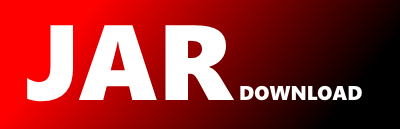
com.amazonaws.services.fms.model.PolicySummary Maven / Gradle / Ivy
Show all versions of aws-java-sdk-fms Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.fms.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Details of the Firewall Manager policy.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class PolicySummary implements Serializable, Cloneable, StructuredPojo {
/**
*
* The Amazon Resource Name (ARN) of the specified policy.
*
*/
private String policyArn;
/**
*
* The ID of the specified policy.
*
*/
private String policyId;
/**
*
* The name of the specified policy.
*
*/
private String policyName;
/**
*
* The type of resource protected by or in scope of the policy. This is in the format shown in the Amazon
* Web Services Resource Types Reference.
*
*/
private String resourceType;
/**
*
* The service that the policy is using to protect the resources. This specifies the type of policy that is created,
* either an WAF policy, a Shield Advanced policy, or a security group policy.
*
*/
private String securityServiceType;
/**
*
* Indicates if the policy should be automatically applied to new resources.
*
*/
private Boolean remediationEnabled;
/**
*
* Indicates whether Firewall Manager should automatically remove protections from resources that leave the policy
* scope and clean up resources that Firewall Manager is managing for accounts when those accounts leave policy
* scope. For example, Firewall Manager will disassociate a Firewall Manager managed web ACL from a protected
* customer resource when the customer resource leaves policy scope.
*
*
* By default, Firewall Manager doesn't remove protections or delete Firewall Manager managed resources.
*
*
* This option is not available for Shield Advanced or WAF Classic policies.
*
*/
private Boolean deleteUnusedFMManagedResources;
/**
*
* Indicates whether the policy is in or out of an admin's policy or Region scope.
*
*
* -
*
* ACTIVE
- The administrator can manage and delete the policy.
*
*
* -
*
* OUT_OF_ADMIN_SCOPE
- The administrator can view the policy, but they can't edit or delete the
* policy. Existing policy protections stay in place. Any new resources that come into scope of the policy won't be
* protected.
*
*
*
*/
private String policyStatus;
/**
*
* The Amazon Resource Name (ARN) of the specified policy.
*
*
* @param policyArn
* The Amazon Resource Name (ARN) of the specified policy.
*/
public void setPolicyArn(String policyArn) {
this.policyArn = policyArn;
}
/**
*
* The Amazon Resource Name (ARN) of the specified policy.
*
*
* @return The Amazon Resource Name (ARN) of the specified policy.
*/
public String getPolicyArn() {
return this.policyArn;
}
/**
*
* The Amazon Resource Name (ARN) of the specified policy.
*
*
* @param policyArn
* The Amazon Resource Name (ARN) of the specified policy.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PolicySummary withPolicyArn(String policyArn) {
setPolicyArn(policyArn);
return this;
}
/**
*
* The ID of the specified policy.
*
*
* @param policyId
* The ID of the specified policy.
*/
public void setPolicyId(String policyId) {
this.policyId = policyId;
}
/**
*
* The ID of the specified policy.
*
*
* @return The ID of the specified policy.
*/
public String getPolicyId() {
return this.policyId;
}
/**
*
* The ID of the specified policy.
*
*
* @param policyId
* The ID of the specified policy.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PolicySummary withPolicyId(String policyId) {
setPolicyId(policyId);
return this;
}
/**
*
* The name of the specified policy.
*
*
* @param policyName
* The name of the specified policy.
*/
public void setPolicyName(String policyName) {
this.policyName = policyName;
}
/**
*
* The name of the specified policy.
*
*
* @return The name of the specified policy.
*/
public String getPolicyName() {
return this.policyName;
}
/**
*
* The name of the specified policy.
*
*
* @param policyName
* The name of the specified policy.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PolicySummary withPolicyName(String policyName) {
setPolicyName(policyName);
return this;
}
/**
*
* The type of resource protected by or in scope of the policy. This is in the format shown in the Amazon
* Web Services Resource Types Reference.
*
*
* @param resourceType
* The type of resource protected by or in scope of the policy. This is in the format shown in the Amazon Web Services Resource Types Reference.
*/
public void setResourceType(String resourceType) {
this.resourceType = resourceType;
}
/**
*
* The type of resource protected by or in scope of the policy. This is in the format shown in the Amazon
* Web Services Resource Types Reference.
*
*
* @return The type of resource protected by or in scope of the policy. This is in the format shown in the Amazon Web Services Resource Types Reference.
*/
public String getResourceType() {
return this.resourceType;
}
/**
*
* The type of resource protected by or in scope of the policy. This is in the format shown in the Amazon
* Web Services Resource Types Reference.
*
*
* @param resourceType
* The type of resource protected by or in scope of the policy. This is in the format shown in the Amazon Web Services Resource Types Reference.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PolicySummary withResourceType(String resourceType) {
setResourceType(resourceType);
return this;
}
/**
*
* The service that the policy is using to protect the resources. This specifies the type of policy that is created,
* either an WAF policy, a Shield Advanced policy, or a security group policy.
*
*
* @param securityServiceType
* The service that the policy is using to protect the resources. This specifies the type of policy that is
* created, either an WAF policy, a Shield Advanced policy, or a security group policy.
* @see SecurityServiceType
*/
public void setSecurityServiceType(String securityServiceType) {
this.securityServiceType = securityServiceType;
}
/**
*
* The service that the policy is using to protect the resources. This specifies the type of policy that is created,
* either an WAF policy, a Shield Advanced policy, or a security group policy.
*
*
* @return The service that the policy is using to protect the resources. This specifies the type of policy that is
* created, either an WAF policy, a Shield Advanced policy, or a security group policy.
* @see SecurityServiceType
*/
public String getSecurityServiceType() {
return this.securityServiceType;
}
/**
*
* The service that the policy is using to protect the resources. This specifies the type of policy that is created,
* either an WAF policy, a Shield Advanced policy, or a security group policy.
*
*
* @param securityServiceType
* The service that the policy is using to protect the resources. This specifies the type of policy that is
* created, either an WAF policy, a Shield Advanced policy, or a security group policy.
* @return Returns a reference to this object so that method calls can be chained together.
* @see SecurityServiceType
*/
public PolicySummary withSecurityServiceType(String securityServiceType) {
setSecurityServiceType(securityServiceType);
return this;
}
/**
*
* The service that the policy is using to protect the resources. This specifies the type of policy that is created,
* either an WAF policy, a Shield Advanced policy, or a security group policy.
*
*
* @param securityServiceType
* The service that the policy is using to protect the resources. This specifies the type of policy that is
* created, either an WAF policy, a Shield Advanced policy, or a security group policy.
* @return Returns a reference to this object so that method calls can be chained together.
* @see SecurityServiceType
*/
public PolicySummary withSecurityServiceType(SecurityServiceType securityServiceType) {
this.securityServiceType = securityServiceType.toString();
return this;
}
/**
*
* Indicates if the policy should be automatically applied to new resources.
*
*
* @param remediationEnabled
* Indicates if the policy should be automatically applied to new resources.
*/
public void setRemediationEnabled(Boolean remediationEnabled) {
this.remediationEnabled = remediationEnabled;
}
/**
*
* Indicates if the policy should be automatically applied to new resources.
*
*
* @return Indicates if the policy should be automatically applied to new resources.
*/
public Boolean getRemediationEnabled() {
return this.remediationEnabled;
}
/**
*
* Indicates if the policy should be automatically applied to new resources.
*
*
* @param remediationEnabled
* Indicates if the policy should be automatically applied to new resources.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PolicySummary withRemediationEnabled(Boolean remediationEnabled) {
setRemediationEnabled(remediationEnabled);
return this;
}
/**
*
* Indicates if the policy should be automatically applied to new resources.
*
*
* @return Indicates if the policy should be automatically applied to new resources.
*/
public Boolean isRemediationEnabled() {
return this.remediationEnabled;
}
/**
*
* Indicates whether Firewall Manager should automatically remove protections from resources that leave the policy
* scope and clean up resources that Firewall Manager is managing for accounts when those accounts leave policy
* scope. For example, Firewall Manager will disassociate a Firewall Manager managed web ACL from a protected
* customer resource when the customer resource leaves policy scope.
*
*
* By default, Firewall Manager doesn't remove protections or delete Firewall Manager managed resources.
*
*
* This option is not available for Shield Advanced or WAF Classic policies.
*
*
* @param deleteUnusedFMManagedResources
* Indicates whether Firewall Manager should automatically remove protections from resources that leave the
* policy scope and clean up resources that Firewall Manager is managing for accounts when those accounts
* leave policy scope. For example, Firewall Manager will disassociate a Firewall Manager managed web ACL
* from a protected customer resource when the customer resource leaves policy scope.
*
* By default, Firewall Manager doesn't remove protections or delete Firewall Manager managed resources.
*
*
* This option is not available for Shield Advanced or WAF Classic policies.
*/
public void setDeleteUnusedFMManagedResources(Boolean deleteUnusedFMManagedResources) {
this.deleteUnusedFMManagedResources = deleteUnusedFMManagedResources;
}
/**
*
* Indicates whether Firewall Manager should automatically remove protections from resources that leave the policy
* scope and clean up resources that Firewall Manager is managing for accounts when those accounts leave policy
* scope. For example, Firewall Manager will disassociate a Firewall Manager managed web ACL from a protected
* customer resource when the customer resource leaves policy scope.
*
*
* By default, Firewall Manager doesn't remove protections or delete Firewall Manager managed resources.
*
*
* This option is not available for Shield Advanced or WAF Classic policies.
*
*
* @return Indicates whether Firewall Manager should automatically remove protections from resources that leave the
* policy scope and clean up resources that Firewall Manager is managing for accounts when those accounts
* leave policy scope. For example, Firewall Manager will disassociate a Firewall Manager managed web ACL
* from a protected customer resource when the customer resource leaves policy scope.
*
* By default, Firewall Manager doesn't remove protections or delete Firewall Manager managed resources.
*
*
* This option is not available for Shield Advanced or WAF Classic policies.
*/
public Boolean getDeleteUnusedFMManagedResources() {
return this.deleteUnusedFMManagedResources;
}
/**
*
* Indicates whether Firewall Manager should automatically remove protections from resources that leave the policy
* scope and clean up resources that Firewall Manager is managing for accounts when those accounts leave policy
* scope. For example, Firewall Manager will disassociate a Firewall Manager managed web ACL from a protected
* customer resource when the customer resource leaves policy scope.
*
*
* By default, Firewall Manager doesn't remove protections or delete Firewall Manager managed resources.
*
*
* This option is not available for Shield Advanced or WAF Classic policies.
*
*
* @param deleteUnusedFMManagedResources
* Indicates whether Firewall Manager should automatically remove protections from resources that leave the
* policy scope and clean up resources that Firewall Manager is managing for accounts when those accounts
* leave policy scope. For example, Firewall Manager will disassociate a Firewall Manager managed web ACL
* from a protected customer resource when the customer resource leaves policy scope.
*
* By default, Firewall Manager doesn't remove protections or delete Firewall Manager managed resources.
*
*
* This option is not available for Shield Advanced or WAF Classic policies.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PolicySummary withDeleteUnusedFMManagedResources(Boolean deleteUnusedFMManagedResources) {
setDeleteUnusedFMManagedResources(deleteUnusedFMManagedResources);
return this;
}
/**
*
* Indicates whether Firewall Manager should automatically remove protections from resources that leave the policy
* scope and clean up resources that Firewall Manager is managing for accounts when those accounts leave policy
* scope. For example, Firewall Manager will disassociate a Firewall Manager managed web ACL from a protected
* customer resource when the customer resource leaves policy scope.
*
*
* By default, Firewall Manager doesn't remove protections or delete Firewall Manager managed resources.
*
*
* This option is not available for Shield Advanced or WAF Classic policies.
*
*
* @return Indicates whether Firewall Manager should automatically remove protections from resources that leave the
* policy scope and clean up resources that Firewall Manager is managing for accounts when those accounts
* leave policy scope. For example, Firewall Manager will disassociate a Firewall Manager managed web ACL
* from a protected customer resource when the customer resource leaves policy scope.
*
* By default, Firewall Manager doesn't remove protections or delete Firewall Manager managed resources.
*
*
* This option is not available for Shield Advanced or WAF Classic policies.
*/
public Boolean isDeleteUnusedFMManagedResources() {
return this.deleteUnusedFMManagedResources;
}
/**
*
* Indicates whether the policy is in or out of an admin's policy or Region scope.
*
*
* -
*
* ACTIVE
- The administrator can manage and delete the policy.
*
*
* -
*
* OUT_OF_ADMIN_SCOPE
- The administrator can view the policy, but they can't edit or delete the
* policy. Existing policy protections stay in place. Any new resources that come into scope of the policy won't be
* protected.
*
*
*
*
* @param policyStatus
* Indicates whether the policy is in or out of an admin's policy or Region scope.
*
* -
*
* ACTIVE
- The administrator can manage and delete the policy.
*
*
* -
*
* OUT_OF_ADMIN_SCOPE
- The administrator can view the policy, but they can't edit or delete the
* policy. Existing policy protections stay in place. Any new resources that come into scope of the policy
* won't be protected.
*
*
* @see CustomerPolicyStatus
*/
public void setPolicyStatus(String policyStatus) {
this.policyStatus = policyStatus;
}
/**
*
* Indicates whether the policy is in or out of an admin's policy or Region scope.
*
*
* -
*
* ACTIVE
- The administrator can manage and delete the policy.
*
*
* -
*
* OUT_OF_ADMIN_SCOPE
- The administrator can view the policy, but they can't edit or delete the
* policy. Existing policy protections stay in place. Any new resources that come into scope of the policy won't be
* protected.
*
*
*
*
* @return Indicates whether the policy is in or out of an admin's policy or Region scope.
*
* -
*
* ACTIVE
- The administrator can manage and delete the policy.
*
*
* -
*
* OUT_OF_ADMIN_SCOPE
- The administrator can view the policy, but they can't edit or delete
* the policy. Existing policy protections stay in place. Any new resources that come into scope of the
* policy won't be protected.
*
*
* @see CustomerPolicyStatus
*/
public String getPolicyStatus() {
return this.policyStatus;
}
/**
*
* Indicates whether the policy is in or out of an admin's policy or Region scope.
*
*
* -
*
* ACTIVE
- The administrator can manage and delete the policy.
*
*
* -
*
* OUT_OF_ADMIN_SCOPE
- The administrator can view the policy, but they can't edit or delete the
* policy. Existing policy protections stay in place. Any new resources that come into scope of the policy won't be
* protected.
*
*
*
*
* @param policyStatus
* Indicates whether the policy is in or out of an admin's policy or Region scope.
*
* -
*
* ACTIVE
- The administrator can manage and delete the policy.
*
*
* -
*
* OUT_OF_ADMIN_SCOPE
- The administrator can view the policy, but they can't edit or delete the
* policy. Existing policy protections stay in place. Any new resources that come into scope of the policy
* won't be protected.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see CustomerPolicyStatus
*/
public PolicySummary withPolicyStatus(String policyStatus) {
setPolicyStatus(policyStatus);
return this;
}
/**
*
* Indicates whether the policy is in or out of an admin's policy or Region scope.
*
*
* -
*
* ACTIVE
- The administrator can manage and delete the policy.
*
*
* -
*
* OUT_OF_ADMIN_SCOPE
- The administrator can view the policy, but they can't edit or delete the
* policy. Existing policy protections stay in place. Any new resources that come into scope of the policy won't be
* protected.
*
*
*
*
* @param policyStatus
* Indicates whether the policy is in or out of an admin's policy or Region scope.
*
* -
*
* ACTIVE
- The administrator can manage and delete the policy.
*
*
* -
*
* OUT_OF_ADMIN_SCOPE
- The administrator can view the policy, but they can't edit or delete the
* policy. Existing policy protections stay in place. Any new resources that come into scope of the policy
* won't be protected.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see CustomerPolicyStatus
*/
public PolicySummary withPolicyStatus(CustomerPolicyStatus policyStatus) {
this.policyStatus = policyStatus.toString();
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getPolicyArn() != null)
sb.append("PolicyArn: ").append(getPolicyArn()).append(",");
if (getPolicyId() != null)
sb.append("PolicyId: ").append(getPolicyId()).append(",");
if (getPolicyName() != null)
sb.append("PolicyName: ").append(getPolicyName()).append(",");
if (getResourceType() != null)
sb.append("ResourceType: ").append(getResourceType()).append(",");
if (getSecurityServiceType() != null)
sb.append("SecurityServiceType: ").append(getSecurityServiceType()).append(",");
if (getRemediationEnabled() != null)
sb.append("RemediationEnabled: ").append(getRemediationEnabled()).append(",");
if (getDeleteUnusedFMManagedResources() != null)
sb.append("DeleteUnusedFMManagedResources: ").append(getDeleteUnusedFMManagedResources()).append(",");
if (getPolicyStatus() != null)
sb.append("PolicyStatus: ").append(getPolicyStatus());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof PolicySummary == false)
return false;
PolicySummary other = (PolicySummary) obj;
if (other.getPolicyArn() == null ^ this.getPolicyArn() == null)
return false;
if (other.getPolicyArn() != null && other.getPolicyArn().equals(this.getPolicyArn()) == false)
return false;
if (other.getPolicyId() == null ^ this.getPolicyId() == null)
return false;
if (other.getPolicyId() != null && other.getPolicyId().equals(this.getPolicyId()) == false)
return false;
if (other.getPolicyName() == null ^ this.getPolicyName() == null)
return false;
if (other.getPolicyName() != null && other.getPolicyName().equals(this.getPolicyName()) == false)
return false;
if (other.getResourceType() == null ^ this.getResourceType() == null)
return false;
if (other.getResourceType() != null && other.getResourceType().equals(this.getResourceType()) == false)
return false;
if (other.getSecurityServiceType() == null ^ this.getSecurityServiceType() == null)
return false;
if (other.getSecurityServiceType() != null && other.getSecurityServiceType().equals(this.getSecurityServiceType()) == false)
return false;
if (other.getRemediationEnabled() == null ^ this.getRemediationEnabled() == null)
return false;
if (other.getRemediationEnabled() != null && other.getRemediationEnabled().equals(this.getRemediationEnabled()) == false)
return false;
if (other.getDeleteUnusedFMManagedResources() == null ^ this.getDeleteUnusedFMManagedResources() == null)
return false;
if (other.getDeleteUnusedFMManagedResources() != null
&& other.getDeleteUnusedFMManagedResources().equals(this.getDeleteUnusedFMManagedResources()) == false)
return false;
if (other.getPolicyStatus() == null ^ this.getPolicyStatus() == null)
return false;
if (other.getPolicyStatus() != null && other.getPolicyStatus().equals(this.getPolicyStatus()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getPolicyArn() == null) ? 0 : getPolicyArn().hashCode());
hashCode = prime * hashCode + ((getPolicyId() == null) ? 0 : getPolicyId().hashCode());
hashCode = prime * hashCode + ((getPolicyName() == null) ? 0 : getPolicyName().hashCode());
hashCode = prime * hashCode + ((getResourceType() == null) ? 0 : getResourceType().hashCode());
hashCode = prime * hashCode + ((getSecurityServiceType() == null) ? 0 : getSecurityServiceType().hashCode());
hashCode = prime * hashCode + ((getRemediationEnabled() == null) ? 0 : getRemediationEnabled().hashCode());
hashCode = prime * hashCode + ((getDeleteUnusedFMManagedResources() == null) ? 0 : getDeleteUnusedFMManagedResources().hashCode());
hashCode = prime * hashCode + ((getPolicyStatus() == null) ? 0 : getPolicyStatus().hashCode());
return hashCode;
}
@Override
public PolicySummary clone() {
try {
return (PolicySummary) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.fms.model.transform.PolicySummaryMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}