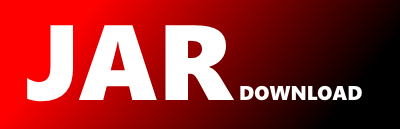
com.amazonaws.services.finspace.model.KxDataviewSegmentConfiguration Maven / Gradle / Ivy
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.finspace.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* The configuration that contains the database path of the data that you want to place on each selected volume. Each
* segment must have a unique database path for each volume. If you do not explicitly specify any database path for a
* volume, they are accessible from the cluster through the default S3/object store segment.
*
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class KxDataviewSegmentConfiguration implements Serializable, Cloneable, StructuredPojo {
/**
*
* The database path of the data that you want to place on each selected volume for the segment. Each segment must
* have a unique database path for each volume.
*
*/
private java.util.List dbPaths;
/**
*
* The name of the volume where you want to add data.
*
*/
private String volumeName;
/**
*
* Enables on-demand caching on the selected database path when a particular file or a column of the database is
* accessed. When on demand caching is True, dataviews perform minimal loading of files on the filesystem as
* needed. When it is set to False, everything is cached. The default value is False.
*
*/
private Boolean onDemand;
/**
*
* The database path of the data that you want to place on each selected volume for the segment. Each segment must
* have a unique database path for each volume.
*
*
* @return The database path of the data that you want to place on each selected volume for the segment. Each
* segment must have a unique database path for each volume.
*/
public java.util.List getDbPaths() {
return dbPaths;
}
/**
*
* The database path of the data that you want to place on each selected volume for the segment. Each segment must
* have a unique database path for each volume.
*
*
* @param dbPaths
* The database path of the data that you want to place on each selected volume for the segment. Each segment
* must have a unique database path for each volume.
*/
public void setDbPaths(java.util.Collection dbPaths) {
if (dbPaths == null) {
this.dbPaths = null;
return;
}
this.dbPaths = new java.util.ArrayList(dbPaths);
}
/**
*
* The database path of the data that you want to place on each selected volume for the segment. Each segment must
* have a unique database path for each volume.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setDbPaths(java.util.Collection)} or {@link #withDbPaths(java.util.Collection)} if you want to override
* the existing values.
*
*
* @param dbPaths
* The database path of the data that you want to place on each selected volume for the segment. Each segment
* must have a unique database path for each volume.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public KxDataviewSegmentConfiguration withDbPaths(String... dbPaths) {
if (this.dbPaths == null) {
setDbPaths(new java.util.ArrayList(dbPaths.length));
}
for (String ele : dbPaths) {
this.dbPaths.add(ele);
}
return this;
}
/**
*
* The database path of the data that you want to place on each selected volume for the segment. Each segment must
* have a unique database path for each volume.
*
*
* @param dbPaths
* The database path of the data that you want to place on each selected volume for the segment. Each segment
* must have a unique database path for each volume.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public KxDataviewSegmentConfiguration withDbPaths(java.util.Collection dbPaths) {
setDbPaths(dbPaths);
return this;
}
/**
*
* The name of the volume where you want to add data.
*
*
* @param volumeName
* The name of the volume where you want to add data.
*/
public void setVolumeName(String volumeName) {
this.volumeName = volumeName;
}
/**
*
* The name of the volume where you want to add data.
*
*
* @return The name of the volume where you want to add data.
*/
public String getVolumeName() {
return this.volumeName;
}
/**
*
* The name of the volume where you want to add data.
*
*
* @param volumeName
* The name of the volume where you want to add data.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public KxDataviewSegmentConfiguration withVolumeName(String volumeName) {
setVolumeName(volumeName);
return this;
}
/**
*
* Enables on-demand caching on the selected database path when a particular file or a column of the database is
* accessed. When on demand caching is True, dataviews perform minimal loading of files on the filesystem as
* needed. When it is set to False, everything is cached. The default value is False.
*
*
* @param onDemand
* Enables on-demand caching on the selected database path when a particular file or a column of the database
* is accessed. When on demand caching is True, dataviews perform minimal loading of files on the
* filesystem as needed. When it is set to False, everything is cached. The default value is
* False.
*/
public void setOnDemand(Boolean onDemand) {
this.onDemand = onDemand;
}
/**
*
* Enables on-demand caching on the selected database path when a particular file or a column of the database is
* accessed. When on demand caching is True, dataviews perform minimal loading of files on the filesystem as
* needed. When it is set to False, everything is cached. The default value is False.
*
*
* @return Enables on-demand caching on the selected database path when a particular file or a column of the
* database is accessed. When on demand caching is True, dataviews perform minimal loading of files
* on the filesystem as needed. When it is set to False, everything is cached. The default value is
* False.
*/
public Boolean getOnDemand() {
return this.onDemand;
}
/**
*
* Enables on-demand caching on the selected database path when a particular file or a column of the database is
* accessed. When on demand caching is True, dataviews perform minimal loading of files on the filesystem as
* needed. When it is set to False, everything is cached. The default value is False.
*
*
* @param onDemand
* Enables on-demand caching on the selected database path when a particular file or a column of the database
* is accessed. When on demand caching is True, dataviews perform minimal loading of files on the
* filesystem as needed. When it is set to False, everything is cached. The default value is
* False.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public KxDataviewSegmentConfiguration withOnDemand(Boolean onDemand) {
setOnDemand(onDemand);
return this;
}
/**
*
* Enables on-demand caching on the selected database path when a particular file or a column of the database is
* accessed. When on demand caching is True, dataviews perform minimal loading of files on the filesystem as
* needed. When it is set to False, everything is cached. The default value is False.
*
*
* @return Enables on-demand caching on the selected database path when a particular file or a column of the
* database is accessed. When on demand caching is True, dataviews perform minimal loading of files
* on the filesystem as needed. When it is set to False, everything is cached. The default value is
* False.
*/
public Boolean isOnDemand() {
return this.onDemand;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getDbPaths() != null)
sb.append("DbPaths: ").append(getDbPaths()).append(",");
if (getVolumeName() != null)
sb.append("VolumeName: ").append(getVolumeName()).append(",");
if (getOnDemand() != null)
sb.append("OnDemand: ").append(getOnDemand());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof KxDataviewSegmentConfiguration == false)
return false;
KxDataviewSegmentConfiguration other = (KxDataviewSegmentConfiguration) obj;
if (other.getDbPaths() == null ^ this.getDbPaths() == null)
return false;
if (other.getDbPaths() != null && other.getDbPaths().equals(this.getDbPaths()) == false)
return false;
if (other.getVolumeName() == null ^ this.getVolumeName() == null)
return false;
if (other.getVolumeName() != null && other.getVolumeName().equals(this.getVolumeName()) == false)
return false;
if (other.getOnDemand() == null ^ this.getOnDemand() == null)
return false;
if (other.getOnDemand() != null && other.getOnDemand().equals(this.getOnDemand()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getDbPaths() == null) ? 0 : getDbPaths().hashCode());
hashCode = prime * hashCode + ((getVolumeName() == null) ? 0 : getVolumeName().hashCode());
hashCode = prime * hashCode + ((getOnDemand() == null) ? 0 : getOnDemand().hashCode());
return hashCode;
}
@Override
public KxDataviewSegmentConfiguration clone() {
try {
return (KxDataviewSegmentConfiguration) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.finspace.model.transform.KxDataviewSegmentConfigurationMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}