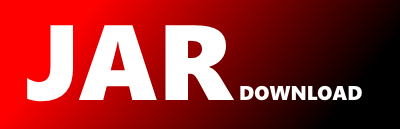
com.amazonaws.services.finspace.model.CreateKxChangesetResult Maven / Gradle / Ivy
Show all versions of aws-java-sdk-finspace Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.finspace.model;
import java.io.Serializable;
import javax.annotation.Generated;
/**
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class CreateKxChangesetResult extends com.amazonaws.AmazonWebServiceResult implements Serializable, Cloneable {
/**
*
* A unique identifier for the changeset.
*
*/
private String changesetId;
/**
*
* The name of the kdb database.
*
*/
private String databaseName;
/**
*
* A unique identifier for the kdb environment.
*
*/
private String environmentId;
/**
*
* A list of change requests.
*
*/
private java.util.List changeRequests;
/**
*
* The timestamp at which the changeset was created in FinSpace. The value is determined as epoch time in
* milliseconds. For example, the value for Monday, November 1, 2021 12:00:00 PM UTC is specified as 1635768000000.
*
*/
private java.util.Date createdTimestamp;
/**
*
* The timestamp at which the changeset was updated in FinSpace. The value is determined as epoch time in
* milliseconds. For example, the value for Monday, November 1, 2021 12:00:00 PM UTC is specified as 1635768000000.
*
*/
private java.util.Date lastModifiedTimestamp;
/**
*
* Status of the changeset creation process.
*
*
* -
*
* Pending – Changeset creation is pending.
*
*
* -
*
* Processing – Changeset creation is running.
*
*
* -
*
* Failed – Changeset creation has failed.
*
*
* -
*
* Complete – Changeset creation has succeeded.
*
*
*
*/
private String status;
/**
*
* The details of the error that you receive when creating a changeset. It consists of the type of error and the
* error message.
*
*/
private ErrorInfo errorInfo;
/**
*
* A unique identifier for the changeset.
*
*
* @param changesetId
* A unique identifier for the changeset.
*/
public void setChangesetId(String changesetId) {
this.changesetId = changesetId;
}
/**
*
* A unique identifier for the changeset.
*
*
* @return A unique identifier for the changeset.
*/
public String getChangesetId() {
return this.changesetId;
}
/**
*
* A unique identifier for the changeset.
*
*
* @param changesetId
* A unique identifier for the changeset.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateKxChangesetResult withChangesetId(String changesetId) {
setChangesetId(changesetId);
return this;
}
/**
*
* The name of the kdb database.
*
*
* @param databaseName
* The name of the kdb database.
*/
public void setDatabaseName(String databaseName) {
this.databaseName = databaseName;
}
/**
*
* The name of the kdb database.
*
*
* @return The name of the kdb database.
*/
public String getDatabaseName() {
return this.databaseName;
}
/**
*
* The name of the kdb database.
*
*
* @param databaseName
* The name of the kdb database.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateKxChangesetResult withDatabaseName(String databaseName) {
setDatabaseName(databaseName);
return this;
}
/**
*
* A unique identifier for the kdb environment.
*
*
* @param environmentId
* A unique identifier for the kdb environment.
*/
public void setEnvironmentId(String environmentId) {
this.environmentId = environmentId;
}
/**
*
* A unique identifier for the kdb environment.
*
*
* @return A unique identifier for the kdb environment.
*/
public String getEnvironmentId() {
return this.environmentId;
}
/**
*
* A unique identifier for the kdb environment.
*
*
* @param environmentId
* A unique identifier for the kdb environment.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateKxChangesetResult withEnvironmentId(String environmentId) {
setEnvironmentId(environmentId);
return this;
}
/**
*
* A list of change requests.
*
*
* @return A list of change requests.
*/
public java.util.List getChangeRequests() {
return changeRequests;
}
/**
*
* A list of change requests.
*
*
* @param changeRequests
* A list of change requests.
*/
public void setChangeRequests(java.util.Collection changeRequests) {
if (changeRequests == null) {
this.changeRequests = null;
return;
}
this.changeRequests = new java.util.ArrayList(changeRequests);
}
/**
*
* A list of change requests.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setChangeRequests(java.util.Collection)} or {@link #withChangeRequests(java.util.Collection)} if you want
* to override the existing values.
*
*
* @param changeRequests
* A list of change requests.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateKxChangesetResult withChangeRequests(ChangeRequest... changeRequests) {
if (this.changeRequests == null) {
setChangeRequests(new java.util.ArrayList(changeRequests.length));
}
for (ChangeRequest ele : changeRequests) {
this.changeRequests.add(ele);
}
return this;
}
/**
*
* A list of change requests.
*
*
* @param changeRequests
* A list of change requests.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateKxChangesetResult withChangeRequests(java.util.Collection changeRequests) {
setChangeRequests(changeRequests);
return this;
}
/**
*
* The timestamp at which the changeset was created in FinSpace. The value is determined as epoch time in
* milliseconds. For example, the value for Monday, November 1, 2021 12:00:00 PM UTC is specified as 1635768000000.
*
*
* @param createdTimestamp
* The timestamp at which the changeset was created in FinSpace. The value is determined as epoch time in
* milliseconds. For example, the value for Monday, November 1, 2021 12:00:00 PM UTC is specified as
* 1635768000000.
*/
public void setCreatedTimestamp(java.util.Date createdTimestamp) {
this.createdTimestamp = createdTimestamp;
}
/**
*
* The timestamp at which the changeset was created in FinSpace. The value is determined as epoch time in
* milliseconds. For example, the value for Monday, November 1, 2021 12:00:00 PM UTC is specified as 1635768000000.
*
*
* @return The timestamp at which the changeset was created in FinSpace. The value is determined as epoch time in
* milliseconds. For example, the value for Monday, November 1, 2021 12:00:00 PM UTC is specified as
* 1635768000000.
*/
public java.util.Date getCreatedTimestamp() {
return this.createdTimestamp;
}
/**
*
* The timestamp at which the changeset was created in FinSpace. The value is determined as epoch time in
* milliseconds. For example, the value for Monday, November 1, 2021 12:00:00 PM UTC is specified as 1635768000000.
*
*
* @param createdTimestamp
* The timestamp at which the changeset was created in FinSpace. The value is determined as epoch time in
* milliseconds. For example, the value for Monday, November 1, 2021 12:00:00 PM UTC is specified as
* 1635768000000.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateKxChangesetResult withCreatedTimestamp(java.util.Date createdTimestamp) {
setCreatedTimestamp(createdTimestamp);
return this;
}
/**
*
* The timestamp at which the changeset was updated in FinSpace. The value is determined as epoch time in
* milliseconds. For example, the value for Monday, November 1, 2021 12:00:00 PM UTC is specified as 1635768000000.
*
*
* @param lastModifiedTimestamp
* The timestamp at which the changeset was updated in FinSpace. The value is determined as epoch time in
* milliseconds. For example, the value for Monday, November 1, 2021 12:00:00 PM UTC is specified as
* 1635768000000.
*/
public void setLastModifiedTimestamp(java.util.Date lastModifiedTimestamp) {
this.lastModifiedTimestamp = lastModifiedTimestamp;
}
/**
*
* The timestamp at which the changeset was updated in FinSpace. The value is determined as epoch time in
* milliseconds. For example, the value for Monday, November 1, 2021 12:00:00 PM UTC is specified as 1635768000000.
*
*
* @return The timestamp at which the changeset was updated in FinSpace. The value is determined as epoch time in
* milliseconds. For example, the value for Monday, November 1, 2021 12:00:00 PM UTC is specified as
* 1635768000000.
*/
public java.util.Date getLastModifiedTimestamp() {
return this.lastModifiedTimestamp;
}
/**
*
* The timestamp at which the changeset was updated in FinSpace. The value is determined as epoch time in
* milliseconds. For example, the value for Monday, November 1, 2021 12:00:00 PM UTC is specified as 1635768000000.
*
*
* @param lastModifiedTimestamp
* The timestamp at which the changeset was updated in FinSpace. The value is determined as epoch time in
* milliseconds. For example, the value for Monday, November 1, 2021 12:00:00 PM UTC is specified as
* 1635768000000.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateKxChangesetResult withLastModifiedTimestamp(java.util.Date lastModifiedTimestamp) {
setLastModifiedTimestamp(lastModifiedTimestamp);
return this;
}
/**
*
* Status of the changeset creation process.
*
*
* -
*
* Pending – Changeset creation is pending.
*
*
* -
*
* Processing – Changeset creation is running.
*
*
* -
*
* Failed – Changeset creation has failed.
*
*
* -
*
* Complete – Changeset creation has succeeded.
*
*
*
*
* @param status
* Status of the changeset creation process.
*
* -
*
* Pending – Changeset creation is pending.
*
*
* -
*
* Processing – Changeset creation is running.
*
*
* -
*
* Failed – Changeset creation has failed.
*
*
* -
*
* Complete – Changeset creation has succeeded.
*
*
* @see ChangesetStatus
*/
public void setStatus(String status) {
this.status = status;
}
/**
*
* Status of the changeset creation process.
*
*
* -
*
* Pending – Changeset creation is pending.
*
*
* -
*
* Processing – Changeset creation is running.
*
*
* -
*
* Failed – Changeset creation has failed.
*
*
* -
*
* Complete – Changeset creation has succeeded.
*
*
*
*
* @return Status of the changeset creation process.
*
* -
*
* Pending – Changeset creation is pending.
*
*
* -
*
* Processing – Changeset creation is running.
*
*
* -
*
* Failed – Changeset creation has failed.
*
*
* -
*
* Complete – Changeset creation has succeeded.
*
*
* @see ChangesetStatus
*/
public String getStatus() {
return this.status;
}
/**
*
* Status of the changeset creation process.
*
*
* -
*
* Pending – Changeset creation is pending.
*
*
* -
*
* Processing – Changeset creation is running.
*
*
* -
*
* Failed – Changeset creation has failed.
*
*
* -
*
* Complete – Changeset creation has succeeded.
*
*
*
*
* @param status
* Status of the changeset creation process.
*
* -
*
* Pending – Changeset creation is pending.
*
*
* -
*
* Processing – Changeset creation is running.
*
*
* -
*
* Failed – Changeset creation has failed.
*
*
* -
*
* Complete – Changeset creation has succeeded.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see ChangesetStatus
*/
public CreateKxChangesetResult withStatus(String status) {
setStatus(status);
return this;
}
/**
*
* Status of the changeset creation process.
*
*
* -
*
* Pending – Changeset creation is pending.
*
*
* -
*
* Processing – Changeset creation is running.
*
*
* -
*
* Failed – Changeset creation has failed.
*
*
* -
*
* Complete – Changeset creation has succeeded.
*
*
*
*
* @param status
* Status of the changeset creation process.
*
* -
*
* Pending – Changeset creation is pending.
*
*
* -
*
* Processing – Changeset creation is running.
*
*
* -
*
* Failed – Changeset creation has failed.
*
*
* -
*
* Complete – Changeset creation has succeeded.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see ChangesetStatus
*/
public CreateKxChangesetResult withStatus(ChangesetStatus status) {
this.status = status.toString();
return this;
}
/**
*
* The details of the error that you receive when creating a changeset. It consists of the type of error and the
* error message.
*
*
* @param errorInfo
* The details of the error that you receive when creating a changeset. It consists of the type of error and
* the error message.
*/
public void setErrorInfo(ErrorInfo errorInfo) {
this.errorInfo = errorInfo;
}
/**
*
* The details of the error that you receive when creating a changeset. It consists of the type of error and the
* error message.
*
*
* @return The details of the error that you receive when creating a changeset. It consists of the type of error and
* the error message.
*/
public ErrorInfo getErrorInfo() {
return this.errorInfo;
}
/**
*
* The details of the error that you receive when creating a changeset. It consists of the type of error and the
* error message.
*
*
* @param errorInfo
* The details of the error that you receive when creating a changeset. It consists of the type of error and
* the error message.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateKxChangesetResult withErrorInfo(ErrorInfo errorInfo) {
setErrorInfo(errorInfo);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getChangesetId() != null)
sb.append("ChangesetId: ").append(getChangesetId()).append(",");
if (getDatabaseName() != null)
sb.append("DatabaseName: ").append(getDatabaseName()).append(",");
if (getEnvironmentId() != null)
sb.append("EnvironmentId: ").append(getEnvironmentId()).append(",");
if (getChangeRequests() != null)
sb.append("ChangeRequests: ").append(getChangeRequests()).append(",");
if (getCreatedTimestamp() != null)
sb.append("CreatedTimestamp: ").append(getCreatedTimestamp()).append(",");
if (getLastModifiedTimestamp() != null)
sb.append("LastModifiedTimestamp: ").append(getLastModifiedTimestamp()).append(",");
if (getStatus() != null)
sb.append("Status: ").append(getStatus()).append(",");
if (getErrorInfo() != null)
sb.append("ErrorInfo: ").append(getErrorInfo());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof CreateKxChangesetResult == false)
return false;
CreateKxChangesetResult other = (CreateKxChangesetResult) obj;
if (other.getChangesetId() == null ^ this.getChangesetId() == null)
return false;
if (other.getChangesetId() != null && other.getChangesetId().equals(this.getChangesetId()) == false)
return false;
if (other.getDatabaseName() == null ^ this.getDatabaseName() == null)
return false;
if (other.getDatabaseName() != null && other.getDatabaseName().equals(this.getDatabaseName()) == false)
return false;
if (other.getEnvironmentId() == null ^ this.getEnvironmentId() == null)
return false;
if (other.getEnvironmentId() != null && other.getEnvironmentId().equals(this.getEnvironmentId()) == false)
return false;
if (other.getChangeRequests() == null ^ this.getChangeRequests() == null)
return false;
if (other.getChangeRequests() != null && other.getChangeRequests().equals(this.getChangeRequests()) == false)
return false;
if (other.getCreatedTimestamp() == null ^ this.getCreatedTimestamp() == null)
return false;
if (other.getCreatedTimestamp() != null && other.getCreatedTimestamp().equals(this.getCreatedTimestamp()) == false)
return false;
if (other.getLastModifiedTimestamp() == null ^ this.getLastModifiedTimestamp() == null)
return false;
if (other.getLastModifiedTimestamp() != null && other.getLastModifiedTimestamp().equals(this.getLastModifiedTimestamp()) == false)
return false;
if (other.getStatus() == null ^ this.getStatus() == null)
return false;
if (other.getStatus() != null && other.getStatus().equals(this.getStatus()) == false)
return false;
if (other.getErrorInfo() == null ^ this.getErrorInfo() == null)
return false;
if (other.getErrorInfo() != null && other.getErrorInfo().equals(this.getErrorInfo()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getChangesetId() == null) ? 0 : getChangesetId().hashCode());
hashCode = prime * hashCode + ((getDatabaseName() == null) ? 0 : getDatabaseName().hashCode());
hashCode = prime * hashCode + ((getEnvironmentId() == null) ? 0 : getEnvironmentId().hashCode());
hashCode = prime * hashCode + ((getChangeRequests() == null) ? 0 : getChangeRequests().hashCode());
hashCode = prime * hashCode + ((getCreatedTimestamp() == null) ? 0 : getCreatedTimestamp().hashCode());
hashCode = prime * hashCode + ((getLastModifiedTimestamp() == null) ? 0 : getLastModifiedTimestamp().hashCode());
hashCode = prime * hashCode + ((getStatus() == null) ? 0 : getStatus().hashCode());
hashCode = prime * hashCode + ((getErrorInfo() == null) ? 0 : getErrorInfo().hashCode());
return hashCode;
}
@Override
public CreateKxChangesetResult clone() {
try {
return (CreateKxChangesetResult) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
}