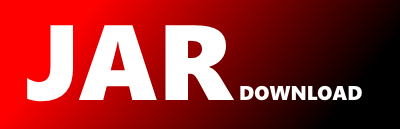
com.amazonaws.services.fms.model.PolicyComplianceDetail Maven / Gradle / Ivy
Show all versions of aws-java-sdk-fms Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.fms.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Describes the noncompliant resources in a member account for a specific Firewall Manager policy. A maximum of 100
* entries are displayed. If more than 100 resources are noncompliant, EvaluationLimitExceeded
is set to
* True
.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class PolicyComplianceDetail implements Serializable, Cloneable, StructuredPojo {
/**
*
* The Amazon Web Services account that created the Firewall Manager policy.
*
*/
private String policyOwner;
/**
*
* The ID of the Firewall Manager policy.
*
*/
private String policyId;
/**
*
* The Amazon Web Services account ID.
*
*/
private String memberAccount;
/**
*
* An array of resources that aren't protected by the WAF or Shield Advanced policy or that aren't in compliance
* with the security group policy.
*
*/
private java.util.List violators;
/**
*
* Indicates if over 100 resources are noncompliant with the Firewall Manager policy.
*
*/
private Boolean evaluationLimitExceeded;
/**
*
* A timestamp that indicates when the returned information should be considered out of date.
*
*/
private java.util.Date expiredAt;
/**
*
* Details about problems with dependent services, such as WAF or Config, and the error message received that
* indicates the problem with the service.
*
*/
private java.util.Map issueInfoMap;
/**
*
* The Amazon Web Services account that created the Firewall Manager policy.
*
*
* @param policyOwner
* The Amazon Web Services account that created the Firewall Manager policy.
*/
public void setPolicyOwner(String policyOwner) {
this.policyOwner = policyOwner;
}
/**
*
* The Amazon Web Services account that created the Firewall Manager policy.
*
*
* @return The Amazon Web Services account that created the Firewall Manager policy.
*/
public String getPolicyOwner() {
return this.policyOwner;
}
/**
*
* The Amazon Web Services account that created the Firewall Manager policy.
*
*
* @param policyOwner
* The Amazon Web Services account that created the Firewall Manager policy.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PolicyComplianceDetail withPolicyOwner(String policyOwner) {
setPolicyOwner(policyOwner);
return this;
}
/**
*
* The ID of the Firewall Manager policy.
*
*
* @param policyId
* The ID of the Firewall Manager policy.
*/
public void setPolicyId(String policyId) {
this.policyId = policyId;
}
/**
*
* The ID of the Firewall Manager policy.
*
*
* @return The ID of the Firewall Manager policy.
*/
public String getPolicyId() {
return this.policyId;
}
/**
*
* The ID of the Firewall Manager policy.
*
*
* @param policyId
* The ID of the Firewall Manager policy.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PolicyComplianceDetail withPolicyId(String policyId) {
setPolicyId(policyId);
return this;
}
/**
*
* The Amazon Web Services account ID.
*
*
* @param memberAccount
* The Amazon Web Services account ID.
*/
public void setMemberAccount(String memberAccount) {
this.memberAccount = memberAccount;
}
/**
*
* The Amazon Web Services account ID.
*
*
* @return The Amazon Web Services account ID.
*/
public String getMemberAccount() {
return this.memberAccount;
}
/**
*
* The Amazon Web Services account ID.
*
*
* @param memberAccount
* The Amazon Web Services account ID.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PolicyComplianceDetail withMemberAccount(String memberAccount) {
setMemberAccount(memberAccount);
return this;
}
/**
*
* An array of resources that aren't protected by the WAF or Shield Advanced policy or that aren't in compliance
* with the security group policy.
*
*
* @return An array of resources that aren't protected by the WAF or Shield Advanced policy or that aren't in
* compliance with the security group policy.
*/
public java.util.List getViolators() {
return violators;
}
/**
*
* An array of resources that aren't protected by the WAF or Shield Advanced policy or that aren't in compliance
* with the security group policy.
*
*
* @param violators
* An array of resources that aren't protected by the WAF or Shield Advanced policy or that aren't in
* compliance with the security group policy.
*/
public void setViolators(java.util.Collection violators) {
if (violators == null) {
this.violators = null;
return;
}
this.violators = new java.util.ArrayList(violators);
}
/**
*
* An array of resources that aren't protected by the WAF or Shield Advanced policy or that aren't in compliance
* with the security group policy.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setViolators(java.util.Collection)} or {@link #withViolators(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param violators
* An array of resources that aren't protected by the WAF or Shield Advanced policy or that aren't in
* compliance with the security group policy.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PolicyComplianceDetail withViolators(ComplianceViolator... violators) {
if (this.violators == null) {
setViolators(new java.util.ArrayList(violators.length));
}
for (ComplianceViolator ele : violators) {
this.violators.add(ele);
}
return this;
}
/**
*
* An array of resources that aren't protected by the WAF or Shield Advanced policy or that aren't in compliance
* with the security group policy.
*
*
* @param violators
* An array of resources that aren't protected by the WAF or Shield Advanced policy or that aren't in
* compliance with the security group policy.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PolicyComplianceDetail withViolators(java.util.Collection violators) {
setViolators(violators);
return this;
}
/**
*
* Indicates if over 100 resources are noncompliant with the Firewall Manager policy.
*
*
* @param evaluationLimitExceeded
* Indicates if over 100 resources are noncompliant with the Firewall Manager policy.
*/
public void setEvaluationLimitExceeded(Boolean evaluationLimitExceeded) {
this.evaluationLimitExceeded = evaluationLimitExceeded;
}
/**
*
* Indicates if over 100 resources are noncompliant with the Firewall Manager policy.
*
*
* @return Indicates if over 100 resources are noncompliant with the Firewall Manager policy.
*/
public Boolean getEvaluationLimitExceeded() {
return this.evaluationLimitExceeded;
}
/**
*
* Indicates if over 100 resources are noncompliant with the Firewall Manager policy.
*
*
* @param evaluationLimitExceeded
* Indicates if over 100 resources are noncompliant with the Firewall Manager policy.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PolicyComplianceDetail withEvaluationLimitExceeded(Boolean evaluationLimitExceeded) {
setEvaluationLimitExceeded(evaluationLimitExceeded);
return this;
}
/**
*
* Indicates if over 100 resources are noncompliant with the Firewall Manager policy.
*
*
* @return Indicates if over 100 resources are noncompliant with the Firewall Manager policy.
*/
public Boolean isEvaluationLimitExceeded() {
return this.evaluationLimitExceeded;
}
/**
*
* A timestamp that indicates when the returned information should be considered out of date.
*
*
* @param expiredAt
* A timestamp that indicates when the returned information should be considered out of date.
*/
public void setExpiredAt(java.util.Date expiredAt) {
this.expiredAt = expiredAt;
}
/**
*
* A timestamp that indicates when the returned information should be considered out of date.
*
*
* @return A timestamp that indicates when the returned information should be considered out of date.
*/
public java.util.Date getExpiredAt() {
return this.expiredAt;
}
/**
*
* A timestamp that indicates when the returned information should be considered out of date.
*
*
* @param expiredAt
* A timestamp that indicates when the returned information should be considered out of date.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PolicyComplianceDetail withExpiredAt(java.util.Date expiredAt) {
setExpiredAt(expiredAt);
return this;
}
/**
*
* Details about problems with dependent services, such as WAF or Config, and the error message received that
* indicates the problem with the service.
*
*
* @return Details about problems with dependent services, such as WAF or Config, and the error message received
* that indicates the problem with the service.
*/
public java.util.Map getIssueInfoMap() {
return issueInfoMap;
}
/**
*
* Details about problems with dependent services, such as WAF or Config, and the error message received that
* indicates the problem with the service.
*
*
* @param issueInfoMap
* Details about problems with dependent services, such as WAF or Config, and the error message received that
* indicates the problem with the service.
*/
public void setIssueInfoMap(java.util.Map issueInfoMap) {
this.issueInfoMap = issueInfoMap;
}
/**
*
* Details about problems with dependent services, such as WAF or Config, and the error message received that
* indicates the problem with the service.
*
*
* @param issueInfoMap
* Details about problems with dependent services, such as WAF or Config, and the error message received that
* indicates the problem with the service.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PolicyComplianceDetail withIssueInfoMap(java.util.Map issueInfoMap) {
setIssueInfoMap(issueInfoMap);
return this;
}
/**
* Add a single IssueInfoMap entry
*
* @see PolicyComplianceDetail#withIssueInfoMap
* @returns a reference to this object so that method calls can be chained together.
*/
public PolicyComplianceDetail addIssueInfoMapEntry(String key, String value) {
if (null == this.issueInfoMap) {
this.issueInfoMap = new java.util.HashMap();
}
if (this.issueInfoMap.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.issueInfoMap.put(key, value);
return this;
}
/**
* Removes all the entries added into IssueInfoMap.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PolicyComplianceDetail clearIssueInfoMapEntries() {
this.issueInfoMap = null;
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getPolicyOwner() != null)
sb.append("PolicyOwner: ").append(getPolicyOwner()).append(",");
if (getPolicyId() != null)
sb.append("PolicyId: ").append(getPolicyId()).append(",");
if (getMemberAccount() != null)
sb.append("MemberAccount: ").append(getMemberAccount()).append(",");
if (getViolators() != null)
sb.append("Violators: ").append(getViolators()).append(",");
if (getEvaluationLimitExceeded() != null)
sb.append("EvaluationLimitExceeded: ").append(getEvaluationLimitExceeded()).append(",");
if (getExpiredAt() != null)
sb.append("ExpiredAt: ").append(getExpiredAt()).append(",");
if (getIssueInfoMap() != null)
sb.append("IssueInfoMap: ").append(getIssueInfoMap());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof PolicyComplianceDetail == false)
return false;
PolicyComplianceDetail other = (PolicyComplianceDetail) obj;
if (other.getPolicyOwner() == null ^ this.getPolicyOwner() == null)
return false;
if (other.getPolicyOwner() != null && other.getPolicyOwner().equals(this.getPolicyOwner()) == false)
return false;
if (other.getPolicyId() == null ^ this.getPolicyId() == null)
return false;
if (other.getPolicyId() != null && other.getPolicyId().equals(this.getPolicyId()) == false)
return false;
if (other.getMemberAccount() == null ^ this.getMemberAccount() == null)
return false;
if (other.getMemberAccount() != null && other.getMemberAccount().equals(this.getMemberAccount()) == false)
return false;
if (other.getViolators() == null ^ this.getViolators() == null)
return false;
if (other.getViolators() != null && other.getViolators().equals(this.getViolators()) == false)
return false;
if (other.getEvaluationLimitExceeded() == null ^ this.getEvaluationLimitExceeded() == null)
return false;
if (other.getEvaluationLimitExceeded() != null && other.getEvaluationLimitExceeded().equals(this.getEvaluationLimitExceeded()) == false)
return false;
if (other.getExpiredAt() == null ^ this.getExpiredAt() == null)
return false;
if (other.getExpiredAt() != null && other.getExpiredAt().equals(this.getExpiredAt()) == false)
return false;
if (other.getIssueInfoMap() == null ^ this.getIssueInfoMap() == null)
return false;
if (other.getIssueInfoMap() != null && other.getIssueInfoMap().equals(this.getIssueInfoMap()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getPolicyOwner() == null) ? 0 : getPolicyOwner().hashCode());
hashCode = prime * hashCode + ((getPolicyId() == null) ? 0 : getPolicyId().hashCode());
hashCode = prime * hashCode + ((getMemberAccount() == null) ? 0 : getMemberAccount().hashCode());
hashCode = prime * hashCode + ((getViolators() == null) ? 0 : getViolators().hashCode());
hashCode = prime * hashCode + ((getEvaluationLimitExceeded() == null) ? 0 : getEvaluationLimitExceeded().hashCode());
hashCode = prime * hashCode + ((getExpiredAt() == null) ? 0 : getExpiredAt().hashCode());
hashCode = prime * hashCode + ((getIssueInfoMap() == null) ? 0 : getIssueInfoMap().hashCode());
return hashCode;
}
@Override
public PolicyComplianceDetail clone() {
try {
return (PolicyComplianceDetail) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.fms.model.transform.PolicyComplianceDetailMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}