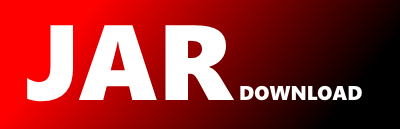
com.amazonaws.services.fsx.model.DataRepositoryTask Maven / Gradle / Ivy
Show all versions of aws-java-sdk-fsx Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.fsx.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* A description of the data repository task.
*
*
* -
*
* You use import and export data repository tasks to perform bulk transfer operations between an Amazon FSx for Lustre
* file system and a linked data repository.
*
*
* -
*
* You use release data repository tasks to release files that have been exported to a linked S3 bucket from your Amazon
* FSx for Lustre file system.
*
*
* -
*
* An Amazon File Cache resource uses a task to automatically release files from the cache.
*
*
*
*
* To learn more about data repository tasks, see Data Repository Tasks.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class DataRepositoryTask implements Serializable, Cloneable, StructuredPojo {
/**
*
* The system-generated, unique 17-digit ID of the data repository task.
*
*/
private String taskId;
/**
*
* The lifecycle status of the data repository task, as follows:
*
*
* -
*
* PENDING
- The task has not started.
*
*
* -
*
* EXECUTING
- The task is in process.
*
*
* -
*
* FAILED
- The task was not able to be completed. For example, there may be files the task failed to
* process. The DataRepositoryTaskFailureDetails property provides more information about task failures.
*
*
* -
*
* SUCCEEDED
- The task has completed successfully.
*
*
* -
*
* CANCELED
- The task was canceled and it did not complete.
*
*
* -
*
* CANCELING
- The task is in process of being canceled.
*
*
*
*
*
* You cannot delete an FSx for Lustre file system if there are data repository tasks for the file system in the
* PENDING
or EXECUTING
states. Please retry when the data repository task is finished
* (with a status of CANCELED
, SUCCEEDED
, or FAILED
). You can use the
* DescribeDataRepositoryTask action to monitor the task status. Contact the FSx team if you need to delete your
* file system immediately.
*
*
*/
private String lifecycle;
/**
*
* The type of data repository task.
*
*
* -
*
* EXPORT_TO_REPOSITORY
tasks export from your Amazon FSx for Lustre file system to a linked data
* repository.
*
*
* -
*
* IMPORT_METADATA_FROM_REPOSITORY
tasks import metadata changes from a linked S3 bucket to your Amazon
* FSx for Lustre file system.
*
*
* -
*
* RELEASE_DATA_FROM_FILESYSTEM
tasks release files in your Amazon FSx for Lustre file system that have
* been exported to a linked S3 bucket and that meet your specified release criteria.
*
*
* -
*
* AUTO_RELEASE_DATA
tasks automatically release files from an Amazon File Cache resource.
*
*
*
*/
private String type;
private java.util.Date creationTime;
/**
*
* The time the system began processing the task.
*
*/
private java.util.Date startTime;
/**
*
* The time the system completed processing the task, populated after the task is complete.
*
*/
private java.util.Date endTime;
private String resourceARN;
private java.util.List tags;
/**
*
* The globally unique ID of the file system.
*
*/
private String fileSystemId;
/**
*
* An array of paths that specify the data for the data repository task to process. For example, in an
* EXPORT_TO_REPOSITORY task, the paths specify which data to export to the linked data repository.
*
*
* (Default) If Paths
is not specified, Amazon FSx uses the file system root directory.
*
*/
private java.util.List paths;
/**
*
* Failure message describing why the task failed, it is populated only when Lifecycle
is set to
* FAILED
.
*
*/
private DataRepositoryTaskFailureDetails failureDetails;
/**
*
* Provides the status of the number of files that the task has processed successfully and failed to process.
*
*/
private DataRepositoryTaskStatus status;
private CompletionReport report;
/**
*
* Specifies the amount of data to release, in GiB, by an Amazon File Cache AUTO_RELEASE_DATA task that
* automatically releases files from the cache.
*
*/
private Long capacityToRelease;
/**
*
* The system-generated, unique ID of the cache.
*
*/
private String fileCacheId;
/**
*
* The configuration that specifies the last accessed time criteria for files that will be released from an Amazon
* FSx for Lustre file system.
*
*/
private ReleaseConfiguration releaseConfiguration;
/**
*
* The system-generated, unique 17-digit ID of the data repository task.
*
*
* @param taskId
* The system-generated, unique 17-digit ID of the data repository task.
*/
public void setTaskId(String taskId) {
this.taskId = taskId;
}
/**
*
* The system-generated, unique 17-digit ID of the data repository task.
*
*
* @return The system-generated, unique 17-digit ID of the data repository task.
*/
public String getTaskId() {
return this.taskId;
}
/**
*
* The system-generated, unique 17-digit ID of the data repository task.
*
*
* @param taskId
* The system-generated, unique 17-digit ID of the data repository task.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DataRepositoryTask withTaskId(String taskId) {
setTaskId(taskId);
return this;
}
/**
*
* The lifecycle status of the data repository task, as follows:
*
*
* -
*
* PENDING
- The task has not started.
*
*
* -
*
* EXECUTING
- The task is in process.
*
*
* -
*
* FAILED
- The task was not able to be completed. For example, there may be files the task failed to
* process. The DataRepositoryTaskFailureDetails property provides more information about task failures.
*
*
* -
*
* SUCCEEDED
- The task has completed successfully.
*
*
* -
*
* CANCELED
- The task was canceled and it did not complete.
*
*
* -
*
* CANCELING
- The task is in process of being canceled.
*
*
*
*
*
* You cannot delete an FSx for Lustre file system if there are data repository tasks for the file system in the
* PENDING
or EXECUTING
states. Please retry when the data repository task is finished
* (with a status of CANCELED
, SUCCEEDED
, or FAILED
). You can use the
* DescribeDataRepositoryTask action to monitor the task status. Contact the FSx team if you need to delete your
* file system immediately.
*
*
*
* @param lifecycle
* The lifecycle status of the data repository task, as follows:
*
* -
*
* PENDING
- The task has not started.
*
*
* -
*
* EXECUTING
- The task is in process.
*
*
* -
*
* FAILED
- The task was not able to be completed. For example, there may be files the task
* failed to process. The DataRepositoryTaskFailureDetails property provides more information about
* task failures.
*
*
* -
*
* SUCCEEDED
- The task has completed successfully.
*
*
* -
*
* CANCELED
- The task was canceled and it did not complete.
*
*
* -
*
* CANCELING
- The task is in process of being canceled.
*
*
*
*
*
* You cannot delete an FSx for Lustre file system if there are data repository tasks for the file system in
* the PENDING
or EXECUTING
states. Please retry when the data repository task is
* finished (with a status of CANCELED
, SUCCEEDED
, or FAILED
). You can
* use the DescribeDataRepositoryTask action to monitor the task status. Contact the FSx team if you need to
* delete your file system immediately.
*
* @see DataRepositoryTaskLifecycle
*/
public void setLifecycle(String lifecycle) {
this.lifecycle = lifecycle;
}
/**
*
* The lifecycle status of the data repository task, as follows:
*
*
* -
*
* PENDING
- The task has not started.
*
*
* -
*
* EXECUTING
- The task is in process.
*
*
* -
*
* FAILED
- The task was not able to be completed. For example, there may be files the task failed to
* process. The DataRepositoryTaskFailureDetails property provides more information about task failures.
*
*
* -
*
* SUCCEEDED
- The task has completed successfully.
*
*
* -
*
* CANCELED
- The task was canceled and it did not complete.
*
*
* -
*
* CANCELING
- The task is in process of being canceled.
*
*
*
*
*
* You cannot delete an FSx for Lustre file system if there are data repository tasks for the file system in the
* PENDING
or EXECUTING
states. Please retry when the data repository task is finished
* (with a status of CANCELED
, SUCCEEDED
, or FAILED
). You can use the
* DescribeDataRepositoryTask action to monitor the task status. Contact the FSx team if you need to delete your
* file system immediately.
*
*
*
* @return The lifecycle status of the data repository task, as follows:
*
* -
*
* PENDING
- The task has not started.
*
*
* -
*
* EXECUTING
- The task is in process.
*
*
* -
*
* FAILED
- The task was not able to be completed. For example, there may be files the task
* failed to process. The DataRepositoryTaskFailureDetails property provides more information about
* task failures.
*
*
* -
*
* SUCCEEDED
- The task has completed successfully.
*
*
* -
*
* CANCELED
- The task was canceled and it did not complete.
*
*
* -
*
* CANCELING
- The task is in process of being canceled.
*
*
*
*
*
* You cannot delete an FSx for Lustre file system if there are data repository tasks for the file system in
* the PENDING
or EXECUTING
states. Please retry when the data repository task is
* finished (with a status of CANCELED
, SUCCEEDED
, or FAILED
). You
* can use the DescribeDataRepositoryTask action to monitor the task status. Contact the FSx team if you
* need to delete your file system immediately.
*
* @see DataRepositoryTaskLifecycle
*/
public String getLifecycle() {
return this.lifecycle;
}
/**
*
* The lifecycle status of the data repository task, as follows:
*
*
* -
*
* PENDING
- The task has not started.
*
*
* -
*
* EXECUTING
- The task is in process.
*
*
* -
*
* FAILED
- The task was not able to be completed. For example, there may be files the task failed to
* process. The DataRepositoryTaskFailureDetails property provides more information about task failures.
*
*
* -
*
* SUCCEEDED
- The task has completed successfully.
*
*
* -
*
* CANCELED
- The task was canceled and it did not complete.
*
*
* -
*
* CANCELING
- The task is in process of being canceled.
*
*
*
*
*
* You cannot delete an FSx for Lustre file system if there are data repository tasks for the file system in the
* PENDING
or EXECUTING
states. Please retry when the data repository task is finished
* (with a status of CANCELED
, SUCCEEDED
, or FAILED
). You can use the
* DescribeDataRepositoryTask action to monitor the task status. Contact the FSx team if you need to delete your
* file system immediately.
*
*
*
* @param lifecycle
* The lifecycle status of the data repository task, as follows:
*
* -
*
* PENDING
- The task has not started.
*
*
* -
*
* EXECUTING
- The task is in process.
*
*
* -
*
* FAILED
- The task was not able to be completed. For example, there may be files the task
* failed to process. The DataRepositoryTaskFailureDetails property provides more information about
* task failures.
*
*
* -
*
* SUCCEEDED
- The task has completed successfully.
*
*
* -
*
* CANCELED
- The task was canceled and it did not complete.
*
*
* -
*
* CANCELING
- The task is in process of being canceled.
*
*
*
*
*
* You cannot delete an FSx for Lustre file system if there are data repository tasks for the file system in
* the PENDING
or EXECUTING
states. Please retry when the data repository task is
* finished (with a status of CANCELED
, SUCCEEDED
, or FAILED
). You can
* use the DescribeDataRepositoryTask action to monitor the task status. Contact the FSx team if you need to
* delete your file system immediately.
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see DataRepositoryTaskLifecycle
*/
public DataRepositoryTask withLifecycle(String lifecycle) {
setLifecycle(lifecycle);
return this;
}
/**
*
* The lifecycle status of the data repository task, as follows:
*
*
* -
*
* PENDING
- The task has not started.
*
*
* -
*
* EXECUTING
- The task is in process.
*
*
* -
*
* FAILED
- The task was not able to be completed. For example, there may be files the task failed to
* process. The DataRepositoryTaskFailureDetails property provides more information about task failures.
*
*
* -
*
* SUCCEEDED
- The task has completed successfully.
*
*
* -
*
* CANCELED
- The task was canceled and it did not complete.
*
*
* -
*
* CANCELING
- The task is in process of being canceled.
*
*
*
*
*
* You cannot delete an FSx for Lustre file system if there are data repository tasks for the file system in the
* PENDING
or EXECUTING
states. Please retry when the data repository task is finished
* (with a status of CANCELED
, SUCCEEDED
, or FAILED
). You can use the
* DescribeDataRepositoryTask action to monitor the task status. Contact the FSx team if you need to delete your
* file system immediately.
*
*
*
* @param lifecycle
* The lifecycle status of the data repository task, as follows:
*
* -
*
* PENDING
- The task has not started.
*
*
* -
*
* EXECUTING
- The task is in process.
*
*
* -
*
* FAILED
- The task was not able to be completed. For example, there may be files the task
* failed to process. The DataRepositoryTaskFailureDetails property provides more information about
* task failures.
*
*
* -
*
* SUCCEEDED
- The task has completed successfully.
*
*
* -
*
* CANCELED
- The task was canceled and it did not complete.
*
*
* -
*
* CANCELING
- The task is in process of being canceled.
*
*
*
*
*
* You cannot delete an FSx for Lustre file system if there are data repository tasks for the file system in
* the PENDING
or EXECUTING
states. Please retry when the data repository task is
* finished (with a status of CANCELED
, SUCCEEDED
, or FAILED
). You can
* use the DescribeDataRepositoryTask action to monitor the task status. Contact the FSx team if you need to
* delete your file system immediately.
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see DataRepositoryTaskLifecycle
*/
public DataRepositoryTask withLifecycle(DataRepositoryTaskLifecycle lifecycle) {
this.lifecycle = lifecycle.toString();
return this;
}
/**
*
* The type of data repository task.
*
*
* -
*
* EXPORT_TO_REPOSITORY
tasks export from your Amazon FSx for Lustre file system to a linked data
* repository.
*
*
* -
*
* IMPORT_METADATA_FROM_REPOSITORY
tasks import metadata changes from a linked S3 bucket to your Amazon
* FSx for Lustre file system.
*
*
* -
*
* RELEASE_DATA_FROM_FILESYSTEM
tasks release files in your Amazon FSx for Lustre file system that have
* been exported to a linked S3 bucket and that meet your specified release criteria.
*
*
* -
*
* AUTO_RELEASE_DATA
tasks automatically release files from an Amazon File Cache resource.
*
*
*
*
* @param type
* The type of data repository task.
*
* -
*
* EXPORT_TO_REPOSITORY
tasks export from your Amazon FSx for Lustre file system to a linked
* data repository.
*
*
* -
*
* IMPORT_METADATA_FROM_REPOSITORY
tasks import metadata changes from a linked S3 bucket to your
* Amazon FSx for Lustre file system.
*
*
* -
*
* RELEASE_DATA_FROM_FILESYSTEM
tasks release files in your Amazon FSx for Lustre file system
* that have been exported to a linked S3 bucket and that meet your specified release criteria.
*
*
* -
*
* AUTO_RELEASE_DATA
tasks automatically release files from an Amazon File Cache resource.
*
*
* @see DataRepositoryTaskType
*/
public void setType(String type) {
this.type = type;
}
/**
*
* The type of data repository task.
*
*
* -
*
* EXPORT_TO_REPOSITORY
tasks export from your Amazon FSx for Lustre file system to a linked data
* repository.
*
*
* -
*
* IMPORT_METADATA_FROM_REPOSITORY
tasks import metadata changes from a linked S3 bucket to your Amazon
* FSx for Lustre file system.
*
*
* -
*
* RELEASE_DATA_FROM_FILESYSTEM
tasks release files in your Amazon FSx for Lustre file system that have
* been exported to a linked S3 bucket and that meet your specified release criteria.
*
*
* -
*
* AUTO_RELEASE_DATA
tasks automatically release files from an Amazon File Cache resource.
*
*
*
*
* @return The type of data repository task.
*
* -
*
* EXPORT_TO_REPOSITORY
tasks export from your Amazon FSx for Lustre file system to a linked
* data repository.
*
*
* -
*
* IMPORT_METADATA_FROM_REPOSITORY
tasks import metadata changes from a linked S3 bucket to
* your Amazon FSx for Lustre file system.
*
*
* -
*
* RELEASE_DATA_FROM_FILESYSTEM
tasks release files in your Amazon FSx for Lustre file system
* that have been exported to a linked S3 bucket and that meet your specified release criteria.
*
*
* -
*
* AUTO_RELEASE_DATA
tasks automatically release files from an Amazon File Cache resource.
*
*
* @see DataRepositoryTaskType
*/
public String getType() {
return this.type;
}
/**
*
* The type of data repository task.
*
*
* -
*
* EXPORT_TO_REPOSITORY
tasks export from your Amazon FSx for Lustre file system to a linked data
* repository.
*
*
* -
*
* IMPORT_METADATA_FROM_REPOSITORY
tasks import metadata changes from a linked S3 bucket to your Amazon
* FSx for Lustre file system.
*
*
* -
*
* RELEASE_DATA_FROM_FILESYSTEM
tasks release files in your Amazon FSx for Lustre file system that have
* been exported to a linked S3 bucket and that meet your specified release criteria.
*
*
* -
*
* AUTO_RELEASE_DATA
tasks automatically release files from an Amazon File Cache resource.
*
*
*
*
* @param type
* The type of data repository task.
*
* -
*
* EXPORT_TO_REPOSITORY
tasks export from your Amazon FSx for Lustre file system to a linked
* data repository.
*
*
* -
*
* IMPORT_METADATA_FROM_REPOSITORY
tasks import metadata changes from a linked S3 bucket to your
* Amazon FSx for Lustre file system.
*
*
* -
*
* RELEASE_DATA_FROM_FILESYSTEM
tasks release files in your Amazon FSx for Lustre file system
* that have been exported to a linked S3 bucket and that meet your specified release criteria.
*
*
* -
*
* AUTO_RELEASE_DATA
tasks automatically release files from an Amazon File Cache resource.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see DataRepositoryTaskType
*/
public DataRepositoryTask withType(String type) {
setType(type);
return this;
}
/**
*
* The type of data repository task.
*
*
* -
*
* EXPORT_TO_REPOSITORY
tasks export from your Amazon FSx for Lustre file system to a linked data
* repository.
*
*
* -
*
* IMPORT_METADATA_FROM_REPOSITORY
tasks import metadata changes from a linked S3 bucket to your Amazon
* FSx for Lustre file system.
*
*
* -
*
* RELEASE_DATA_FROM_FILESYSTEM
tasks release files in your Amazon FSx for Lustre file system that have
* been exported to a linked S3 bucket and that meet your specified release criteria.
*
*
* -
*
* AUTO_RELEASE_DATA
tasks automatically release files from an Amazon File Cache resource.
*
*
*
*
* @param type
* The type of data repository task.
*
* -
*
* EXPORT_TO_REPOSITORY
tasks export from your Amazon FSx for Lustre file system to a linked
* data repository.
*
*
* -
*
* IMPORT_METADATA_FROM_REPOSITORY
tasks import metadata changes from a linked S3 bucket to your
* Amazon FSx for Lustre file system.
*
*
* -
*
* RELEASE_DATA_FROM_FILESYSTEM
tasks release files in your Amazon FSx for Lustre file system
* that have been exported to a linked S3 bucket and that meet your specified release criteria.
*
*
* -
*
* AUTO_RELEASE_DATA
tasks automatically release files from an Amazon File Cache resource.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see DataRepositoryTaskType
*/
public DataRepositoryTask withType(DataRepositoryTaskType type) {
this.type = type.toString();
return this;
}
/**
* @param creationTime
*/
public void setCreationTime(java.util.Date creationTime) {
this.creationTime = creationTime;
}
/**
* @return
*/
public java.util.Date getCreationTime() {
return this.creationTime;
}
/**
* @param creationTime
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DataRepositoryTask withCreationTime(java.util.Date creationTime) {
setCreationTime(creationTime);
return this;
}
/**
*
* The time the system began processing the task.
*
*
* @param startTime
* The time the system began processing the task.
*/
public void setStartTime(java.util.Date startTime) {
this.startTime = startTime;
}
/**
*
* The time the system began processing the task.
*
*
* @return The time the system began processing the task.
*/
public java.util.Date getStartTime() {
return this.startTime;
}
/**
*
* The time the system began processing the task.
*
*
* @param startTime
* The time the system began processing the task.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DataRepositoryTask withStartTime(java.util.Date startTime) {
setStartTime(startTime);
return this;
}
/**
*
* The time the system completed processing the task, populated after the task is complete.
*
*
* @param endTime
* The time the system completed processing the task, populated after the task is complete.
*/
public void setEndTime(java.util.Date endTime) {
this.endTime = endTime;
}
/**
*
* The time the system completed processing the task, populated after the task is complete.
*
*
* @return The time the system completed processing the task, populated after the task is complete.
*/
public java.util.Date getEndTime() {
return this.endTime;
}
/**
*
* The time the system completed processing the task, populated after the task is complete.
*
*
* @param endTime
* The time the system completed processing the task, populated after the task is complete.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DataRepositoryTask withEndTime(java.util.Date endTime) {
setEndTime(endTime);
return this;
}
/**
* @param resourceARN
*/
public void setResourceARN(String resourceARN) {
this.resourceARN = resourceARN;
}
/**
* @return
*/
public String getResourceARN() {
return this.resourceARN;
}
/**
* @param resourceARN
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DataRepositoryTask withResourceARN(String resourceARN) {
setResourceARN(resourceARN);
return this;
}
/**
* @return
*/
public java.util.List getTags() {
return tags;
}
/**
* @param tags
*/
public void setTags(java.util.Collection tags) {
if (tags == null) {
this.tags = null;
return;
}
this.tags = new java.util.ArrayList(tags);
}
/**
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTags(java.util.Collection)} or {@link #withTags(java.util.Collection)} if you want to override the
* existing values.
*
*
* @param tags
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DataRepositoryTask withTags(Tag... tags) {
if (this.tags == null) {
setTags(new java.util.ArrayList(tags.length));
}
for (Tag ele : tags) {
this.tags.add(ele);
}
return this;
}
/**
* @param tags
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DataRepositoryTask withTags(java.util.Collection tags) {
setTags(tags);
return this;
}
/**
*
* The globally unique ID of the file system.
*
*
* @param fileSystemId
* The globally unique ID of the file system.
*/
public void setFileSystemId(String fileSystemId) {
this.fileSystemId = fileSystemId;
}
/**
*
* The globally unique ID of the file system.
*
*
* @return The globally unique ID of the file system.
*/
public String getFileSystemId() {
return this.fileSystemId;
}
/**
*
* The globally unique ID of the file system.
*
*
* @param fileSystemId
* The globally unique ID of the file system.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DataRepositoryTask withFileSystemId(String fileSystemId) {
setFileSystemId(fileSystemId);
return this;
}
/**
*
* An array of paths that specify the data for the data repository task to process. For example, in an
* EXPORT_TO_REPOSITORY task, the paths specify which data to export to the linked data repository.
*
*
* (Default) If Paths
is not specified, Amazon FSx uses the file system root directory.
*
*
* @return An array of paths that specify the data for the data repository task to process. For example, in an
* EXPORT_TO_REPOSITORY task, the paths specify which data to export to the linked data repository.
*
* (Default) If Paths
is not specified, Amazon FSx uses the file system root directory.
*/
public java.util.List getPaths() {
return paths;
}
/**
*
* An array of paths that specify the data for the data repository task to process. For example, in an
* EXPORT_TO_REPOSITORY task, the paths specify which data to export to the linked data repository.
*
*
* (Default) If Paths
is not specified, Amazon FSx uses the file system root directory.
*
*
* @param paths
* An array of paths that specify the data for the data repository task to process. For example, in an
* EXPORT_TO_REPOSITORY task, the paths specify which data to export to the linked data repository.
*
* (Default) If Paths
is not specified, Amazon FSx uses the file system root directory.
*/
public void setPaths(java.util.Collection paths) {
if (paths == null) {
this.paths = null;
return;
}
this.paths = new java.util.ArrayList(paths);
}
/**
*
* An array of paths that specify the data for the data repository task to process. For example, in an
* EXPORT_TO_REPOSITORY task, the paths specify which data to export to the linked data repository.
*
*
* (Default) If Paths
is not specified, Amazon FSx uses the file system root directory.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setPaths(java.util.Collection)} or {@link #withPaths(java.util.Collection)} if you want to override the
* existing values.
*
*
* @param paths
* An array of paths that specify the data for the data repository task to process. For example, in an
* EXPORT_TO_REPOSITORY task, the paths specify which data to export to the linked data repository.
*
* (Default) If Paths
is not specified, Amazon FSx uses the file system root directory.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DataRepositoryTask withPaths(String... paths) {
if (this.paths == null) {
setPaths(new java.util.ArrayList(paths.length));
}
for (String ele : paths) {
this.paths.add(ele);
}
return this;
}
/**
*
* An array of paths that specify the data for the data repository task to process. For example, in an
* EXPORT_TO_REPOSITORY task, the paths specify which data to export to the linked data repository.
*
*
* (Default) If Paths
is not specified, Amazon FSx uses the file system root directory.
*
*
* @param paths
* An array of paths that specify the data for the data repository task to process. For example, in an
* EXPORT_TO_REPOSITORY task, the paths specify which data to export to the linked data repository.
*
* (Default) If Paths
is not specified, Amazon FSx uses the file system root directory.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DataRepositoryTask withPaths(java.util.Collection paths) {
setPaths(paths);
return this;
}
/**
*
* Failure message describing why the task failed, it is populated only when Lifecycle
is set to
* FAILED
.
*
*
* @param failureDetails
* Failure message describing why the task failed, it is populated only when Lifecycle
is set to
* FAILED
.
*/
public void setFailureDetails(DataRepositoryTaskFailureDetails failureDetails) {
this.failureDetails = failureDetails;
}
/**
*
* Failure message describing why the task failed, it is populated only when Lifecycle
is set to
* FAILED
.
*
*
* @return Failure message describing why the task failed, it is populated only when Lifecycle
is set
* to FAILED
.
*/
public DataRepositoryTaskFailureDetails getFailureDetails() {
return this.failureDetails;
}
/**
*
* Failure message describing why the task failed, it is populated only when Lifecycle
is set to
* FAILED
.
*
*
* @param failureDetails
* Failure message describing why the task failed, it is populated only when Lifecycle
is set to
* FAILED
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DataRepositoryTask withFailureDetails(DataRepositoryTaskFailureDetails failureDetails) {
setFailureDetails(failureDetails);
return this;
}
/**
*
* Provides the status of the number of files that the task has processed successfully and failed to process.
*
*
* @param status
* Provides the status of the number of files that the task has processed successfully and failed to process.
*/
public void setStatus(DataRepositoryTaskStatus status) {
this.status = status;
}
/**
*
* Provides the status of the number of files that the task has processed successfully and failed to process.
*
*
* @return Provides the status of the number of files that the task has processed successfully and failed to
* process.
*/
public DataRepositoryTaskStatus getStatus() {
return this.status;
}
/**
*
* Provides the status of the number of files that the task has processed successfully and failed to process.
*
*
* @param status
* Provides the status of the number of files that the task has processed successfully and failed to process.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DataRepositoryTask withStatus(DataRepositoryTaskStatus status) {
setStatus(status);
return this;
}
/**
* @param report
*/
public void setReport(CompletionReport report) {
this.report = report;
}
/**
* @return
*/
public CompletionReport getReport() {
return this.report;
}
/**
* @param report
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DataRepositoryTask withReport(CompletionReport report) {
setReport(report);
return this;
}
/**
*
* Specifies the amount of data to release, in GiB, by an Amazon File Cache AUTO_RELEASE_DATA task that
* automatically releases files from the cache.
*
*
* @param capacityToRelease
* Specifies the amount of data to release, in GiB, by an Amazon File Cache AUTO_RELEASE_DATA task that
* automatically releases files from the cache.
*/
public void setCapacityToRelease(Long capacityToRelease) {
this.capacityToRelease = capacityToRelease;
}
/**
*
* Specifies the amount of data to release, in GiB, by an Amazon File Cache AUTO_RELEASE_DATA task that
* automatically releases files from the cache.
*
*
* @return Specifies the amount of data to release, in GiB, by an Amazon File Cache AUTO_RELEASE_DATA task that
* automatically releases files from the cache.
*/
public Long getCapacityToRelease() {
return this.capacityToRelease;
}
/**
*
* Specifies the amount of data to release, in GiB, by an Amazon File Cache AUTO_RELEASE_DATA task that
* automatically releases files from the cache.
*
*
* @param capacityToRelease
* Specifies the amount of data to release, in GiB, by an Amazon File Cache AUTO_RELEASE_DATA task that
* automatically releases files from the cache.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DataRepositoryTask withCapacityToRelease(Long capacityToRelease) {
setCapacityToRelease(capacityToRelease);
return this;
}
/**
*
* The system-generated, unique ID of the cache.
*
*
* @param fileCacheId
* The system-generated, unique ID of the cache.
*/
public void setFileCacheId(String fileCacheId) {
this.fileCacheId = fileCacheId;
}
/**
*
* The system-generated, unique ID of the cache.
*
*
* @return The system-generated, unique ID of the cache.
*/
public String getFileCacheId() {
return this.fileCacheId;
}
/**
*
* The system-generated, unique ID of the cache.
*
*
* @param fileCacheId
* The system-generated, unique ID of the cache.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DataRepositoryTask withFileCacheId(String fileCacheId) {
setFileCacheId(fileCacheId);
return this;
}
/**
*
* The configuration that specifies the last accessed time criteria for files that will be released from an Amazon
* FSx for Lustre file system.
*
*
* @param releaseConfiguration
* The configuration that specifies the last accessed time criteria for files that will be released from an
* Amazon FSx for Lustre file system.
*/
public void setReleaseConfiguration(ReleaseConfiguration releaseConfiguration) {
this.releaseConfiguration = releaseConfiguration;
}
/**
*
* The configuration that specifies the last accessed time criteria for files that will be released from an Amazon
* FSx for Lustre file system.
*
*
* @return The configuration that specifies the last accessed time criteria for files that will be released from an
* Amazon FSx for Lustre file system.
*/
public ReleaseConfiguration getReleaseConfiguration() {
return this.releaseConfiguration;
}
/**
*
* The configuration that specifies the last accessed time criteria for files that will be released from an Amazon
* FSx for Lustre file system.
*
*
* @param releaseConfiguration
* The configuration that specifies the last accessed time criteria for files that will be released from an
* Amazon FSx for Lustre file system.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DataRepositoryTask withReleaseConfiguration(ReleaseConfiguration releaseConfiguration) {
setReleaseConfiguration(releaseConfiguration);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getTaskId() != null)
sb.append("TaskId: ").append(getTaskId()).append(",");
if (getLifecycle() != null)
sb.append("Lifecycle: ").append(getLifecycle()).append(",");
if (getType() != null)
sb.append("Type: ").append(getType()).append(",");
if (getCreationTime() != null)
sb.append("CreationTime: ").append(getCreationTime()).append(",");
if (getStartTime() != null)
sb.append("StartTime: ").append(getStartTime()).append(",");
if (getEndTime() != null)
sb.append("EndTime: ").append(getEndTime()).append(",");
if (getResourceARN() != null)
sb.append("ResourceARN: ").append(getResourceARN()).append(",");
if (getTags() != null)
sb.append("Tags: ").append(getTags()).append(",");
if (getFileSystemId() != null)
sb.append("FileSystemId: ").append(getFileSystemId()).append(",");
if (getPaths() != null)
sb.append("Paths: ").append(getPaths()).append(",");
if (getFailureDetails() != null)
sb.append("FailureDetails: ").append(getFailureDetails()).append(",");
if (getStatus() != null)
sb.append("Status: ").append(getStatus()).append(",");
if (getReport() != null)
sb.append("Report: ").append(getReport()).append(",");
if (getCapacityToRelease() != null)
sb.append("CapacityToRelease: ").append(getCapacityToRelease()).append(",");
if (getFileCacheId() != null)
sb.append("FileCacheId: ").append(getFileCacheId()).append(",");
if (getReleaseConfiguration() != null)
sb.append("ReleaseConfiguration: ").append(getReleaseConfiguration());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof DataRepositoryTask == false)
return false;
DataRepositoryTask other = (DataRepositoryTask) obj;
if (other.getTaskId() == null ^ this.getTaskId() == null)
return false;
if (other.getTaskId() != null && other.getTaskId().equals(this.getTaskId()) == false)
return false;
if (other.getLifecycle() == null ^ this.getLifecycle() == null)
return false;
if (other.getLifecycle() != null && other.getLifecycle().equals(this.getLifecycle()) == false)
return false;
if (other.getType() == null ^ this.getType() == null)
return false;
if (other.getType() != null && other.getType().equals(this.getType()) == false)
return false;
if (other.getCreationTime() == null ^ this.getCreationTime() == null)
return false;
if (other.getCreationTime() != null && other.getCreationTime().equals(this.getCreationTime()) == false)
return false;
if (other.getStartTime() == null ^ this.getStartTime() == null)
return false;
if (other.getStartTime() != null && other.getStartTime().equals(this.getStartTime()) == false)
return false;
if (other.getEndTime() == null ^ this.getEndTime() == null)
return false;
if (other.getEndTime() != null && other.getEndTime().equals(this.getEndTime()) == false)
return false;
if (other.getResourceARN() == null ^ this.getResourceARN() == null)
return false;
if (other.getResourceARN() != null && other.getResourceARN().equals(this.getResourceARN()) == false)
return false;
if (other.getTags() == null ^ this.getTags() == null)
return false;
if (other.getTags() != null && other.getTags().equals(this.getTags()) == false)
return false;
if (other.getFileSystemId() == null ^ this.getFileSystemId() == null)
return false;
if (other.getFileSystemId() != null && other.getFileSystemId().equals(this.getFileSystemId()) == false)
return false;
if (other.getPaths() == null ^ this.getPaths() == null)
return false;
if (other.getPaths() != null && other.getPaths().equals(this.getPaths()) == false)
return false;
if (other.getFailureDetails() == null ^ this.getFailureDetails() == null)
return false;
if (other.getFailureDetails() != null && other.getFailureDetails().equals(this.getFailureDetails()) == false)
return false;
if (other.getStatus() == null ^ this.getStatus() == null)
return false;
if (other.getStatus() != null && other.getStatus().equals(this.getStatus()) == false)
return false;
if (other.getReport() == null ^ this.getReport() == null)
return false;
if (other.getReport() != null && other.getReport().equals(this.getReport()) == false)
return false;
if (other.getCapacityToRelease() == null ^ this.getCapacityToRelease() == null)
return false;
if (other.getCapacityToRelease() != null && other.getCapacityToRelease().equals(this.getCapacityToRelease()) == false)
return false;
if (other.getFileCacheId() == null ^ this.getFileCacheId() == null)
return false;
if (other.getFileCacheId() != null && other.getFileCacheId().equals(this.getFileCacheId()) == false)
return false;
if (other.getReleaseConfiguration() == null ^ this.getReleaseConfiguration() == null)
return false;
if (other.getReleaseConfiguration() != null && other.getReleaseConfiguration().equals(this.getReleaseConfiguration()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getTaskId() == null) ? 0 : getTaskId().hashCode());
hashCode = prime * hashCode + ((getLifecycle() == null) ? 0 : getLifecycle().hashCode());
hashCode = prime * hashCode + ((getType() == null) ? 0 : getType().hashCode());
hashCode = prime * hashCode + ((getCreationTime() == null) ? 0 : getCreationTime().hashCode());
hashCode = prime * hashCode + ((getStartTime() == null) ? 0 : getStartTime().hashCode());
hashCode = prime * hashCode + ((getEndTime() == null) ? 0 : getEndTime().hashCode());
hashCode = prime * hashCode + ((getResourceARN() == null) ? 0 : getResourceARN().hashCode());
hashCode = prime * hashCode + ((getTags() == null) ? 0 : getTags().hashCode());
hashCode = prime * hashCode + ((getFileSystemId() == null) ? 0 : getFileSystemId().hashCode());
hashCode = prime * hashCode + ((getPaths() == null) ? 0 : getPaths().hashCode());
hashCode = prime * hashCode + ((getFailureDetails() == null) ? 0 : getFailureDetails().hashCode());
hashCode = prime * hashCode + ((getStatus() == null) ? 0 : getStatus().hashCode());
hashCode = prime * hashCode + ((getReport() == null) ? 0 : getReport().hashCode());
hashCode = prime * hashCode + ((getCapacityToRelease() == null) ? 0 : getCapacityToRelease().hashCode());
hashCode = prime * hashCode + ((getFileCacheId() == null) ? 0 : getFileCacheId().hashCode());
hashCode = prime * hashCode + ((getReleaseConfiguration() == null) ? 0 : getReleaseConfiguration().hashCode());
return hashCode;
}
@Override
public DataRepositoryTask clone() {
try {
return (DataRepositoryTask) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.fsx.model.transform.DataRepositoryTaskMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}