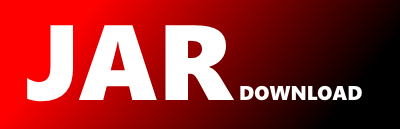
com.amazonaws.services.forecast.model.CreatePredictorRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-forecast Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.forecast.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class CreatePredictorRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* A name for the predictor.
*
*/
private String predictorName;
/**
*
* The Amazon Resource Name (ARN) of the algorithm to use for model training. Required if PerformAutoML
* is not set to true
.
*
*
* Supported algorithms:
*
*
* -
*
* arn:aws:forecast:::algorithm/ARIMA
*
*
* -
*
* arn:aws:forecast:::algorithm/CNN-QR
*
*
* -
*
* arn:aws:forecast:::algorithm/Deep_AR_Plus
*
*
* -
*
* arn:aws:forecast:::algorithm/ETS
*
*
* -
*
* arn:aws:forecast:::algorithm/NPTS
*
*
* -
*
* arn:aws:forecast:::algorithm/Prophet
*
*
*
*/
private String algorithmArn;
/**
*
* Specifies the number of time-steps that the model is trained to predict. The forecast horizon is also called the
* prediction length.
*
*
* For example, if you configure a dataset for daily data collection (using the DataFrequency
parameter
* of the CreateDataset operation) and set the forecast horizon to 10, the model returns predictions for 10
* days.
*
*
* The maximum forecast horizon is the lesser of 500 time-steps or 1/3 of the TARGET_TIME_SERIES dataset length.
*
*/
private Integer forecastHorizon;
/**
*
* Specifies the forecast types used to train a predictor. You can specify up to five forecast types. Forecast types
* can be quantiles from 0.01 to 0.99, by increments of 0.01 or higher. You can also specify the mean forecast with
* mean
.
*
*
* The default value is ["0.10", "0.50", "0.9"]
.
*
*/
private java.util.List forecastTypes;
/**
*
* Whether to perform AutoML. When Amazon Forecast performs AutoML, it evaluates the algorithms it provides and
* chooses the best algorithm and configuration for your training dataset.
*
*
* The default value is false
. In this case, you are required to specify an algorithm.
*
*
* Set PerformAutoML
to true
to have Amazon Forecast perform AutoML. This is a good option
* if you aren't sure which algorithm is suitable for your training data. In this case, PerformHPO
must
* be false.
*
*/
private Boolean performAutoML;
/**
*
*
* The LatencyOptimized
AutoML override strategy is only available in private beta. Contact Amazon Web
* Services Support or your account manager to learn more about access privileges.
*
*
*
* Used to overide the default AutoML strategy, which is to optimize predictor accuracy. To apply an AutoML strategy
* that minimizes training time, use LatencyOptimized
.
*
*
* This parameter is only valid for predictors trained using AutoML.
*
*/
private String autoMLOverrideStrategy;
/**
*
* Whether to perform hyperparameter optimization (HPO). HPO finds optimal hyperparameter values for your training
* data. The process of performing HPO is known as running a hyperparameter tuning job.
*
*
* The default value is false
. In this case, Amazon Forecast uses default hyperparameter values from
* the chosen algorithm.
*
*
* To override the default values, set PerformHPO
to true
and, optionally, supply the
* HyperParameterTuningJobConfig object. The tuning job specifies a metric to optimize, which hyperparameters
* participate in tuning, and the valid range for each tunable hyperparameter. In this case, you are required to
* specify an algorithm and PerformAutoML
must be false.
*
*
* The following algorithms support HPO:
*
*
* -
*
* DeepAR+
*
*
* -
*
* CNN-QR
*
*
*
*/
private Boolean performHPO;
/**
*
* The hyperparameters to override for model training. The hyperparameters that you can override are listed in the
* individual algorithms. For the list of supported algorithms, see aws-forecast-choosing-recipes.
*
*/
private java.util.Map trainingParameters;
/**
*
* Used to override the default evaluation parameters of the specified algorithm. Amazon Forecast evaluates a
* predictor by splitting a dataset into training data and testing data. The evaluation parameters define how to
* perform the split and the number of iterations.
*
*/
private EvaluationParameters evaluationParameters;
/**
*
* Provides hyperparameter override values for the algorithm. If you don't provide this parameter, Amazon Forecast
* uses default values. The individual algorithms specify which hyperparameters support hyperparameter optimization
* (HPO). For more information, see aws-forecast-choosing-recipes.
*
*
* If you included the HPOConfig
object, you must set PerformHPO
to true.
*
*/
private HyperParameterTuningJobConfig hPOConfig;
/**
*
* Describes the dataset group that contains the data to use to train the predictor.
*
*/
private InputDataConfig inputDataConfig;
/**
*
* The featurization configuration.
*
*/
private FeaturizationConfig featurizationConfig;
/**
*
* An Key Management Service (KMS) key and the Identity and Access Management (IAM) role that Amazon Forecast can
* assume to access the key.
*
*/
private EncryptionConfig encryptionConfig;
/**
*
* The optional metadata that you apply to the predictor to help you categorize and organize them. Each tag consists
* of a key and an optional value, both of which you define.
*
*
* The following basic restrictions apply to tags:
*
*
* -
*
* Maximum number of tags per resource - 50.
*
*
* -
*
* For each resource, each tag key must be unique, and each tag key can have only one value.
*
*
* -
*
* Maximum key length - 128 Unicode characters in UTF-8.
*
*
* -
*
* Maximum value length - 256 Unicode characters in UTF-8.
*
*
* -
*
* If your tagging schema is used across multiple services and resources, remember that other services may have
* restrictions on allowed characters. Generally allowed characters are: letters, numbers, and spaces representable
* in UTF-8, and the following characters: + - = . _ : / @.
*
*
* -
*
* Tag keys and values are case sensitive.
*
*
* -
*
* Do not use aws:
, AWS:
, or any upper or lowercase combination of such as a prefix for
* keys as it is reserved for Amazon Web Services use. You cannot edit or delete tag keys with this prefix. Values
* can have this prefix. If a tag value has aws
as its prefix but the key does not, then Forecast
* considers it to be a user tag and will count against the limit of 50 tags. Tags with only the key prefix of
* aws
do not count against your tags per resource limit.
*
*
*
*/
private java.util.List tags;
/**
*
* The accuracy metric used to optimize the predictor.
*
*/
private String optimizationMetric;
/**
*
* A name for the predictor.
*
*
* @param predictorName
* A name for the predictor.
*/
public void setPredictorName(String predictorName) {
this.predictorName = predictorName;
}
/**
*
* A name for the predictor.
*
*
* @return A name for the predictor.
*/
public String getPredictorName() {
return this.predictorName;
}
/**
*
* A name for the predictor.
*
*
* @param predictorName
* A name for the predictor.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreatePredictorRequest withPredictorName(String predictorName) {
setPredictorName(predictorName);
return this;
}
/**
*
* The Amazon Resource Name (ARN) of the algorithm to use for model training. Required if PerformAutoML
* is not set to true
.
*
*
* Supported algorithms:
*
*
* -
*
* arn:aws:forecast:::algorithm/ARIMA
*
*
* -
*
* arn:aws:forecast:::algorithm/CNN-QR
*
*
* -
*
* arn:aws:forecast:::algorithm/Deep_AR_Plus
*
*
* -
*
* arn:aws:forecast:::algorithm/ETS
*
*
* -
*
* arn:aws:forecast:::algorithm/NPTS
*
*
* -
*
* arn:aws:forecast:::algorithm/Prophet
*
*
*
*
* @param algorithmArn
* The Amazon Resource Name (ARN) of the algorithm to use for model training. Required if
* PerformAutoML
is not set to true
.
*
* Supported algorithms:
*
*
* -
*
* arn:aws:forecast:::algorithm/ARIMA
*
*
* -
*
* arn:aws:forecast:::algorithm/CNN-QR
*
*
* -
*
* arn:aws:forecast:::algorithm/Deep_AR_Plus
*
*
* -
*
* arn:aws:forecast:::algorithm/ETS
*
*
* -
*
* arn:aws:forecast:::algorithm/NPTS
*
*
* -
*
* arn:aws:forecast:::algorithm/Prophet
*
*
*/
public void setAlgorithmArn(String algorithmArn) {
this.algorithmArn = algorithmArn;
}
/**
*
* The Amazon Resource Name (ARN) of the algorithm to use for model training. Required if PerformAutoML
* is not set to true
.
*
*
* Supported algorithms:
*
*
* -
*
* arn:aws:forecast:::algorithm/ARIMA
*
*
* -
*
* arn:aws:forecast:::algorithm/CNN-QR
*
*
* -
*
* arn:aws:forecast:::algorithm/Deep_AR_Plus
*
*
* -
*
* arn:aws:forecast:::algorithm/ETS
*
*
* -
*
* arn:aws:forecast:::algorithm/NPTS
*
*
* -
*
* arn:aws:forecast:::algorithm/Prophet
*
*
*
*
* @return The Amazon Resource Name (ARN) of the algorithm to use for model training. Required if
* PerformAutoML
is not set to true
.
*
* Supported algorithms:
*
*
* -
*
* arn:aws:forecast:::algorithm/ARIMA
*
*
* -
*
* arn:aws:forecast:::algorithm/CNN-QR
*
*
* -
*
* arn:aws:forecast:::algorithm/Deep_AR_Plus
*
*
* -
*
* arn:aws:forecast:::algorithm/ETS
*
*
* -
*
* arn:aws:forecast:::algorithm/NPTS
*
*
* -
*
* arn:aws:forecast:::algorithm/Prophet
*
*
*/
public String getAlgorithmArn() {
return this.algorithmArn;
}
/**
*
* The Amazon Resource Name (ARN) of the algorithm to use for model training. Required if PerformAutoML
* is not set to true
.
*
*
* Supported algorithms:
*
*
* -
*
* arn:aws:forecast:::algorithm/ARIMA
*
*
* -
*
* arn:aws:forecast:::algorithm/CNN-QR
*
*
* -
*
* arn:aws:forecast:::algorithm/Deep_AR_Plus
*
*
* -
*
* arn:aws:forecast:::algorithm/ETS
*
*
* -
*
* arn:aws:forecast:::algorithm/NPTS
*
*
* -
*
* arn:aws:forecast:::algorithm/Prophet
*
*
*
*
* @param algorithmArn
* The Amazon Resource Name (ARN) of the algorithm to use for model training. Required if
* PerformAutoML
is not set to true
.
*
* Supported algorithms:
*
*
* -
*
* arn:aws:forecast:::algorithm/ARIMA
*
*
* -
*
* arn:aws:forecast:::algorithm/CNN-QR
*
*
* -
*
* arn:aws:forecast:::algorithm/Deep_AR_Plus
*
*
* -
*
* arn:aws:forecast:::algorithm/ETS
*
*
* -
*
* arn:aws:forecast:::algorithm/NPTS
*
*
* -
*
* arn:aws:forecast:::algorithm/Prophet
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreatePredictorRequest withAlgorithmArn(String algorithmArn) {
setAlgorithmArn(algorithmArn);
return this;
}
/**
*
* Specifies the number of time-steps that the model is trained to predict. The forecast horizon is also called the
* prediction length.
*
*
* For example, if you configure a dataset for daily data collection (using the DataFrequency
parameter
* of the CreateDataset operation) and set the forecast horizon to 10, the model returns predictions for 10
* days.
*
*
* The maximum forecast horizon is the lesser of 500 time-steps or 1/3 of the TARGET_TIME_SERIES dataset length.
*
*
* @param forecastHorizon
* Specifies the number of time-steps that the model is trained to predict. The forecast horizon is also
* called the prediction length.
*
* For example, if you configure a dataset for daily data collection (using the DataFrequency
* parameter of the CreateDataset operation) and set the forecast horizon to 10, the model returns
* predictions for 10 days.
*
*
* The maximum forecast horizon is the lesser of 500 time-steps or 1/3 of the TARGET_TIME_SERIES dataset
* length.
*/
public void setForecastHorizon(Integer forecastHorizon) {
this.forecastHorizon = forecastHorizon;
}
/**
*
* Specifies the number of time-steps that the model is trained to predict. The forecast horizon is also called the
* prediction length.
*
*
* For example, if you configure a dataset for daily data collection (using the DataFrequency
parameter
* of the CreateDataset operation) and set the forecast horizon to 10, the model returns predictions for 10
* days.
*
*
* The maximum forecast horizon is the lesser of 500 time-steps or 1/3 of the TARGET_TIME_SERIES dataset length.
*
*
* @return Specifies the number of time-steps that the model is trained to predict. The forecast horizon is also
* called the prediction length.
*
* For example, if you configure a dataset for daily data collection (using the DataFrequency
* parameter of the CreateDataset operation) and set the forecast horizon to 10, the model returns
* predictions for 10 days.
*
*
* The maximum forecast horizon is the lesser of 500 time-steps or 1/3 of the TARGET_TIME_SERIES dataset
* length.
*/
public Integer getForecastHorizon() {
return this.forecastHorizon;
}
/**
*
* Specifies the number of time-steps that the model is trained to predict. The forecast horizon is also called the
* prediction length.
*
*
* For example, if you configure a dataset for daily data collection (using the DataFrequency
parameter
* of the CreateDataset operation) and set the forecast horizon to 10, the model returns predictions for 10
* days.
*
*
* The maximum forecast horizon is the lesser of 500 time-steps or 1/3 of the TARGET_TIME_SERIES dataset length.
*
*
* @param forecastHorizon
* Specifies the number of time-steps that the model is trained to predict. The forecast horizon is also
* called the prediction length.
*
* For example, if you configure a dataset for daily data collection (using the DataFrequency
* parameter of the CreateDataset operation) and set the forecast horizon to 10, the model returns
* predictions for 10 days.
*
*
* The maximum forecast horizon is the lesser of 500 time-steps or 1/3 of the TARGET_TIME_SERIES dataset
* length.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreatePredictorRequest withForecastHorizon(Integer forecastHorizon) {
setForecastHorizon(forecastHorizon);
return this;
}
/**
*
* Specifies the forecast types used to train a predictor. You can specify up to five forecast types. Forecast types
* can be quantiles from 0.01 to 0.99, by increments of 0.01 or higher. You can also specify the mean forecast with
* mean
.
*
*
* The default value is ["0.10", "0.50", "0.9"]
.
*
*
* @return Specifies the forecast types used to train a predictor. You can specify up to five forecast types.
* Forecast types can be quantiles from 0.01 to 0.99, by increments of 0.01 or higher. You can also specify
* the mean forecast with mean
.
*
* The default value is ["0.10", "0.50", "0.9"]
.
*/
public java.util.List getForecastTypes() {
return forecastTypes;
}
/**
*
* Specifies the forecast types used to train a predictor. You can specify up to five forecast types. Forecast types
* can be quantiles from 0.01 to 0.99, by increments of 0.01 or higher. You can also specify the mean forecast with
* mean
.
*
*
* The default value is ["0.10", "0.50", "0.9"]
.
*
*
* @param forecastTypes
* Specifies the forecast types used to train a predictor. You can specify up to five forecast types.
* Forecast types can be quantiles from 0.01 to 0.99, by increments of 0.01 or higher. You can also specify
* the mean forecast with mean
.
*
* The default value is ["0.10", "0.50", "0.9"]
.
*/
public void setForecastTypes(java.util.Collection forecastTypes) {
if (forecastTypes == null) {
this.forecastTypes = null;
return;
}
this.forecastTypes = new java.util.ArrayList(forecastTypes);
}
/**
*
* Specifies the forecast types used to train a predictor. You can specify up to five forecast types. Forecast types
* can be quantiles from 0.01 to 0.99, by increments of 0.01 or higher. You can also specify the mean forecast with
* mean
.
*
*
* The default value is ["0.10", "0.50", "0.9"]
.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setForecastTypes(java.util.Collection)} or {@link #withForecastTypes(java.util.Collection)} if you want
* to override the existing values.
*
*
* @param forecastTypes
* Specifies the forecast types used to train a predictor. You can specify up to five forecast types.
* Forecast types can be quantiles from 0.01 to 0.99, by increments of 0.01 or higher. You can also specify
* the mean forecast with mean
.
*
* The default value is ["0.10", "0.50", "0.9"]
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreatePredictorRequest withForecastTypes(String... forecastTypes) {
if (this.forecastTypes == null) {
setForecastTypes(new java.util.ArrayList(forecastTypes.length));
}
for (String ele : forecastTypes) {
this.forecastTypes.add(ele);
}
return this;
}
/**
*
* Specifies the forecast types used to train a predictor. You can specify up to five forecast types. Forecast types
* can be quantiles from 0.01 to 0.99, by increments of 0.01 or higher. You can also specify the mean forecast with
* mean
.
*
*
* The default value is ["0.10", "0.50", "0.9"]
.
*
*
* @param forecastTypes
* Specifies the forecast types used to train a predictor. You can specify up to five forecast types.
* Forecast types can be quantiles from 0.01 to 0.99, by increments of 0.01 or higher. You can also specify
* the mean forecast with mean
.
*
* The default value is ["0.10", "0.50", "0.9"]
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreatePredictorRequest withForecastTypes(java.util.Collection forecastTypes) {
setForecastTypes(forecastTypes);
return this;
}
/**
*
* Whether to perform AutoML. When Amazon Forecast performs AutoML, it evaluates the algorithms it provides and
* chooses the best algorithm and configuration for your training dataset.
*
*
* The default value is false
. In this case, you are required to specify an algorithm.
*
*
* Set PerformAutoML
to true
to have Amazon Forecast perform AutoML. This is a good option
* if you aren't sure which algorithm is suitable for your training data. In this case, PerformHPO
must
* be false.
*
*
* @param performAutoML
* Whether to perform AutoML. When Amazon Forecast performs AutoML, it evaluates the algorithms it provides
* and chooses the best algorithm and configuration for your training dataset.
*
* The default value is false
. In this case, you are required to specify an algorithm.
*
*
* Set PerformAutoML
to true
to have Amazon Forecast perform AutoML. This is a good
* option if you aren't sure which algorithm is suitable for your training data. In this case,
* PerformHPO
must be false.
*/
public void setPerformAutoML(Boolean performAutoML) {
this.performAutoML = performAutoML;
}
/**
*
* Whether to perform AutoML. When Amazon Forecast performs AutoML, it evaluates the algorithms it provides and
* chooses the best algorithm and configuration for your training dataset.
*
*
* The default value is false
. In this case, you are required to specify an algorithm.
*
*
* Set PerformAutoML
to true
to have Amazon Forecast perform AutoML. This is a good option
* if you aren't sure which algorithm is suitable for your training data. In this case, PerformHPO
must
* be false.
*
*
* @return Whether to perform AutoML. When Amazon Forecast performs AutoML, it evaluates the algorithms it provides
* and chooses the best algorithm and configuration for your training dataset.
*
* The default value is false
. In this case, you are required to specify an algorithm.
*
*
* Set PerformAutoML
to true
to have Amazon Forecast perform AutoML. This is a
* good option if you aren't sure which algorithm is suitable for your training data. In this case,
* PerformHPO
must be false.
*/
public Boolean getPerformAutoML() {
return this.performAutoML;
}
/**
*
* Whether to perform AutoML. When Amazon Forecast performs AutoML, it evaluates the algorithms it provides and
* chooses the best algorithm and configuration for your training dataset.
*
*
* The default value is false
. In this case, you are required to specify an algorithm.
*
*
* Set PerformAutoML
to true
to have Amazon Forecast perform AutoML. This is a good option
* if you aren't sure which algorithm is suitable for your training data. In this case, PerformHPO
must
* be false.
*
*
* @param performAutoML
* Whether to perform AutoML. When Amazon Forecast performs AutoML, it evaluates the algorithms it provides
* and chooses the best algorithm and configuration for your training dataset.
*
* The default value is false
. In this case, you are required to specify an algorithm.
*
*
* Set PerformAutoML
to true
to have Amazon Forecast perform AutoML. This is a good
* option if you aren't sure which algorithm is suitable for your training data. In this case,
* PerformHPO
must be false.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreatePredictorRequest withPerformAutoML(Boolean performAutoML) {
setPerformAutoML(performAutoML);
return this;
}
/**
*
* Whether to perform AutoML. When Amazon Forecast performs AutoML, it evaluates the algorithms it provides and
* chooses the best algorithm and configuration for your training dataset.
*
*
* The default value is false
. In this case, you are required to specify an algorithm.
*
*
* Set PerformAutoML
to true
to have Amazon Forecast perform AutoML. This is a good option
* if you aren't sure which algorithm is suitable for your training data. In this case, PerformHPO
must
* be false.
*
*
* @return Whether to perform AutoML. When Amazon Forecast performs AutoML, it evaluates the algorithms it provides
* and chooses the best algorithm and configuration for your training dataset.
*
* The default value is false
. In this case, you are required to specify an algorithm.
*
*
* Set PerformAutoML
to true
to have Amazon Forecast perform AutoML. This is a
* good option if you aren't sure which algorithm is suitable for your training data. In this case,
* PerformHPO
must be false.
*/
public Boolean isPerformAutoML() {
return this.performAutoML;
}
/**
*
*
* The LatencyOptimized
AutoML override strategy is only available in private beta. Contact Amazon Web
* Services Support or your account manager to learn more about access privileges.
*
*
*
* Used to overide the default AutoML strategy, which is to optimize predictor accuracy. To apply an AutoML strategy
* that minimizes training time, use LatencyOptimized
.
*
*
* This parameter is only valid for predictors trained using AutoML.
*
*
* @param autoMLOverrideStrategy
*
* The LatencyOptimized
AutoML override strategy is only available in private beta. Contact
* Amazon Web Services Support or your account manager to learn more about access privileges.
*
*
*
* Used to overide the default AutoML strategy, which is to optimize predictor accuracy. To apply an AutoML
* strategy that minimizes training time, use LatencyOptimized
.
*
*
* This parameter is only valid for predictors trained using AutoML.
* @see AutoMLOverrideStrategy
*/
public void setAutoMLOverrideStrategy(String autoMLOverrideStrategy) {
this.autoMLOverrideStrategy = autoMLOverrideStrategy;
}
/**
*
*
* The LatencyOptimized
AutoML override strategy is only available in private beta. Contact Amazon Web
* Services Support or your account manager to learn more about access privileges.
*
*
*
* Used to overide the default AutoML strategy, which is to optimize predictor accuracy. To apply an AutoML strategy
* that minimizes training time, use LatencyOptimized
.
*
*
* This parameter is only valid for predictors trained using AutoML.
*
*
* @return
* The LatencyOptimized
AutoML override strategy is only available in private beta. Contact
* Amazon Web Services Support or your account manager to learn more about access privileges.
*
*
*
* Used to overide the default AutoML strategy, which is to optimize predictor accuracy. To apply an AutoML
* strategy that minimizes training time, use LatencyOptimized
.
*
*
* This parameter is only valid for predictors trained using AutoML.
* @see AutoMLOverrideStrategy
*/
public String getAutoMLOverrideStrategy() {
return this.autoMLOverrideStrategy;
}
/**
*
*
* The LatencyOptimized
AutoML override strategy is only available in private beta. Contact Amazon Web
* Services Support or your account manager to learn more about access privileges.
*
*
*
* Used to overide the default AutoML strategy, which is to optimize predictor accuracy. To apply an AutoML strategy
* that minimizes training time, use LatencyOptimized
.
*
*
* This parameter is only valid for predictors trained using AutoML.
*
*
* @param autoMLOverrideStrategy
*
* The LatencyOptimized
AutoML override strategy is only available in private beta. Contact
* Amazon Web Services Support or your account manager to learn more about access privileges.
*
*
*
* Used to overide the default AutoML strategy, which is to optimize predictor accuracy. To apply an AutoML
* strategy that minimizes training time, use LatencyOptimized
.
*
*
* This parameter is only valid for predictors trained using AutoML.
* @return Returns a reference to this object so that method calls can be chained together.
* @see AutoMLOverrideStrategy
*/
public CreatePredictorRequest withAutoMLOverrideStrategy(String autoMLOverrideStrategy) {
setAutoMLOverrideStrategy(autoMLOverrideStrategy);
return this;
}
/**
*
*
* The LatencyOptimized
AutoML override strategy is only available in private beta. Contact Amazon Web
* Services Support or your account manager to learn more about access privileges.
*
*
*
* Used to overide the default AutoML strategy, which is to optimize predictor accuracy. To apply an AutoML strategy
* that minimizes training time, use LatencyOptimized
.
*
*
* This parameter is only valid for predictors trained using AutoML.
*
*
* @param autoMLOverrideStrategy
*
* The LatencyOptimized
AutoML override strategy is only available in private beta. Contact
* Amazon Web Services Support or your account manager to learn more about access privileges.
*
*
*
* Used to overide the default AutoML strategy, which is to optimize predictor accuracy. To apply an AutoML
* strategy that minimizes training time, use LatencyOptimized
.
*
*
* This parameter is only valid for predictors trained using AutoML.
* @return Returns a reference to this object so that method calls can be chained together.
* @see AutoMLOverrideStrategy
*/
public CreatePredictorRequest withAutoMLOverrideStrategy(AutoMLOverrideStrategy autoMLOverrideStrategy) {
this.autoMLOverrideStrategy = autoMLOverrideStrategy.toString();
return this;
}
/**
*
* Whether to perform hyperparameter optimization (HPO). HPO finds optimal hyperparameter values for your training
* data. The process of performing HPO is known as running a hyperparameter tuning job.
*
*
* The default value is false
. In this case, Amazon Forecast uses default hyperparameter values from
* the chosen algorithm.
*
*
* To override the default values, set PerformHPO
to true
and, optionally, supply the
* HyperParameterTuningJobConfig object. The tuning job specifies a metric to optimize, which hyperparameters
* participate in tuning, and the valid range for each tunable hyperparameter. In this case, you are required to
* specify an algorithm and PerformAutoML
must be false.
*
*
* The following algorithms support HPO:
*
*
* -
*
* DeepAR+
*
*
* -
*
* CNN-QR
*
*
*
*
* @param performHPO
* Whether to perform hyperparameter optimization (HPO). HPO finds optimal hyperparameter values for your
* training data. The process of performing HPO is known as running a hyperparameter tuning job.
*
* The default value is false
. In this case, Amazon Forecast uses default hyperparameter values
* from the chosen algorithm.
*
*
* To override the default values, set PerformHPO
to true
and, optionally, supply
* the HyperParameterTuningJobConfig object. The tuning job specifies a metric to optimize, which
* hyperparameters participate in tuning, and the valid range for each tunable hyperparameter. In this case,
* you are required to specify an algorithm and PerformAutoML
must be false.
*
*
* The following algorithms support HPO:
*
*
* -
*
* DeepAR+
*
*
* -
*
* CNN-QR
*
*
*/
public void setPerformHPO(Boolean performHPO) {
this.performHPO = performHPO;
}
/**
*
* Whether to perform hyperparameter optimization (HPO). HPO finds optimal hyperparameter values for your training
* data. The process of performing HPO is known as running a hyperparameter tuning job.
*
*
* The default value is false
. In this case, Amazon Forecast uses default hyperparameter values from
* the chosen algorithm.
*
*
* To override the default values, set PerformHPO
to true
and, optionally, supply the
* HyperParameterTuningJobConfig object. The tuning job specifies a metric to optimize, which hyperparameters
* participate in tuning, and the valid range for each tunable hyperparameter. In this case, you are required to
* specify an algorithm and PerformAutoML
must be false.
*
*
* The following algorithms support HPO:
*
*
* -
*
* DeepAR+
*
*
* -
*
* CNN-QR
*
*
*
*
* @return Whether to perform hyperparameter optimization (HPO). HPO finds optimal hyperparameter values for your
* training data. The process of performing HPO is known as running a hyperparameter tuning job.
*
* The default value is false
. In this case, Amazon Forecast uses default hyperparameter values
* from the chosen algorithm.
*
*
* To override the default values, set PerformHPO
to true
and, optionally, supply
* the HyperParameterTuningJobConfig object. The tuning job specifies a metric to optimize, which
* hyperparameters participate in tuning, and the valid range for each tunable hyperparameter. In this case,
* you are required to specify an algorithm and PerformAutoML
must be false.
*
*
* The following algorithms support HPO:
*
*
* -
*
* DeepAR+
*
*
* -
*
* CNN-QR
*
*
*/
public Boolean getPerformHPO() {
return this.performHPO;
}
/**
*
* Whether to perform hyperparameter optimization (HPO). HPO finds optimal hyperparameter values for your training
* data. The process of performing HPO is known as running a hyperparameter tuning job.
*
*
* The default value is false
. In this case, Amazon Forecast uses default hyperparameter values from
* the chosen algorithm.
*
*
* To override the default values, set PerformHPO
to true
and, optionally, supply the
* HyperParameterTuningJobConfig object. The tuning job specifies a metric to optimize, which hyperparameters
* participate in tuning, and the valid range for each tunable hyperparameter. In this case, you are required to
* specify an algorithm and PerformAutoML
must be false.
*
*
* The following algorithms support HPO:
*
*
* -
*
* DeepAR+
*
*
* -
*
* CNN-QR
*
*
*
*
* @param performHPO
* Whether to perform hyperparameter optimization (HPO). HPO finds optimal hyperparameter values for your
* training data. The process of performing HPO is known as running a hyperparameter tuning job.
*
* The default value is false
. In this case, Amazon Forecast uses default hyperparameter values
* from the chosen algorithm.
*
*
* To override the default values, set PerformHPO
to true
and, optionally, supply
* the HyperParameterTuningJobConfig object. The tuning job specifies a metric to optimize, which
* hyperparameters participate in tuning, and the valid range for each tunable hyperparameter. In this case,
* you are required to specify an algorithm and PerformAutoML
must be false.
*
*
* The following algorithms support HPO:
*
*
* -
*
* DeepAR+
*
*
* -
*
* CNN-QR
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreatePredictorRequest withPerformHPO(Boolean performHPO) {
setPerformHPO(performHPO);
return this;
}
/**
*
* Whether to perform hyperparameter optimization (HPO). HPO finds optimal hyperparameter values for your training
* data. The process of performing HPO is known as running a hyperparameter tuning job.
*
*
* The default value is false
. In this case, Amazon Forecast uses default hyperparameter values from
* the chosen algorithm.
*
*
* To override the default values, set PerformHPO
to true
and, optionally, supply the
* HyperParameterTuningJobConfig object. The tuning job specifies a metric to optimize, which hyperparameters
* participate in tuning, and the valid range for each tunable hyperparameter. In this case, you are required to
* specify an algorithm and PerformAutoML
must be false.
*
*
* The following algorithms support HPO:
*
*
* -
*
* DeepAR+
*
*
* -
*
* CNN-QR
*
*
*
*
* @return Whether to perform hyperparameter optimization (HPO). HPO finds optimal hyperparameter values for your
* training data. The process of performing HPO is known as running a hyperparameter tuning job.
*
* The default value is false
. In this case, Amazon Forecast uses default hyperparameter values
* from the chosen algorithm.
*
*
* To override the default values, set PerformHPO
to true
and, optionally, supply
* the HyperParameterTuningJobConfig object. The tuning job specifies a metric to optimize, which
* hyperparameters participate in tuning, and the valid range for each tunable hyperparameter. In this case,
* you are required to specify an algorithm and PerformAutoML
must be false.
*
*
* The following algorithms support HPO:
*
*
* -
*
* DeepAR+
*
*
* -
*
* CNN-QR
*
*
*/
public Boolean isPerformHPO() {
return this.performHPO;
}
/**
*
* The hyperparameters to override for model training. The hyperparameters that you can override are listed in the
* individual algorithms. For the list of supported algorithms, see aws-forecast-choosing-recipes.
*
*
* @return The hyperparameters to override for model training. The hyperparameters that you can override are listed
* in the individual algorithms. For the list of supported algorithms, see
* aws-forecast-choosing-recipes.
*/
public java.util.Map getTrainingParameters() {
return trainingParameters;
}
/**
*
* The hyperparameters to override for model training. The hyperparameters that you can override are listed in the
* individual algorithms. For the list of supported algorithms, see aws-forecast-choosing-recipes.
*
*
* @param trainingParameters
* The hyperparameters to override for model training. The hyperparameters that you can override are listed
* in the individual algorithms. For the list of supported algorithms, see
* aws-forecast-choosing-recipes.
*/
public void setTrainingParameters(java.util.Map trainingParameters) {
this.trainingParameters = trainingParameters;
}
/**
*
* The hyperparameters to override for model training. The hyperparameters that you can override are listed in the
* individual algorithms. For the list of supported algorithms, see aws-forecast-choosing-recipes.
*
*
* @param trainingParameters
* The hyperparameters to override for model training. The hyperparameters that you can override are listed
* in the individual algorithms. For the list of supported algorithms, see
* aws-forecast-choosing-recipes.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreatePredictorRequest withTrainingParameters(java.util.Map trainingParameters) {
setTrainingParameters(trainingParameters);
return this;
}
/**
* Add a single TrainingParameters entry
*
* @see CreatePredictorRequest#withTrainingParameters
* @returns a reference to this object so that method calls can be chained together.
*/
public CreatePredictorRequest addTrainingParametersEntry(String key, String value) {
if (null == this.trainingParameters) {
this.trainingParameters = new java.util.HashMap();
}
if (this.trainingParameters.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.trainingParameters.put(key, value);
return this;
}
/**
* Removes all the entries added into TrainingParameters.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreatePredictorRequest clearTrainingParametersEntries() {
this.trainingParameters = null;
return this;
}
/**
*
* Used to override the default evaluation parameters of the specified algorithm. Amazon Forecast evaluates a
* predictor by splitting a dataset into training data and testing data. The evaluation parameters define how to
* perform the split and the number of iterations.
*
*
* @param evaluationParameters
* Used to override the default evaluation parameters of the specified algorithm. Amazon Forecast evaluates a
* predictor by splitting a dataset into training data and testing data. The evaluation parameters define how
* to perform the split and the number of iterations.
*/
public void setEvaluationParameters(EvaluationParameters evaluationParameters) {
this.evaluationParameters = evaluationParameters;
}
/**
*
* Used to override the default evaluation parameters of the specified algorithm. Amazon Forecast evaluates a
* predictor by splitting a dataset into training data and testing data. The evaluation parameters define how to
* perform the split and the number of iterations.
*
*
* @return Used to override the default evaluation parameters of the specified algorithm. Amazon Forecast evaluates
* a predictor by splitting a dataset into training data and testing data. The evaluation parameters define
* how to perform the split and the number of iterations.
*/
public EvaluationParameters getEvaluationParameters() {
return this.evaluationParameters;
}
/**
*
* Used to override the default evaluation parameters of the specified algorithm. Amazon Forecast evaluates a
* predictor by splitting a dataset into training data and testing data. The evaluation parameters define how to
* perform the split and the number of iterations.
*
*
* @param evaluationParameters
* Used to override the default evaluation parameters of the specified algorithm. Amazon Forecast evaluates a
* predictor by splitting a dataset into training data and testing data. The evaluation parameters define how
* to perform the split and the number of iterations.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreatePredictorRequest withEvaluationParameters(EvaluationParameters evaluationParameters) {
setEvaluationParameters(evaluationParameters);
return this;
}
/**
*
* Provides hyperparameter override values for the algorithm. If you don't provide this parameter, Amazon Forecast
* uses default values. The individual algorithms specify which hyperparameters support hyperparameter optimization
* (HPO). For more information, see aws-forecast-choosing-recipes.
*
*
* If you included the HPOConfig
object, you must set PerformHPO
to true.
*
*
* @param hPOConfig
* Provides hyperparameter override values for the algorithm. If you don't provide this parameter, Amazon
* Forecast uses default values. The individual algorithms specify which hyperparameters support
* hyperparameter optimization (HPO). For more information, see aws-forecast-choosing-recipes.
*
* If you included the HPOConfig
object, you must set PerformHPO
to true.
*/
public void setHPOConfig(HyperParameterTuningJobConfig hPOConfig) {
this.hPOConfig = hPOConfig;
}
/**
*
* Provides hyperparameter override values for the algorithm. If you don't provide this parameter, Amazon Forecast
* uses default values. The individual algorithms specify which hyperparameters support hyperparameter optimization
* (HPO). For more information, see aws-forecast-choosing-recipes.
*
*
* If you included the HPOConfig
object, you must set PerformHPO
to true.
*
*
* @return Provides hyperparameter override values for the algorithm. If you don't provide this parameter, Amazon
* Forecast uses default values. The individual algorithms specify which hyperparameters support
* hyperparameter optimization (HPO). For more information, see aws-forecast-choosing-recipes.
*
* If you included the HPOConfig
object, you must set PerformHPO
to true.
*/
public HyperParameterTuningJobConfig getHPOConfig() {
return this.hPOConfig;
}
/**
*
* Provides hyperparameter override values for the algorithm. If you don't provide this parameter, Amazon Forecast
* uses default values. The individual algorithms specify which hyperparameters support hyperparameter optimization
* (HPO). For more information, see aws-forecast-choosing-recipes.
*
*
* If you included the HPOConfig
object, you must set PerformHPO
to true.
*
*
* @param hPOConfig
* Provides hyperparameter override values for the algorithm. If you don't provide this parameter, Amazon
* Forecast uses default values. The individual algorithms specify which hyperparameters support
* hyperparameter optimization (HPO). For more information, see aws-forecast-choosing-recipes.
*
* If you included the HPOConfig
object, you must set PerformHPO
to true.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreatePredictorRequest withHPOConfig(HyperParameterTuningJobConfig hPOConfig) {
setHPOConfig(hPOConfig);
return this;
}
/**
*
* Describes the dataset group that contains the data to use to train the predictor.
*
*
* @param inputDataConfig
* Describes the dataset group that contains the data to use to train the predictor.
*/
public void setInputDataConfig(InputDataConfig inputDataConfig) {
this.inputDataConfig = inputDataConfig;
}
/**
*
* Describes the dataset group that contains the data to use to train the predictor.
*
*
* @return Describes the dataset group that contains the data to use to train the predictor.
*/
public InputDataConfig getInputDataConfig() {
return this.inputDataConfig;
}
/**
*
* Describes the dataset group that contains the data to use to train the predictor.
*
*
* @param inputDataConfig
* Describes the dataset group that contains the data to use to train the predictor.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreatePredictorRequest withInputDataConfig(InputDataConfig inputDataConfig) {
setInputDataConfig(inputDataConfig);
return this;
}
/**
*
* The featurization configuration.
*
*
* @param featurizationConfig
* The featurization configuration.
*/
public void setFeaturizationConfig(FeaturizationConfig featurizationConfig) {
this.featurizationConfig = featurizationConfig;
}
/**
*
* The featurization configuration.
*
*
* @return The featurization configuration.
*/
public FeaturizationConfig getFeaturizationConfig() {
return this.featurizationConfig;
}
/**
*
* The featurization configuration.
*
*
* @param featurizationConfig
* The featurization configuration.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreatePredictorRequest withFeaturizationConfig(FeaturizationConfig featurizationConfig) {
setFeaturizationConfig(featurizationConfig);
return this;
}
/**
*
* An Key Management Service (KMS) key and the Identity and Access Management (IAM) role that Amazon Forecast can
* assume to access the key.
*
*
* @param encryptionConfig
* An Key Management Service (KMS) key and the Identity and Access Management (IAM) role that Amazon Forecast
* can assume to access the key.
*/
public void setEncryptionConfig(EncryptionConfig encryptionConfig) {
this.encryptionConfig = encryptionConfig;
}
/**
*
* An Key Management Service (KMS) key and the Identity and Access Management (IAM) role that Amazon Forecast can
* assume to access the key.
*
*
* @return An Key Management Service (KMS) key and the Identity and Access Management (IAM) role that Amazon
* Forecast can assume to access the key.
*/
public EncryptionConfig getEncryptionConfig() {
return this.encryptionConfig;
}
/**
*
* An Key Management Service (KMS) key and the Identity and Access Management (IAM) role that Amazon Forecast can
* assume to access the key.
*
*
* @param encryptionConfig
* An Key Management Service (KMS) key and the Identity and Access Management (IAM) role that Amazon Forecast
* can assume to access the key.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreatePredictorRequest withEncryptionConfig(EncryptionConfig encryptionConfig) {
setEncryptionConfig(encryptionConfig);
return this;
}
/**
*
* The optional metadata that you apply to the predictor to help you categorize and organize them. Each tag consists
* of a key and an optional value, both of which you define.
*
*
* The following basic restrictions apply to tags:
*
*
* -
*
* Maximum number of tags per resource - 50.
*
*
* -
*
* For each resource, each tag key must be unique, and each tag key can have only one value.
*
*
* -
*
* Maximum key length - 128 Unicode characters in UTF-8.
*
*
* -
*
* Maximum value length - 256 Unicode characters in UTF-8.
*
*
* -
*
* If your tagging schema is used across multiple services and resources, remember that other services may have
* restrictions on allowed characters. Generally allowed characters are: letters, numbers, and spaces representable
* in UTF-8, and the following characters: + - = . _ : / @.
*
*
* -
*
* Tag keys and values are case sensitive.
*
*
* -
*
* Do not use aws:
, AWS:
, or any upper or lowercase combination of such as a prefix for
* keys as it is reserved for Amazon Web Services use. You cannot edit or delete tag keys with this prefix. Values
* can have this prefix. If a tag value has aws
as its prefix but the key does not, then Forecast
* considers it to be a user tag and will count against the limit of 50 tags. Tags with only the key prefix of
* aws
do not count against your tags per resource limit.
*
*
*
*
* @return The optional metadata that you apply to the predictor to help you categorize and organize them. Each tag
* consists of a key and an optional value, both of which you define.
*
* The following basic restrictions apply to tags:
*
*
* -
*
* Maximum number of tags per resource - 50.
*
*
* -
*
* For each resource, each tag key must be unique, and each tag key can have only one value.
*
*
* -
*
* Maximum key length - 128 Unicode characters in UTF-8.
*
*
* -
*
* Maximum value length - 256 Unicode characters in UTF-8.
*
*
* -
*
* If your tagging schema is used across multiple services and resources, remember that other services may
* have restrictions on allowed characters. Generally allowed characters are: letters, numbers, and spaces
* representable in UTF-8, and the following characters: + - = . _ : / @.
*
*
* -
*
* Tag keys and values are case sensitive.
*
*
* -
*
* Do not use aws:
, AWS:
, or any upper or lowercase combination of such as a
* prefix for keys as it is reserved for Amazon Web Services use. You cannot edit or delete tag keys with
* this prefix. Values can have this prefix. If a tag value has aws
as its prefix but the key
* does not, then Forecast considers it to be a user tag and will count against the limit of 50 tags. Tags
* with only the key prefix of aws
do not count against your tags per resource limit.
*
*
*/
public java.util.List getTags() {
return tags;
}
/**
*
* The optional metadata that you apply to the predictor to help you categorize and organize them. Each tag consists
* of a key and an optional value, both of which you define.
*
*
* The following basic restrictions apply to tags:
*
*
* -
*
* Maximum number of tags per resource - 50.
*
*
* -
*
* For each resource, each tag key must be unique, and each tag key can have only one value.
*
*
* -
*
* Maximum key length - 128 Unicode characters in UTF-8.
*
*
* -
*
* Maximum value length - 256 Unicode characters in UTF-8.
*
*
* -
*
* If your tagging schema is used across multiple services and resources, remember that other services may have
* restrictions on allowed characters. Generally allowed characters are: letters, numbers, and spaces representable
* in UTF-8, and the following characters: + - = . _ : / @.
*
*
* -
*
* Tag keys and values are case sensitive.
*
*
* -
*
* Do not use aws:
, AWS:
, or any upper or lowercase combination of such as a prefix for
* keys as it is reserved for Amazon Web Services use. You cannot edit or delete tag keys with this prefix. Values
* can have this prefix. If a tag value has aws
as its prefix but the key does not, then Forecast
* considers it to be a user tag and will count against the limit of 50 tags. Tags with only the key prefix of
* aws
do not count against your tags per resource limit.
*
*
*
*
* @param tags
* The optional metadata that you apply to the predictor to help you categorize and organize them. Each tag
* consists of a key and an optional value, both of which you define.
*
* The following basic restrictions apply to tags:
*
*
* -
*
* Maximum number of tags per resource - 50.
*
*
* -
*
* For each resource, each tag key must be unique, and each tag key can have only one value.
*
*
* -
*
* Maximum key length - 128 Unicode characters in UTF-8.
*
*
* -
*
* Maximum value length - 256 Unicode characters in UTF-8.
*
*
* -
*
* If your tagging schema is used across multiple services and resources, remember that other services may
* have restrictions on allowed characters. Generally allowed characters are: letters, numbers, and spaces
* representable in UTF-8, and the following characters: + - = . _ : / @.
*
*
* -
*
* Tag keys and values are case sensitive.
*
*
* -
*
* Do not use aws:
, AWS:
, or any upper or lowercase combination of such as a prefix
* for keys as it is reserved for Amazon Web Services use. You cannot edit or delete tag keys with this
* prefix. Values can have this prefix. If a tag value has aws
as its prefix but the key does
* not, then Forecast considers it to be a user tag and will count against the limit of 50 tags. Tags with
* only the key prefix of aws
do not count against your tags per resource limit.
*
*
*/
public void setTags(java.util.Collection tags) {
if (tags == null) {
this.tags = null;
return;
}
this.tags = new java.util.ArrayList(tags);
}
/**
*
* The optional metadata that you apply to the predictor to help you categorize and organize them. Each tag consists
* of a key and an optional value, both of which you define.
*
*
* The following basic restrictions apply to tags:
*
*
* -
*
* Maximum number of tags per resource - 50.
*
*
* -
*
* For each resource, each tag key must be unique, and each tag key can have only one value.
*
*
* -
*
* Maximum key length - 128 Unicode characters in UTF-8.
*
*
* -
*
* Maximum value length - 256 Unicode characters in UTF-8.
*
*
* -
*
* If your tagging schema is used across multiple services and resources, remember that other services may have
* restrictions on allowed characters. Generally allowed characters are: letters, numbers, and spaces representable
* in UTF-8, and the following characters: + - = . _ : / @.
*
*
* -
*
* Tag keys and values are case sensitive.
*
*
* -
*
* Do not use aws:
, AWS:
, or any upper or lowercase combination of such as a prefix for
* keys as it is reserved for Amazon Web Services use. You cannot edit or delete tag keys with this prefix. Values
* can have this prefix. If a tag value has aws
as its prefix but the key does not, then Forecast
* considers it to be a user tag and will count against the limit of 50 tags. Tags with only the key prefix of
* aws
do not count against your tags per resource limit.
*
*
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTags(java.util.Collection)} or {@link #withTags(java.util.Collection)} if you want to override the
* existing values.
*
*
* @param tags
* The optional metadata that you apply to the predictor to help you categorize and organize them. Each tag
* consists of a key and an optional value, both of which you define.
*
* The following basic restrictions apply to tags:
*
*
* -
*
* Maximum number of tags per resource - 50.
*
*
* -
*
* For each resource, each tag key must be unique, and each tag key can have only one value.
*
*
* -
*
* Maximum key length - 128 Unicode characters in UTF-8.
*
*
* -
*
* Maximum value length - 256 Unicode characters in UTF-8.
*
*
* -
*
* If your tagging schema is used across multiple services and resources, remember that other services may
* have restrictions on allowed characters. Generally allowed characters are: letters, numbers, and spaces
* representable in UTF-8, and the following characters: + - = . _ : / @.
*
*
* -
*
* Tag keys and values are case sensitive.
*
*
* -
*
* Do not use aws:
, AWS:
, or any upper or lowercase combination of such as a prefix
* for keys as it is reserved for Amazon Web Services use. You cannot edit or delete tag keys with this
* prefix. Values can have this prefix. If a tag value has aws
as its prefix but the key does
* not, then Forecast considers it to be a user tag and will count against the limit of 50 tags. Tags with
* only the key prefix of aws
do not count against your tags per resource limit.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreatePredictorRequest withTags(Tag... tags) {
if (this.tags == null) {
setTags(new java.util.ArrayList(tags.length));
}
for (Tag ele : tags) {
this.tags.add(ele);
}
return this;
}
/**
*
* The optional metadata that you apply to the predictor to help you categorize and organize them. Each tag consists
* of a key and an optional value, both of which you define.
*
*
* The following basic restrictions apply to tags:
*
*
* -
*
* Maximum number of tags per resource - 50.
*
*
* -
*
* For each resource, each tag key must be unique, and each tag key can have only one value.
*
*
* -
*
* Maximum key length - 128 Unicode characters in UTF-8.
*
*
* -
*
* Maximum value length - 256 Unicode characters in UTF-8.
*
*
* -
*
* If your tagging schema is used across multiple services and resources, remember that other services may have
* restrictions on allowed characters. Generally allowed characters are: letters, numbers, and spaces representable
* in UTF-8, and the following characters: + - = . _ : / @.
*
*
* -
*
* Tag keys and values are case sensitive.
*
*
* -
*
* Do not use aws:
, AWS:
, or any upper or lowercase combination of such as a prefix for
* keys as it is reserved for Amazon Web Services use. You cannot edit or delete tag keys with this prefix. Values
* can have this prefix. If a tag value has aws
as its prefix but the key does not, then Forecast
* considers it to be a user tag and will count against the limit of 50 tags. Tags with only the key prefix of
* aws
do not count against your tags per resource limit.
*
*
*
*
* @param tags
* The optional metadata that you apply to the predictor to help you categorize and organize them. Each tag
* consists of a key and an optional value, both of which you define.
*
* The following basic restrictions apply to tags:
*
*
* -
*
* Maximum number of tags per resource - 50.
*
*
* -
*
* For each resource, each tag key must be unique, and each tag key can have only one value.
*
*
* -
*
* Maximum key length - 128 Unicode characters in UTF-8.
*
*
* -
*
* Maximum value length - 256 Unicode characters in UTF-8.
*
*
* -
*
* If your tagging schema is used across multiple services and resources, remember that other services may
* have restrictions on allowed characters. Generally allowed characters are: letters, numbers, and spaces
* representable in UTF-8, and the following characters: + - = . _ : / @.
*
*
* -
*
* Tag keys and values are case sensitive.
*
*
* -
*
* Do not use aws:
, AWS:
, or any upper or lowercase combination of such as a prefix
* for keys as it is reserved for Amazon Web Services use. You cannot edit or delete tag keys with this
* prefix. Values can have this prefix. If a tag value has aws
as its prefix but the key does
* not, then Forecast considers it to be a user tag and will count against the limit of 50 tags. Tags with
* only the key prefix of aws
do not count against your tags per resource limit.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreatePredictorRequest withTags(java.util.Collection tags) {
setTags(tags);
return this;
}
/**
*
* The accuracy metric used to optimize the predictor.
*
*
* @param optimizationMetric
* The accuracy metric used to optimize the predictor.
* @see OptimizationMetric
*/
public void setOptimizationMetric(String optimizationMetric) {
this.optimizationMetric = optimizationMetric;
}
/**
*
* The accuracy metric used to optimize the predictor.
*
*
* @return The accuracy metric used to optimize the predictor.
* @see OptimizationMetric
*/
public String getOptimizationMetric() {
return this.optimizationMetric;
}
/**
*
* The accuracy metric used to optimize the predictor.
*
*
* @param optimizationMetric
* The accuracy metric used to optimize the predictor.
* @return Returns a reference to this object so that method calls can be chained together.
* @see OptimizationMetric
*/
public CreatePredictorRequest withOptimizationMetric(String optimizationMetric) {
setOptimizationMetric(optimizationMetric);
return this;
}
/**
*
* The accuracy metric used to optimize the predictor.
*
*
* @param optimizationMetric
* The accuracy metric used to optimize the predictor.
* @return Returns a reference to this object so that method calls can be chained together.
* @see OptimizationMetric
*/
public CreatePredictorRequest withOptimizationMetric(OptimizationMetric optimizationMetric) {
this.optimizationMetric = optimizationMetric.toString();
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getPredictorName() != null)
sb.append("PredictorName: ").append(getPredictorName()).append(",");
if (getAlgorithmArn() != null)
sb.append("AlgorithmArn: ").append(getAlgorithmArn()).append(",");
if (getForecastHorizon() != null)
sb.append("ForecastHorizon: ").append(getForecastHorizon()).append(",");
if (getForecastTypes() != null)
sb.append("ForecastTypes: ").append(getForecastTypes()).append(",");
if (getPerformAutoML() != null)
sb.append("PerformAutoML: ").append(getPerformAutoML()).append(",");
if (getAutoMLOverrideStrategy() != null)
sb.append("AutoMLOverrideStrategy: ").append(getAutoMLOverrideStrategy()).append(",");
if (getPerformHPO() != null)
sb.append("PerformHPO: ").append(getPerformHPO()).append(",");
if (getTrainingParameters() != null)
sb.append("TrainingParameters: ").append(getTrainingParameters()).append(",");
if (getEvaluationParameters() != null)
sb.append("EvaluationParameters: ").append(getEvaluationParameters()).append(",");
if (getHPOConfig() != null)
sb.append("HPOConfig: ").append(getHPOConfig()).append(",");
if (getInputDataConfig() != null)
sb.append("InputDataConfig: ").append(getInputDataConfig()).append(",");
if (getFeaturizationConfig() != null)
sb.append("FeaturizationConfig: ").append(getFeaturizationConfig()).append(",");
if (getEncryptionConfig() != null)
sb.append("EncryptionConfig: ").append(getEncryptionConfig()).append(",");
if (getTags() != null)
sb.append("Tags: ").append(getTags()).append(",");
if (getOptimizationMetric() != null)
sb.append("OptimizationMetric: ").append(getOptimizationMetric());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof CreatePredictorRequest == false)
return false;
CreatePredictorRequest other = (CreatePredictorRequest) obj;
if (other.getPredictorName() == null ^ this.getPredictorName() == null)
return false;
if (other.getPredictorName() != null && other.getPredictorName().equals(this.getPredictorName()) == false)
return false;
if (other.getAlgorithmArn() == null ^ this.getAlgorithmArn() == null)
return false;
if (other.getAlgorithmArn() != null && other.getAlgorithmArn().equals(this.getAlgorithmArn()) == false)
return false;
if (other.getForecastHorizon() == null ^ this.getForecastHorizon() == null)
return false;
if (other.getForecastHorizon() != null && other.getForecastHorizon().equals(this.getForecastHorizon()) == false)
return false;
if (other.getForecastTypes() == null ^ this.getForecastTypes() == null)
return false;
if (other.getForecastTypes() != null && other.getForecastTypes().equals(this.getForecastTypes()) == false)
return false;
if (other.getPerformAutoML() == null ^ this.getPerformAutoML() == null)
return false;
if (other.getPerformAutoML() != null && other.getPerformAutoML().equals(this.getPerformAutoML()) == false)
return false;
if (other.getAutoMLOverrideStrategy() == null ^ this.getAutoMLOverrideStrategy() == null)
return false;
if (other.getAutoMLOverrideStrategy() != null && other.getAutoMLOverrideStrategy().equals(this.getAutoMLOverrideStrategy()) == false)
return false;
if (other.getPerformHPO() == null ^ this.getPerformHPO() == null)
return false;
if (other.getPerformHPO() != null && other.getPerformHPO().equals(this.getPerformHPO()) == false)
return false;
if (other.getTrainingParameters() == null ^ this.getTrainingParameters() == null)
return false;
if (other.getTrainingParameters() != null && other.getTrainingParameters().equals(this.getTrainingParameters()) == false)
return false;
if (other.getEvaluationParameters() == null ^ this.getEvaluationParameters() == null)
return false;
if (other.getEvaluationParameters() != null && other.getEvaluationParameters().equals(this.getEvaluationParameters()) == false)
return false;
if (other.getHPOConfig() == null ^ this.getHPOConfig() == null)
return false;
if (other.getHPOConfig() != null && other.getHPOConfig().equals(this.getHPOConfig()) == false)
return false;
if (other.getInputDataConfig() == null ^ this.getInputDataConfig() == null)
return false;
if (other.getInputDataConfig() != null && other.getInputDataConfig().equals(this.getInputDataConfig()) == false)
return false;
if (other.getFeaturizationConfig() == null ^ this.getFeaturizationConfig() == null)
return false;
if (other.getFeaturizationConfig() != null && other.getFeaturizationConfig().equals(this.getFeaturizationConfig()) == false)
return false;
if (other.getEncryptionConfig() == null ^ this.getEncryptionConfig() == null)
return false;
if (other.getEncryptionConfig() != null && other.getEncryptionConfig().equals(this.getEncryptionConfig()) == false)
return false;
if (other.getTags() == null ^ this.getTags() == null)
return false;
if (other.getTags() != null && other.getTags().equals(this.getTags()) == false)
return false;
if (other.getOptimizationMetric() == null ^ this.getOptimizationMetric() == null)
return false;
if (other.getOptimizationMetric() != null && other.getOptimizationMetric().equals(this.getOptimizationMetric()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getPredictorName() == null) ? 0 : getPredictorName().hashCode());
hashCode = prime * hashCode + ((getAlgorithmArn() == null) ? 0 : getAlgorithmArn().hashCode());
hashCode = prime * hashCode + ((getForecastHorizon() == null) ? 0 : getForecastHorizon().hashCode());
hashCode = prime * hashCode + ((getForecastTypes() == null) ? 0 : getForecastTypes().hashCode());
hashCode = prime * hashCode + ((getPerformAutoML() == null) ? 0 : getPerformAutoML().hashCode());
hashCode = prime * hashCode + ((getAutoMLOverrideStrategy() == null) ? 0 : getAutoMLOverrideStrategy().hashCode());
hashCode = prime * hashCode + ((getPerformHPO() == null) ? 0 : getPerformHPO().hashCode());
hashCode = prime * hashCode + ((getTrainingParameters() == null) ? 0 : getTrainingParameters().hashCode());
hashCode = prime * hashCode + ((getEvaluationParameters() == null) ? 0 : getEvaluationParameters().hashCode());
hashCode = prime * hashCode + ((getHPOConfig() == null) ? 0 : getHPOConfig().hashCode());
hashCode = prime * hashCode + ((getInputDataConfig() == null) ? 0 : getInputDataConfig().hashCode());
hashCode = prime * hashCode + ((getFeaturizationConfig() == null) ? 0 : getFeaturizationConfig().hashCode());
hashCode = prime * hashCode + ((getEncryptionConfig() == null) ? 0 : getEncryptionConfig().hashCode());
hashCode = prime * hashCode + ((getTags() == null) ? 0 : getTags().hashCode());
hashCode = prime * hashCode + ((getOptimizationMetric() == null) ? 0 : getOptimizationMetric().hashCode());
return hashCode;
}
@Override
public CreatePredictorRequest clone() {
return (CreatePredictorRequest) super.clone();
}
}