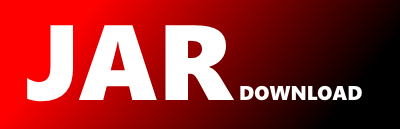
com.amazonaws.services.frauddetector.model.LabelSchema Maven / Gradle / Ivy
Show all versions of aws-java-sdk-frauddetector Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.frauddetector.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* The label schema.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class LabelSchema implements Serializable, Cloneable, StructuredPojo {
/**
*
* The label mapper maps the Amazon Fraud Detector supported model classification labels (FRAUD
,
* LEGIT
) to the appropriate event type labels. For example, if "FRAUD
" and "
* LEGIT
" are Amazon Fraud Detector supported labels, this mapper could be:
* {"FRAUD" => ["0"]
, "LEGIT" => ["1"]}
or {"FRAUD" => ["false"]
,
* "LEGIT" => ["true"]}
or {"FRAUD" => ["fraud", "abuse"]
,
* "LEGIT" => ["legit", "safe"]}
. The value part of the mapper is a list, because you may have
* multiple label variants from your event type for a single Amazon Fraud Detector label.
*
*/
private java.util.Map> labelMapper;
/**
*
* The action to take for unlabeled events.
*
*
* -
*
* Use IGNORE
if you want the unlabeled events to be ignored. This is recommended when the majority of
* the events in the dataset are labeled.
*
*
* -
*
* Use FRAUD
if you want to categorize all unlabeled events as “Fraud”. This is recommended when most
* of the events in your dataset are fraudulent.
*
*
* -
*
* Use LEGIT
if you want to categorize all unlabeled events as “Legit”. This is recommended when most
* of the events in your dataset are legitimate.
*
*
* -
*
* Use AUTO
if you want Amazon Fraud Detector to decide how to use the unlabeled data. This is
* recommended when there is significant unlabeled events in the dataset.
*
*
*
*
* By default, Amazon Fraud Detector ignores the unlabeled data.
*
*/
private String unlabeledEventsTreatment;
/**
*
* The label mapper maps the Amazon Fraud Detector supported model classification labels (FRAUD
,
* LEGIT
) to the appropriate event type labels. For example, if "FRAUD
" and "
* LEGIT
" are Amazon Fraud Detector supported labels, this mapper could be:
* {"FRAUD" => ["0"]
, "LEGIT" => ["1"]}
or {"FRAUD" => ["false"]
,
* "LEGIT" => ["true"]}
or {"FRAUD" => ["fraud", "abuse"]
,
* "LEGIT" => ["legit", "safe"]}
. The value part of the mapper is a list, because you may have
* multiple label variants from your event type for a single Amazon Fraud Detector label.
*
*
* @return The label mapper maps the Amazon Fraud Detector supported model classification labels (FRAUD
* , LEGIT
) to the appropriate event type labels. For example, if "FRAUD
" and "
* LEGIT
" are Amazon Fraud Detector supported labels, this mapper could be:
* {"FRAUD" => ["0"]
, "LEGIT" => ["1"]}
or
* {"FRAUD" => ["false"]
, "LEGIT" => ["true"]}
or
* {"FRAUD" => ["fraud", "abuse"]
, "LEGIT" => ["legit", "safe"]}
. The value
* part of the mapper is a list, because you may have multiple label variants from your event type for a
* single Amazon Fraud Detector label.
*/
public java.util.Map> getLabelMapper() {
return labelMapper;
}
/**
*
* The label mapper maps the Amazon Fraud Detector supported model classification labels (FRAUD
,
* LEGIT
) to the appropriate event type labels. For example, if "FRAUD
" and "
* LEGIT
" are Amazon Fraud Detector supported labels, this mapper could be:
* {"FRAUD" => ["0"]
, "LEGIT" => ["1"]}
or {"FRAUD" => ["false"]
,
* "LEGIT" => ["true"]}
or {"FRAUD" => ["fraud", "abuse"]
,
* "LEGIT" => ["legit", "safe"]}
. The value part of the mapper is a list, because you may have
* multiple label variants from your event type for a single Amazon Fraud Detector label.
*
*
* @param labelMapper
* The label mapper maps the Amazon Fraud Detector supported model classification labels (FRAUD
,
* LEGIT
) to the appropriate event type labels. For example, if "FRAUD
" and "
* LEGIT
" are Amazon Fraud Detector supported labels, this mapper could be:
* {"FRAUD" => ["0"]
, "LEGIT" => ["1"]}
or
* {"FRAUD" => ["false"]
, "LEGIT" => ["true"]}
or
* {"FRAUD" => ["fraud", "abuse"]
, "LEGIT" => ["legit", "safe"]}
. The value
* part of the mapper is a list, because you may have multiple label variants from your event type for a
* single Amazon Fraud Detector label.
*/
public void setLabelMapper(java.util.Map> labelMapper) {
this.labelMapper = labelMapper;
}
/**
*
* The label mapper maps the Amazon Fraud Detector supported model classification labels (FRAUD
,
* LEGIT
) to the appropriate event type labels. For example, if "FRAUD
" and "
* LEGIT
" are Amazon Fraud Detector supported labels, this mapper could be:
* {"FRAUD" => ["0"]
, "LEGIT" => ["1"]}
or {"FRAUD" => ["false"]
,
* "LEGIT" => ["true"]}
or {"FRAUD" => ["fraud", "abuse"]
,
* "LEGIT" => ["legit", "safe"]}
. The value part of the mapper is a list, because you may have
* multiple label variants from your event type for a single Amazon Fraud Detector label.
*
*
* @param labelMapper
* The label mapper maps the Amazon Fraud Detector supported model classification labels (FRAUD
,
* LEGIT
) to the appropriate event type labels. For example, if "FRAUD
" and "
* LEGIT
" are Amazon Fraud Detector supported labels, this mapper could be:
* {"FRAUD" => ["0"]
, "LEGIT" => ["1"]}
or
* {"FRAUD" => ["false"]
, "LEGIT" => ["true"]}
or
* {"FRAUD" => ["fraud", "abuse"]
, "LEGIT" => ["legit", "safe"]}
. The value
* part of the mapper is a list, because you may have multiple label variants from your event type for a
* single Amazon Fraud Detector label.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public LabelSchema withLabelMapper(java.util.Map> labelMapper) {
setLabelMapper(labelMapper);
return this;
}
/**
* Add a single LabelMapper entry
*
* @see LabelSchema#withLabelMapper
* @returns a reference to this object so that method calls can be chained together.
*/
public LabelSchema addLabelMapperEntry(String key, java.util.List value) {
if (null == this.labelMapper) {
this.labelMapper = new java.util.HashMap>();
}
if (this.labelMapper.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.labelMapper.put(key, value);
return this;
}
/**
* Removes all the entries added into LabelMapper.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public LabelSchema clearLabelMapperEntries() {
this.labelMapper = null;
return this;
}
/**
*
* The action to take for unlabeled events.
*
*
* -
*
* Use IGNORE
if you want the unlabeled events to be ignored. This is recommended when the majority of
* the events in the dataset are labeled.
*
*
* -
*
* Use FRAUD
if you want to categorize all unlabeled events as “Fraud”. This is recommended when most
* of the events in your dataset are fraudulent.
*
*
* -
*
* Use LEGIT
if you want to categorize all unlabeled events as “Legit”. This is recommended when most
* of the events in your dataset are legitimate.
*
*
* -
*
* Use AUTO
if you want Amazon Fraud Detector to decide how to use the unlabeled data. This is
* recommended when there is significant unlabeled events in the dataset.
*
*
*
*
* By default, Amazon Fraud Detector ignores the unlabeled data.
*
*
* @param unlabeledEventsTreatment
* The action to take for unlabeled events.
*
* -
*
* Use IGNORE
if you want the unlabeled events to be ignored. This is recommended when the
* majority of the events in the dataset are labeled.
*
*
* -
*
* Use FRAUD
if you want to categorize all unlabeled events as “Fraud”. This is recommended when
* most of the events in your dataset are fraudulent.
*
*
* -
*
* Use LEGIT
if you want to categorize all unlabeled events as “Legit”. This is recommended when
* most of the events in your dataset are legitimate.
*
*
* -
*
* Use AUTO
if you want Amazon Fraud Detector to decide how to use the unlabeled data. This is
* recommended when there is significant unlabeled events in the dataset.
*
*
*
*
* By default, Amazon Fraud Detector ignores the unlabeled data.
* @see UnlabeledEventsTreatment
*/
public void setUnlabeledEventsTreatment(String unlabeledEventsTreatment) {
this.unlabeledEventsTreatment = unlabeledEventsTreatment;
}
/**
*
* The action to take for unlabeled events.
*
*
* -
*
* Use IGNORE
if you want the unlabeled events to be ignored. This is recommended when the majority of
* the events in the dataset are labeled.
*
*
* -
*
* Use FRAUD
if you want to categorize all unlabeled events as “Fraud”. This is recommended when most
* of the events in your dataset are fraudulent.
*
*
* -
*
* Use LEGIT
if you want to categorize all unlabeled events as “Legit”. This is recommended when most
* of the events in your dataset are legitimate.
*
*
* -
*
* Use AUTO
if you want Amazon Fraud Detector to decide how to use the unlabeled data. This is
* recommended when there is significant unlabeled events in the dataset.
*
*
*
*
* By default, Amazon Fraud Detector ignores the unlabeled data.
*
*
* @return The action to take for unlabeled events.
*
* -
*
* Use IGNORE
if you want the unlabeled events to be ignored. This is recommended when the
* majority of the events in the dataset are labeled.
*
*
* -
*
* Use FRAUD
if you want to categorize all unlabeled events as “Fraud”. This is recommended
* when most of the events in your dataset are fraudulent.
*
*
* -
*
* Use LEGIT
if you want to categorize all unlabeled events as “Legit”. This is recommended
* when most of the events in your dataset are legitimate.
*
*
* -
*
* Use AUTO
if you want Amazon Fraud Detector to decide how to use the unlabeled data. This is
* recommended when there is significant unlabeled events in the dataset.
*
*
*
*
* By default, Amazon Fraud Detector ignores the unlabeled data.
* @see UnlabeledEventsTreatment
*/
public String getUnlabeledEventsTreatment() {
return this.unlabeledEventsTreatment;
}
/**
*
* The action to take for unlabeled events.
*
*
* -
*
* Use IGNORE
if you want the unlabeled events to be ignored. This is recommended when the majority of
* the events in the dataset are labeled.
*
*
* -
*
* Use FRAUD
if you want to categorize all unlabeled events as “Fraud”. This is recommended when most
* of the events in your dataset are fraudulent.
*
*
* -
*
* Use LEGIT
if you want to categorize all unlabeled events as “Legit”. This is recommended when most
* of the events in your dataset are legitimate.
*
*
* -
*
* Use AUTO
if you want Amazon Fraud Detector to decide how to use the unlabeled data. This is
* recommended when there is significant unlabeled events in the dataset.
*
*
*
*
* By default, Amazon Fraud Detector ignores the unlabeled data.
*
*
* @param unlabeledEventsTreatment
* The action to take for unlabeled events.
*
* -
*
* Use IGNORE
if you want the unlabeled events to be ignored. This is recommended when the
* majority of the events in the dataset are labeled.
*
*
* -
*
* Use FRAUD
if you want to categorize all unlabeled events as “Fraud”. This is recommended when
* most of the events in your dataset are fraudulent.
*
*
* -
*
* Use LEGIT
if you want to categorize all unlabeled events as “Legit”. This is recommended when
* most of the events in your dataset are legitimate.
*
*
* -
*
* Use AUTO
if you want Amazon Fraud Detector to decide how to use the unlabeled data. This is
* recommended when there is significant unlabeled events in the dataset.
*
*
*
*
* By default, Amazon Fraud Detector ignores the unlabeled data.
* @return Returns a reference to this object so that method calls can be chained together.
* @see UnlabeledEventsTreatment
*/
public LabelSchema withUnlabeledEventsTreatment(String unlabeledEventsTreatment) {
setUnlabeledEventsTreatment(unlabeledEventsTreatment);
return this;
}
/**
*
* The action to take for unlabeled events.
*
*
* -
*
* Use IGNORE
if you want the unlabeled events to be ignored. This is recommended when the majority of
* the events in the dataset are labeled.
*
*
* -
*
* Use FRAUD
if you want to categorize all unlabeled events as “Fraud”. This is recommended when most
* of the events in your dataset are fraudulent.
*
*
* -
*
* Use LEGIT
if you want to categorize all unlabeled events as “Legit”. This is recommended when most
* of the events in your dataset are legitimate.
*
*
* -
*
* Use AUTO
if you want Amazon Fraud Detector to decide how to use the unlabeled data. This is
* recommended when there is significant unlabeled events in the dataset.
*
*
*
*
* By default, Amazon Fraud Detector ignores the unlabeled data.
*
*
* @param unlabeledEventsTreatment
* The action to take for unlabeled events.
*
* -
*
* Use IGNORE
if you want the unlabeled events to be ignored. This is recommended when the
* majority of the events in the dataset are labeled.
*
*
* -
*
* Use FRAUD
if you want to categorize all unlabeled events as “Fraud”. This is recommended when
* most of the events in your dataset are fraudulent.
*
*
* -
*
* Use LEGIT
if you want to categorize all unlabeled events as “Legit”. This is recommended when
* most of the events in your dataset are legitimate.
*
*
* -
*
* Use AUTO
if you want Amazon Fraud Detector to decide how to use the unlabeled data. This is
* recommended when there is significant unlabeled events in the dataset.
*
*
*
*
* By default, Amazon Fraud Detector ignores the unlabeled data.
* @return Returns a reference to this object so that method calls can be chained together.
* @see UnlabeledEventsTreatment
*/
public LabelSchema withUnlabeledEventsTreatment(UnlabeledEventsTreatment unlabeledEventsTreatment) {
this.unlabeledEventsTreatment = unlabeledEventsTreatment.toString();
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getLabelMapper() != null)
sb.append("LabelMapper: ").append(getLabelMapper()).append(",");
if (getUnlabeledEventsTreatment() != null)
sb.append("UnlabeledEventsTreatment: ").append(getUnlabeledEventsTreatment());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof LabelSchema == false)
return false;
LabelSchema other = (LabelSchema) obj;
if (other.getLabelMapper() == null ^ this.getLabelMapper() == null)
return false;
if (other.getLabelMapper() != null && other.getLabelMapper().equals(this.getLabelMapper()) == false)
return false;
if (other.getUnlabeledEventsTreatment() == null ^ this.getUnlabeledEventsTreatment() == null)
return false;
if (other.getUnlabeledEventsTreatment() != null && other.getUnlabeledEventsTreatment().equals(this.getUnlabeledEventsTreatment()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getLabelMapper() == null) ? 0 : getLabelMapper().hashCode());
hashCode = prime * hashCode + ((getUnlabeledEventsTreatment() == null) ? 0 : getUnlabeledEventsTreatment().hashCode());
return hashCode;
}
@Override
public LabelSchema clone() {
try {
return (LabelSchema) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.frauddetector.model.transform.LabelSchemaMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}