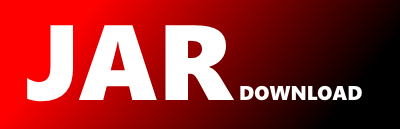
com.amazonaws.services.fms.AWSFMSAsync Maven / Gradle / Ivy
Show all versions of aws-java-sdk-fms Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.fms;
import javax.annotation.Generated;
import com.amazonaws.services.fms.model.*;
/**
* Interface for accessing FMS asynchronously. Each asynchronous method will return a Java Future object representing
* the asynchronous operation; overloads which accept an {@code AsyncHandler} can be used to receive notification when
* an asynchronous operation completes.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.fms.AbstractAWSFMSAsync} instead.
*
*
*
* This is the Firewall Manager API Reference. This guide is for developers who need detailed information about
* the Firewall Manager API actions, data types, and errors. For detailed information about Firewall Manager features,
* see the Firewall Manager Developer
* Guide.
*
*
* Some API actions require explicit resource permissions. For information, see the developer guide topic Service roles for Firewall Manager.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AWSFMSAsync extends AWSFMS {
/**
*
* Sets a Firewall Manager default administrator account. The Firewall Manager default administrator account can
* manage third-party firewalls and has full administrative scope that allows administration of all policy types,
* accounts, organizational units, and Regions. This account must be a member account of the organization in
* Organizations whose resources you want to protect.
*
*
* For information about working with Firewall Manager administrator accounts, see Managing Firewall
* Manager administrators in the Firewall Manager Developer Guide.
*
*
* @param associateAdminAccountRequest
* @return A Java Future containing the result of the AssociateAdminAccount operation returned by the service.
* @sample AWSFMSAsync.AssociateAdminAccount
* @see AWS API
* Documentation
*/
java.util.concurrent.Future associateAdminAccountAsync(AssociateAdminAccountRequest associateAdminAccountRequest);
/**
*
* Sets a Firewall Manager default administrator account. The Firewall Manager default administrator account can
* manage third-party firewalls and has full administrative scope that allows administration of all policy types,
* accounts, organizational units, and Regions. This account must be a member account of the organization in
* Organizations whose resources you want to protect.
*
*
* For information about working with Firewall Manager administrator accounts, see Managing Firewall
* Manager administrators in the Firewall Manager Developer Guide.
*
*
* @param associateAdminAccountRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AssociateAdminAccount operation returned by the service.
* @sample AWSFMSAsyncHandler.AssociateAdminAccount
* @see AWS API
* Documentation
*/
java.util.concurrent.Future associateAdminAccountAsync(AssociateAdminAccountRequest associateAdminAccountRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Sets the Firewall Manager policy administrator as a tenant administrator of a third-party firewall service. A
* tenant is an instance of the third-party firewall service that's associated with your Amazon Web Services
* customer account.
*
*
* @param associateThirdPartyFirewallRequest
* @return A Java Future containing the result of the AssociateThirdPartyFirewall operation returned by the service.
* @sample AWSFMSAsync.AssociateThirdPartyFirewall
* @see AWS API Documentation
*/
java.util.concurrent.Future associateThirdPartyFirewallAsync(
AssociateThirdPartyFirewallRequest associateThirdPartyFirewallRequest);
/**
*
* Sets the Firewall Manager policy administrator as a tenant administrator of a third-party firewall service. A
* tenant is an instance of the third-party firewall service that's associated with your Amazon Web Services
* customer account.
*
*
* @param associateThirdPartyFirewallRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AssociateThirdPartyFirewall operation returned by the service.
* @sample AWSFMSAsyncHandler.AssociateThirdPartyFirewall
* @see AWS API Documentation
*/
java.util.concurrent.Future associateThirdPartyFirewallAsync(
AssociateThirdPartyFirewallRequest associateThirdPartyFirewallRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Associate resources to a Firewall Manager resource set.
*
*
* @param batchAssociateResourceRequest
* @return A Java Future containing the result of the BatchAssociateResource operation returned by the service.
* @sample AWSFMSAsync.BatchAssociateResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future batchAssociateResourceAsync(BatchAssociateResourceRequest batchAssociateResourceRequest);
/**
*
* Associate resources to a Firewall Manager resource set.
*
*
* @param batchAssociateResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the BatchAssociateResource operation returned by the service.
* @sample AWSFMSAsyncHandler.BatchAssociateResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future batchAssociateResourceAsync(BatchAssociateResourceRequest batchAssociateResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Disassociates resources from a Firewall Manager resource set.
*
*
* @param batchDisassociateResourceRequest
* @return A Java Future containing the result of the BatchDisassociateResource operation returned by the service.
* @sample AWSFMSAsync.BatchDisassociateResource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future batchDisassociateResourceAsync(
BatchDisassociateResourceRequest batchDisassociateResourceRequest);
/**
*
* Disassociates resources from a Firewall Manager resource set.
*
*
* @param batchDisassociateResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the BatchDisassociateResource operation returned by the service.
* @sample AWSFMSAsyncHandler.BatchDisassociateResource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future batchDisassociateResourceAsync(
BatchDisassociateResourceRequest batchDisassociateResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Permanently deletes an Firewall Manager applications list.
*
*
* @param deleteAppsListRequest
* @return A Java Future containing the result of the DeleteAppsList operation returned by the service.
* @sample AWSFMSAsync.DeleteAppsList
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteAppsListAsync(DeleteAppsListRequest deleteAppsListRequest);
/**
*
* Permanently deletes an Firewall Manager applications list.
*
*
* @param deleteAppsListRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteAppsList operation returned by the service.
* @sample AWSFMSAsyncHandler.DeleteAppsList
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteAppsListAsync(DeleteAppsListRequest deleteAppsListRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes an Firewall Manager association with the IAM role and the Amazon Simple Notification Service (SNS) topic
* that is used to record Firewall Manager SNS logs.
*
*
* @param deleteNotificationChannelRequest
* @return A Java Future containing the result of the DeleteNotificationChannel operation returned by the service.
* @sample AWSFMSAsync.DeleteNotificationChannel
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteNotificationChannelAsync(
DeleteNotificationChannelRequest deleteNotificationChannelRequest);
/**
*
* Deletes an Firewall Manager association with the IAM role and the Amazon Simple Notification Service (SNS) topic
* that is used to record Firewall Manager SNS logs.
*
*
* @param deleteNotificationChannelRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteNotificationChannel operation returned by the service.
* @sample AWSFMSAsyncHandler.DeleteNotificationChannel
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteNotificationChannelAsync(
DeleteNotificationChannelRequest deleteNotificationChannelRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Permanently deletes an Firewall Manager policy.
*
*
* @param deletePolicyRequest
* @return A Java Future containing the result of the DeletePolicy operation returned by the service.
* @sample AWSFMSAsync.DeletePolicy
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deletePolicyAsync(DeletePolicyRequest deletePolicyRequest);
/**
*
* Permanently deletes an Firewall Manager policy.
*
*
* @param deletePolicyRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeletePolicy operation returned by the service.
* @sample AWSFMSAsyncHandler.DeletePolicy
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deletePolicyAsync(DeletePolicyRequest deletePolicyRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Permanently deletes an Firewall Manager protocols list.
*
*
* @param deleteProtocolsListRequest
* @return A Java Future containing the result of the DeleteProtocolsList operation returned by the service.
* @sample AWSFMSAsync.DeleteProtocolsList
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteProtocolsListAsync(DeleteProtocolsListRequest deleteProtocolsListRequest);
/**
*
* Permanently deletes an Firewall Manager protocols list.
*
*
* @param deleteProtocolsListRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteProtocolsList operation returned by the service.
* @sample AWSFMSAsyncHandler.DeleteProtocolsList
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteProtocolsListAsync(DeleteProtocolsListRequest deleteProtocolsListRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the specified ResourceSet.
*
*
* @param deleteResourceSetRequest
* @return A Java Future containing the result of the DeleteResourceSet operation returned by the service.
* @sample AWSFMSAsync.DeleteResourceSet
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteResourceSetAsync(DeleteResourceSetRequest deleteResourceSetRequest);
/**
*
* Deletes the specified ResourceSet.
*
*
* @param deleteResourceSetRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteResourceSet operation returned by the service.
* @sample AWSFMSAsyncHandler.DeleteResourceSet
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteResourceSetAsync(DeleteResourceSetRequest deleteResourceSetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Disassociates an Firewall Manager administrator account. To set a different account as an Firewall Manager
* administrator, submit a PutAdminAccount request. To set an account as a default administrator account, you
* must submit an AssociateAdminAccount request.
*
*
* Disassociation of the default administrator account follows the first in, last out principle. If you are the
* default administrator, all Firewall Manager administrators within the organization must first disassociate their
* accounts before you can disassociate your account.
*
*
* @param disassociateAdminAccountRequest
* @return A Java Future containing the result of the DisassociateAdminAccount operation returned by the service.
* @sample AWSFMSAsync.DisassociateAdminAccount
* @see AWS
* API Documentation
*/
java.util.concurrent.Future disassociateAdminAccountAsync(DisassociateAdminAccountRequest disassociateAdminAccountRequest);
/**
*
* Disassociates an Firewall Manager administrator account. To set a different account as an Firewall Manager
* administrator, submit a PutAdminAccount request. To set an account as a default administrator account, you
* must submit an AssociateAdminAccount request.
*
*
* Disassociation of the default administrator account follows the first in, last out principle. If you are the
* default administrator, all Firewall Manager administrators within the organization must first disassociate their
* accounts before you can disassociate your account.
*
*
* @param disassociateAdminAccountRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DisassociateAdminAccount operation returned by the service.
* @sample AWSFMSAsyncHandler.DisassociateAdminAccount
* @see AWS
* API Documentation
*/
java.util.concurrent.Future disassociateAdminAccountAsync(DisassociateAdminAccountRequest disassociateAdminAccountRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Disassociates a Firewall Manager policy administrator from a third-party firewall tenant. When you call
* DisassociateThirdPartyFirewall
, the third-party firewall vendor deletes all of the firewalls that
* are associated with the account.
*
*
* @param disassociateThirdPartyFirewallRequest
* @return A Java Future containing the result of the DisassociateThirdPartyFirewall operation returned by the
* service.
* @sample AWSFMSAsync.DisassociateThirdPartyFirewall
* @see AWS API Documentation
*/
java.util.concurrent.Future disassociateThirdPartyFirewallAsync(
DisassociateThirdPartyFirewallRequest disassociateThirdPartyFirewallRequest);
/**
*
* Disassociates a Firewall Manager policy administrator from a third-party firewall tenant. When you call
* DisassociateThirdPartyFirewall
, the third-party firewall vendor deletes all of the firewalls that
* are associated with the account.
*
*
* @param disassociateThirdPartyFirewallRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DisassociateThirdPartyFirewall operation returned by the
* service.
* @sample AWSFMSAsyncHandler.DisassociateThirdPartyFirewall
* @see AWS API Documentation
*/
java.util.concurrent.Future disassociateThirdPartyFirewallAsync(
DisassociateThirdPartyFirewallRequest disassociateThirdPartyFirewallRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns the Organizations account that is associated with Firewall Manager as the Firewall Manager default
* administrator.
*
*
* @param getAdminAccountRequest
* @return A Java Future containing the result of the GetAdminAccount operation returned by the service.
* @sample AWSFMSAsync.GetAdminAccount
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getAdminAccountAsync(GetAdminAccountRequest getAdminAccountRequest);
/**
*
* Returns the Organizations account that is associated with Firewall Manager as the Firewall Manager default
* administrator.
*
*
* @param getAdminAccountRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetAdminAccount operation returned by the service.
* @sample AWSFMSAsyncHandler.GetAdminAccount
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getAdminAccountAsync(GetAdminAccountRequest getAdminAccountRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns information about the specified account's administrative scope. The administrative scope defines the
* resources that an Firewall Manager administrator can manage.
*
*
* @param getAdminScopeRequest
* @return A Java Future containing the result of the GetAdminScope operation returned by the service.
* @sample AWSFMSAsync.GetAdminScope
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getAdminScopeAsync(GetAdminScopeRequest getAdminScopeRequest);
/**
*
* Returns information about the specified account's administrative scope. The administrative scope defines the
* resources that an Firewall Manager administrator can manage.
*
*
* @param getAdminScopeRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetAdminScope operation returned by the service.
* @sample AWSFMSAsyncHandler.GetAdminScope
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getAdminScopeAsync(GetAdminScopeRequest getAdminScopeRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns information about the specified Firewall Manager applications list.
*
*
* @param getAppsListRequest
* @return A Java Future containing the result of the GetAppsList operation returned by the service.
* @sample AWSFMSAsync.GetAppsList
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getAppsListAsync(GetAppsListRequest getAppsListRequest);
/**
*
* Returns information about the specified Firewall Manager applications list.
*
*
* @param getAppsListRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetAppsList operation returned by the service.
* @sample AWSFMSAsyncHandler.GetAppsList
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getAppsListAsync(GetAppsListRequest getAppsListRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns detailed compliance information about the specified member account. Details include resources that are in
* and out of compliance with the specified policy.
*
*
* The reasons for resources being considered compliant depend on the Firewall Manager policy type.
*
*
* @param getComplianceDetailRequest
* @return A Java Future containing the result of the GetComplianceDetail operation returned by the service.
* @sample AWSFMSAsync.GetComplianceDetail
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getComplianceDetailAsync(GetComplianceDetailRequest getComplianceDetailRequest);
/**
*
* Returns detailed compliance information about the specified member account. Details include resources that are in
* and out of compliance with the specified policy.
*
*
* The reasons for resources being considered compliant depend on the Firewall Manager policy type.
*
*
* @param getComplianceDetailRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetComplianceDetail operation returned by the service.
* @sample AWSFMSAsyncHandler.GetComplianceDetail
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getComplianceDetailAsync(GetComplianceDetailRequest getComplianceDetailRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Information about the Amazon Simple Notification Service (SNS) topic that is used to record Firewall Manager SNS
* logs.
*
*
* @param getNotificationChannelRequest
* @return A Java Future containing the result of the GetNotificationChannel operation returned by the service.
* @sample AWSFMSAsync.GetNotificationChannel
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getNotificationChannelAsync(GetNotificationChannelRequest getNotificationChannelRequest);
/**
*
* Information about the Amazon Simple Notification Service (SNS) topic that is used to record Firewall Manager SNS
* logs.
*
*
* @param getNotificationChannelRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetNotificationChannel operation returned by the service.
* @sample AWSFMSAsyncHandler.GetNotificationChannel
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getNotificationChannelAsync(GetNotificationChannelRequest getNotificationChannelRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns information about the specified Firewall Manager policy.
*
*
* @param getPolicyRequest
* @return A Java Future containing the result of the GetPolicy operation returned by the service.
* @sample AWSFMSAsync.GetPolicy
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getPolicyAsync(GetPolicyRequest getPolicyRequest);
/**
*
* Returns information about the specified Firewall Manager policy.
*
*
* @param getPolicyRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetPolicy operation returned by the service.
* @sample AWSFMSAsyncHandler.GetPolicy
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getPolicyAsync(GetPolicyRequest getPolicyRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* If you created a Shield Advanced policy, returns policy-level attack summary information in the event of a
* potential DDoS attack. Other policy types are currently unsupported.
*
*
* @param getProtectionStatusRequest
* @return A Java Future containing the result of the GetProtectionStatus operation returned by the service.
* @sample AWSFMSAsync.GetProtectionStatus
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getProtectionStatusAsync(GetProtectionStatusRequest getProtectionStatusRequest);
/**
*
* If you created a Shield Advanced policy, returns policy-level attack summary information in the event of a
* potential DDoS attack. Other policy types are currently unsupported.
*
*
* @param getProtectionStatusRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetProtectionStatus operation returned by the service.
* @sample AWSFMSAsyncHandler.GetProtectionStatus
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getProtectionStatusAsync(GetProtectionStatusRequest getProtectionStatusRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns information about the specified Firewall Manager protocols list.
*
*
* @param getProtocolsListRequest
* @return A Java Future containing the result of the GetProtocolsList operation returned by the service.
* @sample AWSFMSAsync.GetProtocolsList
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getProtocolsListAsync(GetProtocolsListRequest getProtocolsListRequest);
/**
*
* Returns information about the specified Firewall Manager protocols list.
*
*
* @param getProtocolsListRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetProtocolsList operation returned by the service.
* @sample AWSFMSAsyncHandler.GetProtocolsList
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getProtocolsListAsync(GetProtocolsListRequest getProtocolsListRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets information about a specific resource set.
*
*
* @param getResourceSetRequest
* @return A Java Future containing the result of the GetResourceSet operation returned by the service.
* @sample AWSFMSAsync.GetResourceSet
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getResourceSetAsync(GetResourceSetRequest getResourceSetRequest);
/**
*
* Gets information about a specific resource set.
*
*
* @param getResourceSetRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetResourceSet operation returned by the service.
* @sample AWSFMSAsyncHandler.GetResourceSet
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getResourceSetAsync(GetResourceSetRequest getResourceSetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* The onboarding status of a Firewall Manager admin account to third-party firewall vendor tenant.
*
*
* @param getThirdPartyFirewallAssociationStatusRequest
* @return A Java Future containing the result of the GetThirdPartyFirewallAssociationStatus operation returned by
* the service.
* @sample AWSFMSAsync.GetThirdPartyFirewallAssociationStatus
* @see AWS API Documentation
*/
java.util.concurrent.Future getThirdPartyFirewallAssociationStatusAsync(
GetThirdPartyFirewallAssociationStatusRequest getThirdPartyFirewallAssociationStatusRequest);
/**
*
* The onboarding status of a Firewall Manager admin account to third-party firewall vendor tenant.
*
*
* @param getThirdPartyFirewallAssociationStatusRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetThirdPartyFirewallAssociationStatus operation returned by
* the service.
* @sample AWSFMSAsyncHandler.GetThirdPartyFirewallAssociationStatus
* @see AWS API Documentation
*/
java.util.concurrent.Future getThirdPartyFirewallAssociationStatusAsync(
GetThirdPartyFirewallAssociationStatusRequest getThirdPartyFirewallAssociationStatusRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves violations for a resource based on the specified Firewall Manager policy and Amazon Web Services
* account.
*
*
* @param getViolationDetailsRequest
* @return A Java Future containing the result of the GetViolationDetails operation returned by the service.
* @sample AWSFMSAsync.GetViolationDetails
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getViolationDetailsAsync(GetViolationDetailsRequest getViolationDetailsRequest);
/**
*
* Retrieves violations for a resource based on the specified Firewall Manager policy and Amazon Web Services
* account.
*
*
* @param getViolationDetailsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetViolationDetails operation returned by the service.
* @sample AWSFMSAsyncHandler.GetViolationDetails
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getViolationDetailsAsync(GetViolationDetailsRequest getViolationDetailsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a AdminAccounts
object that lists the Firewall Manager administrators within the
* organization that are onboarded to Firewall Manager by AssociateAdminAccount.
*
*
* This operation can be called only from the organization's management account.
*
*
* @param listAdminAccountsForOrganizationRequest
* @return A Java Future containing the result of the ListAdminAccountsForOrganization operation returned by the
* service.
* @sample AWSFMSAsync.ListAdminAccountsForOrganization
* @see AWS API Documentation
*/
java.util.concurrent.Future listAdminAccountsForOrganizationAsync(
ListAdminAccountsForOrganizationRequest listAdminAccountsForOrganizationRequest);
/**
*
* Returns a AdminAccounts
object that lists the Firewall Manager administrators within the
* organization that are onboarded to Firewall Manager by AssociateAdminAccount.
*
*
* This operation can be called only from the organization's management account.
*
*
* @param listAdminAccountsForOrganizationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListAdminAccountsForOrganization operation returned by the
* service.
* @sample AWSFMSAsyncHandler.ListAdminAccountsForOrganization
* @see AWS API Documentation
*/
java.util.concurrent.Future listAdminAccountsForOrganizationAsync(
ListAdminAccountsForOrganizationRequest listAdminAccountsForOrganizationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the accounts that are managing the specified Organizations member account. This is useful for any member
* account so that they can view the accounts who are managing their account. This operation only returns the
* managing administrators that have the requested account within their AdminScope.
*
*
* @param listAdminsManagingAccountRequest
* @return A Java Future containing the result of the ListAdminsManagingAccount operation returned by the service.
* @sample AWSFMSAsync.ListAdminsManagingAccount
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listAdminsManagingAccountAsync(
ListAdminsManagingAccountRequest listAdminsManagingAccountRequest);
/**
*
* Lists the accounts that are managing the specified Organizations member account. This is useful for any member
* account so that they can view the accounts who are managing their account. This operation only returns the
* managing administrators that have the requested account within their AdminScope.
*
*
* @param listAdminsManagingAccountRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListAdminsManagingAccount operation returned by the service.
* @sample AWSFMSAsyncHandler.ListAdminsManagingAccount
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listAdminsManagingAccountAsync(
ListAdminsManagingAccountRequest listAdminsManagingAccountRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns an array of AppsListDataSummary
objects.
*
*
* @param listAppsListsRequest
* @return A Java Future containing the result of the ListAppsLists operation returned by the service.
* @sample AWSFMSAsync.ListAppsLists
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listAppsListsAsync(ListAppsListsRequest listAppsListsRequest);
/**
*
* Returns an array of AppsListDataSummary
objects.
*
*
* @param listAppsListsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListAppsLists operation returned by the service.
* @sample AWSFMSAsyncHandler.ListAppsLists
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listAppsListsAsync(ListAppsListsRequest listAppsListsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns an array of PolicyComplianceStatus
objects. Use PolicyComplianceStatus
to get a
* summary of which member accounts are protected by the specified policy.
*
*
* @param listComplianceStatusRequest
* @return A Java Future containing the result of the ListComplianceStatus operation returned by the service.
* @sample AWSFMSAsync.ListComplianceStatus
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listComplianceStatusAsync(ListComplianceStatusRequest listComplianceStatusRequest);
/**
*
* Returns an array of PolicyComplianceStatus
objects. Use PolicyComplianceStatus
to get a
* summary of which member accounts are protected by the specified policy.
*
*
* @param listComplianceStatusRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListComplianceStatus operation returned by the service.
* @sample AWSFMSAsyncHandler.ListComplianceStatus
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listComplianceStatusAsync(ListComplianceStatusRequest listComplianceStatusRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns an array of resources in the organization's accounts that are available to be associated with a resource
* set.
*
*
* @param listDiscoveredResourcesRequest
* @return A Java Future containing the result of the ListDiscoveredResources operation returned by the service.
* @sample AWSFMSAsync.ListDiscoveredResources
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listDiscoveredResourcesAsync(ListDiscoveredResourcesRequest listDiscoveredResourcesRequest);
/**
*
* Returns an array of resources in the organization's accounts that are available to be associated with a resource
* set.
*
*
* @param listDiscoveredResourcesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListDiscoveredResources operation returned by the service.
* @sample AWSFMSAsyncHandler.ListDiscoveredResources
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listDiscoveredResourcesAsync(ListDiscoveredResourcesRequest listDiscoveredResourcesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a MemberAccounts
object that lists the member accounts in the administrator's Amazon Web
* Services organization.
*
*
* Either an Firewall Manager administrator or the organization's management account can make this request.
*
*
* @param listMemberAccountsRequest
* @return A Java Future containing the result of the ListMemberAccounts operation returned by the service.
* @sample AWSFMSAsync.ListMemberAccounts
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listMemberAccountsAsync(ListMemberAccountsRequest listMemberAccountsRequest);
/**
*
* Returns a MemberAccounts
object that lists the member accounts in the administrator's Amazon Web
* Services organization.
*
*
* Either an Firewall Manager administrator or the organization's management account can make this request.
*
*
* @param listMemberAccountsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListMemberAccounts operation returned by the service.
* @sample AWSFMSAsyncHandler.ListMemberAccounts
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listMemberAccountsAsync(ListMemberAccountsRequest listMemberAccountsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns an array of PolicySummary
objects.
*
*
* @param listPoliciesRequest
* @return A Java Future containing the result of the ListPolicies operation returned by the service.
* @sample AWSFMSAsync.ListPolicies
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listPoliciesAsync(ListPoliciesRequest listPoliciesRequest);
/**
*
* Returns an array of PolicySummary
objects.
*
*
* @param listPoliciesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListPolicies operation returned by the service.
* @sample AWSFMSAsyncHandler.ListPolicies
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listPoliciesAsync(ListPoliciesRequest listPoliciesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns an array of ProtocolsListDataSummary
objects.
*
*
* @param listProtocolsListsRequest
* @return A Java Future containing the result of the ListProtocolsLists operation returned by the service.
* @sample AWSFMSAsync.ListProtocolsLists
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listProtocolsListsAsync(ListProtocolsListsRequest listProtocolsListsRequest);
/**
*
* Returns an array of ProtocolsListDataSummary
objects.
*
*
* @param listProtocolsListsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListProtocolsLists operation returned by the service.
* @sample AWSFMSAsyncHandler.ListProtocolsLists
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listProtocolsListsAsync(ListProtocolsListsRequest listProtocolsListsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns an array of resources that are currently associated to a resource set.
*
*
* @param listResourceSetResourcesRequest
* @return A Java Future containing the result of the ListResourceSetResources operation returned by the service.
* @sample AWSFMSAsync.ListResourceSetResources
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listResourceSetResourcesAsync(ListResourceSetResourcesRequest listResourceSetResourcesRequest);
/**
*
* Returns an array of resources that are currently associated to a resource set.
*
*
* @param listResourceSetResourcesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListResourceSetResources operation returned by the service.
* @sample AWSFMSAsyncHandler.ListResourceSetResources
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listResourceSetResourcesAsync(ListResourceSetResourcesRequest listResourceSetResourcesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns an array of ResourceSetSummary
objects.
*
*
* @param listResourceSetsRequest
* @return A Java Future containing the result of the ListResourceSets operation returned by the service.
* @sample AWSFMSAsync.ListResourceSets
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listResourceSetsAsync(ListResourceSetsRequest listResourceSetsRequest);
/**
*
* Returns an array of ResourceSetSummary
objects.
*
*
* @param listResourceSetsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListResourceSets operation returned by the service.
* @sample AWSFMSAsyncHandler.ListResourceSets
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listResourceSetsAsync(ListResourceSetsRequest listResourceSetsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves the list of tags for the specified Amazon Web Services resource.
*
*
* @param listTagsForResourceRequest
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AWSFMSAsync.ListTagsForResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest);
/**
*
* Retrieves the list of tags for the specified Amazon Web Services resource.
*
*
* @param listTagsForResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AWSFMSAsyncHandler.ListTagsForResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves a list of all of the third-party firewall policies that are associated with the third-party firewall
* administrator's account.
*
*
* @param listThirdPartyFirewallFirewallPoliciesRequest
* @return A Java Future containing the result of the ListThirdPartyFirewallFirewallPolicies operation returned by
* the service.
* @sample AWSFMSAsync.ListThirdPartyFirewallFirewallPolicies
* @see AWS API Documentation
*/
java.util.concurrent.Future listThirdPartyFirewallFirewallPoliciesAsync(
ListThirdPartyFirewallFirewallPoliciesRequest listThirdPartyFirewallFirewallPoliciesRequest);
/**
*
* Retrieves a list of all of the third-party firewall policies that are associated with the third-party firewall
* administrator's account.
*
*
* @param listThirdPartyFirewallFirewallPoliciesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListThirdPartyFirewallFirewallPolicies operation returned by
* the service.
* @sample AWSFMSAsyncHandler.ListThirdPartyFirewallFirewallPolicies
* @see AWS API Documentation
*/
java.util.concurrent.Future listThirdPartyFirewallFirewallPoliciesAsync(
ListThirdPartyFirewallFirewallPoliciesRequest listThirdPartyFirewallFirewallPoliciesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates or updates an Firewall Manager administrator account. The account must be a member of the organization
* that was onboarded to Firewall Manager by AssociateAdminAccount. Only the organization's management
* account can create an Firewall Manager administrator account. When you create an Firewall Manager administrator
* account, the service checks to see if the account is already a delegated administrator within Organizations. If
* the account isn't a delegated administrator, Firewall Manager calls Organizations to delegate the account within
* Organizations. For more information about administrator accounts within Organizations, see Managing the Amazon
* Web Services Accounts in Your Organization.
*
*
* @param putAdminAccountRequest
* @return A Java Future containing the result of the PutAdminAccount operation returned by the service.
* @sample AWSFMSAsync.PutAdminAccount
* @see AWS API
* Documentation
*/
java.util.concurrent.Future putAdminAccountAsync(PutAdminAccountRequest putAdminAccountRequest);
/**
*
* Creates or updates an Firewall Manager administrator account. The account must be a member of the organization
* that was onboarded to Firewall Manager by AssociateAdminAccount. Only the organization's management
* account can create an Firewall Manager administrator account. When you create an Firewall Manager administrator
* account, the service checks to see if the account is already a delegated administrator within Organizations. If
* the account isn't a delegated administrator, Firewall Manager calls Organizations to delegate the account within
* Organizations. For more information about administrator accounts within Organizations, see Managing the Amazon
* Web Services Accounts in Your Organization.
*
*
* @param putAdminAccountRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the PutAdminAccount operation returned by the service.
* @sample AWSFMSAsyncHandler.PutAdminAccount
* @see AWS API
* Documentation
*/
java.util.concurrent.Future putAdminAccountAsync(PutAdminAccountRequest putAdminAccountRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates an Firewall Manager applications list.
*
*
* @param putAppsListRequest
* @return A Java Future containing the result of the PutAppsList operation returned by the service.
* @sample AWSFMSAsync.PutAppsList
* @see AWS API
* Documentation
*/
java.util.concurrent.Future putAppsListAsync(PutAppsListRequest putAppsListRequest);
/**
*
* Creates an Firewall Manager applications list.
*
*
* @param putAppsListRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the PutAppsList operation returned by the service.
* @sample AWSFMSAsyncHandler.PutAppsList
* @see AWS API
* Documentation
*/
java.util.concurrent.Future putAppsListAsync(PutAppsListRequest putAppsListRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Designates the IAM role and Amazon Simple Notification Service (SNS) topic that Firewall Manager uses to record
* SNS logs.
*
*
* To perform this action outside of the console, you must first configure the SNS topic's access policy to allow
* the SnsRoleName
to publish SNS logs. If the SnsRoleName
provided is a role other than
* the AWSServiceRoleForFMS
service-linked role, this role must have a trust relationship configured to
* allow the Firewall Manager service principal fms.amazonaws.com
to assume this role. For information
* about configuring an SNS access policy, see Service roles for Firewall Manager in the Firewall Manager Developer Guide.
*
*
* @param putNotificationChannelRequest
* @return A Java Future containing the result of the PutNotificationChannel operation returned by the service.
* @sample AWSFMSAsync.PutNotificationChannel
* @see AWS API
* Documentation
*/
java.util.concurrent.Future putNotificationChannelAsync(PutNotificationChannelRequest putNotificationChannelRequest);
/**
*
* Designates the IAM role and Amazon Simple Notification Service (SNS) topic that Firewall Manager uses to record
* SNS logs.
*
*
* To perform this action outside of the console, you must first configure the SNS topic's access policy to allow
* the SnsRoleName
to publish SNS logs. If the SnsRoleName
provided is a role other than
* the AWSServiceRoleForFMS
service-linked role, this role must have a trust relationship configured to
* allow the Firewall Manager service principal fms.amazonaws.com
to assume this role. For information
* about configuring an SNS access policy, see Service roles for Firewall Manager in the Firewall Manager Developer Guide.
*
*
* @param putNotificationChannelRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the PutNotificationChannel operation returned by the service.
* @sample AWSFMSAsyncHandler.PutNotificationChannel
* @see AWS API
* Documentation
*/
java.util.concurrent.Future putNotificationChannelAsync(PutNotificationChannelRequest putNotificationChannelRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates an Firewall Manager policy.
*
*
* A Firewall Manager policy is specific to the individual policy type. If you want to enforce multiple policy types
* across accounts, you can create multiple policies. You can create more than one policy for each type.
*
*
* If you add a new account to an organization that you created with Organizations, Firewall Manager automatically
* applies the policy to the resources in that account that are within scope of the policy.
*
*
* Firewall Manager provides the following types of policies:
*
*
* -
*
* WAF policy - This policy applies WAF web ACL protections to specified accounts and resources.
*
*
* -
*
* Shield Advanced policy - This policy applies Shield Advanced protection to specified accounts and
* resources.
*
*
* -
*
* Security Groups policy - This type of policy gives you control over security groups that are in use
* throughout your organization in Organizations and lets you enforce a baseline set of rules across your
* organization.
*
*
* -
*
* Network ACL policy - This type of policy gives you control over the network ACLs that are in use
* throughout your organization in Organizations and lets you enforce a baseline set of first and last network ACL
* rules across your organization.
*
*
* -
*
* Network Firewall policy - This policy applies Network Firewall protection to your organization's VPCs.
*
*
* -
*
* DNS Firewall policy - This policy applies Amazon Route 53 Resolver DNS Firewall protections to your
* organization's VPCs.
*
*
* -
*
* Third-party firewall policy - This policy applies third-party firewall protections. Third-party firewalls
* are available by subscription through the Amazon Web Services Marketplace console at Amazon Web Services Marketplace.
*
*
* -
*
* Palo Alto Networks Cloud NGFW policy - This policy applies Palo Alto Networks Cloud Next Generation
* Firewall (NGFW) protections and Palo Alto Networks Cloud NGFW rulestacks to your organization's VPCs.
*
*
* -
*
* Fortigate CNF policy - This policy applies Fortigate Cloud Native Firewall (CNF) protections. Fortigate
* CNF is a cloud-centered solution that blocks Zero-Day threats and secures cloud infrastructures with
* industry-leading advanced threat prevention, smart web application firewalls (WAF), and API protection.
*
*
*
*
*
*
* @param putPolicyRequest
* @return A Java Future containing the result of the PutPolicy operation returned by the service.
* @sample AWSFMSAsync.PutPolicy
* @see AWS API
* Documentation
*/
java.util.concurrent.Future putPolicyAsync(PutPolicyRequest putPolicyRequest);
/**
*
* Creates an Firewall Manager policy.
*
*
* A Firewall Manager policy is specific to the individual policy type. If you want to enforce multiple policy types
* across accounts, you can create multiple policies. You can create more than one policy for each type.
*
*
* If you add a new account to an organization that you created with Organizations, Firewall Manager automatically
* applies the policy to the resources in that account that are within scope of the policy.
*
*
* Firewall Manager provides the following types of policies:
*
*
* -
*
* WAF policy - This policy applies WAF web ACL protections to specified accounts and resources.
*
*
* -
*
* Shield Advanced policy - This policy applies Shield Advanced protection to specified accounts and
* resources.
*
*
* -
*
* Security Groups policy - This type of policy gives you control over security groups that are in use
* throughout your organization in Organizations and lets you enforce a baseline set of rules across your
* organization.
*
*
* -
*
* Network ACL policy - This type of policy gives you control over the network ACLs that are in use
* throughout your organization in Organizations and lets you enforce a baseline set of first and last network ACL
* rules across your organization.
*
*
* -
*
* Network Firewall policy - This policy applies Network Firewall protection to your organization's VPCs.
*
*
* -
*
* DNS Firewall policy - This policy applies Amazon Route 53 Resolver DNS Firewall protections to your
* organization's VPCs.
*
*
* -
*
* Third-party firewall policy - This policy applies third-party firewall protections. Third-party firewalls
* are available by subscription through the Amazon Web Services Marketplace console at Amazon Web Services Marketplace.
*
*
* -
*
* Palo Alto Networks Cloud NGFW policy - This policy applies Palo Alto Networks Cloud Next Generation
* Firewall (NGFW) protections and Palo Alto Networks Cloud NGFW rulestacks to your organization's VPCs.
*
*
* -
*
* Fortigate CNF policy - This policy applies Fortigate Cloud Native Firewall (CNF) protections. Fortigate
* CNF is a cloud-centered solution that blocks Zero-Day threats and secures cloud infrastructures with
* industry-leading advanced threat prevention, smart web application firewalls (WAF), and API protection.
*
*
*
*
*
*
* @param putPolicyRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the PutPolicy operation returned by the service.
* @sample AWSFMSAsyncHandler.PutPolicy
* @see AWS API
* Documentation
*/
java.util.concurrent.Future putPolicyAsync(PutPolicyRequest putPolicyRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates an Firewall Manager protocols list.
*
*
* @param putProtocolsListRequest
* @return A Java Future containing the result of the PutProtocolsList operation returned by the service.
* @sample AWSFMSAsync.PutProtocolsList
* @see AWS API
* Documentation
*/
java.util.concurrent.Future putProtocolsListAsync(PutProtocolsListRequest putProtocolsListRequest);
/**
*
* Creates an Firewall Manager protocols list.
*
*
* @param putProtocolsListRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the PutProtocolsList operation returned by the service.
* @sample AWSFMSAsyncHandler.PutProtocolsList
* @see AWS API
* Documentation
*/
java.util.concurrent.Future putProtocolsListAsync(PutProtocolsListRequest putProtocolsListRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates the resource set.
*
*
* An Firewall Manager resource set defines the resources to import into an Firewall Manager policy from another
* Amazon Web Services service.
*
*
* @param putResourceSetRequest
* @return A Java Future containing the result of the PutResourceSet operation returned by the service.
* @sample AWSFMSAsync.PutResourceSet
* @see AWS API
* Documentation
*/
java.util.concurrent.Future putResourceSetAsync(PutResourceSetRequest putResourceSetRequest);
/**
*
* Creates the resource set.
*
*
* An Firewall Manager resource set defines the resources to import into an Firewall Manager policy from another
* Amazon Web Services service.
*
*
* @param putResourceSetRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the PutResourceSet operation returned by the service.
* @sample AWSFMSAsyncHandler.PutResourceSet
* @see AWS API
* Documentation
*/
java.util.concurrent.Future putResourceSetAsync(PutResourceSetRequest putResourceSetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Adds one or more tags to an Amazon Web Services resource.
*
*
* @param tagResourceRequest
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AWSFMSAsync.TagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest);
/**
*
* Adds one or more tags to an Amazon Web Services resource.
*
*
* @param tagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AWSFMSAsyncHandler.TagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Removes one or more tags from an Amazon Web Services resource.
*
*
* @param untagResourceRequest
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AWSFMSAsync.UntagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest);
/**
*
* Removes one or more tags from an Amazon Web Services resource.
*
*
* @param untagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AWSFMSAsyncHandler.UntagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
}