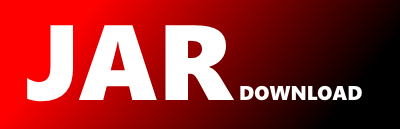
com.amazonaws.services.finspace.model.Environment Maven / Gradle / Ivy
Show all versions of aws-java-sdk-finspace Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.finspace.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Represents an FinSpace environment.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class Environment implements Serializable, Cloneable, StructuredPojo {
/**
*
* The name of the FinSpace environment.
*
*/
private String name;
/**
*
* The identifier of the FinSpace environment.
*
*/
private String environmentId;
/**
*
* The ID of the AWS account in which the FinSpace environment is created.
*
*/
private String awsAccountId;
/**
*
* The current status of creation of the FinSpace environment.
*
*/
private String status;
/**
*
* The sign-in URL for the web application of your FinSpace environment.
*
*/
private String environmentUrl;
/**
*
* The description of the FinSpace environment.
*
*/
private String description;
/**
*
* The Amazon Resource Name (ARN) of your FinSpace environment.
*
*/
private String environmentArn;
/**
*
* The URL of the integrated FinSpace notebook environment in your web application.
*
*/
private String sageMakerStudioDomainUrl;
/**
*
* The KMS key id used to encrypt in the FinSpace environment.
*
*/
private String kmsKeyId;
/**
*
* The AWS account ID of the dedicated service account associated with your FinSpace environment.
*
*/
private String dedicatedServiceAccountId;
/**
*
* The authentication mode for the environment.
*
*/
private String federationMode;
/**
*
* Configuration information when authentication mode is FEDERATED.
*
*/
private FederationParameters federationParameters;
/**
*
* The name of the FinSpace environment.
*
*
* @param name
* The name of the FinSpace environment.
*/
public void setName(String name) {
this.name = name;
}
/**
*
* The name of the FinSpace environment.
*
*
* @return The name of the FinSpace environment.
*/
public String getName() {
return this.name;
}
/**
*
* The name of the FinSpace environment.
*
*
* @param name
* The name of the FinSpace environment.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Environment withName(String name) {
setName(name);
return this;
}
/**
*
* The identifier of the FinSpace environment.
*
*
* @param environmentId
* The identifier of the FinSpace environment.
*/
public void setEnvironmentId(String environmentId) {
this.environmentId = environmentId;
}
/**
*
* The identifier of the FinSpace environment.
*
*
* @return The identifier of the FinSpace environment.
*/
public String getEnvironmentId() {
return this.environmentId;
}
/**
*
* The identifier of the FinSpace environment.
*
*
* @param environmentId
* The identifier of the FinSpace environment.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Environment withEnvironmentId(String environmentId) {
setEnvironmentId(environmentId);
return this;
}
/**
*
* The ID of the AWS account in which the FinSpace environment is created.
*
*
* @param awsAccountId
* The ID of the AWS account in which the FinSpace environment is created.
*/
public void setAwsAccountId(String awsAccountId) {
this.awsAccountId = awsAccountId;
}
/**
*
* The ID of the AWS account in which the FinSpace environment is created.
*
*
* @return The ID of the AWS account in which the FinSpace environment is created.
*/
public String getAwsAccountId() {
return this.awsAccountId;
}
/**
*
* The ID of the AWS account in which the FinSpace environment is created.
*
*
* @param awsAccountId
* The ID of the AWS account in which the FinSpace environment is created.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Environment withAwsAccountId(String awsAccountId) {
setAwsAccountId(awsAccountId);
return this;
}
/**
*
* The current status of creation of the FinSpace environment.
*
*
* @param status
* The current status of creation of the FinSpace environment.
* @see EnvironmentStatus
*/
public void setStatus(String status) {
this.status = status;
}
/**
*
* The current status of creation of the FinSpace environment.
*
*
* @return The current status of creation of the FinSpace environment.
* @see EnvironmentStatus
*/
public String getStatus() {
return this.status;
}
/**
*
* The current status of creation of the FinSpace environment.
*
*
* @param status
* The current status of creation of the FinSpace environment.
* @return Returns a reference to this object so that method calls can be chained together.
* @see EnvironmentStatus
*/
public Environment withStatus(String status) {
setStatus(status);
return this;
}
/**
*
* The current status of creation of the FinSpace environment.
*
*
* @param status
* The current status of creation of the FinSpace environment.
* @return Returns a reference to this object so that method calls can be chained together.
* @see EnvironmentStatus
*/
public Environment withStatus(EnvironmentStatus status) {
this.status = status.toString();
return this;
}
/**
*
* The sign-in URL for the web application of your FinSpace environment.
*
*
* @param environmentUrl
* The sign-in URL for the web application of your FinSpace environment.
*/
public void setEnvironmentUrl(String environmentUrl) {
this.environmentUrl = environmentUrl;
}
/**
*
* The sign-in URL for the web application of your FinSpace environment.
*
*
* @return The sign-in URL for the web application of your FinSpace environment.
*/
public String getEnvironmentUrl() {
return this.environmentUrl;
}
/**
*
* The sign-in URL for the web application of your FinSpace environment.
*
*
* @param environmentUrl
* The sign-in URL for the web application of your FinSpace environment.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Environment withEnvironmentUrl(String environmentUrl) {
setEnvironmentUrl(environmentUrl);
return this;
}
/**
*
* The description of the FinSpace environment.
*
*
* @param description
* The description of the FinSpace environment.
*/
public void setDescription(String description) {
this.description = description;
}
/**
*
* The description of the FinSpace environment.
*
*
* @return The description of the FinSpace environment.
*/
public String getDescription() {
return this.description;
}
/**
*
* The description of the FinSpace environment.
*
*
* @param description
* The description of the FinSpace environment.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Environment withDescription(String description) {
setDescription(description);
return this;
}
/**
*
* The Amazon Resource Name (ARN) of your FinSpace environment.
*
*
* @param environmentArn
* The Amazon Resource Name (ARN) of your FinSpace environment.
*/
public void setEnvironmentArn(String environmentArn) {
this.environmentArn = environmentArn;
}
/**
*
* The Amazon Resource Name (ARN) of your FinSpace environment.
*
*
* @return The Amazon Resource Name (ARN) of your FinSpace environment.
*/
public String getEnvironmentArn() {
return this.environmentArn;
}
/**
*
* The Amazon Resource Name (ARN) of your FinSpace environment.
*
*
* @param environmentArn
* The Amazon Resource Name (ARN) of your FinSpace environment.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Environment withEnvironmentArn(String environmentArn) {
setEnvironmentArn(environmentArn);
return this;
}
/**
*
* The URL of the integrated FinSpace notebook environment in your web application.
*
*
* @param sageMakerStudioDomainUrl
* The URL of the integrated FinSpace notebook environment in your web application.
*/
public void setSageMakerStudioDomainUrl(String sageMakerStudioDomainUrl) {
this.sageMakerStudioDomainUrl = sageMakerStudioDomainUrl;
}
/**
*
* The URL of the integrated FinSpace notebook environment in your web application.
*
*
* @return The URL of the integrated FinSpace notebook environment in your web application.
*/
public String getSageMakerStudioDomainUrl() {
return this.sageMakerStudioDomainUrl;
}
/**
*
* The URL of the integrated FinSpace notebook environment in your web application.
*
*
* @param sageMakerStudioDomainUrl
* The URL of the integrated FinSpace notebook environment in your web application.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Environment withSageMakerStudioDomainUrl(String sageMakerStudioDomainUrl) {
setSageMakerStudioDomainUrl(sageMakerStudioDomainUrl);
return this;
}
/**
*
* The KMS key id used to encrypt in the FinSpace environment.
*
*
* @param kmsKeyId
* The KMS key id used to encrypt in the FinSpace environment.
*/
public void setKmsKeyId(String kmsKeyId) {
this.kmsKeyId = kmsKeyId;
}
/**
*
* The KMS key id used to encrypt in the FinSpace environment.
*
*
* @return The KMS key id used to encrypt in the FinSpace environment.
*/
public String getKmsKeyId() {
return this.kmsKeyId;
}
/**
*
* The KMS key id used to encrypt in the FinSpace environment.
*
*
* @param kmsKeyId
* The KMS key id used to encrypt in the FinSpace environment.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Environment withKmsKeyId(String kmsKeyId) {
setKmsKeyId(kmsKeyId);
return this;
}
/**
*
* The AWS account ID of the dedicated service account associated with your FinSpace environment.
*
*
* @param dedicatedServiceAccountId
* The AWS account ID of the dedicated service account associated with your FinSpace environment.
*/
public void setDedicatedServiceAccountId(String dedicatedServiceAccountId) {
this.dedicatedServiceAccountId = dedicatedServiceAccountId;
}
/**
*
* The AWS account ID of the dedicated service account associated with your FinSpace environment.
*
*
* @return The AWS account ID of the dedicated service account associated with your FinSpace environment.
*/
public String getDedicatedServiceAccountId() {
return this.dedicatedServiceAccountId;
}
/**
*
* The AWS account ID of the dedicated service account associated with your FinSpace environment.
*
*
* @param dedicatedServiceAccountId
* The AWS account ID of the dedicated service account associated with your FinSpace environment.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Environment withDedicatedServiceAccountId(String dedicatedServiceAccountId) {
setDedicatedServiceAccountId(dedicatedServiceAccountId);
return this;
}
/**
*
* The authentication mode for the environment.
*
*
* @param federationMode
* The authentication mode for the environment.
* @see FederationMode
*/
public void setFederationMode(String federationMode) {
this.federationMode = federationMode;
}
/**
*
* The authentication mode for the environment.
*
*
* @return The authentication mode for the environment.
* @see FederationMode
*/
public String getFederationMode() {
return this.federationMode;
}
/**
*
* The authentication mode for the environment.
*
*
* @param federationMode
* The authentication mode for the environment.
* @return Returns a reference to this object so that method calls can be chained together.
* @see FederationMode
*/
public Environment withFederationMode(String federationMode) {
setFederationMode(federationMode);
return this;
}
/**
*
* The authentication mode for the environment.
*
*
* @param federationMode
* The authentication mode for the environment.
* @return Returns a reference to this object so that method calls can be chained together.
* @see FederationMode
*/
public Environment withFederationMode(FederationMode federationMode) {
this.federationMode = federationMode.toString();
return this;
}
/**
*
* Configuration information when authentication mode is FEDERATED.
*
*
* @param federationParameters
* Configuration information when authentication mode is FEDERATED.
*/
public void setFederationParameters(FederationParameters federationParameters) {
this.federationParameters = federationParameters;
}
/**
*
* Configuration information when authentication mode is FEDERATED.
*
*
* @return Configuration information when authentication mode is FEDERATED.
*/
public FederationParameters getFederationParameters() {
return this.federationParameters;
}
/**
*
* Configuration information when authentication mode is FEDERATED.
*
*
* @param federationParameters
* Configuration information when authentication mode is FEDERATED.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Environment withFederationParameters(FederationParameters federationParameters) {
setFederationParameters(federationParameters);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getName() != null)
sb.append("Name: ").append(getName()).append(",");
if (getEnvironmentId() != null)
sb.append("EnvironmentId: ").append(getEnvironmentId()).append(",");
if (getAwsAccountId() != null)
sb.append("AwsAccountId: ").append(getAwsAccountId()).append(",");
if (getStatus() != null)
sb.append("Status: ").append(getStatus()).append(",");
if (getEnvironmentUrl() != null)
sb.append("EnvironmentUrl: ").append(getEnvironmentUrl()).append(",");
if (getDescription() != null)
sb.append("Description: ").append(getDescription()).append(",");
if (getEnvironmentArn() != null)
sb.append("EnvironmentArn: ").append(getEnvironmentArn()).append(",");
if (getSageMakerStudioDomainUrl() != null)
sb.append("SageMakerStudioDomainUrl: ").append(getSageMakerStudioDomainUrl()).append(",");
if (getKmsKeyId() != null)
sb.append("KmsKeyId: ").append(getKmsKeyId()).append(",");
if (getDedicatedServiceAccountId() != null)
sb.append("DedicatedServiceAccountId: ").append(getDedicatedServiceAccountId()).append(",");
if (getFederationMode() != null)
sb.append("FederationMode: ").append(getFederationMode()).append(",");
if (getFederationParameters() != null)
sb.append("FederationParameters: ").append(getFederationParameters());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof Environment == false)
return false;
Environment other = (Environment) obj;
if (other.getName() == null ^ this.getName() == null)
return false;
if (other.getName() != null && other.getName().equals(this.getName()) == false)
return false;
if (other.getEnvironmentId() == null ^ this.getEnvironmentId() == null)
return false;
if (other.getEnvironmentId() != null && other.getEnvironmentId().equals(this.getEnvironmentId()) == false)
return false;
if (other.getAwsAccountId() == null ^ this.getAwsAccountId() == null)
return false;
if (other.getAwsAccountId() != null && other.getAwsAccountId().equals(this.getAwsAccountId()) == false)
return false;
if (other.getStatus() == null ^ this.getStatus() == null)
return false;
if (other.getStatus() != null && other.getStatus().equals(this.getStatus()) == false)
return false;
if (other.getEnvironmentUrl() == null ^ this.getEnvironmentUrl() == null)
return false;
if (other.getEnvironmentUrl() != null && other.getEnvironmentUrl().equals(this.getEnvironmentUrl()) == false)
return false;
if (other.getDescription() == null ^ this.getDescription() == null)
return false;
if (other.getDescription() != null && other.getDescription().equals(this.getDescription()) == false)
return false;
if (other.getEnvironmentArn() == null ^ this.getEnvironmentArn() == null)
return false;
if (other.getEnvironmentArn() != null && other.getEnvironmentArn().equals(this.getEnvironmentArn()) == false)
return false;
if (other.getSageMakerStudioDomainUrl() == null ^ this.getSageMakerStudioDomainUrl() == null)
return false;
if (other.getSageMakerStudioDomainUrl() != null && other.getSageMakerStudioDomainUrl().equals(this.getSageMakerStudioDomainUrl()) == false)
return false;
if (other.getKmsKeyId() == null ^ this.getKmsKeyId() == null)
return false;
if (other.getKmsKeyId() != null && other.getKmsKeyId().equals(this.getKmsKeyId()) == false)
return false;
if (other.getDedicatedServiceAccountId() == null ^ this.getDedicatedServiceAccountId() == null)
return false;
if (other.getDedicatedServiceAccountId() != null && other.getDedicatedServiceAccountId().equals(this.getDedicatedServiceAccountId()) == false)
return false;
if (other.getFederationMode() == null ^ this.getFederationMode() == null)
return false;
if (other.getFederationMode() != null && other.getFederationMode().equals(this.getFederationMode()) == false)
return false;
if (other.getFederationParameters() == null ^ this.getFederationParameters() == null)
return false;
if (other.getFederationParameters() != null && other.getFederationParameters().equals(this.getFederationParameters()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getName() == null) ? 0 : getName().hashCode());
hashCode = prime * hashCode + ((getEnvironmentId() == null) ? 0 : getEnvironmentId().hashCode());
hashCode = prime * hashCode + ((getAwsAccountId() == null) ? 0 : getAwsAccountId().hashCode());
hashCode = prime * hashCode + ((getStatus() == null) ? 0 : getStatus().hashCode());
hashCode = prime * hashCode + ((getEnvironmentUrl() == null) ? 0 : getEnvironmentUrl().hashCode());
hashCode = prime * hashCode + ((getDescription() == null) ? 0 : getDescription().hashCode());
hashCode = prime * hashCode + ((getEnvironmentArn() == null) ? 0 : getEnvironmentArn().hashCode());
hashCode = prime * hashCode + ((getSageMakerStudioDomainUrl() == null) ? 0 : getSageMakerStudioDomainUrl().hashCode());
hashCode = prime * hashCode + ((getKmsKeyId() == null) ? 0 : getKmsKeyId().hashCode());
hashCode = prime * hashCode + ((getDedicatedServiceAccountId() == null) ? 0 : getDedicatedServiceAccountId().hashCode());
hashCode = prime * hashCode + ((getFederationMode() == null) ? 0 : getFederationMode().hashCode());
hashCode = prime * hashCode + ((getFederationParameters() == null) ? 0 : getFederationParameters().hashCode());
return hashCode;
}
@Override
public Environment clone() {
try {
return (Environment) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.finspace.model.transform.EnvironmentMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}