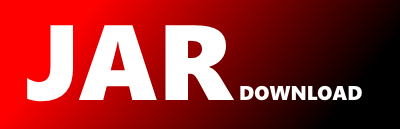
com.amazonaws.services.finspacedata.AWSFinSpaceDataClient Maven / Gradle / Ivy
Show all versions of aws-java-sdk-finspacedata Show documentation
/*
* Copyright 2017-2022 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.finspacedata;
import org.w3c.dom.*;
import java.net.*;
import java.util.*;
import javax.annotation.Generated;
import org.apache.commons.logging.*;
import com.amazonaws.*;
import com.amazonaws.annotation.SdkInternalApi;
import com.amazonaws.auth.*;
import com.amazonaws.handlers.*;
import com.amazonaws.http.*;
import com.amazonaws.internal.*;
import com.amazonaws.internal.auth.*;
import com.amazonaws.metrics.*;
import com.amazonaws.regions.*;
import com.amazonaws.transform.*;
import com.amazonaws.util.*;
import com.amazonaws.protocol.json.*;
import com.amazonaws.util.AWSRequestMetrics.Field;
import com.amazonaws.annotation.ThreadSafe;
import com.amazonaws.client.AwsSyncClientParams;
import com.amazonaws.client.builder.AdvancedConfig;
import com.amazonaws.services.finspacedata.AWSFinSpaceDataClientBuilder;
import com.amazonaws.AmazonServiceException;
import com.amazonaws.services.finspacedata.model.*;
import com.amazonaws.services.finspacedata.model.transform.*;
/**
* Client for accessing FinSpace Data. All service calls made using this client are blocking, and will not return until
* the service call completes.
*
*
* The FinSpace APIs let you take actions inside the FinSpace.
*
*/
@ThreadSafe
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class AWSFinSpaceDataClient extends AmazonWebServiceClient implements AWSFinSpaceData {
/** Provider for AWS credentials. */
private final AWSCredentialsProvider awsCredentialsProvider;
private static final Log log = LogFactory.getLog(AWSFinSpaceData.class);
/** Default signing name for the service. */
private static final String DEFAULT_SIGNING_NAME = "finspace-api";
/** Client configuration factory providing ClientConfigurations tailored to this client */
protected static final ClientConfigurationFactory configFactory = new ClientConfigurationFactory();
private final AdvancedConfig advancedConfig;
private static final com.amazonaws.protocol.json.SdkJsonProtocolFactory protocolFactory = new com.amazonaws.protocol.json.SdkJsonProtocolFactory(
new JsonClientMetadata()
.withProtocolVersion("1.1")
.withSupportsCbor(false)
.withSupportsIon(false)
.withContentTypeOverride("application/json")
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("AccessDeniedException").withExceptionUnmarshaller(
com.amazonaws.services.finspacedata.model.transform.AccessDeniedExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ConflictException").withExceptionUnmarshaller(
com.amazonaws.services.finspacedata.model.transform.ConflictExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ResourceNotFoundException").withExceptionUnmarshaller(
com.amazonaws.services.finspacedata.model.transform.ResourceNotFoundExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ThrottlingException").withExceptionUnmarshaller(
com.amazonaws.services.finspacedata.model.transform.ThrottlingExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ValidationException").withExceptionUnmarshaller(
com.amazonaws.services.finspacedata.model.transform.ValidationExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InternalServerException").withExceptionUnmarshaller(
com.amazonaws.services.finspacedata.model.transform.InternalServerExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("LimitExceededException").withExceptionUnmarshaller(
com.amazonaws.services.finspacedata.model.transform.LimitExceededExceptionUnmarshaller.getInstance()))
.withBaseServiceExceptionClass(com.amazonaws.services.finspacedata.model.AWSFinSpaceDataException.class));
public static AWSFinSpaceDataClientBuilder builder() {
return AWSFinSpaceDataClientBuilder.standard();
}
/**
* Constructs a new client to invoke service methods on FinSpace Data using the specified parameters.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param clientParams
* Object providing client parameters.
*/
AWSFinSpaceDataClient(AwsSyncClientParams clientParams) {
this(clientParams, false);
}
/**
* Constructs a new client to invoke service methods on FinSpace Data using the specified parameters.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param clientParams
* Object providing client parameters.
*/
AWSFinSpaceDataClient(AwsSyncClientParams clientParams, boolean endpointDiscoveryEnabled) {
super(clientParams);
this.awsCredentialsProvider = clientParams.getCredentialsProvider();
this.advancedConfig = clientParams.getAdvancedConfig();
init();
}
private void init() {
setServiceNameIntern(DEFAULT_SIGNING_NAME);
setEndpointPrefix(ENDPOINT_PREFIX);
// calling this.setEndPoint(...) will also modify the signer accordingly
setEndpoint("finspace-api.us-east-1.amazonaws.com");
HandlerChainFactory chainFactory = new HandlerChainFactory();
requestHandler2s.addAll(chainFactory.newRequestHandlerChain("/com/amazonaws/services/finspacedata/request.handlers"));
requestHandler2s.addAll(chainFactory.newRequestHandler2Chain("/com/amazonaws/services/finspacedata/request.handler2s"));
requestHandler2s.addAll(chainFactory.getGlobalHandlers());
}
/**
*
* Creates a new Changeset in a FinSpace Dataset.
*
*
* @param createChangesetRequest
* The request for a CreateChangeset operation.
* @return Result of the CreateChangeset operation returned by the service.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws LimitExceededException
* A limit has exceeded.
* @throws ConflictException
* The request conflicts with an existing resource.
* @sample AWSFinSpaceData.CreateChangeset
* @see AWS API
* Documentation
*/
@Override
public CreateChangesetResult createChangeset(CreateChangesetRequest request) {
request = beforeClientExecution(request);
return executeCreateChangeset(request);
}
@SdkInternalApi
final CreateChangesetResult executeCreateChangeset(CreateChangesetRequest createChangesetRequest) {
ExecutionContext executionContext = createExecutionContext(createChangesetRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateChangesetRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createChangesetRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "finspace data");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateChangeset");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateChangesetResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a Dataview for a Dataset.
*
*
* @param createDataViewRequest
* Request for creating a data view.
* @return Result of the CreateDataView operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws LimitExceededException
* A limit has exceeded.
* @throws ConflictException
* The request conflicts with an existing resource.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @sample AWSFinSpaceData.CreateDataView
* @see AWS API
* Documentation
*/
@Override
public CreateDataViewResult createDataView(CreateDataViewRequest request) {
request = beforeClientExecution(request);
return executeCreateDataView(request);
}
@SdkInternalApi
final CreateDataViewResult executeCreateDataView(CreateDataViewRequest createDataViewRequest) {
ExecutionContext executionContext = createExecutionContext(createDataViewRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateDataViewRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createDataViewRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "finspace data");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateDataView");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateDataViewResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a new FinSpace Dataset.
*
*
* @param createDatasetRequest
* The request for a CreateDataset operation
* @return Result of the CreateDataset operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws LimitExceededException
* A limit has exceeded.
* @throws ConflictException
* The request conflicts with an existing resource.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @sample AWSFinSpaceData.CreateDataset
* @see AWS API
* Documentation
*/
@Override
public CreateDatasetResult createDataset(CreateDatasetRequest request) {
request = beforeClientExecution(request);
return executeCreateDataset(request);
}
@SdkInternalApi
final CreateDatasetResult executeCreateDataset(CreateDatasetRequest createDatasetRequest) {
ExecutionContext executionContext = createExecutionContext(createDatasetRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateDatasetRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createDatasetRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "finspace data");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateDataset");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateDatasetResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a group of permissions for various actions that a user can perform in FinSpace.
*
*
* @param createPermissionGroupRequest
* @return Result of the CreatePermissionGroup operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws LimitExceededException
* A limit has exceeded.
* @throws ConflictException
* The request conflicts with an existing resource.
* @sample AWSFinSpaceData.CreatePermissionGroup
* @see AWS
* API Documentation
*/
@Override
public CreatePermissionGroupResult createPermissionGroup(CreatePermissionGroupRequest request) {
request = beforeClientExecution(request);
return executeCreatePermissionGroup(request);
}
@SdkInternalApi
final CreatePermissionGroupResult executeCreatePermissionGroup(CreatePermissionGroupRequest createPermissionGroupRequest) {
ExecutionContext executionContext = createExecutionContext(createPermissionGroupRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreatePermissionGroupRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createPermissionGroupRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "finspace data");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreatePermissionGroup");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new CreatePermissionGroupResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a new user in FinSpace.
*
*
* @param createUserRequest
* @return Result of the CreateUser operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws LimitExceededException
* A limit has exceeded.
* @throws ConflictException
* The request conflicts with an existing resource.
* @sample AWSFinSpaceData.CreateUser
* @see AWS API
* Documentation
*/
@Override
public CreateUserResult createUser(CreateUserRequest request) {
request = beforeClientExecution(request);
return executeCreateUser(request);
}
@SdkInternalApi
final CreateUserResult executeCreateUser(CreateUserRequest createUserRequest) {
ExecutionContext executionContext = createExecutionContext(createUserRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateUserRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createUserRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "finspace data");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateUser");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateUserResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes a FinSpace Dataset.
*
*
* @param deleteDatasetRequest
* The request for a DeleteDataset operation.
* @return Result of the DeleteDataset operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws LimitExceededException
* A limit has exceeded.
* @throws ConflictException
* The request conflicts with an existing resource.
* @sample AWSFinSpaceData.DeleteDataset
* @see AWS API
* Documentation
*/
@Override
public DeleteDatasetResult deleteDataset(DeleteDatasetRequest request) {
request = beforeClientExecution(request);
return executeDeleteDataset(request);
}
@SdkInternalApi
final DeleteDatasetResult executeDeleteDataset(DeleteDatasetRequest deleteDatasetRequest) {
ExecutionContext executionContext = createExecutionContext(deleteDatasetRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteDatasetRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteDatasetRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "finspace data");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteDataset");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteDatasetResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes a permission group. This action is irreversible.
*
*
* @param deletePermissionGroupRequest
* @return Result of the DeletePermissionGroup operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws LimitExceededException
* A limit has exceeded.
* @throws ConflictException
* The request conflicts with an existing resource.
* @sample AWSFinSpaceData.DeletePermissionGroup
* @see AWS
* API Documentation
*/
@Override
public DeletePermissionGroupResult deletePermissionGroup(DeletePermissionGroupRequest request) {
request = beforeClientExecution(request);
return executeDeletePermissionGroup(request);
}
@SdkInternalApi
final DeletePermissionGroupResult executeDeletePermissionGroup(DeletePermissionGroupRequest deletePermissionGroupRequest) {
ExecutionContext executionContext = createExecutionContext(deletePermissionGroupRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeletePermissionGroupRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deletePermissionGroupRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "finspace data");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeletePermissionGroup");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DeletePermissionGroupResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Denies access to the FinSpace web application and API for the specified user.
*
*
* @param disableUserRequest
* @return Result of the DisableUser operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws ConflictException
* The request conflicts with an existing resource.
* @sample AWSFinSpaceData.DisableUser
* @see AWS API
* Documentation
*/
@Override
public DisableUserResult disableUser(DisableUserRequest request) {
request = beforeClientExecution(request);
return executeDisableUser(request);
}
@SdkInternalApi
final DisableUserResult executeDisableUser(DisableUserRequest disableUserRequest) {
ExecutionContext executionContext = createExecutionContext(disableUserRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DisableUserRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(disableUserRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "finspace data");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DisableUser");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DisableUserResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Allows the specified user to access the FinSpace web application and API.
*
*
* @param enableUserRequest
* @return Result of the EnableUser operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws LimitExceededException
* A limit has exceeded.
* @throws ConflictException
* The request conflicts with an existing resource.
* @sample AWSFinSpaceData.EnableUser
* @see AWS API
* Documentation
*/
@Override
public EnableUserResult enableUser(EnableUserRequest request) {
request = beforeClientExecution(request);
return executeEnableUser(request);
}
@SdkInternalApi
final EnableUserResult executeEnableUser(EnableUserRequest enableUserRequest) {
ExecutionContext executionContext = createExecutionContext(enableUserRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new EnableUserRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(enableUserRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "finspace data");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "EnableUser");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true).withHasStreamingSuccessResponse(false), new EnableUserResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Get information about a Changeset.
*
*
* @param getChangesetRequest
* Request to describe a changeset.
* @return Result of the GetChangeset operation returned by the service.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ConflictException
* The request conflicts with an existing resource.
* @sample AWSFinSpaceData.GetChangeset
* @see AWS API
* Documentation
*/
@Override
public GetChangesetResult getChangeset(GetChangesetRequest request) {
request = beforeClientExecution(request);
return executeGetChangeset(request);
}
@SdkInternalApi
final GetChangesetResult executeGetChangeset(GetChangesetRequest getChangesetRequest) {
ExecutionContext executionContext = createExecutionContext(getChangesetRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetChangesetRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getChangesetRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "finspace data");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetChangeset");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetChangesetResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets information about a Dataview.
*
*
* @param getDataViewRequest
* Request for retrieving a data view detail. Grouped / accessible within a dataset by its dataset id.
* @return Result of the GetDataView operation returned by the service.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws ConflictException
* The request conflicts with an existing resource.
* @sample AWSFinSpaceData.GetDataView
* @see AWS API
* Documentation
*/
@Override
public GetDataViewResult getDataView(GetDataViewRequest request) {
request = beforeClientExecution(request);
return executeGetDataView(request);
}
@SdkInternalApi
final GetDataViewResult executeGetDataView(GetDataViewRequest getDataViewRequest) {
ExecutionContext executionContext = createExecutionContext(getDataViewRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetDataViewRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getDataViewRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "finspace data");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetDataView");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetDataViewResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns information about a Dataset.
*
*
* @param getDatasetRequest
* Request for the GetDataset operation.
* @return Result of the GetDataset operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws ConflictException
* The request conflicts with an existing resource.
* @sample AWSFinSpaceData.GetDataset
* @see AWS API
* Documentation
*/
@Override
public GetDatasetResult getDataset(GetDatasetRequest request) {
request = beforeClientExecution(request);
return executeGetDataset(request);
}
@SdkInternalApi
final GetDatasetResult executeGetDataset(GetDatasetRequest getDatasetRequest) {
ExecutionContext executionContext = createExecutionContext(getDatasetRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetDatasetRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getDatasetRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "finspace data");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetDataset");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetDatasetResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Request programmatic credentials to use with FinSpace SDK.
*
*
* @param getProgrammaticAccessCredentialsRequest
* Request for GetProgrammaticAccessCredentials operation
* @return Result of the GetProgrammaticAccessCredentials operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @sample AWSFinSpaceData.GetProgrammaticAccessCredentials
* @see AWS API Documentation
*/
@Override
public GetProgrammaticAccessCredentialsResult getProgrammaticAccessCredentials(GetProgrammaticAccessCredentialsRequest request) {
request = beforeClientExecution(request);
return executeGetProgrammaticAccessCredentials(request);
}
@SdkInternalApi
final GetProgrammaticAccessCredentialsResult executeGetProgrammaticAccessCredentials(
GetProgrammaticAccessCredentialsRequest getProgrammaticAccessCredentialsRequest) {
ExecutionContext executionContext = createExecutionContext(getProgrammaticAccessCredentialsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetProgrammaticAccessCredentialsRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(getProgrammaticAccessCredentialsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "finspace data");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetProgrammaticAccessCredentials");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new GetProgrammaticAccessCredentialsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves details for a specific user.
*
*
* @param getUserRequest
* @return Result of the GetUser operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @sample AWSFinSpaceData.GetUser
* @see AWS API
* Documentation
*/
@Override
public GetUserResult getUser(GetUserRequest request) {
request = beforeClientExecution(request);
return executeGetUser(request);
}
@SdkInternalApi
final GetUserResult executeGetUser(GetUserRequest getUserRequest) {
ExecutionContext executionContext = createExecutionContext(getUserRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetUserRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getUserRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "finspace data");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetUser");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetUserResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* A temporary Amazon S3 location, where you can copy your files from a source location to stage or use as a scratch
* space in FinSpace notebook.
*
*
* @param getWorkingLocationRequest
* @return Result of the GetWorkingLocation operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @sample AWSFinSpaceData.GetWorkingLocation
* @see AWS
* API Documentation
*/
@Override
public GetWorkingLocationResult getWorkingLocation(GetWorkingLocationRequest request) {
request = beforeClientExecution(request);
return executeGetWorkingLocation(request);
}
@SdkInternalApi
final GetWorkingLocationResult executeGetWorkingLocation(GetWorkingLocationRequest getWorkingLocationRequest) {
ExecutionContext executionContext = createExecutionContext(getWorkingLocationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetWorkingLocationRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getWorkingLocationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "finspace data");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetWorkingLocation");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetWorkingLocationResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists the FinSpace Changesets for a Dataset.
*
*
* @param listChangesetsRequest
* Request to ListChangesetsRequest. It exposes minimal query filters.
* @return Result of the ListChangesets operation returned by the service.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ConflictException
* The request conflicts with an existing resource.
* @sample AWSFinSpaceData.ListChangesets
* @see AWS API
* Documentation
*/
@Override
public ListChangesetsResult listChangesets(ListChangesetsRequest request) {
request = beforeClientExecution(request);
return executeListChangesets(request);
}
@SdkInternalApi
final ListChangesetsResult executeListChangesets(ListChangesetsRequest listChangesetsRequest) {
ExecutionContext executionContext = createExecutionContext(listChangesetsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListChangesetsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listChangesetsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "finspace data");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListChangesets");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListChangesetsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists all available Dataviews for a Dataset.
*
*
* @param listDataViewsRequest
* Request for a list data views.
* @return Result of the ListDataViews operation returned by the service.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws ConflictException
* The request conflicts with an existing resource.
* @sample AWSFinSpaceData.ListDataViews
* @see AWS API
* Documentation
*/
@Override
public ListDataViewsResult listDataViews(ListDataViewsRequest request) {
request = beforeClientExecution(request);
return executeListDataViews(request);
}
@SdkInternalApi
final ListDataViewsResult executeListDataViews(ListDataViewsRequest listDataViewsRequest) {
ExecutionContext executionContext = createExecutionContext(listDataViewsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListDataViewsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listDataViewsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "finspace data");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListDataViews");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListDataViewsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists all of the active Datasets that a user has access to.
*
*
* @param listDatasetsRequest
* Request for the ListDatasets operation.
* @return Result of the ListDatasets operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws ConflictException
* The request conflicts with an existing resource.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @sample AWSFinSpaceData.ListDatasets
* @see AWS API
* Documentation
*/
@Override
public ListDatasetsResult listDatasets(ListDatasetsRequest request) {
request = beforeClientExecution(request);
return executeListDatasets(request);
}
@SdkInternalApi
final ListDatasetsResult executeListDatasets(ListDatasetsRequest listDatasetsRequest) {
ExecutionContext executionContext = createExecutionContext(listDatasetsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListDatasetsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listDatasetsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "finspace data");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListDatasets");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListDatasetsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists all available permission groups in FinSpace.
*
*
* @param listPermissionGroupsRequest
* @return Result of the ListPermissionGroups operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @sample AWSFinSpaceData.ListPermissionGroups
* @see AWS
* API Documentation
*/
@Override
public ListPermissionGroupsResult listPermissionGroups(ListPermissionGroupsRequest request) {
request = beforeClientExecution(request);
return executeListPermissionGroups(request);
}
@SdkInternalApi
final ListPermissionGroupsResult executeListPermissionGroups(ListPermissionGroupsRequest listPermissionGroupsRequest) {
ExecutionContext executionContext = createExecutionContext(listPermissionGroupsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListPermissionGroupsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listPermissionGroupsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "finspace data");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListPermissionGroups");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListPermissionGroupsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists all available user accounts in FinSpace.
*
*
* @param listUsersRequest
* @return Result of the ListUsers operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @sample AWSFinSpaceData.ListUsers
* @see AWS API
* Documentation
*/
@Override
public ListUsersResult listUsers(ListUsersRequest request) {
request = beforeClientExecution(request);
return executeListUsers(request);
}
@SdkInternalApi
final ListUsersResult executeListUsers(ListUsersRequest listUsersRequest) {
ExecutionContext executionContext = createExecutionContext(listUsersRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListUsersRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listUsersRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "finspace data");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListUsers");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListUsersResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Resets the password for a specified user ID and generates a temporary one. Only a superuser can reset password
* for other users. Resetting the password immediately invalidates the previous password associated with the user.
*
*
* @param resetUserPasswordRequest
* @return Result of the ResetUserPassword operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws ConflictException
* The request conflicts with an existing resource.
* @sample AWSFinSpaceData.ResetUserPassword
* @see AWS API
* Documentation
*/
@Override
public ResetUserPasswordResult resetUserPassword(ResetUserPasswordRequest request) {
request = beforeClientExecution(request);
return executeResetUserPassword(request);
}
@SdkInternalApi
final ResetUserPasswordResult executeResetUserPassword(ResetUserPasswordRequest resetUserPasswordRequest) {
ExecutionContext executionContext = createExecutionContext(resetUserPasswordRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ResetUserPasswordRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(resetUserPasswordRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "finspace data");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ResetUserPassword");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ResetUserPasswordResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates a FinSpace Changeset.
*
*
* @param updateChangesetRequest
* Request to update an existing changeset.
* @return Result of the UpdateChangeset operation returned by the service.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ConflictException
* The request conflicts with an existing resource.
* @sample AWSFinSpaceData.UpdateChangeset
* @see AWS API
* Documentation
*/
@Override
public UpdateChangesetResult updateChangeset(UpdateChangesetRequest request) {
request = beforeClientExecution(request);
return executeUpdateChangeset(request);
}
@SdkInternalApi
final UpdateChangesetResult executeUpdateChangeset(UpdateChangesetRequest updateChangesetRequest) {
ExecutionContext executionContext = createExecutionContext(updateChangesetRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateChangesetRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(updateChangesetRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "finspace data");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateChangeset");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new UpdateChangesetResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates a FinSpace Dataset.
*
*
* @param updateDatasetRequest
* The request for an UpdateDataset operation
* @return Result of the UpdateDataset operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ConflictException
* The request conflicts with an existing resource.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @sample AWSFinSpaceData.UpdateDataset
* @see AWS API
* Documentation
*/
@Override
public UpdateDatasetResult updateDataset(UpdateDatasetRequest request) {
request = beforeClientExecution(request);
return executeUpdateDataset(request);
}
@SdkInternalApi
final UpdateDatasetResult executeUpdateDataset(UpdateDatasetRequest updateDatasetRequest) {
ExecutionContext executionContext = createExecutionContext(updateDatasetRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateDatasetRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(updateDatasetRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "finspace data");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateDataset");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new UpdateDatasetResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Modifies the details of a permission group. You cannot modify a permissionGroupID
.
*
*
* @param updatePermissionGroupRequest
* @return Result of the UpdatePermissionGroup operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws ConflictException
* The request conflicts with an existing resource.
* @sample AWSFinSpaceData.UpdatePermissionGroup
* @see AWS
* API Documentation
*/
@Override
public UpdatePermissionGroupResult updatePermissionGroup(UpdatePermissionGroupRequest request) {
request = beforeClientExecution(request);
return executeUpdatePermissionGroup(request);
}
@SdkInternalApi
final UpdatePermissionGroupResult executeUpdatePermissionGroup(UpdatePermissionGroupRequest updatePermissionGroupRequest) {
ExecutionContext executionContext = createExecutionContext(updatePermissionGroupRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdatePermissionGroupRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(updatePermissionGroupRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "finspace data");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdatePermissionGroup");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new UpdatePermissionGroupResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Modifies the details of the specified user account. You cannot update the userId
for a user.
*
*
* @param updateUserRequest
* @return Result of the UpdateUser operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws ConflictException
* The request conflicts with an existing resource.
* @sample AWSFinSpaceData.UpdateUser
* @see AWS API
* Documentation
*/
@Override
public UpdateUserResult updateUser(UpdateUserRequest request) {
request = beforeClientExecution(request);
return executeUpdateUser(request);
}
@SdkInternalApi
final UpdateUserResult executeUpdateUser(UpdateUserRequest updateUserRequest) {
ExecutionContext executionContext = createExecutionContext(updateUserRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateUserRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(updateUserRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "finspace data");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateUser");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true).withHasStreamingSuccessResponse(false), new UpdateUserResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
* Returns additional metadata for a previously executed successful, request, typically used for debugging issues
* where a service isn't acting as expected. This data isn't considered part of the result data returned by an
* operation, so it's available through this separate, diagnostic interface.
*
* Response metadata is only cached for a limited period of time, so if you need to access this extra diagnostic
* information for an executed request, you should use this method to retrieve it as soon as possible after
* executing the request.
*
* @param request
* The originally executed request
*
* @return The response metadata for the specified request, or null if none is available.
*/
public ResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request) {
return client.getResponseMetadataForRequest(request);
}
/**
* Normal invoke with authentication. Credentials are required and may be overriden at the request level.
**/
private Response invoke(Request request, HttpResponseHandler> responseHandler,
ExecutionContext executionContext) {
return invoke(request, responseHandler, executionContext, null, null);
}
/**
* Normal invoke with authentication. Credentials are required and may be overriden at the request level.
**/
private Response invoke(Request request, HttpResponseHandler> responseHandler,
ExecutionContext executionContext, URI cachedEndpoint, URI uriFromEndpointTrait) {
executionContext.setCredentialsProvider(CredentialUtils.getCredentialsProvider(request.getOriginalRequest(), awsCredentialsProvider));
return doInvoke(request, responseHandler, executionContext, cachedEndpoint, uriFromEndpointTrait);
}
/**
* Invoke with no authentication. Credentials are not required and any credentials set on the client or request will
* be ignored for this operation.
**/
private Response anonymousInvoke(Request request,
HttpResponseHandler> responseHandler, ExecutionContext executionContext) {
return doInvoke(request, responseHandler, executionContext, null, null);
}
/**
* Invoke the request using the http client. Assumes credentials (or lack thereof) have been configured in the
* ExecutionContext beforehand.
**/
private Response doInvoke(Request request, HttpResponseHandler> responseHandler,
ExecutionContext executionContext, URI discoveredEndpoint, URI uriFromEndpointTrait) {
if (discoveredEndpoint != null) {
request.setEndpoint(discoveredEndpoint);
request.getOriginalRequest().getRequestClientOptions().appendUserAgent("endpoint-discovery");
} else if (uriFromEndpointTrait != null) {
request.setEndpoint(uriFromEndpointTrait);
} else {
request.setEndpoint(endpoint);
}
request.setTimeOffset(timeOffset);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler(new JsonErrorResponseMetadata());
return client.execute(request, responseHandler, errorResponseHandler, executionContext);
}
@com.amazonaws.annotation.SdkInternalApi
static com.amazonaws.protocol.json.SdkJsonProtocolFactory getProtocolFactory() {
return protocolFactory;
}
@Override
public void shutdown() {
super.shutdown();
}
}