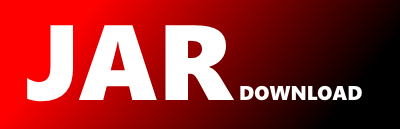
com.amazonaws.services.frauddetector.model.CreateModelVersionRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-frauddetector Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.frauddetector.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS
* API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class CreateModelVersionRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The model ID.
*
*/
private String modelId;
/**
*
* The model type.
*
*/
private String modelType;
/**
*
* The training data source location in Amazon S3.
*
*/
private String trainingDataSource;
/**
*
* The training data schema.
*
*/
private TrainingDataSchema trainingDataSchema;
/**
*
* Details of the external events data used for model version training. Required if trainingDataSource
* is EXTERNAL_EVENTS
.
*
*/
private ExternalEventsDetail externalEventsDetail;
/**
*
* Details of the ingested events data used for model version training. Required if trainingDataSource
* is INGESTED_EVENTS
.
*
*/
private IngestedEventsDetail ingestedEventsDetail;
/**
*
* A collection of key and value pairs.
*
*/
private java.util.List tags;
/**
*
* The model ID.
*
*
* @param modelId
* The model ID.
*/
public void setModelId(String modelId) {
this.modelId = modelId;
}
/**
*
* The model ID.
*
*
* @return The model ID.
*/
public String getModelId() {
return this.modelId;
}
/**
*
* The model ID.
*
*
* @param modelId
* The model ID.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateModelVersionRequest withModelId(String modelId) {
setModelId(modelId);
return this;
}
/**
*
* The model type.
*
*
* @param modelType
* The model type.
* @see ModelTypeEnum
*/
public void setModelType(String modelType) {
this.modelType = modelType;
}
/**
*
* The model type.
*
*
* @return The model type.
* @see ModelTypeEnum
*/
public String getModelType() {
return this.modelType;
}
/**
*
* The model type.
*
*
* @param modelType
* The model type.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ModelTypeEnum
*/
public CreateModelVersionRequest withModelType(String modelType) {
setModelType(modelType);
return this;
}
/**
*
* The model type.
*
*
* @param modelType
* The model type.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ModelTypeEnum
*/
public CreateModelVersionRequest withModelType(ModelTypeEnum modelType) {
this.modelType = modelType.toString();
return this;
}
/**
*
* The training data source location in Amazon S3.
*
*
* @param trainingDataSource
* The training data source location in Amazon S3.
* @see TrainingDataSourceEnum
*/
public void setTrainingDataSource(String trainingDataSource) {
this.trainingDataSource = trainingDataSource;
}
/**
*
* The training data source location in Amazon S3.
*
*
* @return The training data source location in Amazon S3.
* @see TrainingDataSourceEnum
*/
public String getTrainingDataSource() {
return this.trainingDataSource;
}
/**
*
* The training data source location in Amazon S3.
*
*
* @param trainingDataSource
* The training data source location in Amazon S3.
* @return Returns a reference to this object so that method calls can be chained together.
* @see TrainingDataSourceEnum
*/
public CreateModelVersionRequest withTrainingDataSource(String trainingDataSource) {
setTrainingDataSource(trainingDataSource);
return this;
}
/**
*
* The training data source location in Amazon S3.
*
*
* @param trainingDataSource
* The training data source location in Amazon S3.
* @return Returns a reference to this object so that method calls can be chained together.
* @see TrainingDataSourceEnum
*/
public CreateModelVersionRequest withTrainingDataSource(TrainingDataSourceEnum trainingDataSource) {
this.trainingDataSource = trainingDataSource.toString();
return this;
}
/**
*
* The training data schema.
*
*
* @param trainingDataSchema
* The training data schema.
*/
public void setTrainingDataSchema(TrainingDataSchema trainingDataSchema) {
this.trainingDataSchema = trainingDataSchema;
}
/**
*
* The training data schema.
*
*
* @return The training data schema.
*/
public TrainingDataSchema getTrainingDataSchema() {
return this.trainingDataSchema;
}
/**
*
* The training data schema.
*
*
* @param trainingDataSchema
* The training data schema.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateModelVersionRequest withTrainingDataSchema(TrainingDataSchema trainingDataSchema) {
setTrainingDataSchema(trainingDataSchema);
return this;
}
/**
*
* Details of the external events data used for model version training. Required if trainingDataSource
* is EXTERNAL_EVENTS
.
*
*
* @param externalEventsDetail
* Details of the external events data used for model version training. Required if
* trainingDataSource
is EXTERNAL_EVENTS
.
*/
public void setExternalEventsDetail(ExternalEventsDetail externalEventsDetail) {
this.externalEventsDetail = externalEventsDetail;
}
/**
*
* Details of the external events data used for model version training. Required if trainingDataSource
* is EXTERNAL_EVENTS
.
*
*
* @return Details of the external events data used for model version training. Required if
* trainingDataSource
is EXTERNAL_EVENTS
.
*/
public ExternalEventsDetail getExternalEventsDetail() {
return this.externalEventsDetail;
}
/**
*
* Details of the external events data used for model version training. Required if trainingDataSource
* is EXTERNAL_EVENTS
.
*
*
* @param externalEventsDetail
* Details of the external events data used for model version training. Required if
* trainingDataSource
is EXTERNAL_EVENTS
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateModelVersionRequest withExternalEventsDetail(ExternalEventsDetail externalEventsDetail) {
setExternalEventsDetail(externalEventsDetail);
return this;
}
/**
*
* Details of the ingested events data used for model version training. Required if trainingDataSource
* is INGESTED_EVENTS
.
*
*
* @param ingestedEventsDetail
* Details of the ingested events data used for model version training. Required if
* trainingDataSource
is INGESTED_EVENTS
.
*/
public void setIngestedEventsDetail(IngestedEventsDetail ingestedEventsDetail) {
this.ingestedEventsDetail = ingestedEventsDetail;
}
/**
*
* Details of the ingested events data used for model version training. Required if trainingDataSource
* is INGESTED_EVENTS
.
*
*
* @return Details of the ingested events data used for model version training. Required if
* trainingDataSource
is INGESTED_EVENTS
.
*/
public IngestedEventsDetail getIngestedEventsDetail() {
return this.ingestedEventsDetail;
}
/**
*
* Details of the ingested events data used for model version training. Required if trainingDataSource
* is INGESTED_EVENTS
.
*
*
* @param ingestedEventsDetail
* Details of the ingested events data used for model version training. Required if
* trainingDataSource
is INGESTED_EVENTS
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateModelVersionRequest withIngestedEventsDetail(IngestedEventsDetail ingestedEventsDetail) {
setIngestedEventsDetail(ingestedEventsDetail);
return this;
}
/**
*
* A collection of key and value pairs.
*
*
* @return A collection of key and value pairs.
*/
public java.util.List getTags() {
return tags;
}
/**
*
* A collection of key and value pairs.
*
*
* @param tags
* A collection of key and value pairs.
*/
public void setTags(java.util.Collection tags) {
if (tags == null) {
this.tags = null;
return;
}
this.tags = new java.util.ArrayList(tags);
}
/**
*
* A collection of key and value pairs.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTags(java.util.Collection)} or {@link #withTags(java.util.Collection)} if you want to override the
* existing values.
*
*
* @param tags
* A collection of key and value pairs.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateModelVersionRequest withTags(Tag... tags) {
if (this.tags == null) {
setTags(new java.util.ArrayList(tags.length));
}
for (Tag ele : tags) {
this.tags.add(ele);
}
return this;
}
/**
*
* A collection of key and value pairs.
*
*
* @param tags
* A collection of key and value pairs.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateModelVersionRequest withTags(java.util.Collection tags) {
setTags(tags);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getModelId() != null)
sb.append("ModelId: ").append(getModelId()).append(",");
if (getModelType() != null)
sb.append("ModelType: ").append(getModelType()).append(",");
if (getTrainingDataSource() != null)
sb.append("TrainingDataSource: ").append(getTrainingDataSource()).append(",");
if (getTrainingDataSchema() != null)
sb.append("TrainingDataSchema: ").append(getTrainingDataSchema()).append(",");
if (getExternalEventsDetail() != null)
sb.append("ExternalEventsDetail: ").append(getExternalEventsDetail()).append(",");
if (getIngestedEventsDetail() != null)
sb.append("IngestedEventsDetail: ").append(getIngestedEventsDetail()).append(",");
if (getTags() != null)
sb.append("Tags: ").append(getTags());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof CreateModelVersionRequest == false)
return false;
CreateModelVersionRequest other = (CreateModelVersionRequest) obj;
if (other.getModelId() == null ^ this.getModelId() == null)
return false;
if (other.getModelId() != null && other.getModelId().equals(this.getModelId()) == false)
return false;
if (other.getModelType() == null ^ this.getModelType() == null)
return false;
if (other.getModelType() != null && other.getModelType().equals(this.getModelType()) == false)
return false;
if (other.getTrainingDataSource() == null ^ this.getTrainingDataSource() == null)
return false;
if (other.getTrainingDataSource() != null && other.getTrainingDataSource().equals(this.getTrainingDataSource()) == false)
return false;
if (other.getTrainingDataSchema() == null ^ this.getTrainingDataSchema() == null)
return false;
if (other.getTrainingDataSchema() != null && other.getTrainingDataSchema().equals(this.getTrainingDataSchema()) == false)
return false;
if (other.getExternalEventsDetail() == null ^ this.getExternalEventsDetail() == null)
return false;
if (other.getExternalEventsDetail() != null && other.getExternalEventsDetail().equals(this.getExternalEventsDetail()) == false)
return false;
if (other.getIngestedEventsDetail() == null ^ this.getIngestedEventsDetail() == null)
return false;
if (other.getIngestedEventsDetail() != null && other.getIngestedEventsDetail().equals(this.getIngestedEventsDetail()) == false)
return false;
if (other.getTags() == null ^ this.getTags() == null)
return false;
if (other.getTags() != null && other.getTags().equals(this.getTags()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getModelId() == null) ? 0 : getModelId().hashCode());
hashCode = prime * hashCode + ((getModelType() == null) ? 0 : getModelType().hashCode());
hashCode = prime * hashCode + ((getTrainingDataSource() == null) ? 0 : getTrainingDataSource().hashCode());
hashCode = prime * hashCode + ((getTrainingDataSchema() == null) ? 0 : getTrainingDataSchema().hashCode());
hashCode = prime * hashCode + ((getExternalEventsDetail() == null) ? 0 : getExternalEventsDetail().hashCode());
hashCode = prime * hashCode + ((getIngestedEventsDetail() == null) ? 0 : getIngestedEventsDetail().hashCode());
hashCode = prime * hashCode + ((getTags() == null) ? 0 : getTags().hashCode());
return hashCode;
}
@Override
public CreateModelVersionRequest clone() {
return (CreateModelVersionRequest) super.clone();
}
}