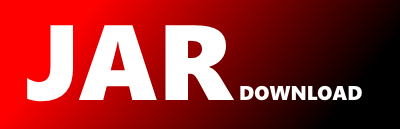
com.amazonaws.services.fsx.model.UpdateFileSystemRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-fsx Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.fsx.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* The request object for the UpdateFileSystem
operation.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class UpdateFileSystemRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The ID of the file system that you are updating.
*
*/
private String fileSystemId;
/**
*
* A string of up to 63 ASCII characters that Amazon FSx uses to ensure idempotent updates. This string is
* automatically filled on your behalf when you use the Command Line Interface (CLI) or an Amazon Web Services SDK.
*
*/
private String clientRequestToken;
/**
*
* Use this parameter to increase the storage capacity of an FSx for Windows File Server, FSx for Lustre, FSx for
* OpenZFS, or FSx for ONTAP file system. Specifies the storage capacity target value, in GiB, to increase the
* storage capacity for the file system that you're updating.
*
*
*
* You can't make a storage capacity increase request if there is an existing storage capacity increase request in
* progress.
*
*
*
* For Lustre file systems, the storage capacity target value can be the following:
*
*
* -
*
* For SCRATCH_2
, PERSISTENT_1
, and PERSISTENT_2 SSD
deployment types, valid
* values are in multiples of 2400 GiB. The value must be greater than the current storage capacity.
*
*
* -
*
* For PERSISTENT HDD
file systems, valid values are multiples of 6000 GiB for 12-MBps throughput per
* TiB file systems and multiples of 1800 GiB for 40-MBps throughput per TiB file systems. The values must be
* greater than the current storage capacity.
*
*
* -
*
* For SCRATCH_1
file systems, you can't increase the storage capacity.
*
*
*
*
* For more information, see Managing storage and
* throughput capacity in the FSx for Lustre User Guide.
*
*
* For FSx for OpenZFS file systems, the storage capacity target value must be at least 10 percent greater than the
* current storage capacity value. For more information, see Managing storage
* capacity in the FSx for OpenZFS User Guide.
*
*
* For Windows file systems, the storage capacity target value must be at least 10 percent greater than the current
* storage capacity value. To increase storage capacity, the file system must have at least 16 MBps of throughput
* capacity. For more information, see Managing storage
* capacity in the Amazon FSxfor Windows File Server User Guide.
*
*
* For ONTAP file systems, the storage capacity target value must be at least 10 percent greater than the current
* storage capacity value. For more information, see Managing storage capacity
* and provisioned IOPS in the Amazon FSx for NetApp ONTAP User Guide.
*
*/
private Integer storageCapacity;
/**
*
* The configuration updates for an Amazon FSx for Windows File Server file system.
*
*/
private UpdateFileSystemWindowsConfiguration windowsConfiguration;
private UpdateFileSystemLustreConfiguration lustreConfiguration;
private UpdateFileSystemOntapConfiguration ontapConfiguration;
/**
*
* The configuration updates for an FSx for OpenZFS file system.
*
*/
private UpdateFileSystemOpenZFSConfiguration openZFSConfiguration;
private String storageType;
/**
*
* The ID of the file system that you are updating.
*
*
* @param fileSystemId
* The ID of the file system that you are updating.
*/
public void setFileSystemId(String fileSystemId) {
this.fileSystemId = fileSystemId;
}
/**
*
* The ID of the file system that you are updating.
*
*
* @return The ID of the file system that you are updating.
*/
public String getFileSystemId() {
return this.fileSystemId;
}
/**
*
* The ID of the file system that you are updating.
*
*
* @param fileSystemId
* The ID of the file system that you are updating.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateFileSystemRequest withFileSystemId(String fileSystemId) {
setFileSystemId(fileSystemId);
return this;
}
/**
*
* A string of up to 63 ASCII characters that Amazon FSx uses to ensure idempotent updates. This string is
* automatically filled on your behalf when you use the Command Line Interface (CLI) or an Amazon Web Services SDK.
*
*
* @param clientRequestToken
* A string of up to 63 ASCII characters that Amazon FSx uses to ensure idempotent updates. This string is
* automatically filled on your behalf when you use the Command Line Interface (CLI) or an Amazon Web
* Services SDK.
*/
public void setClientRequestToken(String clientRequestToken) {
this.clientRequestToken = clientRequestToken;
}
/**
*
* A string of up to 63 ASCII characters that Amazon FSx uses to ensure idempotent updates. This string is
* automatically filled on your behalf when you use the Command Line Interface (CLI) or an Amazon Web Services SDK.
*
*
* @return A string of up to 63 ASCII characters that Amazon FSx uses to ensure idempotent updates. This string is
* automatically filled on your behalf when you use the Command Line Interface (CLI) or an Amazon Web
* Services SDK.
*/
public String getClientRequestToken() {
return this.clientRequestToken;
}
/**
*
* A string of up to 63 ASCII characters that Amazon FSx uses to ensure idempotent updates. This string is
* automatically filled on your behalf when you use the Command Line Interface (CLI) or an Amazon Web Services SDK.
*
*
* @param clientRequestToken
* A string of up to 63 ASCII characters that Amazon FSx uses to ensure idempotent updates. This string is
* automatically filled on your behalf when you use the Command Line Interface (CLI) or an Amazon Web
* Services SDK.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateFileSystemRequest withClientRequestToken(String clientRequestToken) {
setClientRequestToken(clientRequestToken);
return this;
}
/**
*
* Use this parameter to increase the storage capacity of an FSx for Windows File Server, FSx for Lustre, FSx for
* OpenZFS, or FSx for ONTAP file system. Specifies the storage capacity target value, in GiB, to increase the
* storage capacity for the file system that you're updating.
*
*
*
* You can't make a storage capacity increase request if there is an existing storage capacity increase request in
* progress.
*
*
*
* For Lustre file systems, the storage capacity target value can be the following:
*
*
* -
*
* For SCRATCH_2
, PERSISTENT_1
, and PERSISTENT_2 SSD
deployment types, valid
* values are in multiples of 2400 GiB. The value must be greater than the current storage capacity.
*
*
* -
*
* For PERSISTENT HDD
file systems, valid values are multiples of 6000 GiB for 12-MBps throughput per
* TiB file systems and multiples of 1800 GiB for 40-MBps throughput per TiB file systems. The values must be
* greater than the current storage capacity.
*
*
* -
*
* For SCRATCH_1
file systems, you can't increase the storage capacity.
*
*
*
*
* For more information, see Managing storage and
* throughput capacity in the FSx for Lustre User Guide.
*
*
* For FSx for OpenZFS file systems, the storage capacity target value must be at least 10 percent greater than the
* current storage capacity value. For more information, see Managing storage
* capacity in the FSx for OpenZFS User Guide.
*
*
* For Windows file systems, the storage capacity target value must be at least 10 percent greater than the current
* storage capacity value. To increase storage capacity, the file system must have at least 16 MBps of throughput
* capacity. For more information, see Managing storage
* capacity in the Amazon FSxfor Windows File Server User Guide.
*
*
* For ONTAP file systems, the storage capacity target value must be at least 10 percent greater than the current
* storage capacity value. For more information, see Managing storage capacity
* and provisioned IOPS in the Amazon FSx for NetApp ONTAP User Guide.
*
*
* @param storageCapacity
* Use this parameter to increase the storage capacity of an FSx for Windows File Server, FSx for Lustre, FSx
* for OpenZFS, or FSx for ONTAP file system. Specifies the storage capacity target value, in GiB, to
* increase the storage capacity for the file system that you're updating.
*
* You can't make a storage capacity increase request if there is an existing storage capacity increase
* request in progress.
*
*
*
* For Lustre file systems, the storage capacity target value can be the following:
*
*
* -
*
* For SCRATCH_2
, PERSISTENT_1
, and PERSISTENT_2 SSD
deployment types,
* valid values are in multiples of 2400 GiB. The value must be greater than the current storage capacity.
*
*
* -
*
* For PERSISTENT HDD
file systems, valid values are multiples of 6000 GiB for 12-MBps
* throughput per TiB file systems and multiples of 1800 GiB for 40-MBps throughput per TiB file systems. The
* values must be greater than the current storage capacity.
*
*
* -
*
* For SCRATCH_1
file systems, you can't increase the storage capacity.
*
*
*
*
* For more information, see Managing storage
* and throughput capacity in the FSx for Lustre User Guide.
*
*
* For FSx for OpenZFS file systems, the storage capacity target value must be at least 10 percent greater
* than the current storage capacity value. For more information, see Managing storage
* capacity in the FSx for OpenZFS User Guide.
*
*
* For Windows file systems, the storage capacity target value must be at least 10 percent greater than the
* current storage capacity value. To increase storage capacity, the file system must have at least 16 MBps
* of throughput capacity. For more information, see Managing storage
* capacity in the Amazon FSxfor Windows File Server User Guide.
*
*
* For ONTAP file systems, the storage capacity target value must be at least 10 percent greater than the
* current storage capacity value. For more information, see Managing storage
* capacity and provisioned IOPS in the Amazon FSx for NetApp ONTAP User Guide.
*/
public void setStorageCapacity(Integer storageCapacity) {
this.storageCapacity = storageCapacity;
}
/**
*
* Use this parameter to increase the storage capacity of an FSx for Windows File Server, FSx for Lustre, FSx for
* OpenZFS, or FSx for ONTAP file system. Specifies the storage capacity target value, in GiB, to increase the
* storage capacity for the file system that you're updating.
*
*
*
* You can't make a storage capacity increase request if there is an existing storage capacity increase request in
* progress.
*
*
*
* For Lustre file systems, the storage capacity target value can be the following:
*
*
* -
*
* For SCRATCH_2
, PERSISTENT_1
, and PERSISTENT_2 SSD
deployment types, valid
* values are in multiples of 2400 GiB. The value must be greater than the current storage capacity.
*
*
* -
*
* For PERSISTENT HDD
file systems, valid values are multiples of 6000 GiB for 12-MBps throughput per
* TiB file systems and multiples of 1800 GiB for 40-MBps throughput per TiB file systems. The values must be
* greater than the current storage capacity.
*
*
* -
*
* For SCRATCH_1
file systems, you can't increase the storage capacity.
*
*
*
*
* For more information, see Managing storage and
* throughput capacity in the FSx for Lustre User Guide.
*
*
* For FSx for OpenZFS file systems, the storage capacity target value must be at least 10 percent greater than the
* current storage capacity value. For more information, see Managing storage
* capacity in the FSx for OpenZFS User Guide.
*
*
* For Windows file systems, the storage capacity target value must be at least 10 percent greater than the current
* storage capacity value. To increase storage capacity, the file system must have at least 16 MBps of throughput
* capacity. For more information, see Managing storage
* capacity in the Amazon FSxfor Windows File Server User Guide.
*
*
* For ONTAP file systems, the storage capacity target value must be at least 10 percent greater than the current
* storage capacity value. For more information, see Managing storage capacity
* and provisioned IOPS in the Amazon FSx for NetApp ONTAP User Guide.
*
*
* @return Use this parameter to increase the storage capacity of an FSx for Windows File Server, FSx for Lustre,
* FSx for OpenZFS, or FSx for ONTAP file system. Specifies the storage capacity target value, in GiB, to
* increase the storage capacity for the file system that you're updating.
*
* You can't make a storage capacity increase request if there is an existing storage capacity increase
* request in progress.
*
*
*
* For Lustre file systems, the storage capacity target value can be the following:
*
*
* -
*
* For SCRATCH_2
, PERSISTENT_1
, and PERSISTENT_2 SSD
deployment
* types, valid values are in multiples of 2400 GiB. The value must be greater than the current storage
* capacity.
*
*
* -
*
* For PERSISTENT HDD
file systems, valid values are multiples of 6000 GiB for 12-MBps
* throughput per TiB file systems and multiples of 1800 GiB for 40-MBps throughput per TiB file systems.
* The values must be greater than the current storage capacity.
*
*
* -
*
* For SCRATCH_1
file systems, you can't increase the storage capacity.
*
*
*
*
* For more information, see Managing storage
* and throughput capacity in the FSx for Lustre User Guide.
*
*
* For FSx for OpenZFS file systems, the storage capacity target value must be at least 10 percent greater
* than the current storage capacity value. For more information, see Managing
* storage capacity in the FSx for OpenZFS User Guide.
*
*
* For Windows file systems, the storage capacity target value must be at least 10 percent greater than the
* current storage capacity value. To increase storage capacity, the file system must have at least 16 MBps
* of throughput capacity. For more information, see Managing
* storage capacity in the Amazon FSxfor Windows File Server User Guide.
*
*
* For ONTAP file systems, the storage capacity target value must be at least 10 percent greater than the
* current storage capacity value. For more information, see Managing storage
* capacity and provisioned IOPS in the Amazon FSx for NetApp ONTAP User Guide.
*/
public Integer getStorageCapacity() {
return this.storageCapacity;
}
/**
*
* Use this parameter to increase the storage capacity of an FSx for Windows File Server, FSx for Lustre, FSx for
* OpenZFS, or FSx for ONTAP file system. Specifies the storage capacity target value, in GiB, to increase the
* storage capacity for the file system that you're updating.
*
*
*
* You can't make a storage capacity increase request if there is an existing storage capacity increase request in
* progress.
*
*
*
* For Lustre file systems, the storage capacity target value can be the following:
*
*
* -
*
* For SCRATCH_2
, PERSISTENT_1
, and PERSISTENT_2 SSD
deployment types, valid
* values are in multiples of 2400 GiB. The value must be greater than the current storage capacity.
*
*
* -
*
* For PERSISTENT HDD
file systems, valid values are multiples of 6000 GiB for 12-MBps throughput per
* TiB file systems and multiples of 1800 GiB for 40-MBps throughput per TiB file systems. The values must be
* greater than the current storage capacity.
*
*
* -
*
* For SCRATCH_1
file systems, you can't increase the storage capacity.
*
*
*
*
* For more information, see Managing storage and
* throughput capacity in the FSx for Lustre User Guide.
*
*
* For FSx for OpenZFS file systems, the storage capacity target value must be at least 10 percent greater than the
* current storage capacity value. For more information, see Managing storage
* capacity in the FSx for OpenZFS User Guide.
*
*
* For Windows file systems, the storage capacity target value must be at least 10 percent greater than the current
* storage capacity value. To increase storage capacity, the file system must have at least 16 MBps of throughput
* capacity. For more information, see Managing storage
* capacity in the Amazon FSxfor Windows File Server User Guide.
*
*
* For ONTAP file systems, the storage capacity target value must be at least 10 percent greater than the current
* storage capacity value. For more information, see Managing storage capacity
* and provisioned IOPS in the Amazon FSx for NetApp ONTAP User Guide.
*
*
* @param storageCapacity
* Use this parameter to increase the storage capacity of an FSx for Windows File Server, FSx for Lustre, FSx
* for OpenZFS, or FSx for ONTAP file system. Specifies the storage capacity target value, in GiB, to
* increase the storage capacity for the file system that you're updating.
*
* You can't make a storage capacity increase request if there is an existing storage capacity increase
* request in progress.
*
*
*
* For Lustre file systems, the storage capacity target value can be the following:
*
*
* -
*
* For SCRATCH_2
, PERSISTENT_1
, and PERSISTENT_2 SSD
deployment types,
* valid values are in multiples of 2400 GiB. The value must be greater than the current storage capacity.
*
*
* -
*
* For PERSISTENT HDD
file systems, valid values are multiples of 6000 GiB for 12-MBps
* throughput per TiB file systems and multiples of 1800 GiB for 40-MBps throughput per TiB file systems. The
* values must be greater than the current storage capacity.
*
*
* -
*
* For SCRATCH_1
file systems, you can't increase the storage capacity.
*
*
*
*
* For more information, see Managing storage
* and throughput capacity in the FSx for Lustre User Guide.
*
*
* For FSx for OpenZFS file systems, the storage capacity target value must be at least 10 percent greater
* than the current storage capacity value. For more information, see Managing storage
* capacity in the FSx for OpenZFS User Guide.
*
*
* For Windows file systems, the storage capacity target value must be at least 10 percent greater than the
* current storage capacity value. To increase storage capacity, the file system must have at least 16 MBps
* of throughput capacity. For more information, see Managing storage
* capacity in the Amazon FSxfor Windows File Server User Guide.
*
*
* For ONTAP file systems, the storage capacity target value must be at least 10 percent greater than the
* current storage capacity value. For more information, see Managing storage
* capacity and provisioned IOPS in the Amazon FSx for NetApp ONTAP User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateFileSystemRequest withStorageCapacity(Integer storageCapacity) {
setStorageCapacity(storageCapacity);
return this;
}
/**
*
* The configuration updates for an Amazon FSx for Windows File Server file system.
*
*
* @param windowsConfiguration
* The configuration updates for an Amazon FSx for Windows File Server file system.
*/
public void setWindowsConfiguration(UpdateFileSystemWindowsConfiguration windowsConfiguration) {
this.windowsConfiguration = windowsConfiguration;
}
/**
*
* The configuration updates for an Amazon FSx for Windows File Server file system.
*
*
* @return The configuration updates for an Amazon FSx for Windows File Server file system.
*/
public UpdateFileSystemWindowsConfiguration getWindowsConfiguration() {
return this.windowsConfiguration;
}
/**
*
* The configuration updates for an Amazon FSx for Windows File Server file system.
*
*
* @param windowsConfiguration
* The configuration updates for an Amazon FSx for Windows File Server file system.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateFileSystemRequest withWindowsConfiguration(UpdateFileSystemWindowsConfiguration windowsConfiguration) {
setWindowsConfiguration(windowsConfiguration);
return this;
}
/**
* @param lustreConfiguration
*/
public void setLustreConfiguration(UpdateFileSystemLustreConfiguration lustreConfiguration) {
this.lustreConfiguration = lustreConfiguration;
}
/**
* @return
*/
public UpdateFileSystemLustreConfiguration getLustreConfiguration() {
return this.lustreConfiguration;
}
/**
* @param lustreConfiguration
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateFileSystemRequest withLustreConfiguration(UpdateFileSystemLustreConfiguration lustreConfiguration) {
setLustreConfiguration(lustreConfiguration);
return this;
}
/**
* @param ontapConfiguration
*/
public void setOntapConfiguration(UpdateFileSystemOntapConfiguration ontapConfiguration) {
this.ontapConfiguration = ontapConfiguration;
}
/**
* @return
*/
public UpdateFileSystemOntapConfiguration getOntapConfiguration() {
return this.ontapConfiguration;
}
/**
* @param ontapConfiguration
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateFileSystemRequest withOntapConfiguration(UpdateFileSystemOntapConfiguration ontapConfiguration) {
setOntapConfiguration(ontapConfiguration);
return this;
}
/**
*
* The configuration updates for an FSx for OpenZFS file system.
*
*
* @param openZFSConfiguration
* The configuration updates for an FSx for OpenZFS file system.
*/
public void setOpenZFSConfiguration(UpdateFileSystemOpenZFSConfiguration openZFSConfiguration) {
this.openZFSConfiguration = openZFSConfiguration;
}
/**
*
* The configuration updates for an FSx for OpenZFS file system.
*
*
* @return The configuration updates for an FSx for OpenZFS file system.
*/
public UpdateFileSystemOpenZFSConfiguration getOpenZFSConfiguration() {
return this.openZFSConfiguration;
}
/**
*
* The configuration updates for an FSx for OpenZFS file system.
*
*
* @param openZFSConfiguration
* The configuration updates for an FSx for OpenZFS file system.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateFileSystemRequest withOpenZFSConfiguration(UpdateFileSystemOpenZFSConfiguration openZFSConfiguration) {
setOpenZFSConfiguration(openZFSConfiguration);
return this;
}
/**
* @param storageType
* @see StorageType
*/
public void setStorageType(String storageType) {
this.storageType = storageType;
}
/**
* @return
* @see StorageType
*/
public String getStorageType() {
return this.storageType;
}
/**
* @param storageType
* @return Returns a reference to this object so that method calls can be chained together.
* @see StorageType
*/
public UpdateFileSystemRequest withStorageType(String storageType) {
setStorageType(storageType);
return this;
}
/**
* @param storageType
* @return Returns a reference to this object so that method calls can be chained together.
* @see StorageType
*/
public UpdateFileSystemRequest withStorageType(StorageType storageType) {
this.storageType = storageType.toString();
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getFileSystemId() != null)
sb.append("FileSystemId: ").append(getFileSystemId()).append(",");
if (getClientRequestToken() != null)
sb.append("ClientRequestToken: ").append(getClientRequestToken()).append(",");
if (getStorageCapacity() != null)
sb.append("StorageCapacity: ").append(getStorageCapacity()).append(",");
if (getWindowsConfiguration() != null)
sb.append("WindowsConfiguration: ").append(getWindowsConfiguration()).append(",");
if (getLustreConfiguration() != null)
sb.append("LustreConfiguration: ").append(getLustreConfiguration()).append(",");
if (getOntapConfiguration() != null)
sb.append("OntapConfiguration: ").append(getOntapConfiguration()).append(",");
if (getOpenZFSConfiguration() != null)
sb.append("OpenZFSConfiguration: ").append(getOpenZFSConfiguration()).append(",");
if (getStorageType() != null)
sb.append("StorageType: ").append(getStorageType());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof UpdateFileSystemRequest == false)
return false;
UpdateFileSystemRequest other = (UpdateFileSystemRequest) obj;
if (other.getFileSystemId() == null ^ this.getFileSystemId() == null)
return false;
if (other.getFileSystemId() != null && other.getFileSystemId().equals(this.getFileSystemId()) == false)
return false;
if (other.getClientRequestToken() == null ^ this.getClientRequestToken() == null)
return false;
if (other.getClientRequestToken() != null && other.getClientRequestToken().equals(this.getClientRequestToken()) == false)
return false;
if (other.getStorageCapacity() == null ^ this.getStorageCapacity() == null)
return false;
if (other.getStorageCapacity() != null && other.getStorageCapacity().equals(this.getStorageCapacity()) == false)
return false;
if (other.getWindowsConfiguration() == null ^ this.getWindowsConfiguration() == null)
return false;
if (other.getWindowsConfiguration() != null && other.getWindowsConfiguration().equals(this.getWindowsConfiguration()) == false)
return false;
if (other.getLustreConfiguration() == null ^ this.getLustreConfiguration() == null)
return false;
if (other.getLustreConfiguration() != null && other.getLustreConfiguration().equals(this.getLustreConfiguration()) == false)
return false;
if (other.getOntapConfiguration() == null ^ this.getOntapConfiguration() == null)
return false;
if (other.getOntapConfiguration() != null && other.getOntapConfiguration().equals(this.getOntapConfiguration()) == false)
return false;
if (other.getOpenZFSConfiguration() == null ^ this.getOpenZFSConfiguration() == null)
return false;
if (other.getOpenZFSConfiguration() != null && other.getOpenZFSConfiguration().equals(this.getOpenZFSConfiguration()) == false)
return false;
if (other.getStorageType() == null ^ this.getStorageType() == null)
return false;
if (other.getStorageType() != null && other.getStorageType().equals(this.getStorageType()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getFileSystemId() == null) ? 0 : getFileSystemId().hashCode());
hashCode = prime * hashCode + ((getClientRequestToken() == null) ? 0 : getClientRequestToken().hashCode());
hashCode = prime * hashCode + ((getStorageCapacity() == null) ? 0 : getStorageCapacity().hashCode());
hashCode = prime * hashCode + ((getWindowsConfiguration() == null) ? 0 : getWindowsConfiguration().hashCode());
hashCode = prime * hashCode + ((getLustreConfiguration() == null) ? 0 : getLustreConfiguration().hashCode());
hashCode = prime * hashCode + ((getOntapConfiguration() == null) ? 0 : getOntapConfiguration().hashCode());
hashCode = prime * hashCode + ((getOpenZFSConfiguration() == null) ? 0 : getOpenZFSConfiguration().hashCode());
hashCode = prime * hashCode + ((getStorageType() == null) ? 0 : getStorageType().hashCode());
return hashCode;
}
@Override
public UpdateFileSystemRequest clone() {
return (UpdateFileSystemRequest) super.clone();
}
}