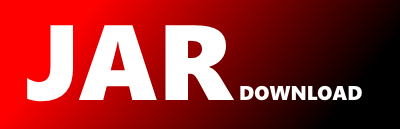
com.amazonaws.services.fsx.AmazonFSxClient Maven / Gradle / Ivy
Show all versions of aws-java-sdk-fsx Show documentation
/*
* Copyright 2015-2020 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.fsx;
import org.w3c.dom.*;
import java.net.*;
import java.util.*;
import javax.annotation.Generated;
import org.apache.commons.logging.*;
import com.amazonaws.*;
import com.amazonaws.annotation.SdkInternalApi;
import com.amazonaws.auth.*;
import com.amazonaws.handlers.*;
import com.amazonaws.http.*;
import com.amazonaws.internal.*;
import com.amazonaws.internal.auth.*;
import com.amazonaws.metrics.*;
import com.amazonaws.regions.*;
import com.amazonaws.transform.*;
import com.amazonaws.util.*;
import com.amazonaws.protocol.json.*;
import com.amazonaws.util.AWSRequestMetrics.Field;
import com.amazonaws.annotation.ThreadSafe;
import com.amazonaws.client.AwsSyncClientParams;
import com.amazonaws.client.builder.AdvancedConfig;
import com.amazonaws.services.fsx.AmazonFSxClientBuilder;
import com.amazonaws.AmazonServiceException;
import com.amazonaws.services.fsx.model.*;
import com.amazonaws.services.fsx.model.transform.*;
/**
* Client for accessing Amazon FSx. All service calls made using this client are blocking, and will not return until the
* service call completes.
*
*
* Amazon FSx is a fully managed service that makes it easy for storage and application administrators to launch and use
* shared file storage.
*
*/
@ThreadSafe
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class AmazonFSxClient extends AmazonWebServiceClient implements AmazonFSx {
/** Provider for AWS credentials. */
private final AWSCredentialsProvider awsCredentialsProvider;
private static final Log log = LogFactory.getLog(AmazonFSx.class);
/** Default signing name for the service. */
private static final String DEFAULT_SIGNING_NAME = "fsx";
/** Client configuration factory providing ClientConfigurations tailored to this client */
protected static final ClientConfigurationFactory configFactory = new ClientConfigurationFactory();
private final AdvancedConfig advancedConfig;
private static final com.amazonaws.protocol.json.SdkJsonProtocolFactory protocolFactory = new com.amazonaws.protocol.json.SdkJsonProtocolFactory(
new JsonClientMetadata()
.withProtocolVersion("1.1")
.withSupportsCbor(false)
.withSupportsIon(false)
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ServiceLimitExceeded").withExceptionUnmarshaller(
com.amazonaws.services.fsx.model.transform.ServiceLimitExceededExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ResourceNotFound").withExceptionUnmarshaller(
com.amazonaws.services.fsx.model.transform.ResourceNotFoundExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("NotServiceResourceError").withExceptionUnmarshaller(
com.amazonaws.services.fsx.model.transform.NotServiceResourceErrorExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("UnsupportedOperation").withExceptionUnmarshaller(
com.amazonaws.services.fsx.model.transform.UnsupportedOperationExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InvalidImportPath").withExceptionUnmarshaller(
com.amazonaws.services.fsx.model.transform.InvalidImportPathExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InvalidNetworkSettings").withExceptionUnmarshaller(
com.amazonaws.services.fsx.model.transform.InvalidNetworkSettingsExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("MissingFileSystemConfiguration").withExceptionUnmarshaller(
com.amazonaws.services.fsx.model.transform.MissingFileSystemConfigurationExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("DataRepositoryTaskExecuting").withExceptionUnmarshaller(
com.amazonaws.services.fsx.model.transform.DataRepositoryTaskExecutingExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("FileSystemNotFound").withExceptionUnmarshaller(
com.amazonaws.services.fsx.model.transform.FileSystemNotFoundExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("BackupRestoring").withExceptionUnmarshaller(
com.amazonaws.services.fsx.model.transform.BackupRestoringExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("IncompatibleParameterError").withExceptionUnmarshaller(
com.amazonaws.services.fsx.model.transform.IncompatibleParameterErrorExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("DataRepositoryTaskEnded").withExceptionUnmarshaller(
com.amazonaws.services.fsx.model.transform.DataRepositoryTaskEndedExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InvalidPerUnitStorageThroughput").withExceptionUnmarshaller(
com.amazonaws.services.fsx.model.transform.InvalidPerUnitStorageThroughputExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("BackupInProgress").withExceptionUnmarshaller(
com.amazonaws.services.fsx.model.transform.BackupInProgressExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InvalidExportPath").withExceptionUnmarshaller(
com.amazonaws.services.fsx.model.transform.InvalidExportPathExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ResourceDoesNotSupportTagging").withExceptionUnmarshaller(
com.amazonaws.services.fsx.model.transform.ResourceDoesNotSupportTaggingExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("DataRepositoryTaskNotFound").withExceptionUnmarshaller(
com.amazonaws.services.fsx.model.transform.DataRepositoryTaskNotFoundExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("BackupNotFound").withExceptionUnmarshaller(
com.amazonaws.services.fsx.model.transform.BackupNotFoundExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ActiveDirectoryError").withExceptionUnmarshaller(
com.amazonaws.services.fsx.model.transform.ActiveDirectoryErrorExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("BadRequest").withExceptionUnmarshaller(
com.amazonaws.services.fsx.model.transform.BadRequestExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InternalServerError").withExceptionUnmarshaller(
com.amazonaws.services.fsx.model.transform.InternalServerErrorExceptionUnmarshaller.getInstance()))
.withBaseServiceExceptionClass(com.amazonaws.services.fsx.model.AmazonFSxException.class));
public static AmazonFSxClientBuilder builder() {
return AmazonFSxClientBuilder.standard();
}
/**
* Constructs a new client to invoke service methods on Amazon FSx using the specified parameters.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param clientParams
* Object providing client parameters.
*/
AmazonFSxClient(AwsSyncClientParams clientParams) {
this(clientParams, false);
}
/**
* Constructs a new client to invoke service methods on Amazon FSx using the specified parameters.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param clientParams
* Object providing client parameters.
*/
AmazonFSxClient(AwsSyncClientParams clientParams, boolean endpointDiscoveryEnabled) {
super(clientParams);
this.awsCredentialsProvider = clientParams.getCredentialsProvider();
this.advancedConfig = clientParams.getAdvancedConfig();
init();
}
private void init() {
setServiceNameIntern(DEFAULT_SIGNING_NAME);
setEndpointPrefix(ENDPOINT_PREFIX);
// calling this.setEndPoint(...) will also modify the signer accordingly
setEndpoint("fsx.us-east-1.amazonaws.com");
HandlerChainFactory chainFactory = new HandlerChainFactory();
requestHandler2s.addAll(chainFactory.newRequestHandlerChain("/com/amazonaws/services/fsx/request.handlers"));
requestHandler2s.addAll(chainFactory.newRequestHandler2Chain("/com/amazonaws/services/fsx/request.handler2s"));
requestHandler2s.addAll(chainFactory.getGlobalHandlers());
}
/**
*
* Cancels an existing Amazon FSx for Lustre data repository task if that task is in either the PENDING
* or EXECUTING
state. When you cancel a task, Amazon FSx does the following.
*
*
* -
*
* Any files that FSx has already exported are not reverted.
*
*
* -
*
* FSx continues to export any files that are "in-flight" when the cancel operation is received.
*
*
* -
*
* FSx does not export any files that have not yet been exported.
*
*
*
*
* @param cancelDataRepositoryTaskRequest
* Cancels a data repository task.
* @return Result of the CancelDataRepositoryTask operation returned by the service.
* @throws BadRequestException
* A generic error indicating a failure with a client request.
* @throws UnsupportedOperationException
* The requested operation is not supported for this resource or API.
* @throws DataRepositoryTaskNotFoundException
* The data repository task or tasks you specified could not be found.
* @throws DataRepositoryTaskEndedException
* The data repository task could not be canceled because the task has already ended.
* @throws InternalServerErrorException
* A generic error indicating a server-side failure.
* @sample AmazonFSx.CancelDataRepositoryTask
* @see AWS
* API Documentation
*/
@Override
public CancelDataRepositoryTaskResult cancelDataRepositoryTask(CancelDataRepositoryTaskRequest request) {
request = beforeClientExecution(request);
return executeCancelDataRepositoryTask(request);
}
@SdkInternalApi
final CancelDataRepositoryTaskResult executeCancelDataRepositoryTask(CancelDataRepositoryTaskRequest cancelDataRepositoryTaskRequest) {
ExecutionContext executionContext = createExecutionContext(cancelDataRepositoryTaskRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CancelDataRepositoryTaskRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(cancelDataRepositoryTaskRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "FSx");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CancelDataRepositoryTask");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new CancelDataRepositoryTaskResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a backup of an existing Amazon FSx for Windows File Server file system. Creating regular backups for your
* file system is a best practice that complements the replication that Amazon FSx for Windows File Server performs
* for your file system. It also enables you to restore from user modification of data.
*
*
* If a backup with the specified client request token exists, and the parameters match, this operation returns the
* description of the existing backup. If a backup specified client request token exists, and the parameters don't
* match, this operation returns IncompatibleParameterError
. If a backup with the specified client
* request token doesn't exist, CreateBackup
does the following:
*
*
* -
*
* Creates a new Amazon FSx backup with an assigned ID, and an initial lifecycle state of CREATING
.
*
*
* -
*
* Returns the description of the backup.
*
*
*
*
* By using the idempotent operation, you can retry a CreateBackup
operation without the risk of
* creating an extra backup. This approach can be useful when an initial call fails in a way that makes it unclear
* whether a backup was created. If you use the same client request token and the initial call created a backup, the
* operation returns a successful result because all the parameters are the same.
*
*
* The CreateFileSystem
operation returns while the backup's lifecycle state is still
* CREATING
. You can check the file system creation status by calling the DescribeBackups
* operation, which returns the backup state along with other information.
*
*
*
*
*
* @param createBackupRequest
* The request object for the CreateBackup
operation.
* @return Result of the CreateBackup operation returned by the service.
* @throws BadRequestException
* A generic error indicating a failure with a client request.
* @throws UnsupportedOperationException
* The requested operation is not supported for this resource or API.
* @throws FileSystemNotFoundException
* No Amazon FSx file systems were found based upon supplied parameters.
* @throws BackupInProgressException
* Another backup is already under way. Wait for completion before initiating additional backups of this
* file system.
* @throws IncompatibleParameterErrorException
* The error returned when a second request is received with the same client request token but different
* parameters settings. A client request token should always uniquely identify a single request.
* @throws ServiceLimitExceededException
* An error indicating that a particular service limit was exceeded. You can increase some service limits by
* contacting AWS Support.
* @throws InternalServerErrorException
* A generic error indicating a server-side failure.
* @sample AmazonFSx.CreateBackup
* @see AWS API
* Documentation
*/
@Override
public CreateBackupResult createBackup(CreateBackupRequest request) {
request = beforeClientExecution(request);
return executeCreateBackup(request);
}
@SdkInternalApi
final CreateBackupResult executeCreateBackup(CreateBackupRequest createBackupRequest) {
ExecutionContext executionContext = createExecutionContext(createBackupRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateBackupRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createBackupRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "FSx");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateBackup");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateBackupResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates an Amazon FSx for Lustre data repository task. You use data repository tasks to perform bulk operations
* between your Amazon FSx file system and its linked data repository. An example of a data repository task is
* exporting any data and metadata changes, including POSIX metadata, to files, directories, and symbolic links
* (symlinks) from your FSx file system to its linked data repository. A CreateDataRepositoryTask
* operation will fail if a data repository is not linked to the FSx file system. To learn more about data
* repository tasks, see Using Data Repository
* Tasks. To learn more about linking a data repository to your file system, see Setting the
* Export Prefix.
*
*
* @param createDataRepositoryTaskRequest
* @return Result of the CreateDataRepositoryTask operation returned by the service.
* @throws BadRequestException
* A generic error indicating a failure with a client request.
* @throws UnsupportedOperationException
* The requested operation is not supported for this resource or API.
* @throws FileSystemNotFoundException
* No Amazon FSx file systems were found based upon supplied parameters.
* @throws IncompatibleParameterErrorException
* The error returned when a second request is received with the same client request token but different
* parameters settings. A client request token should always uniquely identify a single request.
* @throws ServiceLimitExceededException
* An error indicating that a particular service limit was exceeded. You can increase some service limits by
* contacting AWS Support.
* @throws InternalServerErrorException
* A generic error indicating a server-side failure.
* @throws DataRepositoryTaskExecutingException
* An existing data repository task is currently executing on the file system. Wait until the existing task
* has completed, then create the new task.
* @sample AmazonFSx.CreateDataRepositoryTask
* @see AWS
* API Documentation
*/
@Override
public CreateDataRepositoryTaskResult createDataRepositoryTask(CreateDataRepositoryTaskRequest request) {
request = beforeClientExecution(request);
return executeCreateDataRepositoryTask(request);
}
@SdkInternalApi
final CreateDataRepositoryTaskResult executeCreateDataRepositoryTask(CreateDataRepositoryTaskRequest createDataRepositoryTaskRequest) {
ExecutionContext executionContext = createExecutionContext(createDataRepositoryTaskRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateDataRepositoryTaskRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(createDataRepositoryTaskRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "FSx");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateDataRepositoryTask");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new CreateDataRepositoryTaskResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a new, empty Amazon FSx file system.
*
*
* If a file system with the specified client request token exists and the parameters match,
* CreateFileSystem
returns the description of the existing file system. If a file system specified
* client request token exists and the parameters don't match, this call returns
* IncompatibleParameterError
. If a file system with the specified client request token doesn't exist,
* CreateFileSystem
does the following:
*
*
* -
*
* Creates a new, empty Amazon FSx file system with an assigned ID, and an initial lifecycle state of
* CREATING
.
*
*
* -
*
* Returns the description of the file system.
*
*
*
*
* This operation requires a client request token in the request that Amazon FSx uses to ensure idempotent creation.
* This means that calling the operation multiple times with the same client request token has no effect. By using
* the idempotent operation, you can retry a CreateFileSystem
operation without the risk of creating an
* extra file system. This approach can be useful when an initial call fails in a way that makes it unclear whether
* a file system was created. Examples are if a transport level timeout occurred, or your connection was reset. If
* you use the same client request token and the initial call created a file system, the client receives success as
* long as the parameters are the same.
*
*
*
* The CreateFileSystem
call returns while the file system's lifecycle state is still
* CREATING
. You can check the file-system creation status by calling the DescribeFileSystems
* operation, which returns the file system state along with other information.
*
*
*
* @param createFileSystemRequest
* The request object used to create a new Amazon FSx file system.
* @return Result of the CreateFileSystem operation returned by the service.
* @throws BadRequestException
* A generic error indicating a failure with a client request.
* @throws ActiveDirectoryErrorException
* An Active Directory error.
* @throws IncompatibleParameterErrorException
* The error returned when a second request is received with the same client request token but different
* parameters settings. A client request token should always uniquely identify a single request.
* @throws InvalidImportPathException
* The path provided for data repository import isn't valid.
* @throws InvalidExportPathException
* The path provided for data repository export isn't valid.
* @throws InvalidNetworkSettingsException
* One or more network settings specified in the request are invalid. InvalidVpcId
means that
* the ID passed for the virtual private cloud (VPC) is invalid. InvalidSubnetIds
returns the
* list of IDs for subnets that are either invalid or not part of the VPC specified.
* InvalidSecurityGroupIds
returns the list of IDs for security groups that are either invalid
* or not part of the VPC specified.
* @throws InvalidPerUnitStorageThroughputException
* An invalid value for PerUnitStorageThroughput
was provided. Please create your file system
* again, using a valid value.
* @throws ServiceLimitExceededException
* An error indicating that a particular service limit was exceeded. You can increase some service limits by
* contacting AWS Support.
* @throws InternalServerErrorException
* A generic error indicating a server-side failure.
* @throws MissingFileSystemConfigurationException
* A file system configuration is required for this operation.
* @sample AmazonFSx.CreateFileSystem
* @see AWS API
* Documentation
*/
@Override
public CreateFileSystemResult createFileSystem(CreateFileSystemRequest request) {
request = beforeClientExecution(request);
return executeCreateFileSystem(request);
}
@SdkInternalApi
final CreateFileSystemResult executeCreateFileSystem(CreateFileSystemRequest createFileSystemRequest) {
ExecutionContext executionContext = createExecutionContext(createFileSystemRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateFileSystemRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createFileSystemRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "FSx");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateFileSystem");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateFileSystemResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a new Amazon FSx file system from an existing Amazon FSx for Windows File Server backup.
*
*
* If a file system with the specified client request token exists and the parameters match, this operation returns
* the description of the file system. If a client request token specified by the file system exists and the
* parameters don't match, this call returns IncompatibleParameterError
. If a file system with the
* specified client request token doesn't exist, this operation does the following:
*
*
* -
*
* Creates a new Amazon FSx file system from backup with an assigned ID, and an initial lifecycle state of
* CREATING
.
*
*
* -
*
* Returns the description of the file system.
*
*
*
*
* Parameters like Active Directory, default share name, automatic backup, and backup settings default to the
* parameters of the file system that was backed up, unless overridden. You can explicitly supply other settings.
*
*
* By using the idempotent operation, you can retry a CreateFileSystemFromBackup
call without the risk
* of creating an extra file system. This approach can be useful when an initial call fails in a way that makes it
* unclear whether a file system was created. Examples are if a transport level timeout occurred, or your connection
* was reset. If you use the same client request token and the initial call created a file system, the client
* receives success as long as the parameters are the same.
*
*
*
* The CreateFileSystemFromBackup
call returns while the file system's lifecycle state is still
* CREATING
. You can check the file-system creation status by calling the DescribeFileSystems
* operation, which returns the file system state along with other information.
*
*
*
* @param createFileSystemFromBackupRequest
* The request object for the CreateFileSystemFromBackup
operation.
* @return Result of the CreateFileSystemFromBackup operation returned by the service.
* @throws BadRequestException
* A generic error indicating a failure with a client request.
* @throws ActiveDirectoryErrorException
* An Active Directory error.
* @throws IncompatibleParameterErrorException
* The error returned when a second request is received with the same client request token but different
* parameters settings. A client request token should always uniquely identify a single request.
* @throws InvalidNetworkSettingsException
* One or more network settings specified in the request are invalid. InvalidVpcId
means that
* the ID passed for the virtual private cloud (VPC) is invalid. InvalidSubnetIds
returns the
* list of IDs for subnets that are either invalid or not part of the VPC specified.
* InvalidSecurityGroupIds
returns the list of IDs for security groups that are either invalid
* or not part of the VPC specified.
* @throws ServiceLimitExceededException
* An error indicating that a particular service limit was exceeded. You can increase some service limits by
* contacting AWS Support.
* @throws BackupNotFoundException
* No Amazon FSx backups were found based upon the supplied parameters.
* @throws InternalServerErrorException
* A generic error indicating a server-side failure.
* @throws MissingFileSystemConfigurationException
* A file system configuration is required for this operation.
* @sample AmazonFSx.CreateFileSystemFromBackup
* @see AWS
* API Documentation
*/
@Override
public CreateFileSystemFromBackupResult createFileSystemFromBackup(CreateFileSystemFromBackupRequest request) {
request = beforeClientExecution(request);
return executeCreateFileSystemFromBackup(request);
}
@SdkInternalApi
final CreateFileSystemFromBackupResult executeCreateFileSystemFromBackup(CreateFileSystemFromBackupRequest createFileSystemFromBackupRequest) {
ExecutionContext executionContext = createExecutionContext(createFileSystemFromBackupRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateFileSystemFromBackupRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(createFileSystemFromBackupRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "FSx");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateFileSystemFromBackup");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new CreateFileSystemFromBackupResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes an Amazon FSx for Windows File Server backup, deleting its contents. After deletion, the backup no longer
* exists, and its data is gone.
*
*
* The DeleteBackup
call returns instantly. The backup will not show up in later
* DescribeBackups
calls.
*
*
*
* The data in a deleted backup is also deleted and can't be recovered by any means.
*
*
*
* @param deleteBackupRequest
* The request object for DeleteBackup
operation.
* @return Result of the DeleteBackup operation returned by the service.
* @throws BadRequestException
* A generic error indicating a failure with a client request.
* @throws BackupInProgressException
* Another backup is already under way. Wait for completion before initiating additional backups of this
* file system.
* @throws BackupNotFoundException
* No Amazon FSx backups were found based upon the supplied parameters.
* @throws BackupRestoringException
* You can't delete a backup while it's being used to restore a file system.
* @throws IncompatibleParameterErrorException
* The error returned when a second request is received with the same client request token but different
* parameters settings. A client request token should always uniquely identify a single request.
* @throws InternalServerErrorException
* A generic error indicating a server-side failure.
* @sample AmazonFSx.DeleteBackup
* @see AWS API
* Documentation
*/
@Override
public DeleteBackupResult deleteBackup(DeleteBackupRequest request) {
request = beforeClientExecution(request);
return executeDeleteBackup(request);
}
@SdkInternalApi
final DeleteBackupResult executeDeleteBackup(DeleteBackupRequest deleteBackupRequest) {
ExecutionContext executionContext = createExecutionContext(deleteBackupRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteBackupRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteBackupRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "FSx");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteBackup");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteBackupResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes a file system, deleting its contents. After deletion, the file system no longer exists, and its data is
* gone. Any existing automatic backups will also be deleted.
*
*
* By default, when you delete an Amazon FSx for Windows File Server file system, a final backup is created upon
* deletion. This final backup is not subject to the file system's retention policy, and must be manually deleted.
*
*
* The DeleteFileSystem
action returns while the file system has the DELETING
status. You
* can check the file system deletion status by calling the DescribeFileSystems action, which returns a list
* of file systems in your account. If you pass the file system ID for a deleted file system, the
* DescribeFileSystems returns a FileSystemNotFound
error.
*
*
*
* Deleting an Amazon FSx for Lustre file system will fail with a 400 BadRequest if a data repository task is in a
* PENDING
or EXECUTING
state.
*
*
*
* The data in a deleted file system is also deleted and can't be recovered by any means.
*
*
*
* @param deleteFileSystemRequest
* The request object for DeleteFileSystem
operation.
* @return Result of the DeleteFileSystem operation returned by the service.
* @throws BadRequestException
* A generic error indicating a failure with a client request.
* @throws IncompatibleParameterErrorException
* The error returned when a second request is received with the same client request token but different
* parameters settings. A client request token should always uniquely identify a single request.
* @throws FileSystemNotFoundException
* No Amazon FSx file systems were found based upon supplied parameters.
* @throws ServiceLimitExceededException
* An error indicating that a particular service limit was exceeded. You can increase some service limits by
* contacting AWS Support.
* @throws InternalServerErrorException
* A generic error indicating a server-side failure.
* @sample AmazonFSx.DeleteFileSystem
* @see AWS API
* Documentation
*/
@Override
public DeleteFileSystemResult deleteFileSystem(DeleteFileSystemRequest request) {
request = beforeClientExecution(request);
return executeDeleteFileSystem(request);
}
@SdkInternalApi
final DeleteFileSystemResult executeDeleteFileSystem(DeleteFileSystemRequest deleteFileSystemRequest) {
ExecutionContext executionContext = createExecutionContext(deleteFileSystemRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteFileSystemRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteFileSystemRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "FSx");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteFileSystem");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteFileSystemResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns the description of specific Amazon FSx for Windows File Server backups, if a BackupIds
value
* is provided for that backup. Otherwise, it returns all backups owned by your AWS account in the AWS Region of the
* endpoint that you're calling.
*
*
* When retrieving all backups, you can optionally specify the MaxResults
parameter to limit the number
* of backups in a response. If more backups remain, Amazon FSx returns a NextToken
value in the
* response. In this case, send a later request with the NextToken
request parameter set to the value
* of NextToken
from the last response.
*
*
* This action is used in an iterative process to retrieve a list of your backups. DescribeBackups
is
* called first without a NextToken
value. Then the action continues to be called with the
* NextToken
parameter set to the value of the last NextToken
value until a response has
* no NextToken
.
*
*
* When using this action, keep the following in mind:
*
*
* -
*
* The implementation might return fewer than MaxResults
file system descriptions while still including
* a NextToken
value.
*
*
* -
*
* The order of backups returned in the response of one DescribeBackups
call and the order of backups
* returned across the responses of a multi-call iteration is unspecified.
*
*
*
*
* @param describeBackupsRequest
* The request object for DescribeBackups
operation.
* @return Result of the DescribeBackups operation returned by the service.
* @throws BadRequestException
* A generic error indicating a failure with a client request.
* @throws FileSystemNotFoundException
* No Amazon FSx file systems were found based upon supplied parameters.
* @throws BackupNotFoundException
* No Amazon FSx backups were found based upon the supplied parameters.
* @throws InternalServerErrorException
* A generic error indicating a server-side failure.
* @sample AmazonFSx.DescribeBackups
* @see AWS API
* Documentation
*/
@Override
public DescribeBackupsResult describeBackups(DescribeBackupsRequest request) {
request = beforeClientExecution(request);
return executeDescribeBackups(request);
}
@SdkInternalApi
final DescribeBackupsResult executeDescribeBackups(DescribeBackupsRequest describeBackupsRequest) {
ExecutionContext executionContext = createExecutionContext(describeBackupsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeBackupsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(describeBackupsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "FSx");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeBackups");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DescribeBackupsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns the description of specific Amazon FSx for Lustre data repository tasks, if one or more
* TaskIds
values are provided in the request, or if filters are used in the request. You can use
* filters to narrow the response to include just tasks for specific file systems, or tasks in a specific lifecycle
* state. Otherwise, it returns all data repository tasks owned by your AWS account in the AWS Region of the
* endpoint that you're calling.
*
*
* When retrieving all tasks, you can paginate the response by using the optional MaxResults
parameter
* to limit the number of tasks returned in a response. If more tasks remain, Amazon FSx returns a
* NextToken
value in the response. In this case, send a later request with the NextToken
* request parameter set to the value of NextToken
from the last response.
*
*
* @param describeDataRepositoryTasksRequest
* @return Result of the DescribeDataRepositoryTasks operation returned by the service.
* @throws BadRequestException
* A generic error indicating a failure with a client request.
* @throws FileSystemNotFoundException
* No Amazon FSx file systems were found based upon supplied parameters.
* @throws DataRepositoryTaskNotFoundException
* The data repository task or tasks you specified could not be found.
* @throws InternalServerErrorException
* A generic error indicating a server-side failure.
* @sample AmazonFSx.DescribeDataRepositoryTasks
* @see AWS API Documentation
*/
@Override
public DescribeDataRepositoryTasksResult describeDataRepositoryTasks(DescribeDataRepositoryTasksRequest request) {
request = beforeClientExecution(request);
return executeDescribeDataRepositoryTasks(request);
}
@SdkInternalApi
final DescribeDataRepositoryTasksResult executeDescribeDataRepositoryTasks(DescribeDataRepositoryTasksRequest describeDataRepositoryTasksRequest) {
ExecutionContext executionContext = createExecutionContext(describeDataRepositoryTasksRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeDataRepositoryTasksRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(describeDataRepositoryTasksRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "FSx");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeDataRepositoryTasks");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DescribeDataRepositoryTasksResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns the description of specific Amazon FSx file systems, if a FileSystemIds
value is provided
* for that file system. Otherwise, it returns descriptions of all file systems owned by your AWS account in the AWS
* Region of the endpoint that you're calling.
*
*
* When retrieving all file system descriptions, you can optionally specify the MaxResults
parameter to
* limit the number of descriptions in a response. If more file system descriptions remain, Amazon FSx returns a
* NextToken
value in the response. In this case, send a later request with the NextToken
* request parameter set to the value of NextToken
from the last response.
*
*
* This action is used in an iterative process to retrieve a list of your file system descriptions.
* DescribeFileSystems
is called first without a NextToken
value. Then the action continues
* to be called with the NextToken
parameter set to the value of the last NextToken
value
* until a response has no NextToken
.
*
*
* When using this action, keep the following in mind:
*
*
* -
*
* The implementation might return fewer than MaxResults
file system descriptions while still including
* a NextToken
value.
*
*
* -
*
* The order of file systems returned in the response of one DescribeFileSystems
call and the order of
* file systems returned across the responses of a multicall iteration is unspecified.
*
*
*
*
* @param describeFileSystemsRequest
* The request object for DescribeFileSystems
operation.
* @return Result of the DescribeFileSystems operation returned by the service.
* @throws BadRequestException
* A generic error indicating a failure with a client request.
* @throws FileSystemNotFoundException
* No Amazon FSx file systems were found based upon supplied parameters.
* @throws InternalServerErrorException
* A generic error indicating a server-side failure.
* @sample AmazonFSx.DescribeFileSystems
* @see AWS API
* Documentation
*/
@Override
public DescribeFileSystemsResult describeFileSystems(DescribeFileSystemsRequest request) {
request = beforeClientExecution(request);
return executeDescribeFileSystems(request);
}
@SdkInternalApi
final DescribeFileSystemsResult executeDescribeFileSystems(DescribeFileSystemsRequest describeFileSystemsRequest) {
ExecutionContext executionContext = createExecutionContext(describeFileSystemsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeFileSystemsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(describeFileSystemsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "FSx");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeFileSystems");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DescribeFileSystemsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists tags for an Amazon FSx file systems and backups in the case of Amazon FSx for Windows File Server.
*
*
* When retrieving all tags, you can optionally specify the MaxResults
parameter to limit the number of
* tags in a response. If more tags remain, Amazon FSx returns a NextToken
value in the response. In
* this case, send a later request with the NextToken
request parameter set to the value of
* NextToken
from the last response.
*
*
* This action is used in an iterative process to retrieve a list of your tags. ListTagsForResource
is
* called first without a NextToken
value. Then the action continues to be called with the
* NextToken
parameter set to the value of the last NextToken
value until a response has
* no NextToken
.
*
*
* When using this action, keep the following in mind:
*
*
* -
*
* The implementation might return fewer than MaxResults
file system descriptions while still including
* a NextToken
value.
*
*
* -
*
* The order of tags returned in the response of one ListTagsForResource
call and the order of tags
* returned across the responses of a multi-call iteration is unspecified.
*
*
*
*
* @param listTagsForResourceRequest
* The request object for ListTagsForResource
operation.
* @return Result of the ListTagsForResource operation returned by the service.
* @throws BadRequestException
* A generic error indicating a failure with a client request.
* @throws InternalServerErrorException
* A generic error indicating a server-side failure.
* @throws ResourceNotFoundException
* The resource specified by the Amazon Resource Name (ARN) can't be found.
* @throws NotServiceResourceErrorException
* The resource specified for the tagging operation is not a resource type owned by Amazon FSx. Use the API
* of the relevant service to perform the operation.
* @throws ResourceDoesNotSupportTaggingException
* The resource specified does not support tagging.
* @sample AmazonFSx.ListTagsForResource
* @see AWS API
* Documentation
*/
@Override
public ListTagsForResourceResult listTagsForResource(ListTagsForResourceRequest request) {
request = beforeClientExecution(request);
return executeListTagsForResource(request);
}
@SdkInternalApi
final ListTagsForResourceResult executeListTagsForResource(ListTagsForResourceRequest listTagsForResourceRequest) {
ExecutionContext executionContext = createExecutionContext(listTagsForResourceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListTagsForResourceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listTagsForResourceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "FSx");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListTagsForResource");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListTagsForResourceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Tags an Amazon FSx resource.
*
*
* @param tagResourceRequest
* The request object for the TagResource
operation.
* @return Result of the TagResource operation returned by the service.
* @throws BadRequestException
* A generic error indicating a failure with a client request.
* @throws InternalServerErrorException
* A generic error indicating a server-side failure.
* @throws ResourceNotFoundException
* The resource specified by the Amazon Resource Name (ARN) can't be found.
* @throws NotServiceResourceErrorException
* The resource specified for the tagging operation is not a resource type owned by Amazon FSx. Use the API
* of the relevant service to perform the operation.
* @throws ResourceDoesNotSupportTaggingException
* The resource specified does not support tagging.
* @sample AmazonFSx.TagResource
* @see AWS API
* Documentation
*/
@Override
public TagResourceResult tagResource(TagResourceRequest request) {
request = beforeClientExecution(request);
return executeTagResource(request);
}
@SdkInternalApi
final TagResourceResult executeTagResource(TagResourceRequest tagResourceRequest) {
ExecutionContext executionContext = createExecutionContext(tagResourceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new TagResourceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(tagResourceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "FSx");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "TagResource");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new TagResourceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* This action removes a tag from an Amazon FSx resource.
*
*
* @param untagResourceRequest
* The request object for UntagResource
action.
* @return Result of the UntagResource operation returned by the service.
* @throws BadRequestException
* A generic error indicating a failure with a client request.
* @throws InternalServerErrorException
* A generic error indicating a server-side failure.
* @throws ResourceNotFoundException
* The resource specified by the Amazon Resource Name (ARN) can't be found.
* @throws NotServiceResourceErrorException
* The resource specified for the tagging operation is not a resource type owned by Amazon FSx. Use the API
* of the relevant service to perform the operation.
* @throws ResourceDoesNotSupportTaggingException
* The resource specified does not support tagging.
* @sample AmazonFSx.UntagResource
* @see AWS API
* Documentation
*/
@Override
public UntagResourceResult untagResource(UntagResourceRequest request) {
request = beforeClientExecution(request);
return executeUntagResource(request);
}
@SdkInternalApi
final UntagResourceResult executeUntagResource(UntagResourceRequest untagResourceRequest) {
ExecutionContext executionContext = createExecutionContext(untagResourceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UntagResourceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(untagResourceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "FSx");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UntagResource");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new UntagResourceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates a file system configuration.
*
*
* @param updateFileSystemRequest
* The request object for the UpdateFileSystem
operation.
* @return Result of the UpdateFileSystem operation returned by the service.
* @throws BadRequestException
* A generic error indicating a failure with a client request.
* @throws UnsupportedOperationException
* The requested operation is not supported for this resource or API.
* @throws IncompatibleParameterErrorException
* The error returned when a second request is received with the same client request token but different
* parameters settings. A client request token should always uniquely identify a single request.
* @throws InternalServerErrorException
* A generic error indicating a server-side failure.
* @throws FileSystemNotFoundException
* No Amazon FSx file systems were found based upon supplied parameters.
* @throws MissingFileSystemConfigurationException
* A file system configuration is required for this operation.
* @sample AmazonFSx.UpdateFileSystem
* @see AWS API
* Documentation
*/
@Override
public UpdateFileSystemResult updateFileSystem(UpdateFileSystemRequest request) {
request = beforeClientExecution(request);
return executeUpdateFileSystem(request);
}
@SdkInternalApi
final UpdateFileSystemResult executeUpdateFileSystem(UpdateFileSystemRequest updateFileSystemRequest) {
ExecutionContext executionContext = createExecutionContext(updateFileSystemRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateFileSystemRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(updateFileSystemRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "FSx");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateFileSystem");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new UpdateFileSystemResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
* Returns additional metadata for a previously executed successful, request, typically used for debugging issues
* where a service isn't acting as expected. This data isn't considered part of the result data returned by an
* operation, so it's available through this separate, diagnostic interface.
*
* Response metadata is only cached for a limited period of time, so if you need to access this extra diagnostic
* information for an executed request, you should use this method to retrieve it as soon as possible after
* executing the request.
*
* @param request
* The originally executed request
*
* @return The response metadata for the specified request, or null if none is available.
*/
public ResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request) {
return client.getResponseMetadataForRequest(request);
}
/**
* Normal invoke with authentication. Credentials are required and may be overriden at the request level.
**/
private Response invoke(Request request, HttpResponseHandler> responseHandler,
ExecutionContext executionContext) {
return invoke(request, responseHandler, executionContext, null, null);
}
/**
* Normal invoke with authentication. Credentials are required and may be overriden at the request level.
**/
private Response invoke(Request request, HttpResponseHandler> responseHandler,
ExecutionContext executionContext, URI cachedEndpoint, URI uriFromEndpointTrait) {
executionContext.setCredentialsProvider(CredentialUtils.getCredentialsProvider(request.getOriginalRequest(), awsCredentialsProvider));
return doInvoke(request, responseHandler, executionContext, cachedEndpoint, uriFromEndpointTrait);
}
/**
* Invoke with no authentication. Credentials are not required and any credentials set on the client or request will
* be ignored for this operation.
**/
private Response anonymousInvoke(Request request,
HttpResponseHandler> responseHandler, ExecutionContext executionContext) {
return doInvoke(request, responseHandler, executionContext, null, null);
}
/**
* Invoke the request using the http client. Assumes credentials (or lack thereof) have been configured in the
* ExecutionContext beforehand.
**/
private Response doInvoke(Request request, HttpResponseHandler> responseHandler,
ExecutionContext executionContext, URI discoveredEndpoint, URI uriFromEndpointTrait) {
if (discoveredEndpoint != null) {
request.setEndpoint(discoveredEndpoint);
request.getOriginalRequest().getRequestClientOptions().appendUserAgent("endpoint-discovery");
} else if (uriFromEndpointTrait != null) {
request.setEndpoint(uriFromEndpointTrait);
} else {
request.setEndpoint(endpoint);
}
request.setTimeOffset(timeOffset);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler(new JsonErrorResponseMetadata());
return client.execute(request, responseHandler, errorResponseHandler, executionContext);
}
@com.amazonaws.annotation.SdkInternalApi
static com.amazonaws.protocol.json.SdkJsonProtocolFactory getProtocolFactory() {
return protocolFactory;
}
}