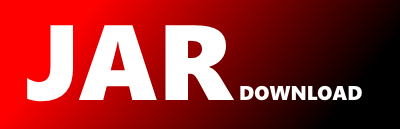
com.amazonaws.services.fms.model.CreateNetworkAclEntriesAction Maven / Gradle / Ivy
Show all versions of aws-java-sdk-fms Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.fms.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Information about the CreateNetworkAclEntries
action in Amazon EC2. This is a remediation option in
* RemediationAction
.
*
*
* @see AWS
* API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class CreateNetworkAclEntriesAction implements Serializable, Cloneable, StructuredPojo {
/**
*
* Brief description of this remediation action.
*
*/
private String description;
/**
*
* The network ACL that's associated with the remediation action.
*
*/
private ActionTarget networkAclId;
/**
*
* Lists the entries that the remediation action would create.
*
*/
private java.util.List networkAclEntriesToBeCreated;
/**
*
* Indicates whether it is possible for Firewall Manager to perform this remediation action. A false value indicates
* that auto remediation is disabled or Firewall Manager is unable to perform the action due to a conflict of some
* kind.
*
*/
private Boolean fMSCanRemediate;
/**
*
* Brief description of this remediation action.
*
*
* @param description
* Brief description of this remediation action.
*/
public void setDescription(String description) {
this.description = description;
}
/**
*
* Brief description of this remediation action.
*
*
* @return Brief description of this remediation action.
*/
public String getDescription() {
return this.description;
}
/**
*
* Brief description of this remediation action.
*
*
* @param description
* Brief description of this remediation action.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateNetworkAclEntriesAction withDescription(String description) {
setDescription(description);
return this;
}
/**
*
* The network ACL that's associated with the remediation action.
*
*
* @param networkAclId
* The network ACL that's associated with the remediation action.
*/
public void setNetworkAclId(ActionTarget networkAclId) {
this.networkAclId = networkAclId;
}
/**
*
* The network ACL that's associated with the remediation action.
*
*
* @return The network ACL that's associated with the remediation action.
*/
public ActionTarget getNetworkAclId() {
return this.networkAclId;
}
/**
*
* The network ACL that's associated with the remediation action.
*
*
* @param networkAclId
* The network ACL that's associated with the remediation action.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateNetworkAclEntriesAction withNetworkAclId(ActionTarget networkAclId) {
setNetworkAclId(networkAclId);
return this;
}
/**
*
* Lists the entries that the remediation action would create.
*
*
* @return Lists the entries that the remediation action would create.
*/
public java.util.List getNetworkAclEntriesToBeCreated() {
return networkAclEntriesToBeCreated;
}
/**
*
* Lists the entries that the remediation action would create.
*
*
* @param networkAclEntriesToBeCreated
* Lists the entries that the remediation action would create.
*/
public void setNetworkAclEntriesToBeCreated(java.util.Collection networkAclEntriesToBeCreated) {
if (networkAclEntriesToBeCreated == null) {
this.networkAclEntriesToBeCreated = null;
return;
}
this.networkAclEntriesToBeCreated = new java.util.ArrayList(networkAclEntriesToBeCreated);
}
/**
*
* Lists the entries that the remediation action would create.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setNetworkAclEntriesToBeCreated(java.util.Collection)} or
* {@link #withNetworkAclEntriesToBeCreated(java.util.Collection)} if you want to override the existing values.
*
*
* @param networkAclEntriesToBeCreated
* Lists the entries that the remediation action would create.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateNetworkAclEntriesAction withNetworkAclEntriesToBeCreated(EntryDescription... networkAclEntriesToBeCreated) {
if (this.networkAclEntriesToBeCreated == null) {
setNetworkAclEntriesToBeCreated(new java.util.ArrayList(networkAclEntriesToBeCreated.length));
}
for (EntryDescription ele : networkAclEntriesToBeCreated) {
this.networkAclEntriesToBeCreated.add(ele);
}
return this;
}
/**
*
* Lists the entries that the remediation action would create.
*
*
* @param networkAclEntriesToBeCreated
* Lists the entries that the remediation action would create.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateNetworkAclEntriesAction withNetworkAclEntriesToBeCreated(java.util.Collection networkAclEntriesToBeCreated) {
setNetworkAclEntriesToBeCreated(networkAclEntriesToBeCreated);
return this;
}
/**
*
* Indicates whether it is possible for Firewall Manager to perform this remediation action. A false value indicates
* that auto remediation is disabled or Firewall Manager is unable to perform the action due to a conflict of some
* kind.
*
*
* @param fMSCanRemediate
* Indicates whether it is possible for Firewall Manager to perform this remediation action. A false value
* indicates that auto remediation is disabled or Firewall Manager is unable to perform the action due to a
* conflict of some kind.
*/
public void setFMSCanRemediate(Boolean fMSCanRemediate) {
this.fMSCanRemediate = fMSCanRemediate;
}
/**
*
* Indicates whether it is possible for Firewall Manager to perform this remediation action. A false value indicates
* that auto remediation is disabled or Firewall Manager is unable to perform the action due to a conflict of some
* kind.
*
*
* @return Indicates whether it is possible for Firewall Manager to perform this remediation action. A false value
* indicates that auto remediation is disabled or Firewall Manager is unable to perform the action due to a
* conflict of some kind.
*/
public Boolean getFMSCanRemediate() {
return this.fMSCanRemediate;
}
/**
*
* Indicates whether it is possible for Firewall Manager to perform this remediation action. A false value indicates
* that auto remediation is disabled or Firewall Manager is unable to perform the action due to a conflict of some
* kind.
*
*
* @param fMSCanRemediate
* Indicates whether it is possible for Firewall Manager to perform this remediation action. A false value
* indicates that auto remediation is disabled or Firewall Manager is unable to perform the action due to a
* conflict of some kind.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateNetworkAclEntriesAction withFMSCanRemediate(Boolean fMSCanRemediate) {
setFMSCanRemediate(fMSCanRemediate);
return this;
}
/**
*
* Indicates whether it is possible for Firewall Manager to perform this remediation action. A false value indicates
* that auto remediation is disabled or Firewall Manager is unable to perform the action due to a conflict of some
* kind.
*
*
* @return Indicates whether it is possible for Firewall Manager to perform this remediation action. A false value
* indicates that auto remediation is disabled or Firewall Manager is unable to perform the action due to a
* conflict of some kind.
*/
public Boolean isFMSCanRemediate() {
return this.fMSCanRemediate;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getDescription() != null)
sb.append("Description: ").append(getDescription()).append(",");
if (getNetworkAclId() != null)
sb.append("NetworkAclId: ").append(getNetworkAclId()).append(",");
if (getNetworkAclEntriesToBeCreated() != null)
sb.append("NetworkAclEntriesToBeCreated: ").append(getNetworkAclEntriesToBeCreated()).append(",");
if (getFMSCanRemediate() != null)
sb.append("FMSCanRemediate: ").append(getFMSCanRemediate());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof CreateNetworkAclEntriesAction == false)
return false;
CreateNetworkAclEntriesAction other = (CreateNetworkAclEntriesAction) obj;
if (other.getDescription() == null ^ this.getDescription() == null)
return false;
if (other.getDescription() != null && other.getDescription().equals(this.getDescription()) == false)
return false;
if (other.getNetworkAclId() == null ^ this.getNetworkAclId() == null)
return false;
if (other.getNetworkAclId() != null && other.getNetworkAclId().equals(this.getNetworkAclId()) == false)
return false;
if (other.getNetworkAclEntriesToBeCreated() == null ^ this.getNetworkAclEntriesToBeCreated() == null)
return false;
if (other.getNetworkAclEntriesToBeCreated() != null && other.getNetworkAclEntriesToBeCreated().equals(this.getNetworkAclEntriesToBeCreated()) == false)
return false;
if (other.getFMSCanRemediate() == null ^ this.getFMSCanRemediate() == null)
return false;
if (other.getFMSCanRemediate() != null && other.getFMSCanRemediate().equals(this.getFMSCanRemediate()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getDescription() == null) ? 0 : getDescription().hashCode());
hashCode = prime * hashCode + ((getNetworkAclId() == null) ? 0 : getNetworkAclId().hashCode());
hashCode = prime * hashCode + ((getNetworkAclEntriesToBeCreated() == null) ? 0 : getNetworkAclEntriesToBeCreated().hashCode());
hashCode = prime * hashCode + ((getFMSCanRemediate() == null) ? 0 : getFMSCanRemediate().hashCode());
return hashCode;
}
@Override
public CreateNetworkAclEntriesAction clone() {
try {
return (CreateNetworkAclEntriesAction) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.fms.model.transform.CreateNetworkAclEntriesActionMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}