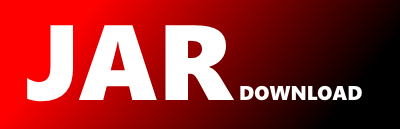
com.amazonaws.services.frauddetector.AmazonFraudDetectorAsync Maven / Gradle / Ivy
Show all versions of aws-java-sdk-frauddetector Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.frauddetector;
import javax.annotation.Generated;
import com.amazonaws.services.frauddetector.model.*;
/**
* Interface for accessing Amazon Fraud Detector asynchronously. Each asynchronous method will return a Java Future
* object representing the asynchronous operation; overloads which accept an {@code AsyncHandler} can be used to receive
* notification when an asynchronous operation completes.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.frauddetector.AbstractAmazonFraudDetectorAsync} instead.
*
*
*
* This is the Amazon Fraud Detector API Reference. This guide is for developers who need detailed information about
* Amazon Fraud Detector API actions, data types, and errors. For more information about Amazon Fraud Detector features,
* see the Amazon Fraud Detector User Guide.
*
*
* We provide the Query API as well as AWS software development kits (SDK) for Amazon Fraud Detector in Java and Python
* programming languages.
*
*
* The Amazon Fraud Detector Query API provides HTTPS requests that use the HTTP verb GET or POST and a Query parameter
* Action
. AWS SDK provides libraries, sample code, tutorials, and other resources for software developers
* who prefer to build applications using language-specific APIs instead of submitting a request over HTTP or HTTPS.
* These libraries provide basic functions that automatically take care of tasks such as cryptographically signing your
* requests, retrying requests, and handling error responses, so that it is easier for you to get started. For more
* information about the AWS SDKs, go to Tools to build on AWS
* page, scroll down to the SDK section, and choose plus (+) sign to expand the section.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AmazonFraudDetectorAsync extends AmazonFraudDetector {
/**
*
* Creates a batch of variables.
*
*
* @param batchCreateVariableRequest
* @return A Java Future containing the result of the BatchCreateVariable operation returned by the service.
* @sample AmazonFraudDetectorAsync.BatchCreateVariable
* @see AWS API Documentation
*/
java.util.concurrent.Future batchCreateVariableAsync(BatchCreateVariableRequest batchCreateVariableRequest);
/**
*
* Creates a batch of variables.
*
*
* @param batchCreateVariableRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the BatchCreateVariable operation returned by the service.
* @sample AmazonFraudDetectorAsyncHandler.BatchCreateVariable
* @see AWS API Documentation
*/
java.util.concurrent.Future batchCreateVariableAsync(BatchCreateVariableRequest batchCreateVariableRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets a batch of variables.
*
*
* @param batchGetVariableRequest
* @return A Java Future containing the result of the BatchGetVariable operation returned by the service.
* @sample AmazonFraudDetectorAsync.BatchGetVariable
* @see AWS
* API Documentation
*/
java.util.concurrent.Future batchGetVariableAsync(BatchGetVariableRequest batchGetVariableRequest);
/**
*
* Gets a batch of variables.
*
*
* @param batchGetVariableRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the BatchGetVariable operation returned by the service.
* @sample AmazonFraudDetectorAsyncHandler.BatchGetVariable
* @see AWS
* API Documentation
*/
java.util.concurrent.Future batchGetVariableAsync(BatchGetVariableRequest batchGetVariableRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Cancels an in-progress batch import job.
*
*
* @param cancelBatchImportJobRequest
* @return A Java Future containing the result of the CancelBatchImportJob operation returned by the service.
* @sample AmazonFraudDetectorAsync.CancelBatchImportJob
* @see AWS API Documentation
*/
java.util.concurrent.Future cancelBatchImportJobAsync(CancelBatchImportJobRequest cancelBatchImportJobRequest);
/**
*
* Cancels an in-progress batch import job.
*
*
* @param cancelBatchImportJobRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CancelBatchImportJob operation returned by the service.
* @sample AmazonFraudDetectorAsyncHandler.CancelBatchImportJob
* @see AWS API Documentation
*/
java.util.concurrent.Future cancelBatchImportJobAsync(CancelBatchImportJobRequest cancelBatchImportJobRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Cancels the specified batch prediction job.
*
*
* @param cancelBatchPredictionJobRequest
* @return A Java Future containing the result of the CancelBatchPredictionJob operation returned by the service.
* @sample AmazonFraudDetectorAsync.CancelBatchPredictionJob
* @see AWS API Documentation
*/
java.util.concurrent.Future cancelBatchPredictionJobAsync(CancelBatchPredictionJobRequest cancelBatchPredictionJobRequest);
/**
*
* Cancels the specified batch prediction job.
*
*
* @param cancelBatchPredictionJobRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CancelBatchPredictionJob operation returned by the service.
* @sample AmazonFraudDetectorAsyncHandler.CancelBatchPredictionJob
* @see AWS API Documentation
*/
java.util.concurrent.Future cancelBatchPredictionJobAsync(CancelBatchPredictionJobRequest cancelBatchPredictionJobRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a batch import job.
*
*
* @param createBatchImportJobRequest
* @return A Java Future containing the result of the CreateBatchImportJob operation returned by the service.
* @sample AmazonFraudDetectorAsync.CreateBatchImportJob
* @see AWS API Documentation
*/
java.util.concurrent.Future createBatchImportJobAsync(CreateBatchImportJobRequest createBatchImportJobRequest);
/**
*
* Creates a batch import job.
*
*
* @param createBatchImportJobRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateBatchImportJob operation returned by the service.
* @sample AmazonFraudDetectorAsyncHandler.CreateBatchImportJob
* @see AWS API Documentation
*/
java.util.concurrent.Future createBatchImportJobAsync(CreateBatchImportJobRequest createBatchImportJobRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a batch prediction job.
*
*
* @param createBatchPredictionJobRequest
* @return A Java Future containing the result of the CreateBatchPredictionJob operation returned by the service.
* @sample AmazonFraudDetectorAsync.CreateBatchPredictionJob
* @see AWS API Documentation
*/
java.util.concurrent.Future createBatchPredictionJobAsync(CreateBatchPredictionJobRequest createBatchPredictionJobRequest);
/**
*
* Creates a batch prediction job.
*
*
* @param createBatchPredictionJobRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateBatchPredictionJob operation returned by the service.
* @sample AmazonFraudDetectorAsyncHandler.CreateBatchPredictionJob
* @see AWS API Documentation
*/
java.util.concurrent.Future createBatchPredictionJobAsync(CreateBatchPredictionJobRequest createBatchPredictionJobRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a detector version. The detector version starts in a DRAFT
status.
*
*
* @param createDetectorVersionRequest
* @return A Java Future containing the result of the CreateDetectorVersion operation returned by the service.
* @sample AmazonFraudDetectorAsync.CreateDetectorVersion
* @see AWS API Documentation
*/
java.util.concurrent.Future createDetectorVersionAsync(CreateDetectorVersionRequest createDetectorVersionRequest);
/**
*
* Creates a detector version. The detector version starts in a DRAFT
status.
*
*
* @param createDetectorVersionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateDetectorVersion operation returned by the service.
* @sample AmazonFraudDetectorAsyncHandler.CreateDetectorVersion
* @see AWS API Documentation
*/
java.util.concurrent.Future createDetectorVersionAsync(CreateDetectorVersionRequest createDetectorVersionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a list.
*
*
* List is a set of input data for a variable in your event dataset. You use the input data in a rule that's
* associated with your detector. For more information, see Lists.
*
*
* @param createListRequest
* @return A Java Future containing the result of the CreateList operation returned by the service.
* @sample AmazonFraudDetectorAsync.CreateList
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createListAsync(CreateListRequest createListRequest);
/**
*
* Creates a list.
*
*
* List is a set of input data for a variable in your event dataset. You use the input data in a rule that's
* associated with your detector. For more information, see Lists.
*
*
* @param createListRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateList operation returned by the service.
* @sample AmazonFraudDetectorAsyncHandler.CreateList
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createListAsync(CreateListRequest createListRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a model using the specified model type.
*
*
* @param createModelRequest
* @return A Java Future containing the result of the CreateModel operation returned by the service.
* @sample AmazonFraudDetectorAsync.CreateModel
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createModelAsync(CreateModelRequest createModelRequest);
/**
*
* Creates a model using the specified model type.
*
*
* @param createModelRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateModel operation returned by the service.
* @sample AmazonFraudDetectorAsyncHandler.CreateModel
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createModelAsync(CreateModelRequest createModelRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a version of the model using the specified model type and model id.
*
*
* @param createModelVersionRequest
* @return A Java Future containing the result of the CreateModelVersion operation returned by the service.
* @sample AmazonFraudDetectorAsync.CreateModelVersion
* @see AWS API Documentation
*/
java.util.concurrent.Future createModelVersionAsync(CreateModelVersionRequest createModelVersionRequest);
/**
*
* Creates a version of the model using the specified model type and model id.
*
*
* @param createModelVersionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateModelVersion operation returned by the service.
* @sample AmazonFraudDetectorAsyncHandler.CreateModelVersion
* @see AWS API Documentation
*/
java.util.concurrent.Future createModelVersionAsync(CreateModelVersionRequest createModelVersionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a rule for use with the specified detector.
*
*
* @param createRuleRequest
* @return A Java Future containing the result of the CreateRule operation returned by the service.
* @sample AmazonFraudDetectorAsync.CreateRule
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createRuleAsync(CreateRuleRequest createRuleRequest);
/**
*
* Creates a rule for use with the specified detector.
*
*
* @param createRuleRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateRule operation returned by the service.
* @sample AmazonFraudDetectorAsyncHandler.CreateRule
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createRuleAsync(CreateRuleRequest createRuleRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a variable.
*
*
* @param createVariableRequest
* @return A Java Future containing the result of the CreateVariable operation returned by the service.
* @sample AmazonFraudDetectorAsync.CreateVariable
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createVariableAsync(CreateVariableRequest createVariableRequest);
/**
*
* Creates a variable.
*
*
* @param createVariableRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateVariable operation returned by the service.
* @sample AmazonFraudDetectorAsyncHandler.CreateVariable
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createVariableAsync(CreateVariableRequest createVariableRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the specified batch import job ID record. This action does not delete the data that was batch imported.
*
*
* @param deleteBatchImportJobRequest
* @return A Java Future containing the result of the DeleteBatchImportJob operation returned by the service.
* @sample AmazonFraudDetectorAsync.DeleteBatchImportJob
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteBatchImportJobAsync(DeleteBatchImportJobRequest deleteBatchImportJobRequest);
/**
*
* Deletes the specified batch import job ID record. This action does not delete the data that was batch imported.
*
*
* @param deleteBatchImportJobRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteBatchImportJob operation returned by the service.
* @sample AmazonFraudDetectorAsyncHandler.DeleteBatchImportJob
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteBatchImportJobAsync(DeleteBatchImportJobRequest deleteBatchImportJobRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a batch prediction job.
*
*
* @param deleteBatchPredictionJobRequest
* @return A Java Future containing the result of the DeleteBatchPredictionJob operation returned by the service.
* @sample AmazonFraudDetectorAsync.DeleteBatchPredictionJob
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteBatchPredictionJobAsync(DeleteBatchPredictionJobRequest deleteBatchPredictionJobRequest);
/**
*
* Deletes a batch prediction job.
*
*
* @param deleteBatchPredictionJobRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteBatchPredictionJob operation returned by the service.
* @sample AmazonFraudDetectorAsyncHandler.DeleteBatchPredictionJob
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteBatchPredictionJobAsync(DeleteBatchPredictionJobRequest deleteBatchPredictionJobRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the detector. Before deleting a detector, you must first delete all detector versions and rule versions
* associated with the detector.
*
*
* When you delete a detector, Amazon Fraud Detector permanently deletes the detector and the data is no longer
* stored in Amazon Fraud Detector.
*
*
* @param deleteDetectorRequest
* @return A Java Future containing the result of the DeleteDetector operation returned by the service.
* @sample AmazonFraudDetectorAsync.DeleteDetector
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteDetectorAsync(DeleteDetectorRequest deleteDetectorRequest);
/**
*
* Deletes the detector. Before deleting a detector, you must first delete all detector versions and rule versions
* associated with the detector.
*
*
* When you delete a detector, Amazon Fraud Detector permanently deletes the detector and the data is no longer
* stored in Amazon Fraud Detector.
*
*
* @param deleteDetectorRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteDetector operation returned by the service.
* @sample AmazonFraudDetectorAsyncHandler.DeleteDetector
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteDetectorAsync(DeleteDetectorRequest deleteDetectorRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the detector version. You cannot delete detector versions that are in ACTIVE
status.
*
*
* When you delete a detector version, Amazon Fraud Detector permanently deletes the detector and the data is no
* longer stored in Amazon Fraud Detector.
*
*
* @param deleteDetectorVersionRequest
* @return A Java Future containing the result of the DeleteDetectorVersion operation returned by the service.
* @sample AmazonFraudDetectorAsync.DeleteDetectorVersion
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteDetectorVersionAsync(DeleteDetectorVersionRequest deleteDetectorVersionRequest);
/**
*
* Deletes the detector version. You cannot delete detector versions that are in ACTIVE
status.
*
*
* When you delete a detector version, Amazon Fraud Detector permanently deletes the detector and the data is no
* longer stored in Amazon Fraud Detector.
*
*
* @param deleteDetectorVersionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteDetectorVersion operation returned by the service.
* @sample AmazonFraudDetectorAsyncHandler.DeleteDetectorVersion
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteDetectorVersionAsync(DeleteDetectorVersionRequest deleteDetectorVersionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes an entity type.
*
*
* You cannot delete an entity type that is included in an event type.
*
*
* When you delete an entity type, Amazon Fraud Detector permanently deletes that entity type and the data is no
* longer stored in Amazon Fraud Detector.
*
*
* @param deleteEntityTypeRequest
* @return A Java Future containing the result of the DeleteEntityType operation returned by the service.
* @sample AmazonFraudDetectorAsync.DeleteEntityType
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteEntityTypeAsync(DeleteEntityTypeRequest deleteEntityTypeRequest);
/**
*
* Deletes an entity type.
*
*
* You cannot delete an entity type that is included in an event type.
*
*
* When you delete an entity type, Amazon Fraud Detector permanently deletes that entity type and the data is no
* longer stored in Amazon Fraud Detector.
*
*
* @param deleteEntityTypeRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteEntityType operation returned by the service.
* @sample AmazonFraudDetectorAsyncHandler.DeleteEntityType
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteEntityTypeAsync(DeleteEntityTypeRequest deleteEntityTypeRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the specified event.
*
*
* When you delete an event, Amazon Fraud Detector permanently deletes that event and the event data is no longer
* stored in Amazon Fraud Detector. If deleteAuditHistory
is True
, event data is available
* through search for up to 30 seconds after the delete operation is completed.
*
*
* @param deleteEventRequest
* @return A Java Future containing the result of the DeleteEvent operation returned by the service.
* @sample AmazonFraudDetectorAsync.DeleteEvent
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteEventAsync(DeleteEventRequest deleteEventRequest);
/**
*
* Deletes the specified event.
*
*
* When you delete an event, Amazon Fraud Detector permanently deletes that event and the event data is no longer
* stored in Amazon Fraud Detector. If deleteAuditHistory
is True
, event data is available
* through search for up to 30 seconds after the delete operation is completed.
*
*
* @param deleteEventRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteEvent operation returned by the service.
* @sample AmazonFraudDetectorAsyncHandler.DeleteEvent
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteEventAsync(DeleteEventRequest deleteEventRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes an event type.
*
*
* You cannot delete an event type that is used in a detector or a model.
*
*
* When you delete an event type, Amazon Fraud Detector permanently deletes that event type and the data is no
* longer stored in Amazon Fraud Detector.
*
*
* @param deleteEventTypeRequest
* @return A Java Future containing the result of the DeleteEventType operation returned by the service.
* @sample AmazonFraudDetectorAsync.DeleteEventType
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteEventTypeAsync(DeleteEventTypeRequest deleteEventTypeRequest);
/**
*
* Deletes an event type.
*
*
* You cannot delete an event type that is used in a detector or a model.
*
*
* When you delete an event type, Amazon Fraud Detector permanently deletes that event type and the data is no
* longer stored in Amazon Fraud Detector.
*
*
* @param deleteEventTypeRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteEventType operation returned by the service.
* @sample AmazonFraudDetectorAsyncHandler.DeleteEventType
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteEventTypeAsync(DeleteEventTypeRequest deleteEventTypeRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes all events of a particular event type.
*
*
* @param deleteEventsByEventTypeRequest
* @return A Java Future containing the result of the DeleteEventsByEventType operation returned by the service.
* @sample AmazonFraudDetectorAsync.DeleteEventsByEventType
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteEventsByEventTypeAsync(DeleteEventsByEventTypeRequest deleteEventsByEventTypeRequest);
/**
*
* Deletes all events of a particular event type.
*
*
* @param deleteEventsByEventTypeRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteEventsByEventType operation returned by the service.
* @sample AmazonFraudDetectorAsyncHandler.DeleteEventsByEventType
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteEventsByEventTypeAsync(DeleteEventsByEventTypeRequest deleteEventsByEventTypeRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Removes a SageMaker model from Amazon Fraud Detector.
*
*
* You can remove an Amazon SageMaker model if it is not associated with a detector version. Removing a SageMaker
* model disconnects it from Amazon Fraud Detector, but the model remains available in SageMaker.
*
*
* @param deleteExternalModelRequest
* @return A Java Future containing the result of the DeleteExternalModel operation returned by the service.
* @sample AmazonFraudDetectorAsync.DeleteExternalModel
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteExternalModelAsync(DeleteExternalModelRequest deleteExternalModelRequest);
/**
*
* Removes a SageMaker model from Amazon Fraud Detector.
*
*
* You can remove an Amazon SageMaker model if it is not associated with a detector version. Removing a SageMaker
* model disconnects it from Amazon Fraud Detector, but the model remains available in SageMaker.
*
*
* @param deleteExternalModelRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteExternalModel operation returned by the service.
* @sample AmazonFraudDetectorAsyncHandler.DeleteExternalModel
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteExternalModelAsync(DeleteExternalModelRequest deleteExternalModelRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a label.
*
*
* You cannot delete labels that are included in an event type in Amazon Fraud Detector.
*
*
* You cannot delete a label assigned to an event ID. You must first delete the relevant event ID.
*
*
* When you delete a label, Amazon Fraud Detector permanently deletes that label and the data is no longer stored in
* Amazon Fraud Detector.
*
*
* @param deleteLabelRequest
* @return A Java Future containing the result of the DeleteLabel operation returned by the service.
* @sample AmazonFraudDetectorAsync.DeleteLabel
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteLabelAsync(DeleteLabelRequest deleteLabelRequest);
/**
*
* Deletes a label.
*
*
* You cannot delete labels that are included in an event type in Amazon Fraud Detector.
*
*
* You cannot delete a label assigned to an event ID. You must first delete the relevant event ID.
*
*
* When you delete a label, Amazon Fraud Detector permanently deletes that label and the data is no longer stored in
* Amazon Fraud Detector.
*
*
* @param deleteLabelRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteLabel operation returned by the service.
* @sample AmazonFraudDetectorAsyncHandler.DeleteLabel
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteLabelAsync(DeleteLabelRequest deleteLabelRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the list, provided it is not used in a rule.
*
*
* When you delete a list, Amazon Fraud Detector permanently deletes that list and the elements in the list.
*
*
* @param deleteListRequest
* @return A Java Future containing the result of the DeleteList operation returned by the service.
* @sample AmazonFraudDetectorAsync.DeleteList
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteListAsync(DeleteListRequest deleteListRequest);
/**
*
* Deletes the list, provided it is not used in a rule.
*
*
* When you delete a list, Amazon Fraud Detector permanently deletes that list and the elements in the list.
*
*
* @param deleteListRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteList operation returned by the service.
* @sample AmazonFraudDetectorAsyncHandler.DeleteList
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteListAsync(DeleteListRequest deleteListRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a model.
*
*
* You can delete models and model versions in Amazon Fraud Detector, provided that they are not associated with a
* detector version.
*
*
* When you delete a model, Amazon Fraud Detector permanently deletes that model and the data is no longer stored in
* Amazon Fraud Detector.
*
*
* @param deleteModelRequest
* @return A Java Future containing the result of the DeleteModel operation returned by the service.
* @sample AmazonFraudDetectorAsync.DeleteModel
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteModelAsync(DeleteModelRequest deleteModelRequest);
/**
*
* Deletes a model.
*
*
* You can delete models and model versions in Amazon Fraud Detector, provided that they are not associated with a
* detector version.
*
*
* When you delete a model, Amazon Fraud Detector permanently deletes that model and the data is no longer stored in
* Amazon Fraud Detector.
*
*
* @param deleteModelRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteModel operation returned by the service.
* @sample AmazonFraudDetectorAsyncHandler.DeleteModel
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteModelAsync(DeleteModelRequest deleteModelRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a model version.
*
*
* You can delete models and model versions in Amazon Fraud Detector, provided that they are not associated with a
* detector version.
*
*
* When you delete a model version, Amazon Fraud Detector permanently deletes that model version and the data is no
* longer stored in Amazon Fraud Detector.
*
*
* @param deleteModelVersionRequest
* @return A Java Future containing the result of the DeleteModelVersion operation returned by the service.
* @sample AmazonFraudDetectorAsync.DeleteModelVersion
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteModelVersionAsync(DeleteModelVersionRequest deleteModelVersionRequest);
/**
*
* Deletes a model version.
*
*
* You can delete models and model versions in Amazon Fraud Detector, provided that they are not associated with a
* detector version.
*
*
* When you delete a model version, Amazon Fraud Detector permanently deletes that model version and the data is no
* longer stored in Amazon Fraud Detector.
*
*
* @param deleteModelVersionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteModelVersion operation returned by the service.
* @sample AmazonFraudDetectorAsyncHandler.DeleteModelVersion
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteModelVersionAsync(DeleteModelVersionRequest deleteModelVersionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes an outcome.
*
*
* You cannot delete an outcome that is used in a rule version.
*
*
* When you delete an outcome, Amazon Fraud Detector permanently deletes that outcome and the data is no longer
* stored in Amazon Fraud Detector.
*
*
* @param deleteOutcomeRequest
* @return A Java Future containing the result of the DeleteOutcome operation returned by the service.
* @sample AmazonFraudDetectorAsync.DeleteOutcome
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteOutcomeAsync(DeleteOutcomeRequest deleteOutcomeRequest);
/**
*
* Deletes an outcome.
*
*
* You cannot delete an outcome that is used in a rule version.
*
*
* When you delete an outcome, Amazon Fraud Detector permanently deletes that outcome and the data is no longer
* stored in Amazon Fraud Detector.
*
*
* @param deleteOutcomeRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteOutcome operation returned by the service.
* @sample AmazonFraudDetectorAsyncHandler.DeleteOutcome
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteOutcomeAsync(DeleteOutcomeRequest deleteOutcomeRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the rule. You cannot delete a rule if it is used by an ACTIVE
or INACTIVE
* detector version.
*
*
* When you delete a rule, Amazon Fraud Detector permanently deletes that rule and the data is no longer stored in
* Amazon Fraud Detector.
*
*
* @param deleteRuleRequest
* @return A Java Future containing the result of the DeleteRule operation returned by the service.
* @sample AmazonFraudDetectorAsync.DeleteRule
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteRuleAsync(DeleteRuleRequest deleteRuleRequest);
/**
*
* Deletes the rule. You cannot delete a rule if it is used by an ACTIVE
or INACTIVE
* detector version.
*
*
* When you delete a rule, Amazon Fraud Detector permanently deletes that rule and the data is no longer stored in
* Amazon Fraud Detector.
*
*
* @param deleteRuleRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteRule operation returned by the service.
* @sample AmazonFraudDetectorAsyncHandler.DeleteRule
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteRuleAsync(DeleteRuleRequest deleteRuleRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a variable.
*
*
* You can't delete variables that are included in an event type in Amazon Fraud Detector.
*
*
* Amazon Fraud Detector automatically deletes model output variables and SageMaker model output variables when you
* delete the model. You can't delete these variables manually.
*
*
* When you delete a variable, Amazon Fraud Detector permanently deletes that variable and the data is no longer
* stored in Amazon Fraud Detector.
*
*
* @param deleteVariableRequest
* @return A Java Future containing the result of the DeleteVariable operation returned by the service.
* @sample AmazonFraudDetectorAsync.DeleteVariable
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteVariableAsync(DeleteVariableRequest deleteVariableRequest);
/**
*
* Deletes a variable.
*
*
* You can't delete variables that are included in an event type in Amazon Fraud Detector.
*
*
* Amazon Fraud Detector automatically deletes model output variables and SageMaker model output variables when you
* delete the model. You can't delete these variables manually.
*
*
* When you delete a variable, Amazon Fraud Detector permanently deletes that variable and the data is no longer
* stored in Amazon Fraud Detector.
*
*
* @param deleteVariableRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteVariable operation returned by the service.
* @sample AmazonFraudDetectorAsyncHandler.DeleteVariable
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteVariableAsync(DeleteVariableRequest deleteVariableRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets all versions for a specified detector.
*
*
* @param describeDetectorRequest
* @return A Java Future containing the result of the DescribeDetector operation returned by the service.
* @sample AmazonFraudDetectorAsync.DescribeDetector
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeDetectorAsync(DescribeDetectorRequest describeDetectorRequest);
/**
*
* Gets all versions for a specified detector.
*
*
* @param describeDetectorRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeDetector operation returned by the service.
* @sample AmazonFraudDetectorAsyncHandler.DescribeDetector
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeDetectorAsync(DescribeDetectorRequest describeDetectorRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets all of the model versions for the specified model type or for the specified model type and model ID. You can
* also get details for a single, specified model version.
*
*
* @param describeModelVersionsRequest
* @return A Java Future containing the result of the DescribeModelVersions operation returned by the service.
* @sample AmazonFraudDetectorAsync.DescribeModelVersions
* @see AWS API Documentation
*/
java.util.concurrent.Future describeModelVersionsAsync(DescribeModelVersionsRequest describeModelVersionsRequest);
/**
*
* Gets all of the model versions for the specified model type or for the specified model type and model ID. You can
* also get details for a single, specified model version.
*
*
* @param describeModelVersionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeModelVersions operation returned by the service.
* @sample AmazonFraudDetectorAsyncHandler.DescribeModelVersions
* @see AWS API Documentation
*/
java.util.concurrent.Future describeModelVersionsAsync(DescribeModelVersionsRequest describeModelVersionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets all batch import jobs or a specific job of the specified ID. This is a paginated API. If you provide a null
* maxResults
, this action retrieves a maximum of 50 records per page. If you provide a
* maxResults
, the value must be between 1 and 50. To get the next page results, provide the pagination
* token from the GetBatchImportJobsResponse
as part of your request. A null pagination token fetches
* the records from the beginning.
*
*
* @param getBatchImportJobsRequest
* @return A Java Future containing the result of the GetBatchImportJobs operation returned by the service.
* @sample AmazonFraudDetectorAsync.GetBatchImportJobs
* @see AWS API Documentation
*/
java.util.concurrent.Future getBatchImportJobsAsync(GetBatchImportJobsRequest getBatchImportJobsRequest);
/**
*
* Gets all batch import jobs or a specific job of the specified ID. This is a paginated API. If you provide a null
* maxResults
, this action retrieves a maximum of 50 records per page. If you provide a
* maxResults
, the value must be between 1 and 50. To get the next page results, provide the pagination
* token from the GetBatchImportJobsResponse
as part of your request. A null pagination token fetches
* the records from the beginning.
*
*
* @param getBatchImportJobsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetBatchImportJobs operation returned by the service.
* @sample AmazonFraudDetectorAsyncHandler.GetBatchImportJobs
* @see AWS API Documentation
*/
java.util.concurrent.Future getBatchImportJobsAsync(GetBatchImportJobsRequest getBatchImportJobsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets all batch prediction jobs or a specific job if you specify a job ID. This is a paginated API. If you provide
* a null maxResults, this action retrieves a maximum of 50 records per page. If you provide a maxResults, the value
* must be between 1 and 50. To get the next page results, provide the pagination token from the
* GetBatchPredictionJobsResponse as part of your request. A null pagination token fetches the records from the
* beginning.
*
*
* @param getBatchPredictionJobsRequest
* @return A Java Future containing the result of the GetBatchPredictionJobs operation returned by the service.
* @sample AmazonFraudDetectorAsync.GetBatchPredictionJobs
* @see AWS API Documentation
*/
java.util.concurrent.Future getBatchPredictionJobsAsync(GetBatchPredictionJobsRequest getBatchPredictionJobsRequest);
/**
*
* Gets all batch prediction jobs or a specific job if you specify a job ID. This is a paginated API. If you provide
* a null maxResults, this action retrieves a maximum of 50 records per page. If you provide a maxResults, the value
* must be between 1 and 50. To get the next page results, provide the pagination token from the
* GetBatchPredictionJobsResponse as part of your request. A null pagination token fetches the records from the
* beginning.
*
*
* @param getBatchPredictionJobsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetBatchPredictionJobs operation returned by the service.
* @sample AmazonFraudDetectorAsyncHandler.GetBatchPredictionJobs
* @see AWS API Documentation
*/
java.util.concurrent.Future getBatchPredictionJobsAsync(GetBatchPredictionJobsRequest getBatchPredictionJobsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves the status of a DeleteEventsByEventType
action.
*
*
* @param getDeleteEventsByEventTypeStatusRequest
* @return A Java Future containing the result of the GetDeleteEventsByEventTypeStatus operation returned by the
* service.
* @sample AmazonFraudDetectorAsync.GetDeleteEventsByEventTypeStatus
* @see AWS API Documentation
*/
java.util.concurrent.Future getDeleteEventsByEventTypeStatusAsync(
GetDeleteEventsByEventTypeStatusRequest getDeleteEventsByEventTypeStatusRequest);
/**
*
* Retrieves the status of a DeleteEventsByEventType
action.
*
*
* @param getDeleteEventsByEventTypeStatusRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetDeleteEventsByEventTypeStatus operation returned by the
* service.
* @sample AmazonFraudDetectorAsyncHandler.GetDeleteEventsByEventTypeStatus
* @see AWS API Documentation
*/
java.util.concurrent.Future getDeleteEventsByEventTypeStatusAsync(
GetDeleteEventsByEventTypeStatusRequest getDeleteEventsByEventTypeStatusRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets a particular detector version.
*
*
* @param getDetectorVersionRequest
* @return A Java Future containing the result of the GetDetectorVersion operation returned by the service.
* @sample AmazonFraudDetectorAsync.GetDetectorVersion
* @see AWS API Documentation
*/
java.util.concurrent.Future getDetectorVersionAsync(GetDetectorVersionRequest getDetectorVersionRequest);
/**
*
* Gets a particular detector version.
*
*
* @param getDetectorVersionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetDetectorVersion operation returned by the service.
* @sample AmazonFraudDetectorAsyncHandler.GetDetectorVersion
* @see AWS API Documentation
*/
java.util.concurrent.Future getDetectorVersionAsync(GetDetectorVersionRequest getDetectorVersionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets all detectors or a single detector if a detectorId
is specified. This is a paginated API. If
* you provide a null maxResults
, this action retrieves a maximum of 10 records per page. If you
* provide a maxResults
, the value must be between 5 and 10. To get the next page results, provide the
* pagination token from the GetDetectorsResponse
as part of your request. A null pagination token
* fetches the records from the beginning.
*
*
* @param getDetectorsRequest
* @return A Java Future containing the result of the GetDetectors operation returned by the service.
* @sample AmazonFraudDetectorAsync.GetDetectors
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getDetectorsAsync(GetDetectorsRequest getDetectorsRequest);
/**
*
* Gets all detectors or a single detector if a detectorId
is specified. This is a paginated API. If
* you provide a null maxResults
, this action retrieves a maximum of 10 records per page. If you
* provide a maxResults
, the value must be between 5 and 10. To get the next page results, provide the
* pagination token from the GetDetectorsResponse
as part of your request. A null pagination token
* fetches the records from the beginning.
*
*
* @param getDetectorsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetDetectors operation returned by the service.
* @sample AmazonFraudDetectorAsyncHandler.GetDetectors
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getDetectorsAsync(GetDetectorsRequest getDetectorsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets all entity types or a specific entity type if a name is specified. This is a paginated API. If you provide a
* null maxResults
, this action retrieves a maximum of 10 records per page. If you provide a
* maxResults
, the value must be between 5 and 10. To get the next page results, provide the pagination
* token from the GetEntityTypesResponse
as part of your request. A null pagination token fetches the
* records from the beginning.
*
*
* @param getEntityTypesRequest
* @return A Java Future containing the result of the GetEntityTypes operation returned by the service.
* @sample AmazonFraudDetectorAsync.GetEntityTypes
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getEntityTypesAsync(GetEntityTypesRequest getEntityTypesRequest);
/**
*
* Gets all entity types or a specific entity type if a name is specified. This is a paginated API. If you provide a
* null maxResults
, this action retrieves a maximum of 10 records per page. If you provide a
* maxResults
, the value must be between 5 and 10. To get the next page results, provide the pagination
* token from the GetEntityTypesResponse
as part of your request. A null pagination token fetches the
* records from the beginning.
*
*
* @param getEntityTypesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetEntityTypes operation returned by the service.
* @sample AmazonFraudDetectorAsyncHandler.GetEntityTypes
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getEntityTypesAsync(GetEntityTypesRequest getEntityTypesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves details of events stored with Amazon Fraud Detector. This action does not retrieve prediction results.
*
*
* @param getEventRequest
* @return A Java Future containing the result of the GetEvent operation returned by the service.
* @sample AmazonFraudDetectorAsync.GetEvent
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getEventAsync(GetEventRequest getEventRequest);
/**
*
* Retrieves details of events stored with Amazon Fraud Detector. This action does not retrieve prediction results.
*
*
* @param getEventRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetEvent operation returned by the service.
* @sample AmazonFraudDetectorAsyncHandler.GetEvent
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getEventAsync(GetEventRequest getEventRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Evaluates an event against a detector version. If a version ID is not provided, the detector’s (
* ACTIVE
) version is used.
*
*
* @param getEventPredictionRequest
* @return A Java Future containing the result of the GetEventPrediction operation returned by the service.
* @sample AmazonFraudDetectorAsync.GetEventPrediction
* @see AWS API Documentation
*/
java.util.concurrent.Future getEventPredictionAsync(GetEventPredictionRequest getEventPredictionRequest);
/**
*
* Evaluates an event against a detector version. If a version ID is not provided, the detector’s (
* ACTIVE
) version is used.
*
*
* @param getEventPredictionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetEventPrediction operation returned by the service.
* @sample AmazonFraudDetectorAsyncHandler.GetEventPrediction
* @see AWS API Documentation
*/
java.util.concurrent.Future getEventPredictionAsync(GetEventPredictionRequest getEventPredictionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets details of the past fraud predictions for the specified event ID, event type, detector ID, and detector
* version ID that was generated in the specified time period.
*
*
* @param getEventPredictionMetadataRequest
* @return A Java Future containing the result of the GetEventPredictionMetadata operation returned by the service.
* @sample AmazonFraudDetectorAsync.GetEventPredictionMetadata
* @see AWS API Documentation
*/
java.util.concurrent.Future getEventPredictionMetadataAsync(
GetEventPredictionMetadataRequest getEventPredictionMetadataRequest);
/**
*
* Gets details of the past fraud predictions for the specified event ID, event type, detector ID, and detector
* version ID that was generated in the specified time period.
*
*
* @param getEventPredictionMetadataRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetEventPredictionMetadata operation returned by the service.
* @sample AmazonFraudDetectorAsyncHandler.GetEventPredictionMetadata
* @see AWS API Documentation
*/
java.util.concurrent.Future getEventPredictionMetadataAsync(
GetEventPredictionMetadataRequest getEventPredictionMetadataRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets all event types or a specific event type if name is provided. This is a paginated API. If you provide a null
* maxResults
, this action retrieves a maximum of 10 records per page. If you provide a
* maxResults
, the value must be between 5 and 10. To get the next page results, provide the pagination
* token from the GetEventTypesResponse
as part of your request. A null pagination token fetches the
* records from the beginning.
*
*
* @param getEventTypesRequest
* @return A Java Future containing the result of the GetEventTypes operation returned by the service.
* @sample AmazonFraudDetectorAsync.GetEventTypes
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getEventTypesAsync(GetEventTypesRequest getEventTypesRequest);
/**
*
* Gets all event types or a specific event type if name is provided. This is a paginated API. If you provide a null
* maxResults
, this action retrieves a maximum of 10 records per page. If you provide a
* maxResults
, the value must be between 5 and 10. To get the next page results, provide the pagination
* token from the GetEventTypesResponse
as part of your request. A null pagination token fetches the
* records from the beginning.
*
*
* @param getEventTypesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetEventTypes operation returned by the service.
* @sample AmazonFraudDetectorAsyncHandler.GetEventTypes
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getEventTypesAsync(GetEventTypesRequest getEventTypesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets the details for one or more Amazon SageMaker models that have been imported into the service. This is a
* paginated API. If you provide a null maxResults
, this actions retrieves a maximum of 10 records per
* page. If you provide a maxResults
, the value must be between 5 and 10. To get the next page results,
* provide the pagination token from the GetExternalModelsResult
as part of your request. A null
* pagination token fetches the records from the beginning.
*
*
* @param getExternalModelsRequest
* @return A Java Future containing the result of the GetExternalModels operation returned by the service.
* @sample AmazonFraudDetectorAsync.GetExternalModels
* @see AWS API Documentation
*/
java.util.concurrent.Future getExternalModelsAsync(GetExternalModelsRequest getExternalModelsRequest);
/**
*
* Gets the details for one or more Amazon SageMaker models that have been imported into the service. This is a
* paginated API. If you provide a null maxResults
, this actions retrieves a maximum of 10 records per
* page. If you provide a maxResults
, the value must be between 5 and 10. To get the next page results,
* provide the pagination token from the GetExternalModelsResult
as part of your request. A null
* pagination token fetches the records from the beginning.
*
*
* @param getExternalModelsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetExternalModels operation returned by the service.
* @sample AmazonFraudDetectorAsyncHandler.GetExternalModels
* @see AWS API Documentation
*/
java.util.concurrent.Future getExternalModelsAsync(GetExternalModelsRequest getExternalModelsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets the encryption key if a KMS key has been specified to be used to encrypt content in Amazon Fraud Detector.
*
*
* @param getKMSEncryptionKeyRequest
* @return A Java Future containing the result of the GetKMSEncryptionKey operation returned by the service.
* @sample AmazonFraudDetectorAsync.GetKMSEncryptionKey
* @see AWS API Documentation
*/
java.util.concurrent.Future getKMSEncryptionKeyAsync(GetKMSEncryptionKeyRequest getKMSEncryptionKeyRequest);
/**
*
* Gets the encryption key if a KMS key has been specified to be used to encrypt content in Amazon Fraud Detector.
*
*
* @param getKMSEncryptionKeyRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetKMSEncryptionKey operation returned by the service.
* @sample AmazonFraudDetectorAsyncHandler.GetKMSEncryptionKey
* @see AWS API Documentation
*/
java.util.concurrent.Future getKMSEncryptionKeyAsync(GetKMSEncryptionKeyRequest getKMSEncryptionKeyRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets all labels or a specific label if name is provided. This is a paginated API. If you provide a null
* maxResults
, this action retrieves a maximum of 50 records per page. If you provide a
* maxResults
, the value must be between 10 and 50. To get the next page results, provide the
* pagination token from the GetGetLabelsResponse
as part of your request. A null pagination token
* fetches the records from the beginning.
*
*
* @param getLabelsRequest
* @return A Java Future containing the result of the GetLabels operation returned by the service.
* @sample AmazonFraudDetectorAsync.GetLabels
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getLabelsAsync(GetLabelsRequest getLabelsRequest);
/**
*
* Gets all labels or a specific label if name is provided. This is a paginated API. If you provide a null
* maxResults
, this action retrieves a maximum of 50 records per page. If you provide a
* maxResults
, the value must be between 10 and 50. To get the next page results, provide the
* pagination token from the GetGetLabelsResponse
as part of your request. A null pagination token
* fetches the records from the beginning.
*
*
* @param getLabelsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetLabels operation returned by the service.
* @sample AmazonFraudDetectorAsyncHandler.GetLabels
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getLabelsAsync(GetLabelsRequest getLabelsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets all the elements in the specified list.
*
*
* @param getListElementsRequest
* @return A Java Future containing the result of the GetListElements operation returned by the service.
* @sample AmazonFraudDetectorAsync.GetListElements
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getListElementsAsync(GetListElementsRequest getListElementsRequest);
/**
*
* Gets all the elements in the specified list.
*
*
* @param getListElementsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetListElements operation returned by the service.
* @sample AmazonFraudDetectorAsyncHandler.GetListElements
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getListElementsAsync(GetListElementsRequest getListElementsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets the metadata of either all the lists under the account or the specified list.
*
*
* @param getListsMetadataRequest
* @return A Java Future containing the result of the GetListsMetadata operation returned by the service.
* @sample AmazonFraudDetectorAsync.GetListsMetadata
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getListsMetadataAsync(GetListsMetadataRequest getListsMetadataRequest);
/**
*
* Gets the metadata of either all the lists under the account or the specified list.
*
*
* @param getListsMetadataRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetListsMetadata operation returned by the service.
* @sample AmazonFraudDetectorAsyncHandler.GetListsMetadata
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getListsMetadataAsync(GetListsMetadataRequest getListsMetadataRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets the details of the specified model version.
*
*
* @param getModelVersionRequest
* @return A Java Future containing the result of the GetModelVersion operation returned by the service.
* @sample AmazonFraudDetectorAsync.GetModelVersion
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getModelVersionAsync(GetModelVersionRequest getModelVersionRequest);
/**
*
* Gets the details of the specified model version.
*
*
* @param getModelVersionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetModelVersion operation returned by the service.
* @sample AmazonFraudDetectorAsyncHandler.GetModelVersion
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getModelVersionAsync(GetModelVersionRequest getModelVersionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets one or more models. Gets all models for the Amazon Web Services account if no model type and no model id
* provided. Gets all models for the Amazon Web Services account and model type, if the model type is specified but
* model id is not provided. Gets a specific model if (model type, model id) tuple is specified.
*
*
* This is a paginated API. If you provide a null maxResults
, this action retrieves a maximum of 10
* records per page. If you provide a maxResults
, the value must be between 1 and 10. To get the next
* page results, provide the pagination token from the response as part of your request. A null pagination token
* fetches the records from the beginning.
*
*
* @param getModelsRequest
* @return A Java Future containing the result of the GetModels operation returned by the service.
* @sample AmazonFraudDetectorAsync.GetModels
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getModelsAsync(GetModelsRequest getModelsRequest);
/**
*
* Gets one or more models. Gets all models for the Amazon Web Services account if no model type and no model id
* provided. Gets all models for the Amazon Web Services account and model type, if the model type is specified but
* model id is not provided. Gets a specific model if (model type, model id) tuple is specified.
*
*
* This is a paginated API. If you provide a null maxResults
, this action retrieves a maximum of 10
* records per page. If you provide a maxResults
, the value must be between 1 and 10. To get the next
* page results, provide the pagination token from the response as part of your request. A null pagination token
* fetches the records from the beginning.
*
*
* @param getModelsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetModels operation returned by the service.
* @sample AmazonFraudDetectorAsyncHandler.GetModels
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getModelsAsync(GetModelsRequest getModelsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets one or more outcomes. This is a paginated API. If you provide a null maxResults
, this actions
* retrieves a maximum of 100 records per page. If you provide a maxResults
, the value must be between
* 50 and 100. To get the next page results, provide the pagination token from the GetOutcomesResult
as
* part of your request. A null pagination token fetches the records from the beginning.
*
*
* @param getOutcomesRequest
* @return A Java Future containing the result of the GetOutcomes operation returned by the service.
* @sample AmazonFraudDetectorAsync.GetOutcomes
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getOutcomesAsync(GetOutcomesRequest getOutcomesRequest);
/**
*
* Gets one or more outcomes. This is a paginated API. If you provide a null maxResults
, this actions
* retrieves a maximum of 100 records per page. If you provide a maxResults
, the value must be between
* 50 and 100. To get the next page results, provide the pagination token from the GetOutcomesResult
as
* part of your request. A null pagination token fetches the records from the beginning.
*
*
* @param getOutcomesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetOutcomes operation returned by the service.
* @sample AmazonFraudDetectorAsyncHandler.GetOutcomes
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getOutcomesAsync(GetOutcomesRequest getOutcomesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Get all rules for a detector (paginated) if ruleId
and ruleVersion
are not specified.
* Gets all rules for the detector and the ruleId
if present (paginated). Gets a specific rule if both
* the ruleId
and the ruleVersion
are specified.
*
*
* This is a paginated API. Providing null maxResults results in retrieving maximum of 100 records per page. If you
* provide maxResults the value must be between 50 and 100. To get the next page result, a provide a pagination
* token from GetRulesResult as part of your request. Null pagination token fetches the records from the beginning.
*
*
* @param getRulesRequest
* @return A Java Future containing the result of the GetRules operation returned by the service.
* @sample AmazonFraudDetectorAsync.GetRules
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getRulesAsync(GetRulesRequest getRulesRequest);
/**
*
* Get all rules for a detector (paginated) if ruleId
and ruleVersion
are not specified.
* Gets all rules for the detector and the ruleId
if present (paginated). Gets a specific rule if both
* the ruleId
and the ruleVersion
are specified.
*
*
* This is a paginated API. Providing null maxResults results in retrieving maximum of 100 records per page. If you
* provide maxResults the value must be between 50 and 100. To get the next page result, a provide a pagination
* token from GetRulesResult as part of your request. Null pagination token fetches the records from the beginning.
*
*
* @param getRulesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetRules operation returned by the service.
* @sample AmazonFraudDetectorAsyncHandler.GetRules
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getRulesAsync(GetRulesRequest getRulesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets all of the variables or the specific variable. This is a paginated API. Providing null
* maxSizePerPage
results in retrieving maximum of 100 records per page. If you provide
* maxSizePerPage
the value must be between 50 and 100. To get the next page result, a provide a
* pagination token from GetVariablesResult
as part of your request. Null pagination token fetches the
* records from the beginning.
*
*
* @param getVariablesRequest
* @return A Java Future containing the result of the GetVariables operation returned by the service.
* @sample AmazonFraudDetectorAsync.GetVariables
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getVariablesAsync(GetVariablesRequest getVariablesRequest);
/**
*
* Gets all of the variables or the specific variable. This is a paginated API. Providing null
* maxSizePerPage
results in retrieving maximum of 100 records per page. If you provide
* maxSizePerPage
the value must be between 50 and 100. To get the next page result, a provide a
* pagination token from GetVariablesResult
as part of your request. Null pagination token fetches the
* records from the beginning.
*
*
* @param getVariablesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetVariables operation returned by the service.
* @sample AmazonFraudDetectorAsyncHandler.GetVariables
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getVariablesAsync(GetVariablesRequest getVariablesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets a list of past predictions. The list can be filtered by detector ID, detector version ID, event ID, event
* type, or by specifying a time period. If filter is not specified, the most recent prediction is returned.
*
*
* For example, the following filter lists all past predictions for xyz
event type -
* { "eventType":{ "value": "xyz" }” }
*
*
* This is a paginated API. If you provide a null maxResults
, this action will retrieve a maximum of 10
* records per page. If you provide a maxResults
, the value must be between 50 and 100. To get the next
* page results, provide the nextToken
from the response as part of your request. A null
* nextToken
fetches the records from the beginning.
*
*
* @param listEventPredictionsRequest
* @return A Java Future containing the result of the ListEventPredictions operation returned by the service.
* @sample AmazonFraudDetectorAsync.ListEventPredictions
* @see AWS API Documentation
*/
java.util.concurrent.Future listEventPredictionsAsync(ListEventPredictionsRequest listEventPredictionsRequest);
/**
*
* Gets a list of past predictions. The list can be filtered by detector ID, detector version ID, event ID, event
* type, or by specifying a time period. If filter is not specified, the most recent prediction is returned.
*
*
* For example, the following filter lists all past predictions for xyz
event type -
* { "eventType":{ "value": "xyz" }” }
*
*
* This is a paginated API. If you provide a null maxResults
, this action will retrieve a maximum of 10
* records per page. If you provide a maxResults
, the value must be between 50 and 100. To get the next
* page results, provide the nextToken
from the response as part of your request. A null
* nextToken
fetches the records from the beginning.
*
*
* @param listEventPredictionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListEventPredictions operation returned by the service.
* @sample AmazonFraudDetectorAsyncHandler.ListEventPredictions
* @see AWS API Documentation
*/
java.util.concurrent.Future listEventPredictionsAsync(ListEventPredictionsRequest listEventPredictionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists all tags associated with the resource. This is a paginated API. To get the next page results, provide the
* pagination token from the response as part of your request. A null pagination token fetches the records from the
* beginning.
*
*
* @param listTagsForResourceRequest
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AmazonFraudDetectorAsync.ListTagsForResource
* @see AWS API Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest);
/**
*
* Lists all tags associated with the resource. This is a paginated API. To get the next page results, provide the
* pagination token from the response as part of your request. A null pagination token fetches the records from the
* beginning.
*
*
* @param listTagsForResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AmazonFraudDetectorAsyncHandler.ListTagsForResource
* @see AWS API Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates or updates a detector.
*
*
* @param putDetectorRequest
* @return A Java Future containing the result of the PutDetector operation returned by the service.
* @sample AmazonFraudDetectorAsync.PutDetector
* @see AWS API
* Documentation
*/
java.util.concurrent.Future putDetectorAsync(PutDetectorRequest putDetectorRequest);
/**
*
* Creates or updates a detector.
*
*
* @param putDetectorRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the PutDetector operation returned by the service.
* @sample AmazonFraudDetectorAsyncHandler.PutDetector
* @see AWS API
* Documentation
*/
java.util.concurrent.Future putDetectorAsync(PutDetectorRequest putDetectorRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates or updates an entity type. An entity represents who is performing the event. As part of a fraud
* prediction, you pass the entity ID to indicate the specific entity who performed the event. An entity type
* classifies the entity. Example classifications include customer, merchant, or account.
*
*
* @param putEntityTypeRequest
* @return A Java Future containing the result of the PutEntityType operation returned by the service.
* @sample AmazonFraudDetectorAsync.PutEntityType
* @see AWS
* API Documentation
*/
java.util.concurrent.Future putEntityTypeAsync(PutEntityTypeRequest putEntityTypeRequest);
/**
*
* Creates or updates an entity type. An entity represents who is performing the event. As part of a fraud
* prediction, you pass the entity ID to indicate the specific entity who performed the event. An entity type
* classifies the entity. Example classifications include customer, merchant, or account.
*
*
* @param putEntityTypeRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the PutEntityType operation returned by the service.
* @sample AmazonFraudDetectorAsyncHandler.PutEntityType
* @see AWS
* API Documentation
*/
java.util.concurrent.Future putEntityTypeAsync(PutEntityTypeRequest putEntityTypeRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates or updates an event type. An event is a business activity that is evaluated for fraud risk. With Amazon
* Fraud Detector, you generate fraud predictions for events. An event type defines the structure for an event sent
* to Amazon Fraud Detector. This includes the variables sent as part of the event, the entity performing the event
* (such as a customer), and the labels that classify the event. Example event types include online payment
* transactions, account registrations, and authentications.
*
*
* @param putEventTypeRequest
* @return A Java Future containing the result of the PutEventType operation returned by the service.
* @sample AmazonFraudDetectorAsync.PutEventType
* @see AWS API
* Documentation
*/
java.util.concurrent.Future putEventTypeAsync(PutEventTypeRequest putEventTypeRequest);
/**
*
* Creates or updates an event type. An event is a business activity that is evaluated for fraud risk. With Amazon
* Fraud Detector, you generate fraud predictions for events. An event type defines the structure for an event sent
* to Amazon Fraud Detector. This includes the variables sent as part of the event, the entity performing the event
* (such as a customer), and the labels that classify the event. Example event types include online payment
* transactions, account registrations, and authentications.
*
*
* @param putEventTypeRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the PutEventType operation returned by the service.
* @sample AmazonFraudDetectorAsyncHandler.PutEventType
* @see AWS API
* Documentation
*/
java.util.concurrent.Future putEventTypeAsync(PutEventTypeRequest putEventTypeRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates or updates an Amazon SageMaker model endpoint. You can also use this action to update the configuration
* of the model endpoint, including the IAM role and/or the mapped variables.
*
*
* @param putExternalModelRequest
* @return A Java Future containing the result of the PutExternalModel operation returned by the service.
* @sample AmazonFraudDetectorAsync.PutExternalModel
* @see AWS
* API Documentation
*/
java.util.concurrent.Future putExternalModelAsync(PutExternalModelRequest putExternalModelRequest);
/**
*
* Creates or updates an Amazon SageMaker model endpoint. You can also use this action to update the configuration
* of the model endpoint, including the IAM role and/or the mapped variables.
*
*
* @param putExternalModelRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the PutExternalModel operation returned by the service.
* @sample AmazonFraudDetectorAsyncHandler.PutExternalModel
* @see AWS
* API Documentation
*/
java.util.concurrent.Future putExternalModelAsync(PutExternalModelRequest putExternalModelRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Specifies the KMS key to be used to encrypt content in Amazon Fraud Detector.
*
*
* @param putKMSEncryptionKeyRequest
* @return A Java Future containing the result of the PutKMSEncryptionKey operation returned by the service.
* @sample AmazonFraudDetectorAsync.PutKMSEncryptionKey
* @see AWS API Documentation
*/
java.util.concurrent.Future putKMSEncryptionKeyAsync(PutKMSEncryptionKeyRequest putKMSEncryptionKeyRequest);
/**
*
* Specifies the KMS key to be used to encrypt content in Amazon Fraud Detector.
*
*
* @param putKMSEncryptionKeyRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the PutKMSEncryptionKey operation returned by the service.
* @sample AmazonFraudDetectorAsyncHandler.PutKMSEncryptionKey
* @see AWS API Documentation
*/
java.util.concurrent.Future putKMSEncryptionKeyAsync(PutKMSEncryptionKeyRequest putKMSEncryptionKeyRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates or updates label. A label classifies an event as fraudulent or legitimate. Labels are associated with
* event types and used to train supervised machine learning models in Amazon Fraud Detector.
*
*
* @param putLabelRequest
* @return A Java Future containing the result of the PutLabel operation returned by the service.
* @sample AmazonFraudDetectorAsync.PutLabel
* @see AWS API
* Documentation
*/
java.util.concurrent.Future putLabelAsync(PutLabelRequest putLabelRequest);
/**
*
* Creates or updates label. A label classifies an event as fraudulent or legitimate. Labels are associated with
* event types and used to train supervised machine learning models in Amazon Fraud Detector.
*
*
* @param putLabelRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the PutLabel operation returned by the service.
* @sample AmazonFraudDetectorAsyncHandler.PutLabel
* @see AWS API
* Documentation
*/
java.util.concurrent.Future putLabelAsync(PutLabelRequest putLabelRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates or updates an outcome.
*
*
* @param putOutcomeRequest
* @return A Java Future containing the result of the PutOutcome operation returned by the service.
* @sample AmazonFraudDetectorAsync.PutOutcome
* @see AWS API
* Documentation
*/
java.util.concurrent.Future putOutcomeAsync(PutOutcomeRequest putOutcomeRequest);
/**
*
* Creates or updates an outcome.
*
*
* @param putOutcomeRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the PutOutcome operation returned by the service.
* @sample AmazonFraudDetectorAsyncHandler.PutOutcome
* @see AWS API
* Documentation
*/
java.util.concurrent.Future putOutcomeAsync(PutOutcomeRequest putOutcomeRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Stores events in Amazon Fraud Detector without generating fraud predictions for those events. For example, you
* can use SendEvent
to upload a historical dataset, which you can then later use to train a model.
*
*
* @param sendEventRequest
* @return A Java Future containing the result of the SendEvent operation returned by the service.
* @sample AmazonFraudDetectorAsync.SendEvent
* @see AWS API
* Documentation
*/
java.util.concurrent.Future sendEventAsync(SendEventRequest sendEventRequest);
/**
*
* Stores events in Amazon Fraud Detector without generating fraud predictions for those events. For example, you
* can use SendEvent
to upload a historical dataset, which you can then later use to train a model.
*
*
* @param sendEventRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the SendEvent operation returned by the service.
* @sample AmazonFraudDetectorAsyncHandler.SendEvent
* @see AWS API
* Documentation
*/
java.util.concurrent.Future sendEventAsync(SendEventRequest sendEventRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Assigns tags to a resource.
*
*
* @param tagResourceRequest
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AmazonFraudDetectorAsync.TagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest);
/**
*
* Assigns tags to a resource.
*
*
* @param tagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AmazonFraudDetectorAsyncHandler.TagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Removes tags from a resource.
*
*
* @param untagResourceRequest
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AmazonFraudDetectorAsync.UntagResource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest);
/**
*
* Removes tags from a resource.
*
*
* @param untagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AmazonFraudDetectorAsyncHandler.UntagResource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates a detector version. The detector version attributes that you can update include models, external model
* endpoints, rules, rule execution mode, and description. You can only update a DRAFT
detector
* version.
*
*
* @param updateDetectorVersionRequest
* @return A Java Future containing the result of the UpdateDetectorVersion operation returned by the service.
* @sample AmazonFraudDetectorAsync.UpdateDetectorVersion
* @see AWS API Documentation
*/
java.util.concurrent.Future updateDetectorVersionAsync(UpdateDetectorVersionRequest updateDetectorVersionRequest);
/**
*
* Updates a detector version. The detector version attributes that you can update include models, external model
* endpoints, rules, rule execution mode, and description. You can only update a DRAFT
detector
* version.
*
*
* @param updateDetectorVersionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateDetectorVersion operation returned by the service.
* @sample AmazonFraudDetectorAsyncHandler.UpdateDetectorVersion
* @see AWS API Documentation
*/
java.util.concurrent.Future updateDetectorVersionAsync(UpdateDetectorVersionRequest updateDetectorVersionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates the detector version's description. You can update the metadata for any detector version (
* DRAFT, ACTIVE,
or INACTIVE
).
*
*
* @param updateDetectorVersionMetadataRequest
* @return A Java Future containing the result of the UpdateDetectorVersionMetadata operation returned by the
* service.
* @sample AmazonFraudDetectorAsync.UpdateDetectorVersionMetadata
* @see AWS API Documentation
*/
java.util.concurrent.Future updateDetectorVersionMetadataAsync(
UpdateDetectorVersionMetadataRequest updateDetectorVersionMetadataRequest);
/**
*
* Updates the detector version's description. You can update the metadata for any detector version (
* DRAFT, ACTIVE,
or INACTIVE
).
*
*
* @param updateDetectorVersionMetadataRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateDetectorVersionMetadata operation returned by the
* service.
* @sample AmazonFraudDetectorAsyncHandler.UpdateDetectorVersionMetadata
* @see AWS API Documentation
*/
java.util.concurrent.Future updateDetectorVersionMetadataAsync(
UpdateDetectorVersionMetadataRequest updateDetectorVersionMetadataRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates the detector version’s status. You can perform the following promotions or demotions using
* UpdateDetectorVersionStatus
: DRAFT
to ACTIVE
, ACTIVE
to
* INACTIVE
, and INACTIVE
to ACTIVE
.
*
*
* @param updateDetectorVersionStatusRequest
* @return A Java Future containing the result of the UpdateDetectorVersionStatus operation returned by the service.
* @sample AmazonFraudDetectorAsync.UpdateDetectorVersionStatus
* @see AWS API Documentation
*/
java.util.concurrent.Future updateDetectorVersionStatusAsync(
UpdateDetectorVersionStatusRequest updateDetectorVersionStatusRequest);
/**
*
* Updates the detector version’s status. You can perform the following promotions or demotions using
* UpdateDetectorVersionStatus
: DRAFT
to ACTIVE
, ACTIVE
to
* INACTIVE
, and INACTIVE
to ACTIVE
.
*
*
* @param updateDetectorVersionStatusRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateDetectorVersionStatus operation returned by the service.
* @sample AmazonFraudDetectorAsyncHandler.UpdateDetectorVersionStatus
* @see AWS API Documentation
*/
java.util.concurrent.Future updateDetectorVersionStatusAsync(
UpdateDetectorVersionStatusRequest updateDetectorVersionStatusRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates the specified event with a new label.
*
*
* @param updateEventLabelRequest
* @return A Java Future containing the result of the UpdateEventLabel operation returned by the service.
* @sample AmazonFraudDetectorAsync.UpdateEventLabel
* @see AWS
* API Documentation
*/
java.util.concurrent.Future updateEventLabelAsync(UpdateEventLabelRequest updateEventLabelRequest);
/**
*
* Updates the specified event with a new label.
*
*
* @param updateEventLabelRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateEventLabel operation returned by the service.
* @sample AmazonFraudDetectorAsyncHandler.UpdateEventLabel
* @see AWS
* API Documentation
*/
java.util.concurrent.Future updateEventLabelAsync(UpdateEventLabelRequest updateEventLabelRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates a list.
*
*
* @param updateListRequest
* @return A Java Future containing the result of the UpdateList operation returned by the service.
* @sample AmazonFraudDetectorAsync.UpdateList
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateListAsync(UpdateListRequest updateListRequest);
/**
*
* Updates a list.
*
*
* @param updateListRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateList operation returned by the service.
* @sample AmazonFraudDetectorAsyncHandler.UpdateList
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateListAsync(UpdateListRequest updateListRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates model description.
*
*
* @param updateModelRequest
* @return A Java Future containing the result of the UpdateModel operation returned by the service.
* @sample AmazonFraudDetectorAsync.UpdateModel
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateModelAsync(UpdateModelRequest updateModelRequest);
/**
*
* Updates model description.
*
*
* @param updateModelRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateModel operation returned by the service.
* @sample AmazonFraudDetectorAsyncHandler.UpdateModel
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateModelAsync(UpdateModelRequest updateModelRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates a model version. Updating a model version retrains an existing model version using updated training data
* and produces a new minor version of the model. You can update the training data set location and data access role
* attributes using this action. This action creates and trains a new minor version of the model, for example
* version 1.01, 1.02, 1.03.
*
*
* @param updateModelVersionRequest
* @return A Java Future containing the result of the UpdateModelVersion operation returned by the service.
* @sample AmazonFraudDetectorAsync.UpdateModelVersion
* @see AWS API Documentation
*/
java.util.concurrent.Future updateModelVersionAsync(UpdateModelVersionRequest updateModelVersionRequest);
/**
*
* Updates a model version. Updating a model version retrains an existing model version using updated training data
* and produces a new minor version of the model. You can update the training data set location and data access role
* attributes using this action. This action creates and trains a new minor version of the model, for example
* version 1.01, 1.02, 1.03.
*
*
* @param updateModelVersionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateModelVersion operation returned by the service.
* @sample AmazonFraudDetectorAsyncHandler.UpdateModelVersion
* @see AWS API Documentation
*/
java.util.concurrent.Future updateModelVersionAsync(UpdateModelVersionRequest updateModelVersionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates the status of a model version.
*
*
* You can perform the following status updates:
*
*
* -
*
* Change the TRAINING_IN_PROGRESS
status to TRAINING_CANCELLED
.
*
*
* -
*
* Change the TRAINING_COMPLETE
status to ACTIVE
.
*
*
* -
*
* Change ACTIVE
to INACTIVE
.
*
*
*
*
* @param updateModelVersionStatusRequest
* @return A Java Future containing the result of the UpdateModelVersionStatus operation returned by the service.
* @sample AmazonFraudDetectorAsync.UpdateModelVersionStatus
* @see AWS API Documentation
*/
java.util.concurrent.Future updateModelVersionStatusAsync(UpdateModelVersionStatusRequest updateModelVersionStatusRequest);
/**
*
* Updates the status of a model version.
*
*
* You can perform the following status updates:
*
*
* -
*
* Change the TRAINING_IN_PROGRESS
status to TRAINING_CANCELLED
.
*
*
* -
*
* Change the TRAINING_COMPLETE
status to ACTIVE
.
*
*
* -
*
* Change ACTIVE
to INACTIVE
.
*
*
*
*
* @param updateModelVersionStatusRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateModelVersionStatus operation returned by the service.
* @sample AmazonFraudDetectorAsyncHandler.UpdateModelVersionStatus
* @see AWS API Documentation
*/
java.util.concurrent.Future updateModelVersionStatusAsync(UpdateModelVersionStatusRequest updateModelVersionStatusRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates a rule's metadata. The description attribute can be updated.
*
*
* @param updateRuleMetadataRequest
* @return A Java Future containing the result of the UpdateRuleMetadata operation returned by the service.
* @sample AmazonFraudDetectorAsync.UpdateRuleMetadata
* @see AWS API Documentation
*/
java.util.concurrent.Future updateRuleMetadataAsync(UpdateRuleMetadataRequest updateRuleMetadataRequest);
/**
*
* Updates a rule's metadata. The description attribute can be updated.
*
*
* @param updateRuleMetadataRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateRuleMetadata operation returned by the service.
* @sample AmazonFraudDetectorAsyncHandler.UpdateRuleMetadata
* @see AWS API Documentation
*/
java.util.concurrent.Future updateRuleMetadataAsync(UpdateRuleMetadataRequest updateRuleMetadataRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates a rule version resulting in a new rule version. Updates a rule version resulting in a new rule version
* (version 1, 2, 3 ...).
*
*
* @param updateRuleVersionRequest
* @return A Java Future containing the result of the UpdateRuleVersion operation returned by the service.
* @sample AmazonFraudDetectorAsync.UpdateRuleVersion
* @see AWS API Documentation
*/
java.util.concurrent.Future updateRuleVersionAsync(UpdateRuleVersionRequest updateRuleVersionRequest);
/**
*
* Updates a rule version resulting in a new rule version. Updates a rule version resulting in a new rule version
* (version 1, 2, 3 ...).
*
*
* @param updateRuleVersionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateRuleVersion operation returned by the service.
* @sample AmazonFraudDetectorAsyncHandler.UpdateRuleVersion
* @see AWS API Documentation
*/
java.util.concurrent.Future updateRuleVersionAsync(UpdateRuleVersionRequest updateRuleVersionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates a variable.
*
*
* @param updateVariableRequest
* @return A Java Future containing the result of the UpdateVariable operation returned by the service.
* @sample AmazonFraudDetectorAsync.UpdateVariable
* @see AWS
* API Documentation
*/
java.util.concurrent.Future updateVariableAsync(UpdateVariableRequest updateVariableRequest);
/**
*
* Updates a variable.
*
*
* @param updateVariableRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateVariable operation returned by the service.
* @sample AmazonFraudDetectorAsyncHandler.UpdateVariable
* @see AWS
* API Documentation
*/
java.util.concurrent.Future updateVariableAsync(UpdateVariableRequest updateVariableRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
}