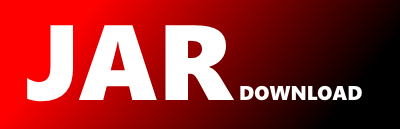
com.amazonaws.services.forecast.model.FeaturizationConfig Maven / Gradle / Ivy
Show all versions of aws-java-sdk-forecast Show documentation
/*
* Copyright 2015-2020 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.forecast.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* In a CreatePredictor operation, the specified algorithm trains a model using the specified dataset group. You
* can optionally tell the operation to modify data fields prior to training a model. These modifications are referred
* to as featurization.
*
*
* You define featurization using the FeaturizationConfig
object. You specify an array of transformations,
* one for each field that you want to featurize. You then include the FeaturizationConfig
object in your
* CreatePredictor
request. Amazon Forecast applies the featurization to the
* TARGET_TIME_SERIES
dataset before model training.
*
*
* You can create multiple featurization configurations. For example, you might call the CreatePredictor
* operation twice by specifying different featurization configurations.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class FeaturizationConfig implements Serializable, Cloneable, StructuredPojo {
/**
*
* The frequency of predictions in a forecast.
*
*
* Valid intervals are Y (Year), M (Month), W (Week), D (Day), H (Hour), 30min (30 minutes), 15min (15 minutes),
* 10min (10 minutes), 5min (5 minutes), and 1min (1 minute). For example, "Y" indicates every year and "5min"
* indicates every five minutes.
*
*
* The frequency must be greater than or equal to the TARGET_TIME_SERIES dataset frequency.
*
*
* When a RELATED_TIME_SERIES dataset is provided, the frequency must be equal to the RELATED_TIME_SERIES dataset
* frequency.
*
*/
private String forecastFrequency;
/**
*
* An array of dimension (field) names that specify how to group the generated forecast.
*
*
* For example, suppose that you are generating a forecast for item sales across all of your stores, and your
* dataset contains a store_id
field. If you want the sales forecast for each item by store, you would
* specify store_id
as the dimension.
*
*
* All forecast dimensions specified in the TARGET_TIME_SERIES
dataset don't need to be specified in
* the CreatePredictor
request. All forecast dimensions specified in the
* RELATED_TIME_SERIES
dataset must be specified in the CreatePredictor
request.
*
*/
private java.util.List forecastDimensions;
/**
*
* An array of featurization (transformation) information for the fields of a dataset. Only a single featurization
* is supported.
*
*/
private java.util.List featurizations;
/**
*
* The frequency of predictions in a forecast.
*
*
* Valid intervals are Y (Year), M (Month), W (Week), D (Day), H (Hour), 30min (30 minutes), 15min (15 minutes),
* 10min (10 minutes), 5min (5 minutes), and 1min (1 minute). For example, "Y" indicates every year and "5min"
* indicates every five minutes.
*
*
* The frequency must be greater than or equal to the TARGET_TIME_SERIES dataset frequency.
*
*
* When a RELATED_TIME_SERIES dataset is provided, the frequency must be equal to the RELATED_TIME_SERIES dataset
* frequency.
*
*
* @param forecastFrequency
* The frequency of predictions in a forecast.
*
* Valid intervals are Y (Year), M (Month), W (Week), D (Day), H (Hour), 30min (30 minutes), 15min (15
* minutes), 10min (10 minutes), 5min (5 minutes), and 1min (1 minute). For example, "Y" indicates every year
* and "5min" indicates every five minutes.
*
*
* The frequency must be greater than or equal to the TARGET_TIME_SERIES dataset frequency.
*
*
* When a RELATED_TIME_SERIES dataset is provided, the frequency must be equal to the RELATED_TIME_SERIES
* dataset frequency.
*/
public void setForecastFrequency(String forecastFrequency) {
this.forecastFrequency = forecastFrequency;
}
/**
*
* The frequency of predictions in a forecast.
*
*
* Valid intervals are Y (Year), M (Month), W (Week), D (Day), H (Hour), 30min (30 minutes), 15min (15 minutes),
* 10min (10 minutes), 5min (5 minutes), and 1min (1 minute). For example, "Y" indicates every year and "5min"
* indicates every five minutes.
*
*
* The frequency must be greater than or equal to the TARGET_TIME_SERIES dataset frequency.
*
*
* When a RELATED_TIME_SERIES dataset is provided, the frequency must be equal to the RELATED_TIME_SERIES dataset
* frequency.
*
*
* @return The frequency of predictions in a forecast.
*
* Valid intervals are Y (Year), M (Month), W (Week), D (Day), H (Hour), 30min (30 minutes), 15min (15
* minutes), 10min (10 minutes), 5min (5 minutes), and 1min (1 minute). For example, "Y" indicates every
* year and "5min" indicates every five minutes.
*
*
* The frequency must be greater than or equal to the TARGET_TIME_SERIES dataset frequency.
*
*
* When a RELATED_TIME_SERIES dataset is provided, the frequency must be equal to the RELATED_TIME_SERIES
* dataset frequency.
*/
public String getForecastFrequency() {
return this.forecastFrequency;
}
/**
*
* The frequency of predictions in a forecast.
*
*
* Valid intervals are Y (Year), M (Month), W (Week), D (Day), H (Hour), 30min (30 minutes), 15min (15 minutes),
* 10min (10 minutes), 5min (5 minutes), and 1min (1 minute). For example, "Y" indicates every year and "5min"
* indicates every five minutes.
*
*
* The frequency must be greater than or equal to the TARGET_TIME_SERIES dataset frequency.
*
*
* When a RELATED_TIME_SERIES dataset is provided, the frequency must be equal to the RELATED_TIME_SERIES dataset
* frequency.
*
*
* @param forecastFrequency
* The frequency of predictions in a forecast.
*
* Valid intervals are Y (Year), M (Month), W (Week), D (Day), H (Hour), 30min (30 minutes), 15min (15
* minutes), 10min (10 minutes), 5min (5 minutes), and 1min (1 minute). For example, "Y" indicates every year
* and "5min" indicates every five minutes.
*
*
* The frequency must be greater than or equal to the TARGET_TIME_SERIES dataset frequency.
*
*
* When a RELATED_TIME_SERIES dataset is provided, the frequency must be equal to the RELATED_TIME_SERIES
* dataset frequency.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public FeaturizationConfig withForecastFrequency(String forecastFrequency) {
setForecastFrequency(forecastFrequency);
return this;
}
/**
*
* An array of dimension (field) names that specify how to group the generated forecast.
*
*
* For example, suppose that you are generating a forecast for item sales across all of your stores, and your
* dataset contains a store_id
field. If you want the sales forecast for each item by store, you would
* specify store_id
as the dimension.
*
*
* All forecast dimensions specified in the TARGET_TIME_SERIES
dataset don't need to be specified in
* the CreatePredictor
request. All forecast dimensions specified in the
* RELATED_TIME_SERIES
dataset must be specified in the CreatePredictor
request.
*
*
* @return An array of dimension (field) names that specify how to group the generated forecast.
*
* For example, suppose that you are generating a forecast for item sales across all of your stores, and
* your dataset contains a store_id
field. If you want the sales forecast for each item by
* store, you would specify store_id
as the dimension.
*
*
* All forecast dimensions specified in the TARGET_TIME_SERIES
dataset don't need to be
* specified in the CreatePredictor
request. All forecast dimensions specified in the
* RELATED_TIME_SERIES
dataset must be specified in the CreatePredictor
request.
*/
public java.util.List getForecastDimensions() {
return forecastDimensions;
}
/**
*
* An array of dimension (field) names that specify how to group the generated forecast.
*
*
* For example, suppose that you are generating a forecast for item sales across all of your stores, and your
* dataset contains a store_id
field. If you want the sales forecast for each item by store, you would
* specify store_id
as the dimension.
*
*
* All forecast dimensions specified in the TARGET_TIME_SERIES
dataset don't need to be specified in
* the CreatePredictor
request. All forecast dimensions specified in the
* RELATED_TIME_SERIES
dataset must be specified in the CreatePredictor
request.
*
*
* @param forecastDimensions
* An array of dimension (field) names that specify how to group the generated forecast.
*
* For example, suppose that you are generating a forecast for item sales across all of your stores, and your
* dataset contains a store_id
field. If you want the sales forecast for each item by store, you
* would specify store_id
as the dimension.
*
*
* All forecast dimensions specified in the TARGET_TIME_SERIES
dataset don't need to be
* specified in the CreatePredictor
request. All forecast dimensions specified in the
* RELATED_TIME_SERIES
dataset must be specified in the CreatePredictor
request.
*/
public void setForecastDimensions(java.util.Collection forecastDimensions) {
if (forecastDimensions == null) {
this.forecastDimensions = null;
return;
}
this.forecastDimensions = new java.util.ArrayList(forecastDimensions);
}
/**
*
* An array of dimension (field) names that specify how to group the generated forecast.
*
*
* For example, suppose that you are generating a forecast for item sales across all of your stores, and your
* dataset contains a store_id
field. If you want the sales forecast for each item by store, you would
* specify store_id
as the dimension.
*
*
* All forecast dimensions specified in the TARGET_TIME_SERIES
dataset don't need to be specified in
* the CreatePredictor
request. All forecast dimensions specified in the
* RELATED_TIME_SERIES
dataset must be specified in the CreatePredictor
request.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setForecastDimensions(java.util.Collection)} or {@link #withForecastDimensions(java.util.Collection)} if
* you want to override the existing values.
*
*
* @param forecastDimensions
* An array of dimension (field) names that specify how to group the generated forecast.
*
* For example, suppose that you are generating a forecast for item sales across all of your stores, and your
* dataset contains a store_id
field. If you want the sales forecast for each item by store, you
* would specify store_id
as the dimension.
*
*
* All forecast dimensions specified in the TARGET_TIME_SERIES
dataset don't need to be
* specified in the CreatePredictor
request. All forecast dimensions specified in the
* RELATED_TIME_SERIES
dataset must be specified in the CreatePredictor
request.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public FeaturizationConfig withForecastDimensions(String... forecastDimensions) {
if (this.forecastDimensions == null) {
setForecastDimensions(new java.util.ArrayList(forecastDimensions.length));
}
for (String ele : forecastDimensions) {
this.forecastDimensions.add(ele);
}
return this;
}
/**
*
* An array of dimension (field) names that specify how to group the generated forecast.
*
*
* For example, suppose that you are generating a forecast for item sales across all of your stores, and your
* dataset contains a store_id
field. If you want the sales forecast for each item by store, you would
* specify store_id
as the dimension.
*
*
* All forecast dimensions specified in the TARGET_TIME_SERIES
dataset don't need to be specified in
* the CreatePredictor
request. All forecast dimensions specified in the
* RELATED_TIME_SERIES
dataset must be specified in the CreatePredictor
request.
*
*
* @param forecastDimensions
* An array of dimension (field) names that specify how to group the generated forecast.
*
* For example, suppose that you are generating a forecast for item sales across all of your stores, and your
* dataset contains a store_id
field. If you want the sales forecast for each item by store, you
* would specify store_id
as the dimension.
*
*
* All forecast dimensions specified in the TARGET_TIME_SERIES
dataset don't need to be
* specified in the CreatePredictor
request. All forecast dimensions specified in the
* RELATED_TIME_SERIES
dataset must be specified in the CreatePredictor
request.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public FeaturizationConfig withForecastDimensions(java.util.Collection forecastDimensions) {
setForecastDimensions(forecastDimensions);
return this;
}
/**
*
* An array of featurization (transformation) information for the fields of a dataset. Only a single featurization
* is supported.
*
*
* @return An array of featurization (transformation) information for the fields of a dataset. Only a single
* featurization is supported.
*/
public java.util.List getFeaturizations() {
return featurizations;
}
/**
*
* An array of featurization (transformation) information for the fields of a dataset. Only a single featurization
* is supported.
*
*
* @param featurizations
* An array of featurization (transformation) information for the fields of a dataset. Only a single
* featurization is supported.
*/
public void setFeaturizations(java.util.Collection featurizations) {
if (featurizations == null) {
this.featurizations = null;
return;
}
this.featurizations = new java.util.ArrayList(featurizations);
}
/**
*
* An array of featurization (transformation) information for the fields of a dataset. Only a single featurization
* is supported.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setFeaturizations(java.util.Collection)} or {@link #withFeaturizations(java.util.Collection)} if you want
* to override the existing values.
*
*
* @param featurizations
* An array of featurization (transformation) information for the fields of a dataset. Only a single
* featurization is supported.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public FeaturizationConfig withFeaturizations(Featurization... featurizations) {
if (this.featurizations == null) {
setFeaturizations(new java.util.ArrayList(featurizations.length));
}
for (Featurization ele : featurizations) {
this.featurizations.add(ele);
}
return this;
}
/**
*
* An array of featurization (transformation) information for the fields of a dataset. Only a single featurization
* is supported.
*
*
* @param featurizations
* An array of featurization (transformation) information for the fields of a dataset. Only a single
* featurization is supported.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public FeaturizationConfig withFeaturizations(java.util.Collection featurizations) {
setFeaturizations(featurizations);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getForecastFrequency() != null)
sb.append("ForecastFrequency: ").append(getForecastFrequency()).append(",");
if (getForecastDimensions() != null)
sb.append("ForecastDimensions: ").append(getForecastDimensions()).append(",");
if (getFeaturizations() != null)
sb.append("Featurizations: ").append(getFeaturizations());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof FeaturizationConfig == false)
return false;
FeaturizationConfig other = (FeaturizationConfig) obj;
if (other.getForecastFrequency() == null ^ this.getForecastFrequency() == null)
return false;
if (other.getForecastFrequency() != null && other.getForecastFrequency().equals(this.getForecastFrequency()) == false)
return false;
if (other.getForecastDimensions() == null ^ this.getForecastDimensions() == null)
return false;
if (other.getForecastDimensions() != null && other.getForecastDimensions().equals(this.getForecastDimensions()) == false)
return false;
if (other.getFeaturizations() == null ^ this.getFeaturizations() == null)
return false;
if (other.getFeaturizations() != null && other.getFeaturizations().equals(this.getFeaturizations()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getForecastFrequency() == null) ? 0 : getForecastFrequency().hashCode());
hashCode = prime * hashCode + ((getForecastDimensions() == null) ? 0 : getForecastDimensions().hashCode());
hashCode = prime * hashCode + ((getFeaturizations() == null) ? 0 : getFeaturizations().hashCode());
return hashCode;
}
@Override
public FeaturizationConfig clone() {
try {
return (FeaturizationConfig) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.forecast.model.transform.FeaturizationConfigMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}