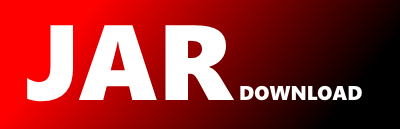
com.amazonaws.services.finspace.model.UpdateKxEnvironmentResult Maven / Gradle / Ivy
Show all versions of aws-java-sdk-finspace Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.finspace.model;
import java.io.Serializable;
import javax.annotation.Generated;
/**
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class UpdateKxEnvironmentResult extends com.amazonaws.AmazonWebServiceResult implements Serializable, Cloneable {
/**
*
* The name of the kdb environment.
*
*/
private String name;
/**
*
* A unique identifier for the kdb environment.
*
*/
private String environmentId;
/**
*
* The unique identifier of the AWS account that is used to create the kdb environment.
*
*/
private String awsAccountId;
/**
*
* The status of the kdb environment.
*
*/
private String status;
/**
*
* The status of the network configuration.
*
*/
private String tgwStatus;
/**
*
* The status of DNS configuration.
*
*/
private String dnsStatus;
/**
*
* Specifies the error message that appears if a flow fails.
*
*/
private String errorMessage;
/**
*
* The description of the environment.
*
*/
private String description;
/**
*
* The ARN identifier of the environment.
*
*/
private String environmentArn;
/**
*
* The KMS key ID to encrypt your data in the FinSpace environment.
*
*/
private String kmsKeyId;
/**
*
* A unique identifier for the AWS environment infrastructure account.
*
*/
private String dedicatedServiceAccountId;
private TransitGatewayConfiguration transitGatewayConfiguration;
/**
*
* A list of DNS server name and server IP. This is used to set up Route-53 outbound resolvers.
*
*/
private java.util.List customDNSConfiguration;
/**
*
* The timestamp at which the kdb environment was created in FinSpace.
*
*/
private java.util.Date creationTimestamp;
/**
*
* The timestamp at which the kdb environment was updated.
*
*/
private java.util.Date updateTimestamp;
/**
*
* The identifier of the availability zones where subnets for the environment are created.
*
*/
private java.util.List availabilityZoneIds;
/**
*
* The name of the kdb environment.
*
*
* @param name
* The name of the kdb environment.
*/
public void setName(String name) {
this.name = name;
}
/**
*
* The name of the kdb environment.
*
*
* @return The name of the kdb environment.
*/
public String getName() {
return this.name;
}
/**
*
* The name of the kdb environment.
*
*
* @param name
* The name of the kdb environment.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateKxEnvironmentResult withName(String name) {
setName(name);
return this;
}
/**
*
* A unique identifier for the kdb environment.
*
*
* @param environmentId
* A unique identifier for the kdb environment.
*/
public void setEnvironmentId(String environmentId) {
this.environmentId = environmentId;
}
/**
*
* A unique identifier for the kdb environment.
*
*
* @return A unique identifier for the kdb environment.
*/
public String getEnvironmentId() {
return this.environmentId;
}
/**
*
* A unique identifier for the kdb environment.
*
*
* @param environmentId
* A unique identifier for the kdb environment.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateKxEnvironmentResult withEnvironmentId(String environmentId) {
setEnvironmentId(environmentId);
return this;
}
/**
*
* The unique identifier of the AWS account that is used to create the kdb environment.
*
*
* @param awsAccountId
* The unique identifier of the AWS account that is used to create the kdb environment.
*/
public void setAwsAccountId(String awsAccountId) {
this.awsAccountId = awsAccountId;
}
/**
*
* The unique identifier of the AWS account that is used to create the kdb environment.
*
*
* @return The unique identifier of the AWS account that is used to create the kdb environment.
*/
public String getAwsAccountId() {
return this.awsAccountId;
}
/**
*
* The unique identifier of the AWS account that is used to create the kdb environment.
*
*
* @param awsAccountId
* The unique identifier of the AWS account that is used to create the kdb environment.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateKxEnvironmentResult withAwsAccountId(String awsAccountId) {
setAwsAccountId(awsAccountId);
return this;
}
/**
*
* The status of the kdb environment.
*
*
* @param status
* The status of the kdb environment.
* @see EnvironmentStatus
*/
public void setStatus(String status) {
this.status = status;
}
/**
*
* The status of the kdb environment.
*
*
* @return The status of the kdb environment.
* @see EnvironmentStatus
*/
public String getStatus() {
return this.status;
}
/**
*
* The status of the kdb environment.
*
*
* @param status
* The status of the kdb environment.
* @return Returns a reference to this object so that method calls can be chained together.
* @see EnvironmentStatus
*/
public UpdateKxEnvironmentResult withStatus(String status) {
setStatus(status);
return this;
}
/**
*
* The status of the kdb environment.
*
*
* @param status
* The status of the kdb environment.
* @return Returns a reference to this object so that method calls can be chained together.
* @see EnvironmentStatus
*/
public UpdateKxEnvironmentResult withStatus(EnvironmentStatus status) {
this.status = status.toString();
return this;
}
/**
*
* The status of the network configuration.
*
*
* @param tgwStatus
* The status of the network configuration.
* @see TgwStatus
*/
public void setTgwStatus(String tgwStatus) {
this.tgwStatus = tgwStatus;
}
/**
*
* The status of the network configuration.
*
*
* @return The status of the network configuration.
* @see TgwStatus
*/
public String getTgwStatus() {
return this.tgwStatus;
}
/**
*
* The status of the network configuration.
*
*
* @param tgwStatus
* The status of the network configuration.
* @return Returns a reference to this object so that method calls can be chained together.
* @see TgwStatus
*/
public UpdateKxEnvironmentResult withTgwStatus(String tgwStatus) {
setTgwStatus(tgwStatus);
return this;
}
/**
*
* The status of the network configuration.
*
*
* @param tgwStatus
* The status of the network configuration.
* @return Returns a reference to this object so that method calls can be chained together.
* @see TgwStatus
*/
public UpdateKxEnvironmentResult withTgwStatus(TgwStatus tgwStatus) {
this.tgwStatus = tgwStatus.toString();
return this;
}
/**
*
* The status of DNS configuration.
*
*
* @param dnsStatus
* The status of DNS configuration.
* @see DnsStatus
*/
public void setDnsStatus(String dnsStatus) {
this.dnsStatus = dnsStatus;
}
/**
*
* The status of DNS configuration.
*
*
* @return The status of DNS configuration.
* @see DnsStatus
*/
public String getDnsStatus() {
return this.dnsStatus;
}
/**
*
* The status of DNS configuration.
*
*
* @param dnsStatus
* The status of DNS configuration.
* @return Returns a reference to this object so that method calls can be chained together.
* @see DnsStatus
*/
public UpdateKxEnvironmentResult withDnsStatus(String dnsStatus) {
setDnsStatus(dnsStatus);
return this;
}
/**
*
* The status of DNS configuration.
*
*
* @param dnsStatus
* The status of DNS configuration.
* @return Returns a reference to this object so that method calls can be chained together.
* @see DnsStatus
*/
public UpdateKxEnvironmentResult withDnsStatus(DnsStatus dnsStatus) {
this.dnsStatus = dnsStatus.toString();
return this;
}
/**
*
* Specifies the error message that appears if a flow fails.
*
*
* @param errorMessage
* Specifies the error message that appears if a flow fails.
*/
public void setErrorMessage(String errorMessage) {
this.errorMessage = errorMessage;
}
/**
*
* Specifies the error message that appears if a flow fails.
*
*
* @return Specifies the error message that appears if a flow fails.
*/
public String getErrorMessage() {
return this.errorMessage;
}
/**
*
* Specifies the error message that appears if a flow fails.
*
*
* @param errorMessage
* Specifies the error message that appears if a flow fails.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateKxEnvironmentResult withErrorMessage(String errorMessage) {
setErrorMessage(errorMessage);
return this;
}
/**
*
* The description of the environment.
*
*
* @param description
* The description of the environment.
*/
public void setDescription(String description) {
this.description = description;
}
/**
*
* The description of the environment.
*
*
* @return The description of the environment.
*/
public String getDescription() {
return this.description;
}
/**
*
* The description of the environment.
*
*
* @param description
* The description of the environment.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateKxEnvironmentResult withDescription(String description) {
setDescription(description);
return this;
}
/**
*
* The ARN identifier of the environment.
*
*
* @param environmentArn
* The ARN identifier of the environment.
*/
public void setEnvironmentArn(String environmentArn) {
this.environmentArn = environmentArn;
}
/**
*
* The ARN identifier of the environment.
*
*
* @return The ARN identifier of the environment.
*/
public String getEnvironmentArn() {
return this.environmentArn;
}
/**
*
* The ARN identifier of the environment.
*
*
* @param environmentArn
* The ARN identifier of the environment.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateKxEnvironmentResult withEnvironmentArn(String environmentArn) {
setEnvironmentArn(environmentArn);
return this;
}
/**
*
* The KMS key ID to encrypt your data in the FinSpace environment.
*
*
* @param kmsKeyId
* The KMS key ID to encrypt your data in the FinSpace environment.
*/
public void setKmsKeyId(String kmsKeyId) {
this.kmsKeyId = kmsKeyId;
}
/**
*
* The KMS key ID to encrypt your data in the FinSpace environment.
*
*
* @return The KMS key ID to encrypt your data in the FinSpace environment.
*/
public String getKmsKeyId() {
return this.kmsKeyId;
}
/**
*
* The KMS key ID to encrypt your data in the FinSpace environment.
*
*
* @param kmsKeyId
* The KMS key ID to encrypt your data in the FinSpace environment.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateKxEnvironmentResult withKmsKeyId(String kmsKeyId) {
setKmsKeyId(kmsKeyId);
return this;
}
/**
*
* A unique identifier for the AWS environment infrastructure account.
*
*
* @param dedicatedServiceAccountId
* A unique identifier for the AWS environment infrastructure account.
*/
public void setDedicatedServiceAccountId(String dedicatedServiceAccountId) {
this.dedicatedServiceAccountId = dedicatedServiceAccountId;
}
/**
*
* A unique identifier for the AWS environment infrastructure account.
*
*
* @return A unique identifier for the AWS environment infrastructure account.
*/
public String getDedicatedServiceAccountId() {
return this.dedicatedServiceAccountId;
}
/**
*
* A unique identifier for the AWS environment infrastructure account.
*
*
* @param dedicatedServiceAccountId
* A unique identifier for the AWS environment infrastructure account.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateKxEnvironmentResult withDedicatedServiceAccountId(String dedicatedServiceAccountId) {
setDedicatedServiceAccountId(dedicatedServiceAccountId);
return this;
}
/**
* @param transitGatewayConfiguration
*/
public void setTransitGatewayConfiguration(TransitGatewayConfiguration transitGatewayConfiguration) {
this.transitGatewayConfiguration = transitGatewayConfiguration;
}
/**
* @return
*/
public TransitGatewayConfiguration getTransitGatewayConfiguration() {
return this.transitGatewayConfiguration;
}
/**
* @param transitGatewayConfiguration
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateKxEnvironmentResult withTransitGatewayConfiguration(TransitGatewayConfiguration transitGatewayConfiguration) {
setTransitGatewayConfiguration(transitGatewayConfiguration);
return this;
}
/**
*
* A list of DNS server name and server IP. This is used to set up Route-53 outbound resolvers.
*
*
* @return A list of DNS server name and server IP. This is used to set up Route-53 outbound resolvers.
*/
public java.util.List getCustomDNSConfiguration() {
return customDNSConfiguration;
}
/**
*
* A list of DNS server name and server IP. This is used to set up Route-53 outbound resolvers.
*
*
* @param customDNSConfiguration
* A list of DNS server name and server IP. This is used to set up Route-53 outbound resolvers.
*/
public void setCustomDNSConfiguration(java.util.Collection customDNSConfiguration) {
if (customDNSConfiguration == null) {
this.customDNSConfiguration = null;
return;
}
this.customDNSConfiguration = new java.util.ArrayList(customDNSConfiguration);
}
/**
*
* A list of DNS server name and server IP. This is used to set up Route-53 outbound resolvers.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setCustomDNSConfiguration(java.util.Collection)} or
* {@link #withCustomDNSConfiguration(java.util.Collection)} if you want to override the existing values.
*
*
* @param customDNSConfiguration
* A list of DNS server name and server IP. This is used to set up Route-53 outbound resolvers.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateKxEnvironmentResult withCustomDNSConfiguration(CustomDNSServer... customDNSConfiguration) {
if (this.customDNSConfiguration == null) {
setCustomDNSConfiguration(new java.util.ArrayList(customDNSConfiguration.length));
}
for (CustomDNSServer ele : customDNSConfiguration) {
this.customDNSConfiguration.add(ele);
}
return this;
}
/**
*
* A list of DNS server name and server IP. This is used to set up Route-53 outbound resolvers.
*
*
* @param customDNSConfiguration
* A list of DNS server name and server IP. This is used to set up Route-53 outbound resolvers.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateKxEnvironmentResult withCustomDNSConfiguration(java.util.Collection customDNSConfiguration) {
setCustomDNSConfiguration(customDNSConfiguration);
return this;
}
/**
*
* The timestamp at which the kdb environment was created in FinSpace.
*
*
* @param creationTimestamp
* The timestamp at which the kdb environment was created in FinSpace.
*/
public void setCreationTimestamp(java.util.Date creationTimestamp) {
this.creationTimestamp = creationTimestamp;
}
/**
*
* The timestamp at which the kdb environment was created in FinSpace.
*
*
* @return The timestamp at which the kdb environment was created in FinSpace.
*/
public java.util.Date getCreationTimestamp() {
return this.creationTimestamp;
}
/**
*
* The timestamp at which the kdb environment was created in FinSpace.
*
*
* @param creationTimestamp
* The timestamp at which the kdb environment was created in FinSpace.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateKxEnvironmentResult withCreationTimestamp(java.util.Date creationTimestamp) {
setCreationTimestamp(creationTimestamp);
return this;
}
/**
*
* The timestamp at which the kdb environment was updated.
*
*
* @param updateTimestamp
* The timestamp at which the kdb environment was updated.
*/
public void setUpdateTimestamp(java.util.Date updateTimestamp) {
this.updateTimestamp = updateTimestamp;
}
/**
*
* The timestamp at which the kdb environment was updated.
*
*
* @return The timestamp at which the kdb environment was updated.
*/
public java.util.Date getUpdateTimestamp() {
return this.updateTimestamp;
}
/**
*
* The timestamp at which the kdb environment was updated.
*
*
* @param updateTimestamp
* The timestamp at which the kdb environment was updated.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateKxEnvironmentResult withUpdateTimestamp(java.util.Date updateTimestamp) {
setUpdateTimestamp(updateTimestamp);
return this;
}
/**
*
* The identifier of the availability zones where subnets for the environment are created.
*
*
* @return The identifier of the availability zones where subnets for the environment are created.
*/
public java.util.List getAvailabilityZoneIds() {
return availabilityZoneIds;
}
/**
*
* The identifier of the availability zones where subnets for the environment are created.
*
*
* @param availabilityZoneIds
* The identifier of the availability zones where subnets for the environment are created.
*/
public void setAvailabilityZoneIds(java.util.Collection availabilityZoneIds) {
if (availabilityZoneIds == null) {
this.availabilityZoneIds = null;
return;
}
this.availabilityZoneIds = new java.util.ArrayList(availabilityZoneIds);
}
/**
*
* The identifier of the availability zones where subnets for the environment are created.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setAvailabilityZoneIds(java.util.Collection)} or {@link #withAvailabilityZoneIds(java.util.Collection)}
* if you want to override the existing values.
*
*
* @param availabilityZoneIds
* The identifier of the availability zones where subnets for the environment are created.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateKxEnvironmentResult withAvailabilityZoneIds(String... availabilityZoneIds) {
if (this.availabilityZoneIds == null) {
setAvailabilityZoneIds(new java.util.ArrayList(availabilityZoneIds.length));
}
for (String ele : availabilityZoneIds) {
this.availabilityZoneIds.add(ele);
}
return this;
}
/**
*
* The identifier of the availability zones where subnets for the environment are created.
*
*
* @param availabilityZoneIds
* The identifier of the availability zones where subnets for the environment are created.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateKxEnvironmentResult withAvailabilityZoneIds(java.util.Collection availabilityZoneIds) {
setAvailabilityZoneIds(availabilityZoneIds);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getName() != null)
sb.append("Name: ").append(getName()).append(",");
if (getEnvironmentId() != null)
sb.append("EnvironmentId: ").append(getEnvironmentId()).append(",");
if (getAwsAccountId() != null)
sb.append("AwsAccountId: ").append(getAwsAccountId()).append(",");
if (getStatus() != null)
sb.append("Status: ").append(getStatus()).append(",");
if (getTgwStatus() != null)
sb.append("TgwStatus: ").append(getTgwStatus()).append(",");
if (getDnsStatus() != null)
sb.append("DnsStatus: ").append(getDnsStatus()).append(",");
if (getErrorMessage() != null)
sb.append("ErrorMessage: ").append(getErrorMessage()).append(",");
if (getDescription() != null)
sb.append("Description: ").append(getDescription()).append(",");
if (getEnvironmentArn() != null)
sb.append("EnvironmentArn: ").append(getEnvironmentArn()).append(",");
if (getKmsKeyId() != null)
sb.append("KmsKeyId: ").append(getKmsKeyId()).append(",");
if (getDedicatedServiceAccountId() != null)
sb.append("DedicatedServiceAccountId: ").append(getDedicatedServiceAccountId()).append(",");
if (getTransitGatewayConfiguration() != null)
sb.append("TransitGatewayConfiguration: ").append(getTransitGatewayConfiguration()).append(",");
if (getCustomDNSConfiguration() != null)
sb.append("CustomDNSConfiguration: ").append(getCustomDNSConfiguration()).append(",");
if (getCreationTimestamp() != null)
sb.append("CreationTimestamp: ").append(getCreationTimestamp()).append(",");
if (getUpdateTimestamp() != null)
sb.append("UpdateTimestamp: ").append(getUpdateTimestamp()).append(",");
if (getAvailabilityZoneIds() != null)
sb.append("AvailabilityZoneIds: ").append(getAvailabilityZoneIds());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof UpdateKxEnvironmentResult == false)
return false;
UpdateKxEnvironmentResult other = (UpdateKxEnvironmentResult) obj;
if (other.getName() == null ^ this.getName() == null)
return false;
if (other.getName() != null && other.getName().equals(this.getName()) == false)
return false;
if (other.getEnvironmentId() == null ^ this.getEnvironmentId() == null)
return false;
if (other.getEnvironmentId() != null && other.getEnvironmentId().equals(this.getEnvironmentId()) == false)
return false;
if (other.getAwsAccountId() == null ^ this.getAwsAccountId() == null)
return false;
if (other.getAwsAccountId() != null && other.getAwsAccountId().equals(this.getAwsAccountId()) == false)
return false;
if (other.getStatus() == null ^ this.getStatus() == null)
return false;
if (other.getStatus() != null && other.getStatus().equals(this.getStatus()) == false)
return false;
if (other.getTgwStatus() == null ^ this.getTgwStatus() == null)
return false;
if (other.getTgwStatus() != null && other.getTgwStatus().equals(this.getTgwStatus()) == false)
return false;
if (other.getDnsStatus() == null ^ this.getDnsStatus() == null)
return false;
if (other.getDnsStatus() != null && other.getDnsStatus().equals(this.getDnsStatus()) == false)
return false;
if (other.getErrorMessage() == null ^ this.getErrorMessage() == null)
return false;
if (other.getErrorMessage() != null && other.getErrorMessage().equals(this.getErrorMessage()) == false)
return false;
if (other.getDescription() == null ^ this.getDescription() == null)
return false;
if (other.getDescription() != null && other.getDescription().equals(this.getDescription()) == false)
return false;
if (other.getEnvironmentArn() == null ^ this.getEnvironmentArn() == null)
return false;
if (other.getEnvironmentArn() != null && other.getEnvironmentArn().equals(this.getEnvironmentArn()) == false)
return false;
if (other.getKmsKeyId() == null ^ this.getKmsKeyId() == null)
return false;
if (other.getKmsKeyId() != null && other.getKmsKeyId().equals(this.getKmsKeyId()) == false)
return false;
if (other.getDedicatedServiceAccountId() == null ^ this.getDedicatedServiceAccountId() == null)
return false;
if (other.getDedicatedServiceAccountId() != null && other.getDedicatedServiceAccountId().equals(this.getDedicatedServiceAccountId()) == false)
return false;
if (other.getTransitGatewayConfiguration() == null ^ this.getTransitGatewayConfiguration() == null)
return false;
if (other.getTransitGatewayConfiguration() != null && other.getTransitGatewayConfiguration().equals(this.getTransitGatewayConfiguration()) == false)
return false;
if (other.getCustomDNSConfiguration() == null ^ this.getCustomDNSConfiguration() == null)
return false;
if (other.getCustomDNSConfiguration() != null && other.getCustomDNSConfiguration().equals(this.getCustomDNSConfiguration()) == false)
return false;
if (other.getCreationTimestamp() == null ^ this.getCreationTimestamp() == null)
return false;
if (other.getCreationTimestamp() != null && other.getCreationTimestamp().equals(this.getCreationTimestamp()) == false)
return false;
if (other.getUpdateTimestamp() == null ^ this.getUpdateTimestamp() == null)
return false;
if (other.getUpdateTimestamp() != null && other.getUpdateTimestamp().equals(this.getUpdateTimestamp()) == false)
return false;
if (other.getAvailabilityZoneIds() == null ^ this.getAvailabilityZoneIds() == null)
return false;
if (other.getAvailabilityZoneIds() != null && other.getAvailabilityZoneIds().equals(this.getAvailabilityZoneIds()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getName() == null) ? 0 : getName().hashCode());
hashCode = prime * hashCode + ((getEnvironmentId() == null) ? 0 : getEnvironmentId().hashCode());
hashCode = prime * hashCode + ((getAwsAccountId() == null) ? 0 : getAwsAccountId().hashCode());
hashCode = prime * hashCode + ((getStatus() == null) ? 0 : getStatus().hashCode());
hashCode = prime * hashCode + ((getTgwStatus() == null) ? 0 : getTgwStatus().hashCode());
hashCode = prime * hashCode + ((getDnsStatus() == null) ? 0 : getDnsStatus().hashCode());
hashCode = prime * hashCode + ((getErrorMessage() == null) ? 0 : getErrorMessage().hashCode());
hashCode = prime * hashCode + ((getDescription() == null) ? 0 : getDescription().hashCode());
hashCode = prime * hashCode + ((getEnvironmentArn() == null) ? 0 : getEnvironmentArn().hashCode());
hashCode = prime * hashCode + ((getKmsKeyId() == null) ? 0 : getKmsKeyId().hashCode());
hashCode = prime * hashCode + ((getDedicatedServiceAccountId() == null) ? 0 : getDedicatedServiceAccountId().hashCode());
hashCode = prime * hashCode + ((getTransitGatewayConfiguration() == null) ? 0 : getTransitGatewayConfiguration().hashCode());
hashCode = prime * hashCode + ((getCustomDNSConfiguration() == null) ? 0 : getCustomDNSConfiguration().hashCode());
hashCode = prime * hashCode + ((getCreationTimestamp() == null) ? 0 : getCreationTimestamp().hashCode());
hashCode = prime * hashCode + ((getUpdateTimestamp() == null) ? 0 : getUpdateTimestamp().hashCode());
hashCode = prime * hashCode + ((getAvailabilityZoneIds() == null) ? 0 : getAvailabilityZoneIds().hashCode());
return hashCode;
}
@Override
public UpdateKxEnvironmentResult clone() {
try {
return (UpdateKxEnvironmentResult) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
}