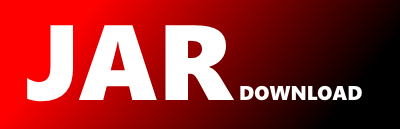
com.amazonaws.services.forecast.model.CreateDatasetGroupRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-forecast Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.forecast.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class CreateDatasetGroupRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* A name for the dataset group.
*
*/
private String datasetGroupName;
/**
*
* The domain associated with the dataset group. When you add a dataset to a dataset group, this value and the value
* specified for the Domain
parameter of the CreateDataset operation must
* match.
*
*
* The Domain
and DatasetType
that you choose determine the fields that must be present in
* training data that you import to a dataset. For example, if you choose the RETAIL
domain and
* TARGET_TIME_SERIES
as the DatasetType
, Amazon Forecast requires that
* item_id
, timestamp
, and demand
fields are present in your data. For more
* information, see Dataset
* groups.
*
*/
private String domain;
/**
*
* An array of Amazon Resource Names (ARNs) of the datasets that you want to include in the dataset group.
*
*/
private java.util.List datasetArns;
/**
*
* The optional metadata that you apply to the dataset group to help you categorize and organize them. Each tag
* consists of a key and an optional value, both of which you define.
*
*
* The following basic restrictions apply to tags:
*
*
* -
*
* Maximum number of tags per resource - 50.
*
*
* -
*
* For each resource, each tag key must be unique, and each tag key can have only one value.
*
*
* -
*
* Maximum key length - 128 Unicode characters in UTF-8.
*
*
* -
*
* Maximum value length - 256 Unicode characters in UTF-8.
*
*
* -
*
* If your tagging schema is used across multiple services and resources, remember that other services may have
* restrictions on allowed characters. Generally allowed characters are: letters, numbers, and spaces representable
* in UTF-8, and the following characters: + - = . _ : / @.
*
*
* -
*
* Tag keys and values are case sensitive.
*
*
* -
*
* Do not use aws:
, AWS:
, or any upper or lowercase combination of such as a prefix for
* keys as it is reserved for Amazon Web Services use. You cannot edit or delete tag keys with this prefix. Values
* can have this prefix. If a tag value has aws
as its prefix but the key does not, then Forecast
* considers it to be a user tag and will count against the limit of 50 tags. Tags with only the key prefix of
* aws
do not count against your tags per resource limit.
*
*
*
*/
private java.util.List tags;
/**
*
* A name for the dataset group.
*
*
* @param datasetGroupName
* A name for the dataset group.
*/
public void setDatasetGroupName(String datasetGroupName) {
this.datasetGroupName = datasetGroupName;
}
/**
*
* A name for the dataset group.
*
*
* @return A name for the dataset group.
*/
public String getDatasetGroupName() {
return this.datasetGroupName;
}
/**
*
* A name for the dataset group.
*
*
* @param datasetGroupName
* A name for the dataset group.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateDatasetGroupRequest withDatasetGroupName(String datasetGroupName) {
setDatasetGroupName(datasetGroupName);
return this;
}
/**
*
* The domain associated with the dataset group. When you add a dataset to a dataset group, this value and the value
* specified for the Domain
parameter of the CreateDataset operation must
* match.
*
*
* The Domain
and DatasetType
that you choose determine the fields that must be present in
* training data that you import to a dataset. For example, if you choose the RETAIL
domain and
* TARGET_TIME_SERIES
as the DatasetType
, Amazon Forecast requires that
* item_id
, timestamp
, and demand
fields are present in your data. For more
* information, see Dataset
* groups.
*
*
* @param domain
* The domain associated with the dataset group. When you add a dataset to a dataset group, this value and
* the value specified for the Domain
parameter of the CreateDataset operation
* must match.
*
* The Domain
and DatasetType
that you choose determine the fields that must be
* present in training data that you import to a dataset. For example, if you choose the RETAIL
* domain and TARGET_TIME_SERIES
as the DatasetType
, Amazon Forecast requires that
* item_id
, timestamp
, and demand
fields are present in your data. For
* more information, see Dataset groups.
* @see Domain
*/
public void setDomain(String domain) {
this.domain = domain;
}
/**
*
* The domain associated with the dataset group. When you add a dataset to a dataset group, this value and the value
* specified for the Domain
parameter of the CreateDataset operation must
* match.
*
*
* The Domain
and DatasetType
that you choose determine the fields that must be present in
* training data that you import to a dataset. For example, if you choose the RETAIL
domain and
* TARGET_TIME_SERIES
as the DatasetType
, Amazon Forecast requires that
* item_id
, timestamp
, and demand
fields are present in your data. For more
* information, see Dataset
* groups.
*
*
* @return The domain associated with the dataset group. When you add a dataset to a dataset group, this value and
* the value specified for the Domain
parameter of the CreateDataset operation
* must match.
*
* The Domain
and DatasetType
that you choose determine the fields that must be
* present in training data that you import to a dataset. For example, if you choose the RETAIL
* domain and TARGET_TIME_SERIES
as the DatasetType
, Amazon Forecast requires that
* item_id
, timestamp
, and demand
fields are present in your data.
* For more information, see Dataset groups.
* @see Domain
*/
public String getDomain() {
return this.domain;
}
/**
*
* The domain associated with the dataset group. When you add a dataset to a dataset group, this value and the value
* specified for the Domain
parameter of the CreateDataset operation must
* match.
*
*
* The Domain
and DatasetType
that you choose determine the fields that must be present in
* training data that you import to a dataset. For example, if you choose the RETAIL
domain and
* TARGET_TIME_SERIES
as the DatasetType
, Amazon Forecast requires that
* item_id
, timestamp
, and demand
fields are present in your data. For more
* information, see Dataset
* groups.
*
*
* @param domain
* The domain associated with the dataset group. When you add a dataset to a dataset group, this value and
* the value specified for the Domain
parameter of the CreateDataset operation
* must match.
*
* The Domain
and DatasetType
that you choose determine the fields that must be
* present in training data that you import to a dataset. For example, if you choose the RETAIL
* domain and TARGET_TIME_SERIES
as the DatasetType
, Amazon Forecast requires that
* item_id
, timestamp
, and demand
fields are present in your data. For
* more information, see Dataset groups.
* @return Returns a reference to this object so that method calls can be chained together.
* @see Domain
*/
public CreateDatasetGroupRequest withDomain(String domain) {
setDomain(domain);
return this;
}
/**
*
* The domain associated with the dataset group. When you add a dataset to a dataset group, this value and the value
* specified for the Domain
parameter of the CreateDataset operation must
* match.
*
*
* The Domain
and DatasetType
that you choose determine the fields that must be present in
* training data that you import to a dataset. For example, if you choose the RETAIL
domain and
* TARGET_TIME_SERIES
as the DatasetType
, Amazon Forecast requires that
* item_id
, timestamp
, and demand
fields are present in your data. For more
* information, see Dataset
* groups.
*
*
* @param domain
* The domain associated with the dataset group. When you add a dataset to a dataset group, this value and
* the value specified for the Domain
parameter of the CreateDataset operation
* must match.
*
* The Domain
and DatasetType
that you choose determine the fields that must be
* present in training data that you import to a dataset. For example, if you choose the RETAIL
* domain and TARGET_TIME_SERIES
as the DatasetType
, Amazon Forecast requires that
* item_id
, timestamp
, and demand
fields are present in your data. For
* more information, see Dataset groups.
* @return Returns a reference to this object so that method calls can be chained together.
* @see Domain
*/
public CreateDatasetGroupRequest withDomain(Domain domain) {
this.domain = domain.toString();
return this;
}
/**
*
* An array of Amazon Resource Names (ARNs) of the datasets that you want to include in the dataset group.
*
*
* @return An array of Amazon Resource Names (ARNs) of the datasets that you want to include in the dataset group.
*/
public java.util.List getDatasetArns() {
return datasetArns;
}
/**
*
* An array of Amazon Resource Names (ARNs) of the datasets that you want to include in the dataset group.
*
*
* @param datasetArns
* An array of Amazon Resource Names (ARNs) of the datasets that you want to include in the dataset group.
*/
public void setDatasetArns(java.util.Collection datasetArns) {
if (datasetArns == null) {
this.datasetArns = null;
return;
}
this.datasetArns = new java.util.ArrayList(datasetArns);
}
/**
*
* An array of Amazon Resource Names (ARNs) of the datasets that you want to include in the dataset group.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setDatasetArns(java.util.Collection)} or {@link #withDatasetArns(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param datasetArns
* An array of Amazon Resource Names (ARNs) of the datasets that you want to include in the dataset group.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateDatasetGroupRequest withDatasetArns(String... datasetArns) {
if (this.datasetArns == null) {
setDatasetArns(new java.util.ArrayList(datasetArns.length));
}
for (String ele : datasetArns) {
this.datasetArns.add(ele);
}
return this;
}
/**
*
* An array of Amazon Resource Names (ARNs) of the datasets that you want to include in the dataset group.
*
*
* @param datasetArns
* An array of Amazon Resource Names (ARNs) of the datasets that you want to include in the dataset group.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateDatasetGroupRequest withDatasetArns(java.util.Collection datasetArns) {
setDatasetArns(datasetArns);
return this;
}
/**
*
* The optional metadata that you apply to the dataset group to help you categorize and organize them. Each tag
* consists of a key and an optional value, both of which you define.
*
*
* The following basic restrictions apply to tags:
*
*
* -
*
* Maximum number of tags per resource - 50.
*
*
* -
*
* For each resource, each tag key must be unique, and each tag key can have only one value.
*
*
* -
*
* Maximum key length - 128 Unicode characters in UTF-8.
*
*
* -
*
* Maximum value length - 256 Unicode characters in UTF-8.
*
*
* -
*
* If your tagging schema is used across multiple services and resources, remember that other services may have
* restrictions on allowed characters. Generally allowed characters are: letters, numbers, and spaces representable
* in UTF-8, and the following characters: + - = . _ : / @.
*
*
* -
*
* Tag keys and values are case sensitive.
*
*
* -
*
* Do not use aws:
, AWS:
, or any upper or lowercase combination of such as a prefix for
* keys as it is reserved for Amazon Web Services use. You cannot edit or delete tag keys with this prefix. Values
* can have this prefix. If a tag value has aws
as its prefix but the key does not, then Forecast
* considers it to be a user tag and will count against the limit of 50 tags. Tags with only the key prefix of
* aws
do not count against your tags per resource limit.
*
*
*
*
* @return The optional metadata that you apply to the dataset group to help you categorize and organize them. Each
* tag consists of a key and an optional value, both of which you define.
*
* The following basic restrictions apply to tags:
*
*
* -
*
* Maximum number of tags per resource - 50.
*
*
* -
*
* For each resource, each tag key must be unique, and each tag key can have only one value.
*
*
* -
*
* Maximum key length - 128 Unicode characters in UTF-8.
*
*
* -
*
* Maximum value length - 256 Unicode characters in UTF-8.
*
*
* -
*
* If your tagging schema is used across multiple services and resources, remember that other services may
* have restrictions on allowed characters. Generally allowed characters are: letters, numbers, and spaces
* representable in UTF-8, and the following characters: + - = . _ : / @.
*
*
* -
*
* Tag keys and values are case sensitive.
*
*
* -
*
* Do not use aws:
, AWS:
, or any upper or lowercase combination of such as a
* prefix for keys as it is reserved for Amazon Web Services use. You cannot edit or delete tag keys with
* this prefix. Values can have this prefix. If a tag value has aws
as its prefix but the key
* does not, then Forecast considers it to be a user tag and will count against the limit of 50 tags. Tags
* with only the key prefix of aws
do not count against your tags per resource limit.
*
*
*/
public java.util.List getTags() {
return tags;
}
/**
*
* The optional metadata that you apply to the dataset group to help you categorize and organize them. Each tag
* consists of a key and an optional value, both of which you define.
*
*
* The following basic restrictions apply to tags:
*
*
* -
*
* Maximum number of tags per resource - 50.
*
*
* -
*
* For each resource, each tag key must be unique, and each tag key can have only one value.
*
*
* -
*
* Maximum key length - 128 Unicode characters in UTF-8.
*
*
* -
*
* Maximum value length - 256 Unicode characters in UTF-8.
*
*
* -
*
* If your tagging schema is used across multiple services and resources, remember that other services may have
* restrictions on allowed characters. Generally allowed characters are: letters, numbers, and spaces representable
* in UTF-8, and the following characters: + - = . _ : / @.
*
*
* -
*
* Tag keys and values are case sensitive.
*
*
* -
*
* Do not use aws:
, AWS:
, or any upper or lowercase combination of such as a prefix for
* keys as it is reserved for Amazon Web Services use. You cannot edit or delete tag keys with this prefix. Values
* can have this prefix. If a tag value has aws
as its prefix but the key does not, then Forecast
* considers it to be a user tag and will count against the limit of 50 tags. Tags with only the key prefix of
* aws
do not count against your tags per resource limit.
*
*
*
*
* @param tags
* The optional metadata that you apply to the dataset group to help you categorize and organize them. Each
* tag consists of a key and an optional value, both of which you define.
*
* The following basic restrictions apply to tags:
*
*
* -
*
* Maximum number of tags per resource - 50.
*
*
* -
*
* For each resource, each tag key must be unique, and each tag key can have only one value.
*
*
* -
*
* Maximum key length - 128 Unicode characters in UTF-8.
*
*
* -
*
* Maximum value length - 256 Unicode characters in UTF-8.
*
*
* -
*
* If your tagging schema is used across multiple services and resources, remember that other services may
* have restrictions on allowed characters. Generally allowed characters are: letters, numbers, and spaces
* representable in UTF-8, and the following characters: + - = . _ : / @.
*
*
* -
*
* Tag keys and values are case sensitive.
*
*
* -
*
* Do not use aws:
, AWS:
, or any upper or lowercase combination of such as a prefix
* for keys as it is reserved for Amazon Web Services use. You cannot edit or delete tag keys with this
* prefix. Values can have this prefix. If a tag value has aws
as its prefix but the key does
* not, then Forecast considers it to be a user tag and will count against the limit of 50 tags. Tags with
* only the key prefix of aws
do not count against your tags per resource limit.
*
*
*/
public void setTags(java.util.Collection tags) {
if (tags == null) {
this.tags = null;
return;
}
this.tags = new java.util.ArrayList(tags);
}
/**
*
* The optional metadata that you apply to the dataset group to help you categorize and organize them. Each tag
* consists of a key and an optional value, both of which you define.
*
*
* The following basic restrictions apply to tags:
*
*
* -
*
* Maximum number of tags per resource - 50.
*
*
* -
*
* For each resource, each tag key must be unique, and each tag key can have only one value.
*
*
* -
*
* Maximum key length - 128 Unicode characters in UTF-8.
*
*
* -
*
* Maximum value length - 256 Unicode characters in UTF-8.
*
*
* -
*
* If your tagging schema is used across multiple services and resources, remember that other services may have
* restrictions on allowed characters. Generally allowed characters are: letters, numbers, and spaces representable
* in UTF-8, and the following characters: + - = . _ : / @.
*
*
* -
*
* Tag keys and values are case sensitive.
*
*
* -
*
* Do not use aws:
, AWS:
, or any upper or lowercase combination of such as a prefix for
* keys as it is reserved for Amazon Web Services use. You cannot edit or delete tag keys with this prefix. Values
* can have this prefix. If a tag value has aws
as its prefix but the key does not, then Forecast
* considers it to be a user tag and will count against the limit of 50 tags. Tags with only the key prefix of
* aws
do not count against your tags per resource limit.
*
*
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTags(java.util.Collection)} or {@link #withTags(java.util.Collection)} if you want to override the
* existing values.
*
*
* @param tags
* The optional metadata that you apply to the dataset group to help you categorize and organize them. Each
* tag consists of a key and an optional value, both of which you define.
*
* The following basic restrictions apply to tags:
*
*
* -
*
* Maximum number of tags per resource - 50.
*
*
* -
*
* For each resource, each tag key must be unique, and each tag key can have only one value.
*
*
* -
*
* Maximum key length - 128 Unicode characters in UTF-8.
*
*
* -
*
* Maximum value length - 256 Unicode characters in UTF-8.
*
*
* -
*
* If your tagging schema is used across multiple services and resources, remember that other services may
* have restrictions on allowed characters. Generally allowed characters are: letters, numbers, and spaces
* representable in UTF-8, and the following characters: + - = . _ : / @.
*
*
* -
*
* Tag keys and values are case sensitive.
*
*
* -
*
* Do not use aws:
, AWS:
, or any upper or lowercase combination of such as a prefix
* for keys as it is reserved for Amazon Web Services use. You cannot edit or delete tag keys with this
* prefix. Values can have this prefix. If a tag value has aws
as its prefix but the key does
* not, then Forecast considers it to be a user tag and will count against the limit of 50 tags. Tags with
* only the key prefix of aws
do not count against your tags per resource limit.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateDatasetGroupRequest withTags(Tag... tags) {
if (this.tags == null) {
setTags(new java.util.ArrayList(tags.length));
}
for (Tag ele : tags) {
this.tags.add(ele);
}
return this;
}
/**
*
* The optional metadata that you apply to the dataset group to help you categorize and organize them. Each tag
* consists of a key and an optional value, both of which you define.
*
*
* The following basic restrictions apply to tags:
*
*
* -
*
* Maximum number of tags per resource - 50.
*
*
* -
*
* For each resource, each tag key must be unique, and each tag key can have only one value.
*
*
* -
*
* Maximum key length - 128 Unicode characters in UTF-8.
*
*
* -
*
* Maximum value length - 256 Unicode characters in UTF-8.
*
*
* -
*
* If your tagging schema is used across multiple services and resources, remember that other services may have
* restrictions on allowed characters. Generally allowed characters are: letters, numbers, and spaces representable
* in UTF-8, and the following characters: + - = . _ : / @.
*
*
* -
*
* Tag keys and values are case sensitive.
*
*
* -
*
* Do not use aws:
, AWS:
, or any upper or lowercase combination of such as a prefix for
* keys as it is reserved for Amazon Web Services use. You cannot edit or delete tag keys with this prefix. Values
* can have this prefix. If a tag value has aws
as its prefix but the key does not, then Forecast
* considers it to be a user tag and will count against the limit of 50 tags. Tags with only the key prefix of
* aws
do not count against your tags per resource limit.
*
*
*
*
* @param tags
* The optional metadata that you apply to the dataset group to help you categorize and organize them. Each
* tag consists of a key and an optional value, both of which you define.
*
* The following basic restrictions apply to tags:
*
*
* -
*
* Maximum number of tags per resource - 50.
*
*
* -
*
* For each resource, each tag key must be unique, and each tag key can have only one value.
*
*
* -
*
* Maximum key length - 128 Unicode characters in UTF-8.
*
*
* -
*
* Maximum value length - 256 Unicode characters in UTF-8.
*
*
* -
*
* If your tagging schema is used across multiple services and resources, remember that other services may
* have restrictions on allowed characters. Generally allowed characters are: letters, numbers, and spaces
* representable in UTF-8, and the following characters: + - = . _ : / @.
*
*
* -
*
* Tag keys and values are case sensitive.
*
*
* -
*
* Do not use aws:
, AWS:
, or any upper or lowercase combination of such as a prefix
* for keys as it is reserved for Amazon Web Services use. You cannot edit or delete tag keys with this
* prefix. Values can have this prefix. If a tag value has aws
as its prefix but the key does
* not, then Forecast considers it to be a user tag and will count against the limit of 50 tags. Tags with
* only the key prefix of aws
do not count against your tags per resource limit.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateDatasetGroupRequest withTags(java.util.Collection tags) {
setTags(tags);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getDatasetGroupName() != null)
sb.append("DatasetGroupName: ").append(getDatasetGroupName()).append(",");
if (getDomain() != null)
sb.append("Domain: ").append(getDomain()).append(",");
if (getDatasetArns() != null)
sb.append("DatasetArns: ").append(getDatasetArns()).append(",");
if (getTags() != null)
sb.append("Tags: ").append(getTags());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof CreateDatasetGroupRequest == false)
return false;
CreateDatasetGroupRequest other = (CreateDatasetGroupRequest) obj;
if (other.getDatasetGroupName() == null ^ this.getDatasetGroupName() == null)
return false;
if (other.getDatasetGroupName() != null && other.getDatasetGroupName().equals(this.getDatasetGroupName()) == false)
return false;
if (other.getDomain() == null ^ this.getDomain() == null)
return false;
if (other.getDomain() != null && other.getDomain().equals(this.getDomain()) == false)
return false;
if (other.getDatasetArns() == null ^ this.getDatasetArns() == null)
return false;
if (other.getDatasetArns() != null && other.getDatasetArns().equals(this.getDatasetArns()) == false)
return false;
if (other.getTags() == null ^ this.getTags() == null)
return false;
if (other.getTags() != null && other.getTags().equals(this.getTags()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getDatasetGroupName() == null) ? 0 : getDatasetGroupName().hashCode());
hashCode = prime * hashCode + ((getDomain() == null) ? 0 : getDomain().hashCode());
hashCode = prime * hashCode + ((getDatasetArns() == null) ? 0 : getDatasetArns().hashCode());
hashCode = prime * hashCode + ((getTags() == null) ? 0 : getTags().hashCode());
return hashCode;
}
@Override
public CreateDatasetGroupRequest clone() {
return (CreateDatasetGroupRequest) super.clone();
}
}