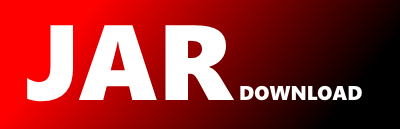
com.amazonaws.services.finspacedata.model.CreateDataViewRequest Maven / Gradle / Ivy
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.finspacedata.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
* Request for creating a data view.
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class CreateDataViewRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* A token that ensures idempotency. This token expires in 10 minutes.
*
*/
private String clientToken;
/**
*
* The unique Dataset identifier that is used to create a Dataview.
*
*/
private String datasetId;
/**
*
* Flag to indicate Dataview should be updated automatically.
*
*/
private Boolean autoUpdate;
/**
*
* Columns to be used for sorting the data.
*
*/
private java.util.List sortColumns;
/**
*
* Ordered set of column names used to partition data.
*
*/
private java.util.List partitionColumns;
/**
*
* Beginning time to use for the Dataview. The value is determined as epoch time in milliseconds. For example, the
* value for Monday, November 1, 2021 12:00:00 PM UTC is specified as 1635768000000.
*
*/
private Long asOfTimestamp;
/**
*
* Options that define the destination type for the Dataview.
*
*/
private DataViewDestinationTypeParams destinationTypeParams;
/**
*
* A token that ensures idempotency. This token expires in 10 minutes.
*
*
* @param clientToken
* A token that ensures idempotency. This token expires in 10 minutes.
*/
public void setClientToken(String clientToken) {
this.clientToken = clientToken;
}
/**
*
* A token that ensures idempotency. This token expires in 10 minutes.
*
*
* @return A token that ensures idempotency. This token expires in 10 minutes.
*/
public String getClientToken() {
return this.clientToken;
}
/**
*
* A token that ensures idempotency. This token expires in 10 minutes.
*
*
* @param clientToken
* A token that ensures idempotency. This token expires in 10 minutes.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateDataViewRequest withClientToken(String clientToken) {
setClientToken(clientToken);
return this;
}
/**
*
* The unique Dataset identifier that is used to create a Dataview.
*
*
* @param datasetId
* The unique Dataset identifier that is used to create a Dataview.
*/
public void setDatasetId(String datasetId) {
this.datasetId = datasetId;
}
/**
*
* The unique Dataset identifier that is used to create a Dataview.
*
*
* @return The unique Dataset identifier that is used to create a Dataview.
*/
public String getDatasetId() {
return this.datasetId;
}
/**
*
* The unique Dataset identifier that is used to create a Dataview.
*
*
* @param datasetId
* The unique Dataset identifier that is used to create a Dataview.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateDataViewRequest withDatasetId(String datasetId) {
setDatasetId(datasetId);
return this;
}
/**
*
* Flag to indicate Dataview should be updated automatically.
*
*
* @param autoUpdate
* Flag to indicate Dataview should be updated automatically.
*/
public void setAutoUpdate(Boolean autoUpdate) {
this.autoUpdate = autoUpdate;
}
/**
*
* Flag to indicate Dataview should be updated automatically.
*
*
* @return Flag to indicate Dataview should be updated automatically.
*/
public Boolean getAutoUpdate() {
return this.autoUpdate;
}
/**
*
* Flag to indicate Dataview should be updated automatically.
*
*
* @param autoUpdate
* Flag to indicate Dataview should be updated automatically.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateDataViewRequest withAutoUpdate(Boolean autoUpdate) {
setAutoUpdate(autoUpdate);
return this;
}
/**
*
* Flag to indicate Dataview should be updated automatically.
*
*
* @return Flag to indicate Dataview should be updated automatically.
*/
public Boolean isAutoUpdate() {
return this.autoUpdate;
}
/**
*
* Columns to be used for sorting the data.
*
*
* @return Columns to be used for sorting the data.
*/
public java.util.List getSortColumns() {
return sortColumns;
}
/**
*
* Columns to be used for sorting the data.
*
*
* @param sortColumns
* Columns to be used for sorting the data.
*/
public void setSortColumns(java.util.Collection sortColumns) {
if (sortColumns == null) {
this.sortColumns = null;
return;
}
this.sortColumns = new java.util.ArrayList(sortColumns);
}
/**
*
* Columns to be used for sorting the data.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setSortColumns(java.util.Collection)} or {@link #withSortColumns(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param sortColumns
* Columns to be used for sorting the data.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateDataViewRequest withSortColumns(String... sortColumns) {
if (this.sortColumns == null) {
setSortColumns(new java.util.ArrayList(sortColumns.length));
}
for (String ele : sortColumns) {
this.sortColumns.add(ele);
}
return this;
}
/**
*
* Columns to be used for sorting the data.
*
*
* @param sortColumns
* Columns to be used for sorting the data.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateDataViewRequest withSortColumns(java.util.Collection sortColumns) {
setSortColumns(sortColumns);
return this;
}
/**
*
* Ordered set of column names used to partition data.
*
*
* @return Ordered set of column names used to partition data.
*/
public java.util.List getPartitionColumns() {
return partitionColumns;
}
/**
*
* Ordered set of column names used to partition data.
*
*
* @param partitionColumns
* Ordered set of column names used to partition data.
*/
public void setPartitionColumns(java.util.Collection partitionColumns) {
if (partitionColumns == null) {
this.partitionColumns = null;
return;
}
this.partitionColumns = new java.util.ArrayList(partitionColumns);
}
/**
*
* Ordered set of column names used to partition data.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setPartitionColumns(java.util.Collection)} or {@link #withPartitionColumns(java.util.Collection)} if you
* want to override the existing values.
*
*
* @param partitionColumns
* Ordered set of column names used to partition data.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateDataViewRequest withPartitionColumns(String... partitionColumns) {
if (this.partitionColumns == null) {
setPartitionColumns(new java.util.ArrayList(partitionColumns.length));
}
for (String ele : partitionColumns) {
this.partitionColumns.add(ele);
}
return this;
}
/**
*
* Ordered set of column names used to partition data.
*
*
* @param partitionColumns
* Ordered set of column names used to partition data.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateDataViewRequest withPartitionColumns(java.util.Collection partitionColumns) {
setPartitionColumns(partitionColumns);
return this;
}
/**
*
* Beginning time to use for the Dataview. The value is determined as epoch time in milliseconds. For example, the
* value for Monday, November 1, 2021 12:00:00 PM UTC is specified as 1635768000000.
*
*
* @param asOfTimestamp
* Beginning time to use for the Dataview. The value is determined as epoch time in milliseconds. For
* example, the value for Monday, November 1, 2021 12:00:00 PM UTC is specified as 1635768000000.
*/
public void setAsOfTimestamp(Long asOfTimestamp) {
this.asOfTimestamp = asOfTimestamp;
}
/**
*
* Beginning time to use for the Dataview. The value is determined as epoch time in milliseconds. For example, the
* value for Monday, November 1, 2021 12:00:00 PM UTC is specified as 1635768000000.
*
*
* @return Beginning time to use for the Dataview. The value is determined as epoch time in milliseconds. For
* example, the value for Monday, November 1, 2021 12:00:00 PM UTC is specified as 1635768000000.
*/
public Long getAsOfTimestamp() {
return this.asOfTimestamp;
}
/**
*
* Beginning time to use for the Dataview. The value is determined as epoch time in milliseconds. For example, the
* value for Monday, November 1, 2021 12:00:00 PM UTC is specified as 1635768000000.
*
*
* @param asOfTimestamp
* Beginning time to use for the Dataview. The value is determined as epoch time in milliseconds. For
* example, the value for Monday, November 1, 2021 12:00:00 PM UTC is specified as 1635768000000.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateDataViewRequest withAsOfTimestamp(Long asOfTimestamp) {
setAsOfTimestamp(asOfTimestamp);
return this;
}
/**
*
* Options that define the destination type for the Dataview.
*
*
* @param destinationTypeParams
* Options that define the destination type for the Dataview.
*/
public void setDestinationTypeParams(DataViewDestinationTypeParams destinationTypeParams) {
this.destinationTypeParams = destinationTypeParams;
}
/**
*
* Options that define the destination type for the Dataview.
*
*
* @return Options that define the destination type for the Dataview.
*/
public DataViewDestinationTypeParams getDestinationTypeParams() {
return this.destinationTypeParams;
}
/**
*
* Options that define the destination type for the Dataview.
*
*
* @param destinationTypeParams
* Options that define the destination type for the Dataview.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateDataViewRequest withDestinationTypeParams(DataViewDestinationTypeParams destinationTypeParams) {
setDestinationTypeParams(destinationTypeParams);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getClientToken() != null)
sb.append("ClientToken: ").append(getClientToken()).append(",");
if (getDatasetId() != null)
sb.append("DatasetId: ").append(getDatasetId()).append(",");
if (getAutoUpdate() != null)
sb.append("AutoUpdate: ").append(getAutoUpdate()).append(",");
if (getSortColumns() != null)
sb.append("SortColumns: ").append(getSortColumns()).append(",");
if (getPartitionColumns() != null)
sb.append("PartitionColumns: ").append(getPartitionColumns()).append(",");
if (getAsOfTimestamp() != null)
sb.append("AsOfTimestamp: ").append(getAsOfTimestamp()).append(",");
if (getDestinationTypeParams() != null)
sb.append("DestinationTypeParams: ").append(getDestinationTypeParams());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof CreateDataViewRequest == false)
return false;
CreateDataViewRequest other = (CreateDataViewRequest) obj;
if (other.getClientToken() == null ^ this.getClientToken() == null)
return false;
if (other.getClientToken() != null && other.getClientToken().equals(this.getClientToken()) == false)
return false;
if (other.getDatasetId() == null ^ this.getDatasetId() == null)
return false;
if (other.getDatasetId() != null && other.getDatasetId().equals(this.getDatasetId()) == false)
return false;
if (other.getAutoUpdate() == null ^ this.getAutoUpdate() == null)
return false;
if (other.getAutoUpdate() != null && other.getAutoUpdate().equals(this.getAutoUpdate()) == false)
return false;
if (other.getSortColumns() == null ^ this.getSortColumns() == null)
return false;
if (other.getSortColumns() != null && other.getSortColumns().equals(this.getSortColumns()) == false)
return false;
if (other.getPartitionColumns() == null ^ this.getPartitionColumns() == null)
return false;
if (other.getPartitionColumns() != null && other.getPartitionColumns().equals(this.getPartitionColumns()) == false)
return false;
if (other.getAsOfTimestamp() == null ^ this.getAsOfTimestamp() == null)
return false;
if (other.getAsOfTimestamp() != null && other.getAsOfTimestamp().equals(this.getAsOfTimestamp()) == false)
return false;
if (other.getDestinationTypeParams() == null ^ this.getDestinationTypeParams() == null)
return false;
if (other.getDestinationTypeParams() != null && other.getDestinationTypeParams().equals(this.getDestinationTypeParams()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getClientToken() == null) ? 0 : getClientToken().hashCode());
hashCode = prime * hashCode + ((getDatasetId() == null) ? 0 : getDatasetId().hashCode());
hashCode = prime * hashCode + ((getAutoUpdate() == null) ? 0 : getAutoUpdate().hashCode());
hashCode = prime * hashCode + ((getSortColumns() == null) ? 0 : getSortColumns().hashCode());
hashCode = prime * hashCode + ((getPartitionColumns() == null) ? 0 : getPartitionColumns().hashCode());
hashCode = prime * hashCode + ((getAsOfTimestamp() == null) ? 0 : getAsOfTimestamp().hashCode());
hashCode = prime * hashCode + ((getDestinationTypeParams() == null) ? 0 : getDestinationTypeParams().hashCode());
return hashCode;
}
@Override
public CreateDataViewRequest clone() {
return (CreateDataViewRequest) super.clone();
}
}