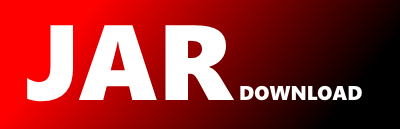
com.amazonaws.services.fsx.model.CreateFileSystemOntapConfiguration Maven / Gradle / Ivy
Show all versions of aws-java-sdk-fsx Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.fsx.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* The ONTAP configuration properties of the FSx for ONTAP file system that you are creating.
*
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class CreateFileSystemOntapConfiguration implements Serializable, Cloneable, StructuredPojo {
private Integer automaticBackupRetentionDays;
private String dailyAutomaticBackupStartTime;
/**
*
* Specifies the FSx for ONTAP file system deployment type to use in creating the file system.
*
*
* -
*
* MULTI_AZ_1
- A high availability file system configured for Multi-AZ redundancy to tolerate
* temporary Availability Zone (AZ) unavailability. This is a first-generation FSx for ONTAP file system.
*
*
* -
*
* MULTI_AZ_2
- A high availability file system configured for Multi-AZ redundancy to tolerate
* temporary AZ unavailability. This is a second-generation FSx for ONTAP file system.
*
*
* -
*
* SINGLE_AZ_1
- A file system configured for Single-AZ redundancy. This is a first-generation FSx for
* ONTAP file system.
*
*
* -
*
* SINGLE_AZ_2
- A file system configured with multiple high-availability (HA) pairs for Single-AZ
* redundancy. This is a second-generation FSx for ONTAP file system.
*
*
*
*
* For information about the use cases for Multi-AZ and Single-AZ deployments, refer to Choosing a file system
* deployment type.
*
*/
private String deploymentType;
/**
*
* (Multi-AZ only) Specifies the IP address range in which the endpoints to access your file system will be created.
* By default in the Amazon FSx API, Amazon FSx selects an unused IP address range for you from the 198.19.* range.
* By default in the Amazon FSx console, Amazon FSx chooses the last 64 IP addresses from the VPC’s primary CIDR
* range to use as the endpoint IP address range for the file system. You can have overlapping endpoint IP addresses
* for file systems deployed in the same VPC/route tables, as long as they don't overlap with any subnet.
*
*/
private String endpointIpAddressRange;
/**
*
* The ONTAP administrative password for the fsxadmin
user with which you administer your file system
* using the NetApp ONTAP CLI and REST API.
*
*/
private String fsxAdminPassword;
/**
*
* The SSD IOPS configuration for the FSx for ONTAP file system.
*
*/
private DiskIopsConfiguration diskIopsConfiguration;
/**
*
* Required when DeploymentType
is set to MULTI_AZ_1
or MULTI_AZ_2
. This
* specifies the subnet in which you want the preferred file server to be located.
*
*/
private String preferredSubnetId;
/**
*
* (Multi-AZ only) Specifies the route tables in which Amazon FSx creates the rules for routing traffic to the
* correct file server. You should specify all virtual private cloud (VPC) route tables associated with the subnets
* in which your clients are located. By default, Amazon FSx selects your VPC's default route table.
*
*
*
* Amazon FSx manages these route tables for Multi-AZ file systems using tag-based authentication. These route
* tables are tagged with Key: AmazonFSx; Value: ManagedByAmazonFSx
. When creating FSx for ONTAP
* Multi-AZ file systems using CloudFormation we recommend that you add the
* Key: AmazonFSx; Value: ManagedByAmazonFSx
tag manually.
*
*
*/
private java.util.List routeTableIds;
/**
*
* Sets the throughput capacity for the file system that you're creating in megabytes per second (MBps). For more
* information, see Managing throughput
* capacity in the FSx for ONTAP User Guide.
*
*
* Amazon FSx responds with an HTTP status code 400 (Bad Request) for the following conditions:
*
*
* -
*
* The value of ThroughputCapacity
and ThroughputCapacityPerHAPair
are not the same value.
*
*
* -
*
* The value of ThroughputCapacity
when divided by the value of HAPairs
is outside of the
* valid range for ThroughputCapacity
.
*
*
*
*/
private Integer throughputCapacity;
private String weeklyMaintenanceStartTime;
/**
*
* Specifies how many high-availability (HA) pairs of file servers will power your file system. First-generation
* file systems are powered by 1 HA pair. Second-generation multi-AZ file systems are powered by 1 HA pair. Second
* generation single-AZ file systems are powered by up to 12 HA pairs. The default value is 1. The value of this
* property affects the values of StorageCapacity
, Iops
, and
* ThroughputCapacity
. For more information, see High-availability (HA) pairs in the FSx for ONTAP user guide. Block storage protocol support (iSCSI and NVMe
* over TCP) is disabled on file systems with more than 6 HA pairs. For more information, see Using
* block storage protocols.
*
*
* Amazon FSx responds with an HTTP status code 400 (Bad Request) for the following conditions:
*
*
* -
*
* The value of HAPairs
is less than 1 or greater than 12.
*
*
* -
*
* The value of HAPairs
is greater than 1 and the value of DeploymentType
is
* SINGLE_AZ_1
, MULTI_AZ_1
, or MULTI_AZ_2
.
*
*
*
*/
private Integer hAPairs;
/**
*
* Use to choose the throughput capacity per HA pair, rather than the total throughput for the file system.
*
*
* You can define either the ThroughputCapacityPerHAPair
or the ThroughputCapacity
when
* creating a file system, but not both.
*
*
* This field and ThroughputCapacity
are the same for file systems powered by one HA pair.
*
*
* -
*
* For SINGLE_AZ_1
and MULTI_AZ_1
file systems, valid values are 128, 256, 512, 1024,
* 2048, or 4096 MBps.
*
*
* -
*
* For SINGLE_AZ_2
, valid values are 1536, 3072, or 6144 MBps.
*
*
* -
*
* For MULTI_AZ_2
, valid values are 384, 768, 1536, 3072, or 6144 MBps.
*
*
*
*
* Amazon FSx responds with an HTTP status code 400 (Bad Request) for the following conditions:
*
*
* -
*
* The value of ThroughputCapacity
and ThroughputCapacityPerHAPair
are not the same value
* for file systems with one HA pair.
*
*
* -
*
* The value of deployment type is SINGLE_AZ_2
and ThroughputCapacity
/
* ThroughputCapacityPerHAPair
is not a valid HA pair (a value between 1 and 12).
*
*
* -
*
* The value of ThroughputCapacityPerHAPair
is not a valid value.
*
*
*
*/
private Integer throughputCapacityPerHAPair;
/**
* @param automaticBackupRetentionDays
*/
public void setAutomaticBackupRetentionDays(Integer automaticBackupRetentionDays) {
this.automaticBackupRetentionDays = automaticBackupRetentionDays;
}
/**
* @return
*/
public Integer getAutomaticBackupRetentionDays() {
return this.automaticBackupRetentionDays;
}
/**
* @param automaticBackupRetentionDays
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateFileSystemOntapConfiguration withAutomaticBackupRetentionDays(Integer automaticBackupRetentionDays) {
setAutomaticBackupRetentionDays(automaticBackupRetentionDays);
return this;
}
/**
* @param dailyAutomaticBackupStartTime
*/
public void setDailyAutomaticBackupStartTime(String dailyAutomaticBackupStartTime) {
this.dailyAutomaticBackupStartTime = dailyAutomaticBackupStartTime;
}
/**
* @return
*/
public String getDailyAutomaticBackupStartTime() {
return this.dailyAutomaticBackupStartTime;
}
/**
* @param dailyAutomaticBackupStartTime
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateFileSystemOntapConfiguration withDailyAutomaticBackupStartTime(String dailyAutomaticBackupStartTime) {
setDailyAutomaticBackupStartTime(dailyAutomaticBackupStartTime);
return this;
}
/**
*
* Specifies the FSx for ONTAP file system deployment type to use in creating the file system.
*
*
* -
*
* MULTI_AZ_1
- A high availability file system configured for Multi-AZ redundancy to tolerate
* temporary Availability Zone (AZ) unavailability. This is a first-generation FSx for ONTAP file system.
*
*
* -
*
* MULTI_AZ_2
- A high availability file system configured for Multi-AZ redundancy to tolerate
* temporary AZ unavailability. This is a second-generation FSx for ONTAP file system.
*
*
* -
*
* SINGLE_AZ_1
- A file system configured for Single-AZ redundancy. This is a first-generation FSx for
* ONTAP file system.
*
*
* -
*
* SINGLE_AZ_2
- A file system configured with multiple high-availability (HA) pairs for Single-AZ
* redundancy. This is a second-generation FSx for ONTAP file system.
*
*
*
*
* For information about the use cases for Multi-AZ and Single-AZ deployments, refer to Choosing a file system
* deployment type.
*
*
* @param deploymentType
* Specifies the FSx for ONTAP file system deployment type to use in creating the file system.
*
* -
*
* MULTI_AZ_1
- A high availability file system configured for Multi-AZ redundancy to tolerate
* temporary Availability Zone (AZ) unavailability. This is a first-generation FSx for ONTAP file system.
*
*
* -
*
* MULTI_AZ_2
- A high availability file system configured for Multi-AZ redundancy to tolerate
* temporary AZ unavailability. This is a second-generation FSx for ONTAP file system.
*
*
* -
*
* SINGLE_AZ_1
- A file system configured for Single-AZ redundancy. This is a first-generation
* FSx for ONTAP file system.
*
*
* -
*
* SINGLE_AZ_2
- A file system configured with multiple high-availability (HA) pairs for
* Single-AZ redundancy. This is a second-generation FSx for ONTAP file system.
*
*
*
*
* For information about the use cases for Multi-AZ and Single-AZ deployments, refer to Choosing a file system
* deployment type.
* @see OntapDeploymentType
*/
public void setDeploymentType(String deploymentType) {
this.deploymentType = deploymentType;
}
/**
*
* Specifies the FSx for ONTAP file system deployment type to use in creating the file system.
*
*
* -
*
* MULTI_AZ_1
- A high availability file system configured for Multi-AZ redundancy to tolerate
* temporary Availability Zone (AZ) unavailability. This is a first-generation FSx for ONTAP file system.
*
*
* -
*
* MULTI_AZ_2
- A high availability file system configured for Multi-AZ redundancy to tolerate
* temporary AZ unavailability. This is a second-generation FSx for ONTAP file system.
*
*
* -
*
* SINGLE_AZ_1
- A file system configured for Single-AZ redundancy. This is a first-generation FSx for
* ONTAP file system.
*
*
* -
*
* SINGLE_AZ_2
- A file system configured with multiple high-availability (HA) pairs for Single-AZ
* redundancy. This is a second-generation FSx for ONTAP file system.
*
*
*
*
* For information about the use cases for Multi-AZ and Single-AZ deployments, refer to Choosing a file system
* deployment type.
*
*
* @return Specifies the FSx for ONTAP file system deployment type to use in creating the file system.
*
* -
*
* MULTI_AZ_1
- A high availability file system configured for Multi-AZ redundancy to tolerate
* temporary Availability Zone (AZ) unavailability. This is a first-generation FSx for ONTAP file system.
*
*
* -
*
* MULTI_AZ_2
- A high availability file system configured for Multi-AZ redundancy to tolerate
* temporary AZ unavailability. This is a second-generation FSx for ONTAP file system.
*
*
* -
*
* SINGLE_AZ_1
- A file system configured for Single-AZ redundancy. This is a first-generation
* FSx for ONTAP file system.
*
*
* -
*
* SINGLE_AZ_2
- A file system configured with multiple high-availability (HA) pairs for
* Single-AZ redundancy. This is a second-generation FSx for ONTAP file system.
*
*
*
*
* For information about the use cases for Multi-AZ and Single-AZ deployments, refer to Choosing a file system
* deployment type.
* @see OntapDeploymentType
*/
public String getDeploymentType() {
return this.deploymentType;
}
/**
*
* Specifies the FSx for ONTAP file system deployment type to use in creating the file system.
*
*
* -
*
* MULTI_AZ_1
- A high availability file system configured for Multi-AZ redundancy to tolerate
* temporary Availability Zone (AZ) unavailability. This is a first-generation FSx for ONTAP file system.
*
*
* -
*
* MULTI_AZ_2
- A high availability file system configured for Multi-AZ redundancy to tolerate
* temporary AZ unavailability. This is a second-generation FSx for ONTAP file system.
*
*
* -
*
* SINGLE_AZ_1
- A file system configured for Single-AZ redundancy. This is a first-generation FSx for
* ONTAP file system.
*
*
* -
*
* SINGLE_AZ_2
- A file system configured with multiple high-availability (HA) pairs for Single-AZ
* redundancy. This is a second-generation FSx for ONTAP file system.
*
*
*
*
* For information about the use cases for Multi-AZ and Single-AZ deployments, refer to Choosing a file system
* deployment type.
*
*
* @param deploymentType
* Specifies the FSx for ONTAP file system deployment type to use in creating the file system.
*
* -
*
* MULTI_AZ_1
- A high availability file system configured for Multi-AZ redundancy to tolerate
* temporary Availability Zone (AZ) unavailability. This is a first-generation FSx for ONTAP file system.
*
*
* -
*
* MULTI_AZ_2
- A high availability file system configured for Multi-AZ redundancy to tolerate
* temporary AZ unavailability. This is a second-generation FSx for ONTAP file system.
*
*
* -
*
* SINGLE_AZ_1
- A file system configured for Single-AZ redundancy. This is a first-generation
* FSx for ONTAP file system.
*
*
* -
*
* SINGLE_AZ_2
- A file system configured with multiple high-availability (HA) pairs for
* Single-AZ redundancy. This is a second-generation FSx for ONTAP file system.
*
*
*
*
* For information about the use cases for Multi-AZ and Single-AZ deployments, refer to Choosing a file system
* deployment type.
* @return Returns a reference to this object so that method calls can be chained together.
* @see OntapDeploymentType
*/
public CreateFileSystemOntapConfiguration withDeploymentType(String deploymentType) {
setDeploymentType(deploymentType);
return this;
}
/**
*
* Specifies the FSx for ONTAP file system deployment type to use in creating the file system.
*
*
* -
*
* MULTI_AZ_1
- A high availability file system configured for Multi-AZ redundancy to tolerate
* temporary Availability Zone (AZ) unavailability. This is a first-generation FSx for ONTAP file system.
*
*
* -
*
* MULTI_AZ_2
- A high availability file system configured for Multi-AZ redundancy to tolerate
* temporary AZ unavailability. This is a second-generation FSx for ONTAP file system.
*
*
* -
*
* SINGLE_AZ_1
- A file system configured for Single-AZ redundancy. This is a first-generation FSx for
* ONTAP file system.
*
*
* -
*
* SINGLE_AZ_2
- A file system configured with multiple high-availability (HA) pairs for Single-AZ
* redundancy. This is a second-generation FSx for ONTAP file system.
*
*
*
*
* For information about the use cases for Multi-AZ and Single-AZ deployments, refer to Choosing a file system
* deployment type.
*
*
* @param deploymentType
* Specifies the FSx for ONTAP file system deployment type to use in creating the file system.
*
* -
*
* MULTI_AZ_1
- A high availability file system configured for Multi-AZ redundancy to tolerate
* temporary Availability Zone (AZ) unavailability. This is a first-generation FSx for ONTAP file system.
*
*
* -
*
* MULTI_AZ_2
- A high availability file system configured for Multi-AZ redundancy to tolerate
* temporary AZ unavailability. This is a second-generation FSx for ONTAP file system.
*
*
* -
*
* SINGLE_AZ_1
- A file system configured for Single-AZ redundancy. This is a first-generation
* FSx for ONTAP file system.
*
*
* -
*
* SINGLE_AZ_2
- A file system configured with multiple high-availability (HA) pairs for
* Single-AZ redundancy. This is a second-generation FSx for ONTAP file system.
*
*
*
*
* For information about the use cases for Multi-AZ and Single-AZ deployments, refer to Choosing a file system
* deployment type.
* @return Returns a reference to this object so that method calls can be chained together.
* @see OntapDeploymentType
*/
public CreateFileSystemOntapConfiguration withDeploymentType(OntapDeploymentType deploymentType) {
this.deploymentType = deploymentType.toString();
return this;
}
/**
*
* (Multi-AZ only) Specifies the IP address range in which the endpoints to access your file system will be created.
* By default in the Amazon FSx API, Amazon FSx selects an unused IP address range for you from the 198.19.* range.
* By default in the Amazon FSx console, Amazon FSx chooses the last 64 IP addresses from the VPC’s primary CIDR
* range to use as the endpoint IP address range for the file system. You can have overlapping endpoint IP addresses
* for file systems deployed in the same VPC/route tables, as long as they don't overlap with any subnet.
*
*
* @param endpointIpAddressRange
* (Multi-AZ only) Specifies the IP address range in which the endpoints to access your file system will be
* created. By default in the Amazon FSx API, Amazon FSx selects an unused IP address range for you from the
* 198.19.* range. By default in the Amazon FSx console, Amazon FSx chooses the last 64 IP addresses from the
* VPC’s primary CIDR range to use as the endpoint IP address range for the file system. You can have
* overlapping endpoint IP addresses for file systems deployed in the same VPC/route tables, as long as they
* don't overlap with any subnet.
*/
public void setEndpointIpAddressRange(String endpointIpAddressRange) {
this.endpointIpAddressRange = endpointIpAddressRange;
}
/**
*
* (Multi-AZ only) Specifies the IP address range in which the endpoints to access your file system will be created.
* By default in the Amazon FSx API, Amazon FSx selects an unused IP address range for you from the 198.19.* range.
* By default in the Amazon FSx console, Amazon FSx chooses the last 64 IP addresses from the VPC’s primary CIDR
* range to use as the endpoint IP address range for the file system. You can have overlapping endpoint IP addresses
* for file systems deployed in the same VPC/route tables, as long as they don't overlap with any subnet.
*
*
* @return (Multi-AZ only) Specifies the IP address range in which the endpoints to access your file system will be
* created. By default in the Amazon FSx API, Amazon FSx selects an unused IP address range for you from the
* 198.19.* range. By default in the Amazon FSx console, Amazon FSx chooses the last 64 IP addresses from
* the VPC’s primary CIDR range to use as the endpoint IP address range for the file system. You can have
* overlapping endpoint IP addresses for file systems deployed in the same VPC/route tables, as long as they
* don't overlap with any subnet.
*/
public String getEndpointIpAddressRange() {
return this.endpointIpAddressRange;
}
/**
*
* (Multi-AZ only) Specifies the IP address range in which the endpoints to access your file system will be created.
* By default in the Amazon FSx API, Amazon FSx selects an unused IP address range for you from the 198.19.* range.
* By default in the Amazon FSx console, Amazon FSx chooses the last 64 IP addresses from the VPC’s primary CIDR
* range to use as the endpoint IP address range for the file system. You can have overlapping endpoint IP addresses
* for file systems deployed in the same VPC/route tables, as long as they don't overlap with any subnet.
*
*
* @param endpointIpAddressRange
* (Multi-AZ only) Specifies the IP address range in which the endpoints to access your file system will be
* created. By default in the Amazon FSx API, Amazon FSx selects an unused IP address range for you from the
* 198.19.* range. By default in the Amazon FSx console, Amazon FSx chooses the last 64 IP addresses from the
* VPC’s primary CIDR range to use as the endpoint IP address range for the file system. You can have
* overlapping endpoint IP addresses for file systems deployed in the same VPC/route tables, as long as they
* don't overlap with any subnet.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateFileSystemOntapConfiguration withEndpointIpAddressRange(String endpointIpAddressRange) {
setEndpointIpAddressRange(endpointIpAddressRange);
return this;
}
/**
*
* The ONTAP administrative password for the fsxadmin
user with which you administer your file system
* using the NetApp ONTAP CLI and REST API.
*
*
* @param fsxAdminPassword
* The ONTAP administrative password for the fsxadmin
user with which you administer your file
* system using the NetApp ONTAP CLI and REST API.
*/
public void setFsxAdminPassword(String fsxAdminPassword) {
this.fsxAdminPassword = fsxAdminPassword;
}
/**
*
* The ONTAP administrative password for the fsxadmin
user with which you administer your file system
* using the NetApp ONTAP CLI and REST API.
*
*
* @return The ONTAP administrative password for the fsxadmin
user with which you administer your file
* system using the NetApp ONTAP CLI and REST API.
*/
public String getFsxAdminPassword() {
return this.fsxAdminPassword;
}
/**
*
* The ONTAP administrative password for the fsxadmin
user with which you administer your file system
* using the NetApp ONTAP CLI and REST API.
*
*
* @param fsxAdminPassword
* The ONTAP administrative password for the fsxadmin
user with which you administer your file
* system using the NetApp ONTAP CLI and REST API.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateFileSystemOntapConfiguration withFsxAdminPassword(String fsxAdminPassword) {
setFsxAdminPassword(fsxAdminPassword);
return this;
}
/**
*
* The SSD IOPS configuration for the FSx for ONTAP file system.
*
*
* @param diskIopsConfiguration
* The SSD IOPS configuration for the FSx for ONTAP file system.
*/
public void setDiskIopsConfiguration(DiskIopsConfiguration diskIopsConfiguration) {
this.diskIopsConfiguration = diskIopsConfiguration;
}
/**
*
* The SSD IOPS configuration for the FSx for ONTAP file system.
*
*
* @return The SSD IOPS configuration for the FSx for ONTAP file system.
*/
public DiskIopsConfiguration getDiskIopsConfiguration() {
return this.diskIopsConfiguration;
}
/**
*
* The SSD IOPS configuration for the FSx for ONTAP file system.
*
*
* @param diskIopsConfiguration
* The SSD IOPS configuration for the FSx for ONTAP file system.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateFileSystemOntapConfiguration withDiskIopsConfiguration(DiskIopsConfiguration diskIopsConfiguration) {
setDiskIopsConfiguration(diskIopsConfiguration);
return this;
}
/**
*
* Required when DeploymentType
is set to MULTI_AZ_1
or MULTI_AZ_2
. This
* specifies the subnet in which you want the preferred file server to be located.
*
*
* @param preferredSubnetId
* Required when DeploymentType
is set to MULTI_AZ_1
or MULTI_AZ_2
.
* This specifies the subnet in which you want the preferred file server to be located.
*/
public void setPreferredSubnetId(String preferredSubnetId) {
this.preferredSubnetId = preferredSubnetId;
}
/**
*
* Required when DeploymentType
is set to MULTI_AZ_1
or MULTI_AZ_2
. This
* specifies the subnet in which you want the preferred file server to be located.
*
*
* @return Required when DeploymentType
is set to MULTI_AZ_1
or MULTI_AZ_2
.
* This specifies the subnet in which you want the preferred file server to be located.
*/
public String getPreferredSubnetId() {
return this.preferredSubnetId;
}
/**
*
* Required when DeploymentType
is set to MULTI_AZ_1
or MULTI_AZ_2
. This
* specifies the subnet in which you want the preferred file server to be located.
*
*
* @param preferredSubnetId
* Required when DeploymentType
is set to MULTI_AZ_1
or MULTI_AZ_2
.
* This specifies the subnet in which you want the preferred file server to be located.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateFileSystemOntapConfiguration withPreferredSubnetId(String preferredSubnetId) {
setPreferredSubnetId(preferredSubnetId);
return this;
}
/**
*
* (Multi-AZ only) Specifies the route tables in which Amazon FSx creates the rules for routing traffic to the
* correct file server. You should specify all virtual private cloud (VPC) route tables associated with the subnets
* in which your clients are located. By default, Amazon FSx selects your VPC's default route table.
*
*
*
* Amazon FSx manages these route tables for Multi-AZ file systems using tag-based authentication. These route
* tables are tagged with Key: AmazonFSx; Value: ManagedByAmazonFSx
. When creating FSx for ONTAP
* Multi-AZ file systems using CloudFormation we recommend that you add the
* Key: AmazonFSx; Value: ManagedByAmazonFSx
tag manually.
*
*
*
* @return (Multi-AZ only) Specifies the route tables in which Amazon FSx creates the rules for routing traffic to
* the correct file server. You should specify all virtual private cloud (VPC) route tables associated with
* the subnets in which your clients are located. By default, Amazon FSx selects your VPC's default route
* table.
*
* Amazon FSx manages these route tables for Multi-AZ file systems using tag-based authentication. These
* route tables are tagged with Key: AmazonFSx; Value: ManagedByAmazonFSx
. When creating FSx
* for ONTAP Multi-AZ file systems using CloudFormation we recommend that you add the
* Key: AmazonFSx; Value: ManagedByAmazonFSx
tag manually.
*
*/
public java.util.List getRouteTableIds() {
return routeTableIds;
}
/**
*
* (Multi-AZ only) Specifies the route tables in which Amazon FSx creates the rules for routing traffic to the
* correct file server. You should specify all virtual private cloud (VPC) route tables associated with the subnets
* in which your clients are located. By default, Amazon FSx selects your VPC's default route table.
*
*
*
* Amazon FSx manages these route tables for Multi-AZ file systems using tag-based authentication. These route
* tables are tagged with Key: AmazonFSx; Value: ManagedByAmazonFSx
. When creating FSx for ONTAP
* Multi-AZ file systems using CloudFormation we recommend that you add the
* Key: AmazonFSx; Value: ManagedByAmazonFSx
tag manually.
*
*
*
* @param routeTableIds
* (Multi-AZ only) Specifies the route tables in which Amazon FSx creates the rules for routing traffic to
* the correct file server. You should specify all virtual private cloud (VPC) route tables associated with
* the subnets in which your clients are located. By default, Amazon FSx selects your VPC's default route
* table.
*
* Amazon FSx manages these route tables for Multi-AZ file systems using tag-based authentication. These
* route tables are tagged with Key: AmazonFSx; Value: ManagedByAmazonFSx
. When creating FSx for
* ONTAP Multi-AZ file systems using CloudFormation we recommend that you add the
* Key: AmazonFSx; Value: ManagedByAmazonFSx
tag manually.
*
*/
public void setRouteTableIds(java.util.Collection routeTableIds) {
if (routeTableIds == null) {
this.routeTableIds = null;
return;
}
this.routeTableIds = new java.util.ArrayList(routeTableIds);
}
/**
*
* (Multi-AZ only) Specifies the route tables in which Amazon FSx creates the rules for routing traffic to the
* correct file server. You should specify all virtual private cloud (VPC) route tables associated with the subnets
* in which your clients are located. By default, Amazon FSx selects your VPC's default route table.
*
*
*
* Amazon FSx manages these route tables for Multi-AZ file systems using tag-based authentication. These route
* tables are tagged with Key: AmazonFSx; Value: ManagedByAmazonFSx
. When creating FSx for ONTAP
* Multi-AZ file systems using CloudFormation we recommend that you add the
* Key: AmazonFSx; Value: ManagedByAmazonFSx
tag manually.
*
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setRouteTableIds(java.util.Collection)} or {@link #withRouteTableIds(java.util.Collection)} if you want
* to override the existing values.
*
*
* @param routeTableIds
* (Multi-AZ only) Specifies the route tables in which Amazon FSx creates the rules for routing traffic to
* the correct file server. You should specify all virtual private cloud (VPC) route tables associated with
* the subnets in which your clients are located. By default, Amazon FSx selects your VPC's default route
* table.
*
* Amazon FSx manages these route tables for Multi-AZ file systems using tag-based authentication. These
* route tables are tagged with Key: AmazonFSx; Value: ManagedByAmazonFSx
. When creating FSx for
* ONTAP Multi-AZ file systems using CloudFormation we recommend that you add the
* Key: AmazonFSx; Value: ManagedByAmazonFSx
tag manually.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateFileSystemOntapConfiguration withRouteTableIds(String... routeTableIds) {
if (this.routeTableIds == null) {
setRouteTableIds(new java.util.ArrayList(routeTableIds.length));
}
for (String ele : routeTableIds) {
this.routeTableIds.add(ele);
}
return this;
}
/**
*
* (Multi-AZ only) Specifies the route tables in which Amazon FSx creates the rules for routing traffic to the
* correct file server. You should specify all virtual private cloud (VPC) route tables associated with the subnets
* in which your clients are located. By default, Amazon FSx selects your VPC's default route table.
*
*
*
* Amazon FSx manages these route tables for Multi-AZ file systems using tag-based authentication. These route
* tables are tagged with Key: AmazonFSx; Value: ManagedByAmazonFSx
. When creating FSx for ONTAP
* Multi-AZ file systems using CloudFormation we recommend that you add the
* Key: AmazonFSx; Value: ManagedByAmazonFSx
tag manually.
*
*
*
* @param routeTableIds
* (Multi-AZ only) Specifies the route tables in which Amazon FSx creates the rules for routing traffic to
* the correct file server. You should specify all virtual private cloud (VPC) route tables associated with
* the subnets in which your clients are located. By default, Amazon FSx selects your VPC's default route
* table.
*
* Amazon FSx manages these route tables for Multi-AZ file systems using tag-based authentication. These
* route tables are tagged with Key: AmazonFSx; Value: ManagedByAmazonFSx
. When creating FSx for
* ONTAP Multi-AZ file systems using CloudFormation we recommend that you add the
* Key: AmazonFSx; Value: ManagedByAmazonFSx
tag manually.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateFileSystemOntapConfiguration withRouteTableIds(java.util.Collection routeTableIds) {
setRouteTableIds(routeTableIds);
return this;
}
/**
*
* Sets the throughput capacity for the file system that you're creating in megabytes per second (MBps). For more
* information, see Managing throughput
* capacity in the FSx for ONTAP User Guide.
*
*
* Amazon FSx responds with an HTTP status code 400 (Bad Request) for the following conditions:
*
*
* -
*
* The value of ThroughputCapacity
and ThroughputCapacityPerHAPair
are not the same value.
*
*
* -
*
* The value of ThroughputCapacity
when divided by the value of HAPairs
is outside of the
* valid range for ThroughputCapacity
.
*
*
*
*
* @param throughputCapacity
* Sets the throughput capacity for the file system that you're creating in megabytes per second (MBps). For
* more information, see Managing
* throughput capacity in the FSx for ONTAP User Guide.
*
* Amazon FSx responds with an HTTP status code 400 (Bad Request) for the following conditions:
*
*
* -
*
* The value of ThroughputCapacity
and ThroughputCapacityPerHAPair
are not the same
* value.
*
*
* -
*
* The value of ThroughputCapacity
when divided by the value of HAPairs
is outside
* of the valid range for ThroughputCapacity
.
*
*
*/
public void setThroughputCapacity(Integer throughputCapacity) {
this.throughputCapacity = throughputCapacity;
}
/**
*
* Sets the throughput capacity for the file system that you're creating in megabytes per second (MBps). For more
* information, see Managing throughput
* capacity in the FSx for ONTAP User Guide.
*
*
* Amazon FSx responds with an HTTP status code 400 (Bad Request) for the following conditions:
*
*
* -
*
* The value of ThroughputCapacity
and ThroughputCapacityPerHAPair
are not the same value.
*
*
* -
*
* The value of ThroughputCapacity
when divided by the value of HAPairs
is outside of the
* valid range for ThroughputCapacity
.
*
*
*
*
* @return Sets the throughput capacity for the file system that you're creating in megabytes per second (MBps). For
* more information, see Managing
* throughput capacity in the FSx for ONTAP User Guide.
*
* Amazon FSx responds with an HTTP status code 400 (Bad Request) for the following conditions:
*
*
* -
*
* The value of ThroughputCapacity
and ThroughputCapacityPerHAPair
are not the
* same value.
*
*
* -
*
* The value of ThroughputCapacity
when divided by the value of HAPairs
is outside
* of the valid range for ThroughputCapacity
.
*
*
*/
public Integer getThroughputCapacity() {
return this.throughputCapacity;
}
/**
*
* Sets the throughput capacity for the file system that you're creating in megabytes per second (MBps). For more
* information, see Managing throughput
* capacity in the FSx for ONTAP User Guide.
*
*
* Amazon FSx responds with an HTTP status code 400 (Bad Request) for the following conditions:
*
*
* -
*
* The value of ThroughputCapacity
and ThroughputCapacityPerHAPair
are not the same value.
*
*
* -
*
* The value of ThroughputCapacity
when divided by the value of HAPairs
is outside of the
* valid range for ThroughputCapacity
.
*
*
*
*
* @param throughputCapacity
* Sets the throughput capacity for the file system that you're creating in megabytes per second (MBps). For
* more information, see Managing
* throughput capacity in the FSx for ONTAP User Guide.
*
* Amazon FSx responds with an HTTP status code 400 (Bad Request) for the following conditions:
*
*
* -
*
* The value of ThroughputCapacity
and ThroughputCapacityPerHAPair
are not the same
* value.
*
*
* -
*
* The value of ThroughputCapacity
when divided by the value of HAPairs
is outside
* of the valid range for ThroughputCapacity
.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateFileSystemOntapConfiguration withThroughputCapacity(Integer throughputCapacity) {
setThroughputCapacity(throughputCapacity);
return this;
}
/**
* @param weeklyMaintenanceStartTime
*/
public void setWeeklyMaintenanceStartTime(String weeklyMaintenanceStartTime) {
this.weeklyMaintenanceStartTime = weeklyMaintenanceStartTime;
}
/**
* @return
*/
public String getWeeklyMaintenanceStartTime() {
return this.weeklyMaintenanceStartTime;
}
/**
* @param weeklyMaintenanceStartTime
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateFileSystemOntapConfiguration withWeeklyMaintenanceStartTime(String weeklyMaintenanceStartTime) {
setWeeklyMaintenanceStartTime(weeklyMaintenanceStartTime);
return this;
}
/**
*
* Specifies how many high-availability (HA) pairs of file servers will power your file system. First-generation
* file systems are powered by 1 HA pair. Second-generation multi-AZ file systems are powered by 1 HA pair. Second
* generation single-AZ file systems are powered by up to 12 HA pairs. The default value is 1. The value of this
* property affects the values of StorageCapacity
, Iops
, and
* ThroughputCapacity
. For more information, see High-availability (HA) pairs in the FSx for ONTAP user guide. Block storage protocol support (iSCSI and NVMe
* over TCP) is disabled on file systems with more than 6 HA pairs. For more information, see Using
* block storage protocols.
*
*
* Amazon FSx responds with an HTTP status code 400 (Bad Request) for the following conditions:
*
*
* -
*
* The value of HAPairs
is less than 1 or greater than 12.
*
*
* -
*
* The value of HAPairs
is greater than 1 and the value of DeploymentType
is
* SINGLE_AZ_1
, MULTI_AZ_1
, or MULTI_AZ_2
.
*
*
*
*
* @param hAPairs
* Specifies how many high-availability (HA) pairs of file servers will power your file system.
* First-generation file systems are powered by 1 HA pair. Second-generation multi-AZ file systems are
* powered by 1 HA pair. Second generation single-AZ file systems are powered by up to 12 HA pairs. The
* default value is 1. The value of this property affects the values of StorageCapacity
,
* Iops
, and ThroughputCapacity
. For more information, see High-availability (HA) pairs in the FSx for ONTAP user guide. Block storage protocol support (iSCSI
* and NVMe over TCP) is disabled on file systems with more than 6 HA pairs. For more information, see Using
* block storage protocols.
*
* Amazon FSx responds with an HTTP status code 400 (Bad Request) for the following conditions:
*
*
* -
*
* The value of HAPairs
is less than 1 or greater than 12.
*
*
* -
*
* The value of HAPairs
is greater than 1 and the value of DeploymentType
is
* SINGLE_AZ_1
, MULTI_AZ_1
, or MULTI_AZ_2
.
*
*
*/
public void setHAPairs(Integer hAPairs) {
this.hAPairs = hAPairs;
}
/**
*
* Specifies how many high-availability (HA) pairs of file servers will power your file system. First-generation
* file systems are powered by 1 HA pair. Second-generation multi-AZ file systems are powered by 1 HA pair. Second
* generation single-AZ file systems are powered by up to 12 HA pairs. The default value is 1. The value of this
* property affects the values of StorageCapacity
, Iops
, and
* ThroughputCapacity
. For more information, see High-availability (HA) pairs in the FSx for ONTAP user guide. Block storage protocol support (iSCSI and NVMe
* over TCP) is disabled on file systems with more than 6 HA pairs. For more information, see Using
* block storage protocols.
*
*
* Amazon FSx responds with an HTTP status code 400 (Bad Request) for the following conditions:
*
*
* -
*
* The value of HAPairs
is less than 1 or greater than 12.
*
*
* -
*
* The value of HAPairs
is greater than 1 and the value of DeploymentType
is
* SINGLE_AZ_1
, MULTI_AZ_1
, or MULTI_AZ_2
.
*
*
*
*
* @return Specifies how many high-availability (HA) pairs of file servers will power your file system.
* First-generation file systems are powered by 1 HA pair. Second-generation multi-AZ file systems are
* powered by 1 HA pair. Second generation single-AZ file systems are powered by up to 12 HA pairs. The
* default value is 1. The value of this property affects the values of StorageCapacity
,
* Iops
, and ThroughputCapacity
. For more information, see High-availability (HA) pairs in the FSx for ONTAP user guide. Block storage protocol support (iSCSI
* and NVMe over TCP) is disabled on file systems with more than 6 HA pairs. For more information, see
* Using block storage protocols.
*
* Amazon FSx responds with an HTTP status code 400 (Bad Request) for the following conditions:
*
*
* -
*
* The value of HAPairs
is less than 1 or greater than 12.
*
*
* -
*
* The value of HAPairs
is greater than 1 and the value of DeploymentType
is
* SINGLE_AZ_1
, MULTI_AZ_1
, or MULTI_AZ_2
.
*
*
*/
public Integer getHAPairs() {
return this.hAPairs;
}
/**
*
* Specifies how many high-availability (HA) pairs of file servers will power your file system. First-generation
* file systems are powered by 1 HA pair. Second-generation multi-AZ file systems are powered by 1 HA pair. Second
* generation single-AZ file systems are powered by up to 12 HA pairs. The default value is 1. The value of this
* property affects the values of StorageCapacity
, Iops
, and
* ThroughputCapacity
. For more information, see High-availability (HA) pairs in the FSx for ONTAP user guide. Block storage protocol support (iSCSI and NVMe
* over TCP) is disabled on file systems with more than 6 HA pairs. For more information, see Using
* block storage protocols.
*
*
* Amazon FSx responds with an HTTP status code 400 (Bad Request) for the following conditions:
*
*
* -
*
* The value of HAPairs
is less than 1 or greater than 12.
*
*
* -
*
* The value of HAPairs
is greater than 1 and the value of DeploymentType
is
* SINGLE_AZ_1
, MULTI_AZ_1
, or MULTI_AZ_2
.
*
*
*
*
* @param hAPairs
* Specifies how many high-availability (HA) pairs of file servers will power your file system.
* First-generation file systems are powered by 1 HA pair. Second-generation multi-AZ file systems are
* powered by 1 HA pair. Second generation single-AZ file systems are powered by up to 12 HA pairs. The
* default value is 1. The value of this property affects the values of StorageCapacity
,
* Iops
, and ThroughputCapacity
. For more information, see High-availability (HA) pairs in the FSx for ONTAP user guide. Block storage protocol support (iSCSI
* and NVMe over TCP) is disabled on file systems with more than 6 HA pairs. For more information, see Using
* block storage protocols.
*
* Amazon FSx responds with an HTTP status code 400 (Bad Request) for the following conditions:
*
*
* -
*
* The value of HAPairs
is less than 1 or greater than 12.
*
*
* -
*
* The value of HAPairs
is greater than 1 and the value of DeploymentType
is
* SINGLE_AZ_1
, MULTI_AZ_1
, or MULTI_AZ_2
.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateFileSystemOntapConfiguration withHAPairs(Integer hAPairs) {
setHAPairs(hAPairs);
return this;
}
/**
*
* Use to choose the throughput capacity per HA pair, rather than the total throughput for the file system.
*
*
* You can define either the ThroughputCapacityPerHAPair
or the ThroughputCapacity
when
* creating a file system, but not both.
*
*
* This field and ThroughputCapacity
are the same for file systems powered by one HA pair.
*
*
* -
*
* For SINGLE_AZ_1
and MULTI_AZ_1
file systems, valid values are 128, 256, 512, 1024,
* 2048, or 4096 MBps.
*
*
* -
*
* For SINGLE_AZ_2
, valid values are 1536, 3072, or 6144 MBps.
*
*
* -
*
* For MULTI_AZ_2
, valid values are 384, 768, 1536, 3072, or 6144 MBps.
*
*
*
*
* Amazon FSx responds with an HTTP status code 400 (Bad Request) for the following conditions:
*
*
* -
*
* The value of ThroughputCapacity
and ThroughputCapacityPerHAPair
are not the same value
* for file systems with one HA pair.
*
*
* -
*
* The value of deployment type is SINGLE_AZ_2
and ThroughputCapacity
/
* ThroughputCapacityPerHAPair
is not a valid HA pair (a value between 1 and 12).
*
*
* -
*
* The value of ThroughputCapacityPerHAPair
is not a valid value.
*
*
*
*
* @param throughputCapacityPerHAPair
* Use to choose the throughput capacity per HA pair, rather than the total throughput for the file system.
*
*
* You can define either the ThroughputCapacityPerHAPair
or the ThroughputCapacity
* when creating a file system, but not both.
*
*
* This field and ThroughputCapacity
are the same for file systems powered by one HA pair.
*
*
* -
*
* For SINGLE_AZ_1
and MULTI_AZ_1
file systems, valid values are 128, 256, 512,
* 1024, 2048, or 4096 MBps.
*
*
* -
*
* For SINGLE_AZ_2
, valid values are 1536, 3072, or 6144 MBps.
*
*
* -
*
* For MULTI_AZ_2
, valid values are 384, 768, 1536, 3072, or 6144 MBps.
*
*
*
*
* Amazon FSx responds with an HTTP status code 400 (Bad Request) for the following conditions:
*
*
* -
*
* The value of ThroughputCapacity
and ThroughputCapacityPerHAPair
are not the same
* value for file systems with one HA pair.
*
*
* -
*
* The value of deployment type is SINGLE_AZ_2
and ThroughputCapacity
/
* ThroughputCapacityPerHAPair
is not a valid HA pair (a value between 1 and 12).
*
*
* -
*
* The value of ThroughputCapacityPerHAPair
is not a valid value.
*
*
*/
public void setThroughputCapacityPerHAPair(Integer throughputCapacityPerHAPair) {
this.throughputCapacityPerHAPair = throughputCapacityPerHAPair;
}
/**
*
* Use to choose the throughput capacity per HA pair, rather than the total throughput for the file system.
*
*
* You can define either the ThroughputCapacityPerHAPair
or the ThroughputCapacity
when
* creating a file system, but not both.
*
*
* This field and ThroughputCapacity
are the same for file systems powered by one HA pair.
*
*
* -
*
* For SINGLE_AZ_1
and MULTI_AZ_1
file systems, valid values are 128, 256, 512, 1024,
* 2048, or 4096 MBps.
*
*
* -
*
* For SINGLE_AZ_2
, valid values are 1536, 3072, or 6144 MBps.
*
*
* -
*
* For MULTI_AZ_2
, valid values are 384, 768, 1536, 3072, or 6144 MBps.
*
*
*
*
* Amazon FSx responds with an HTTP status code 400 (Bad Request) for the following conditions:
*
*
* -
*
* The value of ThroughputCapacity
and ThroughputCapacityPerHAPair
are not the same value
* for file systems with one HA pair.
*
*
* -
*
* The value of deployment type is SINGLE_AZ_2
and ThroughputCapacity
/
* ThroughputCapacityPerHAPair
is not a valid HA pair (a value between 1 and 12).
*
*
* -
*
* The value of ThroughputCapacityPerHAPair
is not a valid value.
*
*
*
*
* @return Use to choose the throughput capacity per HA pair, rather than the total throughput for the file system.
*
*
* You can define either the ThroughputCapacityPerHAPair
or the ThroughputCapacity
* when creating a file system, but not both.
*
*
* This field and ThroughputCapacity
are the same for file systems powered by one HA pair.
*
*
* -
*
* For SINGLE_AZ_1
and MULTI_AZ_1
file systems, valid values are 128, 256, 512,
* 1024, 2048, or 4096 MBps.
*
*
* -
*
* For SINGLE_AZ_2
, valid values are 1536, 3072, or 6144 MBps.
*
*
* -
*
* For MULTI_AZ_2
, valid values are 384, 768, 1536, 3072, or 6144 MBps.
*
*
*
*
* Amazon FSx responds with an HTTP status code 400 (Bad Request) for the following conditions:
*
*
* -
*
* The value of ThroughputCapacity
and ThroughputCapacityPerHAPair
are not the
* same value for file systems with one HA pair.
*
*
* -
*
* The value of deployment type is SINGLE_AZ_2
and ThroughputCapacity
/
* ThroughputCapacityPerHAPair
is not a valid HA pair (a value between 1 and 12).
*
*
* -
*
* The value of ThroughputCapacityPerHAPair
is not a valid value.
*
*
*/
public Integer getThroughputCapacityPerHAPair() {
return this.throughputCapacityPerHAPair;
}
/**
*
* Use to choose the throughput capacity per HA pair, rather than the total throughput for the file system.
*
*
* You can define either the ThroughputCapacityPerHAPair
or the ThroughputCapacity
when
* creating a file system, but not both.
*
*
* This field and ThroughputCapacity
are the same for file systems powered by one HA pair.
*
*
* -
*
* For SINGLE_AZ_1
and MULTI_AZ_1
file systems, valid values are 128, 256, 512, 1024,
* 2048, or 4096 MBps.
*
*
* -
*
* For SINGLE_AZ_2
, valid values are 1536, 3072, or 6144 MBps.
*
*
* -
*
* For MULTI_AZ_2
, valid values are 384, 768, 1536, 3072, or 6144 MBps.
*
*
*
*
* Amazon FSx responds with an HTTP status code 400 (Bad Request) for the following conditions:
*
*
* -
*
* The value of ThroughputCapacity
and ThroughputCapacityPerHAPair
are not the same value
* for file systems with one HA pair.
*
*
* -
*
* The value of deployment type is SINGLE_AZ_2
and ThroughputCapacity
/
* ThroughputCapacityPerHAPair
is not a valid HA pair (a value between 1 and 12).
*
*
* -
*
* The value of ThroughputCapacityPerHAPair
is not a valid value.
*
*
*
*
* @param throughputCapacityPerHAPair
* Use to choose the throughput capacity per HA pair, rather than the total throughput for the file system.
*
*
* You can define either the ThroughputCapacityPerHAPair
or the ThroughputCapacity
* when creating a file system, but not both.
*
*
* This field and ThroughputCapacity
are the same for file systems powered by one HA pair.
*
*
* -
*
* For SINGLE_AZ_1
and MULTI_AZ_1
file systems, valid values are 128, 256, 512,
* 1024, 2048, or 4096 MBps.
*
*
* -
*
* For SINGLE_AZ_2
, valid values are 1536, 3072, or 6144 MBps.
*
*
* -
*
* For MULTI_AZ_2
, valid values are 384, 768, 1536, 3072, or 6144 MBps.
*
*
*
*
* Amazon FSx responds with an HTTP status code 400 (Bad Request) for the following conditions:
*
*
* -
*
* The value of ThroughputCapacity
and ThroughputCapacityPerHAPair
are not the same
* value for file systems with one HA pair.
*
*
* -
*
* The value of deployment type is SINGLE_AZ_2
and ThroughputCapacity
/
* ThroughputCapacityPerHAPair
is not a valid HA pair (a value between 1 and 12).
*
*
* -
*
* The value of ThroughputCapacityPerHAPair
is not a valid value.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateFileSystemOntapConfiguration withThroughputCapacityPerHAPair(Integer throughputCapacityPerHAPair) {
setThroughputCapacityPerHAPair(throughputCapacityPerHAPair);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getAutomaticBackupRetentionDays() != null)
sb.append("AutomaticBackupRetentionDays: ").append(getAutomaticBackupRetentionDays()).append(",");
if (getDailyAutomaticBackupStartTime() != null)
sb.append("DailyAutomaticBackupStartTime: ").append(getDailyAutomaticBackupStartTime()).append(",");
if (getDeploymentType() != null)
sb.append("DeploymentType: ").append(getDeploymentType()).append(",");
if (getEndpointIpAddressRange() != null)
sb.append("EndpointIpAddressRange: ").append(getEndpointIpAddressRange()).append(",");
if (getFsxAdminPassword() != null)
sb.append("FsxAdminPassword: ").append("***Sensitive Data Redacted***").append(",");
if (getDiskIopsConfiguration() != null)
sb.append("DiskIopsConfiguration: ").append(getDiskIopsConfiguration()).append(",");
if (getPreferredSubnetId() != null)
sb.append("PreferredSubnetId: ").append(getPreferredSubnetId()).append(",");
if (getRouteTableIds() != null)
sb.append("RouteTableIds: ").append(getRouteTableIds()).append(",");
if (getThroughputCapacity() != null)
sb.append("ThroughputCapacity: ").append(getThroughputCapacity()).append(",");
if (getWeeklyMaintenanceStartTime() != null)
sb.append("WeeklyMaintenanceStartTime: ").append(getWeeklyMaintenanceStartTime()).append(",");
if (getHAPairs() != null)
sb.append("HAPairs: ").append(getHAPairs()).append(",");
if (getThroughputCapacityPerHAPair() != null)
sb.append("ThroughputCapacityPerHAPair: ").append(getThroughputCapacityPerHAPair());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof CreateFileSystemOntapConfiguration == false)
return false;
CreateFileSystemOntapConfiguration other = (CreateFileSystemOntapConfiguration) obj;
if (other.getAutomaticBackupRetentionDays() == null ^ this.getAutomaticBackupRetentionDays() == null)
return false;
if (other.getAutomaticBackupRetentionDays() != null && other.getAutomaticBackupRetentionDays().equals(this.getAutomaticBackupRetentionDays()) == false)
return false;
if (other.getDailyAutomaticBackupStartTime() == null ^ this.getDailyAutomaticBackupStartTime() == null)
return false;
if (other.getDailyAutomaticBackupStartTime() != null
&& other.getDailyAutomaticBackupStartTime().equals(this.getDailyAutomaticBackupStartTime()) == false)
return false;
if (other.getDeploymentType() == null ^ this.getDeploymentType() == null)
return false;
if (other.getDeploymentType() != null && other.getDeploymentType().equals(this.getDeploymentType()) == false)
return false;
if (other.getEndpointIpAddressRange() == null ^ this.getEndpointIpAddressRange() == null)
return false;
if (other.getEndpointIpAddressRange() != null && other.getEndpointIpAddressRange().equals(this.getEndpointIpAddressRange()) == false)
return false;
if (other.getFsxAdminPassword() == null ^ this.getFsxAdminPassword() == null)
return false;
if (other.getFsxAdminPassword() != null && other.getFsxAdminPassword().equals(this.getFsxAdminPassword()) == false)
return false;
if (other.getDiskIopsConfiguration() == null ^ this.getDiskIopsConfiguration() == null)
return false;
if (other.getDiskIopsConfiguration() != null && other.getDiskIopsConfiguration().equals(this.getDiskIopsConfiguration()) == false)
return false;
if (other.getPreferredSubnetId() == null ^ this.getPreferredSubnetId() == null)
return false;
if (other.getPreferredSubnetId() != null && other.getPreferredSubnetId().equals(this.getPreferredSubnetId()) == false)
return false;
if (other.getRouteTableIds() == null ^ this.getRouteTableIds() == null)
return false;
if (other.getRouteTableIds() != null && other.getRouteTableIds().equals(this.getRouteTableIds()) == false)
return false;
if (other.getThroughputCapacity() == null ^ this.getThroughputCapacity() == null)
return false;
if (other.getThroughputCapacity() != null && other.getThroughputCapacity().equals(this.getThroughputCapacity()) == false)
return false;
if (other.getWeeklyMaintenanceStartTime() == null ^ this.getWeeklyMaintenanceStartTime() == null)
return false;
if (other.getWeeklyMaintenanceStartTime() != null && other.getWeeklyMaintenanceStartTime().equals(this.getWeeklyMaintenanceStartTime()) == false)
return false;
if (other.getHAPairs() == null ^ this.getHAPairs() == null)
return false;
if (other.getHAPairs() != null && other.getHAPairs().equals(this.getHAPairs()) == false)
return false;
if (other.getThroughputCapacityPerHAPair() == null ^ this.getThroughputCapacityPerHAPair() == null)
return false;
if (other.getThroughputCapacityPerHAPair() != null && other.getThroughputCapacityPerHAPair().equals(this.getThroughputCapacityPerHAPair()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getAutomaticBackupRetentionDays() == null) ? 0 : getAutomaticBackupRetentionDays().hashCode());
hashCode = prime * hashCode + ((getDailyAutomaticBackupStartTime() == null) ? 0 : getDailyAutomaticBackupStartTime().hashCode());
hashCode = prime * hashCode + ((getDeploymentType() == null) ? 0 : getDeploymentType().hashCode());
hashCode = prime * hashCode + ((getEndpointIpAddressRange() == null) ? 0 : getEndpointIpAddressRange().hashCode());
hashCode = prime * hashCode + ((getFsxAdminPassword() == null) ? 0 : getFsxAdminPassword().hashCode());
hashCode = prime * hashCode + ((getDiskIopsConfiguration() == null) ? 0 : getDiskIopsConfiguration().hashCode());
hashCode = prime * hashCode + ((getPreferredSubnetId() == null) ? 0 : getPreferredSubnetId().hashCode());
hashCode = prime * hashCode + ((getRouteTableIds() == null) ? 0 : getRouteTableIds().hashCode());
hashCode = prime * hashCode + ((getThroughputCapacity() == null) ? 0 : getThroughputCapacity().hashCode());
hashCode = prime * hashCode + ((getWeeklyMaintenanceStartTime() == null) ? 0 : getWeeklyMaintenanceStartTime().hashCode());
hashCode = prime * hashCode + ((getHAPairs() == null) ? 0 : getHAPairs().hashCode());
hashCode = prime * hashCode + ((getThroughputCapacityPerHAPair() == null) ? 0 : getThroughputCapacityPerHAPair().hashCode());
return hashCode;
}
@Override
public CreateFileSystemOntapConfiguration clone() {
try {
return (CreateFileSystemOntapConfiguration) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.fsx.model.transform.CreateFileSystemOntapConfigurationMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}