change request object:
*
*
* -
*
* This request adds a single sym file at database root location.
*
*
* { "changeType": "PUT", "s3Path":"s3://bucket/db/sym", "dbPath":"/"}
*
*
* -
*
* This request adds files in the given s3Path
under the 2020.01.02 partition of the database.
*
*
* { "changeType": "PUT", "s3Path":"s3://bucket/db/2020.01.02/", "dbPath":"/2020.01.02/"}
*
*
* -
*
* This request adds files in the given s3Path
under the taq table partition of the
* database.
*
*
* [ { "changeType": "PUT", "s3Path":"s3://bucket/db/2020.01.02/taq/", "dbPath":"/2020.01.02/taq/"}]
*
*
* -
*
* This request deletes the 2020.01.02 partition of the database.
*
*
* [{ "changeType": "DELETE", "dbPath": "/2020.01.02/"} ]
*
*
* -
*
* The DELETE request allows you to delete the existing files under the 2020.01.02 partition of the
* database, and the PUT request adds a new taq table under it.
*
*
* [ {"changeType": "DELETE", "dbPath":"/2020.01.02/"}, {"changeType": "PUT", "s3Path":"s3://bucket/db/2020.01.02/taq/", "dbPath":"/2020.01.02/taq/"}]
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateKxChangesetRequest withChangeRequests(ChangeRequest... changeRequests) {
if (this.changeRequests == null) {
setChangeRequests(new java.util.ArrayList(changeRequests.length));
}
for (ChangeRequest ele : changeRequests) {
this.changeRequests.add(ele);
}
return this;
}
/**
*
* A list of change request objects that are run in order. A change request object consists of
* changeType
, s3Path
, and dbPath
. A changeType can have the following
* values:
*
*
* -
*
* PUT – Adds or updates files in a database.
*
*
* -
*
* DELETE – Deletes files in a database.
*
*
*
*
* All the change requests require a mandatory dbPath
attribute that defines the path within the
* database directory. All database paths must start with a leading / and end with a trailing /. The
* s3Path
attribute defines the s3 source file path and is required for a PUT change type. The
* s3path
must end with a trailing / if it is a directory and must end without a trailing / if it is a
* file.
*
*
* Here are few examples of how you can use the change request object:
*
*
* -
*
* This request adds a single sym file at database root location.
*
*
* { "changeType": "PUT", "s3Path":"s3://bucket/db/sym", "dbPath":"/"}
*
*
* -
*
* This request adds files in the given s3Path
under the 2020.01.02 partition of the database.
*
*
* { "changeType": "PUT", "s3Path":"s3://bucket/db/2020.01.02/", "dbPath":"/2020.01.02/"}
*
*
* -
*
* This request adds files in the given s3Path
under the taq table partition of the database.
*
*
* [ { "changeType": "PUT", "s3Path":"s3://bucket/db/2020.01.02/taq/", "dbPath":"/2020.01.02/taq/"}]
*
*
* -
*
* This request deletes the 2020.01.02 partition of the database.
*
*
* [{ "changeType": "DELETE", "dbPath": "/2020.01.02/"} ]
*
*
* -
*
* The DELETE request allows you to delete the existing files under the 2020.01.02 partition of the database,
* and the PUT request adds a new taq table under it.
*
*
* [ {"changeType": "DELETE", "dbPath":"/2020.01.02/"}, {"changeType": "PUT", "s3Path":"s3://bucket/db/2020.01.02/taq/", "dbPath":"/2020.01.02/taq/"}]
*
*
*
*
* @param changeRequests
* A list of change request objects that are run in order. A change request object consists of
* changeType
, s3Path
, and dbPath
. A changeType can have the
* following values:
*
* -
*
* PUT – Adds or updates files in a database.
*
*
* -
*
* DELETE – Deletes files in a database.
*
*
*
*
* All the change requests require a mandatory dbPath
attribute that defines the path within the
* database directory. All database paths must start with a leading / and end with a trailing /. The
* s3Path
attribute defines the s3 source file path and is required for a PUT change type. The
* s3path
must end with a trailing / if it is a directory and must end without a trailing / if
* it is a file.
*
*
* Here are few examples of how you can use the change request object:
*
*
* -
*
* This request adds a single sym file at database root location.
*
*
* { "changeType": "PUT", "s3Path":"s3://bucket/db/sym", "dbPath":"/"}
*
*
* -
*
* This request adds files in the given s3Path
under the 2020.01.02 partition of the database.
*
*
* { "changeType": "PUT", "s3Path":"s3://bucket/db/2020.01.02/", "dbPath":"/2020.01.02/"}
*
*
* -
*
* This request adds files in the given s3Path
under the taq table partition of the
* database.
*
*
* [ { "changeType": "PUT", "s3Path":"s3://bucket/db/2020.01.02/taq/", "dbPath":"/2020.01.02/taq/"}]
*
*
* -
*
* This request deletes the 2020.01.02 partition of the database.
*
*
* [{ "changeType": "DELETE", "dbPath": "/2020.01.02/"} ]
*
*
* -
*
* The DELETE request allows you to delete the existing files under the 2020.01.02 partition of the
* database, and the PUT request adds a new taq table under it.
*
*
* [ {"changeType": "DELETE", "dbPath":"/2020.01.02/"}, {"changeType": "PUT", "s3Path":"s3://bucket/db/2020.01.02/taq/", "dbPath":"/2020.01.02/taq/"}]
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateKxChangesetRequest withChangeRequests(java.util.Collection changeRequests) {
setChangeRequests(changeRequests);
return this;
}
/**
*
* A token that ensures idempotency. This token expires in 10 minutes.
*
*
* @param clientToken
* A token that ensures idempotency. This token expires in 10 minutes.
*/
public void setClientToken(String clientToken) {
this.clientToken = clientToken;
}
/**
*
* A token that ensures idempotency. This token expires in 10 minutes.
*
*
* @return A token that ensures idempotency. This token expires in 10 minutes.
*/
public String getClientToken() {
return this.clientToken;
}
/**
*
* A token that ensures idempotency. This token expires in 10 minutes.
*
*
* @param clientToken
* A token that ensures idempotency. This token expires in 10 minutes.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateKxChangesetRequest withClientToken(String clientToken) {
setClientToken(clientToken);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getEnvironmentId() != null)
sb.append("EnvironmentId: ").append(getEnvironmentId()).append(",");
if (getDatabaseName() != null)
sb.append("DatabaseName: ").append(getDatabaseName()).append(",");
if (getChangeRequests() != null)
sb.append("ChangeRequests: ").append(getChangeRequests()).append(",");
if (getClientToken() != null)
sb.append("ClientToken: ").append(getClientToken());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof CreateKxChangesetRequest == false)
return false;
CreateKxChangesetRequest other = (CreateKxChangesetRequest) obj;
if (other.getEnvironmentId() == null ^ this.getEnvironmentId() == null)
return false;
if (other.getEnvironmentId() != null && other.getEnvironmentId().equals(this.getEnvironmentId()) == false)
return false;
if (other.getDatabaseName() == null ^ this.getDatabaseName() == null)
return false;
if (other.getDatabaseName() != null && other.getDatabaseName().equals(this.getDatabaseName()) == false)
return false;
if (other.getChangeRequests() == null ^ this.getChangeRequests() == null)
return false;
if (other.getChangeRequests() != null && other.getChangeRequests().equals(this.getChangeRequests()) == false)
return false;
if (other.getClientToken() == null ^ this.getClientToken() == null)
return false;
if (other.getClientToken() != null && other.getClientToken().equals(this.getClientToken()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getEnvironmentId() == null) ? 0 : getEnvironmentId().hashCode());
hashCode = prime * hashCode + ((getDatabaseName() == null) ? 0 : getDatabaseName().hashCode());
hashCode = prime * hashCode + ((getChangeRequests() == null) ? 0 : getChangeRequests().hashCode());
hashCode = prime * hashCode + ((getClientToken() == null) ? 0 : getClientToken().hashCode());
return hashCode;
}
@Override
public CreateKxChangesetRequest clone() {
return (CreateKxChangesetRequest) super.clone();
}
}