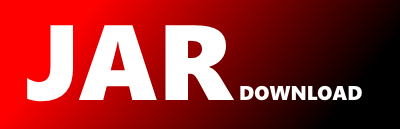
com.amazonaws.services.fsx.AmazonFSxAsync Maven / Gradle / Ivy
Show all versions of aws-java-sdk-fsx Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.fsx;
import javax.annotation.Generated;
import com.amazonaws.services.fsx.model.*;
/**
* Interface for accessing Amazon FSx asynchronously. Each asynchronous method will return a Java Future object
* representing the asynchronous operation; overloads which accept an {@code AsyncHandler} can be used to receive
* notification when an asynchronous operation completes.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.fsx.AbstractAmazonFSxAsync} instead.
*
*
*
* Amazon FSx is a fully managed service that makes it easy for storage and application administrators to launch and use
* shared file storage.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AmazonFSxAsync extends AmazonFSx {
/**
*
* Use this action to associate one or more Domain Name Server (DNS) aliases with an existing Amazon FSx for Windows
* File Server file system. A file system can have a maximum of 50 DNS aliases associated with it at any one time.
* If you try to associate a DNS alias that is already associated with the file system, FSx takes no action on that
* alias in the request. For more information, see Working with DNS Aliases
* and
* Walkthrough 5: Using DNS aliases to access your file system, including additional steps you must take to be
* able to access your file system using a DNS alias.
*
*
* The system response shows the DNS aliases that Amazon FSx is attempting to associate with the file system. Use
* the API operation to monitor the status of the aliases Amazon FSx is associating with the file system.
*
*
* @param associateFileSystemAliasesRequest
* The request object specifying one or more DNS alias names to associate with an Amazon FSx for Windows File
* Server file system.
* @return A Java Future containing the result of the AssociateFileSystemAliases operation returned by the service.
* @sample AmazonFSxAsync.AssociateFileSystemAliases
* @see AWS
* API Documentation
*/
java.util.concurrent.Future associateFileSystemAliasesAsync(
AssociateFileSystemAliasesRequest associateFileSystemAliasesRequest);
/**
*
* Use this action to associate one or more Domain Name Server (DNS) aliases with an existing Amazon FSx for Windows
* File Server file system. A file system can have a maximum of 50 DNS aliases associated with it at any one time.
* If you try to associate a DNS alias that is already associated with the file system, FSx takes no action on that
* alias in the request. For more information, see Working with DNS Aliases
* and
* Walkthrough 5: Using DNS aliases to access your file system, including additional steps you must take to be
* able to access your file system using a DNS alias.
*
*
* The system response shows the DNS aliases that Amazon FSx is attempting to associate with the file system. Use
* the API operation to monitor the status of the aliases Amazon FSx is associating with the file system.
*
*
* @param associateFileSystemAliasesRequest
* The request object specifying one or more DNS alias names to associate with an Amazon FSx for Windows File
* Server file system.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AssociateFileSystemAliases operation returned by the service.
* @sample AmazonFSxAsyncHandler.AssociateFileSystemAliases
* @see AWS
* API Documentation
*/
java.util.concurrent.Future associateFileSystemAliasesAsync(
AssociateFileSystemAliasesRequest associateFileSystemAliasesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Cancels an existing Amazon FSx for Lustre data repository task if that task is in either the PENDING
* or EXECUTING
state. When you cancel am export task, Amazon FSx does the following.
*
*
* -
*
* Any files that FSx has already exported are not reverted.
*
*
* -
*
* FSx continues to export any files that are in-flight when the cancel operation is received.
*
*
* -
*
* FSx does not export any files that have not yet been exported.
*
*
*
*
* For a release task, Amazon FSx will stop releasing files upon cancellation. Any files that have already been
* released will remain in the released state.
*
*
* @param cancelDataRepositoryTaskRequest
* Cancels a data repository task.
* @return A Java Future containing the result of the CancelDataRepositoryTask operation returned by the service.
* @sample AmazonFSxAsync.CancelDataRepositoryTask
* @see AWS
* API Documentation
*/
java.util.concurrent.Future cancelDataRepositoryTaskAsync(CancelDataRepositoryTaskRequest cancelDataRepositoryTaskRequest);
/**
*
* Cancels an existing Amazon FSx for Lustre data repository task if that task is in either the PENDING
* or EXECUTING
state. When you cancel am export task, Amazon FSx does the following.
*
*
* -
*
* Any files that FSx has already exported are not reverted.
*
*
* -
*
* FSx continues to export any files that are in-flight when the cancel operation is received.
*
*
* -
*
* FSx does not export any files that have not yet been exported.
*
*
*
*
* For a release task, Amazon FSx will stop releasing files upon cancellation. Any files that have already been
* released will remain in the released state.
*
*
* @param cancelDataRepositoryTaskRequest
* Cancels a data repository task.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CancelDataRepositoryTask operation returned by the service.
* @sample AmazonFSxAsyncHandler.CancelDataRepositoryTask
* @see AWS
* API Documentation
*/
java.util.concurrent.Future cancelDataRepositoryTaskAsync(CancelDataRepositoryTaskRequest cancelDataRepositoryTaskRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Copies an existing backup within the same Amazon Web Services account to another Amazon Web Services Region
* (cross-Region copy) or within the same Amazon Web Services Region (in-Region copy). You can have up to five
* backup copy requests in progress to a single destination Region per account.
*
*
* You can use cross-Region backup copies for cross-Region disaster recovery. You can periodically take backups and
* copy them to another Region so that in the event of a disaster in the primary Region, you can restore from backup
* and recover availability quickly in the other Region. You can make cross-Region copies only within your Amazon
* Web Services partition. A partition is a grouping of Regions. Amazon Web Services currently has three partitions:
* aws
(Standard Regions), aws-cn
(China Regions), and aws-us-gov
(Amazon Web
* Services GovCloud [US] Regions).
*
*
* You can also use backup copies to clone your file dataset to another Region or within the same Region.
*
*
* You can use the SourceRegion
parameter to specify the Amazon Web Services Region from which the
* backup will be copied. For example, if you make the call from the us-west-1
Region and want to copy
* a backup from the us-east-2
Region, you specify us-east-2
in the
* SourceRegion
parameter to make a cross-Region copy. If you don't specify a Region, the backup copy
* is created in the same Region where the request is sent from (in-Region copy).
*
*
* For more information about creating backup copies, see Copying backups
* in the Amazon FSx for Windows User Guide, Copying backups
* in the Amazon FSx for Lustre User Guide, and Copying backups in
* the Amazon FSx for OpenZFS User Guide.
*
*
* @param copyBackupRequest
* @return A Java Future containing the result of the CopyBackup operation returned by the service.
* @sample AmazonFSxAsync.CopyBackup
* @see AWS API
* Documentation
*/
java.util.concurrent.Future copyBackupAsync(CopyBackupRequest copyBackupRequest);
/**
*
* Copies an existing backup within the same Amazon Web Services account to another Amazon Web Services Region
* (cross-Region copy) or within the same Amazon Web Services Region (in-Region copy). You can have up to five
* backup copy requests in progress to a single destination Region per account.
*
*
* You can use cross-Region backup copies for cross-Region disaster recovery. You can periodically take backups and
* copy them to another Region so that in the event of a disaster in the primary Region, you can restore from backup
* and recover availability quickly in the other Region. You can make cross-Region copies only within your Amazon
* Web Services partition. A partition is a grouping of Regions. Amazon Web Services currently has three partitions:
* aws
(Standard Regions), aws-cn
(China Regions), and aws-us-gov
(Amazon Web
* Services GovCloud [US] Regions).
*
*
* You can also use backup copies to clone your file dataset to another Region or within the same Region.
*
*
* You can use the SourceRegion
parameter to specify the Amazon Web Services Region from which the
* backup will be copied. For example, if you make the call from the us-west-1
Region and want to copy
* a backup from the us-east-2
Region, you specify us-east-2
in the
* SourceRegion
parameter to make a cross-Region copy. If you don't specify a Region, the backup copy
* is created in the same Region where the request is sent from (in-Region copy).
*
*
* For more information about creating backup copies, see Copying backups
* in the Amazon FSx for Windows User Guide, Copying backups
* in the Amazon FSx for Lustre User Guide, and Copying backups in
* the Amazon FSx for OpenZFS User Guide.
*
*
* @param copyBackupRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CopyBackup operation returned by the service.
* @sample AmazonFSxAsyncHandler.CopyBackup
* @see AWS API
* Documentation
*/
java.util.concurrent.Future copyBackupAsync(CopyBackupRequest copyBackupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates an existing volume by using a snapshot from another Amazon FSx for OpenZFS file system. For more
* information, see on-demand data
* replication in the Amazon FSx for OpenZFS User Guide.
*
*
* @param copySnapshotAndUpdateVolumeRequest
* @return A Java Future containing the result of the CopySnapshotAndUpdateVolume operation returned by the service.
* @sample AmazonFSxAsync.CopySnapshotAndUpdateVolume
* @see AWS API Documentation
*/
java.util.concurrent.Future copySnapshotAndUpdateVolumeAsync(
CopySnapshotAndUpdateVolumeRequest copySnapshotAndUpdateVolumeRequest);
/**
*
* Updates an existing volume by using a snapshot from another Amazon FSx for OpenZFS file system. For more
* information, see on-demand data
* replication in the Amazon FSx for OpenZFS User Guide.
*
*
* @param copySnapshotAndUpdateVolumeRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CopySnapshotAndUpdateVolume operation returned by the service.
* @sample AmazonFSxAsyncHandler.CopySnapshotAndUpdateVolume
* @see AWS API Documentation
*/
java.util.concurrent.Future copySnapshotAndUpdateVolumeAsync(
CopySnapshotAndUpdateVolumeRequest copySnapshotAndUpdateVolumeRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a backup of an existing Amazon FSx for Windows File Server file system, Amazon FSx for Lustre file
* system, Amazon FSx for NetApp ONTAP volume, or Amazon FSx for OpenZFS file system. We recommend creating regular
* backups so that you can restore a file system or volume from a backup if an issue arises with the original file
* system or volume.
*
*
* For Amazon FSx for Lustre file systems, you can create a backup only for file systems that have the following
* configuration:
*
*
* -
*
* A Persistent deployment type
*
*
* -
*
* Are not linked to a data repository
*
*
*
*
* For more information about backups, see the following:
*
*
* -
*
* For Amazon FSx for Lustre, see Working with FSx for Lustre
* backups.
*
*
* -
*
* For Amazon FSx for Windows, see Working with FSx for Windows
* backups.
*
*
* -
*
* For Amazon FSx for NetApp ONTAP, see Working with FSx for NetApp ONTAP
* backups.
*
*
* -
*
* For Amazon FSx for OpenZFS, see Working with FSx for OpenZFS
* backups.
*
*
*
*
* If a backup with the specified client request token exists and the parameters match, this operation returns the
* description of the existing backup. If a backup with the specified client request token exists and the parameters
* don't match, this operation returns IncompatibleParameterError
. If a backup with the specified
* client request token doesn't exist, CreateBackup
does the following:
*
*
* -
*
* Creates a new Amazon FSx backup with an assigned ID, and an initial lifecycle state of CREATING
.
*
*
* -
*
* Returns the description of the backup.
*
*
*
*
* By using the idempotent operation, you can retry a CreateBackup
operation without the risk of
* creating an extra backup. This approach can be useful when an initial call fails in a way that makes it unclear
* whether a backup was created. If you use the same client request token and the initial call created a backup, the
* operation returns a successful result because all the parameters are the same.
*
*
* The CreateBackup
operation returns while the backup's lifecycle state is still CREATING
* . You can check the backup creation status by calling the DescribeBackups
* operation, which returns the backup state along with other information.
*
*
* @param createBackupRequest
* The request object for the CreateBackup
operation.
* @return A Java Future containing the result of the CreateBackup operation returned by the service.
* @sample AmazonFSxAsync.CreateBackup
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createBackupAsync(CreateBackupRequest createBackupRequest);
/**
*
* Creates a backup of an existing Amazon FSx for Windows File Server file system, Amazon FSx for Lustre file
* system, Amazon FSx for NetApp ONTAP volume, or Amazon FSx for OpenZFS file system. We recommend creating regular
* backups so that you can restore a file system or volume from a backup if an issue arises with the original file
* system or volume.
*
*
* For Amazon FSx for Lustre file systems, you can create a backup only for file systems that have the following
* configuration:
*
*
* -
*
* A Persistent deployment type
*
*
* -
*
* Are not linked to a data repository
*
*
*
*
* For more information about backups, see the following:
*
*
* -
*
* For Amazon FSx for Lustre, see Working with FSx for Lustre
* backups.
*
*
* -
*
* For Amazon FSx for Windows, see Working with FSx for Windows
* backups.
*
*
* -
*
* For Amazon FSx for NetApp ONTAP, see Working with FSx for NetApp ONTAP
* backups.
*
*
* -
*
* For Amazon FSx for OpenZFS, see Working with FSx for OpenZFS
* backups.
*
*
*
*
* If a backup with the specified client request token exists and the parameters match, this operation returns the
* description of the existing backup. If a backup with the specified client request token exists and the parameters
* don't match, this operation returns IncompatibleParameterError
. If a backup with the specified
* client request token doesn't exist, CreateBackup
does the following:
*
*
* -
*
* Creates a new Amazon FSx backup with an assigned ID, and an initial lifecycle state of CREATING
.
*
*
* -
*
* Returns the description of the backup.
*
*
*
*
* By using the idempotent operation, you can retry a CreateBackup
operation without the risk of
* creating an extra backup. This approach can be useful when an initial call fails in a way that makes it unclear
* whether a backup was created. If you use the same client request token and the initial call created a backup, the
* operation returns a successful result because all the parameters are the same.
*
*
* The CreateBackup
operation returns while the backup's lifecycle state is still CREATING
* . You can check the backup creation status by calling the DescribeBackups
* operation, which returns the backup state along with other information.
*
*
* @param createBackupRequest
* The request object for the CreateBackup
operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateBackup operation returned by the service.
* @sample AmazonFSxAsyncHandler.CreateBackup
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createBackupAsync(CreateBackupRequest createBackupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates an Amazon FSx for Lustre data repository association (DRA). A data repository association is a link
* between a directory on the file system and an Amazon S3 bucket or prefix. You can have a maximum of 8 data
* repository associations on a file system. Data repository associations are supported on all FSx for Lustre 2.12
* and 2.15 file systems, excluding scratch_1
deployment type.
*
*
* Each data repository association must have a unique Amazon FSx file system directory and a unique S3 bucket or
* prefix associated with it. You can configure a data repository association for automatic import only, for
* automatic export only, or for both. To learn more about linking a data repository to your file system, see Linking your file
* system to an S3 bucket.
*
*
*
* CreateDataRepositoryAssociation
isn't supported on Amazon File Cache resources. To create a DRA on
* Amazon File Cache, use the CreateFileCache
operation.
*
*
*
* @param createDataRepositoryAssociationRequest
* @return A Java Future containing the result of the CreateDataRepositoryAssociation operation returned by the
* service.
* @sample AmazonFSxAsync.CreateDataRepositoryAssociation
* @see AWS API Documentation
*/
java.util.concurrent.Future createDataRepositoryAssociationAsync(
CreateDataRepositoryAssociationRequest createDataRepositoryAssociationRequest);
/**
*
* Creates an Amazon FSx for Lustre data repository association (DRA). A data repository association is a link
* between a directory on the file system and an Amazon S3 bucket or prefix. You can have a maximum of 8 data
* repository associations on a file system. Data repository associations are supported on all FSx for Lustre 2.12
* and 2.15 file systems, excluding scratch_1
deployment type.
*
*
* Each data repository association must have a unique Amazon FSx file system directory and a unique S3 bucket or
* prefix associated with it. You can configure a data repository association for automatic import only, for
* automatic export only, or for both. To learn more about linking a data repository to your file system, see Linking your file
* system to an S3 bucket.
*
*
*
* CreateDataRepositoryAssociation
isn't supported on Amazon File Cache resources. To create a DRA on
* Amazon File Cache, use the CreateFileCache
operation.
*
*
*
* @param createDataRepositoryAssociationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateDataRepositoryAssociation operation returned by the
* service.
* @sample AmazonFSxAsyncHandler.CreateDataRepositoryAssociation
* @see AWS API Documentation
*/
java.util.concurrent.Future createDataRepositoryAssociationAsync(
CreateDataRepositoryAssociationRequest createDataRepositoryAssociationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates an Amazon FSx for Lustre data repository task. A CreateDataRepositoryTask
operation will
* fail if a data repository is not linked to the FSx file system.
*
*
* You use import and export data repository tasks to perform bulk operations between your FSx for Lustre file
* system and its linked data repositories. An example of a data repository task is exporting any data and metadata
* changes, including POSIX metadata, to files, directories, and symbolic links (symlinks) from your FSx file system
* to a linked data repository.
*
*
* You use release data repository tasks to release data from your file system for files that are exported to S3.
* The metadata of released files remains on the file system so users or applications can still access released
* files by reading the files again, which will restore data from Amazon S3 to the FSx for Lustre file system.
*
*
* To learn more about data repository tasks, see Data Repository Tasks.
* To learn more about linking a data repository to your file system, see Linking your file
* system to an S3 bucket.
*
*
* @param createDataRepositoryTaskRequest
* @return A Java Future containing the result of the CreateDataRepositoryTask operation returned by the service.
* @sample AmazonFSxAsync.CreateDataRepositoryTask
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createDataRepositoryTaskAsync(CreateDataRepositoryTaskRequest createDataRepositoryTaskRequest);
/**
*
* Creates an Amazon FSx for Lustre data repository task. A CreateDataRepositoryTask
operation will
* fail if a data repository is not linked to the FSx file system.
*
*
* You use import and export data repository tasks to perform bulk operations between your FSx for Lustre file
* system and its linked data repositories. An example of a data repository task is exporting any data and metadata
* changes, including POSIX metadata, to files, directories, and symbolic links (symlinks) from your FSx file system
* to a linked data repository.
*
*
* You use release data repository tasks to release data from your file system for files that are exported to S3.
* The metadata of released files remains on the file system so users or applications can still access released
* files by reading the files again, which will restore data from Amazon S3 to the FSx for Lustre file system.
*
*
* To learn more about data repository tasks, see Data Repository Tasks.
* To learn more about linking a data repository to your file system, see Linking your file
* system to an S3 bucket.
*
*
* @param createDataRepositoryTaskRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateDataRepositoryTask operation returned by the service.
* @sample AmazonFSxAsyncHandler.CreateDataRepositoryTask
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createDataRepositoryTaskAsync(CreateDataRepositoryTaskRequest createDataRepositoryTaskRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a new Amazon File Cache resource.
*
*
* You can use this operation with a client request token in the request that Amazon File Cache uses to ensure
* idempotent creation. If a cache with the specified client request token exists and the parameters match,
* CreateFileCache
returns the description of the existing cache. If a cache with the specified client
* request token exists and the parameters don't match, this call returns IncompatibleParameterError
.
* If a file cache with the specified client request token doesn't exist, CreateFileCache
does the
* following:
*
*
* -
*
* Creates a new, empty Amazon File Cache resourcewith an assigned ID, and an initial lifecycle state of
* CREATING
.
*
*
* -
*
* Returns the description of the cache in JSON format.
*
*
*
*
*
* The CreateFileCache
call returns while the cache's lifecycle state is still CREATING
.
* You can check the cache creation status by calling the DescribeFileCaches
* operation, which returns the cache state along with other information.
*
*
*
* @param createFileCacheRequest
* @return A Java Future containing the result of the CreateFileCache operation returned by the service.
* @sample AmazonFSxAsync.CreateFileCache
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createFileCacheAsync(CreateFileCacheRequest createFileCacheRequest);
/**
*
* Creates a new Amazon File Cache resource.
*
*
* You can use this operation with a client request token in the request that Amazon File Cache uses to ensure
* idempotent creation. If a cache with the specified client request token exists and the parameters match,
* CreateFileCache
returns the description of the existing cache. If a cache with the specified client
* request token exists and the parameters don't match, this call returns IncompatibleParameterError
.
* If a file cache with the specified client request token doesn't exist, CreateFileCache
does the
* following:
*
*
* -
*
* Creates a new, empty Amazon File Cache resourcewith an assigned ID, and an initial lifecycle state of
* CREATING
.
*
*
* -
*
* Returns the description of the cache in JSON format.
*
*
*
*
*
* The CreateFileCache
call returns while the cache's lifecycle state is still CREATING
.
* You can check the cache creation status by calling the DescribeFileCaches
* operation, which returns the cache state along with other information.
*
*
*
* @param createFileCacheRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateFileCache operation returned by the service.
* @sample AmazonFSxAsyncHandler.CreateFileCache
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createFileCacheAsync(CreateFileCacheRequest createFileCacheRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a new, empty Amazon FSx file system. You can create the following supported Amazon FSx file systems using
* the CreateFileSystem
API operation:
*
*
* -
*
* Amazon FSx for Lustre
*
*
* -
*
* Amazon FSx for NetApp ONTAP
*
*
* -
*
* Amazon FSx for OpenZFS
*
*
* -
*
* Amazon FSx for Windows File Server
*
*
*
*
* This operation requires a client request token in the request that Amazon FSx uses to ensure idempotent creation.
* This means that calling the operation multiple times with the same client request token has no effect. By using
* the idempotent operation, you can retry a CreateFileSystem
operation without the risk of creating an
* extra file system. This approach can be useful when an initial call fails in a way that makes it unclear whether
* a file system was created. Examples are if a transport level timeout occurred, or your connection was reset. If
* you use the same client request token and the initial call created a file system, the client receives success as
* long as the parameters are the same.
*
*
* If a file system with the specified client request token exists and the parameters match,
* CreateFileSystem
returns the description of the existing file system. If a file system with the
* specified client request token exists and the parameters don't match, this call returns
* IncompatibleParameterError
. If a file system with the specified client request token doesn't exist,
* CreateFileSystem
does the following:
*
*
* -
*
* Creates a new, empty Amazon FSx file system with an assigned ID, and an initial lifecycle state of
* CREATING
.
*
*
* -
*
* Returns the description of the file system in JSON format.
*
*
*
*
*
* The CreateFileSystem
call returns while the file system's lifecycle state is still
* CREATING
. You can check the file-system creation status by calling the DescribeFileSystems
* operation, which returns the file system state along with other information.
*
*
*
* @param createFileSystemRequest
* The request object used to create a new Amazon FSx file system.
* @return A Java Future containing the result of the CreateFileSystem operation returned by the service.
* @sample AmazonFSxAsync.CreateFileSystem
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createFileSystemAsync(CreateFileSystemRequest createFileSystemRequest);
/**
*
* Creates a new, empty Amazon FSx file system. You can create the following supported Amazon FSx file systems using
* the CreateFileSystem
API operation:
*
*
* -
*
* Amazon FSx for Lustre
*
*
* -
*
* Amazon FSx for NetApp ONTAP
*
*
* -
*
* Amazon FSx for OpenZFS
*
*
* -
*
* Amazon FSx for Windows File Server
*
*
*
*
* This operation requires a client request token in the request that Amazon FSx uses to ensure idempotent creation.
* This means that calling the operation multiple times with the same client request token has no effect. By using
* the idempotent operation, you can retry a CreateFileSystem
operation without the risk of creating an
* extra file system. This approach can be useful when an initial call fails in a way that makes it unclear whether
* a file system was created. Examples are if a transport level timeout occurred, or your connection was reset. If
* you use the same client request token and the initial call created a file system, the client receives success as
* long as the parameters are the same.
*
*
* If a file system with the specified client request token exists and the parameters match,
* CreateFileSystem
returns the description of the existing file system. If a file system with the
* specified client request token exists and the parameters don't match, this call returns
* IncompatibleParameterError
. If a file system with the specified client request token doesn't exist,
* CreateFileSystem
does the following:
*
*
* -
*
* Creates a new, empty Amazon FSx file system with an assigned ID, and an initial lifecycle state of
* CREATING
.
*
*
* -
*
* Returns the description of the file system in JSON format.
*
*
*
*
*
* The CreateFileSystem
call returns while the file system's lifecycle state is still
* CREATING
. You can check the file-system creation status by calling the DescribeFileSystems
* operation, which returns the file system state along with other information.
*
*
*
* @param createFileSystemRequest
* The request object used to create a new Amazon FSx file system.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateFileSystem operation returned by the service.
* @sample AmazonFSxAsyncHandler.CreateFileSystem
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createFileSystemAsync(CreateFileSystemRequest createFileSystemRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a new Amazon FSx for Lustre, Amazon FSx for Windows File Server, or Amazon FSx for OpenZFS file system
* from an existing Amazon FSx backup.
*
*
* If a file system with the specified client request token exists and the parameters match, this operation returns
* the description of the file system. If a file system with the specified client request token exists but the
* parameters don't match, this call returns IncompatibleParameterError
. If a file system with the
* specified client request token doesn't exist, this operation does the following:
*
*
* -
*
* Creates a new Amazon FSx file system from backup with an assigned ID, and an initial lifecycle state of
* CREATING
.
*
*
* -
*
* Returns the description of the file system.
*
*
*
*
* Parameters like the Active Directory, default share name, automatic backup, and backup settings default to the
* parameters of the file system that was backed up, unless overridden. You can explicitly supply other settings.
*
*
* By using the idempotent operation, you can retry a CreateFileSystemFromBackup
call without the risk
* of creating an extra file system. This approach can be useful when an initial call fails in a way that makes it
* unclear whether a file system was created. Examples are if a transport level timeout occurred, or your connection
* was reset. If you use the same client request token and the initial call created a file system, the client
* receives a success message as long as the parameters are the same.
*
*
*
* The CreateFileSystemFromBackup
call returns while the file system's lifecycle state is still
* CREATING
. You can check the file-system creation status by calling the DescribeFileSystems
* operation, which returns the file system state along with other information.
*
*
*
* @param createFileSystemFromBackupRequest
* The request object for the CreateFileSystemFromBackup
operation.
* @return A Java Future containing the result of the CreateFileSystemFromBackup operation returned by the service.
* @sample AmazonFSxAsync.CreateFileSystemFromBackup
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createFileSystemFromBackupAsync(
CreateFileSystemFromBackupRequest createFileSystemFromBackupRequest);
/**
*
* Creates a new Amazon FSx for Lustre, Amazon FSx for Windows File Server, or Amazon FSx for OpenZFS file system
* from an existing Amazon FSx backup.
*
*
* If a file system with the specified client request token exists and the parameters match, this operation returns
* the description of the file system. If a file system with the specified client request token exists but the
* parameters don't match, this call returns IncompatibleParameterError
. If a file system with the
* specified client request token doesn't exist, this operation does the following:
*
*
* -
*
* Creates a new Amazon FSx file system from backup with an assigned ID, and an initial lifecycle state of
* CREATING
.
*
*
* -
*
* Returns the description of the file system.
*
*
*
*
* Parameters like the Active Directory, default share name, automatic backup, and backup settings default to the
* parameters of the file system that was backed up, unless overridden. You can explicitly supply other settings.
*
*
* By using the idempotent operation, you can retry a CreateFileSystemFromBackup
call without the risk
* of creating an extra file system. This approach can be useful when an initial call fails in a way that makes it
* unclear whether a file system was created. Examples are if a transport level timeout occurred, or your connection
* was reset. If you use the same client request token and the initial call created a file system, the client
* receives a success message as long as the parameters are the same.
*
*
*
* The CreateFileSystemFromBackup
call returns while the file system's lifecycle state is still
* CREATING
. You can check the file-system creation status by calling the DescribeFileSystems
* operation, which returns the file system state along with other information.
*
*
*
* @param createFileSystemFromBackupRequest
* The request object for the CreateFileSystemFromBackup
operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateFileSystemFromBackup operation returned by the service.
* @sample AmazonFSxAsyncHandler.CreateFileSystemFromBackup
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createFileSystemFromBackupAsync(
CreateFileSystemFromBackupRequest createFileSystemFromBackupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a snapshot of an existing Amazon FSx for OpenZFS volume. With snapshots, you can easily undo file changes
* and compare file versions by restoring the volume to a previous version.
*
*
* If a snapshot with the specified client request token exists, and the parameters match, this operation returns
* the description of the existing snapshot. If a snapshot with the specified client request token exists, and the
* parameters don't match, this operation returns IncompatibleParameterError
. If a snapshot with the
* specified client request token doesn't exist, CreateSnapshot
does the following:
*
*
* -
*
* Creates a new OpenZFS snapshot with an assigned ID, and an initial lifecycle state of CREATING
.
*
*
* -
*
* Returns the description of the snapshot.
*
*
*
*
* By using the idempotent operation, you can retry a CreateSnapshot
operation without the risk of
* creating an extra snapshot. This approach can be useful when an initial call fails in a way that makes it unclear
* whether a snapshot was created. If you use the same client request token and the initial call created a snapshot,
* the operation returns a successful result because all the parameters are the same.
*
*
* The CreateSnapshot
operation returns while the snapshot's lifecycle state is still
* CREATING
. You can check the snapshot creation status by calling the DescribeSnapshots
* operation, which returns the snapshot state along with other information.
*
*
* @param createSnapshotRequest
* @return A Java Future containing the result of the CreateSnapshot operation returned by the service.
* @sample AmazonFSxAsync.CreateSnapshot
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createSnapshotAsync(CreateSnapshotRequest createSnapshotRequest);
/**
*
* Creates a snapshot of an existing Amazon FSx for OpenZFS volume. With snapshots, you can easily undo file changes
* and compare file versions by restoring the volume to a previous version.
*
*
* If a snapshot with the specified client request token exists, and the parameters match, this operation returns
* the description of the existing snapshot. If a snapshot with the specified client request token exists, and the
* parameters don't match, this operation returns IncompatibleParameterError
. If a snapshot with the
* specified client request token doesn't exist, CreateSnapshot
does the following:
*
*
* -
*
* Creates a new OpenZFS snapshot with an assigned ID, and an initial lifecycle state of CREATING
.
*
*
* -
*
* Returns the description of the snapshot.
*
*
*
*
* By using the idempotent operation, you can retry a CreateSnapshot
operation without the risk of
* creating an extra snapshot. This approach can be useful when an initial call fails in a way that makes it unclear
* whether a snapshot was created. If you use the same client request token and the initial call created a snapshot,
* the operation returns a successful result because all the parameters are the same.
*
*
* The CreateSnapshot
operation returns while the snapshot's lifecycle state is still
* CREATING
. You can check the snapshot creation status by calling the DescribeSnapshots
* operation, which returns the snapshot state along with other information.
*
*
* @param createSnapshotRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateSnapshot operation returned by the service.
* @sample AmazonFSxAsyncHandler.CreateSnapshot
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createSnapshotAsync(CreateSnapshotRequest createSnapshotRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a storage virtual machine (SVM) for an Amazon FSx for ONTAP file system.
*
*
* @param createStorageVirtualMachineRequest
* @return A Java Future containing the result of the CreateStorageVirtualMachine operation returned by the service.
* @sample AmazonFSxAsync.CreateStorageVirtualMachine
* @see AWS API Documentation
*/
java.util.concurrent.Future createStorageVirtualMachineAsync(
CreateStorageVirtualMachineRequest createStorageVirtualMachineRequest);
/**
*
* Creates a storage virtual machine (SVM) for an Amazon FSx for ONTAP file system.
*
*
* @param createStorageVirtualMachineRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateStorageVirtualMachine operation returned by the service.
* @sample AmazonFSxAsyncHandler.CreateStorageVirtualMachine
* @see AWS API Documentation
*/
java.util.concurrent.Future createStorageVirtualMachineAsync(
CreateStorageVirtualMachineRequest createStorageVirtualMachineRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates an FSx for ONTAP or Amazon FSx for OpenZFS storage volume.
*
*
* @param createVolumeRequest
* @return A Java Future containing the result of the CreateVolume operation returned by the service.
* @sample AmazonFSxAsync.CreateVolume
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createVolumeAsync(CreateVolumeRequest createVolumeRequest);
/**
*
* Creates an FSx for ONTAP or Amazon FSx for OpenZFS storage volume.
*
*
* @param createVolumeRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateVolume operation returned by the service.
* @sample AmazonFSxAsyncHandler.CreateVolume
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createVolumeAsync(CreateVolumeRequest createVolumeRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a new Amazon FSx for NetApp ONTAP volume from an existing Amazon FSx volume backup.
*
*
* @param createVolumeFromBackupRequest
* @return A Java Future containing the result of the CreateVolumeFromBackup operation returned by the service.
* @sample AmazonFSxAsync.CreateVolumeFromBackup
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createVolumeFromBackupAsync(CreateVolumeFromBackupRequest createVolumeFromBackupRequest);
/**
*
* Creates a new Amazon FSx for NetApp ONTAP volume from an existing Amazon FSx volume backup.
*
*
* @param createVolumeFromBackupRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateVolumeFromBackup operation returned by the service.
* @sample AmazonFSxAsyncHandler.CreateVolumeFromBackup
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createVolumeFromBackupAsync(CreateVolumeFromBackupRequest createVolumeFromBackupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes an Amazon FSx backup. After deletion, the backup no longer exists, and its data is gone.
*
*
* The DeleteBackup
call returns instantly. The backup won't show up in later
* DescribeBackups
calls.
*
*
*
* The data in a deleted backup is also deleted and can't be recovered by any means.
*
*
*
* @param deleteBackupRequest
* The request object for the DeleteBackup
operation.
* @return A Java Future containing the result of the DeleteBackup operation returned by the service.
* @sample AmazonFSxAsync.DeleteBackup
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteBackupAsync(DeleteBackupRequest deleteBackupRequest);
/**
*
* Deletes an Amazon FSx backup. After deletion, the backup no longer exists, and its data is gone.
*
*
* The DeleteBackup
call returns instantly. The backup won't show up in later
* DescribeBackups
calls.
*
*
*
* The data in a deleted backup is also deleted and can't be recovered by any means.
*
*
*
* @param deleteBackupRequest
* The request object for the DeleteBackup
operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteBackup operation returned by the service.
* @sample AmazonFSxAsyncHandler.DeleteBackup
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteBackupAsync(DeleteBackupRequest deleteBackupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a data repository association on an Amazon FSx for Lustre file system. Deleting the data repository
* association unlinks the file system from the Amazon S3 bucket. When deleting a data repository association, you
* have the option of deleting the data in the file system that corresponds to the data repository association. Data
* repository associations are supported on all FSx for Lustre 2.12 and 2.15 file systems, excluding
* scratch_1
deployment type.
*
*
* @param deleteDataRepositoryAssociationRequest
* @return A Java Future containing the result of the DeleteDataRepositoryAssociation operation returned by the
* service.
* @sample AmazonFSxAsync.DeleteDataRepositoryAssociation
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteDataRepositoryAssociationAsync(
DeleteDataRepositoryAssociationRequest deleteDataRepositoryAssociationRequest);
/**
*
* Deletes a data repository association on an Amazon FSx for Lustre file system. Deleting the data repository
* association unlinks the file system from the Amazon S3 bucket. When deleting a data repository association, you
* have the option of deleting the data in the file system that corresponds to the data repository association. Data
* repository associations are supported on all FSx for Lustre 2.12 and 2.15 file systems, excluding
* scratch_1
deployment type.
*
*
* @param deleteDataRepositoryAssociationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteDataRepositoryAssociation operation returned by the
* service.
* @sample AmazonFSxAsyncHandler.DeleteDataRepositoryAssociation
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteDataRepositoryAssociationAsync(
DeleteDataRepositoryAssociationRequest deleteDataRepositoryAssociationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes an Amazon File Cache resource. After deletion, the cache no longer exists, and its data is gone.
*
*
* The DeleteFileCache
operation returns while the cache has the DELETING
status. You can
* check the cache deletion status by calling the DescribeFileCaches
* operation, which returns a list of caches in your account. If you pass the cache ID for a deleted cache, the
* DescribeFileCaches
operation returns a FileCacheNotFound
error.
*
*
*
* The data in a deleted cache is also deleted and can't be recovered by any means.
*
*
*
* @param deleteFileCacheRequest
* @return A Java Future containing the result of the DeleteFileCache operation returned by the service.
* @sample AmazonFSxAsync.DeleteFileCache
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteFileCacheAsync(DeleteFileCacheRequest deleteFileCacheRequest);
/**
*
* Deletes an Amazon File Cache resource. After deletion, the cache no longer exists, and its data is gone.
*
*
* The DeleteFileCache
operation returns while the cache has the DELETING
status. You can
* check the cache deletion status by calling the DescribeFileCaches
* operation, which returns a list of caches in your account. If you pass the cache ID for a deleted cache, the
* DescribeFileCaches
operation returns a FileCacheNotFound
error.
*
*
*
* The data in a deleted cache is also deleted and can't be recovered by any means.
*
*
*
* @param deleteFileCacheRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteFileCache operation returned by the service.
* @sample AmazonFSxAsyncHandler.DeleteFileCache
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteFileCacheAsync(DeleteFileCacheRequest deleteFileCacheRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a file system. After deletion, the file system no longer exists, and its data is gone. Any existing
* automatic backups and snapshots are also deleted.
*
*
* To delete an Amazon FSx for NetApp ONTAP file system, first delete all the volumes and storage virtual machines
* (SVMs) on the file system. Then provide a FileSystemId
value to the DeleteFileSystem
* operation.
*
*
* By default, when you delete an Amazon FSx for Windows File Server file system, a final backup is created upon
* deletion. This final backup isn't subject to the file system's retention policy, and must be manually deleted.
*
*
* To delete an Amazon FSx for Lustre file system, first unmount it from every connected
* Amazon EC2 instance, then provide a FileSystemId
value to the DeleteFileSystem
* operation. By default, Amazon FSx will not take a final backup when the DeleteFileSystem
operation
* is invoked. On file systems not linked to an Amazon S3 bucket, set SkipFinalBackup
to
* false
to take a final backup of the file system you are deleting. Backups cannot be enabled on
* S3-linked file systems. To ensure all of your data is written back to S3 before deleting your file system, you
* can either monitor for the AgeOfOldestQueuedMessage metric to be zero (if using automatic export) or you can run an export data repository
* task. If you have automatic export enabled and want to use an export data repository task, you have to
* disable automatic export before executing the export data repository task.
*
*
* The DeleteFileSystem
operation returns while the file system has the DELETING
status.
* You can check the file system deletion status by calling the DescribeFileSystems
* operation, which returns a list of file systems in your account. If you pass the file system ID for a deleted
* file system, the DescribeFileSystems
operation returns a FileSystemNotFound
error.
*
*
*
* If a data repository task is in a PENDING
or EXECUTING
state, deleting an Amazon FSx
* for Lustre file system will fail with an HTTP status code 400 (Bad Request).
*
*
*
* The data in a deleted file system is also deleted and can't be recovered by any means.
*
*
*
* @param deleteFileSystemRequest
* The request object for DeleteFileSystem
operation.
* @return A Java Future containing the result of the DeleteFileSystem operation returned by the service.
* @sample AmazonFSxAsync.DeleteFileSystem
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteFileSystemAsync(DeleteFileSystemRequest deleteFileSystemRequest);
/**
*
* Deletes a file system. After deletion, the file system no longer exists, and its data is gone. Any existing
* automatic backups and snapshots are also deleted.
*
*
* To delete an Amazon FSx for NetApp ONTAP file system, first delete all the volumes and storage virtual machines
* (SVMs) on the file system. Then provide a FileSystemId
value to the DeleteFileSystem
* operation.
*
*
* By default, when you delete an Amazon FSx for Windows File Server file system, a final backup is created upon
* deletion. This final backup isn't subject to the file system's retention policy, and must be manually deleted.
*
*
* To delete an Amazon FSx for Lustre file system, first unmount it from every connected
* Amazon EC2 instance, then provide a FileSystemId
value to the DeleteFileSystem
* operation. By default, Amazon FSx will not take a final backup when the DeleteFileSystem
operation
* is invoked. On file systems not linked to an Amazon S3 bucket, set SkipFinalBackup
to
* false
to take a final backup of the file system you are deleting. Backups cannot be enabled on
* S3-linked file systems. To ensure all of your data is written back to S3 before deleting your file system, you
* can either monitor for the AgeOfOldestQueuedMessage metric to be zero (if using automatic export) or you can run an export data repository
* task. If you have automatic export enabled and want to use an export data repository task, you have to
* disable automatic export before executing the export data repository task.
*
*
* The DeleteFileSystem
operation returns while the file system has the DELETING
status.
* You can check the file system deletion status by calling the DescribeFileSystems
* operation, which returns a list of file systems in your account. If you pass the file system ID for a deleted
* file system, the DescribeFileSystems
operation returns a FileSystemNotFound
error.
*
*
*
* If a data repository task is in a PENDING
or EXECUTING
state, deleting an Amazon FSx
* for Lustre file system will fail with an HTTP status code 400 (Bad Request).
*
*
*
* The data in a deleted file system is also deleted and can't be recovered by any means.
*
*
*
* @param deleteFileSystemRequest
* The request object for DeleteFileSystem
operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteFileSystem operation returned by the service.
* @sample AmazonFSxAsyncHandler.DeleteFileSystem
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteFileSystemAsync(DeleteFileSystemRequest deleteFileSystemRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes an Amazon FSx for OpenZFS snapshot. After deletion, the snapshot no longer exists, and its data is gone.
* Deleting a snapshot doesn't affect snapshots stored in a file system backup.
*
*
* The DeleteSnapshot
operation returns instantly. The snapshot appears with the lifecycle status of
* DELETING
until the deletion is complete.
*
*
* @param deleteSnapshotRequest
* @return A Java Future containing the result of the DeleteSnapshot operation returned by the service.
* @sample AmazonFSxAsync.DeleteSnapshot
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteSnapshotAsync(DeleteSnapshotRequest deleteSnapshotRequest);
/**
*
* Deletes an Amazon FSx for OpenZFS snapshot. After deletion, the snapshot no longer exists, and its data is gone.
* Deleting a snapshot doesn't affect snapshots stored in a file system backup.
*
*
* The DeleteSnapshot
operation returns instantly. The snapshot appears with the lifecycle status of
* DELETING
until the deletion is complete.
*
*
* @param deleteSnapshotRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteSnapshot operation returned by the service.
* @sample AmazonFSxAsyncHandler.DeleteSnapshot
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteSnapshotAsync(DeleteSnapshotRequest deleteSnapshotRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes an existing Amazon FSx for ONTAP storage virtual machine (SVM). Prior to deleting an SVM, you must delete
* all non-root volumes in the SVM, otherwise the operation will fail.
*
*
* @param deleteStorageVirtualMachineRequest
* @return A Java Future containing the result of the DeleteStorageVirtualMachine operation returned by the service.
* @sample AmazonFSxAsync.DeleteStorageVirtualMachine
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteStorageVirtualMachineAsync(
DeleteStorageVirtualMachineRequest deleteStorageVirtualMachineRequest);
/**
*
* Deletes an existing Amazon FSx for ONTAP storage virtual machine (SVM). Prior to deleting an SVM, you must delete
* all non-root volumes in the SVM, otherwise the operation will fail.
*
*
* @param deleteStorageVirtualMachineRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteStorageVirtualMachine operation returned by the service.
* @sample AmazonFSxAsyncHandler.DeleteStorageVirtualMachine
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteStorageVirtualMachineAsync(
DeleteStorageVirtualMachineRequest deleteStorageVirtualMachineRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes an Amazon FSx for NetApp ONTAP or Amazon FSx for OpenZFS volume.
*
*
* @param deleteVolumeRequest
* @return A Java Future containing the result of the DeleteVolume operation returned by the service.
* @sample AmazonFSxAsync.DeleteVolume
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteVolumeAsync(DeleteVolumeRequest deleteVolumeRequest);
/**
*
* Deletes an Amazon FSx for NetApp ONTAP or Amazon FSx for OpenZFS volume.
*
*
* @param deleteVolumeRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteVolume operation returned by the service.
* @sample AmazonFSxAsyncHandler.DeleteVolume
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteVolumeAsync(DeleteVolumeRequest deleteVolumeRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns the description of a specific Amazon FSx backup, if a BackupIds
value is provided for that
* backup. Otherwise, it returns all backups owned by your Amazon Web Services account in the Amazon Web Services
* Region of the endpoint that you're calling.
*
*
* When retrieving all backups, you can optionally specify the MaxResults
parameter to limit the number
* of backups in a response. If more backups remain, Amazon FSx returns a NextToken
value in the
* response. In this case, send a later request with the NextToken
request parameter set to the value
* of the NextToken
value from the last response.
*
*
* This operation is used in an iterative process to retrieve a list of your backups. DescribeBackups
* is called first without a NextToken
value. Then the operation continues to be called with the
* NextToken
parameter set to the value of the last NextToken
value until a response has
* no NextToken
value.
*
*
* When using this operation, keep the following in mind:
*
*
* -
*
* The operation might return fewer than the MaxResults
value of backup descriptions while still
* including a NextToken
value.
*
*
* -
*
* The order of the backups returned in the response of one DescribeBackups
call and the order of the
* backups returned across the responses of a multi-call iteration is unspecified.
*
*
*
*
* @param describeBackupsRequest
* The request object for the DescribeBackups
operation.
* @return A Java Future containing the result of the DescribeBackups operation returned by the service.
* @sample AmazonFSxAsync.DescribeBackups
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeBackupsAsync(DescribeBackupsRequest describeBackupsRequest);
/**
*
* Returns the description of a specific Amazon FSx backup, if a BackupIds
value is provided for that
* backup. Otherwise, it returns all backups owned by your Amazon Web Services account in the Amazon Web Services
* Region of the endpoint that you're calling.
*
*
* When retrieving all backups, you can optionally specify the MaxResults
parameter to limit the number
* of backups in a response. If more backups remain, Amazon FSx returns a NextToken
value in the
* response. In this case, send a later request with the NextToken
request parameter set to the value
* of the NextToken
value from the last response.
*
*
* This operation is used in an iterative process to retrieve a list of your backups. DescribeBackups
* is called first without a NextToken
value. Then the operation continues to be called with the
* NextToken
parameter set to the value of the last NextToken
value until a response has
* no NextToken
value.
*
*
* When using this operation, keep the following in mind:
*
*
* -
*
* The operation might return fewer than the MaxResults
value of backup descriptions while still
* including a NextToken
value.
*
*
* -
*
* The order of the backups returned in the response of one DescribeBackups
call and the order of the
* backups returned across the responses of a multi-call iteration is unspecified.
*
*
*
*
* @param describeBackupsRequest
* The request object for the DescribeBackups
operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeBackups operation returned by the service.
* @sample AmazonFSxAsyncHandler.DescribeBackups
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeBackupsAsync(DescribeBackupsRequest describeBackupsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns the description of specific Amazon FSx for Lustre or Amazon File Cache data repository associations, if
* one or more AssociationIds
values are provided in the request, or if filters are used in the
* request. Data repository associations are supported on Amazon File Cache resources and all FSx for Lustre 2.12
* and 2,15 file systems, excluding scratch_1
deployment type.
*
*
* You can use filters to narrow the response to include just data repository associations for specific file systems
* (use the file-system-id
filter with the ID of the file system) or caches (use the
* file-cache-id
filter with the ID of the cache), or data repository associations for a specific
* repository type (use the data-repository-type
filter with a value of S3
or
* NFS
). If you don't use filters, the response returns all data repository associations owned by your
* Amazon Web Services account in the Amazon Web Services Region of the endpoint that you're calling.
*
*
* When retrieving all data repository associations, you can paginate the response by using the optional
* MaxResults
parameter to limit the number of data repository associations returned in a response. If
* more data repository associations remain, a NextToken
value is returned in the response. In this
* case, send a later request with the NextToken
request parameter set to the value of
* NextToken
from the last response.
*
*
* @param describeDataRepositoryAssociationsRequest
* @return A Java Future containing the result of the DescribeDataRepositoryAssociations operation returned by the
* service.
* @sample AmazonFSxAsync.DescribeDataRepositoryAssociations
* @see AWS API Documentation
*/
java.util.concurrent.Future describeDataRepositoryAssociationsAsync(
DescribeDataRepositoryAssociationsRequest describeDataRepositoryAssociationsRequest);
/**
*
* Returns the description of specific Amazon FSx for Lustre or Amazon File Cache data repository associations, if
* one or more AssociationIds
values are provided in the request, or if filters are used in the
* request. Data repository associations are supported on Amazon File Cache resources and all FSx for Lustre 2.12
* and 2,15 file systems, excluding scratch_1
deployment type.
*
*
* You can use filters to narrow the response to include just data repository associations for specific file systems
* (use the file-system-id
filter with the ID of the file system) or caches (use the
* file-cache-id
filter with the ID of the cache), or data repository associations for a specific
* repository type (use the data-repository-type
filter with a value of S3
or
* NFS
). If you don't use filters, the response returns all data repository associations owned by your
* Amazon Web Services account in the Amazon Web Services Region of the endpoint that you're calling.
*
*
* When retrieving all data repository associations, you can paginate the response by using the optional
* MaxResults
parameter to limit the number of data repository associations returned in a response. If
* more data repository associations remain, a NextToken
value is returned in the response. In this
* case, send a later request with the NextToken
request parameter set to the value of
* NextToken
from the last response.
*
*
* @param describeDataRepositoryAssociationsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeDataRepositoryAssociations operation returned by the
* service.
* @sample AmazonFSxAsyncHandler.DescribeDataRepositoryAssociations
* @see AWS API Documentation
*/
java.util.concurrent.Future describeDataRepositoryAssociationsAsync(
DescribeDataRepositoryAssociationsRequest describeDataRepositoryAssociationsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns the description of specific Amazon FSx for Lustre or Amazon File Cache data repository tasks, if one or
* more TaskIds
values are provided in the request, or if filters are used in the request. You can use
* filters to narrow the response to include just tasks for specific file systems or caches, or tasks in a specific
* lifecycle state. Otherwise, it returns all data repository tasks owned by your Amazon Web Services account in the
* Amazon Web Services Region of the endpoint that you're calling.
*
*
* When retrieving all tasks, you can paginate the response by using the optional MaxResults
parameter
* to limit the number of tasks returned in a response. If more tasks remain, a NextToken
value is
* returned in the response. In this case, send a later request with the NextToken
request parameter
* set to the value of NextToken
from the last response.
*
*
* @param describeDataRepositoryTasksRequest
* @return A Java Future containing the result of the DescribeDataRepositoryTasks operation returned by the service.
* @sample AmazonFSxAsync.DescribeDataRepositoryTasks
* @see AWS API Documentation
*/
java.util.concurrent.Future describeDataRepositoryTasksAsync(
DescribeDataRepositoryTasksRequest describeDataRepositoryTasksRequest);
/**
*
* Returns the description of specific Amazon FSx for Lustre or Amazon File Cache data repository tasks, if one or
* more TaskIds
values are provided in the request, or if filters are used in the request. You can use
* filters to narrow the response to include just tasks for specific file systems or caches, or tasks in a specific
* lifecycle state. Otherwise, it returns all data repository tasks owned by your Amazon Web Services account in the
* Amazon Web Services Region of the endpoint that you're calling.
*
*
* When retrieving all tasks, you can paginate the response by using the optional MaxResults
parameter
* to limit the number of tasks returned in a response. If more tasks remain, a NextToken
value is
* returned in the response. In this case, send a later request with the NextToken
request parameter
* set to the value of NextToken
from the last response.
*
*
* @param describeDataRepositoryTasksRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeDataRepositoryTasks operation returned by the service.
* @sample AmazonFSxAsyncHandler.DescribeDataRepositoryTasks
* @see AWS API Documentation
*/
java.util.concurrent.Future describeDataRepositoryTasksAsync(
DescribeDataRepositoryTasksRequest describeDataRepositoryTasksRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns the description of a specific Amazon File Cache resource, if a FileCacheIds
value is
* provided for that cache. Otherwise, it returns descriptions of all caches owned by your Amazon Web Services
* account in the Amazon Web Services Region of the endpoint that you're calling.
*
*
* When retrieving all cache descriptions, you can optionally specify the MaxResults
parameter to limit
* the number of descriptions in a response. If more cache descriptions remain, the operation returns a
* NextToken
value in the response. In this case, send a later request with the NextToken
* request parameter set to the value of NextToken
from the last response.
*
*
* This operation is used in an iterative process to retrieve a list of your cache descriptions.
* DescribeFileCaches
is called first without a NextToken
value. Then the operation
* continues to be called with the NextToken
parameter set to the value of the last
* NextToken
value until a response has no NextToken
.
*
*
* When using this operation, keep the following in mind:
*
*
* -
*
* The implementation might return fewer than MaxResults
cache descriptions while still including a
* NextToken
value.
*
*
* -
*
* The order of caches returned in the response of one DescribeFileCaches
call and the order of caches
* returned across the responses of a multicall iteration is unspecified.
*
*
*
*
* @param describeFileCachesRequest
* @return A Java Future containing the result of the DescribeFileCaches operation returned by the service.
* @sample AmazonFSxAsync.DescribeFileCaches
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeFileCachesAsync(DescribeFileCachesRequest describeFileCachesRequest);
/**
*
* Returns the description of a specific Amazon File Cache resource, if a FileCacheIds
value is
* provided for that cache. Otherwise, it returns descriptions of all caches owned by your Amazon Web Services
* account in the Amazon Web Services Region of the endpoint that you're calling.
*
*
* When retrieving all cache descriptions, you can optionally specify the MaxResults
parameter to limit
* the number of descriptions in a response. If more cache descriptions remain, the operation returns a
* NextToken
value in the response. In this case, send a later request with the NextToken
* request parameter set to the value of NextToken
from the last response.
*
*
* This operation is used in an iterative process to retrieve a list of your cache descriptions.
* DescribeFileCaches
is called first without a NextToken
value. Then the operation
* continues to be called with the NextToken
parameter set to the value of the last
* NextToken
value until a response has no NextToken
.
*
*
* When using this operation, keep the following in mind:
*
*
* -
*
* The implementation might return fewer than MaxResults
cache descriptions while still including a
* NextToken
value.
*
*
* -
*
* The order of caches returned in the response of one DescribeFileCaches
call and the order of caches
* returned across the responses of a multicall iteration is unspecified.
*
*
*
*
* @param describeFileCachesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeFileCaches operation returned by the service.
* @sample AmazonFSxAsyncHandler.DescribeFileCaches
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeFileCachesAsync(DescribeFileCachesRequest describeFileCachesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns the DNS aliases that are associated with the specified Amazon FSx for Windows File Server file system. A
* history of all DNS aliases that have been associated with and disassociated from the file system is available in
* the list of AdministrativeAction provided in the DescribeFileSystems operation response.
*
*
* @param describeFileSystemAliasesRequest
* The request object for DescribeFileSystemAliases
operation.
* @return A Java Future containing the result of the DescribeFileSystemAliases operation returned by the service.
* @sample AmazonFSxAsync.DescribeFileSystemAliases
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeFileSystemAliasesAsync(
DescribeFileSystemAliasesRequest describeFileSystemAliasesRequest);
/**
*
* Returns the DNS aliases that are associated with the specified Amazon FSx for Windows File Server file system. A
* history of all DNS aliases that have been associated with and disassociated from the file system is available in
* the list of AdministrativeAction provided in the DescribeFileSystems operation response.
*
*
* @param describeFileSystemAliasesRequest
* The request object for DescribeFileSystemAliases
operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeFileSystemAliases operation returned by the service.
* @sample AmazonFSxAsyncHandler.DescribeFileSystemAliases
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeFileSystemAliasesAsync(
DescribeFileSystemAliasesRequest describeFileSystemAliasesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns the description of specific Amazon FSx file systems, if a FileSystemIds
value is provided
* for that file system. Otherwise, it returns descriptions of all file systems owned by your Amazon Web Services
* account in the Amazon Web Services Region of the endpoint that you're calling.
*
*
* When retrieving all file system descriptions, you can optionally specify the MaxResults
parameter to
* limit the number of descriptions in a response. If more file system descriptions remain, Amazon FSx returns a
* NextToken
value in the response. In this case, send a later request with the NextToken
* request parameter set to the value of NextToken
from the last response.
*
*
* This operation is used in an iterative process to retrieve a list of your file system descriptions.
* DescribeFileSystems
is called first without a NextToken
value. Then the operation
* continues to be called with the NextToken
parameter set to the value of the last
* NextToken
value until a response has no NextToken
.
*
*
* When using this operation, keep the following in mind:
*
*
* -
*
* The implementation might return fewer than MaxResults
file system descriptions while still including
* a NextToken
value.
*
*
* -
*
* The order of file systems returned in the response of one DescribeFileSystems
call and the order of
* file systems returned across the responses of a multicall iteration is unspecified.
*
*
*
*
* @param describeFileSystemsRequest
* The request object for DescribeFileSystems
operation.
* @return A Java Future containing the result of the DescribeFileSystems operation returned by the service.
* @sample AmazonFSxAsync.DescribeFileSystems
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeFileSystemsAsync(DescribeFileSystemsRequest describeFileSystemsRequest);
/**
*
* Returns the description of specific Amazon FSx file systems, if a FileSystemIds
value is provided
* for that file system. Otherwise, it returns descriptions of all file systems owned by your Amazon Web Services
* account in the Amazon Web Services Region of the endpoint that you're calling.
*
*
* When retrieving all file system descriptions, you can optionally specify the MaxResults
parameter to
* limit the number of descriptions in a response. If more file system descriptions remain, Amazon FSx returns a
* NextToken
value in the response. In this case, send a later request with the NextToken
* request parameter set to the value of NextToken
from the last response.
*
*
* This operation is used in an iterative process to retrieve a list of your file system descriptions.
* DescribeFileSystems
is called first without a NextToken
value. Then the operation
* continues to be called with the NextToken
parameter set to the value of the last
* NextToken
value until a response has no NextToken
.
*
*
* When using this operation, keep the following in mind:
*
*
* -
*
* The implementation might return fewer than MaxResults
file system descriptions while still including
* a NextToken
value.
*
*
* -
*
* The order of file systems returned in the response of one DescribeFileSystems
call and the order of
* file systems returned across the responses of a multicall iteration is unspecified.
*
*
*
*
* @param describeFileSystemsRequest
* The request object for DescribeFileSystems
operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeFileSystems operation returned by the service.
* @sample AmazonFSxAsyncHandler.DescribeFileSystems
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeFileSystemsAsync(DescribeFileSystemsRequest describeFileSystemsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Indicates whether participant accounts in your organization can create Amazon FSx for NetApp ONTAP Multi-AZ file
* systems in subnets that are shared by a virtual private cloud (VPC) owner. For more information, see Creating FSx for ONTAP file systems in shared subnets.
*
*
* @param describeSharedVpcConfigurationRequest
* @return A Java Future containing the result of the DescribeSharedVpcConfiguration operation returned by the
* service.
* @sample AmazonFSxAsync.DescribeSharedVpcConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future describeSharedVpcConfigurationAsync(
DescribeSharedVpcConfigurationRequest describeSharedVpcConfigurationRequest);
/**
*
* Indicates whether participant accounts in your organization can create Amazon FSx for NetApp ONTAP Multi-AZ file
* systems in subnets that are shared by a virtual private cloud (VPC) owner. For more information, see Creating FSx for ONTAP file systems in shared subnets.
*
*
* @param describeSharedVpcConfigurationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeSharedVpcConfiguration operation returned by the
* service.
* @sample AmazonFSxAsyncHandler.DescribeSharedVpcConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future describeSharedVpcConfigurationAsync(
DescribeSharedVpcConfigurationRequest describeSharedVpcConfigurationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns the description of specific Amazon FSx for OpenZFS snapshots, if a SnapshotIds
value is
* provided. Otherwise, this operation returns all snapshots owned by your Amazon Web Services account in the Amazon
* Web Services Region of the endpoint that you're calling.
*
*
* When retrieving all snapshots, you can optionally specify the MaxResults
parameter to limit the
* number of snapshots in a response. If more backups remain, Amazon FSx returns a NextToken
value in
* the response. In this case, send a later request with the NextToken
request parameter set to the
* value of NextToken
from the last response.
*
*
* Use this operation in an iterative process to retrieve a list of your snapshots. DescribeSnapshots
* is called first without a NextToken
value. Then the operation continues to be called with the
* NextToken
parameter set to the value of the last NextToken
value until a response has
* no NextToken
value.
*
*
* When using this operation, keep the following in mind:
*
*
* -
*
* The operation might return fewer than the MaxResults
value of snapshot descriptions while still
* including a NextToken
value.
*
*
* -
*
* The order of snapshots returned in the response of one DescribeSnapshots
call and the order of
* backups returned across the responses of a multi-call iteration is unspecified.
*
*
*
*
* @param describeSnapshotsRequest
* @return A Java Future containing the result of the DescribeSnapshots operation returned by the service.
* @sample AmazonFSxAsync.DescribeSnapshots
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeSnapshotsAsync(DescribeSnapshotsRequest describeSnapshotsRequest);
/**
*
* Returns the description of specific Amazon FSx for OpenZFS snapshots, if a SnapshotIds
value is
* provided. Otherwise, this operation returns all snapshots owned by your Amazon Web Services account in the Amazon
* Web Services Region of the endpoint that you're calling.
*
*
* When retrieving all snapshots, you can optionally specify the MaxResults
parameter to limit the
* number of snapshots in a response. If more backups remain, Amazon FSx returns a NextToken
value in
* the response. In this case, send a later request with the NextToken
request parameter set to the
* value of NextToken
from the last response.
*
*
* Use this operation in an iterative process to retrieve a list of your snapshots. DescribeSnapshots
* is called first without a NextToken
value. Then the operation continues to be called with the
* NextToken
parameter set to the value of the last NextToken
value until a response has
* no NextToken
value.
*
*
* When using this operation, keep the following in mind:
*
*
* -
*
* The operation might return fewer than the MaxResults
value of snapshot descriptions while still
* including a NextToken
value.
*
*
* -
*
* The order of snapshots returned in the response of one DescribeSnapshots
call and the order of
* backups returned across the responses of a multi-call iteration is unspecified.
*
*
*
*
* @param describeSnapshotsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeSnapshots operation returned by the service.
* @sample AmazonFSxAsyncHandler.DescribeSnapshots
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeSnapshotsAsync(DescribeSnapshotsRequest describeSnapshotsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes one or more Amazon FSx for NetApp ONTAP storage virtual machines (SVMs).
*
*
* @param describeStorageVirtualMachinesRequest
* @return A Java Future containing the result of the DescribeStorageVirtualMachines operation returned by the
* service.
* @sample AmazonFSxAsync.DescribeStorageVirtualMachines
* @see AWS API Documentation
*/
java.util.concurrent.Future describeStorageVirtualMachinesAsync(
DescribeStorageVirtualMachinesRequest describeStorageVirtualMachinesRequest);
/**
*
* Describes one or more Amazon FSx for NetApp ONTAP storage virtual machines (SVMs).
*
*
* @param describeStorageVirtualMachinesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeStorageVirtualMachines operation returned by the
* service.
* @sample AmazonFSxAsyncHandler.DescribeStorageVirtualMachines
* @see AWS API Documentation
*/
java.util.concurrent.Future describeStorageVirtualMachinesAsync(
DescribeStorageVirtualMachinesRequest describeStorageVirtualMachinesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes one or more Amazon FSx for NetApp ONTAP or Amazon FSx for OpenZFS volumes.
*
*
* @param describeVolumesRequest
* @return A Java Future containing the result of the DescribeVolumes operation returned by the service.
* @sample AmazonFSxAsync.DescribeVolumes
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeVolumesAsync(DescribeVolumesRequest describeVolumesRequest);
/**
*
* Describes one or more Amazon FSx for NetApp ONTAP or Amazon FSx for OpenZFS volumes.
*
*
* @param describeVolumesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeVolumes operation returned by the service.
* @sample AmazonFSxAsyncHandler.DescribeVolumes
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeVolumesAsync(DescribeVolumesRequest describeVolumesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Use this action to disassociate, or remove, one or more Domain Name Service (DNS) aliases from an Amazon FSx for
* Windows File Server file system. If you attempt to disassociate a DNS alias that is not associated with the file
* system, Amazon FSx responds with an HTTP status code 400 (Bad Request). For more information, see Working with DNS
* Aliases.
*
*
* The system generated response showing the DNS aliases that Amazon FSx is attempting to disassociate from the file
* system. Use the API operation to monitor the status of the aliases Amazon FSx is disassociating with the file
* system.
*
*
* @param disassociateFileSystemAliasesRequest
* The request object of DNS aliases to disassociate from an Amazon FSx for Windows File Server file system.
* @return A Java Future containing the result of the DisassociateFileSystemAliases operation returned by the
* service.
* @sample AmazonFSxAsync.DisassociateFileSystemAliases
* @see AWS API Documentation
*/
java.util.concurrent.Future disassociateFileSystemAliasesAsync(
DisassociateFileSystemAliasesRequest disassociateFileSystemAliasesRequest);
/**
*
* Use this action to disassociate, or remove, one or more Domain Name Service (DNS) aliases from an Amazon FSx for
* Windows File Server file system. If you attempt to disassociate a DNS alias that is not associated with the file
* system, Amazon FSx responds with an HTTP status code 400 (Bad Request). For more information, see Working with DNS
* Aliases.
*
*
* The system generated response showing the DNS aliases that Amazon FSx is attempting to disassociate from the file
* system. Use the API operation to monitor the status of the aliases Amazon FSx is disassociating with the file
* system.
*
*
* @param disassociateFileSystemAliasesRequest
* The request object of DNS aliases to disassociate from an Amazon FSx for Windows File Server file system.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DisassociateFileSystemAliases operation returned by the
* service.
* @sample AmazonFSxAsyncHandler.DisassociateFileSystemAliases
* @see AWS API Documentation
*/
java.util.concurrent.Future disassociateFileSystemAliasesAsync(
DisassociateFileSystemAliasesRequest disassociateFileSystemAliasesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists tags for Amazon FSx resources.
*
*
* When retrieving all tags, you can optionally specify the MaxResults
parameter to limit the number of
* tags in a response. If more tags remain, Amazon FSx returns a NextToken
value in the response. In
* this case, send a later request with the NextToken
request parameter set to the value of
* NextToken
from the last response.
*
*
* This action is used in an iterative process to retrieve a list of your tags. ListTagsForResource
is
* called first without a NextToken
value. Then the action continues to be called with the
* NextToken
parameter set to the value of the last NextToken
value until a response has
* no NextToken
.
*
*
* When using this action, keep the following in mind:
*
*
* -
*
* The implementation might return fewer than MaxResults
file system descriptions while still including
* a NextToken
value.
*
*
* -
*
* The order of tags returned in the response of one ListTagsForResource
call and the order of tags
* returned across the responses of a multi-call iteration is unspecified.
*
*
*
*
* @param listTagsForResourceRequest
* The request object for ListTagsForResource
operation.
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AmazonFSxAsync.ListTagsForResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest);
/**
*
* Lists tags for Amazon FSx resources.
*
*
* When retrieving all tags, you can optionally specify the MaxResults
parameter to limit the number of
* tags in a response. If more tags remain, Amazon FSx returns a NextToken
value in the response. In
* this case, send a later request with the NextToken
request parameter set to the value of
* NextToken
from the last response.
*
*
* This action is used in an iterative process to retrieve a list of your tags. ListTagsForResource
is
* called first without a NextToken
value. Then the action continues to be called with the
* NextToken
parameter set to the value of the last NextToken
value until a response has
* no NextToken
.
*
*
* When using this action, keep the following in mind:
*
*
* -
*
* The implementation might return fewer than MaxResults
file system descriptions while still including
* a NextToken
value.
*
*
* -
*
* The order of tags returned in the response of one ListTagsForResource
call and the order of tags
* returned across the responses of a multi-call iteration is unspecified.
*
*
*
*
* @param listTagsForResourceRequest
* The request object for ListTagsForResource
operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AmazonFSxAsyncHandler.ListTagsForResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Releases the file system lock from an Amazon FSx for OpenZFS file system.
*
*
* @param releaseFileSystemNfsV3LocksRequest
* @return A Java Future containing the result of the ReleaseFileSystemNfsV3Locks operation returned by the service.
* @sample AmazonFSxAsync.ReleaseFileSystemNfsV3Locks
* @see AWS API Documentation
*/
java.util.concurrent.Future releaseFileSystemNfsV3LocksAsync(
ReleaseFileSystemNfsV3LocksRequest releaseFileSystemNfsV3LocksRequest);
/**
*
* Releases the file system lock from an Amazon FSx for OpenZFS file system.
*
*
* @param releaseFileSystemNfsV3LocksRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ReleaseFileSystemNfsV3Locks operation returned by the service.
* @sample AmazonFSxAsyncHandler.ReleaseFileSystemNfsV3Locks
* @see AWS API Documentation
*/
java.util.concurrent.Future releaseFileSystemNfsV3LocksAsync(
ReleaseFileSystemNfsV3LocksRequest releaseFileSystemNfsV3LocksRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns an Amazon FSx for OpenZFS volume to the state saved by the specified snapshot.
*
*
* @param restoreVolumeFromSnapshotRequest
* @return A Java Future containing the result of the RestoreVolumeFromSnapshot operation returned by the service.
* @sample AmazonFSxAsync.RestoreVolumeFromSnapshot
* @see AWS
* API Documentation
*/
java.util.concurrent.Future restoreVolumeFromSnapshotAsync(
RestoreVolumeFromSnapshotRequest restoreVolumeFromSnapshotRequest);
/**
*
* Returns an Amazon FSx for OpenZFS volume to the state saved by the specified snapshot.
*
*
* @param restoreVolumeFromSnapshotRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the RestoreVolumeFromSnapshot operation returned by the service.
* @sample AmazonFSxAsyncHandler.RestoreVolumeFromSnapshot
* @see AWS
* API Documentation
*/
java.util.concurrent.Future restoreVolumeFromSnapshotAsync(
RestoreVolumeFromSnapshotRequest restoreVolumeFromSnapshotRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* After performing steps to repair the Active Directory configuration of an FSx for Windows File Server file
* system, use this action to initiate the process of Amazon FSx attempting to reconnect to the file system.
*
*
* @param startMisconfiguredStateRecoveryRequest
* @return A Java Future containing the result of the StartMisconfiguredStateRecovery operation returned by the
* service.
* @sample AmazonFSxAsync.StartMisconfiguredStateRecovery
* @see AWS API Documentation
*/
java.util.concurrent.Future startMisconfiguredStateRecoveryAsync(
StartMisconfiguredStateRecoveryRequest startMisconfiguredStateRecoveryRequest);
/**
*
* After performing steps to repair the Active Directory configuration of an FSx for Windows File Server file
* system, use this action to initiate the process of Amazon FSx attempting to reconnect to the file system.
*
*
* @param startMisconfiguredStateRecoveryRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the StartMisconfiguredStateRecovery operation returned by the
* service.
* @sample AmazonFSxAsyncHandler.StartMisconfiguredStateRecovery
* @see AWS API Documentation
*/
java.util.concurrent.Future startMisconfiguredStateRecoveryAsync(
StartMisconfiguredStateRecoveryRequest startMisconfiguredStateRecoveryRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Tags an Amazon FSx resource.
*
*
* @param tagResourceRequest
* The request object for the TagResource
operation.
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AmazonFSxAsync.TagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest);
/**
*
* Tags an Amazon FSx resource.
*
*
* @param tagResourceRequest
* The request object for the TagResource
operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AmazonFSxAsyncHandler.TagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* This action removes a tag from an Amazon FSx resource.
*
*
* @param untagResourceRequest
* The request object for UntagResource
action.
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AmazonFSxAsync.UntagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest);
/**
*
* This action removes a tag from an Amazon FSx resource.
*
*
* @param untagResourceRequest
* The request object for UntagResource
action.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AmazonFSxAsyncHandler.UntagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates the configuration of an existing data repository association on an Amazon FSx for Lustre file system.
* Data repository associations are supported on all FSx for Lustre 2.12 and 2.15 file systems, excluding
* scratch_1
deployment type.
*
*
* @param updateDataRepositoryAssociationRequest
* @return A Java Future containing the result of the UpdateDataRepositoryAssociation operation returned by the
* service.
* @sample AmazonFSxAsync.UpdateDataRepositoryAssociation
* @see AWS API Documentation
*/
java.util.concurrent.Future updateDataRepositoryAssociationAsync(
UpdateDataRepositoryAssociationRequest updateDataRepositoryAssociationRequest);
/**
*
* Updates the configuration of an existing data repository association on an Amazon FSx for Lustre file system.
* Data repository associations are supported on all FSx for Lustre 2.12 and 2.15 file systems, excluding
* scratch_1
deployment type.
*
*
* @param updateDataRepositoryAssociationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateDataRepositoryAssociation operation returned by the
* service.
* @sample AmazonFSxAsyncHandler.UpdateDataRepositoryAssociation
* @see AWS API Documentation
*/
java.util.concurrent.Future updateDataRepositoryAssociationAsync(
UpdateDataRepositoryAssociationRequest updateDataRepositoryAssociationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates the configuration of an existing Amazon File Cache resource. You can update multiple properties in a
* single request.
*
*
* @param updateFileCacheRequest
* @return A Java Future containing the result of the UpdateFileCache operation returned by the service.
* @sample AmazonFSxAsync.UpdateFileCache
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateFileCacheAsync(UpdateFileCacheRequest updateFileCacheRequest);
/**
*
* Updates the configuration of an existing Amazon File Cache resource. You can update multiple properties in a
* single request.
*
*
* @param updateFileCacheRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateFileCache operation returned by the service.
* @sample AmazonFSxAsyncHandler.UpdateFileCache
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateFileCacheAsync(UpdateFileCacheRequest updateFileCacheRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Use this operation to update the configuration of an existing Amazon FSx file system. You can update multiple
* properties in a single request.
*
*
* For FSx for Windows File Server file systems, you can update the following properties:
*
*
* -
*
* AuditLogConfiguration
*
*
* -
*
* AutomaticBackupRetentionDays
*
*
* -
*
* DailyAutomaticBackupStartTime
*
*
* -
*
* SelfManagedActiveDirectoryConfiguration
*
*
* -
*
* StorageCapacity
*
*
* -
*
* StorageType
*
*
* -
*
* ThroughputCapacity
*
*
* -
*
* DiskIopsConfiguration
*
*
* -
*
* WeeklyMaintenanceStartTime
*
*
*
*
* For FSx for Lustre file systems, you can update the following properties:
*
*
* -
*
* AutoImportPolicy
*
*
* -
*
* AutomaticBackupRetentionDays
*
*
* -
*
* DailyAutomaticBackupStartTime
*
*
* -
*
* DataCompressionType
*
*
* -
*
* LogConfiguration
*
*
* -
*
* LustreRootSquashConfiguration
*
*
* -
*
* MetadataConfiguration
*
*
* -
*
* PerUnitStorageThroughput
*
*
* -
*
* StorageCapacity
*
*
* -
*
* WeeklyMaintenanceStartTime
*
*
*
*
* For FSx for ONTAP file systems, you can update the following properties:
*
*
* -
*
* AddRouteTableIds
*
*
* -
*
* AutomaticBackupRetentionDays
*
*
* -
*
* DailyAutomaticBackupStartTime
*
*
* -
*
* DiskIopsConfiguration
*
*
* -
*
* FsxAdminPassword
*
*
* -
*
* HAPairs
*
*
* -
*
* RemoveRouteTableIds
*
*
* -
*
* StorageCapacity
*
*
* -
*
* ThroughputCapacity
*
*
* -
*
* ThroughputCapacityPerHAPair
*
*
* -
*
* WeeklyMaintenanceStartTime
*
*
*
*
* For FSx for OpenZFS file systems, you can update the following properties:
*
*
* -
*
* AddRouteTableIds
*
*
* -
*
* AutomaticBackupRetentionDays
*
*
* -
*
* CopyTagsToBackups
*
*
* -
*
* CopyTagsToVolumes
*
*
* -
*
* DailyAutomaticBackupStartTime
*
*
* -
*
* DiskIopsConfiguration
*
*
* -
*
* RemoveRouteTableIds
*
*
* -
*
* StorageCapacity
*
*
* -
*
* ThroughputCapacity
*
*
* -
*
* WeeklyMaintenanceStartTime
*
*
*
*
* @param updateFileSystemRequest
* The request object for the UpdateFileSystem
operation.
* @return A Java Future containing the result of the UpdateFileSystem operation returned by the service.
* @sample AmazonFSxAsync.UpdateFileSystem
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateFileSystemAsync(UpdateFileSystemRequest updateFileSystemRequest);
/**
*
* Use this operation to update the configuration of an existing Amazon FSx file system. You can update multiple
* properties in a single request.
*
*
* For FSx for Windows File Server file systems, you can update the following properties:
*
*
* -
*
* AuditLogConfiguration
*
*
* -
*
* AutomaticBackupRetentionDays
*
*
* -
*
* DailyAutomaticBackupStartTime
*
*
* -
*
* SelfManagedActiveDirectoryConfiguration
*
*
* -
*
* StorageCapacity
*
*
* -
*
* StorageType
*
*
* -
*
* ThroughputCapacity
*
*
* -
*
* DiskIopsConfiguration
*
*
* -
*
* WeeklyMaintenanceStartTime
*
*
*
*
* For FSx for Lustre file systems, you can update the following properties:
*
*
* -
*
* AutoImportPolicy
*
*
* -
*
* AutomaticBackupRetentionDays
*
*
* -
*
* DailyAutomaticBackupStartTime
*
*
* -
*
* DataCompressionType
*
*
* -
*
* LogConfiguration
*
*
* -
*
* LustreRootSquashConfiguration
*
*
* -
*
* MetadataConfiguration
*
*
* -
*
* PerUnitStorageThroughput
*
*
* -
*
* StorageCapacity
*
*
* -
*
* WeeklyMaintenanceStartTime
*
*
*
*
* For FSx for ONTAP file systems, you can update the following properties:
*
*
* -
*
* AddRouteTableIds
*
*
* -
*
* AutomaticBackupRetentionDays
*
*
* -
*
* DailyAutomaticBackupStartTime
*
*
* -
*
* DiskIopsConfiguration
*
*
* -
*
* FsxAdminPassword
*
*
* -
*
* HAPairs
*
*
* -
*
* RemoveRouteTableIds
*
*
* -
*
* StorageCapacity
*
*
* -
*
* ThroughputCapacity
*
*
* -
*
* ThroughputCapacityPerHAPair
*
*
* -
*
* WeeklyMaintenanceStartTime
*
*
*
*
* For FSx for OpenZFS file systems, you can update the following properties:
*
*
* -
*
* AddRouteTableIds
*
*
* -
*
* AutomaticBackupRetentionDays
*
*
* -
*
* CopyTagsToBackups
*
*
* -
*
* CopyTagsToVolumes
*
*
* -
*
* DailyAutomaticBackupStartTime
*
*
* -
*
* DiskIopsConfiguration
*
*
* -
*
* RemoveRouteTableIds
*
*
* -
*
* StorageCapacity
*
*
* -
*
* ThroughputCapacity
*
*
* -
*
* WeeklyMaintenanceStartTime
*
*
*
*
* @param updateFileSystemRequest
* The request object for the UpdateFileSystem
operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateFileSystem operation returned by the service.
* @sample AmazonFSxAsyncHandler.UpdateFileSystem
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateFileSystemAsync(UpdateFileSystemRequest updateFileSystemRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Configures whether participant accounts in your organization can create Amazon FSx for NetApp ONTAP Multi-AZ file
* systems in subnets that are shared by a virtual private cloud (VPC) owner. For more information, see the Amazon FSx for NetApp ONTAP User
* Guide.
*
*
*
* We strongly recommend that participant-created Multi-AZ file systems in the shared VPC are deleted before you
* disable this feature. Once the feature is disabled, these file systems will enter a MISCONFIGURED
* state and behave like Single-AZ file systems. For more information, see Important
* considerations before disabling shared VPC support for Multi-AZ file systems.
*
*
*
* @param updateSharedVpcConfigurationRequest
* @return A Java Future containing the result of the UpdateSharedVpcConfiguration operation returned by the
* service.
* @sample AmazonFSxAsync.UpdateSharedVpcConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future updateSharedVpcConfigurationAsync(
UpdateSharedVpcConfigurationRequest updateSharedVpcConfigurationRequest);
/**
*
* Configures whether participant accounts in your organization can create Amazon FSx for NetApp ONTAP Multi-AZ file
* systems in subnets that are shared by a virtual private cloud (VPC) owner. For more information, see the Amazon FSx for NetApp ONTAP User
* Guide.
*
*
*
* We strongly recommend that participant-created Multi-AZ file systems in the shared VPC are deleted before you
* disable this feature. Once the feature is disabled, these file systems will enter a MISCONFIGURED
* state and behave like Single-AZ file systems. For more information, see Important
* considerations before disabling shared VPC support for Multi-AZ file systems.
*
*
*
* @param updateSharedVpcConfigurationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateSharedVpcConfiguration operation returned by the
* service.
* @sample AmazonFSxAsyncHandler.UpdateSharedVpcConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future updateSharedVpcConfigurationAsync(
UpdateSharedVpcConfigurationRequest updateSharedVpcConfigurationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates the name of an Amazon FSx for OpenZFS snapshot.
*
*
* @param updateSnapshotRequest
* @return A Java Future containing the result of the UpdateSnapshot operation returned by the service.
* @sample AmazonFSxAsync.UpdateSnapshot
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateSnapshotAsync(UpdateSnapshotRequest updateSnapshotRequest);
/**
*
* Updates the name of an Amazon FSx for OpenZFS snapshot.
*
*
* @param updateSnapshotRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateSnapshot operation returned by the service.
* @sample AmazonFSxAsyncHandler.UpdateSnapshot
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateSnapshotAsync(UpdateSnapshotRequest updateSnapshotRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates an FSx for ONTAP storage virtual machine (SVM).
*
*
* @param updateStorageVirtualMachineRequest
* @return A Java Future containing the result of the UpdateStorageVirtualMachine operation returned by the service.
* @sample AmazonFSxAsync.UpdateStorageVirtualMachine
* @see AWS API Documentation
*/
java.util.concurrent.Future updateStorageVirtualMachineAsync(
UpdateStorageVirtualMachineRequest updateStorageVirtualMachineRequest);
/**
*
* Updates an FSx for ONTAP storage virtual machine (SVM).
*
*
* @param updateStorageVirtualMachineRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateStorageVirtualMachine operation returned by the service.
* @sample AmazonFSxAsyncHandler.UpdateStorageVirtualMachine
* @see AWS API Documentation
*/
java.util.concurrent.Future updateStorageVirtualMachineAsync(
UpdateStorageVirtualMachineRequest updateStorageVirtualMachineRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates the configuration of an Amazon FSx for NetApp ONTAP or Amazon FSx for OpenZFS volume.
*
*
* @param updateVolumeRequest
* @return A Java Future containing the result of the UpdateVolume operation returned by the service.
* @sample AmazonFSxAsync.UpdateVolume
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateVolumeAsync(UpdateVolumeRequest updateVolumeRequest);
/**
*
* Updates the configuration of an Amazon FSx for NetApp ONTAP or Amazon FSx for OpenZFS volume.
*
*
* @param updateVolumeRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateVolume operation returned by the service.
* @sample AmazonFSxAsyncHandler.UpdateVolume
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateVolumeAsync(UpdateVolumeRequest updateVolumeRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
}