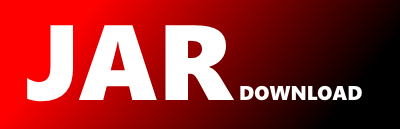
com.amazonaws.services.finspace.model.GetKxScalingGroupResult Maven / Gradle / Ivy
Show all versions of aws-java-sdk-finspace Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.finspace.model;
import java.io.Serializable;
import javax.annotation.Generated;
/**
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class GetKxScalingGroupResult extends com.amazonaws.AmazonWebServiceResult implements Serializable, Cloneable {
/**
*
* A unique identifier for the kdb scaling group.
*
*/
private String scalingGroupName;
/**
*
* The ARN identifier for the scaling group.
*
*/
private String scalingGroupArn;
/**
*
* The memory and CPU capabilities of the scaling group host on which FinSpace Managed kdb clusters will be placed.
*
*
* It can have one of the following values:
*
*
* -
*
* kx.sg.4xlarge
– The host type with a configuration of 108 GiB memory and 16 vCPUs.
*
*
* -
*
* kx.sg.8xlarge
– The host type with a configuration of 216 GiB memory and 32 vCPUs.
*
*
* -
*
* kx.sg.16xlarge
– The host type with a configuration of 432 GiB memory and 64 vCPUs.
*
*
* -
*
* kx.sg.32xlarge
– The host type with a configuration of 864 GiB memory and 128 vCPUs.
*
*
* -
*
* kx.sg1.16xlarge
– The host type with a configuration of 1949 GiB memory and 64 vCPUs.
*
*
* -
*
* kx.sg1.24xlarge
– The host type with a configuration of 2948 GiB memory and 96 vCPUs.
*
*
*
*/
private String hostType;
/**
*
* The list of Managed kdb clusters that are currently active in the given scaling group.
*
*/
private java.util.List clusters;
/**
*
* The identifier of the availability zones.
*
*/
private String availabilityZoneId;
/**
*
* The status of scaling group.
*
*
* -
*
* CREATING – The scaling group creation is in progress.
*
*
* -
*
* CREATE_FAILED – The scaling group creation has failed.
*
*
* -
*
* ACTIVE – The scaling group is active.
*
*
* -
*
* UPDATING – The scaling group is in the process of being updated.
*
*
* -
*
* UPDATE_FAILED – The update action failed.
*
*
* -
*
* DELETING – The scaling group is in the process of being deleted.
*
*
* -
*
* DELETE_FAILED – The system failed to delete the scaling group.
*
*
* -
*
* DELETED – The scaling group is successfully deleted.
*
*
*
*/
private String status;
/**
*
* The error message when a failed state occurs.
*
*/
private String statusReason;
/**
*
* The last time that the scaling group was updated in FinSpace. The value is determined as epoch time in
* milliseconds. For example, the value for Monday, November 1, 2021 12:00:00 PM UTC is specified as 1635768000000.
*
*/
private java.util.Date lastModifiedTimestamp;
/**
*
* The timestamp at which the scaling group was created in FinSpace. The value is determined as epoch time in
* milliseconds. For example, the value for Monday, November 1, 2021 12:00:00 PM UTC is specified as 1635768000000.
*
*/
private java.util.Date createdTimestamp;
/**
*
* A unique identifier for the kdb scaling group.
*
*
* @param scalingGroupName
* A unique identifier for the kdb scaling group.
*/
public void setScalingGroupName(String scalingGroupName) {
this.scalingGroupName = scalingGroupName;
}
/**
*
* A unique identifier for the kdb scaling group.
*
*
* @return A unique identifier for the kdb scaling group.
*/
public String getScalingGroupName() {
return this.scalingGroupName;
}
/**
*
* A unique identifier for the kdb scaling group.
*
*
* @param scalingGroupName
* A unique identifier for the kdb scaling group.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetKxScalingGroupResult withScalingGroupName(String scalingGroupName) {
setScalingGroupName(scalingGroupName);
return this;
}
/**
*
* The ARN identifier for the scaling group.
*
*
* @param scalingGroupArn
* The ARN identifier for the scaling group.
*/
public void setScalingGroupArn(String scalingGroupArn) {
this.scalingGroupArn = scalingGroupArn;
}
/**
*
* The ARN identifier for the scaling group.
*
*
* @return The ARN identifier for the scaling group.
*/
public String getScalingGroupArn() {
return this.scalingGroupArn;
}
/**
*
* The ARN identifier for the scaling group.
*
*
* @param scalingGroupArn
* The ARN identifier for the scaling group.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetKxScalingGroupResult withScalingGroupArn(String scalingGroupArn) {
setScalingGroupArn(scalingGroupArn);
return this;
}
/**
*
* The memory and CPU capabilities of the scaling group host on which FinSpace Managed kdb clusters will be placed.
*
*
* It can have one of the following values:
*
*
* -
*
* kx.sg.4xlarge
– The host type with a configuration of 108 GiB memory and 16 vCPUs.
*
*
* -
*
* kx.sg.8xlarge
– The host type with a configuration of 216 GiB memory and 32 vCPUs.
*
*
* -
*
* kx.sg.16xlarge
– The host type with a configuration of 432 GiB memory and 64 vCPUs.
*
*
* -
*
* kx.sg.32xlarge
– The host type with a configuration of 864 GiB memory and 128 vCPUs.
*
*
* -
*
* kx.sg1.16xlarge
– The host type with a configuration of 1949 GiB memory and 64 vCPUs.
*
*
* -
*
* kx.sg1.24xlarge
– The host type with a configuration of 2948 GiB memory and 96 vCPUs.
*
*
*
*
* @param hostType
* The memory and CPU capabilities of the scaling group host on which FinSpace Managed kdb clusters will be
* placed.
*
* It can have one of the following values:
*
*
* -
*
* kx.sg.4xlarge
– The host type with a configuration of 108 GiB memory and 16 vCPUs.
*
*
* -
*
* kx.sg.8xlarge
– The host type with a configuration of 216 GiB memory and 32 vCPUs.
*
*
* -
*
* kx.sg.16xlarge
– The host type with a configuration of 432 GiB memory and 64 vCPUs.
*
*
* -
*
* kx.sg.32xlarge
– The host type with a configuration of 864 GiB memory and 128 vCPUs.
*
*
* -
*
* kx.sg1.16xlarge
– The host type with a configuration of 1949 GiB memory and 64 vCPUs.
*
*
* -
*
* kx.sg1.24xlarge
– The host type with a configuration of 2948 GiB memory and 96 vCPUs.
*
*
*/
public void setHostType(String hostType) {
this.hostType = hostType;
}
/**
*
* The memory and CPU capabilities of the scaling group host on which FinSpace Managed kdb clusters will be placed.
*
*
* It can have one of the following values:
*
*
* -
*
* kx.sg.4xlarge
– The host type with a configuration of 108 GiB memory and 16 vCPUs.
*
*
* -
*
* kx.sg.8xlarge
– The host type with a configuration of 216 GiB memory and 32 vCPUs.
*
*
* -
*
* kx.sg.16xlarge
– The host type with a configuration of 432 GiB memory and 64 vCPUs.
*
*
* -
*
* kx.sg.32xlarge
– The host type with a configuration of 864 GiB memory and 128 vCPUs.
*
*
* -
*
* kx.sg1.16xlarge
– The host type with a configuration of 1949 GiB memory and 64 vCPUs.
*
*
* -
*
* kx.sg1.24xlarge
– The host type with a configuration of 2948 GiB memory and 96 vCPUs.
*
*
*
*
* @return The memory and CPU capabilities of the scaling group host on which FinSpace Managed kdb clusters will be
* placed.
*
* It can have one of the following values:
*
*
* -
*
* kx.sg.4xlarge
– The host type with a configuration of 108 GiB memory and 16 vCPUs.
*
*
* -
*
* kx.sg.8xlarge
– The host type with a configuration of 216 GiB memory and 32 vCPUs.
*
*
* -
*
* kx.sg.16xlarge
– The host type with a configuration of 432 GiB memory and 64 vCPUs.
*
*
* -
*
* kx.sg.32xlarge
– The host type with a configuration of 864 GiB memory and 128 vCPUs.
*
*
* -
*
* kx.sg1.16xlarge
– The host type with a configuration of 1949 GiB memory and 64 vCPUs.
*
*
* -
*
* kx.sg1.24xlarge
– The host type with a configuration of 2948 GiB memory and 96 vCPUs.
*
*
*/
public String getHostType() {
return this.hostType;
}
/**
*
* The memory and CPU capabilities of the scaling group host on which FinSpace Managed kdb clusters will be placed.
*
*
* It can have one of the following values:
*
*
* -
*
* kx.sg.4xlarge
– The host type with a configuration of 108 GiB memory and 16 vCPUs.
*
*
* -
*
* kx.sg.8xlarge
– The host type with a configuration of 216 GiB memory and 32 vCPUs.
*
*
* -
*
* kx.sg.16xlarge
– The host type with a configuration of 432 GiB memory and 64 vCPUs.
*
*
* -
*
* kx.sg.32xlarge
– The host type with a configuration of 864 GiB memory and 128 vCPUs.
*
*
* -
*
* kx.sg1.16xlarge
– The host type with a configuration of 1949 GiB memory and 64 vCPUs.
*
*
* -
*
* kx.sg1.24xlarge
– The host type with a configuration of 2948 GiB memory and 96 vCPUs.
*
*
*
*
* @param hostType
* The memory and CPU capabilities of the scaling group host on which FinSpace Managed kdb clusters will be
* placed.
*
* It can have one of the following values:
*
*
* -
*
* kx.sg.4xlarge
– The host type with a configuration of 108 GiB memory and 16 vCPUs.
*
*
* -
*
* kx.sg.8xlarge
– The host type with a configuration of 216 GiB memory and 32 vCPUs.
*
*
* -
*
* kx.sg.16xlarge
– The host type with a configuration of 432 GiB memory and 64 vCPUs.
*
*
* -
*
* kx.sg.32xlarge
– The host type with a configuration of 864 GiB memory and 128 vCPUs.
*
*
* -
*
* kx.sg1.16xlarge
– The host type with a configuration of 1949 GiB memory and 64 vCPUs.
*
*
* -
*
* kx.sg1.24xlarge
– The host type with a configuration of 2948 GiB memory and 96 vCPUs.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetKxScalingGroupResult withHostType(String hostType) {
setHostType(hostType);
return this;
}
/**
*
* The list of Managed kdb clusters that are currently active in the given scaling group.
*
*
* @return The list of Managed kdb clusters that are currently active in the given scaling group.
*/
public java.util.List getClusters() {
return clusters;
}
/**
*
* The list of Managed kdb clusters that are currently active in the given scaling group.
*
*
* @param clusters
* The list of Managed kdb clusters that are currently active in the given scaling group.
*/
public void setClusters(java.util.Collection clusters) {
if (clusters == null) {
this.clusters = null;
return;
}
this.clusters = new java.util.ArrayList(clusters);
}
/**
*
* The list of Managed kdb clusters that are currently active in the given scaling group.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setClusters(java.util.Collection)} or {@link #withClusters(java.util.Collection)} if you want to override
* the existing values.
*
*
* @param clusters
* The list of Managed kdb clusters that are currently active in the given scaling group.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetKxScalingGroupResult withClusters(String... clusters) {
if (this.clusters == null) {
setClusters(new java.util.ArrayList(clusters.length));
}
for (String ele : clusters) {
this.clusters.add(ele);
}
return this;
}
/**
*
* The list of Managed kdb clusters that are currently active in the given scaling group.
*
*
* @param clusters
* The list of Managed kdb clusters that are currently active in the given scaling group.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetKxScalingGroupResult withClusters(java.util.Collection clusters) {
setClusters(clusters);
return this;
}
/**
*
* The identifier of the availability zones.
*
*
* @param availabilityZoneId
* The identifier of the availability zones.
*/
public void setAvailabilityZoneId(String availabilityZoneId) {
this.availabilityZoneId = availabilityZoneId;
}
/**
*
* The identifier of the availability zones.
*
*
* @return The identifier of the availability zones.
*/
public String getAvailabilityZoneId() {
return this.availabilityZoneId;
}
/**
*
* The identifier of the availability zones.
*
*
* @param availabilityZoneId
* The identifier of the availability zones.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetKxScalingGroupResult withAvailabilityZoneId(String availabilityZoneId) {
setAvailabilityZoneId(availabilityZoneId);
return this;
}
/**
*
* The status of scaling group.
*
*
* -
*
* CREATING – The scaling group creation is in progress.
*
*
* -
*
* CREATE_FAILED – The scaling group creation has failed.
*
*
* -
*
* ACTIVE – The scaling group is active.
*
*
* -
*
* UPDATING – The scaling group is in the process of being updated.
*
*
* -
*
* UPDATE_FAILED – The update action failed.
*
*
* -
*
* DELETING – The scaling group is in the process of being deleted.
*
*
* -
*
* DELETE_FAILED – The system failed to delete the scaling group.
*
*
* -
*
* DELETED – The scaling group is successfully deleted.
*
*
*
*
* @param status
* The status of scaling group.
*
* -
*
* CREATING – The scaling group creation is in progress.
*
*
* -
*
* CREATE_FAILED – The scaling group creation has failed.
*
*
* -
*
* ACTIVE – The scaling group is active.
*
*
* -
*
* UPDATING – The scaling group is in the process of being updated.
*
*
* -
*
* UPDATE_FAILED – The update action failed.
*
*
* -
*
* DELETING – The scaling group is in the process of being deleted.
*
*
* -
*
* DELETE_FAILED – The system failed to delete the scaling group.
*
*
* -
*
* DELETED – The scaling group is successfully deleted.
*
*
* @see KxScalingGroupStatus
*/
public void setStatus(String status) {
this.status = status;
}
/**
*
* The status of scaling group.
*
*
* -
*
* CREATING – The scaling group creation is in progress.
*
*
* -
*
* CREATE_FAILED – The scaling group creation has failed.
*
*
* -
*
* ACTIVE – The scaling group is active.
*
*
* -
*
* UPDATING – The scaling group is in the process of being updated.
*
*
* -
*
* UPDATE_FAILED – The update action failed.
*
*
* -
*
* DELETING – The scaling group is in the process of being deleted.
*
*
* -
*
* DELETE_FAILED – The system failed to delete the scaling group.
*
*
* -
*
* DELETED – The scaling group is successfully deleted.
*
*
*
*
* @return The status of scaling group.
*
* -
*
* CREATING – The scaling group creation is in progress.
*
*
* -
*
* CREATE_FAILED – The scaling group creation has failed.
*
*
* -
*
* ACTIVE – The scaling group is active.
*
*
* -
*
* UPDATING – The scaling group is in the process of being updated.
*
*
* -
*
* UPDATE_FAILED – The update action failed.
*
*
* -
*
* DELETING – The scaling group is in the process of being deleted.
*
*
* -
*
* DELETE_FAILED – The system failed to delete the scaling group.
*
*
* -
*
* DELETED – The scaling group is successfully deleted.
*
*
* @see KxScalingGroupStatus
*/
public String getStatus() {
return this.status;
}
/**
*
* The status of scaling group.
*
*
* -
*
* CREATING – The scaling group creation is in progress.
*
*
* -
*
* CREATE_FAILED – The scaling group creation has failed.
*
*
* -
*
* ACTIVE – The scaling group is active.
*
*
* -
*
* UPDATING – The scaling group is in the process of being updated.
*
*
* -
*
* UPDATE_FAILED – The update action failed.
*
*
* -
*
* DELETING – The scaling group is in the process of being deleted.
*
*
* -
*
* DELETE_FAILED – The system failed to delete the scaling group.
*
*
* -
*
* DELETED – The scaling group is successfully deleted.
*
*
*
*
* @param status
* The status of scaling group.
*
* -
*
* CREATING – The scaling group creation is in progress.
*
*
* -
*
* CREATE_FAILED – The scaling group creation has failed.
*
*
* -
*
* ACTIVE – The scaling group is active.
*
*
* -
*
* UPDATING – The scaling group is in the process of being updated.
*
*
* -
*
* UPDATE_FAILED – The update action failed.
*
*
* -
*
* DELETING – The scaling group is in the process of being deleted.
*
*
* -
*
* DELETE_FAILED – The system failed to delete the scaling group.
*
*
* -
*
* DELETED – The scaling group is successfully deleted.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see KxScalingGroupStatus
*/
public GetKxScalingGroupResult withStatus(String status) {
setStatus(status);
return this;
}
/**
*
* The status of scaling group.
*
*
* -
*
* CREATING – The scaling group creation is in progress.
*
*
* -
*
* CREATE_FAILED – The scaling group creation has failed.
*
*
* -
*
* ACTIVE – The scaling group is active.
*
*
* -
*
* UPDATING – The scaling group is in the process of being updated.
*
*
* -
*
* UPDATE_FAILED – The update action failed.
*
*
* -
*
* DELETING – The scaling group is in the process of being deleted.
*
*
* -
*
* DELETE_FAILED – The system failed to delete the scaling group.
*
*
* -
*
* DELETED – The scaling group is successfully deleted.
*
*
*
*
* @param status
* The status of scaling group.
*
* -
*
* CREATING – The scaling group creation is in progress.
*
*
* -
*
* CREATE_FAILED – The scaling group creation has failed.
*
*
* -
*
* ACTIVE – The scaling group is active.
*
*
* -
*
* UPDATING – The scaling group is in the process of being updated.
*
*
* -
*
* UPDATE_FAILED – The update action failed.
*
*
* -
*
* DELETING – The scaling group is in the process of being deleted.
*
*
* -
*
* DELETE_FAILED – The system failed to delete the scaling group.
*
*
* -
*
* DELETED – The scaling group is successfully deleted.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see KxScalingGroupStatus
*/
public GetKxScalingGroupResult withStatus(KxScalingGroupStatus status) {
this.status = status.toString();
return this;
}
/**
*
* The error message when a failed state occurs.
*
*
* @param statusReason
* The error message when a failed state occurs.
*/
public void setStatusReason(String statusReason) {
this.statusReason = statusReason;
}
/**
*
* The error message when a failed state occurs.
*
*
* @return The error message when a failed state occurs.
*/
public String getStatusReason() {
return this.statusReason;
}
/**
*
* The error message when a failed state occurs.
*
*
* @param statusReason
* The error message when a failed state occurs.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetKxScalingGroupResult withStatusReason(String statusReason) {
setStatusReason(statusReason);
return this;
}
/**
*
* The last time that the scaling group was updated in FinSpace. The value is determined as epoch time in
* milliseconds. For example, the value for Monday, November 1, 2021 12:00:00 PM UTC is specified as 1635768000000.
*
*
* @param lastModifiedTimestamp
* The last time that the scaling group was updated in FinSpace. The value is determined as epoch time in
* milliseconds. For example, the value for Monday, November 1, 2021 12:00:00 PM UTC is specified as
* 1635768000000.
*/
public void setLastModifiedTimestamp(java.util.Date lastModifiedTimestamp) {
this.lastModifiedTimestamp = lastModifiedTimestamp;
}
/**
*
* The last time that the scaling group was updated in FinSpace. The value is determined as epoch time in
* milliseconds. For example, the value for Monday, November 1, 2021 12:00:00 PM UTC is specified as 1635768000000.
*
*
* @return The last time that the scaling group was updated in FinSpace. The value is determined as epoch time in
* milliseconds. For example, the value for Monday, November 1, 2021 12:00:00 PM UTC is specified as
* 1635768000000.
*/
public java.util.Date getLastModifiedTimestamp() {
return this.lastModifiedTimestamp;
}
/**
*
* The last time that the scaling group was updated in FinSpace. The value is determined as epoch time in
* milliseconds. For example, the value for Monday, November 1, 2021 12:00:00 PM UTC is specified as 1635768000000.
*
*
* @param lastModifiedTimestamp
* The last time that the scaling group was updated in FinSpace. The value is determined as epoch time in
* milliseconds. For example, the value for Monday, November 1, 2021 12:00:00 PM UTC is specified as
* 1635768000000.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetKxScalingGroupResult withLastModifiedTimestamp(java.util.Date lastModifiedTimestamp) {
setLastModifiedTimestamp(lastModifiedTimestamp);
return this;
}
/**
*
* The timestamp at which the scaling group was created in FinSpace. The value is determined as epoch time in
* milliseconds. For example, the value for Monday, November 1, 2021 12:00:00 PM UTC is specified as 1635768000000.
*
*
* @param createdTimestamp
* The timestamp at which the scaling group was created in FinSpace. The value is determined as epoch time in
* milliseconds. For example, the value for Monday, November 1, 2021 12:00:00 PM UTC is specified as
* 1635768000000.
*/
public void setCreatedTimestamp(java.util.Date createdTimestamp) {
this.createdTimestamp = createdTimestamp;
}
/**
*
* The timestamp at which the scaling group was created in FinSpace. The value is determined as epoch time in
* milliseconds. For example, the value for Monday, November 1, 2021 12:00:00 PM UTC is specified as 1635768000000.
*
*
* @return The timestamp at which the scaling group was created in FinSpace. The value is determined as epoch time
* in milliseconds. For example, the value for Monday, November 1, 2021 12:00:00 PM UTC is specified as
* 1635768000000.
*/
public java.util.Date getCreatedTimestamp() {
return this.createdTimestamp;
}
/**
*
* The timestamp at which the scaling group was created in FinSpace. The value is determined as epoch time in
* milliseconds. For example, the value for Monday, November 1, 2021 12:00:00 PM UTC is specified as 1635768000000.
*
*
* @param createdTimestamp
* The timestamp at which the scaling group was created in FinSpace. The value is determined as epoch time in
* milliseconds. For example, the value for Monday, November 1, 2021 12:00:00 PM UTC is specified as
* 1635768000000.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetKxScalingGroupResult withCreatedTimestamp(java.util.Date createdTimestamp) {
setCreatedTimestamp(createdTimestamp);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getScalingGroupName() != null)
sb.append("ScalingGroupName: ").append(getScalingGroupName()).append(",");
if (getScalingGroupArn() != null)
sb.append("ScalingGroupArn: ").append(getScalingGroupArn()).append(",");
if (getHostType() != null)
sb.append("HostType: ").append(getHostType()).append(",");
if (getClusters() != null)
sb.append("Clusters: ").append(getClusters()).append(",");
if (getAvailabilityZoneId() != null)
sb.append("AvailabilityZoneId: ").append(getAvailabilityZoneId()).append(",");
if (getStatus() != null)
sb.append("Status: ").append(getStatus()).append(",");
if (getStatusReason() != null)
sb.append("StatusReason: ").append(getStatusReason()).append(",");
if (getLastModifiedTimestamp() != null)
sb.append("LastModifiedTimestamp: ").append(getLastModifiedTimestamp()).append(",");
if (getCreatedTimestamp() != null)
sb.append("CreatedTimestamp: ").append(getCreatedTimestamp());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof GetKxScalingGroupResult == false)
return false;
GetKxScalingGroupResult other = (GetKxScalingGroupResult) obj;
if (other.getScalingGroupName() == null ^ this.getScalingGroupName() == null)
return false;
if (other.getScalingGroupName() != null && other.getScalingGroupName().equals(this.getScalingGroupName()) == false)
return false;
if (other.getScalingGroupArn() == null ^ this.getScalingGroupArn() == null)
return false;
if (other.getScalingGroupArn() != null && other.getScalingGroupArn().equals(this.getScalingGroupArn()) == false)
return false;
if (other.getHostType() == null ^ this.getHostType() == null)
return false;
if (other.getHostType() != null && other.getHostType().equals(this.getHostType()) == false)
return false;
if (other.getClusters() == null ^ this.getClusters() == null)
return false;
if (other.getClusters() != null && other.getClusters().equals(this.getClusters()) == false)
return false;
if (other.getAvailabilityZoneId() == null ^ this.getAvailabilityZoneId() == null)
return false;
if (other.getAvailabilityZoneId() != null && other.getAvailabilityZoneId().equals(this.getAvailabilityZoneId()) == false)
return false;
if (other.getStatus() == null ^ this.getStatus() == null)
return false;
if (other.getStatus() != null && other.getStatus().equals(this.getStatus()) == false)
return false;
if (other.getStatusReason() == null ^ this.getStatusReason() == null)
return false;
if (other.getStatusReason() != null && other.getStatusReason().equals(this.getStatusReason()) == false)
return false;
if (other.getLastModifiedTimestamp() == null ^ this.getLastModifiedTimestamp() == null)
return false;
if (other.getLastModifiedTimestamp() != null && other.getLastModifiedTimestamp().equals(this.getLastModifiedTimestamp()) == false)
return false;
if (other.getCreatedTimestamp() == null ^ this.getCreatedTimestamp() == null)
return false;
if (other.getCreatedTimestamp() != null && other.getCreatedTimestamp().equals(this.getCreatedTimestamp()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getScalingGroupName() == null) ? 0 : getScalingGroupName().hashCode());
hashCode = prime * hashCode + ((getScalingGroupArn() == null) ? 0 : getScalingGroupArn().hashCode());
hashCode = prime * hashCode + ((getHostType() == null) ? 0 : getHostType().hashCode());
hashCode = prime * hashCode + ((getClusters() == null) ? 0 : getClusters().hashCode());
hashCode = prime * hashCode + ((getAvailabilityZoneId() == null) ? 0 : getAvailabilityZoneId().hashCode());
hashCode = prime * hashCode + ((getStatus() == null) ? 0 : getStatus().hashCode());
hashCode = prime * hashCode + ((getStatusReason() == null) ? 0 : getStatusReason().hashCode());
hashCode = prime * hashCode + ((getLastModifiedTimestamp() == null) ? 0 : getLastModifiedTimestamp().hashCode());
hashCode = prime * hashCode + ((getCreatedTimestamp() == null) ? 0 : getCreatedTimestamp().hashCode());
return hashCode;
}
@Override
public GetKxScalingGroupResult clone() {
try {
return (GetKxScalingGroupResult) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
}