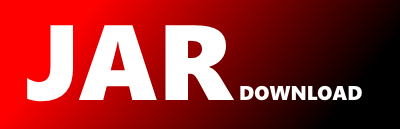
com.amazonaws.services.frauddetector.AmazonFraudDetector Maven / Gradle / Ivy
Show all versions of aws-java-sdk-frauddetector Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.frauddetector;
import javax.annotation.Generated;
import com.amazonaws.*;
import com.amazonaws.regions.*;
import com.amazonaws.services.frauddetector.model.*;
/**
* Interface for accessing Amazon Fraud Detector.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.frauddetector.AbstractAmazonFraudDetector} instead.
*
*
*
* This is the Amazon Fraud Detector API Reference. This guide is for developers who need detailed information about
* Amazon Fraud Detector API actions, data types, and errors. For more information about Amazon Fraud Detector features,
* see the Amazon Fraud Detector User Guide.
*
*
* We provide the Query API as well as AWS software development kits (SDK) for Amazon Fraud Detector in Java and Python
* programming languages.
*
*
* The Amazon Fraud Detector Query API provides HTTPS requests that use the HTTP verb GET or POST and a Query parameter
* Action
. AWS SDK provides libraries, sample code, tutorials, and other resources for software developers
* who prefer to build applications using language-specific APIs instead of submitting a request over HTTP or HTTPS.
* These libraries provide basic functions that automatically take care of tasks such as cryptographically signing your
* requests, retrying requests, and handling error responses, so that it is easier for you to get started. For more
* information about the AWS SDKs, go to Tools to build on AWS
* page, scroll down to the SDK section, and choose plus (+) sign to expand the section.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AmazonFraudDetector {
/**
* The region metadata service name for computing region endpoints. You can use this value to retrieve metadata
* (such as supported regions) of the service.
*
* @see RegionUtils#getRegionsForService(String)
*/
String ENDPOINT_PREFIX = "frauddetector";
/**
*
* Creates a batch of variables.
*
*
* @param batchCreateVariableRequest
* @return Result of the BatchCreateVariable operation returned by the service.
* @throws ValidationException
* An exception indicating a specified value is not allowed.
* @throws InternalServerException
* An exception indicating an internal server error.
* @throws ThrottlingException
* An exception indicating a throttling error.
* @throws AccessDeniedException
* An exception indicating Amazon Fraud Detector does not have the needed permissions. This can occur if you
* submit a request, such as PutExternalModel
, that specifies a role that is not in your
* account.
* @sample AmazonFraudDetector.BatchCreateVariable
* @see AWS API Documentation
*/
BatchCreateVariableResult batchCreateVariable(BatchCreateVariableRequest batchCreateVariableRequest);
/**
*
* Gets a batch of variables.
*
*
* @param batchGetVariableRequest
* @return Result of the BatchGetVariable operation returned by the service.
* @throws ValidationException
* An exception indicating a specified value is not allowed.
* @throws InternalServerException
* An exception indicating an internal server error.
* @throws ThrottlingException
* An exception indicating a throttling error.
* @throws AccessDeniedException
* An exception indicating Amazon Fraud Detector does not have the needed permissions. This can occur if you
* submit a request, such as PutExternalModel
, that specifies a role that is not in your
* account.
* @sample AmazonFraudDetector.BatchGetVariable
* @see AWS
* API Documentation
*/
BatchGetVariableResult batchGetVariable(BatchGetVariableRequest batchGetVariableRequest);
/**
*
* Cancels an in-progress batch import job.
*
*
* @param cancelBatchImportJobRequest
* @return Result of the CancelBatchImportJob operation returned by the service.
* @throws ValidationException
* An exception indicating a specified value is not allowed.
* @throws ResourceNotFoundException
* An exception indicating the specified resource was not found.
* @throws InternalServerException
* An exception indicating an internal server error.
* @throws ThrottlingException
* An exception indicating a throttling error.
* @throws AccessDeniedException
* An exception indicating Amazon Fraud Detector does not have the needed permissions. This can occur if you
* submit a request, such as PutExternalModel
, that specifies a role that is not in your
* account.
* @sample AmazonFraudDetector.CancelBatchImportJob
* @see AWS API Documentation
*/
CancelBatchImportJobResult cancelBatchImportJob(CancelBatchImportJobRequest cancelBatchImportJobRequest);
/**
*
* Cancels the specified batch prediction job.
*
*
* @param cancelBatchPredictionJobRequest
* @return Result of the CancelBatchPredictionJob operation returned by the service.
* @throws ValidationException
* An exception indicating a specified value is not allowed.
* @throws ResourceNotFoundException
* An exception indicating the specified resource was not found.
* @throws InternalServerException
* An exception indicating an internal server error.
* @throws ThrottlingException
* An exception indicating a throttling error.
* @throws AccessDeniedException
* An exception indicating Amazon Fraud Detector does not have the needed permissions. This can occur if you
* submit a request, such as PutExternalModel
, that specifies a role that is not in your
* account.
* @sample AmazonFraudDetector.CancelBatchPredictionJob
* @see AWS API Documentation
*/
CancelBatchPredictionJobResult cancelBatchPredictionJob(CancelBatchPredictionJobRequest cancelBatchPredictionJobRequest);
/**
*
* Creates a batch import job.
*
*
* @param createBatchImportJobRequest
* @return Result of the CreateBatchImportJob operation returned by the service.
* @throws ValidationException
* An exception indicating a specified value is not allowed.
* @throws ResourceNotFoundException
* An exception indicating the specified resource was not found.
* @throws InternalServerException
* An exception indicating an internal server error.
* @throws ThrottlingException
* An exception indicating a throttling error.
* @throws AccessDeniedException
* An exception indicating Amazon Fraud Detector does not have the needed permissions. This can occur if you
* submit a request, such as PutExternalModel
, that specifies a role that is not in your
* account.
* @sample AmazonFraudDetector.CreateBatchImportJob
* @see AWS API Documentation
*/
CreateBatchImportJobResult createBatchImportJob(CreateBatchImportJobRequest createBatchImportJobRequest);
/**
*
* Creates a batch prediction job.
*
*
* @param createBatchPredictionJobRequest
* @return Result of the CreateBatchPredictionJob operation returned by the service.
* @throws ValidationException
* An exception indicating a specified value is not allowed.
* @throws InternalServerException
* An exception indicating an internal server error.
* @throws ThrottlingException
* An exception indicating a throttling error.
* @throws AccessDeniedException
* An exception indicating Amazon Fraud Detector does not have the needed permissions. This can occur if you
* submit a request, such as PutExternalModel
, that specifies a role that is not in your
* account.
* @throws ResourceNotFoundException
* An exception indicating the specified resource was not found.
* @sample AmazonFraudDetector.CreateBatchPredictionJob
* @see AWS API Documentation
*/
CreateBatchPredictionJobResult createBatchPredictionJob(CreateBatchPredictionJobRequest createBatchPredictionJobRequest);
/**
*
* Creates a detector version. The detector version starts in a DRAFT
status.
*
*
* @param createDetectorVersionRequest
* @return Result of the CreateDetectorVersion operation returned by the service.
* @throws ValidationException
* An exception indicating a specified value is not allowed.
* @throws ResourceNotFoundException
* An exception indicating the specified resource was not found.
* @throws InternalServerException
* An exception indicating an internal server error.
* @throws ThrottlingException
* An exception indicating a throttling error.
* @throws AccessDeniedException
* An exception indicating Amazon Fraud Detector does not have the needed permissions. This can occur if you
* submit a request, such as PutExternalModel
, that specifies a role that is not in your
* account.
* @sample AmazonFraudDetector.CreateDetectorVersion
* @see AWS API Documentation
*/
CreateDetectorVersionResult createDetectorVersion(CreateDetectorVersionRequest createDetectorVersionRequest);
/**
*
* Creates a list.
*
*
* List is a set of input data for a variable in your event dataset. You use the input data in a rule that's
* associated with your detector. For more information, see Lists.
*
*
* @param createListRequest
* @return Result of the CreateList operation returned by the service.
* @throws ValidationException
* An exception indicating a specified value is not allowed.
* @throws InternalServerException
* An exception indicating an internal server error.
* @throws ThrottlingException
* An exception indicating a throttling error.
* @throws AccessDeniedException
* An exception indicating Amazon Fraud Detector does not have the needed permissions. This can occur if you
* submit a request, such as PutExternalModel
, that specifies a role that is not in your
* account.
* @sample AmazonFraudDetector.CreateList
* @see AWS API
* Documentation
*/
CreateListResult createList(CreateListRequest createListRequest);
/**
*
* Creates a model using the specified model type.
*
*
* @param createModelRequest
* @return Result of the CreateModel operation returned by the service.
* @throws ValidationException
* An exception indicating a specified value is not allowed.
* @throws InternalServerException
* An exception indicating an internal server error.
* @throws ThrottlingException
* An exception indicating a throttling error.
* @throws AccessDeniedException
* An exception indicating Amazon Fraud Detector does not have the needed permissions. This can occur if you
* submit a request, such as PutExternalModel
, that specifies a role that is not in your
* account.
* @sample AmazonFraudDetector.CreateModel
* @see AWS API
* Documentation
*/
CreateModelResult createModel(CreateModelRequest createModelRequest);
/**
*
* Creates a version of the model using the specified model type and model id.
*
*
* @param createModelVersionRequest
* @return Result of the CreateModelVersion operation returned by the service.
* @throws ValidationException
* An exception indicating a specified value is not allowed.
* @throws ResourceNotFoundException
* An exception indicating the specified resource was not found.
* @throws AccessDeniedException
* An exception indicating Amazon Fraud Detector does not have the needed permissions. This can occur if you
* submit a request, such as PutExternalModel
, that specifies a role that is not in your
* account.
* @throws ThrottlingException
* An exception indicating a throttling error.
* @throws InternalServerException
* An exception indicating an internal server error.
* @sample AmazonFraudDetector.CreateModelVersion
* @see AWS API Documentation
*/
CreateModelVersionResult createModelVersion(CreateModelVersionRequest createModelVersionRequest);
/**
*
* Creates a rule for use with the specified detector.
*
*
* @param createRuleRequest
* @return Result of the CreateRule operation returned by the service.
* @throws ValidationException
* An exception indicating a specified value is not allowed.
* @throws InternalServerException
* An exception indicating an internal server error.
* @throws ThrottlingException
* An exception indicating a throttling error.
* @throws AccessDeniedException
* An exception indicating Amazon Fraud Detector does not have the needed permissions. This can occur if you
* submit a request, such as PutExternalModel
, that specifies a role that is not in your
* account.
* @sample AmazonFraudDetector.CreateRule
* @see AWS API
* Documentation
*/
CreateRuleResult createRule(CreateRuleRequest createRuleRequest);
/**
*
* Creates a variable.
*
*
* @param createVariableRequest
* @return Result of the CreateVariable operation returned by the service.
* @throws ValidationException
* An exception indicating a specified value is not allowed.
* @throws InternalServerException
* An exception indicating an internal server error.
* @throws ThrottlingException
* An exception indicating a throttling error.
* @throws AccessDeniedException
* An exception indicating Amazon Fraud Detector does not have the needed permissions. This can occur if you
* submit a request, such as PutExternalModel
, that specifies a role that is not in your
* account.
* @sample AmazonFraudDetector.CreateVariable
* @see AWS
* API Documentation
*/
CreateVariableResult createVariable(CreateVariableRequest createVariableRequest);
/**
*
* Deletes the specified batch import job ID record. This action does not delete the data that was batch imported.
*
*
* @param deleteBatchImportJobRequest
* @return Result of the DeleteBatchImportJob operation returned by the service.
* @throws ValidationException
* An exception indicating a specified value is not allowed.
* @throws InternalServerException
* An exception indicating an internal server error.
* @throws ThrottlingException
* An exception indicating a throttling error.
* @throws AccessDeniedException
* An exception indicating Amazon Fraud Detector does not have the needed permissions. This can occur if you
* submit a request, such as PutExternalModel
, that specifies a role that is not in your
* account.
* @sample AmazonFraudDetector.DeleteBatchImportJob
* @see AWS API Documentation
*/
DeleteBatchImportJobResult deleteBatchImportJob(DeleteBatchImportJobRequest deleteBatchImportJobRequest);
/**
*
* Deletes a batch prediction job.
*
*
* @param deleteBatchPredictionJobRequest
* @return Result of the DeleteBatchPredictionJob operation returned by the service.
* @throws ValidationException
* An exception indicating a specified value is not allowed.
* @throws InternalServerException
* An exception indicating an internal server error.
* @throws ThrottlingException
* An exception indicating a throttling error.
* @throws AccessDeniedException
* An exception indicating Amazon Fraud Detector does not have the needed permissions. This can occur if you
* submit a request, such as PutExternalModel
, that specifies a role that is not in your
* account.
* @sample AmazonFraudDetector.DeleteBatchPredictionJob
* @see AWS API Documentation
*/
DeleteBatchPredictionJobResult deleteBatchPredictionJob(DeleteBatchPredictionJobRequest deleteBatchPredictionJobRequest);
/**
*
* Deletes the detector. Before deleting a detector, you must first delete all detector versions and rule versions
* associated with the detector.
*
*
* When you delete a detector, Amazon Fraud Detector permanently deletes the detector and the data is no longer
* stored in Amazon Fraud Detector.
*
*
* @param deleteDetectorRequest
* @return Result of the DeleteDetector operation returned by the service.
* @throws ConflictException
* An exception indicating there was a conflict during a delete operation.
* @throws ValidationException
* An exception indicating a specified value is not allowed.
* @throws InternalServerException
* An exception indicating an internal server error.
* @throws ThrottlingException
* An exception indicating a throttling error.
* @throws AccessDeniedException
* An exception indicating Amazon Fraud Detector does not have the needed permissions. This can occur if you
* submit a request, such as PutExternalModel
, that specifies a role that is not in your
* account.
* @sample AmazonFraudDetector.DeleteDetector
* @see AWS
* API Documentation
*/
DeleteDetectorResult deleteDetector(DeleteDetectorRequest deleteDetectorRequest);
/**
*
* Deletes the detector version. You cannot delete detector versions that are in ACTIVE
status.
*
*
* When you delete a detector version, Amazon Fraud Detector permanently deletes the detector and the data is no
* longer stored in Amazon Fraud Detector.
*
*
* @param deleteDetectorVersionRequest
* @return Result of the DeleteDetectorVersion operation returned by the service.
* @throws ValidationException
* An exception indicating a specified value is not allowed.
* @throws ResourceNotFoundException
* An exception indicating the specified resource was not found.
* @throws InternalServerException
* An exception indicating an internal server error.
* @throws ThrottlingException
* An exception indicating a throttling error.
* @throws ConflictException
* An exception indicating there was a conflict during a delete operation.
* @throws AccessDeniedException
* An exception indicating Amazon Fraud Detector does not have the needed permissions. This can occur if you
* submit a request, such as PutExternalModel
, that specifies a role that is not in your
* account.
* @sample AmazonFraudDetector.DeleteDetectorVersion
* @see AWS API Documentation
*/
DeleteDetectorVersionResult deleteDetectorVersion(DeleteDetectorVersionRequest deleteDetectorVersionRequest);
/**
*
* Deletes an entity type.
*
*
* You cannot delete an entity type that is included in an event type.
*
*
* When you delete an entity type, Amazon Fraud Detector permanently deletes that entity type and the data is no
* longer stored in Amazon Fraud Detector.
*
*
* @param deleteEntityTypeRequest
* @return Result of the DeleteEntityType operation returned by the service.
* @throws ValidationException
* An exception indicating a specified value is not allowed.
* @throws ConflictException
* An exception indicating there was a conflict during a delete operation.
* @throws InternalServerException
* An exception indicating an internal server error.
* @throws ThrottlingException
* An exception indicating a throttling error.
* @throws AccessDeniedException
* An exception indicating Amazon Fraud Detector does not have the needed permissions. This can occur if you
* submit a request, such as PutExternalModel
, that specifies a role that is not in your
* account.
* @sample AmazonFraudDetector.DeleteEntityType
* @see AWS
* API Documentation
*/
DeleteEntityTypeResult deleteEntityType(DeleteEntityTypeRequest deleteEntityTypeRequest);
/**
*
* Deletes the specified event.
*
*
* When you delete an event, Amazon Fraud Detector permanently deletes that event and the event data is no longer
* stored in Amazon Fraud Detector. If deleteAuditHistory
is True
, event data is available
* through search for up to 30 seconds after the delete operation is completed.
*
*
* @param deleteEventRequest
* @return Result of the DeleteEvent operation returned by the service.
* @throws InternalServerException
* An exception indicating an internal server error.
* @throws ThrottlingException
* An exception indicating a throttling error.
* @throws AccessDeniedException
* An exception indicating Amazon Fraud Detector does not have the needed permissions. This can occur if you
* submit a request, such as PutExternalModel
, that specifies a role that is not in your
* account.
* @throws ValidationException
* An exception indicating a specified value is not allowed.
* @sample AmazonFraudDetector.DeleteEvent
* @see AWS API
* Documentation
*/
DeleteEventResult deleteEvent(DeleteEventRequest deleteEventRequest);
/**
*
* Deletes an event type.
*
*
* You cannot delete an event type that is used in a detector or a model.
*
*
* When you delete an event type, Amazon Fraud Detector permanently deletes that event type and the data is no
* longer stored in Amazon Fraud Detector.
*
*
* @param deleteEventTypeRequest
* @return Result of the DeleteEventType operation returned by the service.
* @throws ConflictException
* An exception indicating there was a conflict during a delete operation.
* @throws ValidationException
* An exception indicating a specified value is not allowed.
* @throws InternalServerException
* An exception indicating an internal server error.
* @throws ThrottlingException
* An exception indicating a throttling error.
* @throws AccessDeniedException
* An exception indicating Amazon Fraud Detector does not have the needed permissions. This can occur if you
* submit a request, such as PutExternalModel
, that specifies a role that is not in your
* account.
* @sample AmazonFraudDetector.DeleteEventType
* @see AWS
* API Documentation
*/
DeleteEventTypeResult deleteEventType(DeleteEventTypeRequest deleteEventTypeRequest);
/**
*
* Deletes all events of a particular event type.
*
*
* @param deleteEventsByEventTypeRequest
* @return Result of the DeleteEventsByEventType operation returned by the service.
* @throws ConflictException
* An exception indicating there was a conflict during a delete operation.
* @throws ValidationException
* An exception indicating a specified value is not allowed.
* @throws ResourceNotFoundException
* An exception indicating the specified resource was not found.
* @throws InternalServerException
* An exception indicating an internal server error.
* @throws ThrottlingException
* An exception indicating a throttling error.
* @throws AccessDeniedException
* An exception indicating Amazon Fraud Detector does not have the needed permissions. This can occur if you
* submit a request, such as PutExternalModel
, that specifies a role that is not in your
* account.
* @sample AmazonFraudDetector.DeleteEventsByEventType
* @see AWS API Documentation
*/
DeleteEventsByEventTypeResult deleteEventsByEventType(DeleteEventsByEventTypeRequest deleteEventsByEventTypeRequest);
/**
*
* Removes a SageMaker model from Amazon Fraud Detector.
*
*
* You can remove an Amazon SageMaker model if it is not associated with a detector version. Removing a SageMaker
* model disconnects it from Amazon Fraud Detector, but the model remains available in SageMaker.
*
*
* @param deleteExternalModelRequest
* @return Result of the DeleteExternalModel operation returned by the service.
* @throws ValidationException
* An exception indicating a specified value is not allowed.
* @throws ConflictException
* An exception indicating there was a conflict during a delete operation.
* @throws InternalServerException
* An exception indicating an internal server error.
* @throws ThrottlingException
* An exception indicating a throttling error.
* @throws AccessDeniedException
* An exception indicating Amazon Fraud Detector does not have the needed permissions. This can occur if you
* submit a request, such as PutExternalModel
, that specifies a role that is not in your
* account.
* @sample AmazonFraudDetector.DeleteExternalModel
* @see AWS API Documentation
*/
DeleteExternalModelResult deleteExternalModel(DeleteExternalModelRequest deleteExternalModelRequest);
/**
*
* Deletes a label.
*
*
* You cannot delete labels that are included in an event type in Amazon Fraud Detector.
*
*
* You cannot delete a label assigned to an event ID. You must first delete the relevant event ID.
*
*
* When you delete a label, Amazon Fraud Detector permanently deletes that label and the data is no longer stored in
* Amazon Fraud Detector.
*
*
* @param deleteLabelRequest
* @return Result of the DeleteLabel operation returned by the service.
* @throws ValidationException
* An exception indicating a specified value is not allowed.
* @throws ConflictException
* An exception indicating there was a conflict during a delete operation.
* @throws ThrottlingException
* An exception indicating a throttling error.
* @throws InternalServerException
* An exception indicating an internal server error.
* @sample AmazonFraudDetector.DeleteLabel
* @see AWS API
* Documentation
*/
DeleteLabelResult deleteLabel(DeleteLabelRequest deleteLabelRequest);
/**
*
* Deletes the list, provided it is not used in a rule.
*
*
* When you delete a list, Amazon Fraud Detector permanently deletes that list and the elements in the list.
*
*
* @param deleteListRequest
* @return Result of the DeleteList operation returned by the service.
* @throws ValidationException
* An exception indicating a specified value is not allowed.
* @throws InternalServerException
* An exception indicating an internal server error.
* @throws ThrottlingException
* An exception indicating a throttling error.
* @throws AccessDeniedException
* An exception indicating Amazon Fraud Detector does not have the needed permissions. This can occur if you
* submit a request, such as PutExternalModel
, that specifies a role that is not in your
* account.
* @throws ConflictException
* An exception indicating there was a conflict during a delete operation.
* @sample AmazonFraudDetector.DeleteList
* @see AWS API
* Documentation
*/
DeleteListResult deleteList(DeleteListRequest deleteListRequest);
/**
*
* Deletes a model.
*
*
* You can delete models and model versions in Amazon Fraud Detector, provided that they are not associated with a
* detector version.
*
*
* When you delete a model, Amazon Fraud Detector permanently deletes that model and the data is no longer stored in
* Amazon Fraud Detector.
*
*
* @param deleteModelRequest
* @return Result of the DeleteModel operation returned by the service.
* @throws ConflictException
* An exception indicating there was a conflict during a delete operation.
* @throws ValidationException
* An exception indicating a specified value is not allowed.
* @throws InternalServerException
* An exception indicating an internal server error.
* @throws ThrottlingException
* An exception indicating a throttling error.
* @throws AccessDeniedException
* An exception indicating Amazon Fraud Detector does not have the needed permissions. This can occur if you
* submit a request, such as PutExternalModel
, that specifies a role that is not in your
* account.
* @sample AmazonFraudDetector.DeleteModel
* @see AWS API
* Documentation
*/
DeleteModelResult deleteModel(DeleteModelRequest deleteModelRequest);
/**
*
* Deletes a model version.
*
*
* You can delete models and model versions in Amazon Fraud Detector, provided that they are not associated with a
* detector version.
*
*
* When you delete a model version, Amazon Fraud Detector permanently deletes that model version and the data is no
* longer stored in Amazon Fraud Detector.
*
*
* @param deleteModelVersionRequest
* @return Result of the DeleteModelVersion operation returned by the service.
* @throws ValidationException
* An exception indicating a specified value is not allowed.
* @throws InternalServerException
* An exception indicating an internal server error.
* @throws AccessDeniedException
* An exception indicating Amazon Fraud Detector does not have the needed permissions. This can occur if you
* submit a request, such as PutExternalModel
, that specifies a role that is not in your
* account.
* @throws ThrottlingException
* An exception indicating a throttling error.
* @throws ConflictException
* An exception indicating there was a conflict during a delete operation.
* @sample AmazonFraudDetector.DeleteModelVersion
* @see AWS API Documentation
*/
DeleteModelVersionResult deleteModelVersion(DeleteModelVersionRequest deleteModelVersionRequest);
/**
*
* Deletes an outcome.
*
*
* You cannot delete an outcome that is used in a rule version.
*
*
* When you delete an outcome, Amazon Fraud Detector permanently deletes that outcome and the data is no longer
* stored in Amazon Fraud Detector.
*
*
* @param deleteOutcomeRequest
* @return Result of the DeleteOutcome operation returned by the service.
* @throws ValidationException
* An exception indicating a specified value is not allowed.
* @throws InternalServerException
* An exception indicating an internal server error.
* @throws ThrottlingException
* An exception indicating a throttling error.
* @throws ConflictException
* An exception indicating there was a conflict during a delete operation.
* @throws AccessDeniedException
* An exception indicating Amazon Fraud Detector does not have the needed permissions. This can occur if you
* submit a request, such as PutExternalModel
, that specifies a role that is not in your
* account.
* @sample AmazonFraudDetector.DeleteOutcome
* @see AWS
* API Documentation
*/
DeleteOutcomeResult deleteOutcome(DeleteOutcomeRequest deleteOutcomeRequest);
/**
*
* Deletes the rule. You cannot delete a rule if it is used by an ACTIVE
or INACTIVE
* detector version.
*
*
* When you delete a rule, Amazon Fraud Detector permanently deletes that rule and the data is no longer stored in
* Amazon Fraud Detector.
*
*
* @param deleteRuleRequest
* @return Result of the DeleteRule operation returned by the service.
* @throws ConflictException
* An exception indicating there was a conflict during a delete operation.
* @throws ValidationException
* An exception indicating a specified value is not allowed.
* @throws InternalServerException
* An exception indicating an internal server error.
* @throws ThrottlingException
* An exception indicating a throttling error.
* @throws AccessDeniedException
* An exception indicating Amazon Fraud Detector does not have the needed permissions. This can occur if you
* submit a request, such as PutExternalModel
, that specifies a role that is not in your
* account.
* @sample AmazonFraudDetector.DeleteRule
* @see AWS API
* Documentation
*/
DeleteRuleResult deleteRule(DeleteRuleRequest deleteRuleRequest);
/**
*
* Deletes a variable.
*
*
* You can't delete variables that are included in an event type in Amazon Fraud Detector.
*
*
* Amazon Fraud Detector automatically deletes model output variables and SageMaker model output variables when you
* delete the model. You can't delete these variables manually.
*
*
* When you delete a variable, Amazon Fraud Detector permanently deletes that variable and the data is no longer
* stored in Amazon Fraud Detector.
*
*
* @param deleteVariableRequest
* @return Result of the DeleteVariable operation returned by the service.
* @throws ValidationException
* An exception indicating a specified value is not allowed.
* @throws ConflictException
* An exception indicating there was a conflict during a delete operation.
* @throws InternalServerException
* An exception indicating an internal server error.
* @throws ThrottlingException
* An exception indicating a throttling error.
* @throws AccessDeniedException
* An exception indicating Amazon Fraud Detector does not have the needed permissions. This can occur if you
* submit a request, such as PutExternalModel
, that specifies a role that is not in your
* account.
* @sample AmazonFraudDetector.DeleteVariable
* @see AWS
* API Documentation
*/
DeleteVariableResult deleteVariable(DeleteVariableRequest deleteVariableRequest);
/**
*
* Gets all versions for a specified detector.
*
*
* @param describeDetectorRequest
* @return Result of the DescribeDetector operation returned by the service.
* @throws ValidationException
* An exception indicating a specified value is not allowed.
* @throws ResourceNotFoundException
* An exception indicating the specified resource was not found.
* @throws InternalServerException
* An exception indicating an internal server error.
* @throws ThrottlingException
* An exception indicating a throttling error.
* @throws AccessDeniedException
* An exception indicating Amazon Fraud Detector does not have the needed permissions. This can occur if you
* submit a request, such as PutExternalModel
, that specifies a role that is not in your
* account.
* @sample AmazonFraudDetector.DescribeDetector
* @see AWS
* API Documentation
*/
DescribeDetectorResult describeDetector(DescribeDetectorRequest describeDetectorRequest);
/**
*
* Gets all of the model versions for the specified model type or for the specified model type and model ID. You can
* also get details for a single, specified model version.
*
*
* @param describeModelVersionsRequest
* @return Result of the DescribeModelVersions operation returned by the service.
* @throws ValidationException
* An exception indicating a specified value is not allowed.
* @throws ResourceNotFoundException
* An exception indicating the specified resource was not found.
* @throws InternalServerException
* An exception indicating an internal server error.
* @throws ThrottlingException
* An exception indicating a throttling error.
* @throws AccessDeniedException
* An exception indicating Amazon Fraud Detector does not have the needed permissions. This can occur if you
* submit a request, such as PutExternalModel
, that specifies a role that is not in your
* account.
* @sample AmazonFraudDetector.DescribeModelVersions
* @see AWS API Documentation
*/
DescribeModelVersionsResult describeModelVersions(DescribeModelVersionsRequest describeModelVersionsRequest);
/**
*
* Gets all batch import jobs or a specific job of the specified ID. This is a paginated API. If you provide a null
* maxResults
, this action retrieves a maximum of 50 records per page. If you provide a
* maxResults
, the value must be between 1 and 50. To get the next page results, provide the pagination
* token from the GetBatchImportJobsResponse
as part of your request. A null pagination token fetches
* the records from the beginning.
*
*
* @param getBatchImportJobsRequest
* @return Result of the GetBatchImportJobs operation returned by the service.
* @throws ValidationException
* An exception indicating a specified value is not allowed.
* @throws ResourceNotFoundException
* An exception indicating the specified resource was not found.
* @throws InternalServerException
* An exception indicating an internal server error.
* @throws ThrottlingException
* An exception indicating a throttling error.
* @throws AccessDeniedException
* An exception indicating Amazon Fraud Detector does not have the needed permissions. This can occur if you
* submit a request, such as PutExternalModel
, that specifies a role that is not in your
* account.
* @sample AmazonFraudDetector.GetBatchImportJobs
* @see AWS API Documentation
*/
GetBatchImportJobsResult getBatchImportJobs(GetBatchImportJobsRequest getBatchImportJobsRequest);
/**
*
* Gets all batch prediction jobs or a specific job if you specify a job ID. This is a paginated API. If you provide
* a null maxResults, this action retrieves a maximum of 50 records per page. If you provide a maxResults, the value
* must be between 1 and 50. To get the next page results, provide the pagination token from the
* GetBatchPredictionJobsResponse as part of your request. A null pagination token fetches the records from the
* beginning.
*
*
* @param getBatchPredictionJobsRequest
* @return Result of the GetBatchPredictionJobs operation returned by the service.
* @throws ValidationException
* An exception indicating a specified value is not allowed.
* @throws ResourceNotFoundException
* An exception indicating the specified resource was not found.
* @throws InternalServerException
* An exception indicating an internal server error.
* @throws ThrottlingException
* An exception indicating a throttling error.
* @throws AccessDeniedException
* An exception indicating Amazon Fraud Detector does not have the needed permissions. This can occur if you
* submit a request, such as PutExternalModel
, that specifies a role that is not in your
* account.
* @sample AmazonFraudDetector.GetBatchPredictionJobs
* @see AWS API Documentation
*/
GetBatchPredictionJobsResult getBatchPredictionJobs(GetBatchPredictionJobsRequest getBatchPredictionJobsRequest);
/**
*
* Retrieves the status of a DeleteEventsByEventType
action.
*
*
* @param getDeleteEventsByEventTypeStatusRequest
* @return Result of the GetDeleteEventsByEventTypeStatus operation returned by the service.
* @throws ValidationException
* An exception indicating a specified value is not allowed.
* @throws ResourceNotFoundException
* An exception indicating the specified resource was not found.
* @throws InternalServerException
* An exception indicating an internal server error.
* @throws ThrottlingException
* An exception indicating a throttling error.
* @throws AccessDeniedException
* An exception indicating Amazon Fraud Detector does not have the needed permissions. This can occur if you
* submit a request, such as PutExternalModel
, that specifies a role that is not in your
* account.
* @sample AmazonFraudDetector.GetDeleteEventsByEventTypeStatus
* @see AWS API Documentation
*/
GetDeleteEventsByEventTypeStatusResult getDeleteEventsByEventTypeStatus(GetDeleteEventsByEventTypeStatusRequest getDeleteEventsByEventTypeStatusRequest);
/**
*
* Gets a particular detector version.
*
*
* @param getDetectorVersionRequest
* @return Result of the GetDetectorVersion operation returned by the service.
* @throws ValidationException
* An exception indicating a specified value is not allowed.
* @throws ResourceNotFoundException
* An exception indicating the specified resource was not found.
* @throws InternalServerException
* An exception indicating an internal server error.
* @throws ThrottlingException
* An exception indicating a throttling error.
* @throws AccessDeniedException
* An exception indicating Amazon Fraud Detector does not have the needed permissions. This can occur if you
* submit a request, such as PutExternalModel
, that specifies a role that is not in your
* account.
* @sample AmazonFraudDetector.GetDetectorVersion
* @see AWS API Documentation
*/
GetDetectorVersionResult getDetectorVersion(GetDetectorVersionRequest getDetectorVersionRequest);
/**
*
* Gets all detectors or a single detector if a detectorId
is specified. This is a paginated API. If
* you provide a null maxResults
, this action retrieves a maximum of 10 records per page. If you
* provide a maxResults
, the value must be between 5 and 10. To get the next page results, provide the
* pagination token from the GetDetectorsResponse
as part of your request. A null pagination token
* fetches the records from the beginning.
*
*
* @param getDetectorsRequest
* @return Result of the GetDetectors operation returned by the service.
* @throws ValidationException
* An exception indicating a specified value is not allowed.
* @throws ResourceNotFoundException
* An exception indicating the specified resource was not found.
* @throws InternalServerException
* An exception indicating an internal server error.
* @throws ThrottlingException
* An exception indicating a throttling error.
* @throws AccessDeniedException
* An exception indicating Amazon Fraud Detector does not have the needed permissions. This can occur if you
* submit a request, such as PutExternalModel
, that specifies a role that is not in your
* account.
* @sample AmazonFraudDetector.GetDetectors
* @see AWS API
* Documentation
*/
GetDetectorsResult getDetectors(GetDetectorsRequest getDetectorsRequest);
/**
*
* Gets all entity types or a specific entity type if a name is specified. This is a paginated API. If you provide a
* null maxResults
, this action retrieves a maximum of 10 records per page. If you provide a
* maxResults
, the value must be between 5 and 10. To get the next page results, provide the pagination
* token from the GetEntityTypesResponse
as part of your request. A null pagination token fetches the
* records from the beginning.
*
*
* @param getEntityTypesRequest
* @return Result of the GetEntityTypes operation returned by the service.
* @throws ValidationException
* An exception indicating a specified value is not allowed.
* @throws ResourceNotFoundException
* An exception indicating the specified resource was not found.
* @throws InternalServerException
* An exception indicating an internal server error.
* @throws ThrottlingException
* An exception indicating a throttling error.
* @throws AccessDeniedException
* An exception indicating Amazon Fraud Detector does not have the needed permissions. This can occur if you
* submit a request, such as PutExternalModel
, that specifies a role that is not in your
* account.
* @sample AmazonFraudDetector.GetEntityTypes
* @see AWS
* API Documentation
*/
GetEntityTypesResult getEntityTypes(GetEntityTypesRequest getEntityTypesRequest);
/**
*
* Retrieves details of events stored with Amazon Fraud Detector. This action does not retrieve prediction results.
*
*
* @param getEventRequest
* @return Result of the GetEvent operation returned by the service.
* @throws ValidationException
* An exception indicating a specified value is not allowed.
* @throws ResourceNotFoundException
* An exception indicating the specified resource was not found.
* @throws InternalServerException
* An exception indicating an internal server error.
* @throws ThrottlingException
* An exception indicating a throttling error.
* @throws AccessDeniedException
* An exception indicating Amazon Fraud Detector does not have the needed permissions. This can occur if you
* submit a request, such as PutExternalModel
, that specifies a role that is not in your
* account.
* @sample AmazonFraudDetector.GetEvent
* @see AWS API
* Documentation
*/
GetEventResult getEvent(GetEventRequest getEventRequest);
/**
*
* Evaluates an event against a detector version. If a version ID is not provided, the detector’s (
* ACTIVE
) version is used.
*
*
* @param getEventPredictionRequest
* @return Result of the GetEventPrediction operation returned by the service.
* @throws ValidationException
* An exception indicating a specified value is not allowed.
* @throws ResourceNotFoundException
* An exception indicating the specified resource was not found.
* @throws InternalServerException
* An exception indicating an internal server error.
* @throws ThrottlingException
* An exception indicating a throttling error.
* @throws AccessDeniedException
* An exception indicating Amazon Fraud Detector does not have the needed permissions. This can occur if you
* submit a request, such as PutExternalModel
, that specifies a role that is not in your
* account.
* @throws ConflictException
* An exception indicating there was a conflict during a delete operation.
* @throws ResourceUnavailableException
* An exception indicating that the attached customer-owned (external) model threw an exception when Amazon
* Fraud Detector invoked the model.
* @sample AmazonFraudDetector.GetEventPrediction
* @see AWS API Documentation
*/
GetEventPredictionResult getEventPrediction(GetEventPredictionRequest getEventPredictionRequest);
/**
*
* Gets details of the past fraud predictions for the specified event ID, event type, detector ID, and detector
* version ID that was generated in the specified time period.
*
*
* @param getEventPredictionMetadataRequest
* @return Result of the GetEventPredictionMetadata operation returned by the service.
* @throws ValidationException
* An exception indicating a specified value is not allowed.
* @throws ResourceNotFoundException
* An exception indicating the specified resource was not found.
* @throws AccessDeniedException
* An exception indicating Amazon Fraud Detector does not have the needed permissions. This can occur if you
* submit a request, such as PutExternalModel
, that specifies a role that is not in your
* account.
* @throws ThrottlingException
* An exception indicating a throttling error.
* @throws InternalServerException
* An exception indicating an internal server error.
* @sample AmazonFraudDetector.GetEventPredictionMetadata
* @see AWS API Documentation
*/
GetEventPredictionMetadataResult getEventPredictionMetadata(GetEventPredictionMetadataRequest getEventPredictionMetadataRequest);
/**
*
* Gets all event types or a specific event type if name is provided. This is a paginated API. If you provide a null
* maxResults
, this action retrieves a maximum of 10 records per page. If you provide a
* maxResults
, the value must be between 5 and 10. To get the next page results, provide the pagination
* token from the GetEventTypesResponse
as part of your request. A null pagination token fetches the
* records from the beginning.
*
*
* @param getEventTypesRequest
* @return Result of the GetEventTypes operation returned by the service.
* @throws ValidationException
* An exception indicating a specified value is not allowed.
* @throws ResourceNotFoundException
* An exception indicating the specified resource was not found.
* @throws InternalServerException
* An exception indicating an internal server error.
* @throws ThrottlingException
* An exception indicating a throttling error.
* @throws AccessDeniedException
* An exception indicating Amazon Fraud Detector does not have the needed permissions. This can occur if you
* submit a request, such as PutExternalModel
, that specifies a role that is not in your
* account.
* @sample AmazonFraudDetector.GetEventTypes
* @see AWS
* API Documentation
*/
GetEventTypesResult getEventTypes(GetEventTypesRequest getEventTypesRequest);
/**
*
* Gets the details for one or more Amazon SageMaker models that have been imported into the service. This is a
* paginated API. If you provide a null maxResults
, this actions retrieves a maximum of 10 records per
* page. If you provide a maxResults
, the value must be between 5 and 10. To get the next page results,
* provide the pagination token from the GetExternalModelsResult
as part of your request. A null
* pagination token fetches the records from the beginning.
*
*
* @param getExternalModelsRequest
* @return Result of the GetExternalModels operation returned by the service.
* @throws ValidationException
* An exception indicating a specified value is not allowed.
* @throws ResourceNotFoundException
* An exception indicating the specified resource was not found.
* @throws InternalServerException
* An exception indicating an internal server error.
* @throws ThrottlingException
* An exception indicating a throttling error.
* @throws AccessDeniedException
* An exception indicating Amazon Fraud Detector does not have the needed permissions. This can occur if you
* submit a request, such as PutExternalModel
, that specifies a role that is not in your
* account.
* @sample AmazonFraudDetector.GetExternalModels
* @see AWS API Documentation
*/
GetExternalModelsResult getExternalModels(GetExternalModelsRequest getExternalModelsRequest);
/**
*
* Gets the encryption key if a KMS key has been specified to be used to encrypt content in Amazon Fraud Detector.
*
*
* @param getKMSEncryptionKeyRequest
* @return Result of the GetKMSEncryptionKey operation returned by the service.
* @throws ResourceNotFoundException
* An exception indicating the specified resource was not found.
* @throws InternalServerException
* An exception indicating an internal server error.
* @throws ThrottlingException
* An exception indicating a throttling error.
* @throws AccessDeniedException
* An exception indicating Amazon Fraud Detector does not have the needed permissions. This can occur if you
* submit a request, such as PutExternalModel
, that specifies a role that is not in your
* account.
* @sample AmazonFraudDetector.GetKMSEncryptionKey
* @see AWS API Documentation
*/
GetKMSEncryptionKeyResult getKMSEncryptionKey(GetKMSEncryptionKeyRequest getKMSEncryptionKeyRequest);
/**
*
* Gets all labels or a specific label if name is provided. This is a paginated API. If you provide a null
* maxResults
, this action retrieves a maximum of 50 records per page. If you provide a
* maxResults
, the value must be between 10 and 50. To get the next page results, provide the
* pagination token from the GetGetLabelsResponse
as part of your request. A null pagination token
* fetches the records from the beginning.
*
*
* @param getLabelsRequest
* @return Result of the GetLabels operation returned by the service.
* @throws ValidationException
* An exception indicating a specified value is not allowed.
* @throws ResourceNotFoundException
* An exception indicating the specified resource was not found.
* @throws InternalServerException
* An exception indicating an internal server error.
* @throws ThrottlingException
* An exception indicating a throttling error.
* @throws AccessDeniedException
* An exception indicating Amazon Fraud Detector does not have the needed permissions. This can occur if you
* submit a request, such as PutExternalModel
, that specifies a role that is not in your
* account.
* @sample AmazonFraudDetector.GetLabels
* @see AWS API
* Documentation
*/
GetLabelsResult getLabels(GetLabelsRequest getLabelsRequest);
/**
*
* Gets all the elements in the specified list.
*
*
* @param getListElementsRequest
* @return Result of the GetListElements operation returned by the service.
* @throws ValidationException
* An exception indicating a specified value is not allowed.
* @throws ResourceNotFoundException
* An exception indicating the specified resource was not found.
* @throws InternalServerException
* An exception indicating an internal server error.
* @throws ThrottlingException
* An exception indicating a throttling error.
* @throws AccessDeniedException
* An exception indicating Amazon Fraud Detector does not have the needed permissions. This can occur if you
* submit a request, such as PutExternalModel
, that specifies a role that is not in your
* account.
* @sample AmazonFraudDetector.GetListElements
* @see AWS
* API Documentation
*/
GetListElementsResult getListElements(GetListElementsRequest getListElementsRequest);
/**
*
* Gets the metadata of either all the lists under the account or the specified list.
*
*
* @param getListsMetadataRequest
* @return Result of the GetListsMetadata operation returned by the service.
* @throws ValidationException
* An exception indicating a specified value is not allowed.
* @throws ResourceNotFoundException
* An exception indicating the specified resource was not found.
* @throws InternalServerException
* An exception indicating an internal server error.
* @throws ThrottlingException
* An exception indicating a throttling error.
* @throws AccessDeniedException
* An exception indicating Amazon Fraud Detector does not have the needed permissions. This can occur if you
* submit a request, such as PutExternalModel
, that specifies a role that is not in your
* account.
* @sample AmazonFraudDetector.GetListsMetadata
* @see AWS
* API Documentation
*/
GetListsMetadataResult getListsMetadata(GetListsMetadataRequest getListsMetadataRequest);
/**
*
* Gets the details of the specified model version.
*
*
* @param getModelVersionRequest
* @return Result of the GetModelVersion operation returned by the service.
* @throws ValidationException
* An exception indicating a specified value is not allowed.
* @throws ResourceNotFoundException
* An exception indicating the specified resource was not found.
* @throws InternalServerException
* An exception indicating an internal server error.
* @throws ThrottlingException
* An exception indicating a throttling error.
* @throws AccessDeniedException
* An exception indicating Amazon Fraud Detector does not have the needed permissions. This can occur if you
* submit a request, such as PutExternalModel
, that specifies a role that is not in your
* account.
* @sample AmazonFraudDetector.GetModelVersion
* @see AWS
* API Documentation
*/
GetModelVersionResult getModelVersion(GetModelVersionRequest getModelVersionRequest);
/**
*
* Gets one or more models. Gets all models for the Amazon Web Services account if no model type and no model id
* provided. Gets all models for the Amazon Web Services account and model type, if the model type is specified but
* model id is not provided. Gets a specific model if (model type, model id) tuple is specified.
*
*
* This is a paginated API. If you provide a null maxResults
, this action retrieves a maximum of 10
* records per page. If you provide a maxResults
, the value must be between 1 and 10. To get the next
* page results, provide the pagination token from the response as part of your request. A null pagination token
* fetches the records from the beginning.
*
*
* @param getModelsRequest
* @return Result of the GetModels operation returned by the service.
* @throws ValidationException
* An exception indicating a specified value is not allowed.
* @throws ResourceNotFoundException
* An exception indicating the specified resource was not found.
* @throws InternalServerException
* An exception indicating an internal server error.
* @throws ThrottlingException
* An exception indicating a throttling error.
* @throws AccessDeniedException
* An exception indicating Amazon Fraud Detector does not have the needed permissions. This can occur if you
* submit a request, such as PutExternalModel
, that specifies a role that is not in your
* account.
* @sample AmazonFraudDetector.GetModels
* @see AWS API
* Documentation
*/
GetModelsResult getModels(GetModelsRequest getModelsRequest);
/**
*
* Gets one or more outcomes. This is a paginated API. If you provide a null maxResults
, this actions
* retrieves a maximum of 100 records per page. If you provide a maxResults
, the value must be between
* 50 and 100. To get the next page results, provide the pagination token from the GetOutcomesResult
as
* part of your request. A null pagination token fetches the records from the beginning.
*
*
* @param getOutcomesRequest
* @return Result of the GetOutcomes operation returned by the service.
* @throws ValidationException
* An exception indicating a specified value is not allowed.
* @throws ResourceNotFoundException
* An exception indicating the specified resource was not found.
* @throws InternalServerException
* An exception indicating an internal server error.
* @throws ThrottlingException
* An exception indicating a throttling error.
* @throws AccessDeniedException
* An exception indicating Amazon Fraud Detector does not have the needed permissions. This can occur if you
* submit a request, such as PutExternalModel
, that specifies a role that is not in your
* account.
* @sample AmazonFraudDetector.GetOutcomes
* @see AWS API
* Documentation
*/
GetOutcomesResult getOutcomes(GetOutcomesRequest getOutcomesRequest);
/**
*
* Get all rules for a detector (paginated) if ruleId
and ruleVersion
are not specified.
* Gets all rules for the detector and the ruleId
if present (paginated). Gets a specific rule if both
* the ruleId
and the ruleVersion
are specified.
*
*
* This is a paginated API. Providing null maxResults results in retrieving maximum of 100 records per page. If you
* provide maxResults the value must be between 50 and 100. To get the next page result, a provide a pagination
* token from GetRulesResult as part of your request. Null pagination token fetches the records from the beginning.
*
*
* @param getRulesRequest
* @return Result of the GetRules operation returned by the service.
* @throws ValidationException
* An exception indicating a specified value is not allowed.
* @throws ResourceNotFoundException
* An exception indicating the specified resource was not found.
* @throws InternalServerException
* An exception indicating an internal server error.
* @throws ThrottlingException
* An exception indicating a throttling error.
* @throws AccessDeniedException
* An exception indicating Amazon Fraud Detector does not have the needed permissions. This can occur if you
* submit a request, such as PutExternalModel
, that specifies a role that is not in your
* account.
* @sample AmazonFraudDetector.GetRules
* @see AWS API
* Documentation
*/
GetRulesResult getRules(GetRulesRequest getRulesRequest);
/**
*
* Gets all of the variables or the specific variable. This is a paginated API. Providing null
* maxSizePerPage
results in retrieving maximum of 100 records per page. If you provide
* maxSizePerPage
the value must be between 50 and 100. To get the next page result, a provide a
* pagination token from GetVariablesResult
as part of your request. Null pagination token fetches the
* records from the beginning.
*
*
* @param getVariablesRequest
* @return Result of the GetVariables operation returned by the service.
* @throws ValidationException
* An exception indicating a specified value is not allowed.
* @throws ResourceNotFoundException
* An exception indicating the specified resource was not found.
* @throws InternalServerException
* An exception indicating an internal server error.
* @throws ThrottlingException
* An exception indicating a throttling error.
* @throws AccessDeniedException
* An exception indicating Amazon Fraud Detector does not have the needed permissions. This can occur if you
* submit a request, such as PutExternalModel
, that specifies a role that is not in your
* account.
* @sample AmazonFraudDetector.GetVariables
* @see AWS API
* Documentation
*/
GetVariablesResult getVariables(GetVariablesRequest getVariablesRequest);
/**
*
* Gets a list of past predictions. The list can be filtered by detector ID, detector version ID, event ID, event
* type, or by specifying a time period. If filter is not specified, the most recent prediction is returned.
*
*
* For example, the following filter lists all past predictions for xyz
event type -
* { "eventType":{ "value": "xyz" }” }
*
*
* This is a paginated API. If you provide a null maxResults
, this action will retrieve a maximum of 10
* records per page. If you provide a maxResults
, the value must be between 50 and 100. To get the next
* page results, provide the nextToken
from the response as part of your request. A null
* nextToken
fetches the records from the beginning.
*
*
* @param listEventPredictionsRequest
* @return Result of the ListEventPredictions operation returned by the service.
* @throws ValidationException
* An exception indicating a specified value is not allowed.
* @throws AccessDeniedException
* An exception indicating Amazon Fraud Detector does not have the needed permissions. This can occur if you
* submit a request, such as PutExternalModel
, that specifies a role that is not in your
* account.
* @throws ThrottlingException
* An exception indicating a throttling error.
* @throws InternalServerException
* An exception indicating an internal server error.
* @sample AmazonFraudDetector.ListEventPredictions
* @see AWS API Documentation
*/
ListEventPredictionsResult listEventPredictions(ListEventPredictionsRequest listEventPredictionsRequest);
/**
*
* Lists all tags associated with the resource. This is a paginated API. To get the next page results, provide the
* pagination token from the response as part of your request. A null pagination token fetches the records from the
* beginning.
*
*
* @param listTagsForResourceRequest
* @return Result of the ListTagsForResource operation returned by the service.
* @throws ValidationException
* An exception indicating a specified value is not allowed.
* @throws ResourceNotFoundException
* An exception indicating the specified resource was not found.
* @throws ThrottlingException
* An exception indicating a throttling error.
* @throws AccessDeniedException
* An exception indicating Amazon Fraud Detector does not have the needed permissions. This can occur if you
* submit a request, such as PutExternalModel
, that specifies a role that is not in your
* account.
* @sample AmazonFraudDetector.ListTagsForResource
* @see AWS API Documentation
*/
ListTagsForResourceResult listTagsForResource(ListTagsForResourceRequest listTagsForResourceRequest);
/**
*
* Creates or updates a detector.
*
*
* @param putDetectorRequest
* @return Result of the PutDetector operation returned by the service.
* @throws ValidationException
* An exception indicating a specified value is not allowed.
* @throws InternalServerException
* An exception indicating an internal server error.
* @throws ThrottlingException
* An exception indicating a throttling error.
* @throws AccessDeniedException
* An exception indicating Amazon Fraud Detector does not have the needed permissions. This can occur if you
* submit a request, such as PutExternalModel
, that specifies a role that is not in your
* account.
* @throws ConflictException
* An exception indicating there was a conflict during a delete operation.
* @sample AmazonFraudDetector.PutDetector
* @see AWS API
* Documentation
*/
PutDetectorResult putDetector(PutDetectorRequest putDetectorRequest);
/**
*
* Creates or updates an entity type. An entity represents who is performing the event. As part of a fraud
* prediction, you pass the entity ID to indicate the specific entity who performed the event. An entity type
* classifies the entity. Example classifications include customer, merchant, or account.
*
*
* @param putEntityTypeRequest
* @return Result of the PutEntityType operation returned by the service.
* @throws ValidationException
* An exception indicating a specified value is not allowed.
* @throws InternalServerException
* An exception indicating an internal server error.
* @throws ThrottlingException
* An exception indicating a throttling error.
* @throws AccessDeniedException
* An exception indicating Amazon Fraud Detector does not have the needed permissions. This can occur if you
* submit a request, such as PutExternalModel
, that specifies a role that is not in your
* account.
* @throws ConflictException
* An exception indicating there was a conflict during a delete operation.
* @sample AmazonFraudDetector.PutEntityType
* @see AWS
* API Documentation
*/
PutEntityTypeResult putEntityType(PutEntityTypeRequest putEntityTypeRequest);
/**
*
* Creates or updates an event type. An event is a business activity that is evaluated for fraud risk. With Amazon
* Fraud Detector, you generate fraud predictions for events. An event type defines the structure for an event sent
* to Amazon Fraud Detector. This includes the variables sent as part of the event, the entity performing the event
* (such as a customer), and the labels that classify the event. Example event types include online payment
* transactions, account registrations, and authentications.
*
*
* @param putEventTypeRequest
* @return Result of the PutEventType operation returned by the service.
* @throws ValidationException
* An exception indicating a specified value is not allowed.
* @throws InternalServerException
* An exception indicating an internal server error.
* @throws ThrottlingException
* An exception indicating a throttling error.
* @throws AccessDeniedException
* An exception indicating Amazon Fraud Detector does not have the needed permissions. This can occur if you
* submit a request, such as PutExternalModel
, that specifies a role that is not in your
* account.
* @throws ConflictException
* An exception indicating there was a conflict during a delete operation.
* @sample AmazonFraudDetector.PutEventType
* @see AWS API
* Documentation
*/
PutEventTypeResult putEventType(PutEventTypeRequest putEventTypeRequest);
/**
*
* Creates or updates an Amazon SageMaker model endpoint. You can also use this action to update the configuration
* of the model endpoint, including the IAM role and/or the mapped variables.
*
*
* @param putExternalModelRequest
* @return Result of the PutExternalModel operation returned by the service.
* @throws ValidationException
* An exception indicating a specified value is not allowed.
* @throws InternalServerException
* An exception indicating an internal server error.
* @throws ThrottlingException
* An exception indicating a throttling error.
* @throws AccessDeniedException
* An exception indicating Amazon Fraud Detector does not have the needed permissions. This can occur if you
* submit a request, such as PutExternalModel
, that specifies a role that is not in your
* account.
* @throws ConflictException
* An exception indicating there was a conflict during a delete operation.
* @sample AmazonFraudDetector.PutExternalModel
* @see AWS
* API Documentation
*/
PutExternalModelResult putExternalModel(PutExternalModelRequest putExternalModelRequest);
/**
*
* Specifies the KMS key to be used to encrypt content in Amazon Fraud Detector.
*
*
* @param putKMSEncryptionKeyRequest
* @return Result of the PutKMSEncryptionKey operation returned by the service.
* @throws ValidationException
* An exception indicating a specified value is not allowed.
* @throws ResourceNotFoundException
* An exception indicating the specified resource was not found.
* @throws InternalServerException
* An exception indicating an internal server error.
* @throws ThrottlingException
* An exception indicating a throttling error.
* @throws AccessDeniedException
* An exception indicating Amazon Fraud Detector does not have the needed permissions. This can occur if you
* submit a request, such as PutExternalModel
, that specifies a role that is not in your
* account.
* @throws ConflictException
* An exception indicating there was a conflict during a delete operation.
* @sample AmazonFraudDetector.PutKMSEncryptionKey
* @see AWS API Documentation
*/
PutKMSEncryptionKeyResult putKMSEncryptionKey(PutKMSEncryptionKeyRequest putKMSEncryptionKeyRequest);
/**
*
* Creates or updates label. A label classifies an event as fraudulent or legitimate. Labels are associated with
* event types and used to train supervised machine learning models in Amazon Fraud Detector.
*
*
* @param putLabelRequest
* @return Result of the PutLabel operation returned by the service.
* @throws ValidationException
* An exception indicating a specified value is not allowed.
* @throws InternalServerException
* An exception indicating an internal server error.
* @throws ThrottlingException
* An exception indicating a throttling error.
* @throws AccessDeniedException
* An exception indicating Amazon Fraud Detector does not have the needed permissions. This can occur if you
* submit a request, such as PutExternalModel
, that specifies a role that is not in your
* account.
* @throws ConflictException
* An exception indicating there was a conflict during a delete operation.
* @sample AmazonFraudDetector.PutLabel
* @see AWS API
* Documentation
*/
PutLabelResult putLabel(PutLabelRequest putLabelRequest);
/**
*
* Creates or updates an outcome.
*
*
* @param putOutcomeRequest
* @return Result of the PutOutcome operation returned by the service.
* @throws ValidationException
* An exception indicating a specified value is not allowed.
* @throws InternalServerException
* An exception indicating an internal server error.
* @throws ThrottlingException
* An exception indicating a throttling error.
* @throws AccessDeniedException
* An exception indicating Amazon Fraud Detector does not have the needed permissions. This can occur if you
* submit a request, such as PutExternalModel
, that specifies a role that is not in your
* account.
* @throws ConflictException
* An exception indicating there was a conflict during a delete operation.
* @sample AmazonFraudDetector.PutOutcome
* @see AWS API
* Documentation
*/
PutOutcomeResult putOutcome(PutOutcomeRequest putOutcomeRequest);
/**
*
* Stores events in Amazon Fraud Detector without generating fraud predictions for those events. For example, you
* can use SendEvent
to upload a historical dataset, which you can then later use to train a model.
*
*
* @param sendEventRequest
* @return Result of the SendEvent operation returned by the service.
* @throws ValidationException
* An exception indicating a specified value is not allowed.
* @throws ConflictException
* An exception indicating there was a conflict during a delete operation.
* @throws ResourceNotFoundException
* An exception indicating the specified resource was not found.
* @throws InternalServerException
* An exception indicating an internal server error.
* @throws ThrottlingException
* An exception indicating a throttling error.
* @throws AccessDeniedException
* An exception indicating Amazon Fraud Detector does not have the needed permissions. This can occur if you
* submit a request, such as PutExternalModel
, that specifies a role that is not in your
* account.
* @sample AmazonFraudDetector.SendEvent
* @see AWS API
* Documentation
*/
SendEventResult sendEvent(SendEventRequest sendEventRequest);
/**
*
* Assigns tags to a resource.
*
*
* @param tagResourceRequest
* @return Result of the TagResource operation returned by the service.
* @throws ValidationException
* An exception indicating a specified value is not allowed.
* @throws ResourceNotFoundException
* An exception indicating the specified resource was not found.
* @throws ThrottlingException
* An exception indicating a throttling error.
* @throws AccessDeniedException
* An exception indicating Amazon Fraud Detector does not have the needed permissions. This can occur if you
* submit a request, such as PutExternalModel
, that specifies a role that is not in your
* account.
* @sample AmazonFraudDetector.TagResource
* @see AWS API
* Documentation
*/
TagResourceResult tagResource(TagResourceRequest tagResourceRequest);
/**
*
* Removes tags from a resource.
*
*
* @param untagResourceRequest
* @return Result of the UntagResource operation returned by the service.
* @throws ValidationException
* An exception indicating a specified value is not allowed.
* @throws ResourceNotFoundException
* An exception indicating the specified resource was not found.
* @throws ThrottlingException
* An exception indicating a throttling error.
* @throws AccessDeniedException
* An exception indicating Amazon Fraud Detector does not have the needed permissions. This can occur if you
* submit a request, such as PutExternalModel
, that specifies a role that is not in your
* account.
* @sample AmazonFraudDetector.UntagResource
* @see AWS
* API Documentation
*/
UntagResourceResult untagResource(UntagResourceRequest untagResourceRequest);
/**
*
* Updates a detector version. The detector version attributes that you can update include models, external model
* endpoints, rules, rule execution mode, and description. You can only update a DRAFT
detector
* version.
*
*
* @param updateDetectorVersionRequest
* @return Result of the UpdateDetectorVersion operation returned by the service.
* @throws ValidationException
* An exception indicating a specified value is not allowed.
* @throws ResourceNotFoundException
* An exception indicating the specified resource was not found.
* @throws InternalServerException
* An exception indicating an internal server error.
* @throws ThrottlingException
* An exception indicating a throttling error.
* @throws AccessDeniedException
* An exception indicating Amazon Fraud Detector does not have the needed permissions. This can occur if you
* submit a request, such as PutExternalModel
, that specifies a role that is not in your
* account.
* @throws ConflictException
* An exception indicating there was a conflict during a delete operation.
* @sample AmazonFraudDetector.UpdateDetectorVersion
* @see AWS API Documentation
*/
UpdateDetectorVersionResult updateDetectorVersion(UpdateDetectorVersionRequest updateDetectorVersionRequest);
/**
*
* Updates the detector version's description. You can update the metadata for any detector version (
* DRAFT, ACTIVE,
or INACTIVE
).
*
*
* @param updateDetectorVersionMetadataRequest
* @return Result of the UpdateDetectorVersionMetadata operation returned by the service.
* @throws ValidationException
* An exception indicating a specified value is not allowed.
* @throws InternalServerException
* An exception indicating an internal server error.
* @throws ThrottlingException
* An exception indicating a throttling error.
* @throws AccessDeniedException
* An exception indicating Amazon Fraud Detector does not have the needed permissions. This can occur if you
* submit a request, such as PutExternalModel
, that specifies a role that is not in your
* account.
* @throws ConflictException
* An exception indicating there was a conflict during a delete operation.
* @sample AmazonFraudDetector.UpdateDetectorVersionMetadata
* @see AWS API Documentation
*/
UpdateDetectorVersionMetadataResult updateDetectorVersionMetadata(UpdateDetectorVersionMetadataRequest updateDetectorVersionMetadataRequest);
/**
*
* Updates the detector version’s status. You can perform the following promotions or demotions using
* UpdateDetectorVersionStatus
: DRAFT
to ACTIVE
, ACTIVE
to
* INACTIVE
, and INACTIVE
to ACTIVE
.
*
*
* @param updateDetectorVersionStatusRequest
* @return Result of the UpdateDetectorVersionStatus operation returned by the service.
* @throws ValidationException
* An exception indicating a specified value is not allowed.
* @throws ResourceNotFoundException
* An exception indicating the specified resource was not found.
* @throws InternalServerException
* An exception indicating an internal server error.
* @throws ThrottlingException
* An exception indicating a throttling error.
* @throws AccessDeniedException
* An exception indicating Amazon Fraud Detector does not have the needed permissions. This can occur if you
* submit a request, such as PutExternalModel
, that specifies a role that is not in your
* account.
* @throws ConflictException
* An exception indicating there was a conflict during a delete operation.
* @sample AmazonFraudDetector.UpdateDetectorVersionStatus
* @see AWS API Documentation
*/
UpdateDetectorVersionStatusResult updateDetectorVersionStatus(UpdateDetectorVersionStatusRequest updateDetectorVersionStatusRequest);
/**
*
* Updates the specified event with a new label.
*
*
* @param updateEventLabelRequest
* @return Result of the UpdateEventLabel operation returned by the service.
* @throws ValidationException
* An exception indicating a specified value is not allowed.
* @throws ResourceNotFoundException
* An exception indicating the specified resource was not found.
* @throws InternalServerException
* An exception indicating an internal server error.
* @throws ThrottlingException
* An exception indicating a throttling error.
* @throws AccessDeniedException
* An exception indicating Amazon Fraud Detector does not have the needed permissions. This can occur if you
* submit a request, such as PutExternalModel
, that specifies a role that is not in your
* account.
* @throws ConflictException
* An exception indicating there was a conflict during a delete operation.
* @sample AmazonFraudDetector.UpdateEventLabel
* @see AWS
* API Documentation
*/
UpdateEventLabelResult updateEventLabel(UpdateEventLabelRequest updateEventLabelRequest);
/**
*
* Updates a list.
*
*
* @param updateListRequest
* @return Result of the UpdateList operation returned by the service.
* @throws ValidationException
* An exception indicating a specified value is not allowed.
* @throws ResourceNotFoundException
* An exception indicating the specified resource was not found.
* @throws InternalServerException
* An exception indicating an internal server error.
* @throws ThrottlingException
* An exception indicating a throttling error.
* @throws AccessDeniedException
* An exception indicating Amazon Fraud Detector does not have the needed permissions. This can occur if you
* submit a request, such as PutExternalModel
, that specifies a role that is not in your
* account.
* @throws ConflictException
* An exception indicating there was a conflict during a delete operation.
* @sample AmazonFraudDetector.UpdateList
* @see AWS API
* Documentation
*/
UpdateListResult updateList(UpdateListRequest updateListRequest);
/**
*
* Updates model description.
*
*
* @param updateModelRequest
* @return Result of the UpdateModel operation returned by the service.
* @throws ValidationException
* An exception indicating a specified value is not allowed.
* @throws ResourceNotFoundException
* An exception indicating the specified resource was not found.
* @throws InternalServerException
* An exception indicating an internal server error.
* @throws ThrottlingException
* An exception indicating a throttling error.
* @throws AccessDeniedException
* An exception indicating Amazon Fraud Detector does not have the needed permissions. This can occur if you
* submit a request, such as PutExternalModel
, that specifies a role that is not in your
* account.
* @throws ConflictException
* An exception indicating there was a conflict during a delete operation.
* @sample AmazonFraudDetector.UpdateModel
* @see AWS API
* Documentation
*/
UpdateModelResult updateModel(UpdateModelRequest updateModelRequest);
/**
*
* Updates a model version. Updating a model version retrains an existing model version using updated training data
* and produces a new minor version of the model. You can update the training data set location and data access role
* attributes using this action. This action creates and trains a new minor version of the model, for example
* version 1.01, 1.02, 1.03.
*
*
* @param updateModelVersionRequest
* @return Result of the UpdateModelVersion operation returned by the service.
* @throws ValidationException
* An exception indicating a specified value is not allowed.
* @throws ResourceNotFoundException
* An exception indicating the specified resource was not found.
* @throws AccessDeniedException
* An exception indicating Amazon Fraud Detector does not have the needed permissions. This can occur if you
* submit a request, such as PutExternalModel
, that specifies a role that is not in your
* account.
* @throws ThrottlingException
* An exception indicating a throttling error.
* @throws InternalServerException
* An exception indicating an internal server error.
* @throws ConflictException
* An exception indicating there was a conflict during a delete operation.
* @sample AmazonFraudDetector.UpdateModelVersion
* @see AWS API Documentation
*/
UpdateModelVersionResult updateModelVersion(UpdateModelVersionRequest updateModelVersionRequest);
/**
*
* Updates the status of a model version.
*
*
* You can perform the following status updates:
*
*
* -
*
* Change the TRAINING_IN_PROGRESS
status to TRAINING_CANCELLED
.
*
*
* -
*
* Change the TRAINING_COMPLETE
status to ACTIVE
.
*
*
* -
*
* Change ACTIVE
to INACTIVE
.
*
*
*
*
* @param updateModelVersionStatusRequest
* @return Result of the UpdateModelVersionStatus operation returned by the service.
* @throws ValidationException
* An exception indicating a specified value is not allowed.
* @throws ResourceNotFoundException
* An exception indicating the specified resource was not found.
* @throws InternalServerException
* An exception indicating an internal server error.
* @throws ThrottlingException
* An exception indicating a throttling error.
* @throws AccessDeniedException
* An exception indicating Amazon Fraud Detector does not have the needed permissions. This can occur if you
* submit a request, such as PutExternalModel
, that specifies a role that is not in your
* account.
* @throws ConflictException
* An exception indicating there was a conflict during a delete operation.
* @sample AmazonFraudDetector.UpdateModelVersionStatus
* @see AWS API Documentation
*/
UpdateModelVersionStatusResult updateModelVersionStatus(UpdateModelVersionStatusRequest updateModelVersionStatusRequest);
/**
*
* Updates a rule's metadata. The description attribute can be updated.
*
*
* @param updateRuleMetadataRequest
* @return Result of the UpdateRuleMetadata operation returned by the service.
* @throws ValidationException
* An exception indicating a specified value is not allowed.
* @throws ResourceNotFoundException
* An exception indicating the specified resource was not found.
* @throws InternalServerException
* An exception indicating an internal server error.
* @throws ThrottlingException
* An exception indicating a throttling error.
* @throws AccessDeniedException
* An exception indicating Amazon Fraud Detector does not have the needed permissions. This can occur if you
* submit a request, such as PutExternalModel
, that specifies a role that is not in your
* account.
* @throws ConflictException
* An exception indicating there was a conflict during a delete operation.
* @sample AmazonFraudDetector.UpdateRuleMetadata
* @see AWS API Documentation
*/
UpdateRuleMetadataResult updateRuleMetadata(UpdateRuleMetadataRequest updateRuleMetadataRequest);
/**
*
* Updates a rule version resulting in a new rule version. Updates a rule version resulting in a new rule version
* (version 1, 2, 3 ...).
*
*
* @param updateRuleVersionRequest
* @return Result of the UpdateRuleVersion operation returned by the service.
* @throws ValidationException
* An exception indicating a specified value is not allowed.
* @throws ResourceNotFoundException
* An exception indicating the specified resource was not found.
* @throws InternalServerException
* An exception indicating an internal server error.
* @throws ThrottlingException
* An exception indicating a throttling error.
* @throws AccessDeniedException
* An exception indicating Amazon Fraud Detector does not have the needed permissions. This can occur if you
* submit a request, such as PutExternalModel
, that specifies a role that is not in your
* account.
* @throws ConflictException
* An exception indicating there was a conflict during a delete operation.
* @sample AmazonFraudDetector.UpdateRuleVersion
* @see AWS API Documentation
*/
UpdateRuleVersionResult updateRuleVersion(UpdateRuleVersionRequest updateRuleVersionRequest);
/**
*
* Updates a variable.
*
*
* @param updateVariableRequest
* @return Result of the UpdateVariable operation returned by the service.
* @throws ValidationException
* An exception indicating a specified value is not allowed.
* @throws ResourceNotFoundException
* An exception indicating the specified resource was not found.
* @throws InternalServerException
* An exception indicating an internal server error.
* @throws ThrottlingException
* An exception indicating a throttling error.
* @throws AccessDeniedException
* An exception indicating Amazon Fraud Detector does not have the needed permissions. This can occur if you
* submit a request, such as PutExternalModel
, that specifies a role that is not in your
* account.
* @throws ConflictException
* An exception indicating there was a conflict during a delete operation.
* @sample AmazonFraudDetector.UpdateVariable
* @see AWS
* API Documentation
*/
UpdateVariableResult updateVariable(UpdateVariableRequest updateVariableRequest);
/**
* Shuts down this client object, releasing any resources that might be held open. This is an optional method, and
* callers are not expected to call it, but can if they want to explicitly release any open resources. Once a client
* has been shutdown, it should not be used to make any more requests.
*/
void shutdown();
/**
* Returns additional metadata for a previously executed successful request, typically used for debugging issues
* where a service isn't acting as expected. This data isn't considered part of the result data returned by an
* operation, so it's available through this separate, diagnostic interface.
*
* Response metadata is only cached for a limited period of time, so if you need to access this extra diagnostic
* information for an executed request, you should use this method to retrieve it as soon as possible after
* executing a request.
*
* @param request
* The originally executed request.
*
* @return The response metadata for the specified request, or null if none is available.
*/
ResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request);
}