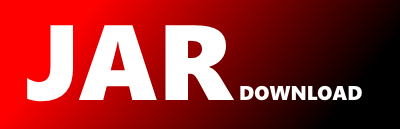
com.amazonaws.services.fsx.model.AdministrativeAction Maven / Gradle / Ivy
Show all versions of aws-java-sdk-fsx Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.fsx.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Describes a specific Amazon FSx administrative action for the current Windows, Lustre, OpenZFS, or ONTAP file system
* or volume.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class AdministrativeAction implements Serializable, Cloneable, StructuredPojo {
private String administrativeActionType;
/**
*
* The percentage-complete status of a STORAGE_OPTIMIZATION
or DOWNLOAD_DATA_FROM_BACKUP
* administrative action. Does not apply to any other administrative action type.
*
*/
private Integer progressPercent;
/**
*
* The time that the administrative action request was received.
*
*/
private java.util.Date requestTime;
/**
*
* The status of the administrative action, as follows:
*
*
* -
*
* FAILED
- Amazon FSx failed to process the administrative action successfully.
*
*
* -
*
* IN_PROGRESS
- Amazon FSx is processing the administrative action.
*
*
* -
*
* PENDING
- Amazon FSx is waiting to process the administrative action.
*
*
* -
*
* COMPLETED
- Amazon FSx has finished processing the administrative task.
*
*
* For a backup restore to a second-generation FSx for ONTAP file system, indicates that all data has been
* downloaded to the volume, and clients now have read-write access to volume.
*
*
* -
*
* UPDATED_OPTIMIZING
- For a storage-capacity increase update, Amazon FSx has updated the file system
* with the new storage capacity, and is now performing the storage-optimization process.
*
*
* -
*
* PENDING
- For a backup restore to a second-generation FSx for ONTAP file system, indicates that the
* file metadata is being downloaded onto the volume. The volume's Lifecycle state is CREATING.
*
*
* -
*
* IN_PROGRESS
- For a backup restore to a second-generation FSx for ONTAP file system, indicates that
* all metadata has been downloaded to the new volume and client can access data with read-only access while Amazon
* FSx downloads the file data to the volume. Track the progress of this process with the
* ProgressPercent
element.
*
*
*
*/
private String status;
/**
*
* The target value for the administration action, provided in the UpdateFileSystem
operation. Returned
* for FILE_SYSTEM_UPDATE
administrative actions.
*
*/
private FileSystem targetFileSystemValues;
private AdministrativeActionFailureDetails failureDetails;
private Volume targetVolumeValues;
private Snapshot targetSnapshotValues;
/**
*
* The number of bytes that have transferred for the FSx for OpenZFS snapshot that you're copying.
*
*/
private Long totalTransferBytes;
/**
*
* The remaining bytes to transfer for the FSx for OpenZFS snapshot that you're copying.
*
*/
private Long remainingTransferBytes;
/**
* @param administrativeActionType
* @see AdministrativeActionType
*/
public void setAdministrativeActionType(String administrativeActionType) {
this.administrativeActionType = administrativeActionType;
}
/**
* @return
* @see AdministrativeActionType
*/
public String getAdministrativeActionType() {
return this.administrativeActionType;
}
/**
* @param administrativeActionType
* @return Returns a reference to this object so that method calls can be chained together.
* @see AdministrativeActionType
*/
public AdministrativeAction withAdministrativeActionType(String administrativeActionType) {
setAdministrativeActionType(administrativeActionType);
return this;
}
/**
* @param administrativeActionType
* @return Returns a reference to this object so that method calls can be chained together.
* @see AdministrativeActionType
*/
public AdministrativeAction withAdministrativeActionType(AdministrativeActionType administrativeActionType) {
this.administrativeActionType = administrativeActionType.toString();
return this;
}
/**
*
* The percentage-complete status of a STORAGE_OPTIMIZATION
or DOWNLOAD_DATA_FROM_BACKUP
* administrative action. Does not apply to any other administrative action type.
*
*
* @param progressPercent
* The percentage-complete status of a STORAGE_OPTIMIZATION
or
* DOWNLOAD_DATA_FROM_BACKUP
administrative action. Does not apply to any other administrative
* action type.
*/
public void setProgressPercent(Integer progressPercent) {
this.progressPercent = progressPercent;
}
/**
*
* The percentage-complete status of a STORAGE_OPTIMIZATION
or DOWNLOAD_DATA_FROM_BACKUP
* administrative action. Does not apply to any other administrative action type.
*
*
* @return The percentage-complete status of a STORAGE_OPTIMIZATION
or
* DOWNLOAD_DATA_FROM_BACKUP
administrative action. Does not apply to any other administrative
* action type.
*/
public Integer getProgressPercent() {
return this.progressPercent;
}
/**
*
* The percentage-complete status of a STORAGE_OPTIMIZATION
or DOWNLOAD_DATA_FROM_BACKUP
* administrative action. Does not apply to any other administrative action type.
*
*
* @param progressPercent
* The percentage-complete status of a STORAGE_OPTIMIZATION
or
* DOWNLOAD_DATA_FROM_BACKUP
administrative action. Does not apply to any other administrative
* action type.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AdministrativeAction withProgressPercent(Integer progressPercent) {
setProgressPercent(progressPercent);
return this;
}
/**
*
* The time that the administrative action request was received.
*
*
* @param requestTime
* The time that the administrative action request was received.
*/
public void setRequestTime(java.util.Date requestTime) {
this.requestTime = requestTime;
}
/**
*
* The time that the administrative action request was received.
*
*
* @return The time that the administrative action request was received.
*/
public java.util.Date getRequestTime() {
return this.requestTime;
}
/**
*
* The time that the administrative action request was received.
*
*
* @param requestTime
* The time that the administrative action request was received.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AdministrativeAction withRequestTime(java.util.Date requestTime) {
setRequestTime(requestTime);
return this;
}
/**
*
* The status of the administrative action, as follows:
*
*
* -
*
* FAILED
- Amazon FSx failed to process the administrative action successfully.
*
*
* -
*
* IN_PROGRESS
- Amazon FSx is processing the administrative action.
*
*
* -
*
* PENDING
- Amazon FSx is waiting to process the administrative action.
*
*
* -
*
* COMPLETED
- Amazon FSx has finished processing the administrative task.
*
*
* For a backup restore to a second-generation FSx for ONTAP file system, indicates that all data has been
* downloaded to the volume, and clients now have read-write access to volume.
*
*
* -
*
* UPDATED_OPTIMIZING
- For a storage-capacity increase update, Amazon FSx has updated the file system
* with the new storage capacity, and is now performing the storage-optimization process.
*
*
* -
*
* PENDING
- For a backup restore to a second-generation FSx for ONTAP file system, indicates that the
* file metadata is being downloaded onto the volume. The volume's Lifecycle state is CREATING.
*
*
* -
*
* IN_PROGRESS
- For a backup restore to a second-generation FSx for ONTAP file system, indicates that
* all metadata has been downloaded to the new volume and client can access data with read-only access while Amazon
* FSx downloads the file data to the volume. Track the progress of this process with the
* ProgressPercent
element.
*
*
*
*
* @param status
* The status of the administrative action, as follows:
*
* -
*
* FAILED
- Amazon FSx failed to process the administrative action successfully.
*
*
* -
*
* IN_PROGRESS
- Amazon FSx is processing the administrative action.
*
*
* -
*
* PENDING
- Amazon FSx is waiting to process the administrative action.
*
*
* -
*
* COMPLETED
- Amazon FSx has finished processing the administrative task.
*
*
* For a backup restore to a second-generation FSx for ONTAP file system, indicates that all data has been
* downloaded to the volume, and clients now have read-write access to volume.
*
*
* -
*
* UPDATED_OPTIMIZING
- For a storage-capacity increase update, Amazon FSx has updated the file
* system with the new storage capacity, and is now performing the storage-optimization process.
*
*
* -
*
* PENDING
- For a backup restore to a second-generation FSx for ONTAP file system, indicates
* that the file metadata is being downloaded onto the volume. The volume's Lifecycle state is CREATING.
*
*
* -
*
* IN_PROGRESS
- For a backup restore to a second-generation FSx for ONTAP file system,
* indicates that all metadata has been downloaded to the new volume and client can access data with
* read-only access while Amazon FSx downloads the file data to the volume. Track the progress of this
* process with the ProgressPercent
element.
*
*
* @see Status
*/
public void setStatus(String status) {
this.status = status;
}
/**
*
* The status of the administrative action, as follows:
*
*
* -
*
* FAILED
- Amazon FSx failed to process the administrative action successfully.
*
*
* -
*
* IN_PROGRESS
- Amazon FSx is processing the administrative action.
*
*
* -
*
* PENDING
- Amazon FSx is waiting to process the administrative action.
*
*
* -
*
* COMPLETED
- Amazon FSx has finished processing the administrative task.
*
*
* For a backup restore to a second-generation FSx for ONTAP file system, indicates that all data has been
* downloaded to the volume, and clients now have read-write access to volume.
*
*
* -
*
* UPDATED_OPTIMIZING
- For a storage-capacity increase update, Amazon FSx has updated the file system
* with the new storage capacity, and is now performing the storage-optimization process.
*
*
* -
*
* PENDING
- For a backup restore to a second-generation FSx for ONTAP file system, indicates that the
* file metadata is being downloaded onto the volume. The volume's Lifecycle state is CREATING.
*
*
* -
*
* IN_PROGRESS
- For a backup restore to a second-generation FSx for ONTAP file system, indicates that
* all metadata has been downloaded to the new volume and client can access data with read-only access while Amazon
* FSx downloads the file data to the volume. Track the progress of this process with the
* ProgressPercent
element.
*
*
*
*
* @return The status of the administrative action, as follows:
*
* -
*
* FAILED
- Amazon FSx failed to process the administrative action successfully.
*
*
* -
*
* IN_PROGRESS
- Amazon FSx is processing the administrative action.
*
*
* -
*
* PENDING
- Amazon FSx is waiting to process the administrative action.
*
*
* -
*
* COMPLETED
- Amazon FSx has finished processing the administrative task.
*
*
* For a backup restore to a second-generation FSx for ONTAP file system, indicates that all data has been
* downloaded to the volume, and clients now have read-write access to volume.
*
*
* -
*
* UPDATED_OPTIMIZING
- For a storage-capacity increase update, Amazon FSx has updated the file
* system with the new storage capacity, and is now performing the storage-optimization process.
*
*
* -
*
* PENDING
- For a backup restore to a second-generation FSx for ONTAP file system, indicates
* that the file metadata is being downloaded onto the volume. The volume's Lifecycle state is CREATING.
*
*
* -
*
* IN_PROGRESS
- For a backup restore to a second-generation FSx for ONTAP file system,
* indicates that all metadata has been downloaded to the new volume and client can access data with
* read-only access while Amazon FSx downloads the file data to the volume. Track the progress of this
* process with the ProgressPercent
element.
*
*
* @see Status
*/
public String getStatus() {
return this.status;
}
/**
*
* The status of the administrative action, as follows:
*
*
* -
*
* FAILED
- Amazon FSx failed to process the administrative action successfully.
*
*
* -
*
* IN_PROGRESS
- Amazon FSx is processing the administrative action.
*
*
* -
*
* PENDING
- Amazon FSx is waiting to process the administrative action.
*
*
* -
*
* COMPLETED
- Amazon FSx has finished processing the administrative task.
*
*
* For a backup restore to a second-generation FSx for ONTAP file system, indicates that all data has been
* downloaded to the volume, and clients now have read-write access to volume.
*
*
* -
*
* UPDATED_OPTIMIZING
- For a storage-capacity increase update, Amazon FSx has updated the file system
* with the new storage capacity, and is now performing the storage-optimization process.
*
*
* -
*
* PENDING
- For a backup restore to a second-generation FSx for ONTAP file system, indicates that the
* file metadata is being downloaded onto the volume. The volume's Lifecycle state is CREATING.
*
*
* -
*
* IN_PROGRESS
- For a backup restore to a second-generation FSx for ONTAP file system, indicates that
* all metadata has been downloaded to the new volume and client can access data with read-only access while Amazon
* FSx downloads the file data to the volume. Track the progress of this process with the
* ProgressPercent
element.
*
*
*
*
* @param status
* The status of the administrative action, as follows:
*
* -
*
* FAILED
- Amazon FSx failed to process the administrative action successfully.
*
*
* -
*
* IN_PROGRESS
- Amazon FSx is processing the administrative action.
*
*
* -
*
* PENDING
- Amazon FSx is waiting to process the administrative action.
*
*
* -
*
* COMPLETED
- Amazon FSx has finished processing the administrative task.
*
*
* For a backup restore to a second-generation FSx for ONTAP file system, indicates that all data has been
* downloaded to the volume, and clients now have read-write access to volume.
*
*
* -
*
* UPDATED_OPTIMIZING
- For a storage-capacity increase update, Amazon FSx has updated the file
* system with the new storage capacity, and is now performing the storage-optimization process.
*
*
* -
*
* PENDING
- For a backup restore to a second-generation FSx for ONTAP file system, indicates
* that the file metadata is being downloaded onto the volume. The volume's Lifecycle state is CREATING.
*
*
* -
*
* IN_PROGRESS
- For a backup restore to a second-generation FSx for ONTAP file system,
* indicates that all metadata has been downloaded to the new volume and client can access data with
* read-only access while Amazon FSx downloads the file data to the volume. Track the progress of this
* process with the ProgressPercent
element.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see Status
*/
public AdministrativeAction withStatus(String status) {
setStatus(status);
return this;
}
/**
*
* The status of the administrative action, as follows:
*
*
* -
*
* FAILED
- Amazon FSx failed to process the administrative action successfully.
*
*
* -
*
* IN_PROGRESS
- Amazon FSx is processing the administrative action.
*
*
* -
*
* PENDING
- Amazon FSx is waiting to process the administrative action.
*
*
* -
*
* COMPLETED
- Amazon FSx has finished processing the administrative task.
*
*
* For a backup restore to a second-generation FSx for ONTAP file system, indicates that all data has been
* downloaded to the volume, and clients now have read-write access to volume.
*
*
* -
*
* UPDATED_OPTIMIZING
- For a storage-capacity increase update, Amazon FSx has updated the file system
* with the new storage capacity, and is now performing the storage-optimization process.
*
*
* -
*
* PENDING
- For a backup restore to a second-generation FSx for ONTAP file system, indicates that the
* file metadata is being downloaded onto the volume. The volume's Lifecycle state is CREATING.
*
*
* -
*
* IN_PROGRESS
- For a backup restore to a second-generation FSx for ONTAP file system, indicates that
* all metadata has been downloaded to the new volume and client can access data with read-only access while Amazon
* FSx downloads the file data to the volume. Track the progress of this process with the
* ProgressPercent
element.
*
*
*
*
* @param status
* The status of the administrative action, as follows:
*
* -
*
* FAILED
- Amazon FSx failed to process the administrative action successfully.
*
*
* -
*
* IN_PROGRESS
- Amazon FSx is processing the administrative action.
*
*
* -
*
* PENDING
- Amazon FSx is waiting to process the administrative action.
*
*
* -
*
* COMPLETED
- Amazon FSx has finished processing the administrative task.
*
*
* For a backup restore to a second-generation FSx for ONTAP file system, indicates that all data has been
* downloaded to the volume, and clients now have read-write access to volume.
*
*
* -
*
* UPDATED_OPTIMIZING
- For a storage-capacity increase update, Amazon FSx has updated the file
* system with the new storage capacity, and is now performing the storage-optimization process.
*
*
* -
*
* PENDING
- For a backup restore to a second-generation FSx for ONTAP file system, indicates
* that the file metadata is being downloaded onto the volume. The volume's Lifecycle state is CREATING.
*
*
* -
*
* IN_PROGRESS
- For a backup restore to a second-generation FSx for ONTAP file system,
* indicates that all metadata has been downloaded to the new volume and client can access data with
* read-only access while Amazon FSx downloads the file data to the volume. Track the progress of this
* process with the ProgressPercent
element.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see Status
*/
public AdministrativeAction withStatus(Status status) {
this.status = status.toString();
return this;
}
/**
*
* The target value for the administration action, provided in the UpdateFileSystem
operation. Returned
* for FILE_SYSTEM_UPDATE
administrative actions.
*
*
* @param targetFileSystemValues
* The target value for the administration action, provided in the UpdateFileSystem
operation.
* Returned for FILE_SYSTEM_UPDATE
administrative actions.
*/
public void setTargetFileSystemValues(FileSystem targetFileSystemValues) {
this.targetFileSystemValues = targetFileSystemValues;
}
/**
*
* The target value for the administration action, provided in the UpdateFileSystem
operation. Returned
* for FILE_SYSTEM_UPDATE
administrative actions.
*
*
* @return The target value for the administration action, provided in the UpdateFileSystem
operation.
* Returned for FILE_SYSTEM_UPDATE
administrative actions.
*/
public FileSystem getTargetFileSystemValues() {
return this.targetFileSystemValues;
}
/**
*
* The target value for the administration action, provided in the UpdateFileSystem
operation. Returned
* for FILE_SYSTEM_UPDATE
administrative actions.
*
*
* @param targetFileSystemValues
* The target value for the administration action, provided in the UpdateFileSystem
operation.
* Returned for FILE_SYSTEM_UPDATE
administrative actions.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AdministrativeAction withTargetFileSystemValues(FileSystem targetFileSystemValues) {
setTargetFileSystemValues(targetFileSystemValues);
return this;
}
/**
* @param failureDetails
*/
public void setFailureDetails(AdministrativeActionFailureDetails failureDetails) {
this.failureDetails = failureDetails;
}
/**
* @return
*/
public AdministrativeActionFailureDetails getFailureDetails() {
return this.failureDetails;
}
/**
* @param failureDetails
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AdministrativeAction withFailureDetails(AdministrativeActionFailureDetails failureDetails) {
setFailureDetails(failureDetails);
return this;
}
/**
* @param targetVolumeValues
*/
public void setTargetVolumeValues(Volume targetVolumeValues) {
this.targetVolumeValues = targetVolumeValues;
}
/**
* @return
*/
public Volume getTargetVolumeValues() {
return this.targetVolumeValues;
}
/**
* @param targetVolumeValues
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AdministrativeAction withTargetVolumeValues(Volume targetVolumeValues) {
setTargetVolumeValues(targetVolumeValues);
return this;
}
/**
* @param targetSnapshotValues
*/
public void setTargetSnapshotValues(Snapshot targetSnapshotValues) {
this.targetSnapshotValues = targetSnapshotValues;
}
/**
* @return
*/
public Snapshot getTargetSnapshotValues() {
return this.targetSnapshotValues;
}
/**
* @param targetSnapshotValues
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AdministrativeAction withTargetSnapshotValues(Snapshot targetSnapshotValues) {
setTargetSnapshotValues(targetSnapshotValues);
return this;
}
/**
*
* The number of bytes that have transferred for the FSx for OpenZFS snapshot that you're copying.
*
*
* @param totalTransferBytes
* The number of bytes that have transferred for the FSx for OpenZFS snapshot that you're copying.
*/
public void setTotalTransferBytes(Long totalTransferBytes) {
this.totalTransferBytes = totalTransferBytes;
}
/**
*
* The number of bytes that have transferred for the FSx for OpenZFS snapshot that you're copying.
*
*
* @return The number of bytes that have transferred for the FSx for OpenZFS snapshot that you're copying.
*/
public Long getTotalTransferBytes() {
return this.totalTransferBytes;
}
/**
*
* The number of bytes that have transferred for the FSx for OpenZFS snapshot that you're copying.
*
*
* @param totalTransferBytes
* The number of bytes that have transferred for the FSx for OpenZFS snapshot that you're copying.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AdministrativeAction withTotalTransferBytes(Long totalTransferBytes) {
setTotalTransferBytes(totalTransferBytes);
return this;
}
/**
*
* The remaining bytes to transfer for the FSx for OpenZFS snapshot that you're copying.
*
*
* @param remainingTransferBytes
* The remaining bytes to transfer for the FSx for OpenZFS snapshot that you're copying.
*/
public void setRemainingTransferBytes(Long remainingTransferBytes) {
this.remainingTransferBytes = remainingTransferBytes;
}
/**
*
* The remaining bytes to transfer for the FSx for OpenZFS snapshot that you're copying.
*
*
* @return The remaining bytes to transfer for the FSx for OpenZFS snapshot that you're copying.
*/
public Long getRemainingTransferBytes() {
return this.remainingTransferBytes;
}
/**
*
* The remaining bytes to transfer for the FSx for OpenZFS snapshot that you're copying.
*
*
* @param remainingTransferBytes
* The remaining bytes to transfer for the FSx for OpenZFS snapshot that you're copying.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AdministrativeAction withRemainingTransferBytes(Long remainingTransferBytes) {
setRemainingTransferBytes(remainingTransferBytes);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getAdministrativeActionType() != null)
sb.append("AdministrativeActionType: ").append(getAdministrativeActionType()).append(",");
if (getProgressPercent() != null)
sb.append("ProgressPercent: ").append(getProgressPercent()).append(",");
if (getRequestTime() != null)
sb.append("RequestTime: ").append(getRequestTime()).append(",");
if (getStatus() != null)
sb.append("Status: ").append(getStatus()).append(",");
if (getTargetFileSystemValues() != null)
sb.append("TargetFileSystemValues: ").append(getTargetFileSystemValues()).append(",");
if (getFailureDetails() != null)
sb.append("FailureDetails: ").append(getFailureDetails()).append(",");
if (getTargetVolumeValues() != null)
sb.append("TargetVolumeValues: ").append(getTargetVolumeValues()).append(",");
if (getTargetSnapshotValues() != null)
sb.append("TargetSnapshotValues: ").append(getTargetSnapshotValues()).append(",");
if (getTotalTransferBytes() != null)
sb.append("TotalTransferBytes: ").append(getTotalTransferBytes()).append(",");
if (getRemainingTransferBytes() != null)
sb.append("RemainingTransferBytes: ").append(getRemainingTransferBytes());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof AdministrativeAction == false)
return false;
AdministrativeAction other = (AdministrativeAction) obj;
if (other.getAdministrativeActionType() == null ^ this.getAdministrativeActionType() == null)
return false;
if (other.getAdministrativeActionType() != null && other.getAdministrativeActionType().equals(this.getAdministrativeActionType()) == false)
return false;
if (other.getProgressPercent() == null ^ this.getProgressPercent() == null)
return false;
if (other.getProgressPercent() != null && other.getProgressPercent().equals(this.getProgressPercent()) == false)
return false;
if (other.getRequestTime() == null ^ this.getRequestTime() == null)
return false;
if (other.getRequestTime() != null && other.getRequestTime().equals(this.getRequestTime()) == false)
return false;
if (other.getStatus() == null ^ this.getStatus() == null)
return false;
if (other.getStatus() != null && other.getStatus().equals(this.getStatus()) == false)
return false;
if (other.getTargetFileSystemValues() == null ^ this.getTargetFileSystemValues() == null)
return false;
if (other.getTargetFileSystemValues() != null && other.getTargetFileSystemValues().equals(this.getTargetFileSystemValues()) == false)
return false;
if (other.getFailureDetails() == null ^ this.getFailureDetails() == null)
return false;
if (other.getFailureDetails() != null && other.getFailureDetails().equals(this.getFailureDetails()) == false)
return false;
if (other.getTargetVolumeValues() == null ^ this.getTargetVolumeValues() == null)
return false;
if (other.getTargetVolumeValues() != null && other.getTargetVolumeValues().equals(this.getTargetVolumeValues()) == false)
return false;
if (other.getTargetSnapshotValues() == null ^ this.getTargetSnapshotValues() == null)
return false;
if (other.getTargetSnapshotValues() != null && other.getTargetSnapshotValues().equals(this.getTargetSnapshotValues()) == false)
return false;
if (other.getTotalTransferBytes() == null ^ this.getTotalTransferBytes() == null)
return false;
if (other.getTotalTransferBytes() != null && other.getTotalTransferBytes().equals(this.getTotalTransferBytes()) == false)
return false;
if (other.getRemainingTransferBytes() == null ^ this.getRemainingTransferBytes() == null)
return false;
if (other.getRemainingTransferBytes() != null && other.getRemainingTransferBytes().equals(this.getRemainingTransferBytes()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getAdministrativeActionType() == null) ? 0 : getAdministrativeActionType().hashCode());
hashCode = prime * hashCode + ((getProgressPercent() == null) ? 0 : getProgressPercent().hashCode());
hashCode = prime * hashCode + ((getRequestTime() == null) ? 0 : getRequestTime().hashCode());
hashCode = prime * hashCode + ((getStatus() == null) ? 0 : getStatus().hashCode());
hashCode = prime * hashCode + ((getTargetFileSystemValues() == null) ? 0 : getTargetFileSystemValues().hashCode());
hashCode = prime * hashCode + ((getFailureDetails() == null) ? 0 : getFailureDetails().hashCode());
hashCode = prime * hashCode + ((getTargetVolumeValues() == null) ? 0 : getTargetVolumeValues().hashCode());
hashCode = prime * hashCode + ((getTargetSnapshotValues() == null) ? 0 : getTargetSnapshotValues().hashCode());
hashCode = prime * hashCode + ((getTotalTransferBytes() == null) ? 0 : getTotalTransferBytes().hashCode());
hashCode = prime * hashCode + ((getRemainingTransferBytes() == null) ? 0 : getRemainingTransferBytes().hashCode());
return hashCode;
}
@Override
public AdministrativeAction clone() {
try {
return (AdministrativeAction) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.fsx.model.transform.AdministrativeActionMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}