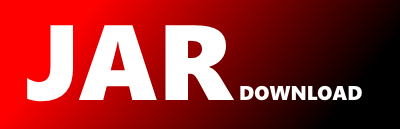
com.amazonaws.services.finspacedata.AWSFinSpaceData Maven / Gradle / Ivy
Show all versions of aws-java-sdk-finspacedata Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.finspacedata;
import javax.annotation.Generated;
import com.amazonaws.*;
import com.amazonaws.regions.*;
import com.amazonaws.services.finspacedata.model.*;
/**
* Interface for accessing FinSpace Data.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.finspacedata.AbstractAWSFinSpaceData} instead.
*
*
*
* The FinSpace APIs let you take actions inside the FinSpace.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AWSFinSpaceData {
/**
* The region metadata service name for computing region endpoints. You can use this value to retrieve metadata
* (such as supported regions) of the service.
*
* @see RegionUtils#getRegionsForService(String)
*/
String ENDPOINT_PREFIX = "finspace-api";
/**
*
* Adds a user to a permission group to grant permissions for actions a user can perform in FinSpace.
*
*
* @param associateUserToPermissionGroupRequest
* @return Result of the AssociateUserToPermissionGroup operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws ConflictException
* The request conflicts with an existing resource.
* @sample AWSFinSpaceData.AssociateUserToPermissionGroup
* @see AWS API Documentation
*/
@Deprecated
AssociateUserToPermissionGroupResult associateUserToPermissionGroup(AssociateUserToPermissionGroupRequest associateUserToPermissionGroupRequest);
/**
*
* Creates a new Changeset in a FinSpace Dataset.
*
*
* @param createChangesetRequest
* The request for a CreateChangeset operation.
* @return Result of the CreateChangeset operation returned by the service.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws LimitExceededException
* A limit has exceeded.
* @throws ConflictException
* The request conflicts with an existing resource.
* @sample AWSFinSpaceData.CreateChangeset
* @see AWS API
* Documentation
*/
@Deprecated
CreateChangesetResult createChangeset(CreateChangesetRequest createChangesetRequest);
/**
*
* Creates a Dataview for a Dataset.
*
*
* @param createDataViewRequest
* Request for creating a data view.
* @return Result of the CreateDataView operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws LimitExceededException
* A limit has exceeded.
* @throws ConflictException
* The request conflicts with an existing resource.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @sample AWSFinSpaceData.CreateDataView
* @see AWS API
* Documentation
*/
@Deprecated
CreateDataViewResult createDataView(CreateDataViewRequest createDataViewRequest);
/**
*
* Creates a new FinSpace Dataset.
*
*
* @param createDatasetRequest
* The request for a CreateDataset operation
* @return Result of the CreateDataset operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws LimitExceededException
* A limit has exceeded.
* @throws ConflictException
* The request conflicts with an existing resource.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @sample AWSFinSpaceData.CreateDataset
* @see AWS API
* Documentation
*/
@Deprecated
CreateDatasetResult createDataset(CreateDatasetRequest createDatasetRequest);
/**
*
* Creates a group of permissions for various actions that a user can perform in FinSpace.
*
*
* @param createPermissionGroupRequest
* @return Result of the CreatePermissionGroup operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws LimitExceededException
* A limit has exceeded.
* @throws ConflictException
* The request conflicts with an existing resource.
* @sample AWSFinSpaceData.CreatePermissionGroup
* @see AWS
* API Documentation
*/
@Deprecated
CreatePermissionGroupResult createPermissionGroup(CreatePermissionGroupRequest createPermissionGroupRequest);
/**
*
* Creates a new user in FinSpace.
*
*
* @param createUserRequest
* @return Result of the CreateUser operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws LimitExceededException
* A limit has exceeded.
* @throws ConflictException
* The request conflicts with an existing resource.
* @sample AWSFinSpaceData.CreateUser
* @see AWS API
* Documentation
*/
@Deprecated
CreateUserResult createUser(CreateUserRequest createUserRequest);
/**
*
* Deletes a FinSpace Dataset.
*
*
* @param deleteDatasetRequest
* The request for a DeleteDataset operation.
* @return Result of the DeleteDataset operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws LimitExceededException
* A limit has exceeded.
* @throws ConflictException
* The request conflicts with an existing resource.
* @sample AWSFinSpaceData.DeleteDataset
* @see AWS API
* Documentation
*/
@Deprecated
DeleteDatasetResult deleteDataset(DeleteDatasetRequest deleteDatasetRequest);
/**
*
* Deletes a permission group. This action is irreversible.
*
*
* @param deletePermissionGroupRequest
* @return Result of the DeletePermissionGroup operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws LimitExceededException
* A limit has exceeded.
* @throws ConflictException
* The request conflicts with an existing resource.
* @sample AWSFinSpaceData.DeletePermissionGroup
* @see AWS
* API Documentation
*/
@Deprecated
DeletePermissionGroupResult deletePermissionGroup(DeletePermissionGroupRequest deletePermissionGroupRequest);
/**
*
* Denies access to the FinSpace web application and API for the specified user.
*
*
* @param disableUserRequest
* @return Result of the DisableUser operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws ConflictException
* The request conflicts with an existing resource.
* @sample AWSFinSpaceData.DisableUser
* @see AWS API
* Documentation
*/
@Deprecated
DisableUserResult disableUser(DisableUserRequest disableUserRequest);
/**
*
* Removes a user from a permission group.
*
*
* @param disassociateUserFromPermissionGroupRequest
* @return Result of the DisassociateUserFromPermissionGroup operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws ConflictException
* The request conflicts with an existing resource.
* @sample AWSFinSpaceData.DisassociateUserFromPermissionGroup
* @see AWS API Documentation
*/
@Deprecated
DisassociateUserFromPermissionGroupResult disassociateUserFromPermissionGroup(
DisassociateUserFromPermissionGroupRequest disassociateUserFromPermissionGroupRequest);
/**
*
* Allows the specified user to access the FinSpace web application and API.
*
*
* @param enableUserRequest
* @return Result of the EnableUser operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws LimitExceededException
* A limit has exceeded.
* @throws ConflictException
* The request conflicts with an existing resource.
* @sample AWSFinSpaceData.EnableUser
* @see AWS API
* Documentation
*/
@Deprecated
EnableUserResult enableUser(EnableUserRequest enableUserRequest);
/**
*
* Get information about a Changeset.
*
*
* @param getChangesetRequest
* Request to describe a changeset.
* @return Result of the GetChangeset operation returned by the service.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ConflictException
* The request conflicts with an existing resource.
* @sample AWSFinSpaceData.GetChangeset
* @see AWS API
* Documentation
*/
@Deprecated
GetChangesetResult getChangeset(GetChangesetRequest getChangesetRequest);
/**
*
* Gets information about a Dataview.
*
*
* @param getDataViewRequest
* Request for retrieving a data view detail. Grouped / accessible within a dataset by its dataset id.
* @return Result of the GetDataView operation returned by the service.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws ConflictException
* The request conflicts with an existing resource.
* @sample AWSFinSpaceData.GetDataView
* @see AWS API
* Documentation
*/
@Deprecated
GetDataViewResult getDataView(GetDataViewRequest getDataViewRequest);
/**
*
* Returns information about a Dataset.
*
*
* @param getDatasetRequest
* Request for the GetDataset operation.
* @return Result of the GetDataset operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws ConflictException
* The request conflicts with an existing resource.
* @sample AWSFinSpaceData.GetDataset
* @see AWS API
* Documentation
*/
@Deprecated
GetDatasetResult getDataset(GetDatasetRequest getDatasetRequest);
/**
*
* Returns the credentials to access the external Dataview from an S3 location. To call this API:
*
*
* -
*
* You must retrieve the programmatic credentials.
*
*
* -
*
* You must be a member of a FinSpace user group, where the dataset that you want to access has
* Read Dataset Data
permissions.
*
*
*
*
* @param getExternalDataViewAccessDetailsRequest
* @return Result of the GetExternalDataViewAccessDetails operation returned by the service.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @sample AWSFinSpaceData.GetExternalDataViewAccessDetails
* @see AWS API Documentation
*/
@Deprecated
GetExternalDataViewAccessDetailsResult getExternalDataViewAccessDetails(GetExternalDataViewAccessDetailsRequest getExternalDataViewAccessDetailsRequest);
/**
*
* Retrieves the details of a specific permission group.
*
*
* @param getPermissionGroupRequest
* @return Result of the GetPermissionGroup operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @sample AWSFinSpaceData.GetPermissionGroup
* @see AWS
* API Documentation
*/
@Deprecated
GetPermissionGroupResult getPermissionGroup(GetPermissionGroupRequest getPermissionGroupRequest);
/**
*
* Request programmatic credentials to use with FinSpace SDK. For more information, see Step
* 2. Access credentials programmatically using IAM access key id and secret access key.
*
*
* @param getProgrammaticAccessCredentialsRequest
* Request for GetProgrammaticAccessCredentials operation
* @return Result of the GetProgrammaticAccessCredentials operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @sample AWSFinSpaceData.GetProgrammaticAccessCredentials
* @see AWS API Documentation
*/
@Deprecated
GetProgrammaticAccessCredentialsResult getProgrammaticAccessCredentials(GetProgrammaticAccessCredentialsRequest getProgrammaticAccessCredentialsRequest);
/**
*
* Retrieves details for a specific user.
*
*
* @param getUserRequest
* @return Result of the GetUser operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @sample AWSFinSpaceData.GetUser
* @see AWS API
* Documentation
*/
@Deprecated
GetUserResult getUser(GetUserRequest getUserRequest);
/**
*
* A temporary Amazon S3 location, where you can copy your files from a source location to stage or use as a scratch
* space in FinSpace notebook.
*
*
* @param getWorkingLocationRequest
* @return Result of the GetWorkingLocation operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @sample AWSFinSpaceData.GetWorkingLocation
* @see AWS
* API Documentation
*/
@Deprecated
GetWorkingLocationResult getWorkingLocation(GetWorkingLocationRequest getWorkingLocationRequest);
/**
*
* Lists the FinSpace Changesets for a Dataset.
*
*
* @param listChangesetsRequest
* Request to ListChangesetsRequest. It exposes minimal query filters.
* @return Result of the ListChangesets operation returned by the service.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ConflictException
* The request conflicts with an existing resource.
* @sample AWSFinSpaceData.ListChangesets
* @see AWS API
* Documentation
*/
@Deprecated
ListChangesetsResult listChangesets(ListChangesetsRequest listChangesetsRequest);
/**
*
* Lists all available Dataviews for a Dataset.
*
*
* @param listDataViewsRequest
* Request for a list data views.
* @return Result of the ListDataViews operation returned by the service.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws ConflictException
* The request conflicts with an existing resource.
* @sample AWSFinSpaceData.ListDataViews
* @see AWS API
* Documentation
*/
@Deprecated
ListDataViewsResult listDataViews(ListDataViewsRequest listDataViewsRequest);
/**
*
* Lists all of the active Datasets that a user has access to.
*
*
* @param listDatasetsRequest
* Request for the ListDatasets operation.
* @return Result of the ListDatasets operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws ConflictException
* The request conflicts with an existing resource.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @sample AWSFinSpaceData.ListDatasets
* @see AWS API
* Documentation
*/
@Deprecated
ListDatasetsResult listDatasets(ListDatasetsRequest listDatasetsRequest);
/**
*
* Lists all available permission groups in FinSpace.
*
*
* @param listPermissionGroupsRequest
* @return Result of the ListPermissionGroups operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @sample AWSFinSpaceData.ListPermissionGroups
* @see AWS
* API Documentation
*/
@Deprecated
ListPermissionGroupsResult listPermissionGroups(ListPermissionGroupsRequest listPermissionGroupsRequest);
/**
*
* Lists all the permission groups that are associated with a specific user.
*
*
* @param listPermissionGroupsByUserRequest
* @return Result of the ListPermissionGroupsByUser operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @sample AWSFinSpaceData.ListPermissionGroupsByUser
* @see AWS API Documentation
*/
@Deprecated
ListPermissionGroupsByUserResult listPermissionGroupsByUser(ListPermissionGroupsByUserRequest listPermissionGroupsByUserRequest);
/**
*
* Lists all available users in FinSpace.
*
*
* @param listUsersRequest
* @return Result of the ListUsers operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @sample AWSFinSpaceData.ListUsers
* @see AWS API
* Documentation
*/
@Deprecated
ListUsersResult listUsers(ListUsersRequest listUsersRequest);
/**
*
* Lists details of all the users in a specific permission group.
*
*
* @param listUsersByPermissionGroupRequest
* @return Result of the ListUsersByPermissionGroup operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @sample AWSFinSpaceData.ListUsersByPermissionGroup
* @see AWS API Documentation
*/
@Deprecated
ListUsersByPermissionGroupResult listUsersByPermissionGroup(ListUsersByPermissionGroupRequest listUsersByPermissionGroupRequest);
/**
*
* Resets the password for a specified user ID and generates a temporary one. Only a superuser can reset password
* for other users. Resetting the password immediately invalidates the previous password associated with the user.
*
*
* @param resetUserPasswordRequest
* @return Result of the ResetUserPassword operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws ConflictException
* The request conflicts with an existing resource.
* @sample AWSFinSpaceData.ResetUserPassword
* @see AWS API
* Documentation
*/
@Deprecated
ResetUserPasswordResult resetUserPassword(ResetUserPasswordRequest resetUserPasswordRequest);
/**
*
* Updates a FinSpace Changeset.
*
*
* @param updateChangesetRequest
* Request to update an existing changeset.
* @return Result of the UpdateChangeset operation returned by the service.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ConflictException
* The request conflicts with an existing resource.
* @sample AWSFinSpaceData.UpdateChangeset
* @see AWS API
* Documentation
*/
@Deprecated
UpdateChangesetResult updateChangeset(UpdateChangesetRequest updateChangesetRequest);
/**
*
* Updates a FinSpace Dataset.
*
*
* @param updateDatasetRequest
* The request for an UpdateDataset operation
* @return Result of the UpdateDataset operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ConflictException
* The request conflicts with an existing resource.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @sample AWSFinSpaceData.UpdateDataset
* @see AWS API
* Documentation
*/
@Deprecated
UpdateDatasetResult updateDataset(UpdateDatasetRequest updateDatasetRequest);
/**
*
* Modifies the details of a permission group. You cannot modify a permissionGroupID
.
*
*
* @param updatePermissionGroupRequest
* @return Result of the UpdatePermissionGroup operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws ConflictException
* The request conflicts with an existing resource.
* @sample AWSFinSpaceData.UpdatePermissionGroup
* @see AWS
* API Documentation
*/
@Deprecated
UpdatePermissionGroupResult updatePermissionGroup(UpdatePermissionGroupRequest updatePermissionGroupRequest);
/**
*
* Modifies the details of the specified user. You cannot update the userId
for a user.
*
*
* @param updateUserRequest
* @return Result of the UpdateUser operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an AWS service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws ConflictException
* The request conflicts with an existing resource.
* @sample AWSFinSpaceData.UpdateUser
* @see AWS API
* Documentation
*/
@Deprecated
UpdateUserResult updateUser(UpdateUserRequest updateUserRequest);
/**
* Shuts down this client object, releasing any resources that might be held open. This is an optional method, and
* callers are not expected to call it, but can if they want to explicitly release any open resources. Once a client
* has been shutdown, it should not be used to make any more requests.
*/
void shutdown();
/**
* Returns additional metadata for a previously executed successful request, typically used for debugging issues
* where a service isn't acting as expected. This data isn't considered part of the result data returned by an
* operation, so it's available through this separate, diagnostic interface.
*
* Response metadata is only cached for a limited period of time, so if you need to access this extra diagnostic
* information for an executed request, you should use this method to retrieve it as soon as possible after
* executing a request.
*
* @param request
* The originally executed request.
*
* @return The response metadata for the specified request, or null if none is available.
*/
ResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request);
}