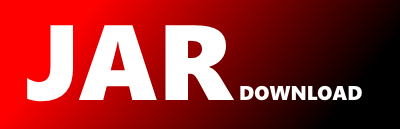
com.amazonaws.services.fms.model.AccountScope Maven / Gradle / Ivy
Show all versions of aws-java-sdk-fms Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.fms.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Configures the accounts within the administrator's Organizations organization that the specified Firewall Manager
* administrator can apply policies to.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class AccountScope implements Serializable, Cloneable, StructuredPojo {
/**
*
* The list of accounts within the organization that the specified Firewall Manager administrator either can or
* cannot apply policies to, based on the value of ExcludeSpecifiedAccounts
. If
* ExcludeSpecifiedAccounts
is set to true
, then the Firewall Manager administrator can
* apply policies to all members of the organization except for the accounts in this list. If
* ExcludeSpecifiedAccounts
is set to false
, then the Firewall Manager administrator can
* only apply policies to the accounts in this list.
*
*/
private java.util.List accounts;
/**
*
* A boolean value that indicates if the administrator can apply policies to all accounts within an organization. If
* true, the administrator can apply policies to all accounts within the organization. You can either enable
* management of all accounts through this operation, or you can specify a list of accounts to manage in
* AccountScope$Accounts
. You cannot specify both.
*
*/
private Boolean allAccountsEnabled;
/**
*
* A boolean value that excludes the accounts in AccountScope$Accounts
from the administrator's scope.
* If true, the Firewall Manager administrator can apply policies to all members of the organization except for the
* accounts listed in AccountScope$Accounts
. You can either specify a list of accounts to exclude by
* AccountScope$Accounts
, or you can enable management of all accounts by
* AccountScope$AllAccountsEnabled
. You cannot specify both.
*
*/
private Boolean excludeSpecifiedAccounts;
/**
*
* The list of accounts within the organization that the specified Firewall Manager administrator either can or
* cannot apply policies to, based on the value of ExcludeSpecifiedAccounts
. If
* ExcludeSpecifiedAccounts
is set to true
, then the Firewall Manager administrator can
* apply policies to all members of the organization except for the accounts in this list. If
* ExcludeSpecifiedAccounts
is set to false
, then the Firewall Manager administrator can
* only apply policies to the accounts in this list.
*
*
* @return The list of accounts within the organization that the specified Firewall Manager administrator either can
* or cannot apply policies to, based on the value of ExcludeSpecifiedAccounts
. If
* ExcludeSpecifiedAccounts
is set to true
, then the Firewall Manager
* administrator can apply policies to all members of the organization except for the accounts in this list.
* If ExcludeSpecifiedAccounts
is set to false
, then the Firewall Manager
* administrator can only apply policies to the accounts in this list.
*/
public java.util.List getAccounts() {
return accounts;
}
/**
*
* The list of accounts within the organization that the specified Firewall Manager administrator either can or
* cannot apply policies to, based on the value of ExcludeSpecifiedAccounts
. If
* ExcludeSpecifiedAccounts
is set to true
, then the Firewall Manager administrator can
* apply policies to all members of the organization except for the accounts in this list. If
* ExcludeSpecifiedAccounts
is set to false
, then the Firewall Manager administrator can
* only apply policies to the accounts in this list.
*
*
* @param accounts
* The list of accounts within the organization that the specified Firewall Manager administrator either can
* or cannot apply policies to, based on the value of ExcludeSpecifiedAccounts
. If
* ExcludeSpecifiedAccounts
is set to true
, then the Firewall Manager administrator
* can apply policies to all members of the organization except for the accounts in this list. If
* ExcludeSpecifiedAccounts
is set to false
, then the Firewall Manager
* administrator can only apply policies to the accounts in this list.
*/
public void setAccounts(java.util.Collection accounts) {
if (accounts == null) {
this.accounts = null;
return;
}
this.accounts = new java.util.ArrayList(accounts);
}
/**
*
* The list of accounts within the organization that the specified Firewall Manager administrator either can or
* cannot apply policies to, based on the value of ExcludeSpecifiedAccounts
. If
* ExcludeSpecifiedAccounts
is set to true
, then the Firewall Manager administrator can
* apply policies to all members of the organization except for the accounts in this list. If
* ExcludeSpecifiedAccounts
is set to false
, then the Firewall Manager administrator can
* only apply policies to the accounts in this list.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setAccounts(java.util.Collection)} or {@link #withAccounts(java.util.Collection)} if you want to override
* the existing values.
*
*
* @param accounts
* The list of accounts within the organization that the specified Firewall Manager administrator either can
* or cannot apply policies to, based on the value of ExcludeSpecifiedAccounts
. If
* ExcludeSpecifiedAccounts
is set to true
, then the Firewall Manager administrator
* can apply policies to all members of the organization except for the accounts in this list. If
* ExcludeSpecifiedAccounts
is set to false
, then the Firewall Manager
* administrator can only apply policies to the accounts in this list.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AccountScope withAccounts(String... accounts) {
if (this.accounts == null) {
setAccounts(new java.util.ArrayList(accounts.length));
}
for (String ele : accounts) {
this.accounts.add(ele);
}
return this;
}
/**
*
* The list of accounts within the organization that the specified Firewall Manager administrator either can or
* cannot apply policies to, based on the value of ExcludeSpecifiedAccounts
. If
* ExcludeSpecifiedAccounts
is set to true
, then the Firewall Manager administrator can
* apply policies to all members of the organization except for the accounts in this list. If
* ExcludeSpecifiedAccounts
is set to false
, then the Firewall Manager administrator can
* only apply policies to the accounts in this list.
*
*
* @param accounts
* The list of accounts within the organization that the specified Firewall Manager administrator either can
* or cannot apply policies to, based on the value of ExcludeSpecifiedAccounts
. If
* ExcludeSpecifiedAccounts
is set to true
, then the Firewall Manager administrator
* can apply policies to all members of the organization except for the accounts in this list. If
* ExcludeSpecifiedAccounts
is set to false
, then the Firewall Manager
* administrator can only apply policies to the accounts in this list.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AccountScope withAccounts(java.util.Collection accounts) {
setAccounts(accounts);
return this;
}
/**
*
* A boolean value that indicates if the administrator can apply policies to all accounts within an organization. If
* true, the administrator can apply policies to all accounts within the organization. You can either enable
* management of all accounts through this operation, or you can specify a list of accounts to manage in
* AccountScope$Accounts
. You cannot specify both.
*
*
* @param allAccountsEnabled
* A boolean value that indicates if the administrator can apply policies to all accounts within an
* organization. If true, the administrator can apply policies to all accounts within the organization. You
* can either enable management of all accounts through this operation, or you can specify a list of accounts
* to manage in AccountScope$Accounts
. You cannot specify both.
*/
public void setAllAccountsEnabled(Boolean allAccountsEnabled) {
this.allAccountsEnabled = allAccountsEnabled;
}
/**
*
* A boolean value that indicates if the administrator can apply policies to all accounts within an organization. If
* true, the administrator can apply policies to all accounts within the organization. You can either enable
* management of all accounts through this operation, or you can specify a list of accounts to manage in
* AccountScope$Accounts
. You cannot specify both.
*
*
* @return A boolean value that indicates if the administrator can apply policies to all accounts within an
* organization. If true, the administrator can apply policies to all accounts within the organization. You
* can either enable management of all accounts through this operation, or you can specify a list of
* accounts to manage in AccountScope$Accounts
. You cannot specify both.
*/
public Boolean getAllAccountsEnabled() {
return this.allAccountsEnabled;
}
/**
*
* A boolean value that indicates if the administrator can apply policies to all accounts within an organization. If
* true, the administrator can apply policies to all accounts within the organization. You can either enable
* management of all accounts through this operation, or you can specify a list of accounts to manage in
* AccountScope$Accounts
. You cannot specify both.
*
*
* @param allAccountsEnabled
* A boolean value that indicates if the administrator can apply policies to all accounts within an
* organization. If true, the administrator can apply policies to all accounts within the organization. You
* can either enable management of all accounts through this operation, or you can specify a list of accounts
* to manage in AccountScope$Accounts
. You cannot specify both.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AccountScope withAllAccountsEnabled(Boolean allAccountsEnabled) {
setAllAccountsEnabled(allAccountsEnabled);
return this;
}
/**
*
* A boolean value that indicates if the administrator can apply policies to all accounts within an organization. If
* true, the administrator can apply policies to all accounts within the organization. You can either enable
* management of all accounts through this operation, or you can specify a list of accounts to manage in
* AccountScope$Accounts
. You cannot specify both.
*
*
* @return A boolean value that indicates if the administrator can apply policies to all accounts within an
* organization. If true, the administrator can apply policies to all accounts within the organization. You
* can either enable management of all accounts through this operation, or you can specify a list of
* accounts to manage in AccountScope$Accounts
. You cannot specify both.
*/
public Boolean isAllAccountsEnabled() {
return this.allAccountsEnabled;
}
/**
*
* A boolean value that excludes the accounts in AccountScope$Accounts
from the administrator's scope.
* If true, the Firewall Manager administrator can apply policies to all members of the organization except for the
* accounts listed in AccountScope$Accounts
. You can either specify a list of accounts to exclude by
* AccountScope$Accounts
, or you can enable management of all accounts by
* AccountScope$AllAccountsEnabled
. You cannot specify both.
*
*
* @param excludeSpecifiedAccounts
* A boolean value that excludes the accounts in AccountScope$Accounts
from the administrator's
* scope. If true, the Firewall Manager administrator can apply policies to all members of the organization
* except for the accounts listed in AccountScope$Accounts
. You can either specify a list of
* accounts to exclude by AccountScope$Accounts
, or you can enable management of all accounts by
* AccountScope$AllAccountsEnabled
. You cannot specify both.
*/
public void setExcludeSpecifiedAccounts(Boolean excludeSpecifiedAccounts) {
this.excludeSpecifiedAccounts = excludeSpecifiedAccounts;
}
/**
*
* A boolean value that excludes the accounts in AccountScope$Accounts
from the administrator's scope.
* If true, the Firewall Manager administrator can apply policies to all members of the organization except for the
* accounts listed in AccountScope$Accounts
. You can either specify a list of accounts to exclude by
* AccountScope$Accounts
, or you can enable management of all accounts by
* AccountScope$AllAccountsEnabled
. You cannot specify both.
*
*
* @return A boolean value that excludes the accounts in AccountScope$Accounts
from the administrator's
* scope. If true, the Firewall Manager administrator can apply policies to all members of the organization
* except for the accounts listed in AccountScope$Accounts
. You can either specify a list of
* accounts to exclude by AccountScope$Accounts
, or you can enable management of all accounts
* by AccountScope$AllAccountsEnabled
. You cannot specify both.
*/
public Boolean getExcludeSpecifiedAccounts() {
return this.excludeSpecifiedAccounts;
}
/**
*
* A boolean value that excludes the accounts in AccountScope$Accounts
from the administrator's scope.
* If true, the Firewall Manager administrator can apply policies to all members of the organization except for the
* accounts listed in AccountScope$Accounts
. You can either specify a list of accounts to exclude by
* AccountScope$Accounts
, or you can enable management of all accounts by
* AccountScope$AllAccountsEnabled
. You cannot specify both.
*
*
* @param excludeSpecifiedAccounts
* A boolean value that excludes the accounts in AccountScope$Accounts
from the administrator's
* scope. If true, the Firewall Manager administrator can apply policies to all members of the organization
* except for the accounts listed in AccountScope$Accounts
. You can either specify a list of
* accounts to exclude by AccountScope$Accounts
, or you can enable management of all accounts by
* AccountScope$AllAccountsEnabled
. You cannot specify both.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AccountScope withExcludeSpecifiedAccounts(Boolean excludeSpecifiedAccounts) {
setExcludeSpecifiedAccounts(excludeSpecifiedAccounts);
return this;
}
/**
*
* A boolean value that excludes the accounts in AccountScope$Accounts
from the administrator's scope.
* If true, the Firewall Manager administrator can apply policies to all members of the organization except for the
* accounts listed in AccountScope$Accounts
. You can either specify a list of accounts to exclude by
* AccountScope$Accounts
, or you can enable management of all accounts by
* AccountScope$AllAccountsEnabled
. You cannot specify both.
*
*
* @return A boolean value that excludes the accounts in AccountScope$Accounts
from the administrator's
* scope. If true, the Firewall Manager administrator can apply policies to all members of the organization
* except for the accounts listed in AccountScope$Accounts
. You can either specify a list of
* accounts to exclude by AccountScope$Accounts
, or you can enable management of all accounts
* by AccountScope$AllAccountsEnabled
. You cannot specify both.
*/
public Boolean isExcludeSpecifiedAccounts() {
return this.excludeSpecifiedAccounts;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getAccounts() != null)
sb.append("Accounts: ").append(getAccounts()).append(",");
if (getAllAccountsEnabled() != null)
sb.append("AllAccountsEnabled: ").append(getAllAccountsEnabled()).append(",");
if (getExcludeSpecifiedAccounts() != null)
sb.append("ExcludeSpecifiedAccounts: ").append(getExcludeSpecifiedAccounts());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof AccountScope == false)
return false;
AccountScope other = (AccountScope) obj;
if (other.getAccounts() == null ^ this.getAccounts() == null)
return false;
if (other.getAccounts() != null && other.getAccounts().equals(this.getAccounts()) == false)
return false;
if (other.getAllAccountsEnabled() == null ^ this.getAllAccountsEnabled() == null)
return false;
if (other.getAllAccountsEnabled() != null && other.getAllAccountsEnabled().equals(this.getAllAccountsEnabled()) == false)
return false;
if (other.getExcludeSpecifiedAccounts() == null ^ this.getExcludeSpecifiedAccounts() == null)
return false;
if (other.getExcludeSpecifiedAccounts() != null && other.getExcludeSpecifiedAccounts().equals(this.getExcludeSpecifiedAccounts()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getAccounts() == null) ? 0 : getAccounts().hashCode());
hashCode = prime * hashCode + ((getAllAccountsEnabled() == null) ? 0 : getAllAccountsEnabled().hashCode());
hashCode = prime * hashCode + ((getExcludeSpecifiedAccounts() == null) ? 0 : getExcludeSpecifiedAccounts().hashCode());
return hashCode;
}
@Override
public AccountScope clone() {
try {
return (AccountScope) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.fms.model.transform.AccountScopeMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}