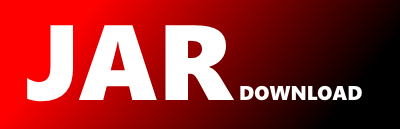
com.amazonaws.services.fsx.model.FileCacheCreating Maven / Gradle / Ivy
Show all versions of aws-java-sdk-fsx Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.fsx.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* The response object for the Amazon File Cache resource being created in the CreateFileCache
operation.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class FileCacheCreating implements Serializable, Cloneable, StructuredPojo {
private String ownerId;
private java.util.Date creationTime;
/**
*
* The system-generated, unique ID of the cache.
*
*/
private String fileCacheId;
/**
*
* The type of cache, which must be LUSTRE
.
*
*/
private String fileCacheType;
/**
*
* The Lustre version of the cache, which must be 2.12
.
*
*/
private String fileCacheTypeVersion;
/**
*
* The lifecycle status of the cache. The following are the possible values and what they mean:
*
*
* -
*
* AVAILABLE
- The cache is in a healthy state, and is reachable and available for use.
*
*
* -
*
* CREATING
- The new cache is being created.
*
*
* -
*
* DELETING
- An existing cache is being deleted.
*
*
* -
*
* UPDATING
- The cache is undergoing a customer-initiated update.
*
*
* -
*
* FAILED
- An existing cache has experienced an unrecoverable failure. When creating a new cache, the
* cache was unable to be created.
*
*
*
*/
private String lifecycle;
/**
*
* A structure providing details of any failures that occurred.
*
*/
private FileCacheFailureDetails failureDetails;
/**
*
* The storage capacity of the cache in gibibytes (GiB).
*
*/
private Integer storageCapacity;
private String vpcId;
private java.util.List subnetIds;
private java.util.List networkInterfaceIds;
/**
*
* The Domain Name System (DNS) name for the cache.
*
*/
private String dNSName;
/**
*
* Specifies the ID of the Key Management Service (KMS) key to use for encrypting data on an Amazon File Cache. If a
* KmsKeyId
isn't specified, the Amazon FSx-managed KMS key for your account is used. For more
* information, see Encrypt in
* the Key Management Service API Reference.
*
*/
private String kmsKeyId;
private String resourceARN;
private java.util.List tags;
/**
*
* A boolean flag indicating whether tags for the cache should be copied to data repository associations.
*
*/
private Boolean copyTagsToDataRepositoryAssociations;
/**
*
* The configuration for the Amazon File Cache resource.
*
*/
private FileCacheLustreConfiguration lustreConfiguration;
/**
*
* A list of IDs of data repository associations that are associated with this cache.
*
*/
private java.util.List dataRepositoryAssociationIds;
/**
* @param ownerId
*/
public void setOwnerId(String ownerId) {
this.ownerId = ownerId;
}
/**
* @return
*/
public String getOwnerId() {
return this.ownerId;
}
/**
* @param ownerId
* @return Returns a reference to this object so that method calls can be chained together.
*/
public FileCacheCreating withOwnerId(String ownerId) {
setOwnerId(ownerId);
return this;
}
/**
* @param creationTime
*/
public void setCreationTime(java.util.Date creationTime) {
this.creationTime = creationTime;
}
/**
* @return
*/
public java.util.Date getCreationTime() {
return this.creationTime;
}
/**
* @param creationTime
* @return Returns a reference to this object so that method calls can be chained together.
*/
public FileCacheCreating withCreationTime(java.util.Date creationTime) {
setCreationTime(creationTime);
return this;
}
/**
*
* The system-generated, unique ID of the cache.
*
*
* @param fileCacheId
* The system-generated, unique ID of the cache.
*/
public void setFileCacheId(String fileCacheId) {
this.fileCacheId = fileCacheId;
}
/**
*
* The system-generated, unique ID of the cache.
*
*
* @return The system-generated, unique ID of the cache.
*/
public String getFileCacheId() {
return this.fileCacheId;
}
/**
*
* The system-generated, unique ID of the cache.
*
*
* @param fileCacheId
* The system-generated, unique ID of the cache.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public FileCacheCreating withFileCacheId(String fileCacheId) {
setFileCacheId(fileCacheId);
return this;
}
/**
*
* The type of cache, which must be LUSTRE
.
*
*
* @param fileCacheType
* The type of cache, which must be LUSTRE
.
* @see FileCacheType
*/
public void setFileCacheType(String fileCacheType) {
this.fileCacheType = fileCacheType;
}
/**
*
* The type of cache, which must be LUSTRE
.
*
*
* @return The type of cache, which must be LUSTRE
.
* @see FileCacheType
*/
public String getFileCacheType() {
return this.fileCacheType;
}
/**
*
* The type of cache, which must be LUSTRE
.
*
*
* @param fileCacheType
* The type of cache, which must be LUSTRE
.
* @return Returns a reference to this object so that method calls can be chained together.
* @see FileCacheType
*/
public FileCacheCreating withFileCacheType(String fileCacheType) {
setFileCacheType(fileCacheType);
return this;
}
/**
*
* The type of cache, which must be LUSTRE
.
*
*
* @param fileCacheType
* The type of cache, which must be LUSTRE
.
* @return Returns a reference to this object so that method calls can be chained together.
* @see FileCacheType
*/
public FileCacheCreating withFileCacheType(FileCacheType fileCacheType) {
this.fileCacheType = fileCacheType.toString();
return this;
}
/**
*
* The Lustre version of the cache, which must be 2.12
.
*
*
* @param fileCacheTypeVersion
* The Lustre version of the cache, which must be 2.12
.
*/
public void setFileCacheTypeVersion(String fileCacheTypeVersion) {
this.fileCacheTypeVersion = fileCacheTypeVersion;
}
/**
*
* The Lustre version of the cache, which must be 2.12
.
*
*
* @return The Lustre version of the cache, which must be 2.12
.
*/
public String getFileCacheTypeVersion() {
return this.fileCacheTypeVersion;
}
/**
*
* The Lustre version of the cache, which must be 2.12
.
*
*
* @param fileCacheTypeVersion
* The Lustre version of the cache, which must be 2.12
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public FileCacheCreating withFileCacheTypeVersion(String fileCacheTypeVersion) {
setFileCacheTypeVersion(fileCacheTypeVersion);
return this;
}
/**
*
* The lifecycle status of the cache. The following are the possible values and what they mean:
*
*
* -
*
* AVAILABLE
- The cache is in a healthy state, and is reachable and available for use.
*
*
* -
*
* CREATING
- The new cache is being created.
*
*
* -
*
* DELETING
- An existing cache is being deleted.
*
*
* -
*
* UPDATING
- The cache is undergoing a customer-initiated update.
*
*
* -
*
* FAILED
- An existing cache has experienced an unrecoverable failure. When creating a new cache, the
* cache was unable to be created.
*
*
*
*
* @param lifecycle
* The lifecycle status of the cache. The following are the possible values and what they mean:
*
* -
*
* AVAILABLE
- The cache is in a healthy state, and is reachable and available for use.
*
*
* -
*
* CREATING
- The new cache is being created.
*
*
* -
*
* DELETING
- An existing cache is being deleted.
*
*
* -
*
* UPDATING
- The cache is undergoing a customer-initiated update.
*
*
* -
*
* FAILED
- An existing cache has experienced an unrecoverable failure. When creating a new
* cache, the cache was unable to be created.
*
*
* @see FileCacheLifecycle
*/
public void setLifecycle(String lifecycle) {
this.lifecycle = lifecycle;
}
/**
*
* The lifecycle status of the cache. The following are the possible values and what they mean:
*
*
* -
*
* AVAILABLE
- The cache is in a healthy state, and is reachable and available for use.
*
*
* -
*
* CREATING
- The new cache is being created.
*
*
* -
*
* DELETING
- An existing cache is being deleted.
*
*
* -
*
* UPDATING
- The cache is undergoing a customer-initiated update.
*
*
* -
*
* FAILED
- An existing cache has experienced an unrecoverable failure. When creating a new cache, the
* cache was unable to be created.
*
*
*
*
* @return The lifecycle status of the cache. The following are the possible values and what they mean:
*
* -
*
* AVAILABLE
- The cache is in a healthy state, and is reachable and available for use.
*
*
* -
*
* CREATING
- The new cache is being created.
*
*
* -
*
* DELETING
- An existing cache is being deleted.
*
*
* -
*
* UPDATING
- The cache is undergoing a customer-initiated update.
*
*
* -
*
* FAILED
- An existing cache has experienced an unrecoverable failure. When creating a new
* cache, the cache was unable to be created.
*
*
* @see FileCacheLifecycle
*/
public String getLifecycle() {
return this.lifecycle;
}
/**
*
* The lifecycle status of the cache. The following are the possible values and what they mean:
*
*
* -
*
* AVAILABLE
- The cache is in a healthy state, and is reachable and available for use.
*
*
* -
*
* CREATING
- The new cache is being created.
*
*
* -
*
* DELETING
- An existing cache is being deleted.
*
*
* -
*
* UPDATING
- The cache is undergoing a customer-initiated update.
*
*
* -
*
* FAILED
- An existing cache has experienced an unrecoverable failure. When creating a new cache, the
* cache was unable to be created.
*
*
*
*
* @param lifecycle
* The lifecycle status of the cache. The following are the possible values and what they mean:
*
* -
*
* AVAILABLE
- The cache is in a healthy state, and is reachable and available for use.
*
*
* -
*
* CREATING
- The new cache is being created.
*
*
* -
*
* DELETING
- An existing cache is being deleted.
*
*
* -
*
* UPDATING
- The cache is undergoing a customer-initiated update.
*
*
* -
*
* FAILED
- An existing cache has experienced an unrecoverable failure. When creating a new
* cache, the cache was unable to be created.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see FileCacheLifecycle
*/
public FileCacheCreating withLifecycle(String lifecycle) {
setLifecycle(lifecycle);
return this;
}
/**
*
* The lifecycle status of the cache. The following are the possible values and what they mean:
*
*
* -
*
* AVAILABLE
- The cache is in a healthy state, and is reachable and available for use.
*
*
* -
*
* CREATING
- The new cache is being created.
*
*
* -
*
* DELETING
- An existing cache is being deleted.
*
*
* -
*
* UPDATING
- The cache is undergoing a customer-initiated update.
*
*
* -
*
* FAILED
- An existing cache has experienced an unrecoverable failure. When creating a new cache, the
* cache was unable to be created.
*
*
*
*
* @param lifecycle
* The lifecycle status of the cache. The following are the possible values and what they mean:
*
* -
*
* AVAILABLE
- The cache is in a healthy state, and is reachable and available for use.
*
*
* -
*
* CREATING
- The new cache is being created.
*
*
* -
*
* DELETING
- An existing cache is being deleted.
*
*
* -
*
* UPDATING
- The cache is undergoing a customer-initiated update.
*
*
* -
*
* FAILED
- An existing cache has experienced an unrecoverable failure. When creating a new
* cache, the cache was unable to be created.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see FileCacheLifecycle
*/
public FileCacheCreating withLifecycle(FileCacheLifecycle lifecycle) {
this.lifecycle = lifecycle.toString();
return this;
}
/**
*
* A structure providing details of any failures that occurred.
*
*
* @param failureDetails
* A structure providing details of any failures that occurred.
*/
public void setFailureDetails(FileCacheFailureDetails failureDetails) {
this.failureDetails = failureDetails;
}
/**
*
* A structure providing details of any failures that occurred.
*
*
* @return A structure providing details of any failures that occurred.
*/
public FileCacheFailureDetails getFailureDetails() {
return this.failureDetails;
}
/**
*
* A structure providing details of any failures that occurred.
*
*
* @param failureDetails
* A structure providing details of any failures that occurred.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public FileCacheCreating withFailureDetails(FileCacheFailureDetails failureDetails) {
setFailureDetails(failureDetails);
return this;
}
/**
*
* The storage capacity of the cache in gibibytes (GiB).
*
*
* @param storageCapacity
* The storage capacity of the cache in gibibytes (GiB).
*/
public void setStorageCapacity(Integer storageCapacity) {
this.storageCapacity = storageCapacity;
}
/**
*
* The storage capacity of the cache in gibibytes (GiB).
*
*
* @return The storage capacity of the cache in gibibytes (GiB).
*/
public Integer getStorageCapacity() {
return this.storageCapacity;
}
/**
*
* The storage capacity of the cache in gibibytes (GiB).
*
*
* @param storageCapacity
* The storage capacity of the cache in gibibytes (GiB).
* @return Returns a reference to this object so that method calls can be chained together.
*/
public FileCacheCreating withStorageCapacity(Integer storageCapacity) {
setStorageCapacity(storageCapacity);
return this;
}
/**
* @param vpcId
*/
public void setVpcId(String vpcId) {
this.vpcId = vpcId;
}
/**
* @return
*/
public String getVpcId() {
return this.vpcId;
}
/**
* @param vpcId
* @return Returns a reference to this object so that method calls can be chained together.
*/
public FileCacheCreating withVpcId(String vpcId) {
setVpcId(vpcId);
return this;
}
/**
* @return
*/
public java.util.List getSubnetIds() {
return subnetIds;
}
/**
* @param subnetIds
*/
public void setSubnetIds(java.util.Collection subnetIds) {
if (subnetIds == null) {
this.subnetIds = null;
return;
}
this.subnetIds = new java.util.ArrayList(subnetIds);
}
/**
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setSubnetIds(java.util.Collection)} or {@link #withSubnetIds(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param subnetIds
* @return Returns a reference to this object so that method calls can be chained together.
*/
public FileCacheCreating withSubnetIds(String... subnetIds) {
if (this.subnetIds == null) {
setSubnetIds(new java.util.ArrayList(subnetIds.length));
}
for (String ele : subnetIds) {
this.subnetIds.add(ele);
}
return this;
}
/**
* @param subnetIds
* @return Returns a reference to this object so that method calls can be chained together.
*/
public FileCacheCreating withSubnetIds(java.util.Collection subnetIds) {
setSubnetIds(subnetIds);
return this;
}
/**
* @return
*/
public java.util.List getNetworkInterfaceIds() {
return networkInterfaceIds;
}
/**
* @param networkInterfaceIds
*/
public void setNetworkInterfaceIds(java.util.Collection networkInterfaceIds) {
if (networkInterfaceIds == null) {
this.networkInterfaceIds = null;
return;
}
this.networkInterfaceIds = new java.util.ArrayList(networkInterfaceIds);
}
/**
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setNetworkInterfaceIds(java.util.Collection)} or {@link #withNetworkInterfaceIds(java.util.Collection)}
* if you want to override the existing values.
*
*
* @param networkInterfaceIds
* @return Returns a reference to this object so that method calls can be chained together.
*/
public FileCacheCreating withNetworkInterfaceIds(String... networkInterfaceIds) {
if (this.networkInterfaceIds == null) {
setNetworkInterfaceIds(new java.util.ArrayList(networkInterfaceIds.length));
}
for (String ele : networkInterfaceIds) {
this.networkInterfaceIds.add(ele);
}
return this;
}
/**
* @param networkInterfaceIds
* @return Returns a reference to this object so that method calls can be chained together.
*/
public FileCacheCreating withNetworkInterfaceIds(java.util.Collection networkInterfaceIds) {
setNetworkInterfaceIds(networkInterfaceIds);
return this;
}
/**
*
* The Domain Name System (DNS) name for the cache.
*
*
* @param dNSName
* The Domain Name System (DNS) name for the cache.
*/
public void setDNSName(String dNSName) {
this.dNSName = dNSName;
}
/**
*
* The Domain Name System (DNS) name for the cache.
*
*
* @return The Domain Name System (DNS) name for the cache.
*/
public String getDNSName() {
return this.dNSName;
}
/**
*
* The Domain Name System (DNS) name for the cache.
*
*
* @param dNSName
* The Domain Name System (DNS) name for the cache.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public FileCacheCreating withDNSName(String dNSName) {
setDNSName(dNSName);
return this;
}
/**
*
* Specifies the ID of the Key Management Service (KMS) key to use for encrypting data on an Amazon File Cache. If a
* KmsKeyId
isn't specified, the Amazon FSx-managed KMS key for your account is used. For more
* information, see Encrypt in
* the Key Management Service API Reference.
*
*
* @param kmsKeyId
* Specifies the ID of the Key Management Service (KMS) key to use for encrypting data on an Amazon File
* Cache. If a KmsKeyId
isn't specified, the Amazon FSx-managed KMS key for your account is
* used. For more information, see Encrypt in the Key
* Management Service API Reference.
*/
public void setKmsKeyId(String kmsKeyId) {
this.kmsKeyId = kmsKeyId;
}
/**
*
* Specifies the ID of the Key Management Service (KMS) key to use for encrypting data on an Amazon File Cache. If a
* KmsKeyId
isn't specified, the Amazon FSx-managed KMS key for your account is used. For more
* information, see Encrypt in
* the Key Management Service API Reference.
*
*
* @return Specifies the ID of the Key Management Service (KMS) key to use for encrypting data on an Amazon File
* Cache. If a KmsKeyId
isn't specified, the Amazon FSx-managed KMS key for your account is
* used. For more information, see Encrypt in the Key
* Management Service API Reference.
*/
public String getKmsKeyId() {
return this.kmsKeyId;
}
/**
*
* Specifies the ID of the Key Management Service (KMS) key to use for encrypting data on an Amazon File Cache. If a
* KmsKeyId
isn't specified, the Amazon FSx-managed KMS key for your account is used. For more
* information, see Encrypt in
* the Key Management Service API Reference.
*
*
* @param kmsKeyId
* Specifies the ID of the Key Management Service (KMS) key to use for encrypting data on an Amazon File
* Cache. If a KmsKeyId
isn't specified, the Amazon FSx-managed KMS key for your account is
* used. For more information, see Encrypt in the Key
* Management Service API Reference.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public FileCacheCreating withKmsKeyId(String kmsKeyId) {
setKmsKeyId(kmsKeyId);
return this;
}
/**
* @param resourceARN
*/
public void setResourceARN(String resourceARN) {
this.resourceARN = resourceARN;
}
/**
* @return
*/
public String getResourceARN() {
return this.resourceARN;
}
/**
* @param resourceARN
* @return Returns a reference to this object so that method calls can be chained together.
*/
public FileCacheCreating withResourceARN(String resourceARN) {
setResourceARN(resourceARN);
return this;
}
/**
* @return
*/
public java.util.List getTags() {
return tags;
}
/**
* @param tags
*/
public void setTags(java.util.Collection tags) {
if (tags == null) {
this.tags = null;
return;
}
this.tags = new java.util.ArrayList(tags);
}
/**
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTags(java.util.Collection)} or {@link #withTags(java.util.Collection)} if you want to override the
* existing values.
*
*
* @param tags
* @return Returns a reference to this object so that method calls can be chained together.
*/
public FileCacheCreating withTags(Tag... tags) {
if (this.tags == null) {
setTags(new java.util.ArrayList(tags.length));
}
for (Tag ele : tags) {
this.tags.add(ele);
}
return this;
}
/**
* @param tags
* @return Returns a reference to this object so that method calls can be chained together.
*/
public FileCacheCreating withTags(java.util.Collection tags) {
setTags(tags);
return this;
}
/**
*
* A boolean flag indicating whether tags for the cache should be copied to data repository associations.
*
*
* @param copyTagsToDataRepositoryAssociations
* A boolean flag indicating whether tags for the cache should be copied to data repository associations.
*/
public void setCopyTagsToDataRepositoryAssociations(Boolean copyTagsToDataRepositoryAssociations) {
this.copyTagsToDataRepositoryAssociations = copyTagsToDataRepositoryAssociations;
}
/**
*
* A boolean flag indicating whether tags for the cache should be copied to data repository associations.
*
*
* @return A boolean flag indicating whether tags for the cache should be copied to data repository associations.
*/
public Boolean getCopyTagsToDataRepositoryAssociations() {
return this.copyTagsToDataRepositoryAssociations;
}
/**
*
* A boolean flag indicating whether tags for the cache should be copied to data repository associations.
*
*
* @param copyTagsToDataRepositoryAssociations
* A boolean flag indicating whether tags for the cache should be copied to data repository associations.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public FileCacheCreating withCopyTagsToDataRepositoryAssociations(Boolean copyTagsToDataRepositoryAssociations) {
setCopyTagsToDataRepositoryAssociations(copyTagsToDataRepositoryAssociations);
return this;
}
/**
*
* A boolean flag indicating whether tags for the cache should be copied to data repository associations.
*
*
* @return A boolean flag indicating whether tags for the cache should be copied to data repository associations.
*/
public Boolean isCopyTagsToDataRepositoryAssociations() {
return this.copyTagsToDataRepositoryAssociations;
}
/**
*
* The configuration for the Amazon File Cache resource.
*
*
* @param lustreConfiguration
* The configuration for the Amazon File Cache resource.
*/
public void setLustreConfiguration(FileCacheLustreConfiguration lustreConfiguration) {
this.lustreConfiguration = lustreConfiguration;
}
/**
*
* The configuration for the Amazon File Cache resource.
*
*
* @return The configuration for the Amazon File Cache resource.
*/
public FileCacheLustreConfiguration getLustreConfiguration() {
return this.lustreConfiguration;
}
/**
*
* The configuration for the Amazon File Cache resource.
*
*
* @param lustreConfiguration
* The configuration for the Amazon File Cache resource.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public FileCacheCreating withLustreConfiguration(FileCacheLustreConfiguration lustreConfiguration) {
setLustreConfiguration(lustreConfiguration);
return this;
}
/**
*
* A list of IDs of data repository associations that are associated with this cache.
*
*
* @return A list of IDs of data repository associations that are associated with this cache.
*/
public java.util.List getDataRepositoryAssociationIds() {
return dataRepositoryAssociationIds;
}
/**
*
* A list of IDs of data repository associations that are associated with this cache.
*
*
* @param dataRepositoryAssociationIds
* A list of IDs of data repository associations that are associated with this cache.
*/
public void setDataRepositoryAssociationIds(java.util.Collection dataRepositoryAssociationIds) {
if (dataRepositoryAssociationIds == null) {
this.dataRepositoryAssociationIds = null;
return;
}
this.dataRepositoryAssociationIds = new java.util.ArrayList(dataRepositoryAssociationIds);
}
/**
*
* A list of IDs of data repository associations that are associated with this cache.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setDataRepositoryAssociationIds(java.util.Collection)} or
* {@link #withDataRepositoryAssociationIds(java.util.Collection)} if you want to override the existing values.
*
*
* @param dataRepositoryAssociationIds
* A list of IDs of data repository associations that are associated with this cache.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public FileCacheCreating withDataRepositoryAssociationIds(String... dataRepositoryAssociationIds) {
if (this.dataRepositoryAssociationIds == null) {
setDataRepositoryAssociationIds(new java.util.ArrayList(dataRepositoryAssociationIds.length));
}
for (String ele : dataRepositoryAssociationIds) {
this.dataRepositoryAssociationIds.add(ele);
}
return this;
}
/**
*
* A list of IDs of data repository associations that are associated with this cache.
*
*
* @param dataRepositoryAssociationIds
* A list of IDs of data repository associations that are associated with this cache.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public FileCacheCreating withDataRepositoryAssociationIds(java.util.Collection dataRepositoryAssociationIds) {
setDataRepositoryAssociationIds(dataRepositoryAssociationIds);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getOwnerId() != null)
sb.append("OwnerId: ").append(getOwnerId()).append(",");
if (getCreationTime() != null)
sb.append("CreationTime: ").append(getCreationTime()).append(",");
if (getFileCacheId() != null)
sb.append("FileCacheId: ").append(getFileCacheId()).append(",");
if (getFileCacheType() != null)
sb.append("FileCacheType: ").append(getFileCacheType()).append(",");
if (getFileCacheTypeVersion() != null)
sb.append("FileCacheTypeVersion: ").append(getFileCacheTypeVersion()).append(",");
if (getLifecycle() != null)
sb.append("Lifecycle: ").append(getLifecycle()).append(",");
if (getFailureDetails() != null)
sb.append("FailureDetails: ").append(getFailureDetails()).append(",");
if (getStorageCapacity() != null)
sb.append("StorageCapacity: ").append(getStorageCapacity()).append(",");
if (getVpcId() != null)
sb.append("VpcId: ").append(getVpcId()).append(",");
if (getSubnetIds() != null)
sb.append("SubnetIds: ").append(getSubnetIds()).append(",");
if (getNetworkInterfaceIds() != null)
sb.append("NetworkInterfaceIds: ").append(getNetworkInterfaceIds()).append(",");
if (getDNSName() != null)
sb.append("DNSName: ").append(getDNSName()).append(",");
if (getKmsKeyId() != null)
sb.append("KmsKeyId: ").append(getKmsKeyId()).append(",");
if (getResourceARN() != null)
sb.append("ResourceARN: ").append(getResourceARN()).append(",");
if (getTags() != null)
sb.append("Tags: ").append(getTags()).append(",");
if (getCopyTagsToDataRepositoryAssociations() != null)
sb.append("CopyTagsToDataRepositoryAssociations: ").append(getCopyTagsToDataRepositoryAssociations()).append(",");
if (getLustreConfiguration() != null)
sb.append("LustreConfiguration: ").append(getLustreConfiguration()).append(",");
if (getDataRepositoryAssociationIds() != null)
sb.append("DataRepositoryAssociationIds: ").append(getDataRepositoryAssociationIds());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof FileCacheCreating == false)
return false;
FileCacheCreating other = (FileCacheCreating) obj;
if (other.getOwnerId() == null ^ this.getOwnerId() == null)
return false;
if (other.getOwnerId() != null && other.getOwnerId().equals(this.getOwnerId()) == false)
return false;
if (other.getCreationTime() == null ^ this.getCreationTime() == null)
return false;
if (other.getCreationTime() != null && other.getCreationTime().equals(this.getCreationTime()) == false)
return false;
if (other.getFileCacheId() == null ^ this.getFileCacheId() == null)
return false;
if (other.getFileCacheId() != null && other.getFileCacheId().equals(this.getFileCacheId()) == false)
return false;
if (other.getFileCacheType() == null ^ this.getFileCacheType() == null)
return false;
if (other.getFileCacheType() != null && other.getFileCacheType().equals(this.getFileCacheType()) == false)
return false;
if (other.getFileCacheTypeVersion() == null ^ this.getFileCacheTypeVersion() == null)
return false;
if (other.getFileCacheTypeVersion() != null && other.getFileCacheTypeVersion().equals(this.getFileCacheTypeVersion()) == false)
return false;
if (other.getLifecycle() == null ^ this.getLifecycle() == null)
return false;
if (other.getLifecycle() != null && other.getLifecycle().equals(this.getLifecycle()) == false)
return false;
if (other.getFailureDetails() == null ^ this.getFailureDetails() == null)
return false;
if (other.getFailureDetails() != null && other.getFailureDetails().equals(this.getFailureDetails()) == false)
return false;
if (other.getStorageCapacity() == null ^ this.getStorageCapacity() == null)
return false;
if (other.getStorageCapacity() != null && other.getStorageCapacity().equals(this.getStorageCapacity()) == false)
return false;
if (other.getVpcId() == null ^ this.getVpcId() == null)
return false;
if (other.getVpcId() != null && other.getVpcId().equals(this.getVpcId()) == false)
return false;
if (other.getSubnetIds() == null ^ this.getSubnetIds() == null)
return false;
if (other.getSubnetIds() != null && other.getSubnetIds().equals(this.getSubnetIds()) == false)
return false;
if (other.getNetworkInterfaceIds() == null ^ this.getNetworkInterfaceIds() == null)
return false;
if (other.getNetworkInterfaceIds() != null && other.getNetworkInterfaceIds().equals(this.getNetworkInterfaceIds()) == false)
return false;
if (other.getDNSName() == null ^ this.getDNSName() == null)
return false;
if (other.getDNSName() != null && other.getDNSName().equals(this.getDNSName()) == false)
return false;
if (other.getKmsKeyId() == null ^ this.getKmsKeyId() == null)
return false;
if (other.getKmsKeyId() != null && other.getKmsKeyId().equals(this.getKmsKeyId()) == false)
return false;
if (other.getResourceARN() == null ^ this.getResourceARN() == null)
return false;
if (other.getResourceARN() != null && other.getResourceARN().equals(this.getResourceARN()) == false)
return false;
if (other.getTags() == null ^ this.getTags() == null)
return false;
if (other.getTags() != null && other.getTags().equals(this.getTags()) == false)
return false;
if (other.getCopyTagsToDataRepositoryAssociations() == null ^ this.getCopyTagsToDataRepositoryAssociations() == null)
return false;
if (other.getCopyTagsToDataRepositoryAssociations() != null
&& other.getCopyTagsToDataRepositoryAssociations().equals(this.getCopyTagsToDataRepositoryAssociations()) == false)
return false;
if (other.getLustreConfiguration() == null ^ this.getLustreConfiguration() == null)
return false;
if (other.getLustreConfiguration() != null && other.getLustreConfiguration().equals(this.getLustreConfiguration()) == false)
return false;
if (other.getDataRepositoryAssociationIds() == null ^ this.getDataRepositoryAssociationIds() == null)
return false;
if (other.getDataRepositoryAssociationIds() != null && other.getDataRepositoryAssociationIds().equals(this.getDataRepositoryAssociationIds()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getOwnerId() == null) ? 0 : getOwnerId().hashCode());
hashCode = prime * hashCode + ((getCreationTime() == null) ? 0 : getCreationTime().hashCode());
hashCode = prime * hashCode + ((getFileCacheId() == null) ? 0 : getFileCacheId().hashCode());
hashCode = prime * hashCode + ((getFileCacheType() == null) ? 0 : getFileCacheType().hashCode());
hashCode = prime * hashCode + ((getFileCacheTypeVersion() == null) ? 0 : getFileCacheTypeVersion().hashCode());
hashCode = prime * hashCode + ((getLifecycle() == null) ? 0 : getLifecycle().hashCode());
hashCode = prime * hashCode + ((getFailureDetails() == null) ? 0 : getFailureDetails().hashCode());
hashCode = prime * hashCode + ((getStorageCapacity() == null) ? 0 : getStorageCapacity().hashCode());
hashCode = prime * hashCode + ((getVpcId() == null) ? 0 : getVpcId().hashCode());
hashCode = prime * hashCode + ((getSubnetIds() == null) ? 0 : getSubnetIds().hashCode());
hashCode = prime * hashCode + ((getNetworkInterfaceIds() == null) ? 0 : getNetworkInterfaceIds().hashCode());
hashCode = prime * hashCode + ((getDNSName() == null) ? 0 : getDNSName().hashCode());
hashCode = prime * hashCode + ((getKmsKeyId() == null) ? 0 : getKmsKeyId().hashCode());
hashCode = prime * hashCode + ((getResourceARN() == null) ? 0 : getResourceARN().hashCode());
hashCode = prime * hashCode + ((getTags() == null) ? 0 : getTags().hashCode());
hashCode = prime * hashCode + ((getCopyTagsToDataRepositoryAssociations() == null) ? 0 : getCopyTagsToDataRepositoryAssociations().hashCode());
hashCode = prime * hashCode + ((getLustreConfiguration() == null) ? 0 : getLustreConfiguration().hashCode());
hashCode = prime * hashCode + ((getDataRepositoryAssociationIds() == null) ? 0 : getDataRepositoryAssociationIds().hashCode());
return hashCode;
}
@Override
public FileCacheCreating clone() {
try {
return (FileCacheCreating) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.fsx.model.transform.FileCacheCreatingMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}