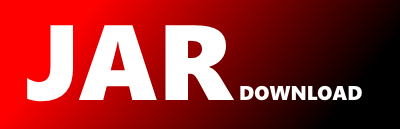
com.amazonaws.services.fms.model.Policy Maven / Gradle / Ivy
Show all versions of aws-java-sdk-fms Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.fms.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* An Firewall Manager policy.
*
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class Policy implements Serializable, Cloneable, StructuredPojo {
/**
*
* The ID of the Firewall Manager policy.
*
*/
private String policyId;
/**
*
* The name of the Firewall Manager policy.
*
*/
private String policyName;
/**
*
* A unique identifier for each update to the policy. When issuing a PutPolicy
request, the
* PolicyUpdateToken
in the request must match the PolicyUpdateToken
of the current policy
* version. To get the PolicyUpdateToken
of the current policy version, use a GetPolicy
* request.
*
*/
private String policyUpdateToken;
/**
*
* Details about the security service that is being used to protect the resources.
*
*/
private SecurityServicePolicyData securityServicePolicyData;
/**
*
* The type of resource protected by or in scope of the policy. This is in the format shown in the Amazon
* Web Services Resource Types Reference. To apply this policy to multiple resource types, specify a resource
* type of ResourceTypeList
and then specify the resource types in a ResourceTypeList
.
*
*
* The following are valid resource types for each Firewall Manager policy type:
*
*
* -
*
* Amazon Web Services WAF Classic - AWS::ApiGateway::Stage
, AWS::CloudFront::Distribution
* , and AWS::ElasticLoadBalancingV2::LoadBalancer
.
*
*
* -
*
* WAF - AWS::ApiGateway::Stage
, AWS::ElasticLoadBalancingV2::LoadBalancer
, and
* AWS::CloudFront::Distribution
.
*
*
* -
*
* Shield Advanced - AWS::ElasticLoadBalancingV2::LoadBalancer
,
* AWS::ElasticLoadBalancing::LoadBalancer
, AWS::EC2::EIP
, and
* AWS::CloudFront::Distribution
.
*
*
* -
*
* Network ACL - AWS::EC2::Subnet
.
*
*
* -
*
* Security group usage audit - AWS::EC2::SecurityGroup
.
*
*
* -
*
* Security group content audit - AWS::EC2::SecurityGroup
, AWS::EC2::NetworkInterface
, and
* AWS::EC2::Instance
.
*
*
* -
*
* DNS Firewall, Network Firewall, and third-party firewall - AWS::EC2::VPC
.
*
*
*
*/
private String resourceType;
/**
*
* An array of ResourceType
objects. Use this only to specify multiple resource types. To specify a
* single resource type, use ResourceType
.
*
*/
private java.util.List resourceTypeList;
/**
*
* An array of ResourceTag
objects.
*
*/
private java.util.List resourceTags;
/**
*
* If set to True
, resources with the tags that are specified in the ResourceTag
array are
* not in scope of the policy. If set to False
, and the ResourceTag
array is not null,
* only resources with the specified tags are in scope of the policy.
*
*/
private Boolean excludeResourceTags;
/**
*
* Indicates if the policy should be automatically applied to new resources.
*
*/
private Boolean remediationEnabled;
/**
*
* Indicates whether Firewall Manager should automatically remove protections from resources that leave the policy
* scope and clean up resources that Firewall Manager is managing for accounts when those accounts leave policy
* scope. For example, Firewall Manager will disassociate a Firewall Manager managed web ACL from a protected
* customer resource when the customer resource leaves policy scope.
*
*
* By default, Firewall Manager doesn't remove protections or delete Firewall Manager managed resources.
*
*
* This option is not available for Shield Advanced or WAF Classic policies.
*
*/
private Boolean deleteUnusedFMManagedResources;
/**
*
* Specifies the Amazon Web Services account IDs and Organizations organizational units (OUs) to include in the
* policy. Specifying an OU is the equivalent of specifying all accounts in the OU and in any of its child OUs,
* including any child OUs and accounts that are added at a later time.
*
*
* You can specify inclusions or exclusions, but not both. If you specify an IncludeMap
, Firewall
* Manager applies the policy to all accounts specified by the IncludeMap
, and does not evaluate any
* ExcludeMap
specifications. If you do not specify an IncludeMap
, then Firewall Manager
* applies the policy to all accounts except for those specified by the ExcludeMap
.
*
*
* You can specify account IDs, OUs, or a combination:
*
*
* -
*
* Specify account IDs by setting the key to ACCOUNT
. For example, the following is a valid map:
* {“ACCOUNT” : [“accountID1”, “accountID2”]}
.
*
*
* -
*
* Specify OUs by setting the key to ORG_UNIT
. For example, the following is a valid map:
* {“ORG_UNIT” : [“ouid111”, “ouid112”]}
.
*
*
* -
*
* Specify accounts and OUs together in a single map, separated with a comma. For example, the following is a valid
* map: {“ACCOUNT” : [“accountID1”, “accountID2”], “ORG_UNIT” : [“ouid111”, “ouid112”]}
.
*
*
*
*/
private java.util.Map> includeMap;
/**
*
* Specifies the Amazon Web Services account IDs and Organizations organizational units (OUs) to exclude from the
* policy. Specifying an OU is the equivalent of specifying all accounts in the OU and in any of its child OUs,
* including any child OUs and accounts that are added at a later time.
*
*
* You can specify inclusions or exclusions, but not both. If you specify an IncludeMap
, Firewall
* Manager applies the policy to all accounts specified by the IncludeMap
, and does not evaluate any
* ExcludeMap
specifications. If you do not specify an IncludeMap
, then Firewall Manager
* applies the policy to all accounts except for those specified by the ExcludeMap
.
*
*
* You can specify account IDs, OUs, or a combination:
*
*
* -
*
* Specify account IDs by setting the key to ACCOUNT
. For example, the following is a valid map:
* {“ACCOUNT” : [“accountID1”, “accountID2”]}
.
*
*
* -
*
* Specify OUs by setting the key to ORG_UNIT
. For example, the following is a valid map:
* {“ORG_UNIT” : [“ouid111”, “ouid112”]}
.
*
*
* -
*
* Specify accounts and OUs together in a single map, separated with a comma. For example, the following is a valid
* map: {“ACCOUNT” : [“accountID1”, “accountID2”], “ORG_UNIT” : [“ouid111”, “ouid112”]}
.
*
*
*
*/
private java.util.Map> excludeMap;
/**
*
* The unique identifiers of the resource sets used by the policy.
*
*/
private java.util.List resourceSetIds;
/**
*
* Your description of the Firewall Manager policy.
*
*/
private String policyDescription;
/**
*
* Indicates whether the policy is in or out of an admin's policy or Region scope.
*
*
* -
*
* ACTIVE
- The administrator can manage and delete the policy.
*
*
* -
*
* OUT_OF_ADMIN_SCOPE
- The administrator can view the policy, but they can't edit or delete the
* policy. Existing policy protections stay in place. Any new resources that come into scope of the policy won't be
* protected.
*
*
*
*/
private String policyStatus;
/**
*
* The ID of the Firewall Manager policy.
*
*
* @param policyId
* The ID of the Firewall Manager policy.
*/
public void setPolicyId(String policyId) {
this.policyId = policyId;
}
/**
*
* The ID of the Firewall Manager policy.
*
*
* @return The ID of the Firewall Manager policy.
*/
public String getPolicyId() {
return this.policyId;
}
/**
*
* The ID of the Firewall Manager policy.
*
*
* @param policyId
* The ID of the Firewall Manager policy.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Policy withPolicyId(String policyId) {
setPolicyId(policyId);
return this;
}
/**
*
* The name of the Firewall Manager policy.
*
*
* @param policyName
* The name of the Firewall Manager policy.
*/
public void setPolicyName(String policyName) {
this.policyName = policyName;
}
/**
*
* The name of the Firewall Manager policy.
*
*
* @return The name of the Firewall Manager policy.
*/
public String getPolicyName() {
return this.policyName;
}
/**
*
* The name of the Firewall Manager policy.
*
*
* @param policyName
* The name of the Firewall Manager policy.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Policy withPolicyName(String policyName) {
setPolicyName(policyName);
return this;
}
/**
*
* A unique identifier for each update to the policy. When issuing a PutPolicy
request, the
* PolicyUpdateToken
in the request must match the PolicyUpdateToken
of the current policy
* version. To get the PolicyUpdateToken
of the current policy version, use a GetPolicy
* request.
*
*
* @param policyUpdateToken
* A unique identifier for each update to the policy. When issuing a PutPolicy
request, the
* PolicyUpdateToken
in the request must match the PolicyUpdateToken
of the current
* policy version. To get the PolicyUpdateToken
of the current policy version, use a
* GetPolicy
request.
*/
public void setPolicyUpdateToken(String policyUpdateToken) {
this.policyUpdateToken = policyUpdateToken;
}
/**
*
* A unique identifier for each update to the policy. When issuing a PutPolicy
request, the
* PolicyUpdateToken
in the request must match the PolicyUpdateToken
of the current policy
* version. To get the PolicyUpdateToken
of the current policy version, use a GetPolicy
* request.
*
*
* @return A unique identifier for each update to the policy. When issuing a PutPolicy
request, the
* PolicyUpdateToken
in the request must match the PolicyUpdateToken
of the
* current policy version. To get the PolicyUpdateToken
of the current policy version, use a
* GetPolicy
request.
*/
public String getPolicyUpdateToken() {
return this.policyUpdateToken;
}
/**
*
* A unique identifier for each update to the policy. When issuing a PutPolicy
request, the
* PolicyUpdateToken
in the request must match the PolicyUpdateToken
of the current policy
* version. To get the PolicyUpdateToken
of the current policy version, use a GetPolicy
* request.
*
*
* @param policyUpdateToken
* A unique identifier for each update to the policy. When issuing a PutPolicy
request, the
* PolicyUpdateToken
in the request must match the PolicyUpdateToken
of the current
* policy version. To get the PolicyUpdateToken
of the current policy version, use a
* GetPolicy
request.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Policy withPolicyUpdateToken(String policyUpdateToken) {
setPolicyUpdateToken(policyUpdateToken);
return this;
}
/**
*
* Details about the security service that is being used to protect the resources.
*
*
* @param securityServicePolicyData
* Details about the security service that is being used to protect the resources.
*/
public void setSecurityServicePolicyData(SecurityServicePolicyData securityServicePolicyData) {
this.securityServicePolicyData = securityServicePolicyData;
}
/**
*
* Details about the security service that is being used to protect the resources.
*
*
* @return Details about the security service that is being used to protect the resources.
*/
public SecurityServicePolicyData getSecurityServicePolicyData() {
return this.securityServicePolicyData;
}
/**
*
* Details about the security service that is being used to protect the resources.
*
*
* @param securityServicePolicyData
* Details about the security service that is being used to protect the resources.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Policy withSecurityServicePolicyData(SecurityServicePolicyData securityServicePolicyData) {
setSecurityServicePolicyData(securityServicePolicyData);
return this;
}
/**
*
* The type of resource protected by or in scope of the policy. This is in the format shown in the Amazon
* Web Services Resource Types Reference. To apply this policy to multiple resource types, specify a resource
* type of ResourceTypeList
and then specify the resource types in a ResourceTypeList
.
*
*
* The following are valid resource types for each Firewall Manager policy type:
*
*
* -
*
* Amazon Web Services WAF Classic - AWS::ApiGateway::Stage
, AWS::CloudFront::Distribution
* , and AWS::ElasticLoadBalancingV2::LoadBalancer
.
*
*
* -
*
* WAF - AWS::ApiGateway::Stage
, AWS::ElasticLoadBalancingV2::LoadBalancer
, and
* AWS::CloudFront::Distribution
.
*
*
* -
*
* Shield Advanced - AWS::ElasticLoadBalancingV2::LoadBalancer
,
* AWS::ElasticLoadBalancing::LoadBalancer
, AWS::EC2::EIP
, and
* AWS::CloudFront::Distribution
.
*
*
* -
*
* Network ACL - AWS::EC2::Subnet
.
*
*
* -
*
* Security group usage audit - AWS::EC2::SecurityGroup
.
*
*
* -
*
* Security group content audit - AWS::EC2::SecurityGroup
, AWS::EC2::NetworkInterface
, and
* AWS::EC2::Instance
.
*
*
* -
*
* DNS Firewall, Network Firewall, and third-party firewall - AWS::EC2::VPC
.
*
*
*
*
* @param resourceType
* The type of resource protected by or in scope of the policy. This is in the format shown in the Amazon Web Services Resource Types Reference. To apply this policy to multiple resource types,
* specify a resource type of ResourceTypeList
and then specify the resource types in a
* ResourceTypeList
.
*
* The following are valid resource types for each Firewall Manager policy type:
*
*
* -
*
* Amazon Web Services WAF Classic - AWS::ApiGateway::Stage
,
* AWS::CloudFront::Distribution
, and AWS::ElasticLoadBalancingV2::LoadBalancer
.
*
*
* -
*
* WAF - AWS::ApiGateway::Stage
, AWS::ElasticLoadBalancingV2::LoadBalancer
, and
* AWS::CloudFront::Distribution
.
*
*
* -
*
* Shield Advanced - AWS::ElasticLoadBalancingV2::LoadBalancer
,
* AWS::ElasticLoadBalancing::LoadBalancer
, AWS::EC2::EIP
, and
* AWS::CloudFront::Distribution
.
*
*
* -
*
* Network ACL - AWS::EC2::Subnet
.
*
*
* -
*
* Security group usage audit - AWS::EC2::SecurityGroup
.
*
*
* -
*
* Security group content audit - AWS::EC2::SecurityGroup
,
* AWS::EC2::NetworkInterface
, and AWS::EC2::Instance
.
*
*
* -
*
* DNS Firewall, Network Firewall, and third-party firewall - AWS::EC2::VPC
.
*
*
*/
public void setResourceType(String resourceType) {
this.resourceType = resourceType;
}
/**
*
* The type of resource protected by or in scope of the policy. This is in the format shown in the Amazon
* Web Services Resource Types Reference. To apply this policy to multiple resource types, specify a resource
* type of ResourceTypeList
and then specify the resource types in a ResourceTypeList
.
*
*
* The following are valid resource types for each Firewall Manager policy type:
*
*
* -
*
* Amazon Web Services WAF Classic - AWS::ApiGateway::Stage
, AWS::CloudFront::Distribution
* , and AWS::ElasticLoadBalancingV2::LoadBalancer
.
*
*
* -
*
* WAF - AWS::ApiGateway::Stage
, AWS::ElasticLoadBalancingV2::LoadBalancer
, and
* AWS::CloudFront::Distribution
.
*
*
* -
*
* Shield Advanced - AWS::ElasticLoadBalancingV2::LoadBalancer
,
* AWS::ElasticLoadBalancing::LoadBalancer
, AWS::EC2::EIP
, and
* AWS::CloudFront::Distribution
.
*
*
* -
*
* Network ACL - AWS::EC2::Subnet
.
*
*
* -
*
* Security group usage audit - AWS::EC2::SecurityGroup
.
*
*
* -
*
* Security group content audit - AWS::EC2::SecurityGroup
, AWS::EC2::NetworkInterface
, and
* AWS::EC2::Instance
.
*
*
* -
*
* DNS Firewall, Network Firewall, and third-party firewall - AWS::EC2::VPC
.
*
*
*
*
* @return The type of resource protected by or in scope of the policy. This is in the format shown in the Amazon Web Services Resource Types Reference. To apply this policy to multiple resource types,
* specify a resource type of ResourceTypeList
and then specify the resource types in a
* ResourceTypeList
.
*
* The following are valid resource types for each Firewall Manager policy type:
*
*
* -
*
* Amazon Web Services WAF Classic - AWS::ApiGateway::Stage
,
* AWS::CloudFront::Distribution
, and AWS::ElasticLoadBalancingV2::LoadBalancer
.
*
*
* -
*
* WAF - AWS::ApiGateway::Stage
, AWS::ElasticLoadBalancingV2::LoadBalancer
, and
* AWS::CloudFront::Distribution
.
*
*
* -
*
* Shield Advanced - AWS::ElasticLoadBalancingV2::LoadBalancer
,
* AWS::ElasticLoadBalancing::LoadBalancer
, AWS::EC2::EIP
, and
* AWS::CloudFront::Distribution
.
*
*
* -
*
* Network ACL - AWS::EC2::Subnet
.
*
*
* -
*
* Security group usage audit - AWS::EC2::SecurityGroup
.
*
*
* -
*
* Security group content audit - AWS::EC2::SecurityGroup
,
* AWS::EC2::NetworkInterface
, and AWS::EC2::Instance
.
*
*
* -
*
* DNS Firewall, Network Firewall, and third-party firewall - AWS::EC2::VPC
.
*
*
*/
public String getResourceType() {
return this.resourceType;
}
/**
*
* The type of resource protected by or in scope of the policy. This is in the format shown in the Amazon
* Web Services Resource Types Reference. To apply this policy to multiple resource types, specify a resource
* type of ResourceTypeList
and then specify the resource types in a ResourceTypeList
.
*
*
* The following are valid resource types for each Firewall Manager policy type:
*
*
* -
*
* Amazon Web Services WAF Classic - AWS::ApiGateway::Stage
, AWS::CloudFront::Distribution
* , and AWS::ElasticLoadBalancingV2::LoadBalancer
.
*
*
* -
*
* WAF - AWS::ApiGateway::Stage
, AWS::ElasticLoadBalancingV2::LoadBalancer
, and
* AWS::CloudFront::Distribution
.
*
*
* -
*
* Shield Advanced - AWS::ElasticLoadBalancingV2::LoadBalancer
,
* AWS::ElasticLoadBalancing::LoadBalancer
, AWS::EC2::EIP
, and
* AWS::CloudFront::Distribution
.
*
*
* -
*
* Network ACL - AWS::EC2::Subnet
.
*
*
* -
*
* Security group usage audit - AWS::EC2::SecurityGroup
.
*
*
* -
*
* Security group content audit - AWS::EC2::SecurityGroup
, AWS::EC2::NetworkInterface
, and
* AWS::EC2::Instance
.
*
*
* -
*
* DNS Firewall, Network Firewall, and third-party firewall - AWS::EC2::VPC
.
*
*
*
*
* @param resourceType
* The type of resource protected by or in scope of the policy. This is in the format shown in the Amazon Web Services Resource Types Reference. To apply this policy to multiple resource types,
* specify a resource type of ResourceTypeList
and then specify the resource types in a
* ResourceTypeList
.
*
* The following are valid resource types for each Firewall Manager policy type:
*
*
* -
*
* Amazon Web Services WAF Classic - AWS::ApiGateway::Stage
,
* AWS::CloudFront::Distribution
, and AWS::ElasticLoadBalancingV2::LoadBalancer
.
*
*
* -
*
* WAF - AWS::ApiGateway::Stage
, AWS::ElasticLoadBalancingV2::LoadBalancer
, and
* AWS::CloudFront::Distribution
.
*
*
* -
*
* Shield Advanced - AWS::ElasticLoadBalancingV2::LoadBalancer
,
* AWS::ElasticLoadBalancing::LoadBalancer
, AWS::EC2::EIP
, and
* AWS::CloudFront::Distribution
.
*
*
* -
*
* Network ACL - AWS::EC2::Subnet
.
*
*
* -
*
* Security group usage audit - AWS::EC2::SecurityGroup
.
*
*
* -
*
* Security group content audit - AWS::EC2::SecurityGroup
,
* AWS::EC2::NetworkInterface
, and AWS::EC2::Instance
.
*
*
* -
*
* DNS Firewall, Network Firewall, and third-party firewall - AWS::EC2::VPC
.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Policy withResourceType(String resourceType) {
setResourceType(resourceType);
return this;
}
/**
*
* An array of ResourceType
objects. Use this only to specify multiple resource types. To specify a
* single resource type, use ResourceType
.
*
*
* @return An array of ResourceType
objects. Use this only to specify multiple resource types. To
* specify a single resource type, use ResourceType
.
*/
public java.util.List getResourceTypeList() {
return resourceTypeList;
}
/**
*
* An array of ResourceType
objects. Use this only to specify multiple resource types. To specify a
* single resource type, use ResourceType
.
*
*
* @param resourceTypeList
* An array of ResourceType
objects. Use this only to specify multiple resource types. To
* specify a single resource type, use ResourceType
.
*/
public void setResourceTypeList(java.util.Collection resourceTypeList) {
if (resourceTypeList == null) {
this.resourceTypeList = null;
return;
}
this.resourceTypeList = new java.util.ArrayList(resourceTypeList);
}
/**
*
* An array of ResourceType
objects. Use this only to specify multiple resource types. To specify a
* single resource type, use ResourceType
.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setResourceTypeList(java.util.Collection)} or {@link #withResourceTypeList(java.util.Collection)} if you
* want to override the existing values.
*
*
* @param resourceTypeList
* An array of ResourceType
objects. Use this only to specify multiple resource types. To
* specify a single resource type, use ResourceType
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Policy withResourceTypeList(String... resourceTypeList) {
if (this.resourceTypeList == null) {
setResourceTypeList(new java.util.ArrayList(resourceTypeList.length));
}
for (String ele : resourceTypeList) {
this.resourceTypeList.add(ele);
}
return this;
}
/**
*
* An array of ResourceType
objects. Use this only to specify multiple resource types. To specify a
* single resource type, use ResourceType
.
*
*
* @param resourceTypeList
* An array of ResourceType
objects. Use this only to specify multiple resource types. To
* specify a single resource type, use ResourceType
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Policy withResourceTypeList(java.util.Collection resourceTypeList) {
setResourceTypeList(resourceTypeList);
return this;
}
/**
*
* An array of ResourceTag
objects.
*
*
* @return An array of ResourceTag
objects.
*/
public java.util.List getResourceTags() {
return resourceTags;
}
/**
*
* An array of ResourceTag
objects.
*
*
* @param resourceTags
* An array of ResourceTag
objects.
*/
public void setResourceTags(java.util.Collection resourceTags) {
if (resourceTags == null) {
this.resourceTags = null;
return;
}
this.resourceTags = new java.util.ArrayList(resourceTags);
}
/**
*
* An array of ResourceTag
objects.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setResourceTags(java.util.Collection)} or {@link #withResourceTags(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param resourceTags
* An array of ResourceTag
objects.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Policy withResourceTags(ResourceTag... resourceTags) {
if (this.resourceTags == null) {
setResourceTags(new java.util.ArrayList(resourceTags.length));
}
for (ResourceTag ele : resourceTags) {
this.resourceTags.add(ele);
}
return this;
}
/**
*
* An array of ResourceTag
objects.
*
*
* @param resourceTags
* An array of ResourceTag
objects.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Policy withResourceTags(java.util.Collection resourceTags) {
setResourceTags(resourceTags);
return this;
}
/**
*
* If set to True
, resources with the tags that are specified in the ResourceTag
array are
* not in scope of the policy. If set to False
, and the ResourceTag
array is not null,
* only resources with the specified tags are in scope of the policy.
*
*
* @param excludeResourceTags
* If set to True
, resources with the tags that are specified in the ResourceTag
* array are not in scope of the policy. If set to False
, and the ResourceTag
array
* is not null, only resources with the specified tags are in scope of the policy.
*/
public void setExcludeResourceTags(Boolean excludeResourceTags) {
this.excludeResourceTags = excludeResourceTags;
}
/**
*
* If set to True
, resources with the tags that are specified in the ResourceTag
array are
* not in scope of the policy. If set to False
, and the ResourceTag
array is not null,
* only resources with the specified tags are in scope of the policy.
*
*
* @return If set to True
, resources with the tags that are specified in the ResourceTag
* array are not in scope of the policy. If set to False
, and the ResourceTag
* array is not null, only resources with the specified tags are in scope of the policy.
*/
public Boolean getExcludeResourceTags() {
return this.excludeResourceTags;
}
/**
*
* If set to True
, resources with the tags that are specified in the ResourceTag
array are
* not in scope of the policy. If set to False
, and the ResourceTag
array is not null,
* only resources with the specified tags are in scope of the policy.
*
*
* @param excludeResourceTags
* If set to True
, resources with the tags that are specified in the ResourceTag
* array are not in scope of the policy. If set to False
, and the ResourceTag
array
* is not null, only resources with the specified tags are in scope of the policy.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Policy withExcludeResourceTags(Boolean excludeResourceTags) {
setExcludeResourceTags(excludeResourceTags);
return this;
}
/**
*
* If set to True
, resources with the tags that are specified in the ResourceTag
array are
* not in scope of the policy. If set to False
, and the ResourceTag
array is not null,
* only resources with the specified tags are in scope of the policy.
*
*
* @return If set to True
, resources with the tags that are specified in the ResourceTag
* array are not in scope of the policy. If set to False
, and the ResourceTag
* array is not null, only resources with the specified tags are in scope of the policy.
*/
public Boolean isExcludeResourceTags() {
return this.excludeResourceTags;
}
/**
*
* Indicates if the policy should be automatically applied to new resources.
*
*
* @param remediationEnabled
* Indicates if the policy should be automatically applied to new resources.
*/
public void setRemediationEnabled(Boolean remediationEnabled) {
this.remediationEnabled = remediationEnabled;
}
/**
*
* Indicates if the policy should be automatically applied to new resources.
*
*
* @return Indicates if the policy should be automatically applied to new resources.
*/
public Boolean getRemediationEnabled() {
return this.remediationEnabled;
}
/**
*
* Indicates if the policy should be automatically applied to new resources.
*
*
* @param remediationEnabled
* Indicates if the policy should be automatically applied to new resources.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Policy withRemediationEnabled(Boolean remediationEnabled) {
setRemediationEnabled(remediationEnabled);
return this;
}
/**
*
* Indicates if the policy should be automatically applied to new resources.
*
*
* @return Indicates if the policy should be automatically applied to new resources.
*/
public Boolean isRemediationEnabled() {
return this.remediationEnabled;
}
/**
*
* Indicates whether Firewall Manager should automatically remove protections from resources that leave the policy
* scope and clean up resources that Firewall Manager is managing for accounts when those accounts leave policy
* scope. For example, Firewall Manager will disassociate a Firewall Manager managed web ACL from a protected
* customer resource when the customer resource leaves policy scope.
*
*
* By default, Firewall Manager doesn't remove protections or delete Firewall Manager managed resources.
*
*
* This option is not available for Shield Advanced or WAF Classic policies.
*
*
* @param deleteUnusedFMManagedResources
* Indicates whether Firewall Manager should automatically remove protections from resources that leave the
* policy scope and clean up resources that Firewall Manager is managing for accounts when those accounts
* leave policy scope. For example, Firewall Manager will disassociate a Firewall Manager managed web ACL
* from a protected customer resource when the customer resource leaves policy scope.
*
* By default, Firewall Manager doesn't remove protections or delete Firewall Manager managed resources.
*
*
* This option is not available for Shield Advanced or WAF Classic policies.
*/
public void setDeleteUnusedFMManagedResources(Boolean deleteUnusedFMManagedResources) {
this.deleteUnusedFMManagedResources = deleteUnusedFMManagedResources;
}
/**
*
* Indicates whether Firewall Manager should automatically remove protections from resources that leave the policy
* scope and clean up resources that Firewall Manager is managing for accounts when those accounts leave policy
* scope. For example, Firewall Manager will disassociate a Firewall Manager managed web ACL from a protected
* customer resource when the customer resource leaves policy scope.
*
*
* By default, Firewall Manager doesn't remove protections or delete Firewall Manager managed resources.
*
*
* This option is not available for Shield Advanced or WAF Classic policies.
*
*
* @return Indicates whether Firewall Manager should automatically remove protections from resources that leave the
* policy scope and clean up resources that Firewall Manager is managing for accounts when those accounts
* leave policy scope. For example, Firewall Manager will disassociate a Firewall Manager managed web ACL
* from a protected customer resource when the customer resource leaves policy scope.
*
* By default, Firewall Manager doesn't remove protections or delete Firewall Manager managed resources.
*
*
* This option is not available for Shield Advanced or WAF Classic policies.
*/
public Boolean getDeleteUnusedFMManagedResources() {
return this.deleteUnusedFMManagedResources;
}
/**
*
* Indicates whether Firewall Manager should automatically remove protections from resources that leave the policy
* scope and clean up resources that Firewall Manager is managing for accounts when those accounts leave policy
* scope. For example, Firewall Manager will disassociate a Firewall Manager managed web ACL from a protected
* customer resource when the customer resource leaves policy scope.
*
*
* By default, Firewall Manager doesn't remove protections or delete Firewall Manager managed resources.
*
*
* This option is not available for Shield Advanced or WAF Classic policies.
*
*
* @param deleteUnusedFMManagedResources
* Indicates whether Firewall Manager should automatically remove protections from resources that leave the
* policy scope and clean up resources that Firewall Manager is managing for accounts when those accounts
* leave policy scope. For example, Firewall Manager will disassociate a Firewall Manager managed web ACL
* from a protected customer resource when the customer resource leaves policy scope.
*
* By default, Firewall Manager doesn't remove protections or delete Firewall Manager managed resources.
*
*
* This option is not available for Shield Advanced or WAF Classic policies.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Policy withDeleteUnusedFMManagedResources(Boolean deleteUnusedFMManagedResources) {
setDeleteUnusedFMManagedResources(deleteUnusedFMManagedResources);
return this;
}
/**
*
* Indicates whether Firewall Manager should automatically remove protections from resources that leave the policy
* scope and clean up resources that Firewall Manager is managing for accounts when those accounts leave policy
* scope. For example, Firewall Manager will disassociate a Firewall Manager managed web ACL from a protected
* customer resource when the customer resource leaves policy scope.
*
*
* By default, Firewall Manager doesn't remove protections or delete Firewall Manager managed resources.
*
*
* This option is not available for Shield Advanced or WAF Classic policies.
*
*
* @return Indicates whether Firewall Manager should automatically remove protections from resources that leave the
* policy scope and clean up resources that Firewall Manager is managing for accounts when those accounts
* leave policy scope. For example, Firewall Manager will disassociate a Firewall Manager managed web ACL
* from a protected customer resource when the customer resource leaves policy scope.
*
* By default, Firewall Manager doesn't remove protections or delete Firewall Manager managed resources.
*
*
* This option is not available for Shield Advanced or WAF Classic policies.
*/
public Boolean isDeleteUnusedFMManagedResources() {
return this.deleteUnusedFMManagedResources;
}
/**
*
* Specifies the Amazon Web Services account IDs and Organizations organizational units (OUs) to include in the
* policy. Specifying an OU is the equivalent of specifying all accounts in the OU and in any of its child OUs,
* including any child OUs and accounts that are added at a later time.
*
*
* You can specify inclusions or exclusions, but not both. If you specify an IncludeMap
, Firewall
* Manager applies the policy to all accounts specified by the IncludeMap
, and does not evaluate any
* ExcludeMap
specifications. If you do not specify an IncludeMap
, then Firewall Manager
* applies the policy to all accounts except for those specified by the ExcludeMap
.
*
*
* You can specify account IDs, OUs, or a combination:
*
*
* -
*
* Specify account IDs by setting the key to ACCOUNT
. For example, the following is a valid map:
* {“ACCOUNT” : [“accountID1”, “accountID2”]}
.
*
*
* -
*
* Specify OUs by setting the key to ORG_UNIT
. For example, the following is a valid map:
* {“ORG_UNIT” : [“ouid111”, “ouid112”]}
.
*
*
* -
*
* Specify accounts and OUs together in a single map, separated with a comma. For example, the following is a valid
* map: {“ACCOUNT” : [“accountID1”, “accountID2”], “ORG_UNIT” : [“ouid111”, “ouid112”]}
.
*
*
*
*
* @return Specifies the Amazon Web Services account IDs and Organizations organizational units (OUs) to include in
* the policy. Specifying an OU is the equivalent of specifying all accounts in the OU and in any of its
* child OUs, including any child OUs and accounts that are added at a later time.
*
* You can specify inclusions or exclusions, but not both. If you specify an IncludeMap
,
* Firewall Manager applies the policy to all accounts specified by the IncludeMap
, and does
* not evaluate any ExcludeMap
specifications. If you do not specify an IncludeMap
* , then Firewall Manager applies the policy to all accounts except for those specified by the
* ExcludeMap
.
*
*
* You can specify account IDs, OUs, or a combination:
*
*
* -
*
* Specify account IDs by setting the key to ACCOUNT
. For example, the following is a valid
* map: {“ACCOUNT” : [“accountID1”, “accountID2”]}
.
*
*
* -
*
* Specify OUs by setting the key to ORG_UNIT
. For example, the following is a valid map:
* {“ORG_UNIT” : [“ouid111”, “ouid112”]}
.
*
*
* -
*
* Specify accounts and OUs together in a single map, separated with a comma. For example, the following is
* a valid map: {“ACCOUNT” : [“accountID1”, “accountID2”], “ORG_UNIT” : [“ouid111”, “ouid112”]}
* .
*
*
*/
public java.util.Map> getIncludeMap() {
return includeMap;
}
/**
*
* Specifies the Amazon Web Services account IDs and Organizations organizational units (OUs) to include in the
* policy. Specifying an OU is the equivalent of specifying all accounts in the OU and in any of its child OUs,
* including any child OUs and accounts that are added at a later time.
*
*
* You can specify inclusions or exclusions, but not both. If you specify an IncludeMap
, Firewall
* Manager applies the policy to all accounts specified by the IncludeMap
, and does not evaluate any
* ExcludeMap
specifications. If you do not specify an IncludeMap
, then Firewall Manager
* applies the policy to all accounts except for those specified by the ExcludeMap
.
*
*
* You can specify account IDs, OUs, or a combination:
*
*
* -
*
* Specify account IDs by setting the key to ACCOUNT
. For example, the following is a valid map:
* {“ACCOUNT” : [“accountID1”, “accountID2”]}
.
*
*
* -
*
* Specify OUs by setting the key to ORG_UNIT
. For example, the following is a valid map:
* {“ORG_UNIT” : [“ouid111”, “ouid112”]}
.
*
*
* -
*
* Specify accounts and OUs together in a single map, separated with a comma. For example, the following is a valid
* map: {“ACCOUNT” : [“accountID1”, “accountID2”], “ORG_UNIT” : [“ouid111”, “ouid112”]}
.
*
*
*
*
* @param includeMap
* Specifies the Amazon Web Services account IDs and Organizations organizational units (OUs) to include in
* the policy. Specifying an OU is the equivalent of specifying all accounts in the OU and in any of its
* child OUs, including any child OUs and accounts that are added at a later time.
*
* You can specify inclusions or exclusions, but not both. If you specify an IncludeMap
,
* Firewall Manager applies the policy to all accounts specified by the IncludeMap
, and does not
* evaluate any ExcludeMap
specifications. If you do not specify an IncludeMap
,
* then Firewall Manager applies the policy to all accounts except for those specified by the
* ExcludeMap
.
*
*
* You can specify account IDs, OUs, or a combination:
*
*
* -
*
* Specify account IDs by setting the key to ACCOUNT
. For example, the following is a valid map:
* {“ACCOUNT” : [“accountID1”, “accountID2”]}
.
*
*
* -
*
* Specify OUs by setting the key to ORG_UNIT
. For example, the following is a valid map:
* {“ORG_UNIT” : [“ouid111”, “ouid112”]}
.
*
*
* -
*
* Specify accounts and OUs together in a single map, separated with a comma. For example, the following is a
* valid map: {“ACCOUNT” : [“accountID1”, “accountID2”], “ORG_UNIT” : [“ouid111”, “ouid112”]}
.
*
*
*/
public void setIncludeMap(java.util.Map> includeMap) {
this.includeMap = includeMap;
}
/**
*
* Specifies the Amazon Web Services account IDs and Organizations organizational units (OUs) to include in the
* policy. Specifying an OU is the equivalent of specifying all accounts in the OU and in any of its child OUs,
* including any child OUs and accounts that are added at a later time.
*
*
* You can specify inclusions or exclusions, but not both. If you specify an IncludeMap
, Firewall
* Manager applies the policy to all accounts specified by the IncludeMap
, and does not evaluate any
* ExcludeMap
specifications. If you do not specify an IncludeMap
, then Firewall Manager
* applies the policy to all accounts except for those specified by the ExcludeMap
.
*
*
* You can specify account IDs, OUs, or a combination:
*
*
* -
*
* Specify account IDs by setting the key to ACCOUNT
. For example, the following is a valid map:
* {“ACCOUNT” : [“accountID1”, “accountID2”]}
.
*
*
* -
*
* Specify OUs by setting the key to ORG_UNIT
. For example, the following is a valid map:
* {“ORG_UNIT” : [“ouid111”, “ouid112”]}
.
*
*
* -
*
* Specify accounts and OUs together in a single map, separated with a comma. For example, the following is a valid
* map: {“ACCOUNT” : [“accountID1”, “accountID2”], “ORG_UNIT” : [“ouid111”, “ouid112”]}
.
*
*
*
*
* @param includeMap
* Specifies the Amazon Web Services account IDs and Organizations organizational units (OUs) to include in
* the policy. Specifying an OU is the equivalent of specifying all accounts in the OU and in any of its
* child OUs, including any child OUs and accounts that are added at a later time.
*
* You can specify inclusions or exclusions, but not both. If you specify an IncludeMap
,
* Firewall Manager applies the policy to all accounts specified by the IncludeMap
, and does not
* evaluate any ExcludeMap
specifications. If you do not specify an IncludeMap
,
* then Firewall Manager applies the policy to all accounts except for those specified by the
* ExcludeMap
.
*
*
* You can specify account IDs, OUs, or a combination:
*
*
* -
*
* Specify account IDs by setting the key to ACCOUNT
. For example, the following is a valid map:
* {“ACCOUNT” : [“accountID1”, “accountID2”]}
.
*
*
* -
*
* Specify OUs by setting the key to ORG_UNIT
. For example, the following is a valid map:
* {“ORG_UNIT” : [“ouid111”, “ouid112”]}
.
*
*
* -
*
* Specify accounts and OUs together in a single map, separated with a comma. For example, the following is a
* valid map: {“ACCOUNT” : [“accountID1”, “accountID2”], “ORG_UNIT” : [“ouid111”, “ouid112”]}
.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Policy withIncludeMap(java.util.Map> includeMap) {
setIncludeMap(includeMap);
return this;
}
/**
* Add a single IncludeMap entry
*
* @see Policy#withIncludeMap
* @returns a reference to this object so that method calls can be chained together.
*/
public Policy addIncludeMapEntry(String key, java.util.List value) {
if (null == this.includeMap) {
this.includeMap = new java.util.HashMap>();
}
if (this.includeMap.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.includeMap.put(key, value);
return this;
}
/**
* Removes all the entries added into IncludeMap.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Policy clearIncludeMapEntries() {
this.includeMap = null;
return this;
}
/**
*
* Specifies the Amazon Web Services account IDs and Organizations organizational units (OUs) to exclude from the
* policy. Specifying an OU is the equivalent of specifying all accounts in the OU and in any of its child OUs,
* including any child OUs and accounts that are added at a later time.
*
*
* You can specify inclusions or exclusions, but not both. If you specify an IncludeMap
, Firewall
* Manager applies the policy to all accounts specified by the IncludeMap
, and does not evaluate any
* ExcludeMap
specifications. If you do not specify an IncludeMap
, then Firewall Manager
* applies the policy to all accounts except for those specified by the ExcludeMap
.
*
*
* You can specify account IDs, OUs, or a combination:
*
*
* -
*
* Specify account IDs by setting the key to ACCOUNT
. For example, the following is a valid map:
* {“ACCOUNT” : [“accountID1”, “accountID2”]}
.
*
*
* -
*
* Specify OUs by setting the key to ORG_UNIT
. For example, the following is a valid map:
* {“ORG_UNIT” : [“ouid111”, “ouid112”]}
.
*
*
* -
*
* Specify accounts and OUs together in a single map, separated with a comma. For example, the following is a valid
* map: {“ACCOUNT” : [“accountID1”, “accountID2”], “ORG_UNIT” : [“ouid111”, “ouid112”]}
.
*
*
*
*
* @return Specifies the Amazon Web Services account IDs and Organizations organizational units (OUs) to exclude
* from the policy. Specifying an OU is the equivalent of specifying all accounts in the OU and in any of
* its child OUs, including any child OUs and accounts that are added at a later time.
*
* You can specify inclusions or exclusions, but not both. If you specify an IncludeMap
,
* Firewall Manager applies the policy to all accounts specified by the IncludeMap
, and does
* not evaluate any ExcludeMap
specifications. If you do not specify an IncludeMap
* , then Firewall Manager applies the policy to all accounts except for those specified by the
* ExcludeMap
.
*
*
* You can specify account IDs, OUs, or a combination:
*
*
* -
*
* Specify account IDs by setting the key to ACCOUNT
. For example, the following is a valid
* map: {“ACCOUNT” : [“accountID1”, “accountID2”]}
.
*
*
* -
*
* Specify OUs by setting the key to ORG_UNIT
. For example, the following is a valid map:
* {“ORG_UNIT” : [“ouid111”, “ouid112”]}
.
*
*
* -
*
* Specify accounts and OUs together in a single map, separated with a comma. For example, the following is
* a valid map: {“ACCOUNT” : [“accountID1”, “accountID2”], “ORG_UNIT” : [“ouid111”, “ouid112”]}
* .
*
*
*/
public java.util.Map> getExcludeMap() {
return excludeMap;
}
/**
*
* Specifies the Amazon Web Services account IDs and Organizations organizational units (OUs) to exclude from the
* policy. Specifying an OU is the equivalent of specifying all accounts in the OU and in any of its child OUs,
* including any child OUs and accounts that are added at a later time.
*
*
* You can specify inclusions or exclusions, but not both. If you specify an IncludeMap
, Firewall
* Manager applies the policy to all accounts specified by the IncludeMap
, and does not evaluate any
* ExcludeMap
specifications. If you do not specify an IncludeMap
, then Firewall Manager
* applies the policy to all accounts except for those specified by the ExcludeMap
.
*
*
* You can specify account IDs, OUs, or a combination:
*
*
* -
*
* Specify account IDs by setting the key to ACCOUNT
. For example, the following is a valid map:
* {“ACCOUNT” : [“accountID1”, “accountID2”]}
.
*
*
* -
*
* Specify OUs by setting the key to ORG_UNIT
. For example, the following is a valid map:
* {“ORG_UNIT” : [“ouid111”, “ouid112”]}
.
*
*
* -
*
* Specify accounts and OUs together in a single map, separated with a comma. For example, the following is a valid
* map: {“ACCOUNT” : [“accountID1”, “accountID2”], “ORG_UNIT” : [“ouid111”, “ouid112”]}
.
*
*
*
*
* @param excludeMap
* Specifies the Amazon Web Services account IDs and Organizations organizational units (OUs) to exclude from
* the policy. Specifying an OU is the equivalent of specifying all accounts in the OU and in any of its
* child OUs, including any child OUs and accounts that are added at a later time.
*
* You can specify inclusions or exclusions, but not both. If you specify an IncludeMap
,
* Firewall Manager applies the policy to all accounts specified by the IncludeMap
, and does not
* evaluate any ExcludeMap
specifications. If you do not specify an IncludeMap
,
* then Firewall Manager applies the policy to all accounts except for those specified by the
* ExcludeMap
.
*
*
* You can specify account IDs, OUs, or a combination:
*
*
* -
*
* Specify account IDs by setting the key to ACCOUNT
. For example, the following is a valid map:
* {“ACCOUNT” : [“accountID1”, “accountID2”]}
.
*
*
* -
*
* Specify OUs by setting the key to ORG_UNIT
. For example, the following is a valid map:
* {“ORG_UNIT” : [“ouid111”, “ouid112”]}
.
*
*
* -
*
* Specify accounts and OUs together in a single map, separated with a comma. For example, the following is a
* valid map: {“ACCOUNT” : [“accountID1”, “accountID2”], “ORG_UNIT” : [“ouid111”, “ouid112”]}
.
*
*
*/
public void setExcludeMap(java.util.Map> excludeMap) {
this.excludeMap = excludeMap;
}
/**
*
* Specifies the Amazon Web Services account IDs and Organizations organizational units (OUs) to exclude from the
* policy. Specifying an OU is the equivalent of specifying all accounts in the OU and in any of its child OUs,
* including any child OUs and accounts that are added at a later time.
*
*
* You can specify inclusions or exclusions, but not both. If you specify an IncludeMap
, Firewall
* Manager applies the policy to all accounts specified by the IncludeMap
, and does not evaluate any
* ExcludeMap
specifications. If you do not specify an IncludeMap
, then Firewall Manager
* applies the policy to all accounts except for those specified by the ExcludeMap
.
*
*
* You can specify account IDs, OUs, or a combination:
*
*
* -
*
* Specify account IDs by setting the key to ACCOUNT
. For example, the following is a valid map:
* {“ACCOUNT” : [“accountID1”, “accountID2”]}
.
*
*
* -
*
* Specify OUs by setting the key to ORG_UNIT
. For example, the following is a valid map:
* {“ORG_UNIT” : [“ouid111”, “ouid112”]}
.
*
*
* -
*
* Specify accounts and OUs together in a single map, separated with a comma. For example, the following is a valid
* map: {“ACCOUNT” : [“accountID1”, “accountID2”], “ORG_UNIT” : [“ouid111”, “ouid112”]}
.
*
*
*
*
* @param excludeMap
* Specifies the Amazon Web Services account IDs and Organizations organizational units (OUs) to exclude from
* the policy. Specifying an OU is the equivalent of specifying all accounts in the OU and in any of its
* child OUs, including any child OUs and accounts that are added at a later time.
*
* You can specify inclusions or exclusions, but not both. If you specify an IncludeMap
,
* Firewall Manager applies the policy to all accounts specified by the IncludeMap
, and does not
* evaluate any ExcludeMap
specifications. If you do not specify an IncludeMap
,
* then Firewall Manager applies the policy to all accounts except for those specified by the
* ExcludeMap
.
*
*
* You can specify account IDs, OUs, or a combination:
*
*
* -
*
* Specify account IDs by setting the key to ACCOUNT
. For example, the following is a valid map:
* {“ACCOUNT” : [“accountID1”, “accountID2”]}
.
*
*
* -
*
* Specify OUs by setting the key to ORG_UNIT
. For example, the following is a valid map:
* {“ORG_UNIT” : [“ouid111”, “ouid112”]}
.
*
*
* -
*
* Specify accounts and OUs together in a single map, separated with a comma. For example, the following is a
* valid map: {“ACCOUNT” : [“accountID1”, “accountID2”], “ORG_UNIT” : [“ouid111”, “ouid112”]}
.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Policy withExcludeMap(java.util.Map> excludeMap) {
setExcludeMap(excludeMap);
return this;
}
/**
* Add a single ExcludeMap entry
*
* @see Policy#withExcludeMap
* @returns a reference to this object so that method calls can be chained together.
*/
public Policy addExcludeMapEntry(String key, java.util.List value) {
if (null == this.excludeMap) {
this.excludeMap = new java.util.HashMap>();
}
if (this.excludeMap.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.excludeMap.put(key, value);
return this;
}
/**
* Removes all the entries added into ExcludeMap.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Policy clearExcludeMapEntries() {
this.excludeMap = null;
return this;
}
/**
*
* The unique identifiers of the resource sets used by the policy.
*
*
* @return The unique identifiers of the resource sets used by the policy.
*/
public java.util.List getResourceSetIds() {
return resourceSetIds;
}
/**
*
* The unique identifiers of the resource sets used by the policy.
*
*
* @param resourceSetIds
* The unique identifiers of the resource sets used by the policy.
*/
public void setResourceSetIds(java.util.Collection resourceSetIds) {
if (resourceSetIds == null) {
this.resourceSetIds = null;
return;
}
this.resourceSetIds = new java.util.ArrayList(resourceSetIds);
}
/**
*
* The unique identifiers of the resource sets used by the policy.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setResourceSetIds(java.util.Collection)} or {@link #withResourceSetIds(java.util.Collection)} if you want
* to override the existing values.
*
*
* @param resourceSetIds
* The unique identifiers of the resource sets used by the policy.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Policy withResourceSetIds(String... resourceSetIds) {
if (this.resourceSetIds == null) {
setResourceSetIds(new java.util.ArrayList(resourceSetIds.length));
}
for (String ele : resourceSetIds) {
this.resourceSetIds.add(ele);
}
return this;
}
/**
*
* The unique identifiers of the resource sets used by the policy.
*
*
* @param resourceSetIds
* The unique identifiers of the resource sets used by the policy.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Policy withResourceSetIds(java.util.Collection resourceSetIds) {
setResourceSetIds(resourceSetIds);
return this;
}
/**
*
* Your description of the Firewall Manager policy.
*
*
* @param policyDescription
* Your description of the Firewall Manager policy.
*/
public void setPolicyDescription(String policyDescription) {
this.policyDescription = policyDescription;
}
/**
*
* Your description of the Firewall Manager policy.
*
*
* @return Your description of the Firewall Manager policy.
*/
public String getPolicyDescription() {
return this.policyDescription;
}
/**
*
* Your description of the Firewall Manager policy.
*
*
* @param policyDescription
* Your description of the Firewall Manager policy.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Policy withPolicyDescription(String policyDescription) {
setPolicyDescription(policyDescription);
return this;
}
/**
*
* Indicates whether the policy is in or out of an admin's policy or Region scope.
*
*
* -
*
* ACTIVE
- The administrator can manage and delete the policy.
*
*
* -
*
* OUT_OF_ADMIN_SCOPE
- The administrator can view the policy, but they can't edit or delete the
* policy. Existing policy protections stay in place. Any new resources that come into scope of the policy won't be
* protected.
*
*
*
*
* @param policyStatus
* Indicates whether the policy is in or out of an admin's policy or Region scope.
*
* -
*
* ACTIVE
- The administrator can manage and delete the policy.
*
*
* -
*
* OUT_OF_ADMIN_SCOPE
- The administrator can view the policy, but they can't edit or delete the
* policy. Existing policy protections stay in place. Any new resources that come into scope of the policy
* won't be protected.
*
*
* @see CustomerPolicyStatus
*/
public void setPolicyStatus(String policyStatus) {
this.policyStatus = policyStatus;
}
/**
*
* Indicates whether the policy is in or out of an admin's policy or Region scope.
*
*
* -
*
* ACTIVE
- The administrator can manage and delete the policy.
*
*
* -
*
* OUT_OF_ADMIN_SCOPE
- The administrator can view the policy, but they can't edit or delete the
* policy. Existing policy protections stay in place. Any new resources that come into scope of the policy won't be
* protected.
*
*
*
*
* @return Indicates whether the policy is in or out of an admin's policy or Region scope.
*
* -
*
* ACTIVE
- The administrator can manage and delete the policy.
*
*
* -
*
* OUT_OF_ADMIN_SCOPE
- The administrator can view the policy, but they can't edit or delete
* the policy. Existing policy protections stay in place. Any new resources that come into scope of the
* policy won't be protected.
*
*
* @see CustomerPolicyStatus
*/
public String getPolicyStatus() {
return this.policyStatus;
}
/**
*
* Indicates whether the policy is in or out of an admin's policy or Region scope.
*
*
* -
*
* ACTIVE
- The administrator can manage and delete the policy.
*
*
* -
*
* OUT_OF_ADMIN_SCOPE
- The administrator can view the policy, but they can't edit or delete the
* policy. Existing policy protections stay in place. Any new resources that come into scope of the policy won't be
* protected.
*
*
*
*
* @param policyStatus
* Indicates whether the policy is in or out of an admin's policy or Region scope.
*
* -
*
* ACTIVE
- The administrator can manage and delete the policy.
*
*
* -
*
* OUT_OF_ADMIN_SCOPE
- The administrator can view the policy, but they can't edit or delete the
* policy. Existing policy protections stay in place. Any new resources that come into scope of the policy
* won't be protected.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see CustomerPolicyStatus
*/
public Policy withPolicyStatus(String policyStatus) {
setPolicyStatus(policyStatus);
return this;
}
/**
*
* Indicates whether the policy is in or out of an admin's policy or Region scope.
*
*
* -
*
* ACTIVE
- The administrator can manage and delete the policy.
*
*
* -
*
* OUT_OF_ADMIN_SCOPE
- The administrator can view the policy, but they can't edit or delete the
* policy. Existing policy protections stay in place. Any new resources that come into scope of the policy won't be
* protected.
*
*
*
*
* @param policyStatus
* Indicates whether the policy is in or out of an admin's policy or Region scope.
*
* -
*
* ACTIVE
- The administrator can manage and delete the policy.
*
*
* -
*
* OUT_OF_ADMIN_SCOPE
- The administrator can view the policy, but they can't edit or delete the
* policy. Existing policy protections stay in place. Any new resources that come into scope of the policy
* won't be protected.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see CustomerPolicyStatus
*/
public Policy withPolicyStatus(CustomerPolicyStatus policyStatus) {
this.policyStatus = policyStatus.toString();
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getPolicyId() != null)
sb.append("PolicyId: ").append(getPolicyId()).append(",");
if (getPolicyName() != null)
sb.append("PolicyName: ").append(getPolicyName()).append(",");
if (getPolicyUpdateToken() != null)
sb.append("PolicyUpdateToken: ").append(getPolicyUpdateToken()).append(",");
if (getSecurityServicePolicyData() != null)
sb.append("SecurityServicePolicyData: ").append(getSecurityServicePolicyData()).append(",");
if (getResourceType() != null)
sb.append("ResourceType: ").append(getResourceType()).append(",");
if (getResourceTypeList() != null)
sb.append("ResourceTypeList: ").append(getResourceTypeList()).append(",");
if (getResourceTags() != null)
sb.append("ResourceTags: ").append(getResourceTags()).append(",");
if (getExcludeResourceTags() != null)
sb.append("ExcludeResourceTags: ").append(getExcludeResourceTags()).append(",");
if (getRemediationEnabled() != null)
sb.append("RemediationEnabled: ").append(getRemediationEnabled()).append(",");
if (getDeleteUnusedFMManagedResources() != null)
sb.append("DeleteUnusedFMManagedResources: ").append(getDeleteUnusedFMManagedResources()).append(",");
if (getIncludeMap() != null)
sb.append("IncludeMap: ").append(getIncludeMap()).append(",");
if (getExcludeMap() != null)
sb.append("ExcludeMap: ").append(getExcludeMap()).append(",");
if (getResourceSetIds() != null)
sb.append("ResourceSetIds: ").append(getResourceSetIds()).append(",");
if (getPolicyDescription() != null)
sb.append("PolicyDescription: ").append(getPolicyDescription()).append(",");
if (getPolicyStatus() != null)
sb.append("PolicyStatus: ").append(getPolicyStatus());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof Policy == false)
return false;
Policy other = (Policy) obj;
if (other.getPolicyId() == null ^ this.getPolicyId() == null)
return false;
if (other.getPolicyId() != null && other.getPolicyId().equals(this.getPolicyId()) == false)
return false;
if (other.getPolicyName() == null ^ this.getPolicyName() == null)
return false;
if (other.getPolicyName() != null && other.getPolicyName().equals(this.getPolicyName()) == false)
return false;
if (other.getPolicyUpdateToken() == null ^ this.getPolicyUpdateToken() == null)
return false;
if (other.getPolicyUpdateToken() != null && other.getPolicyUpdateToken().equals(this.getPolicyUpdateToken()) == false)
return false;
if (other.getSecurityServicePolicyData() == null ^ this.getSecurityServicePolicyData() == null)
return false;
if (other.getSecurityServicePolicyData() != null && other.getSecurityServicePolicyData().equals(this.getSecurityServicePolicyData()) == false)
return false;
if (other.getResourceType() == null ^ this.getResourceType() == null)
return false;
if (other.getResourceType() != null && other.getResourceType().equals(this.getResourceType()) == false)
return false;
if (other.getResourceTypeList() == null ^ this.getResourceTypeList() == null)
return false;
if (other.getResourceTypeList() != null && other.getResourceTypeList().equals(this.getResourceTypeList()) == false)
return false;
if (other.getResourceTags() == null ^ this.getResourceTags() == null)
return false;
if (other.getResourceTags() != null && other.getResourceTags().equals(this.getResourceTags()) == false)
return false;
if (other.getExcludeResourceTags() == null ^ this.getExcludeResourceTags() == null)
return false;
if (other.getExcludeResourceTags() != null && other.getExcludeResourceTags().equals(this.getExcludeResourceTags()) == false)
return false;
if (other.getRemediationEnabled() == null ^ this.getRemediationEnabled() == null)
return false;
if (other.getRemediationEnabled() != null && other.getRemediationEnabled().equals(this.getRemediationEnabled()) == false)
return false;
if (other.getDeleteUnusedFMManagedResources() == null ^ this.getDeleteUnusedFMManagedResources() == null)
return false;
if (other.getDeleteUnusedFMManagedResources() != null
&& other.getDeleteUnusedFMManagedResources().equals(this.getDeleteUnusedFMManagedResources()) == false)
return false;
if (other.getIncludeMap() == null ^ this.getIncludeMap() == null)
return false;
if (other.getIncludeMap() != null && other.getIncludeMap().equals(this.getIncludeMap()) == false)
return false;
if (other.getExcludeMap() == null ^ this.getExcludeMap() == null)
return false;
if (other.getExcludeMap() != null && other.getExcludeMap().equals(this.getExcludeMap()) == false)
return false;
if (other.getResourceSetIds() == null ^ this.getResourceSetIds() == null)
return false;
if (other.getResourceSetIds() != null && other.getResourceSetIds().equals(this.getResourceSetIds()) == false)
return false;
if (other.getPolicyDescription() == null ^ this.getPolicyDescription() == null)
return false;
if (other.getPolicyDescription() != null && other.getPolicyDescription().equals(this.getPolicyDescription()) == false)
return false;
if (other.getPolicyStatus() == null ^ this.getPolicyStatus() == null)
return false;
if (other.getPolicyStatus() != null && other.getPolicyStatus().equals(this.getPolicyStatus()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getPolicyId() == null) ? 0 : getPolicyId().hashCode());
hashCode = prime * hashCode + ((getPolicyName() == null) ? 0 : getPolicyName().hashCode());
hashCode = prime * hashCode + ((getPolicyUpdateToken() == null) ? 0 : getPolicyUpdateToken().hashCode());
hashCode = prime * hashCode + ((getSecurityServicePolicyData() == null) ? 0 : getSecurityServicePolicyData().hashCode());
hashCode = prime * hashCode + ((getResourceType() == null) ? 0 : getResourceType().hashCode());
hashCode = prime * hashCode + ((getResourceTypeList() == null) ? 0 : getResourceTypeList().hashCode());
hashCode = prime * hashCode + ((getResourceTags() == null) ? 0 : getResourceTags().hashCode());
hashCode = prime * hashCode + ((getExcludeResourceTags() == null) ? 0 : getExcludeResourceTags().hashCode());
hashCode = prime * hashCode + ((getRemediationEnabled() == null) ? 0 : getRemediationEnabled().hashCode());
hashCode = prime * hashCode + ((getDeleteUnusedFMManagedResources() == null) ? 0 : getDeleteUnusedFMManagedResources().hashCode());
hashCode = prime * hashCode + ((getIncludeMap() == null) ? 0 : getIncludeMap().hashCode());
hashCode = prime * hashCode + ((getExcludeMap() == null) ? 0 : getExcludeMap().hashCode());
hashCode = prime * hashCode + ((getResourceSetIds() == null) ? 0 : getResourceSetIds().hashCode());
hashCode = prime * hashCode + ((getPolicyDescription() == null) ? 0 : getPolicyDescription().hashCode());
hashCode = prime * hashCode + ((getPolicyStatus() == null) ? 0 : getPolicyStatus().hashCode());
return hashCode;
}
@Override
public Policy clone() {
try {
return (Policy) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.fms.model.transform.PolicyMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}