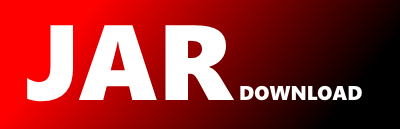
com.amazonaws.services.finspacedata.model.DataViewSummary Maven / Gradle / Ivy
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.finspacedata.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Structure for the summary of a Dataview.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class DataViewSummary implements Serializable, Cloneable, StructuredPojo {
/**
*
* The unique identifier for the Dataview.
*
*/
private String dataViewId;
/**
*
* The ARN identifier of the Dataview.
*
*/
private String dataViewArn;
/**
*
* Th unique identifier for the Dataview Dataset.
*
*/
private String datasetId;
/**
*
* Time range to use for the Dataview. The value is determined as epoch time in milliseconds. For example, the value
* for Monday, November 1, 2021 12:00:00 PM UTC is specified as 1635768000000.
*
*/
private Long asOfTimestamp;
/**
*
* Ordered set of column names used to partition data.
*
*/
private java.util.List partitionColumns;
/**
*
* Columns to be used for sorting the data.
*
*/
private java.util.List sortColumns;
/**
*
* The status of a Dataview creation.
*
*
* -
*
* RUNNING
– Dataview creation is running.
*
*
* -
*
* STARTING
– Dataview creation is starting.
*
*
* -
*
* FAILED
– Dataview creation has failed.
*
*
* -
*
* CANCELLED
– Dataview creation has been cancelled.
*
*
* -
*
* TIMEOUT
– Dataview creation has timed out.
*
*
* -
*
* SUCCESS
– Dataview creation has succeeded.
*
*
* -
*
* PENDING
– Dataview creation is pending.
*
*
* -
*
* FAILED_CLEANUP_FAILED
– Dataview creation failed and resource cleanup failed.
*
*
*
*/
private String status;
/**
*
* The structure with error messages.
*
*/
private DataViewErrorInfo errorInfo;
/**
*
* Information about the Dataview destination.
*
*/
private DataViewDestinationTypeParams destinationTypeProperties;
/**
*
* The flag to indicate Dataview should be updated automatically.
*
*/
private Boolean autoUpdate;
/**
*
* The timestamp at which the Dataview was created in FinSpace. The value is determined as epoch time in
* milliseconds. For example, the value for Monday, November 1, 2021 12:00:00 PM UTC is specified as 1635768000000.
*
*/
private Long createTime;
/**
*
* The last time that a Dataview was modified. The value is determined as epoch time in milliseconds. For example,
* the value for Monday, November 1, 2021 12:00:00 PM UTC is specified as 1635768000000.
*
*/
private Long lastModifiedTime;
/**
*
* The unique identifier for the Dataview.
*
*
* @param dataViewId
* The unique identifier for the Dataview.
*/
public void setDataViewId(String dataViewId) {
this.dataViewId = dataViewId;
}
/**
*
* The unique identifier for the Dataview.
*
*
* @return The unique identifier for the Dataview.
*/
public String getDataViewId() {
return this.dataViewId;
}
/**
*
* The unique identifier for the Dataview.
*
*
* @param dataViewId
* The unique identifier for the Dataview.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DataViewSummary withDataViewId(String dataViewId) {
setDataViewId(dataViewId);
return this;
}
/**
*
* The ARN identifier of the Dataview.
*
*
* @param dataViewArn
* The ARN identifier of the Dataview.
*/
public void setDataViewArn(String dataViewArn) {
this.dataViewArn = dataViewArn;
}
/**
*
* The ARN identifier of the Dataview.
*
*
* @return The ARN identifier of the Dataview.
*/
public String getDataViewArn() {
return this.dataViewArn;
}
/**
*
* The ARN identifier of the Dataview.
*
*
* @param dataViewArn
* The ARN identifier of the Dataview.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DataViewSummary withDataViewArn(String dataViewArn) {
setDataViewArn(dataViewArn);
return this;
}
/**
*
* Th unique identifier for the Dataview Dataset.
*
*
* @param datasetId
* Th unique identifier for the Dataview Dataset.
*/
public void setDatasetId(String datasetId) {
this.datasetId = datasetId;
}
/**
*
* Th unique identifier for the Dataview Dataset.
*
*
* @return Th unique identifier for the Dataview Dataset.
*/
public String getDatasetId() {
return this.datasetId;
}
/**
*
* Th unique identifier for the Dataview Dataset.
*
*
* @param datasetId
* Th unique identifier for the Dataview Dataset.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DataViewSummary withDatasetId(String datasetId) {
setDatasetId(datasetId);
return this;
}
/**
*
* Time range to use for the Dataview. The value is determined as epoch time in milliseconds. For example, the value
* for Monday, November 1, 2021 12:00:00 PM UTC is specified as 1635768000000.
*
*
* @param asOfTimestamp
* Time range to use for the Dataview. The value is determined as epoch time in milliseconds. For example,
* the value for Monday, November 1, 2021 12:00:00 PM UTC is specified as 1635768000000.
*/
public void setAsOfTimestamp(Long asOfTimestamp) {
this.asOfTimestamp = asOfTimestamp;
}
/**
*
* Time range to use for the Dataview. The value is determined as epoch time in milliseconds. For example, the value
* for Monday, November 1, 2021 12:00:00 PM UTC is specified as 1635768000000.
*
*
* @return Time range to use for the Dataview. The value is determined as epoch time in milliseconds. For example,
* the value for Monday, November 1, 2021 12:00:00 PM UTC is specified as 1635768000000.
*/
public Long getAsOfTimestamp() {
return this.asOfTimestamp;
}
/**
*
* Time range to use for the Dataview. The value is determined as epoch time in milliseconds. For example, the value
* for Monday, November 1, 2021 12:00:00 PM UTC is specified as 1635768000000.
*
*
* @param asOfTimestamp
* Time range to use for the Dataview. The value is determined as epoch time in milliseconds. For example,
* the value for Monday, November 1, 2021 12:00:00 PM UTC is specified as 1635768000000.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DataViewSummary withAsOfTimestamp(Long asOfTimestamp) {
setAsOfTimestamp(asOfTimestamp);
return this;
}
/**
*
* Ordered set of column names used to partition data.
*
*
* @return Ordered set of column names used to partition data.
*/
public java.util.List getPartitionColumns() {
return partitionColumns;
}
/**
*
* Ordered set of column names used to partition data.
*
*
* @param partitionColumns
* Ordered set of column names used to partition data.
*/
public void setPartitionColumns(java.util.Collection partitionColumns) {
if (partitionColumns == null) {
this.partitionColumns = null;
return;
}
this.partitionColumns = new java.util.ArrayList(partitionColumns);
}
/**
*
* Ordered set of column names used to partition data.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setPartitionColumns(java.util.Collection)} or {@link #withPartitionColumns(java.util.Collection)} if you
* want to override the existing values.
*
*
* @param partitionColumns
* Ordered set of column names used to partition data.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DataViewSummary withPartitionColumns(String... partitionColumns) {
if (this.partitionColumns == null) {
setPartitionColumns(new java.util.ArrayList(partitionColumns.length));
}
for (String ele : partitionColumns) {
this.partitionColumns.add(ele);
}
return this;
}
/**
*
* Ordered set of column names used to partition data.
*
*
* @param partitionColumns
* Ordered set of column names used to partition data.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DataViewSummary withPartitionColumns(java.util.Collection partitionColumns) {
setPartitionColumns(partitionColumns);
return this;
}
/**
*
* Columns to be used for sorting the data.
*
*
* @return Columns to be used for sorting the data.
*/
public java.util.List getSortColumns() {
return sortColumns;
}
/**
*
* Columns to be used for sorting the data.
*
*
* @param sortColumns
* Columns to be used for sorting the data.
*/
public void setSortColumns(java.util.Collection sortColumns) {
if (sortColumns == null) {
this.sortColumns = null;
return;
}
this.sortColumns = new java.util.ArrayList(sortColumns);
}
/**
*
* Columns to be used for sorting the data.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setSortColumns(java.util.Collection)} or {@link #withSortColumns(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param sortColumns
* Columns to be used for sorting the data.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DataViewSummary withSortColumns(String... sortColumns) {
if (this.sortColumns == null) {
setSortColumns(new java.util.ArrayList(sortColumns.length));
}
for (String ele : sortColumns) {
this.sortColumns.add(ele);
}
return this;
}
/**
*
* Columns to be used for sorting the data.
*
*
* @param sortColumns
* Columns to be used for sorting the data.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DataViewSummary withSortColumns(java.util.Collection sortColumns) {
setSortColumns(sortColumns);
return this;
}
/**
*
* The status of a Dataview creation.
*
*
* -
*
* RUNNING
– Dataview creation is running.
*
*
* -
*
* STARTING
– Dataview creation is starting.
*
*
* -
*
* FAILED
– Dataview creation has failed.
*
*
* -
*
* CANCELLED
– Dataview creation has been cancelled.
*
*
* -
*
* TIMEOUT
– Dataview creation has timed out.
*
*
* -
*
* SUCCESS
– Dataview creation has succeeded.
*
*
* -
*
* PENDING
– Dataview creation is pending.
*
*
* -
*
* FAILED_CLEANUP_FAILED
– Dataview creation failed and resource cleanup failed.
*
*
*
*
* @param status
* The status of a Dataview creation.
*
* -
*
* RUNNING
– Dataview creation is running.
*
*
* -
*
* STARTING
– Dataview creation is starting.
*
*
* -
*
* FAILED
– Dataview creation has failed.
*
*
* -
*
* CANCELLED
– Dataview creation has been cancelled.
*
*
* -
*
* TIMEOUT
– Dataview creation has timed out.
*
*
* -
*
* SUCCESS
– Dataview creation has succeeded.
*
*
* -
*
* PENDING
– Dataview creation is pending.
*
*
* -
*
* FAILED_CLEANUP_FAILED
– Dataview creation failed and resource cleanup failed.
*
*
* @see DataViewStatus
*/
public void setStatus(String status) {
this.status = status;
}
/**
*
* The status of a Dataview creation.
*
*
* -
*
* RUNNING
– Dataview creation is running.
*
*
* -
*
* STARTING
– Dataview creation is starting.
*
*
* -
*
* FAILED
– Dataview creation has failed.
*
*
* -
*
* CANCELLED
– Dataview creation has been cancelled.
*
*
* -
*
* TIMEOUT
– Dataview creation has timed out.
*
*
* -
*
* SUCCESS
– Dataview creation has succeeded.
*
*
* -
*
* PENDING
– Dataview creation is pending.
*
*
* -
*
* FAILED_CLEANUP_FAILED
– Dataview creation failed and resource cleanup failed.
*
*
*
*
* @return The status of a Dataview creation.
*
* -
*
* RUNNING
– Dataview creation is running.
*
*
* -
*
* STARTING
– Dataview creation is starting.
*
*
* -
*
* FAILED
– Dataview creation has failed.
*
*
* -
*
* CANCELLED
– Dataview creation has been cancelled.
*
*
* -
*
* TIMEOUT
– Dataview creation has timed out.
*
*
* -
*
* SUCCESS
– Dataview creation has succeeded.
*
*
* -
*
* PENDING
– Dataview creation is pending.
*
*
* -
*
* FAILED_CLEANUP_FAILED
– Dataview creation failed and resource cleanup failed.
*
*
* @see DataViewStatus
*/
public String getStatus() {
return this.status;
}
/**
*
* The status of a Dataview creation.
*
*
* -
*
* RUNNING
– Dataview creation is running.
*
*
* -
*
* STARTING
– Dataview creation is starting.
*
*
* -
*
* FAILED
– Dataview creation has failed.
*
*
* -
*
* CANCELLED
– Dataview creation has been cancelled.
*
*
* -
*
* TIMEOUT
– Dataview creation has timed out.
*
*
* -
*
* SUCCESS
– Dataview creation has succeeded.
*
*
* -
*
* PENDING
– Dataview creation is pending.
*
*
* -
*
* FAILED_CLEANUP_FAILED
– Dataview creation failed and resource cleanup failed.
*
*
*
*
* @param status
* The status of a Dataview creation.
*
* -
*
* RUNNING
– Dataview creation is running.
*
*
* -
*
* STARTING
– Dataview creation is starting.
*
*
* -
*
* FAILED
– Dataview creation has failed.
*
*
* -
*
* CANCELLED
– Dataview creation has been cancelled.
*
*
* -
*
* TIMEOUT
– Dataview creation has timed out.
*
*
* -
*
* SUCCESS
– Dataview creation has succeeded.
*
*
* -
*
* PENDING
– Dataview creation is pending.
*
*
* -
*
* FAILED_CLEANUP_FAILED
– Dataview creation failed and resource cleanup failed.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see DataViewStatus
*/
public DataViewSummary withStatus(String status) {
setStatus(status);
return this;
}
/**
*
* The status of a Dataview creation.
*
*
* -
*
* RUNNING
– Dataview creation is running.
*
*
* -
*
* STARTING
– Dataview creation is starting.
*
*
* -
*
* FAILED
– Dataview creation has failed.
*
*
* -
*
* CANCELLED
– Dataview creation has been cancelled.
*
*
* -
*
* TIMEOUT
– Dataview creation has timed out.
*
*
* -
*
* SUCCESS
– Dataview creation has succeeded.
*
*
* -
*
* PENDING
– Dataview creation is pending.
*
*
* -
*
* FAILED_CLEANUP_FAILED
– Dataview creation failed and resource cleanup failed.
*
*
*
*
* @param status
* The status of a Dataview creation.
*
* -
*
* RUNNING
– Dataview creation is running.
*
*
* -
*
* STARTING
– Dataview creation is starting.
*
*
* -
*
* FAILED
– Dataview creation has failed.
*
*
* -
*
* CANCELLED
– Dataview creation has been cancelled.
*
*
* -
*
* TIMEOUT
– Dataview creation has timed out.
*
*
* -
*
* SUCCESS
– Dataview creation has succeeded.
*
*
* -
*
* PENDING
– Dataview creation is pending.
*
*
* -
*
* FAILED_CLEANUP_FAILED
– Dataview creation failed and resource cleanup failed.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see DataViewStatus
*/
public DataViewSummary withStatus(DataViewStatus status) {
this.status = status.toString();
return this;
}
/**
*
* The structure with error messages.
*
*
* @param errorInfo
* The structure with error messages.
*/
public void setErrorInfo(DataViewErrorInfo errorInfo) {
this.errorInfo = errorInfo;
}
/**
*
* The structure with error messages.
*
*
* @return The structure with error messages.
*/
public DataViewErrorInfo getErrorInfo() {
return this.errorInfo;
}
/**
*
* The structure with error messages.
*
*
* @param errorInfo
* The structure with error messages.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DataViewSummary withErrorInfo(DataViewErrorInfo errorInfo) {
setErrorInfo(errorInfo);
return this;
}
/**
*
* Information about the Dataview destination.
*
*
* @param destinationTypeProperties
* Information about the Dataview destination.
*/
public void setDestinationTypeProperties(DataViewDestinationTypeParams destinationTypeProperties) {
this.destinationTypeProperties = destinationTypeProperties;
}
/**
*
* Information about the Dataview destination.
*
*
* @return Information about the Dataview destination.
*/
public DataViewDestinationTypeParams getDestinationTypeProperties() {
return this.destinationTypeProperties;
}
/**
*
* Information about the Dataview destination.
*
*
* @param destinationTypeProperties
* Information about the Dataview destination.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DataViewSummary withDestinationTypeProperties(DataViewDestinationTypeParams destinationTypeProperties) {
setDestinationTypeProperties(destinationTypeProperties);
return this;
}
/**
*
* The flag to indicate Dataview should be updated automatically.
*
*
* @param autoUpdate
* The flag to indicate Dataview should be updated automatically.
*/
public void setAutoUpdate(Boolean autoUpdate) {
this.autoUpdate = autoUpdate;
}
/**
*
* The flag to indicate Dataview should be updated automatically.
*
*
* @return The flag to indicate Dataview should be updated automatically.
*/
public Boolean getAutoUpdate() {
return this.autoUpdate;
}
/**
*
* The flag to indicate Dataview should be updated automatically.
*
*
* @param autoUpdate
* The flag to indicate Dataview should be updated automatically.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DataViewSummary withAutoUpdate(Boolean autoUpdate) {
setAutoUpdate(autoUpdate);
return this;
}
/**
*
* The flag to indicate Dataview should be updated automatically.
*
*
* @return The flag to indicate Dataview should be updated automatically.
*/
public Boolean isAutoUpdate() {
return this.autoUpdate;
}
/**
*
* The timestamp at which the Dataview was created in FinSpace. The value is determined as epoch time in
* milliseconds. For example, the value for Monday, November 1, 2021 12:00:00 PM UTC is specified as 1635768000000.
*
*
* @param createTime
* The timestamp at which the Dataview was created in FinSpace. The value is determined as epoch time in
* milliseconds. For example, the value for Monday, November 1, 2021 12:00:00 PM UTC is specified as
* 1635768000000.
*/
public void setCreateTime(Long createTime) {
this.createTime = createTime;
}
/**
*
* The timestamp at which the Dataview was created in FinSpace. The value is determined as epoch time in
* milliseconds. For example, the value for Monday, November 1, 2021 12:00:00 PM UTC is specified as 1635768000000.
*
*
* @return The timestamp at which the Dataview was created in FinSpace. The value is determined as epoch time in
* milliseconds. For example, the value for Monday, November 1, 2021 12:00:00 PM UTC is specified as
* 1635768000000.
*/
public Long getCreateTime() {
return this.createTime;
}
/**
*
* The timestamp at which the Dataview was created in FinSpace. The value is determined as epoch time in
* milliseconds. For example, the value for Monday, November 1, 2021 12:00:00 PM UTC is specified as 1635768000000.
*
*
* @param createTime
* The timestamp at which the Dataview was created in FinSpace. The value is determined as epoch time in
* milliseconds. For example, the value for Monday, November 1, 2021 12:00:00 PM UTC is specified as
* 1635768000000.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DataViewSummary withCreateTime(Long createTime) {
setCreateTime(createTime);
return this;
}
/**
*
* The last time that a Dataview was modified. The value is determined as epoch time in milliseconds. For example,
* the value for Monday, November 1, 2021 12:00:00 PM UTC is specified as 1635768000000.
*
*
* @param lastModifiedTime
* The last time that a Dataview was modified. The value is determined as epoch time in milliseconds. For
* example, the value for Monday, November 1, 2021 12:00:00 PM UTC is specified as 1635768000000.
*/
public void setLastModifiedTime(Long lastModifiedTime) {
this.lastModifiedTime = lastModifiedTime;
}
/**
*
* The last time that a Dataview was modified. The value is determined as epoch time in milliseconds. For example,
* the value for Monday, November 1, 2021 12:00:00 PM UTC is specified as 1635768000000.
*
*
* @return The last time that a Dataview was modified. The value is determined as epoch time in milliseconds. For
* example, the value for Monday, November 1, 2021 12:00:00 PM UTC is specified as 1635768000000.
*/
public Long getLastModifiedTime() {
return this.lastModifiedTime;
}
/**
*
* The last time that a Dataview was modified. The value is determined as epoch time in milliseconds. For example,
* the value for Monday, November 1, 2021 12:00:00 PM UTC is specified as 1635768000000.
*
*
* @param lastModifiedTime
* The last time that a Dataview was modified. The value is determined as epoch time in milliseconds. For
* example, the value for Monday, November 1, 2021 12:00:00 PM UTC is specified as 1635768000000.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DataViewSummary withLastModifiedTime(Long lastModifiedTime) {
setLastModifiedTime(lastModifiedTime);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getDataViewId() != null)
sb.append("DataViewId: ").append(getDataViewId()).append(",");
if (getDataViewArn() != null)
sb.append("DataViewArn: ").append(getDataViewArn()).append(",");
if (getDatasetId() != null)
sb.append("DatasetId: ").append(getDatasetId()).append(",");
if (getAsOfTimestamp() != null)
sb.append("AsOfTimestamp: ").append(getAsOfTimestamp()).append(",");
if (getPartitionColumns() != null)
sb.append("PartitionColumns: ").append(getPartitionColumns()).append(",");
if (getSortColumns() != null)
sb.append("SortColumns: ").append(getSortColumns()).append(",");
if (getStatus() != null)
sb.append("Status: ").append(getStatus()).append(",");
if (getErrorInfo() != null)
sb.append("ErrorInfo: ").append(getErrorInfo()).append(",");
if (getDestinationTypeProperties() != null)
sb.append("DestinationTypeProperties: ").append(getDestinationTypeProperties()).append(",");
if (getAutoUpdate() != null)
sb.append("AutoUpdate: ").append(getAutoUpdate()).append(",");
if (getCreateTime() != null)
sb.append("CreateTime: ").append(getCreateTime()).append(",");
if (getLastModifiedTime() != null)
sb.append("LastModifiedTime: ").append(getLastModifiedTime());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof DataViewSummary == false)
return false;
DataViewSummary other = (DataViewSummary) obj;
if (other.getDataViewId() == null ^ this.getDataViewId() == null)
return false;
if (other.getDataViewId() != null && other.getDataViewId().equals(this.getDataViewId()) == false)
return false;
if (other.getDataViewArn() == null ^ this.getDataViewArn() == null)
return false;
if (other.getDataViewArn() != null && other.getDataViewArn().equals(this.getDataViewArn()) == false)
return false;
if (other.getDatasetId() == null ^ this.getDatasetId() == null)
return false;
if (other.getDatasetId() != null && other.getDatasetId().equals(this.getDatasetId()) == false)
return false;
if (other.getAsOfTimestamp() == null ^ this.getAsOfTimestamp() == null)
return false;
if (other.getAsOfTimestamp() != null && other.getAsOfTimestamp().equals(this.getAsOfTimestamp()) == false)
return false;
if (other.getPartitionColumns() == null ^ this.getPartitionColumns() == null)
return false;
if (other.getPartitionColumns() != null && other.getPartitionColumns().equals(this.getPartitionColumns()) == false)
return false;
if (other.getSortColumns() == null ^ this.getSortColumns() == null)
return false;
if (other.getSortColumns() != null && other.getSortColumns().equals(this.getSortColumns()) == false)
return false;
if (other.getStatus() == null ^ this.getStatus() == null)
return false;
if (other.getStatus() != null && other.getStatus().equals(this.getStatus()) == false)
return false;
if (other.getErrorInfo() == null ^ this.getErrorInfo() == null)
return false;
if (other.getErrorInfo() != null && other.getErrorInfo().equals(this.getErrorInfo()) == false)
return false;
if (other.getDestinationTypeProperties() == null ^ this.getDestinationTypeProperties() == null)
return false;
if (other.getDestinationTypeProperties() != null && other.getDestinationTypeProperties().equals(this.getDestinationTypeProperties()) == false)
return false;
if (other.getAutoUpdate() == null ^ this.getAutoUpdate() == null)
return false;
if (other.getAutoUpdate() != null && other.getAutoUpdate().equals(this.getAutoUpdate()) == false)
return false;
if (other.getCreateTime() == null ^ this.getCreateTime() == null)
return false;
if (other.getCreateTime() != null && other.getCreateTime().equals(this.getCreateTime()) == false)
return false;
if (other.getLastModifiedTime() == null ^ this.getLastModifiedTime() == null)
return false;
if (other.getLastModifiedTime() != null && other.getLastModifiedTime().equals(this.getLastModifiedTime()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getDataViewId() == null) ? 0 : getDataViewId().hashCode());
hashCode = prime * hashCode + ((getDataViewArn() == null) ? 0 : getDataViewArn().hashCode());
hashCode = prime * hashCode + ((getDatasetId() == null) ? 0 : getDatasetId().hashCode());
hashCode = prime * hashCode + ((getAsOfTimestamp() == null) ? 0 : getAsOfTimestamp().hashCode());
hashCode = prime * hashCode + ((getPartitionColumns() == null) ? 0 : getPartitionColumns().hashCode());
hashCode = prime * hashCode + ((getSortColumns() == null) ? 0 : getSortColumns().hashCode());
hashCode = prime * hashCode + ((getStatus() == null) ? 0 : getStatus().hashCode());
hashCode = prime * hashCode + ((getErrorInfo() == null) ? 0 : getErrorInfo().hashCode());
hashCode = prime * hashCode + ((getDestinationTypeProperties() == null) ? 0 : getDestinationTypeProperties().hashCode());
hashCode = prime * hashCode + ((getAutoUpdate() == null) ? 0 : getAutoUpdate().hashCode());
hashCode = prime * hashCode + ((getCreateTime() == null) ? 0 : getCreateTime().hashCode());
hashCode = prime * hashCode + ((getLastModifiedTime() == null) ? 0 : getLastModifiedTime().hashCode());
return hashCode;
}
@Override
public DataViewSummary clone() {
try {
return (DataViewSummary) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.finspacedata.model.transform.DataViewSummaryMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}