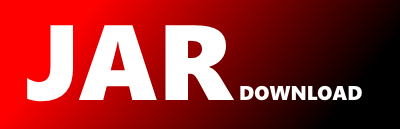
com.amazonaws.services.fms.model.GetViolationDetailsRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-fms Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.fms.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class GetViolationDetailsRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The ID of the Firewall Manager policy that you want the details for. You can get violation details for the
* following policy types:
*
*
* -
*
* DNS Firewall
*
*
* -
*
* Imported Network Firewall
*
*
* -
*
* Network Firewall
*
*
* -
*
* Security group content audit
*
*
* -
*
* Network ACL
*
*
* -
*
* Third-party firewall
*
*
*
*/
private String policyId;
/**
*
* The Amazon Web Services account ID that you want the details for.
*
*/
private String memberAccount;
/**
*
* The ID of the resource that has violations.
*
*/
private String resourceId;
/**
*
* The resource type. This is in the format shown in the Amazon
* Web Services Resource Types Reference. Supported resource types are: AWS::EC2::Instance
,
* AWS::EC2::NetworkInterface
, AWS::EC2::SecurityGroup
,
* AWS::NetworkFirewall::FirewallPolicy
, and AWS::EC2::Subnet
.
*
*/
private String resourceType;
/**
*
* The ID of the Firewall Manager policy that you want the details for. You can get violation details for the
* following policy types:
*
*
* -
*
* DNS Firewall
*
*
* -
*
* Imported Network Firewall
*
*
* -
*
* Network Firewall
*
*
* -
*
* Security group content audit
*
*
* -
*
* Network ACL
*
*
* -
*
* Third-party firewall
*
*
*
*
* @param policyId
* The ID of the Firewall Manager policy that you want the details for. You can get violation details for the
* following policy types:
*
* -
*
* DNS Firewall
*
*
* -
*
* Imported Network Firewall
*
*
* -
*
* Network Firewall
*
*
* -
*
* Security group content audit
*
*
* -
*
* Network ACL
*
*
* -
*
* Third-party firewall
*
*
*/
public void setPolicyId(String policyId) {
this.policyId = policyId;
}
/**
*
* The ID of the Firewall Manager policy that you want the details for. You can get violation details for the
* following policy types:
*
*
* -
*
* DNS Firewall
*
*
* -
*
* Imported Network Firewall
*
*
* -
*
* Network Firewall
*
*
* -
*
* Security group content audit
*
*
* -
*
* Network ACL
*
*
* -
*
* Third-party firewall
*
*
*
*
* @return The ID of the Firewall Manager policy that you want the details for. You can get violation details for
* the following policy types:
*
* -
*
* DNS Firewall
*
*
* -
*
* Imported Network Firewall
*
*
* -
*
* Network Firewall
*
*
* -
*
* Security group content audit
*
*
* -
*
* Network ACL
*
*
* -
*
* Third-party firewall
*
*
*/
public String getPolicyId() {
return this.policyId;
}
/**
*
* The ID of the Firewall Manager policy that you want the details for. You can get violation details for the
* following policy types:
*
*
* -
*
* DNS Firewall
*
*
* -
*
* Imported Network Firewall
*
*
* -
*
* Network Firewall
*
*
* -
*
* Security group content audit
*
*
* -
*
* Network ACL
*
*
* -
*
* Third-party firewall
*
*
*
*
* @param policyId
* The ID of the Firewall Manager policy that you want the details for. You can get violation details for the
* following policy types:
*
* -
*
* DNS Firewall
*
*
* -
*
* Imported Network Firewall
*
*
* -
*
* Network Firewall
*
*
* -
*
* Security group content audit
*
*
* -
*
* Network ACL
*
*
* -
*
* Third-party firewall
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetViolationDetailsRequest withPolicyId(String policyId) {
setPolicyId(policyId);
return this;
}
/**
*
* The Amazon Web Services account ID that you want the details for.
*
*
* @param memberAccount
* The Amazon Web Services account ID that you want the details for.
*/
public void setMemberAccount(String memberAccount) {
this.memberAccount = memberAccount;
}
/**
*
* The Amazon Web Services account ID that you want the details for.
*
*
* @return The Amazon Web Services account ID that you want the details for.
*/
public String getMemberAccount() {
return this.memberAccount;
}
/**
*
* The Amazon Web Services account ID that you want the details for.
*
*
* @param memberAccount
* The Amazon Web Services account ID that you want the details for.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetViolationDetailsRequest withMemberAccount(String memberAccount) {
setMemberAccount(memberAccount);
return this;
}
/**
*
* The ID of the resource that has violations.
*
*
* @param resourceId
* The ID of the resource that has violations.
*/
public void setResourceId(String resourceId) {
this.resourceId = resourceId;
}
/**
*
* The ID of the resource that has violations.
*
*
* @return The ID of the resource that has violations.
*/
public String getResourceId() {
return this.resourceId;
}
/**
*
* The ID of the resource that has violations.
*
*
* @param resourceId
* The ID of the resource that has violations.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetViolationDetailsRequest withResourceId(String resourceId) {
setResourceId(resourceId);
return this;
}
/**
*
* The resource type. This is in the format shown in the Amazon
* Web Services Resource Types Reference. Supported resource types are: AWS::EC2::Instance
,
* AWS::EC2::NetworkInterface
, AWS::EC2::SecurityGroup
,
* AWS::NetworkFirewall::FirewallPolicy
, and AWS::EC2::Subnet
.
*
*
* @param resourceType
* The resource type. This is in the format shown in the Amazon Web Services Resource Types Reference. Supported resource types are:
* AWS::EC2::Instance
, AWS::EC2::NetworkInterface
,
* AWS::EC2::SecurityGroup
, AWS::NetworkFirewall::FirewallPolicy
, and
* AWS::EC2::Subnet
.
*/
public void setResourceType(String resourceType) {
this.resourceType = resourceType;
}
/**
*
* The resource type. This is in the format shown in the Amazon
* Web Services Resource Types Reference. Supported resource types are: AWS::EC2::Instance
,
* AWS::EC2::NetworkInterface
, AWS::EC2::SecurityGroup
,
* AWS::NetworkFirewall::FirewallPolicy
, and AWS::EC2::Subnet
.
*
*
* @return The resource type. This is in the format shown in the Amazon Web Services Resource Types Reference. Supported resource types are:
* AWS::EC2::Instance
, AWS::EC2::NetworkInterface
,
* AWS::EC2::SecurityGroup
, AWS::NetworkFirewall::FirewallPolicy
, and
* AWS::EC2::Subnet
.
*/
public String getResourceType() {
return this.resourceType;
}
/**
*
* The resource type. This is in the format shown in the Amazon
* Web Services Resource Types Reference. Supported resource types are: AWS::EC2::Instance
,
* AWS::EC2::NetworkInterface
, AWS::EC2::SecurityGroup
,
* AWS::NetworkFirewall::FirewallPolicy
, and AWS::EC2::Subnet
.
*
*
* @param resourceType
* The resource type. This is in the format shown in the Amazon Web Services Resource Types Reference. Supported resource types are:
* AWS::EC2::Instance
, AWS::EC2::NetworkInterface
,
* AWS::EC2::SecurityGroup
, AWS::NetworkFirewall::FirewallPolicy
, and
* AWS::EC2::Subnet
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetViolationDetailsRequest withResourceType(String resourceType) {
setResourceType(resourceType);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getPolicyId() != null)
sb.append("PolicyId: ").append(getPolicyId()).append(",");
if (getMemberAccount() != null)
sb.append("MemberAccount: ").append(getMemberAccount()).append(",");
if (getResourceId() != null)
sb.append("ResourceId: ").append(getResourceId()).append(",");
if (getResourceType() != null)
sb.append("ResourceType: ").append(getResourceType());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof GetViolationDetailsRequest == false)
return false;
GetViolationDetailsRequest other = (GetViolationDetailsRequest) obj;
if (other.getPolicyId() == null ^ this.getPolicyId() == null)
return false;
if (other.getPolicyId() != null && other.getPolicyId().equals(this.getPolicyId()) == false)
return false;
if (other.getMemberAccount() == null ^ this.getMemberAccount() == null)
return false;
if (other.getMemberAccount() != null && other.getMemberAccount().equals(this.getMemberAccount()) == false)
return false;
if (other.getResourceId() == null ^ this.getResourceId() == null)
return false;
if (other.getResourceId() != null && other.getResourceId().equals(this.getResourceId()) == false)
return false;
if (other.getResourceType() == null ^ this.getResourceType() == null)
return false;
if (other.getResourceType() != null && other.getResourceType().equals(this.getResourceType()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getPolicyId() == null) ? 0 : getPolicyId().hashCode());
hashCode = prime * hashCode + ((getMemberAccount() == null) ? 0 : getMemberAccount().hashCode());
hashCode = prime * hashCode + ((getResourceId() == null) ? 0 : getResourceId().hashCode());
hashCode = prime * hashCode + ((getResourceType() == null) ? 0 : getResourceType().hashCode());
return hashCode;
}
@Override
public GetViolationDetailsRequest clone() {
return (GetViolationDetailsRequest) super.clone();
}
}