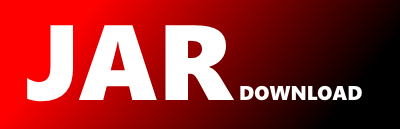
com.amazonaws.services.fms.model.NetworkFirewallPolicyDescription Maven / Gradle / Ivy
Show all versions of aws-java-sdk-fms Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.fms.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* The definition of the Network Firewall firewall policy.
*
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class NetworkFirewallPolicyDescription implements Serializable, Cloneable, StructuredPojo {
/**
*
* The stateless rule groups that are used in the Network Firewall firewall policy.
*
*/
private java.util.List statelessRuleGroups;
/**
*
* The actions to take on packets that don't match any of the stateless rule groups.
*
*/
private java.util.List statelessDefaultActions;
/**
*
* The actions to take on packet fragments that don't match any of the stateless rule groups.
*
*/
private java.util.List statelessFragmentDefaultActions;
/**
*
* Names of custom actions that are available for use in the stateless default actions settings.
*
*/
private java.util.List statelessCustomActions;
/**
*
* The stateful rule groups that are used in the Network Firewall firewall policy.
*
*/
private java.util.List statefulRuleGroups;
/**
*
* The default actions to take on a packet that doesn't match any stateful rules. The stateful default action is
* optional, and is only valid when using the strict rule order.
*
*
* Valid values of the stateful default action:
*
*
* -
*
* aws:drop_strict
*
*
* -
*
* aws:drop_established
*
*
* -
*
* aws:alert_strict
*
*
* -
*
* aws:alert_established
*
*
*
*/
private java.util.List statefulDefaultActions;
/**
*
* Additional options governing how Network Firewall handles stateful rules. The stateful rule groups that you use
* in your policy must have stateful rule options settings that are compatible with these settings.
*
*/
private StatefulEngineOptions statefulEngineOptions;
/**
*
* The stateless rule groups that are used in the Network Firewall firewall policy.
*
*
* @return The stateless rule groups that are used in the Network Firewall firewall policy.
*/
public java.util.List getStatelessRuleGroups() {
return statelessRuleGroups;
}
/**
*
* The stateless rule groups that are used in the Network Firewall firewall policy.
*
*
* @param statelessRuleGroups
* The stateless rule groups that are used in the Network Firewall firewall policy.
*/
public void setStatelessRuleGroups(java.util.Collection statelessRuleGroups) {
if (statelessRuleGroups == null) {
this.statelessRuleGroups = null;
return;
}
this.statelessRuleGroups = new java.util.ArrayList(statelessRuleGroups);
}
/**
*
* The stateless rule groups that are used in the Network Firewall firewall policy.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setStatelessRuleGroups(java.util.Collection)} or {@link #withStatelessRuleGroups(java.util.Collection)}
* if you want to override the existing values.
*
*
* @param statelessRuleGroups
* The stateless rule groups that are used in the Network Firewall firewall policy.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public NetworkFirewallPolicyDescription withStatelessRuleGroups(StatelessRuleGroup... statelessRuleGroups) {
if (this.statelessRuleGroups == null) {
setStatelessRuleGroups(new java.util.ArrayList(statelessRuleGroups.length));
}
for (StatelessRuleGroup ele : statelessRuleGroups) {
this.statelessRuleGroups.add(ele);
}
return this;
}
/**
*
* The stateless rule groups that are used in the Network Firewall firewall policy.
*
*
* @param statelessRuleGroups
* The stateless rule groups that are used in the Network Firewall firewall policy.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public NetworkFirewallPolicyDescription withStatelessRuleGroups(java.util.Collection statelessRuleGroups) {
setStatelessRuleGroups(statelessRuleGroups);
return this;
}
/**
*
* The actions to take on packets that don't match any of the stateless rule groups.
*
*
* @return The actions to take on packets that don't match any of the stateless rule groups.
*/
public java.util.List getStatelessDefaultActions() {
return statelessDefaultActions;
}
/**
*
* The actions to take on packets that don't match any of the stateless rule groups.
*
*
* @param statelessDefaultActions
* The actions to take on packets that don't match any of the stateless rule groups.
*/
public void setStatelessDefaultActions(java.util.Collection statelessDefaultActions) {
if (statelessDefaultActions == null) {
this.statelessDefaultActions = null;
return;
}
this.statelessDefaultActions = new java.util.ArrayList(statelessDefaultActions);
}
/**
*
* The actions to take on packets that don't match any of the stateless rule groups.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setStatelessDefaultActions(java.util.Collection)} or
* {@link #withStatelessDefaultActions(java.util.Collection)} if you want to override the existing values.
*
*
* @param statelessDefaultActions
* The actions to take on packets that don't match any of the stateless rule groups.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public NetworkFirewallPolicyDescription withStatelessDefaultActions(String... statelessDefaultActions) {
if (this.statelessDefaultActions == null) {
setStatelessDefaultActions(new java.util.ArrayList(statelessDefaultActions.length));
}
for (String ele : statelessDefaultActions) {
this.statelessDefaultActions.add(ele);
}
return this;
}
/**
*
* The actions to take on packets that don't match any of the stateless rule groups.
*
*
* @param statelessDefaultActions
* The actions to take on packets that don't match any of the stateless rule groups.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public NetworkFirewallPolicyDescription withStatelessDefaultActions(java.util.Collection statelessDefaultActions) {
setStatelessDefaultActions(statelessDefaultActions);
return this;
}
/**
*
* The actions to take on packet fragments that don't match any of the stateless rule groups.
*
*
* @return The actions to take on packet fragments that don't match any of the stateless rule groups.
*/
public java.util.List getStatelessFragmentDefaultActions() {
return statelessFragmentDefaultActions;
}
/**
*
* The actions to take on packet fragments that don't match any of the stateless rule groups.
*
*
* @param statelessFragmentDefaultActions
* The actions to take on packet fragments that don't match any of the stateless rule groups.
*/
public void setStatelessFragmentDefaultActions(java.util.Collection statelessFragmentDefaultActions) {
if (statelessFragmentDefaultActions == null) {
this.statelessFragmentDefaultActions = null;
return;
}
this.statelessFragmentDefaultActions = new java.util.ArrayList(statelessFragmentDefaultActions);
}
/**
*
* The actions to take on packet fragments that don't match any of the stateless rule groups.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setStatelessFragmentDefaultActions(java.util.Collection)} or
* {@link #withStatelessFragmentDefaultActions(java.util.Collection)} if you want to override the existing values.
*
*
* @param statelessFragmentDefaultActions
* The actions to take on packet fragments that don't match any of the stateless rule groups.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public NetworkFirewallPolicyDescription withStatelessFragmentDefaultActions(String... statelessFragmentDefaultActions) {
if (this.statelessFragmentDefaultActions == null) {
setStatelessFragmentDefaultActions(new java.util.ArrayList(statelessFragmentDefaultActions.length));
}
for (String ele : statelessFragmentDefaultActions) {
this.statelessFragmentDefaultActions.add(ele);
}
return this;
}
/**
*
* The actions to take on packet fragments that don't match any of the stateless rule groups.
*
*
* @param statelessFragmentDefaultActions
* The actions to take on packet fragments that don't match any of the stateless rule groups.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public NetworkFirewallPolicyDescription withStatelessFragmentDefaultActions(java.util.Collection statelessFragmentDefaultActions) {
setStatelessFragmentDefaultActions(statelessFragmentDefaultActions);
return this;
}
/**
*
* Names of custom actions that are available for use in the stateless default actions settings.
*
*
* @return Names of custom actions that are available for use in the stateless default actions settings.
*/
public java.util.List getStatelessCustomActions() {
return statelessCustomActions;
}
/**
*
* Names of custom actions that are available for use in the stateless default actions settings.
*
*
* @param statelessCustomActions
* Names of custom actions that are available for use in the stateless default actions settings.
*/
public void setStatelessCustomActions(java.util.Collection statelessCustomActions) {
if (statelessCustomActions == null) {
this.statelessCustomActions = null;
return;
}
this.statelessCustomActions = new java.util.ArrayList(statelessCustomActions);
}
/**
*
* Names of custom actions that are available for use in the stateless default actions settings.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setStatelessCustomActions(java.util.Collection)} or
* {@link #withStatelessCustomActions(java.util.Collection)} if you want to override the existing values.
*
*
* @param statelessCustomActions
* Names of custom actions that are available for use in the stateless default actions settings.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public NetworkFirewallPolicyDescription withStatelessCustomActions(String... statelessCustomActions) {
if (this.statelessCustomActions == null) {
setStatelessCustomActions(new java.util.ArrayList(statelessCustomActions.length));
}
for (String ele : statelessCustomActions) {
this.statelessCustomActions.add(ele);
}
return this;
}
/**
*
* Names of custom actions that are available for use in the stateless default actions settings.
*
*
* @param statelessCustomActions
* Names of custom actions that are available for use in the stateless default actions settings.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public NetworkFirewallPolicyDescription withStatelessCustomActions(java.util.Collection statelessCustomActions) {
setStatelessCustomActions(statelessCustomActions);
return this;
}
/**
*
* The stateful rule groups that are used in the Network Firewall firewall policy.
*
*
* @return The stateful rule groups that are used in the Network Firewall firewall policy.
*/
public java.util.List getStatefulRuleGroups() {
return statefulRuleGroups;
}
/**
*
* The stateful rule groups that are used in the Network Firewall firewall policy.
*
*
* @param statefulRuleGroups
* The stateful rule groups that are used in the Network Firewall firewall policy.
*/
public void setStatefulRuleGroups(java.util.Collection statefulRuleGroups) {
if (statefulRuleGroups == null) {
this.statefulRuleGroups = null;
return;
}
this.statefulRuleGroups = new java.util.ArrayList(statefulRuleGroups);
}
/**
*
* The stateful rule groups that are used in the Network Firewall firewall policy.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setStatefulRuleGroups(java.util.Collection)} or {@link #withStatefulRuleGroups(java.util.Collection)} if
* you want to override the existing values.
*
*
* @param statefulRuleGroups
* The stateful rule groups that are used in the Network Firewall firewall policy.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public NetworkFirewallPolicyDescription withStatefulRuleGroups(StatefulRuleGroup... statefulRuleGroups) {
if (this.statefulRuleGroups == null) {
setStatefulRuleGroups(new java.util.ArrayList(statefulRuleGroups.length));
}
for (StatefulRuleGroup ele : statefulRuleGroups) {
this.statefulRuleGroups.add(ele);
}
return this;
}
/**
*
* The stateful rule groups that are used in the Network Firewall firewall policy.
*
*
* @param statefulRuleGroups
* The stateful rule groups that are used in the Network Firewall firewall policy.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public NetworkFirewallPolicyDescription withStatefulRuleGroups(java.util.Collection statefulRuleGroups) {
setStatefulRuleGroups(statefulRuleGroups);
return this;
}
/**
*
* The default actions to take on a packet that doesn't match any stateful rules. The stateful default action is
* optional, and is only valid when using the strict rule order.
*
*
* Valid values of the stateful default action:
*
*
* -
*
* aws:drop_strict
*
*
* -
*
* aws:drop_established
*
*
* -
*
* aws:alert_strict
*
*
* -
*
* aws:alert_established
*
*
*
*
* @return The default actions to take on a packet that doesn't match any stateful rules. The stateful default
* action is optional, and is only valid when using the strict rule order.
*
* Valid values of the stateful default action:
*
*
* -
*
* aws:drop_strict
*
*
* -
*
* aws:drop_established
*
*
* -
*
* aws:alert_strict
*
*
* -
*
* aws:alert_established
*
*
*/
public java.util.List getStatefulDefaultActions() {
return statefulDefaultActions;
}
/**
*
* The default actions to take on a packet that doesn't match any stateful rules. The stateful default action is
* optional, and is only valid when using the strict rule order.
*
*
* Valid values of the stateful default action:
*
*
* -
*
* aws:drop_strict
*
*
* -
*
* aws:drop_established
*
*
* -
*
* aws:alert_strict
*
*
* -
*
* aws:alert_established
*
*
*
*
* @param statefulDefaultActions
* The default actions to take on a packet that doesn't match any stateful rules. The stateful default action
* is optional, and is only valid when using the strict rule order.
*
* Valid values of the stateful default action:
*
*
* -
*
* aws:drop_strict
*
*
* -
*
* aws:drop_established
*
*
* -
*
* aws:alert_strict
*
*
* -
*
* aws:alert_established
*
*
*/
public void setStatefulDefaultActions(java.util.Collection statefulDefaultActions) {
if (statefulDefaultActions == null) {
this.statefulDefaultActions = null;
return;
}
this.statefulDefaultActions = new java.util.ArrayList(statefulDefaultActions);
}
/**
*
* The default actions to take on a packet that doesn't match any stateful rules. The stateful default action is
* optional, and is only valid when using the strict rule order.
*
*
* Valid values of the stateful default action:
*
*
* -
*
* aws:drop_strict
*
*
* -
*
* aws:drop_established
*
*
* -
*
* aws:alert_strict
*
*
* -
*
* aws:alert_established
*
*
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setStatefulDefaultActions(java.util.Collection)} or
* {@link #withStatefulDefaultActions(java.util.Collection)} if you want to override the existing values.
*
*
* @param statefulDefaultActions
* The default actions to take on a packet that doesn't match any stateful rules. The stateful default action
* is optional, and is only valid when using the strict rule order.
*
* Valid values of the stateful default action:
*
*
* -
*
* aws:drop_strict
*
*
* -
*
* aws:drop_established
*
*
* -
*
* aws:alert_strict
*
*
* -
*
* aws:alert_established
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public NetworkFirewallPolicyDescription withStatefulDefaultActions(String... statefulDefaultActions) {
if (this.statefulDefaultActions == null) {
setStatefulDefaultActions(new java.util.ArrayList(statefulDefaultActions.length));
}
for (String ele : statefulDefaultActions) {
this.statefulDefaultActions.add(ele);
}
return this;
}
/**
*
* The default actions to take on a packet that doesn't match any stateful rules. The stateful default action is
* optional, and is only valid when using the strict rule order.
*
*
* Valid values of the stateful default action:
*
*
* -
*
* aws:drop_strict
*
*
* -
*
* aws:drop_established
*
*
* -
*
* aws:alert_strict
*
*
* -
*
* aws:alert_established
*
*
*
*
* @param statefulDefaultActions
* The default actions to take on a packet that doesn't match any stateful rules. The stateful default action
* is optional, and is only valid when using the strict rule order.
*
* Valid values of the stateful default action:
*
*
* -
*
* aws:drop_strict
*
*
* -
*
* aws:drop_established
*
*
* -
*
* aws:alert_strict
*
*
* -
*
* aws:alert_established
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public NetworkFirewallPolicyDescription withStatefulDefaultActions(java.util.Collection statefulDefaultActions) {
setStatefulDefaultActions(statefulDefaultActions);
return this;
}
/**
*
* Additional options governing how Network Firewall handles stateful rules. The stateful rule groups that you use
* in your policy must have stateful rule options settings that are compatible with these settings.
*
*
* @param statefulEngineOptions
* Additional options governing how Network Firewall handles stateful rules. The stateful rule groups that
* you use in your policy must have stateful rule options settings that are compatible with these settings.
*/
public void setStatefulEngineOptions(StatefulEngineOptions statefulEngineOptions) {
this.statefulEngineOptions = statefulEngineOptions;
}
/**
*
* Additional options governing how Network Firewall handles stateful rules. The stateful rule groups that you use
* in your policy must have stateful rule options settings that are compatible with these settings.
*
*
* @return Additional options governing how Network Firewall handles stateful rules. The stateful rule groups that
* you use in your policy must have stateful rule options settings that are compatible with these settings.
*/
public StatefulEngineOptions getStatefulEngineOptions() {
return this.statefulEngineOptions;
}
/**
*
* Additional options governing how Network Firewall handles stateful rules. The stateful rule groups that you use
* in your policy must have stateful rule options settings that are compatible with these settings.
*
*
* @param statefulEngineOptions
* Additional options governing how Network Firewall handles stateful rules. The stateful rule groups that
* you use in your policy must have stateful rule options settings that are compatible with these settings.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public NetworkFirewallPolicyDescription withStatefulEngineOptions(StatefulEngineOptions statefulEngineOptions) {
setStatefulEngineOptions(statefulEngineOptions);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getStatelessRuleGroups() != null)
sb.append("StatelessRuleGroups: ").append(getStatelessRuleGroups()).append(",");
if (getStatelessDefaultActions() != null)
sb.append("StatelessDefaultActions: ").append(getStatelessDefaultActions()).append(",");
if (getStatelessFragmentDefaultActions() != null)
sb.append("StatelessFragmentDefaultActions: ").append(getStatelessFragmentDefaultActions()).append(",");
if (getStatelessCustomActions() != null)
sb.append("StatelessCustomActions: ").append(getStatelessCustomActions()).append(",");
if (getStatefulRuleGroups() != null)
sb.append("StatefulRuleGroups: ").append(getStatefulRuleGroups()).append(",");
if (getStatefulDefaultActions() != null)
sb.append("StatefulDefaultActions: ").append(getStatefulDefaultActions()).append(",");
if (getStatefulEngineOptions() != null)
sb.append("StatefulEngineOptions: ").append(getStatefulEngineOptions());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof NetworkFirewallPolicyDescription == false)
return false;
NetworkFirewallPolicyDescription other = (NetworkFirewallPolicyDescription) obj;
if (other.getStatelessRuleGroups() == null ^ this.getStatelessRuleGroups() == null)
return false;
if (other.getStatelessRuleGroups() != null && other.getStatelessRuleGroups().equals(this.getStatelessRuleGroups()) == false)
return false;
if (other.getStatelessDefaultActions() == null ^ this.getStatelessDefaultActions() == null)
return false;
if (other.getStatelessDefaultActions() != null && other.getStatelessDefaultActions().equals(this.getStatelessDefaultActions()) == false)
return false;
if (other.getStatelessFragmentDefaultActions() == null ^ this.getStatelessFragmentDefaultActions() == null)
return false;
if (other.getStatelessFragmentDefaultActions() != null
&& other.getStatelessFragmentDefaultActions().equals(this.getStatelessFragmentDefaultActions()) == false)
return false;
if (other.getStatelessCustomActions() == null ^ this.getStatelessCustomActions() == null)
return false;
if (other.getStatelessCustomActions() != null && other.getStatelessCustomActions().equals(this.getStatelessCustomActions()) == false)
return false;
if (other.getStatefulRuleGroups() == null ^ this.getStatefulRuleGroups() == null)
return false;
if (other.getStatefulRuleGroups() != null && other.getStatefulRuleGroups().equals(this.getStatefulRuleGroups()) == false)
return false;
if (other.getStatefulDefaultActions() == null ^ this.getStatefulDefaultActions() == null)
return false;
if (other.getStatefulDefaultActions() != null && other.getStatefulDefaultActions().equals(this.getStatefulDefaultActions()) == false)
return false;
if (other.getStatefulEngineOptions() == null ^ this.getStatefulEngineOptions() == null)
return false;
if (other.getStatefulEngineOptions() != null && other.getStatefulEngineOptions().equals(this.getStatefulEngineOptions()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getStatelessRuleGroups() == null) ? 0 : getStatelessRuleGroups().hashCode());
hashCode = prime * hashCode + ((getStatelessDefaultActions() == null) ? 0 : getStatelessDefaultActions().hashCode());
hashCode = prime * hashCode + ((getStatelessFragmentDefaultActions() == null) ? 0 : getStatelessFragmentDefaultActions().hashCode());
hashCode = prime * hashCode + ((getStatelessCustomActions() == null) ? 0 : getStatelessCustomActions().hashCode());
hashCode = prime * hashCode + ((getStatefulRuleGroups() == null) ? 0 : getStatefulRuleGroups().hashCode());
hashCode = prime * hashCode + ((getStatefulDefaultActions() == null) ? 0 : getStatefulDefaultActions().hashCode());
hashCode = prime * hashCode + ((getStatefulEngineOptions() == null) ? 0 : getStatefulEngineOptions().hashCode());
return hashCode;
}
@Override
public NetworkFirewallPolicyDescription clone() {
try {
return (NetworkFirewallPolicyDescription) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.fms.model.transform.NetworkFirewallPolicyDescriptionMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}