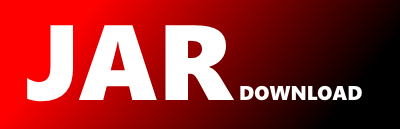
com.amazonaws.services.finspace.model.UpdateKxDataviewResult Maven / Gradle / Ivy
Show all versions of aws-java-sdk-finspace Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.finspace.model;
import java.io.Serializable;
import javax.annotation.Generated;
/**
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class UpdateKxDataviewResult extends com.amazonaws.AmazonWebServiceResult implements Serializable, Cloneable {
/**
*
* A unique identifier for the kdb environment, where you want to update the dataview.
*
*/
private String environmentId;
/**
*
* The name of the database.
*
*/
private String databaseName;
/**
*
* The name of the database under which the dataview was created.
*
*/
private String dataviewName;
/**
*
* The number of availability zones you want to assign per volume. Currently, FinSpace only supports
* SINGLE
for volumes. This places dataview in a single AZ.
*
*/
private String azMode;
/**
*
* The identifier of the availability zones.
*
*/
private String availabilityZoneId;
/**
*
* A unique identifier for the changeset.
*
*/
private String changesetId;
/**
*
* The configuration that contains the database path of the data that you want to place on each selected volume.
* Each segment must have a unique database path for each volume. If you do not explicitly specify any database path
* for a volume, they are accessible from the cluster through the default S3/object store segment.
*
*/
private java.util.List segmentConfigurations;
/**
*
* The current active changeset versions of the database on the given dataview.
*
*/
private java.util.List activeVersions;
/**
*
* The status of dataview creation.
*
*
* -
*
* CREATING
– The dataview creation is in progress.
*
*
* -
*
* UPDATING
– The dataview is in the process of being updated.
*
*
* -
*
* ACTIVE
– The dataview is active.
*
*
*
*/
private String status;
/**
*
* The option to specify whether you want to apply all the future additions and corrections automatically to the
* dataview when new changesets are ingested. The default value is false.
*
*/
private Boolean autoUpdate;
/**
*
* Returns True if the dataview is created as writeable and False otherwise.
*
*/
private Boolean readWrite;
/**
*
* A description of the dataview.
*
*/
private String description;
/**
*
* The timestamp at which the dataview was created in FinSpace. The value is determined as epoch time in
* milliseconds. For example, the value for Monday, November 1, 2021 12:00:00 PM UTC is specified as 1635768000000.
*
*/
private java.util.Date createdTimestamp;
/**
*
* The last time that the dataview was updated in FinSpace. The value is determined as epoch time in milliseconds.
* For example, the value for Monday, November 1, 2021 12:00:00 PM UTC is specified as 1635768000000.
*
*/
private java.util.Date lastModifiedTimestamp;
/**
*
* A unique identifier for the kdb environment, where you want to update the dataview.
*
*
* @param environmentId
* A unique identifier for the kdb environment, where you want to update the dataview.
*/
public void setEnvironmentId(String environmentId) {
this.environmentId = environmentId;
}
/**
*
* A unique identifier for the kdb environment, where you want to update the dataview.
*
*
* @return A unique identifier for the kdb environment, where you want to update the dataview.
*/
public String getEnvironmentId() {
return this.environmentId;
}
/**
*
* A unique identifier for the kdb environment, where you want to update the dataview.
*
*
* @param environmentId
* A unique identifier for the kdb environment, where you want to update the dataview.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateKxDataviewResult withEnvironmentId(String environmentId) {
setEnvironmentId(environmentId);
return this;
}
/**
*
* The name of the database.
*
*
* @param databaseName
* The name of the database.
*/
public void setDatabaseName(String databaseName) {
this.databaseName = databaseName;
}
/**
*
* The name of the database.
*
*
* @return The name of the database.
*/
public String getDatabaseName() {
return this.databaseName;
}
/**
*
* The name of the database.
*
*
* @param databaseName
* The name of the database.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateKxDataviewResult withDatabaseName(String databaseName) {
setDatabaseName(databaseName);
return this;
}
/**
*
* The name of the database under which the dataview was created.
*
*
* @param dataviewName
* The name of the database under which the dataview was created.
*/
public void setDataviewName(String dataviewName) {
this.dataviewName = dataviewName;
}
/**
*
* The name of the database under which the dataview was created.
*
*
* @return The name of the database under which the dataview was created.
*/
public String getDataviewName() {
return this.dataviewName;
}
/**
*
* The name of the database under which the dataview was created.
*
*
* @param dataviewName
* The name of the database under which the dataview was created.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateKxDataviewResult withDataviewName(String dataviewName) {
setDataviewName(dataviewName);
return this;
}
/**
*
* The number of availability zones you want to assign per volume. Currently, FinSpace only supports
* SINGLE
for volumes. This places dataview in a single AZ.
*
*
* @param azMode
* The number of availability zones you want to assign per volume. Currently, FinSpace only supports
* SINGLE
for volumes. This places dataview in a single AZ.
* @see KxAzMode
*/
public void setAzMode(String azMode) {
this.azMode = azMode;
}
/**
*
* The number of availability zones you want to assign per volume. Currently, FinSpace only supports
* SINGLE
for volumes. This places dataview in a single AZ.
*
*
* @return The number of availability zones you want to assign per volume. Currently, FinSpace only supports
* SINGLE
for volumes. This places dataview in a single AZ.
* @see KxAzMode
*/
public String getAzMode() {
return this.azMode;
}
/**
*
* The number of availability zones you want to assign per volume. Currently, FinSpace only supports
* SINGLE
for volumes. This places dataview in a single AZ.
*
*
* @param azMode
* The number of availability zones you want to assign per volume. Currently, FinSpace only supports
* SINGLE
for volumes. This places dataview in a single AZ.
* @return Returns a reference to this object so that method calls can be chained together.
* @see KxAzMode
*/
public UpdateKxDataviewResult withAzMode(String azMode) {
setAzMode(azMode);
return this;
}
/**
*
* The number of availability zones you want to assign per volume. Currently, FinSpace only supports
* SINGLE
for volumes. This places dataview in a single AZ.
*
*
* @param azMode
* The number of availability zones you want to assign per volume. Currently, FinSpace only supports
* SINGLE
for volumes. This places dataview in a single AZ.
* @return Returns a reference to this object so that method calls can be chained together.
* @see KxAzMode
*/
public UpdateKxDataviewResult withAzMode(KxAzMode azMode) {
this.azMode = azMode.toString();
return this;
}
/**
*
* The identifier of the availability zones.
*
*
* @param availabilityZoneId
* The identifier of the availability zones.
*/
public void setAvailabilityZoneId(String availabilityZoneId) {
this.availabilityZoneId = availabilityZoneId;
}
/**
*
* The identifier of the availability zones.
*
*
* @return The identifier of the availability zones.
*/
public String getAvailabilityZoneId() {
return this.availabilityZoneId;
}
/**
*
* The identifier of the availability zones.
*
*
* @param availabilityZoneId
* The identifier of the availability zones.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateKxDataviewResult withAvailabilityZoneId(String availabilityZoneId) {
setAvailabilityZoneId(availabilityZoneId);
return this;
}
/**
*
* A unique identifier for the changeset.
*
*
* @param changesetId
* A unique identifier for the changeset.
*/
public void setChangesetId(String changesetId) {
this.changesetId = changesetId;
}
/**
*
* A unique identifier for the changeset.
*
*
* @return A unique identifier for the changeset.
*/
public String getChangesetId() {
return this.changesetId;
}
/**
*
* A unique identifier for the changeset.
*
*
* @param changesetId
* A unique identifier for the changeset.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateKxDataviewResult withChangesetId(String changesetId) {
setChangesetId(changesetId);
return this;
}
/**
*
* The configuration that contains the database path of the data that you want to place on each selected volume.
* Each segment must have a unique database path for each volume. If you do not explicitly specify any database path
* for a volume, they are accessible from the cluster through the default S3/object store segment.
*
*
* @return The configuration that contains the database path of the data that you want to place on each selected
* volume. Each segment must have a unique database path for each volume. If you do not explicitly specify
* any database path for a volume, they are accessible from the cluster through the default S3/object store
* segment.
*/
public java.util.List getSegmentConfigurations() {
return segmentConfigurations;
}
/**
*
* The configuration that contains the database path of the data that you want to place on each selected volume.
* Each segment must have a unique database path for each volume. If you do not explicitly specify any database path
* for a volume, they are accessible from the cluster through the default S3/object store segment.
*
*
* @param segmentConfigurations
* The configuration that contains the database path of the data that you want to place on each selected
* volume. Each segment must have a unique database path for each volume. If you do not explicitly specify
* any database path for a volume, they are accessible from the cluster through the default S3/object store
* segment.
*/
public void setSegmentConfigurations(java.util.Collection segmentConfigurations) {
if (segmentConfigurations == null) {
this.segmentConfigurations = null;
return;
}
this.segmentConfigurations = new java.util.ArrayList(segmentConfigurations);
}
/**
*
* The configuration that contains the database path of the data that you want to place on each selected volume.
* Each segment must have a unique database path for each volume. If you do not explicitly specify any database path
* for a volume, they are accessible from the cluster through the default S3/object store segment.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setSegmentConfigurations(java.util.Collection)} or
* {@link #withSegmentConfigurations(java.util.Collection)} if you want to override the existing values.
*
*
* @param segmentConfigurations
* The configuration that contains the database path of the data that you want to place on each selected
* volume. Each segment must have a unique database path for each volume. If you do not explicitly specify
* any database path for a volume, they are accessible from the cluster through the default S3/object store
* segment.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateKxDataviewResult withSegmentConfigurations(KxDataviewSegmentConfiguration... segmentConfigurations) {
if (this.segmentConfigurations == null) {
setSegmentConfigurations(new java.util.ArrayList(segmentConfigurations.length));
}
for (KxDataviewSegmentConfiguration ele : segmentConfigurations) {
this.segmentConfigurations.add(ele);
}
return this;
}
/**
*
* The configuration that contains the database path of the data that you want to place on each selected volume.
* Each segment must have a unique database path for each volume. If you do not explicitly specify any database path
* for a volume, they are accessible from the cluster through the default S3/object store segment.
*
*
* @param segmentConfigurations
* The configuration that contains the database path of the data that you want to place on each selected
* volume. Each segment must have a unique database path for each volume. If you do not explicitly specify
* any database path for a volume, they are accessible from the cluster through the default S3/object store
* segment.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateKxDataviewResult withSegmentConfigurations(java.util.Collection segmentConfigurations) {
setSegmentConfigurations(segmentConfigurations);
return this;
}
/**
*
* The current active changeset versions of the database on the given dataview.
*
*
* @return The current active changeset versions of the database on the given dataview.
*/
public java.util.List getActiveVersions() {
return activeVersions;
}
/**
*
* The current active changeset versions of the database on the given dataview.
*
*
* @param activeVersions
* The current active changeset versions of the database on the given dataview.
*/
public void setActiveVersions(java.util.Collection activeVersions) {
if (activeVersions == null) {
this.activeVersions = null;
return;
}
this.activeVersions = new java.util.ArrayList(activeVersions);
}
/**
*
* The current active changeset versions of the database on the given dataview.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setActiveVersions(java.util.Collection)} or {@link #withActiveVersions(java.util.Collection)} if you want
* to override the existing values.
*
*
* @param activeVersions
* The current active changeset versions of the database on the given dataview.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateKxDataviewResult withActiveVersions(KxDataviewActiveVersion... activeVersions) {
if (this.activeVersions == null) {
setActiveVersions(new java.util.ArrayList(activeVersions.length));
}
for (KxDataviewActiveVersion ele : activeVersions) {
this.activeVersions.add(ele);
}
return this;
}
/**
*
* The current active changeset versions of the database on the given dataview.
*
*
* @param activeVersions
* The current active changeset versions of the database on the given dataview.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateKxDataviewResult withActiveVersions(java.util.Collection activeVersions) {
setActiveVersions(activeVersions);
return this;
}
/**
*
* The status of dataview creation.
*
*
* -
*
* CREATING
– The dataview creation is in progress.
*
*
* -
*
* UPDATING
– The dataview is in the process of being updated.
*
*
* -
*
* ACTIVE
– The dataview is active.
*
*
*
*
* @param status
* The status of dataview creation.
*
* -
*
* CREATING
– The dataview creation is in progress.
*
*
* -
*
* UPDATING
– The dataview is in the process of being updated.
*
*
* -
*
* ACTIVE
– The dataview is active.
*
*
* @see KxDataviewStatus
*/
public void setStatus(String status) {
this.status = status;
}
/**
*
* The status of dataview creation.
*
*
* -
*
* CREATING
– The dataview creation is in progress.
*
*
* -
*
* UPDATING
– The dataview is in the process of being updated.
*
*
* -
*
* ACTIVE
– The dataview is active.
*
*
*
*
* @return The status of dataview creation.
*
* -
*
* CREATING
– The dataview creation is in progress.
*
*
* -
*
* UPDATING
– The dataview is in the process of being updated.
*
*
* -
*
* ACTIVE
– The dataview is active.
*
*
* @see KxDataviewStatus
*/
public String getStatus() {
return this.status;
}
/**
*
* The status of dataview creation.
*
*
* -
*
* CREATING
– The dataview creation is in progress.
*
*
* -
*
* UPDATING
– The dataview is in the process of being updated.
*
*
* -
*
* ACTIVE
– The dataview is active.
*
*
*
*
* @param status
* The status of dataview creation.
*
* -
*
* CREATING
– The dataview creation is in progress.
*
*
* -
*
* UPDATING
– The dataview is in the process of being updated.
*
*
* -
*
* ACTIVE
– The dataview is active.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see KxDataviewStatus
*/
public UpdateKxDataviewResult withStatus(String status) {
setStatus(status);
return this;
}
/**
*
* The status of dataview creation.
*
*
* -
*
* CREATING
– The dataview creation is in progress.
*
*
* -
*
* UPDATING
– The dataview is in the process of being updated.
*
*
* -
*
* ACTIVE
– The dataview is active.
*
*
*
*
* @param status
* The status of dataview creation.
*
* -
*
* CREATING
– The dataview creation is in progress.
*
*
* -
*
* UPDATING
– The dataview is in the process of being updated.
*
*
* -
*
* ACTIVE
– The dataview is active.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see KxDataviewStatus
*/
public UpdateKxDataviewResult withStatus(KxDataviewStatus status) {
this.status = status.toString();
return this;
}
/**
*
* The option to specify whether you want to apply all the future additions and corrections automatically to the
* dataview when new changesets are ingested. The default value is false.
*
*
* @param autoUpdate
* The option to specify whether you want to apply all the future additions and corrections automatically to
* the dataview when new changesets are ingested. The default value is false.
*/
public void setAutoUpdate(Boolean autoUpdate) {
this.autoUpdate = autoUpdate;
}
/**
*
* The option to specify whether you want to apply all the future additions and corrections automatically to the
* dataview when new changesets are ingested. The default value is false.
*
*
* @return The option to specify whether you want to apply all the future additions and corrections automatically to
* the dataview when new changesets are ingested. The default value is false.
*/
public Boolean getAutoUpdate() {
return this.autoUpdate;
}
/**
*
* The option to specify whether you want to apply all the future additions and corrections automatically to the
* dataview when new changesets are ingested. The default value is false.
*
*
* @param autoUpdate
* The option to specify whether you want to apply all the future additions and corrections automatically to
* the dataview when new changesets are ingested. The default value is false.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateKxDataviewResult withAutoUpdate(Boolean autoUpdate) {
setAutoUpdate(autoUpdate);
return this;
}
/**
*
* The option to specify whether you want to apply all the future additions and corrections automatically to the
* dataview when new changesets are ingested. The default value is false.
*
*
* @return The option to specify whether you want to apply all the future additions and corrections automatically to
* the dataview when new changesets are ingested. The default value is false.
*/
public Boolean isAutoUpdate() {
return this.autoUpdate;
}
/**
*
* Returns True if the dataview is created as writeable and False otherwise.
*
*
* @param readWrite
* Returns True if the dataview is created as writeable and False otherwise.
*/
public void setReadWrite(Boolean readWrite) {
this.readWrite = readWrite;
}
/**
*
* Returns True if the dataview is created as writeable and False otherwise.
*
*
* @return Returns True if the dataview is created as writeable and False otherwise.
*/
public Boolean getReadWrite() {
return this.readWrite;
}
/**
*
* Returns True if the dataview is created as writeable and False otherwise.
*
*
* @param readWrite
* Returns True if the dataview is created as writeable and False otherwise.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateKxDataviewResult withReadWrite(Boolean readWrite) {
setReadWrite(readWrite);
return this;
}
/**
*
* Returns True if the dataview is created as writeable and False otherwise.
*
*
* @return Returns True if the dataview is created as writeable and False otherwise.
*/
public Boolean isReadWrite() {
return this.readWrite;
}
/**
*
* A description of the dataview.
*
*
* @param description
* A description of the dataview.
*/
public void setDescription(String description) {
this.description = description;
}
/**
*
* A description of the dataview.
*
*
* @return A description of the dataview.
*/
public String getDescription() {
return this.description;
}
/**
*
* A description of the dataview.
*
*
* @param description
* A description of the dataview.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateKxDataviewResult withDescription(String description) {
setDescription(description);
return this;
}
/**
*
* The timestamp at which the dataview was created in FinSpace. The value is determined as epoch time in
* milliseconds. For example, the value for Monday, November 1, 2021 12:00:00 PM UTC is specified as 1635768000000.
*
*
* @param createdTimestamp
* The timestamp at which the dataview was created in FinSpace. The value is determined as epoch time in
* milliseconds. For example, the value for Monday, November 1, 2021 12:00:00 PM UTC is specified as
* 1635768000000.
*/
public void setCreatedTimestamp(java.util.Date createdTimestamp) {
this.createdTimestamp = createdTimestamp;
}
/**
*
* The timestamp at which the dataview was created in FinSpace. The value is determined as epoch time in
* milliseconds. For example, the value for Monday, November 1, 2021 12:00:00 PM UTC is specified as 1635768000000.
*
*
* @return The timestamp at which the dataview was created in FinSpace. The value is determined as epoch time in
* milliseconds. For example, the value for Monday, November 1, 2021 12:00:00 PM UTC is specified as
* 1635768000000.
*/
public java.util.Date getCreatedTimestamp() {
return this.createdTimestamp;
}
/**
*
* The timestamp at which the dataview was created in FinSpace. The value is determined as epoch time in
* milliseconds. For example, the value for Monday, November 1, 2021 12:00:00 PM UTC is specified as 1635768000000.
*
*
* @param createdTimestamp
* The timestamp at which the dataview was created in FinSpace. The value is determined as epoch time in
* milliseconds. For example, the value for Monday, November 1, 2021 12:00:00 PM UTC is specified as
* 1635768000000.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateKxDataviewResult withCreatedTimestamp(java.util.Date createdTimestamp) {
setCreatedTimestamp(createdTimestamp);
return this;
}
/**
*
* The last time that the dataview was updated in FinSpace. The value is determined as epoch time in milliseconds.
* For example, the value for Monday, November 1, 2021 12:00:00 PM UTC is specified as 1635768000000.
*
*
* @param lastModifiedTimestamp
* The last time that the dataview was updated in FinSpace. The value is determined as epoch time in
* milliseconds. For example, the value for Monday, November 1, 2021 12:00:00 PM UTC is specified as
* 1635768000000.
*/
public void setLastModifiedTimestamp(java.util.Date lastModifiedTimestamp) {
this.lastModifiedTimestamp = lastModifiedTimestamp;
}
/**
*
* The last time that the dataview was updated in FinSpace. The value is determined as epoch time in milliseconds.
* For example, the value for Monday, November 1, 2021 12:00:00 PM UTC is specified as 1635768000000.
*
*
* @return The last time that the dataview was updated in FinSpace. The value is determined as epoch time in
* milliseconds. For example, the value for Monday, November 1, 2021 12:00:00 PM UTC is specified as
* 1635768000000.
*/
public java.util.Date getLastModifiedTimestamp() {
return this.lastModifiedTimestamp;
}
/**
*
* The last time that the dataview was updated in FinSpace. The value is determined as epoch time in milliseconds.
* For example, the value for Monday, November 1, 2021 12:00:00 PM UTC is specified as 1635768000000.
*
*
* @param lastModifiedTimestamp
* The last time that the dataview was updated in FinSpace. The value is determined as epoch time in
* milliseconds. For example, the value for Monday, November 1, 2021 12:00:00 PM UTC is specified as
* 1635768000000.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateKxDataviewResult withLastModifiedTimestamp(java.util.Date lastModifiedTimestamp) {
setLastModifiedTimestamp(lastModifiedTimestamp);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getEnvironmentId() != null)
sb.append("EnvironmentId: ").append(getEnvironmentId()).append(",");
if (getDatabaseName() != null)
sb.append("DatabaseName: ").append(getDatabaseName()).append(",");
if (getDataviewName() != null)
sb.append("DataviewName: ").append(getDataviewName()).append(",");
if (getAzMode() != null)
sb.append("AzMode: ").append(getAzMode()).append(",");
if (getAvailabilityZoneId() != null)
sb.append("AvailabilityZoneId: ").append(getAvailabilityZoneId()).append(",");
if (getChangesetId() != null)
sb.append("ChangesetId: ").append(getChangesetId()).append(",");
if (getSegmentConfigurations() != null)
sb.append("SegmentConfigurations: ").append(getSegmentConfigurations()).append(",");
if (getActiveVersions() != null)
sb.append("ActiveVersions: ").append(getActiveVersions()).append(",");
if (getStatus() != null)
sb.append("Status: ").append(getStatus()).append(",");
if (getAutoUpdate() != null)
sb.append("AutoUpdate: ").append(getAutoUpdate()).append(",");
if (getReadWrite() != null)
sb.append("ReadWrite: ").append(getReadWrite()).append(",");
if (getDescription() != null)
sb.append("Description: ").append(getDescription()).append(",");
if (getCreatedTimestamp() != null)
sb.append("CreatedTimestamp: ").append(getCreatedTimestamp()).append(",");
if (getLastModifiedTimestamp() != null)
sb.append("LastModifiedTimestamp: ").append(getLastModifiedTimestamp());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof UpdateKxDataviewResult == false)
return false;
UpdateKxDataviewResult other = (UpdateKxDataviewResult) obj;
if (other.getEnvironmentId() == null ^ this.getEnvironmentId() == null)
return false;
if (other.getEnvironmentId() != null && other.getEnvironmentId().equals(this.getEnvironmentId()) == false)
return false;
if (other.getDatabaseName() == null ^ this.getDatabaseName() == null)
return false;
if (other.getDatabaseName() != null && other.getDatabaseName().equals(this.getDatabaseName()) == false)
return false;
if (other.getDataviewName() == null ^ this.getDataviewName() == null)
return false;
if (other.getDataviewName() != null && other.getDataviewName().equals(this.getDataviewName()) == false)
return false;
if (other.getAzMode() == null ^ this.getAzMode() == null)
return false;
if (other.getAzMode() != null && other.getAzMode().equals(this.getAzMode()) == false)
return false;
if (other.getAvailabilityZoneId() == null ^ this.getAvailabilityZoneId() == null)
return false;
if (other.getAvailabilityZoneId() != null && other.getAvailabilityZoneId().equals(this.getAvailabilityZoneId()) == false)
return false;
if (other.getChangesetId() == null ^ this.getChangesetId() == null)
return false;
if (other.getChangesetId() != null && other.getChangesetId().equals(this.getChangesetId()) == false)
return false;
if (other.getSegmentConfigurations() == null ^ this.getSegmentConfigurations() == null)
return false;
if (other.getSegmentConfigurations() != null && other.getSegmentConfigurations().equals(this.getSegmentConfigurations()) == false)
return false;
if (other.getActiveVersions() == null ^ this.getActiveVersions() == null)
return false;
if (other.getActiveVersions() != null && other.getActiveVersions().equals(this.getActiveVersions()) == false)
return false;
if (other.getStatus() == null ^ this.getStatus() == null)
return false;
if (other.getStatus() != null && other.getStatus().equals(this.getStatus()) == false)
return false;
if (other.getAutoUpdate() == null ^ this.getAutoUpdate() == null)
return false;
if (other.getAutoUpdate() != null && other.getAutoUpdate().equals(this.getAutoUpdate()) == false)
return false;
if (other.getReadWrite() == null ^ this.getReadWrite() == null)
return false;
if (other.getReadWrite() != null && other.getReadWrite().equals(this.getReadWrite()) == false)
return false;
if (other.getDescription() == null ^ this.getDescription() == null)
return false;
if (other.getDescription() != null && other.getDescription().equals(this.getDescription()) == false)
return false;
if (other.getCreatedTimestamp() == null ^ this.getCreatedTimestamp() == null)
return false;
if (other.getCreatedTimestamp() != null && other.getCreatedTimestamp().equals(this.getCreatedTimestamp()) == false)
return false;
if (other.getLastModifiedTimestamp() == null ^ this.getLastModifiedTimestamp() == null)
return false;
if (other.getLastModifiedTimestamp() != null && other.getLastModifiedTimestamp().equals(this.getLastModifiedTimestamp()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getEnvironmentId() == null) ? 0 : getEnvironmentId().hashCode());
hashCode = prime * hashCode + ((getDatabaseName() == null) ? 0 : getDatabaseName().hashCode());
hashCode = prime * hashCode + ((getDataviewName() == null) ? 0 : getDataviewName().hashCode());
hashCode = prime * hashCode + ((getAzMode() == null) ? 0 : getAzMode().hashCode());
hashCode = prime * hashCode + ((getAvailabilityZoneId() == null) ? 0 : getAvailabilityZoneId().hashCode());
hashCode = prime * hashCode + ((getChangesetId() == null) ? 0 : getChangesetId().hashCode());
hashCode = prime * hashCode + ((getSegmentConfigurations() == null) ? 0 : getSegmentConfigurations().hashCode());
hashCode = prime * hashCode + ((getActiveVersions() == null) ? 0 : getActiveVersions().hashCode());
hashCode = prime * hashCode + ((getStatus() == null) ? 0 : getStatus().hashCode());
hashCode = prime * hashCode + ((getAutoUpdate() == null) ? 0 : getAutoUpdate().hashCode());
hashCode = prime * hashCode + ((getReadWrite() == null) ? 0 : getReadWrite().hashCode());
hashCode = prime * hashCode + ((getDescription() == null) ? 0 : getDescription().hashCode());
hashCode = prime * hashCode + ((getCreatedTimestamp() == null) ? 0 : getCreatedTimestamp().hashCode());
hashCode = prime * hashCode + ((getLastModifiedTimestamp() == null) ? 0 : getLastModifiedTimestamp().hashCode());
return hashCode;
}
@Override
public UpdateKxDataviewResult clone() {
try {
return (UpdateKxDataviewResult) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
}