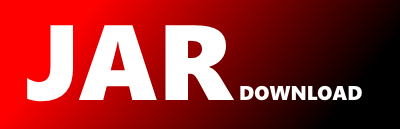
com.amazonaws.services.fms.model.EntryViolation Maven / Gradle / Ivy
Show all versions of aws-java-sdk-fms Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.fms.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Detailed information about an entry violation in a network ACL. The violation is against the network ACL
* specification inside the Firewall Manager network ACL policy. This data object is part of
* InvalidNetworkAclEntriesViolation
.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class EntryViolation implements Serializable, Cloneable, StructuredPojo {
/**
*
* The Firewall Manager-managed network ACL entry that is involved in the entry violation.
*
*/
private EntryDescription expectedEntry;
/**
*
* The evaluation location within the ordered list of entries where the ExpectedEntry
should be,
* according to the network ACL policy specifications.
*
*/
private String expectedEvaluationOrder;
/**
*
* The evaluation location within the ordered list of entries where the ExpectedEntry
is currently
* located.
*
*/
private String actualEvaluationOrder;
/**
*
* The entry that's currently in the ExpectedEvaluationOrder
location, in place of the expected entry.
*
*/
private EntryDescription entryAtExpectedEvaluationOrder;
/**
*
* The list of entries that are in conflict with ExpectedEntry
.
*
*/
private java.util.List entriesWithConflicts;
/**
*
* Descriptions of the violations that Firewall Manager found for these entries.
*
*/
private java.util.List entryViolationReasons;
/**
*
* The Firewall Manager-managed network ACL entry that is involved in the entry violation.
*
*
* @param expectedEntry
* The Firewall Manager-managed network ACL entry that is involved in the entry violation.
*/
public void setExpectedEntry(EntryDescription expectedEntry) {
this.expectedEntry = expectedEntry;
}
/**
*
* The Firewall Manager-managed network ACL entry that is involved in the entry violation.
*
*
* @return The Firewall Manager-managed network ACL entry that is involved in the entry violation.
*/
public EntryDescription getExpectedEntry() {
return this.expectedEntry;
}
/**
*
* The Firewall Manager-managed network ACL entry that is involved in the entry violation.
*
*
* @param expectedEntry
* The Firewall Manager-managed network ACL entry that is involved in the entry violation.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public EntryViolation withExpectedEntry(EntryDescription expectedEntry) {
setExpectedEntry(expectedEntry);
return this;
}
/**
*
* The evaluation location within the ordered list of entries where the ExpectedEntry
should be,
* according to the network ACL policy specifications.
*
*
* @param expectedEvaluationOrder
* The evaluation location within the ordered list of entries where the ExpectedEntry
should be,
* according to the network ACL policy specifications.
*/
public void setExpectedEvaluationOrder(String expectedEvaluationOrder) {
this.expectedEvaluationOrder = expectedEvaluationOrder;
}
/**
*
* The evaluation location within the ordered list of entries where the ExpectedEntry
should be,
* according to the network ACL policy specifications.
*
*
* @return The evaluation location within the ordered list of entries where the ExpectedEntry
should
* be, according to the network ACL policy specifications.
*/
public String getExpectedEvaluationOrder() {
return this.expectedEvaluationOrder;
}
/**
*
* The evaluation location within the ordered list of entries where the ExpectedEntry
should be,
* according to the network ACL policy specifications.
*
*
* @param expectedEvaluationOrder
* The evaluation location within the ordered list of entries where the ExpectedEntry
should be,
* according to the network ACL policy specifications.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public EntryViolation withExpectedEvaluationOrder(String expectedEvaluationOrder) {
setExpectedEvaluationOrder(expectedEvaluationOrder);
return this;
}
/**
*
* The evaluation location within the ordered list of entries where the ExpectedEntry
is currently
* located.
*
*
* @param actualEvaluationOrder
* The evaluation location within the ordered list of entries where the ExpectedEntry
is
* currently located.
*/
public void setActualEvaluationOrder(String actualEvaluationOrder) {
this.actualEvaluationOrder = actualEvaluationOrder;
}
/**
*
* The evaluation location within the ordered list of entries where the ExpectedEntry
is currently
* located.
*
*
* @return The evaluation location within the ordered list of entries where the ExpectedEntry
is
* currently located.
*/
public String getActualEvaluationOrder() {
return this.actualEvaluationOrder;
}
/**
*
* The evaluation location within the ordered list of entries where the ExpectedEntry
is currently
* located.
*
*
* @param actualEvaluationOrder
* The evaluation location within the ordered list of entries where the ExpectedEntry
is
* currently located.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public EntryViolation withActualEvaluationOrder(String actualEvaluationOrder) {
setActualEvaluationOrder(actualEvaluationOrder);
return this;
}
/**
*
* The entry that's currently in the ExpectedEvaluationOrder
location, in place of the expected entry.
*
*
* @param entryAtExpectedEvaluationOrder
* The entry that's currently in the ExpectedEvaluationOrder
location, in place of the expected
* entry.
*/
public void setEntryAtExpectedEvaluationOrder(EntryDescription entryAtExpectedEvaluationOrder) {
this.entryAtExpectedEvaluationOrder = entryAtExpectedEvaluationOrder;
}
/**
*
* The entry that's currently in the ExpectedEvaluationOrder
location, in place of the expected entry.
*
*
* @return The entry that's currently in the ExpectedEvaluationOrder
location, in place of the expected
* entry.
*/
public EntryDescription getEntryAtExpectedEvaluationOrder() {
return this.entryAtExpectedEvaluationOrder;
}
/**
*
* The entry that's currently in the ExpectedEvaluationOrder
location, in place of the expected entry.
*
*
* @param entryAtExpectedEvaluationOrder
* The entry that's currently in the ExpectedEvaluationOrder
location, in place of the expected
* entry.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public EntryViolation withEntryAtExpectedEvaluationOrder(EntryDescription entryAtExpectedEvaluationOrder) {
setEntryAtExpectedEvaluationOrder(entryAtExpectedEvaluationOrder);
return this;
}
/**
*
* The list of entries that are in conflict with ExpectedEntry
.
*
*
* @return The list of entries that are in conflict with ExpectedEntry
.
*/
public java.util.List getEntriesWithConflicts() {
return entriesWithConflicts;
}
/**
*
* The list of entries that are in conflict with ExpectedEntry
.
*
*
* @param entriesWithConflicts
* The list of entries that are in conflict with ExpectedEntry
.
*/
public void setEntriesWithConflicts(java.util.Collection entriesWithConflicts) {
if (entriesWithConflicts == null) {
this.entriesWithConflicts = null;
return;
}
this.entriesWithConflicts = new java.util.ArrayList(entriesWithConflicts);
}
/**
*
* The list of entries that are in conflict with ExpectedEntry
.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setEntriesWithConflicts(java.util.Collection)} or {@link #withEntriesWithConflicts(java.util.Collection)}
* if you want to override the existing values.
*
*
* @param entriesWithConflicts
* The list of entries that are in conflict with ExpectedEntry
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public EntryViolation withEntriesWithConflicts(EntryDescription... entriesWithConflicts) {
if (this.entriesWithConflicts == null) {
setEntriesWithConflicts(new java.util.ArrayList(entriesWithConflicts.length));
}
for (EntryDescription ele : entriesWithConflicts) {
this.entriesWithConflicts.add(ele);
}
return this;
}
/**
*
* The list of entries that are in conflict with ExpectedEntry
.
*
*
* @param entriesWithConflicts
* The list of entries that are in conflict with ExpectedEntry
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public EntryViolation withEntriesWithConflicts(java.util.Collection entriesWithConflicts) {
setEntriesWithConflicts(entriesWithConflicts);
return this;
}
/**
*
* Descriptions of the violations that Firewall Manager found for these entries.
*
*
* @return Descriptions of the violations that Firewall Manager found for these entries.
* @see EntryViolationReason
*/
public java.util.List getEntryViolationReasons() {
return entryViolationReasons;
}
/**
*
* Descriptions of the violations that Firewall Manager found for these entries.
*
*
* @param entryViolationReasons
* Descriptions of the violations that Firewall Manager found for these entries.
* @see EntryViolationReason
*/
public void setEntryViolationReasons(java.util.Collection entryViolationReasons) {
if (entryViolationReasons == null) {
this.entryViolationReasons = null;
return;
}
this.entryViolationReasons = new java.util.ArrayList(entryViolationReasons);
}
/**
*
* Descriptions of the violations that Firewall Manager found for these entries.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setEntryViolationReasons(java.util.Collection)} or
* {@link #withEntryViolationReasons(java.util.Collection)} if you want to override the existing values.
*
*
* @param entryViolationReasons
* Descriptions of the violations that Firewall Manager found for these entries.
* @return Returns a reference to this object so that method calls can be chained together.
* @see EntryViolationReason
*/
public EntryViolation withEntryViolationReasons(String... entryViolationReasons) {
if (this.entryViolationReasons == null) {
setEntryViolationReasons(new java.util.ArrayList(entryViolationReasons.length));
}
for (String ele : entryViolationReasons) {
this.entryViolationReasons.add(ele);
}
return this;
}
/**
*
* Descriptions of the violations that Firewall Manager found for these entries.
*
*
* @param entryViolationReasons
* Descriptions of the violations that Firewall Manager found for these entries.
* @return Returns a reference to this object so that method calls can be chained together.
* @see EntryViolationReason
*/
public EntryViolation withEntryViolationReasons(java.util.Collection entryViolationReasons) {
setEntryViolationReasons(entryViolationReasons);
return this;
}
/**
*
* Descriptions of the violations that Firewall Manager found for these entries.
*
*
* @param entryViolationReasons
* Descriptions of the violations that Firewall Manager found for these entries.
* @return Returns a reference to this object so that method calls can be chained together.
* @see EntryViolationReason
*/
public EntryViolation withEntryViolationReasons(EntryViolationReason... entryViolationReasons) {
java.util.ArrayList entryViolationReasonsCopy = new java.util.ArrayList(entryViolationReasons.length);
for (EntryViolationReason value : entryViolationReasons) {
entryViolationReasonsCopy.add(value.toString());
}
if (getEntryViolationReasons() == null) {
setEntryViolationReasons(entryViolationReasonsCopy);
} else {
getEntryViolationReasons().addAll(entryViolationReasonsCopy);
}
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getExpectedEntry() != null)
sb.append("ExpectedEntry: ").append(getExpectedEntry()).append(",");
if (getExpectedEvaluationOrder() != null)
sb.append("ExpectedEvaluationOrder: ").append(getExpectedEvaluationOrder()).append(",");
if (getActualEvaluationOrder() != null)
sb.append("ActualEvaluationOrder: ").append(getActualEvaluationOrder()).append(",");
if (getEntryAtExpectedEvaluationOrder() != null)
sb.append("EntryAtExpectedEvaluationOrder: ").append(getEntryAtExpectedEvaluationOrder()).append(",");
if (getEntriesWithConflicts() != null)
sb.append("EntriesWithConflicts: ").append(getEntriesWithConflicts()).append(",");
if (getEntryViolationReasons() != null)
sb.append("EntryViolationReasons: ").append(getEntryViolationReasons());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof EntryViolation == false)
return false;
EntryViolation other = (EntryViolation) obj;
if (other.getExpectedEntry() == null ^ this.getExpectedEntry() == null)
return false;
if (other.getExpectedEntry() != null && other.getExpectedEntry().equals(this.getExpectedEntry()) == false)
return false;
if (other.getExpectedEvaluationOrder() == null ^ this.getExpectedEvaluationOrder() == null)
return false;
if (other.getExpectedEvaluationOrder() != null && other.getExpectedEvaluationOrder().equals(this.getExpectedEvaluationOrder()) == false)
return false;
if (other.getActualEvaluationOrder() == null ^ this.getActualEvaluationOrder() == null)
return false;
if (other.getActualEvaluationOrder() != null && other.getActualEvaluationOrder().equals(this.getActualEvaluationOrder()) == false)
return false;
if (other.getEntryAtExpectedEvaluationOrder() == null ^ this.getEntryAtExpectedEvaluationOrder() == null)
return false;
if (other.getEntryAtExpectedEvaluationOrder() != null
&& other.getEntryAtExpectedEvaluationOrder().equals(this.getEntryAtExpectedEvaluationOrder()) == false)
return false;
if (other.getEntriesWithConflicts() == null ^ this.getEntriesWithConflicts() == null)
return false;
if (other.getEntriesWithConflicts() != null && other.getEntriesWithConflicts().equals(this.getEntriesWithConflicts()) == false)
return false;
if (other.getEntryViolationReasons() == null ^ this.getEntryViolationReasons() == null)
return false;
if (other.getEntryViolationReasons() != null && other.getEntryViolationReasons().equals(this.getEntryViolationReasons()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getExpectedEntry() == null) ? 0 : getExpectedEntry().hashCode());
hashCode = prime * hashCode + ((getExpectedEvaluationOrder() == null) ? 0 : getExpectedEvaluationOrder().hashCode());
hashCode = prime * hashCode + ((getActualEvaluationOrder() == null) ? 0 : getActualEvaluationOrder().hashCode());
hashCode = prime * hashCode + ((getEntryAtExpectedEvaluationOrder() == null) ? 0 : getEntryAtExpectedEvaluationOrder().hashCode());
hashCode = prime * hashCode + ((getEntriesWithConflicts() == null) ? 0 : getEntriesWithConflicts().hashCode());
hashCode = prime * hashCode + ((getEntryViolationReasons() == null) ? 0 : getEntryViolationReasons().hashCode());
return hashCode;
}
@Override
public EntryViolation clone() {
try {
return (EntryViolation) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.fms.model.transform.EntryViolationMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}