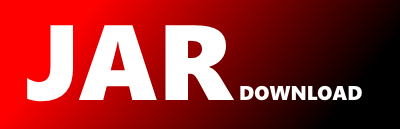
com.amazonaws.services.finspace.model.GetKxVolumeResult Maven / Gradle / Ivy
Show all versions of aws-java-sdk-finspace Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.finspace.model;
import java.io.Serializable;
import javax.annotation.Generated;
/**
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class GetKxVolumeResult extends com.amazonaws.AmazonWebServiceResult implements Serializable, Cloneable {
/**
*
* A unique identifier for the kdb environment, whose clusters can attach to the volume.
*
*/
private String environmentId;
/**
*
* A unique identifier for the volume.
*
*/
private String volumeName;
/**
*
* The type of file system volume. Currently, FinSpace only supports NAS_1
volume type.
*
*/
private String volumeType;
/**
*
* The ARN identifier of the volume.
*
*/
private String volumeArn;
/**
*
* Specifies the configuration for the Network attached storage (NAS_1) file system volume.
*
*/
private KxNAS1Configuration nas1Configuration;
/**
*
* The status of volume creation.
*
*
* -
*
* CREATING – The volume creation is in progress.
*
*
* -
*
* CREATE_FAILED – The volume creation has failed.
*
*
* -
*
* ACTIVE – The volume is active.
*
*
* -
*
* UPDATING – The volume is in the process of being updated.
*
*
* -
*
* UPDATE_FAILED – The update action failed.
*
*
* -
*
* UPDATED – The volume is successfully updated.
*
*
* -
*
* DELETING – The volume is in the process of being deleted.
*
*
* -
*
* DELETE_FAILED – The system failed to delete the volume.
*
*
* -
*
* DELETED – The volume is successfully deleted.
*
*
*
*/
private String status;
/**
*
* The error message when a failed state occurs.
*
*/
private String statusReason;
/**
*
* The timestamp at which the volume was created in FinSpace. The value is determined as epoch time in milliseconds.
* For example, the value for Monday, November 1, 2021 12:00:00 PM UTC is specified as 1635768000000.
*
*/
private java.util.Date createdTimestamp;
/**
*
* A description of the volume.
*
*/
private String description;
/**
*
* The number of availability zones you want to assign per volume. Currently, FinSpace only supports
* SINGLE
for volumes. This places dataview in a single AZ.
*
*/
private String azMode;
/**
*
* The identifier of the availability zones.
*
*/
private java.util.List availabilityZoneIds;
/**
*
* The last time that the volume was updated in FinSpace. The value is determined as epoch time in milliseconds. For
* example, the value for Monday, November 1, 2021 12:00:00 PM UTC is specified as 1635768000000.
*
*/
private java.util.Date lastModifiedTimestamp;
/**
*
* A list of cluster identifiers that a volume is attached to.
*
*/
private java.util.List attachedClusters;
/**
*
* A unique identifier for the kdb environment, whose clusters can attach to the volume.
*
*
* @param environmentId
* A unique identifier for the kdb environment, whose clusters can attach to the volume.
*/
public void setEnvironmentId(String environmentId) {
this.environmentId = environmentId;
}
/**
*
* A unique identifier for the kdb environment, whose clusters can attach to the volume.
*
*
* @return A unique identifier for the kdb environment, whose clusters can attach to the volume.
*/
public String getEnvironmentId() {
return this.environmentId;
}
/**
*
* A unique identifier for the kdb environment, whose clusters can attach to the volume.
*
*
* @param environmentId
* A unique identifier for the kdb environment, whose clusters can attach to the volume.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetKxVolumeResult withEnvironmentId(String environmentId) {
setEnvironmentId(environmentId);
return this;
}
/**
*
* A unique identifier for the volume.
*
*
* @param volumeName
* A unique identifier for the volume.
*/
public void setVolumeName(String volumeName) {
this.volumeName = volumeName;
}
/**
*
* A unique identifier for the volume.
*
*
* @return A unique identifier for the volume.
*/
public String getVolumeName() {
return this.volumeName;
}
/**
*
* A unique identifier for the volume.
*
*
* @param volumeName
* A unique identifier for the volume.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetKxVolumeResult withVolumeName(String volumeName) {
setVolumeName(volumeName);
return this;
}
/**
*
* The type of file system volume. Currently, FinSpace only supports NAS_1
volume type.
*
*
* @param volumeType
* The type of file system volume. Currently, FinSpace only supports NAS_1
volume type.
* @see KxVolumeType
*/
public void setVolumeType(String volumeType) {
this.volumeType = volumeType;
}
/**
*
* The type of file system volume. Currently, FinSpace only supports NAS_1
volume type.
*
*
* @return The type of file system volume. Currently, FinSpace only supports NAS_1
volume type.
* @see KxVolumeType
*/
public String getVolumeType() {
return this.volumeType;
}
/**
*
* The type of file system volume. Currently, FinSpace only supports NAS_1
volume type.
*
*
* @param volumeType
* The type of file system volume. Currently, FinSpace only supports NAS_1
volume type.
* @return Returns a reference to this object so that method calls can be chained together.
* @see KxVolumeType
*/
public GetKxVolumeResult withVolumeType(String volumeType) {
setVolumeType(volumeType);
return this;
}
/**
*
* The type of file system volume. Currently, FinSpace only supports NAS_1
volume type.
*
*
* @param volumeType
* The type of file system volume. Currently, FinSpace only supports NAS_1
volume type.
* @return Returns a reference to this object so that method calls can be chained together.
* @see KxVolumeType
*/
public GetKxVolumeResult withVolumeType(KxVolumeType volumeType) {
this.volumeType = volumeType.toString();
return this;
}
/**
*
* The ARN identifier of the volume.
*
*
* @param volumeArn
* The ARN identifier of the volume.
*/
public void setVolumeArn(String volumeArn) {
this.volumeArn = volumeArn;
}
/**
*
* The ARN identifier of the volume.
*
*
* @return The ARN identifier of the volume.
*/
public String getVolumeArn() {
return this.volumeArn;
}
/**
*
* The ARN identifier of the volume.
*
*
* @param volumeArn
* The ARN identifier of the volume.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetKxVolumeResult withVolumeArn(String volumeArn) {
setVolumeArn(volumeArn);
return this;
}
/**
*
* Specifies the configuration for the Network attached storage (NAS_1) file system volume.
*
*
* @param nas1Configuration
* Specifies the configuration for the Network attached storage (NAS_1) file system volume.
*/
public void setNas1Configuration(KxNAS1Configuration nas1Configuration) {
this.nas1Configuration = nas1Configuration;
}
/**
*
* Specifies the configuration for the Network attached storage (NAS_1) file system volume.
*
*
* @return Specifies the configuration for the Network attached storage (NAS_1) file system volume.
*/
public KxNAS1Configuration getNas1Configuration() {
return this.nas1Configuration;
}
/**
*
* Specifies the configuration for the Network attached storage (NAS_1) file system volume.
*
*
* @param nas1Configuration
* Specifies the configuration for the Network attached storage (NAS_1) file system volume.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetKxVolumeResult withNas1Configuration(KxNAS1Configuration nas1Configuration) {
setNas1Configuration(nas1Configuration);
return this;
}
/**
*
* The status of volume creation.
*
*
* -
*
* CREATING – The volume creation is in progress.
*
*
* -
*
* CREATE_FAILED – The volume creation has failed.
*
*
* -
*
* ACTIVE – The volume is active.
*
*
* -
*
* UPDATING – The volume is in the process of being updated.
*
*
* -
*
* UPDATE_FAILED – The update action failed.
*
*
* -
*
* UPDATED – The volume is successfully updated.
*
*
* -
*
* DELETING – The volume is in the process of being deleted.
*
*
* -
*
* DELETE_FAILED – The system failed to delete the volume.
*
*
* -
*
* DELETED – The volume is successfully deleted.
*
*
*
*
* @param status
* The status of volume creation.
*
* -
*
* CREATING – The volume creation is in progress.
*
*
* -
*
* CREATE_FAILED – The volume creation has failed.
*
*
* -
*
* ACTIVE – The volume is active.
*
*
* -
*
* UPDATING – The volume is in the process of being updated.
*
*
* -
*
* UPDATE_FAILED – The update action failed.
*
*
* -
*
* UPDATED – The volume is successfully updated.
*
*
* -
*
* DELETING – The volume is in the process of being deleted.
*
*
* -
*
* DELETE_FAILED – The system failed to delete the volume.
*
*
* -
*
* DELETED – The volume is successfully deleted.
*
*
* @see KxVolumeStatus
*/
public void setStatus(String status) {
this.status = status;
}
/**
*
* The status of volume creation.
*
*
* -
*
* CREATING – The volume creation is in progress.
*
*
* -
*
* CREATE_FAILED – The volume creation has failed.
*
*
* -
*
* ACTIVE – The volume is active.
*
*
* -
*
* UPDATING – The volume is in the process of being updated.
*
*
* -
*
* UPDATE_FAILED – The update action failed.
*
*
* -
*
* UPDATED – The volume is successfully updated.
*
*
* -
*
* DELETING – The volume is in the process of being deleted.
*
*
* -
*
* DELETE_FAILED – The system failed to delete the volume.
*
*
* -
*
* DELETED – The volume is successfully deleted.
*
*
*
*
* @return The status of volume creation.
*
* -
*
* CREATING – The volume creation is in progress.
*
*
* -
*
* CREATE_FAILED – The volume creation has failed.
*
*
* -
*
* ACTIVE – The volume is active.
*
*
* -
*
* UPDATING – The volume is in the process of being updated.
*
*
* -
*
* UPDATE_FAILED – The update action failed.
*
*
* -
*
* UPDATED – The volume is successfully updated.
*
*
* -
*
* DELETING – The volume is in the process of being deleted.
*
*
* -
*
* DELETE_FAILED – The system failed to delete the volume.
*
*
* -
*
* DELETED – The volume is successfully deleted.
*
*
* @see KxVolumeStatus
*/
public String getStatus() {
return this.status;
}
/**
*
* The status of volume creation.
*
*
* -
*
* CREATING – The volume creation is in progress.
*
*
* -
*
* CREATE_FAILED – The volume creation has failed.
*
*
* -
*
* ACTIVE – The volume is active.
*
*
* -
*
* UPDATING – The volume is in the process of being updated.
*
*
* -
*
* UPDATE_FAILED – The update action failed.
*
*
* -
*
* UPDATED – The volume is successfully updated.
*
*
* -
*
* DELETING – The volume is in the process of being deleted.
*
*
* -
*
* DELETE_FAILED – The system failed to delete the volume.
*
*
* -
*
* DELETED – The volume is successfully deleted.
*
*
*
*
* @param status
* The status of volume creation.
*
* -
*
* CREATING – The volume creation is in progress.
*
*
* -
*
* CREATE_FAILED – The volume creation has failed.
*
*
* -
*
* ACTIVE – The volume is active.
*
*
* -
*
* UPDATING – The volume is in the process of being updated.
*
*
* -
*
* UPDATE_FAILED – The update action failed.
*
*
* -
*
* UPDATED – The volume is successfully updated.
*
*
* -
*
* DELETING – The volume is in the process of being deleted.
*
*
* -
*
* DELETE_FAILED – The system failed to delete the volume.
*
*
* -
*
* DELETED – The volume is successfully deleted.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see KxVolumeStatus
*/
public GetKxVolumeResult withStatus(String status) {
setStatus(status);
return this;
}
/**
*
* The status of volume creation.
*
*
* -
*
* CREATING – The volume creation is in progress.
*
*
* -
*
* CREATE_FAILED – The volume creation has failed.
*
*
* -
*
* ACTIVE – The volume is active.
*
*
* -
*
* UPDATING – The volume is in the process of being updated.
*
*
* -
*
* UPDATE_FAILED – The update action failed.
*
*
* -
*
* UPDATED – The volume is successfully updated.
*
*
* -
*
* DELETING – The volume is in the process of being deleted.
*
*
* -
*
* DELETE_FAILED – The system failed to delete the volume.
*
*
* -
*
* DELETED – The volume is successfully deleted.
*
*
*
*
* @param status
* The status of volume creation.
*
* -
*
* CREATING – The volume creation is in progress.
*
*
* -
*
* CREATE_FAILED – The volume creation has failed.
*
*
* -
*
* ACTIVE – The volume is active.
*
*
* -
*
* UPDATING – The volume is in the process of being updated.
*
*
* -
*
* UPDATE_FAILED – The update action failed.
*
*
* -
*
* UPDATED – The volume is successfully updated.
*
*
* -
*
* DELETING – The volume is in the process of being deleted.
*
*
* -
*
* DELETE_FAILED – The system failed to delete the volume.
*
*
* -
*
* DELETED – The volume is successfully deleted.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see KxVolumeStatus
*/
public GetKxVolumeResult withStatus(KxVolumeStatus status) {
this.status = status.toString();
return this;
}
/**
*
* The error message when a failed state occurs.
*
*
* @param statusReason
* The error message when a failed state occurs.
*/
public void setStatusReason(String statusReason) {
this.statusReason = statusReason;
}
/**
*
* The error message when a failed state occurs.
*
*
* @return The error message when a failed state occurs.
*/
public String getStatusReason() {
return this.statusReason;
}
/**
*
* The error message when a failed state occurs.
*
*
* @param statusReason
* The error message when a failed state occurs.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetKxVolumeResult withStatusReason(String statusReason) {
setStatusReason(statusReason);
return this;
}
/**
*
* The timestamp at which the volume was created in FinSpace. The value is determined as epoch time in milliseconds.
* For example, the value for Monday, November 1, 2021 12:00:00 PM UTC is specified as 1635768000000.
*
*
* @param createdTimestamp
* The timestamp at which the volume was created in FinSpace. The value is determined as epoch time in
* milliseconds. For example, the value for Monday, November 1, 2021 12:00:00 PM UTC is specified as
* 1635768000000.
*/
public void setCreatedTimestamp(java.util.Date createdTimestamp) {
this.createdTimestamp = createdTimestamp;
}
/**
*
* The timestamp at which the volume was created in FinSpace. The value is determined as epoch time in milliseconds.
* For example, the value for Monday, November 1, 2021 12:00:00 PM UTC is specified as 1635768000000.
*
*
* @return The timestamp at which the volume was created in FinSpace. The value is determined as epoch time in
* milliseconds. For example, the value for Monday, November 1, 2021 12:00:00 PM UTC is specified as
* 1635768000000.
*/
public java.util.Date getCreatedTimestamp() {
return this.createdTimestamp;
}
/**
*
* The timestamp at which the volume was created in FinSpace. The value is determined as epoch time in milliseconds.
* For example, the value for Monday, November 1, 2021 12:00:00 PM UTC is specified as 1635768000000.
*
*
* @param createdTimestamp
* The timestamp at which the volume was created in FinSpace. The value is determined as epoch time in
* milliseconds. For example, the value for Monday, November 1, 2021 12:00:00 PM UTC is specified as
* 1635768000000.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetKxVolumeResult withCreatedTimestamp(java.util.Date createdTimestamp) {
setCreatedTimestamp(createdTimestamp);
return this;
}
/**
*
* A description of the volume.
*
*
* @param description
* A description of the volume.
*/
public void setDescription(String description) {
this.description = description;
}
/**
*
* A description of the volume.
*
*
* @return A description of the volume.
*/
public String getDescription() {
return this.description;
}
/**
*
* A description of the volume.
*
*
* @param description
* A description of the volume.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetKxVolumeResult withDescription(String description) {
setDescription(description);
return this;
}
/**
*
* The number of availability zones you want to assign per volume. Currently, FinSpace only supports
* SINGLE
for volumes. This places dataview in a single AZ.
*
*
* @param azMode
* The number of availability zones you want to assign per volume. Currently, FinSpace only supports
* SINGLE
for volumes. This places dataview in a single AZ.
* @see KxAzMode
*/
public void setAzMode(String azMode) {
this.azMode = azMode;
}
/**
*
* The number of availability zones you want to assign per volume. Currently, FinSpace only supports
* SINGLE
for volumes. This places dataview in a single AZ.
*
*
* @return The number of availability zones you want to assign per volume. Currently, FinSpace only supports
* SINGLE
for volumes. This places dataview in a single AZ.
* @see KxAzMode
*/
public String getAzMode() {
return this.azMode;
}
/**
*
* The number of availability zones you want to assign per volume. Currently, FinSpace only supports
* SINGLE
for volumes. This places dataview in a single AZ.
*
*
* @param azMode
* The number of availability zones you want to assign per volume. Currently, FinSpace only supports
* SINGLE
for volumes. This places dataview in a single AZ.
* @return Returns a reference to this object so that method calls can be chained together.
* @see KxAzMode
*/
public GetKxVolumeResult withAzMode(String azMode) {
setAzMode(azMode);
return this;
}
/**
*
* The number of availability zones you want to assign per volume. Currently, FinSpace only supports
* SINGLE
for volumes. This places dataview in a single AZ.
*
*
* @param azMode
* The number of availability zones you want to assign per volume. Currently, FinSpace only supports
* SINGLE
for volumes. This places dataview in a single AZ.
* @return Returns a reference to this object so that method calls can be chained together.
* @see KxAzMode
*/
public GetKxVolumeResult withAzMode(KxAzMode azMode) {
this.azMode = azMode.toString();
return this;
}
/**
*
* The identifier of the availability zones.
*
*
* @return The identifier of the availability zones.
*/
public java.util.List getAvailabilityZoneIds() {
return availabilityZoneIds;
}
/**
*
* The identifier of the availability zones.
*
*
* @param availabilityZoneIds
* The identifier of the availability zones.
*/
public void setAvailabilityZoneIds(java.util.Collection availabilityZoneIds) {
if (availabilityZoneIds == null) {
this.availabilityZoneIds = null;
return;
}
this.availabilityZoneIds = new java.util.ArrayList(availabilityZoneIds);
}
/**
*
* The identifier of the availability zones.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setAvailabilityZoneIds(java.util.Collection)} or {@link #withAvailabilityZoneIds(java.util.Collection)}
* if you want to override the existing values.
*
*
* @param availabilityZoneIds
* The identifier of the availability zones.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetKxVolumeResult withAvailabilityZoneIds(String... availabilityZoneIds) {
if (this.availabilityZoneIds == null) {
setAvailabilityZoneIds(new java.util.ArrayList(availabilityZoneIds.length));
}
for (String ele : availabilityZoneIds) {
this.availabilityZoneIds.add(ele);
}
return this;
}
/**
*
* The identifier of the availability zones.
*
*
* @param availabilityZoneIds
* The identifier of the availability zones.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetKxVolumeResult withAvailabilityZoneIds(java.util.Collection availabilityZoneIds) {
setAvailabilityZoneIds(availabilityZoneIds);
return this;
}
/**
*
* The last time that the volume was updated in FinSpace. The value is determined as epoch time in milliseconds. For
* example, the value for Monday, November 1, 2021 12:00:00 PM UTC is specified as 1635768000000.
*
*
* @param lastModifiedTimestamp
* The last time that the volume was updated in FinSpace. The value is determined as epoch time in
* milliseconds. For example, the value for Monday, November 1, 2021 12:00:00 PM UTC is specified as
* 1635768000000.
*/
public void setLastModifiedTimestamp(java.util.Date lastModifiedTimestamp) {
this.lastModifiedTimestamp = lastModifiedTimestamp;
}
/**
*
* The last time that the volume was updated in FinSpace. The value is determined as epoch time in milliseconds. For
* example, the value for Monday, November 1, 2021 12:00:00 PM UTC is specified as 1635768000000.
*
*
* @return The last time that the volume was updated in FinSpace. The value is determined as epoch time in
* milliseconds. For example, the value for Monday, November 1, 2021 12:00:00 PM UTC is specified as
* 1635768000000.
*/
public java.util.Date getLastModifiedTimestamp() {
return this.lastModifiedTimestamp;
}
/**
*
* The last time that the volume was updated in FinSpace. The value is determined as epoch time in milliseconds. For
* example, the value for Monday, November 1, 2021 12:00:00 PM UTC is specified as 1635768000000.
*
*
* @param lastModifiedTimestamp
* The last time that the volume was updated in FinSpace. The value is determined as epoch time in
* milliseconds. For example, the value for Monday, November 1, 2021 12:00:00 PM UTC is specified as
* 1635768000000.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetKxVolumeResult withLastModifiedTimestamp(java.util.Date lastModifiedTimestamp) {
setLastModifiedTimestamp(lastModifiedTimestamp);
return this;
}
/**
*
* A list of cluster identifiers that a volume is attached to.
*
*
* @return A list of cluster identifiers that a volume is attached to.
*/
public java.util.List getAttachedClusters() {
return attachedClusters;
}
/**
*
* A list of cluster identifiers that a volume is attached to.
*
*
* @param attachedClusters
* A list of cluster identifiers that a volume is attached to.
*/
public void setAttachedClusters(java.util.Collection attachedClusters) {
if (attachedClusters == null) {
this.attachedClusters = null;
return;
}
this.attachedClusters = new java.util.ArrayList(attachedClusters);
}
/**
*
* A list of cluster identifiers that a volume is attached to.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setAttachedClusters(java.util.Collection)} or {@link #withAttachedClusters(java.util.Collection)} if you
* want to override the existing values.
*
*
* @param attachedClusters
* A list of cluster identifiers that a volume is attached to.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetKxVolumeResult withAttachedClusters(KxAttachedCluster... attachedClusters) {
if (this.attachedClusters == null) {
setAttachedClusters(new java.util.ArrayList(attachedClusters.length));
}
for (KxAttachedCluster ele : attachedClusters) {
this.attachedClusters.add(ele);
}
return this;
}
/**
*
* A list of cluster identifiers that a volume is attached to.
*
*
* @param attachedClusters
* A list of cluster identifiers that a volume is attached to.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetKxVolumeResult withAttachedClusters(java.util.Collection attachedClusters) {
setAttachedClusters(attachedClusters);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getEnvironmentId() != null)
sb.append("EnvironmentId: ").append(getEnvironmentId()).append(",");
if (getVolumeName() != null)
sb.append("VolumeName: ").append(getVolumeName()).append(",");
if (getVolumeType() != null)
sb.append("VolumeType: ").append(getVolumeType()).append(",");
if (getVolumeArn() != null)
sb.append("VolumeArn: ").append(getVolumeArn()).append(",");
if (getNas1Configuration() != null)
sb.append("Nas1Configuration: ").append(getNas1Configuration()).append(",");
if (getStatus() != null)
sb.append("Status: ").append(getStatus()).append(",");
if (getStatusReason() != null)
sb.append("StatusReason: ").append(getStatusReason()).append(",");
if (getCreatedTimestamp() != null)
sb.append("CreatedTimestamp: ").append(getCreatedTimestamp()).append(",");
if (getDescription() != null)
sb.append("Description: ").append(getDescription()).append(",");
if (getAzMode() != null)
sb.append("AzMode: ").append(getAzMode()).append(",");
if (getAvailabilityZoneIds() != null)
sb.append("AvailabilityZoneIds: ").append(getAvailabilityZoneIds()).append(",");
if (getLastModifiedTimestamp() != null)
sb.append("LastModifiedTimestamp: ").append(getLastModifiedTimestamp()).append(",");
if (getAttachedClusters() != null)
sb.append("AttachedClusters: ").append(getAttachedClusters());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof GetKxVolumeResult == false)
return false;
GetKxVolumeResult other = (GetKxVolumeResult) obj;
if (other.getEnvironmentId() == null ^ this.getEnvironmentId() == null)
return false;
if (other.getEnvironmentId() != null && other.getEnvironmentId().equals(this.getEnvironmentId()) == false)
return false;
if (other.getVolumeName() == null ^ this.getVolumeName() == null)
return false;
if (other.getVolumeName() != null && other.getVolumeName().equals(this.getVolumeName()) == false)
return false;
if (other.getVolumeType() == null ^ this.getVolumeType() == null)
return false;
if (other.getVolumeType() != null && other.getVolumeType().equals(this.getVolumeType()) == false)
return false;
if (other.getVolumeArn() == null ^ this.getVolumeArn() == null)
return false;
if (other.getVolumeArn() != null && other.getVolumeArn().equals(this.getVolumeArn()) == false)
return false;
if (other.getNas1Configuration() == null ^ this.getNas1Configuration() == null)
return false;
if (other.getNas1Configuration() != null && other.getNas1Configuration().equals(this.getNas1Configuration()) == false)
return false;
if (other.getStatus() == null ^ this.getStatus() == null)
return false;
if (other.getStatus() != null && other.getStatus().equals(this.getStatus()) == false)
return false;
if (other.getStatusReason() == null ^ this.getStatusReason() == null)
return false;
if (other.getStatusReason() != null && other.getStatusReason().equals(this.getStatusReason()) == false)
return false;
if (other.getCreatedTimestamp() == null ^ this.getCreatedTimestamp() == null)
return false;
if (other.getCreatedTimestamp() != null && other.getCreatedTimestamp().equals(this.getCreatedTimestamp()) == false)
return false;
if (other.getDescription() == null ^ this.getDescription() == null)
return false;
if (other.getDescription() != null && other.getDescription().equals(this.getDescription()) == false)
return false;
if (other.getAzMode() == null ^ this.getAzMode() == null)
return false;
if (other.getAzMode() != null && other.getAzMode().equals(this.getAzMode()) == false)
return false;
if (other.getAvailabilityZoneIds() == null ^ this.getAvailabilityZoneIds() == null)
return false;
if (other.getAvailabilityZoneIds() != null && other.getAvailabilityZoneIds().equals(this.getAvailabilityZoneIds()) == false)
return false;
if (other.getLastModifiedTimestamp() == null ^ this.getLastModifiedTimestamp() == null)
return false;
if (other.getLastModifiedTimestamp() != null && other.getLastModifiedTimestamp().equals(this.getLastModifiedTimestamp()) == false)
return false;
if (other.getAttachedClusters() == null ^ this.getAttachedClusters() == null)
return false;
if (other.getAttachedClusters() != null && other.getAttachedClusters().equals(this.getAttachedClusters()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getEnvironmentId() == null) ? 0 : getEnvironmentId().hashCode());
hashCode = prime * hashCode + ((getVolumeName() == null) ? 0 : getVolumeName().hashCode());
hashCode = prime * hashCode + ((getVolumeType() == null) ? 0 : getVolumeType().hashCode());
hashCode = prime * hashCode + ((getVolumeArn() == null) ? 0 : getVolumeArn().hashCode());
hashCode = prime * hashCode + ((getNas1Configuration() == null) ? 0 : getNas1Configuration().hashCode());
hashCode = prime * hashCode + ((getStatus() == null) ? 0 : getStatus().hashCode());
hashCode = prime * hashCode + ((getStatusReason() == null) ? 0 : getStatusReason().hashCode());
hashCode = prime * hashCode + ((getCreatedTimestamp() == null) ? 0 : getCreatedTimestamp().hashCode());
hashCode = prime * hashCode + ((getDescription() == null) ? 0 : getDescription().hashCode());
hashCode = prime * hashCode + ((getAzMode() == null) ? 0 : getAzMode().hashCode());
hashCode = prime * hashCode + ((getAvailabilityZoneIds() == null) ? 0 : getAvailabilityZoneIds().hashCode());
hashCode = prime * hashCode + ((getLastModifiedTimestamp() == null) ? 0 : getLastModifiedTimestamp().hashCode());
hashCode = prime * hashCode + ((getAttachedClusters() == null) ? 0 : getAttachedClusters().hashCode());
return hashCode;
}
@Override
public GetKxVolumeResult clone() {
try {
return (GetKxVolumeResult) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
}