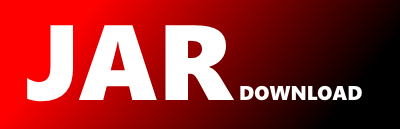
com.amazonaws.services.fsx.model.CreateSnaplockConfiguration Maven / Gradle / Ivy
Show all versions of aws-java-sdk-fsx Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.fsx.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Defines the SnapLock configuration when creating an FSx for ONTAP SnapLock volume.
*
*
* @see AWS
* API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class CreateSnaplockConfiguration implements Serializable, Cloneable, StructuredPojo {
/**
*
* Enables or disables the audit log volume for an FSx for ONTAP SnapLock volume. The default value is
* false
. If you set AuditLogVolume
to true
, the SnapLock volume is created
* as an audit log volume. The minimum retention period for an audit log volume is six months.
*
*
* For more information, see
* SnapLock audit log volumes.
*
*/
private Boolean auditLogVolume;
/**
*
* The configuration object for setting the autocommit period of files in an FSx for ONTAP SnapLock volume.
*
*/
private AutocommitPeriod autocommitPeriod;
/**
*
* Enables, disables, or permanently disables privileged delete on an FSx for ONTAP SnapLock Enterprise volume.
* Enabling privileged delete allows SnapLock administrators to delete WORM files even if they have active retention
* periods. PERMANENTLY_DISABLED
is a terminal state. If privileged delete is permanently disabled on a
* SnapLock volume, you can't re-enable it. The default value is DISABLED
.
*
*
* For more information, see Privileged
* delete.
*
*/
private String privilegedDelete;
/**
*
* Specifies the retention period of an FSx for ONTAP SnapLock volume.
*
*/
private SnaplockRetentionPeriod retentionPeriod;
/**
*
* Specifies the retention mode of an FSx for ONTAP SnapLock volume. After it is set, it can't be changed. You can
* choose one of the following retention modes:
*
*
* -
*
* COMPLIANCE
: Files transitioned to write once, read many (WORM) on a Compliance volume can't be
* deleted until their retention periods expire. This retention mode is used to address government or
* industry-specific mandates or to protect against ransomware attacks. For more information, see SnapLock Compliance.
*
*
* -
*
* ENTERPRISE
: Files transitioned to WORM on an Enterprise volume can be deleted by authorized users
* before their retention periods expire using privileged delete. This retention mode is used to advance an
* organization's data integrity and internal compliance or to test retention settings before using SnapLock
* Compliance. For more information, see SnapLock Enterprise.
*
*
*
*/
private String snaplockType;
/**
*
* Enables or disables volume-append mode on an FSx for ONTAP SnapLock volume. Volume-append mode allows you to
* create WORM-appendable files and write data to them incrementally. The default value is false
.
*
*
* For more information, see Volume-append
* mode.
*
*/
private Boolean volumeAppendModeEnabled;
/**
*
* Enables or disables the audit log volume for an FSx for ONTAP SnapLock volume. The default value is
* false
. If you set AuditLogVolume
to true
, the SnapLock volume is created
* as an audit log volume. The minimum retention period for an audit log volume is six months.
*
*
* For more information, see
* SnapLock audit log volumes.
*
*
* @param auditLogVolume
* Enables or disables the audit log volume for an FSx for ONTAP SnapLock volume. The default value is
* false
. If you set AuditLogVolume
to true
, the SnapLock volume is
* created as an audit log volume. The minimum retention period for an audit log volume is six months.
*
* For more information, see
* SnapLock audit log volumes.
*/
public void setAuditLogVolume(Boolean auditLogVolume) {
this.auditLogVolume = auditLogVolume;
}
/**
*
* Enables or disables the audit log volume for an FSx for ONTAP SnapLock volume. The default value is
* false
. If you set AuditLogVolume
to true
, the SnapLock volume is created
* as an audit log volume. The minimum retention period for an audit log volume is six months.
*
*
* For more information, see
* SnapLock audit log volumes.
*
*
* @return Enables or disables the audit log volume for an FSx for ONTAP SnapLock volume. The default value is
* false
. If you set AuditLogVolume
to true
, the SnapLock volume is
* created as an audit log volume. The minimum retention period for an audit log volume is six months.
*
* For more information, see
* SnapLock audit log volumes.
*/
public Boolean getAuditLogVolume() {
return this.auditLogVolume;
}
/**
*
* Enables or disables the audit log volume for an FSx for ONTAP SnapLock volume. The default value is
* false
. If you set AuditLogVolume
to true
, the SnapLock volume is created
* as an audit log volume. The minimum retention period for an audit log volume is six months.
*
*
* For more information, see
* SnapLock audit log volumes.
*
*
* @param auditLogVolume
* Enables or disables the audit log volume for an FSx for ONTAP SnapLock volume. The default value is
* false
. If you set AuditLogVolume
to true
, the SnapLock volume is
* created as an audit log volume. The minimum retention period for an audit log volume is six months.
*
* For more information, see
* SnapLock audit log volumes.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateSnaplockConfiguration withAuditLogVolume(Boolean auditLogVolume) {
setAuditLogVolume(auditLogVolume);
return this;
}
/**
*
* Enables or disables the audit log volume for an FSx for ONTAP SnapLock volume. The default value is
* false
. If you set AuditLogVolume
to true
, the SnapLock volume is created
* as an audit log volume. The minimum retention period for an audit log volume is six months.
*
*
* For more information, see
* SnapLock audit log volumes.
*
*
* @return Enables or disables the audit log volume for an FSx for ONTAP SnapLock volume. The default value is
* false
. If you set AuditLogVolume
to true
, the SnapLock volume is
* created as an audit log volume. The minimum retention period for an audit log volume is six months.
*
* For more information, see
* SnapLock audit log volumes.
*/
public Boolean isAuditLogVolume() {
return this.auditLogVolume;
}
/**
*
* The configuration object for setting the autocommit period of files in an FSx for ONTAP SnapLock volume.
*
*
* @param autocommitPeriod
* The configuration object for setting the autocommit period of files in an FSx for ONTAP SnapLock volume.
*/
public void setAutocommitPeriod(AutocommitPeriod autocommitPeriod) {
this.autocommitPeriod = autocommitPeriod;
}
/**
*
* The configuration object for setting the autocommit period of files in an FSx for ONTAP SnapLock volume.
*
*
* @return The configuration object for setting the autocommit period of files in an FSx for ONTAP SnapLock volume.
*/
public AutocommitPeriod getAutocommitPeriod() {
return this.autocommitPeriod;
}
/**
*
* The configuration object for setting the autocommit period of files in an FSx for ONTAP SnapLock volume.
*
*
* @param autocommitPeriod
* The configuration object for setting the autocommit period of files in an FSx for ONTAP SnapLock volume.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateSnaplockConfiguration withAutocommitPeriod(AutocommitPeriod autocommitPeriod) {
setAutocommitPeriod(autocommitPeriod);
return this;
}
/**
*
* Enables, disables, or permanently disables privileged delete on an FSx for ONTAP SnapLock Enterprise volume.
* Enabling privileged delete allows SnapLock administrators to delete WORM files even if they have active retention
* periods. PERMANENTLY_DISABLED
is a terminal state. If privileged delete is permanently disabled on a
* SnapLock volume, you can't re-enable it. The default value is DISABLED
.
*
*
* For more information, see Privileged
* delete.
*
*
* @param privilegedDelete
* Enables, disables, or permanently disables privileged delete on an FSx for ONTAP SnapLock Enterprise
* volume. Enabling privileged delete allows SnapLock administrators to delete WORM files even if they have
* active retention periods. PERMANENTLY_DISABLED
is a terminal state. If privileged delete is
* permanently disabled on a SnapLock volume, you can't re-enable it. The default value is
* DISABLED
.
*
* For more information, see Privileged delete.
* @see PrivilegedDelete
*/
public void setPrivilegedDelete(String privilegedDelete) {
this.privilegedDelete = privilegedDelete;
}
/**
*
* Enables, disables, or permanently disables privileged delete on an FSx for ONTAP SnapLock Enterprise volume.
* Enabling privileged delete allows SnapLock administrators to delete WORM files even if they have active retention
* periods. PERMANENTLY_DISABLED
is a terminal state. If privileged delete is permanently disabled on a
* SnapLock volume, you can't re-enable it. The default value is DISABLED
.
*
*
* For more information, see Privileged
* delete.
*
*
* @return Enables, disables, or permanently disables privileged delete on an FSx for ONTAP SnapLock Enterprise
* volume. Enabling privileged delete allows SnapLock administrators to delete WORM files even if they have
* active retention periods. PERMANENTLY_DISABLED
is a terminal state. If privileged delete is
* permanently disabled on a SnapLock volume, you can't re-enable it. The default value is
* DISABLED
.
*
* For more information, see Privileged delete.
* @see PrivilegedDelete
*/
public String getPrivilegedDelete() {
return this.privilegedDelete;
}
/**
*
* Enables, disables, or permanently disables privileged delete on an FSx for ONTAP SnapLock Enterprise volume.
* Enabling privileged delete allows SnapLock administrators to delete WORM files even if they have active retention
* periods. PERMANENTLY_DISABLED
is a terminal state. If privileged delete is permanently disabled on a
* SnapLock volume, you can't re-enable it. The default value is DISABLED
.
*
*
* For more information, see Privileged
* delete.
*
*
* @param privilegedDelete
* Enables, disables, or permanently disables privileged delete on an FSx for ONTAP SnapLock Enterprise
* volume. Enabling privileged delete allows SnapLock administrators to delete WORM files even if they have
* active retention periods. PERMANENTLY_DISABLED
is a terminal state. If privileged delete is
* permanently disabled on a SnapLock volume, you can't re-enable it. The default value is
* DISABLED
.
*
* For more information, see Privileged delete.
* @return Returns a reference to this object so that method calls can be chained together.
* @see PrivilegedDelete
*/
public CreateSnaplockConfiguration withPrivilegedDelete(String privilegedDelete) {
setPrivilegedDelete(privilegedDelete);
return this;
}
/**
*
* Enables, disables, or permanently disables privileged delete on an FSx for ONTAP SnapLock Enterprise volume.
* Enabling privileged delete allows SnapLock administrators to delete WORM files even if they have active retention
* periods. PERMANENTLY_DISABLED
is a terminal state. If privileged delete is permanently disabled on a
* SnapLock volume, you can't re-enable it. The default value is DISABLED
.
*
*
* For more information, see Privileged
* delete.
*
*
* @param privilegedDelete
* Enables, disables, or permanently disables privileged delete on an FSx for ONTAP SnapLock Enterprise
* volume. Enabling privileged delete allows SnapLock administrators to delete WORM files even if they have
* active retention periods. PERMANENTLY_DISABLED
is a terminal state. If privileged delete is
* permanently disabled on a SnapLock volume, you can't re-enable it. The default value is
* DISABLED
.
*
* For more information, see Privileged delete.
* @return Returns a reference to this object so that method calls can be chained together.
* @see PrivilegedDelete
*/
public CreateSnaplockConfiguration withPrivilegedDelete(PrivilegedDelete privilegedDelete) {
this.privilegedDelete = privilegedDelete.toString();
return this;
}
/**
*
* Specifies the retention period of an FSx for ONTAP SnapLock volume.
*
*
* @param retentionPeriod
* Specifies the retention period of an FSx for ONTAP SnapLock volume.
*/
public void setRetentionPeriod(SnaplockRetentionPeriod retentionPeriod) {
this.retentionPeriod = retentionPeriod;
}
/**
*
* Specifies the retention period of an FSx for ONTAP SnapLock volume.
*
*
* @return Specifies the retention period of an FSx for ONTAP SnapLock volume.
*/
public SnaplockRetentionPeriod getRetentionPeriod() {
return this.retentionPeriod;
}
/**
*
* Specifies the retention period of an FSx for ONTAP SnapLock volume.
*
*
* @param retentionPeriod
* Specifies the retention period of an FSx for ONTAP SnapLock volume.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateSnaplockConfiguration withRetentionPeriod(SnaplockRetentionPeriod retentionPeriod) {
setRetentionPeriod(retentionPeriod);
return this;
}
/**
*
* Specifies the retention mode of an FSx for ONTAP SnapLock volume. After it is set, it can't be changed. You can
* choose one of the following retention modes:
*
*
* -
*
* COMPLIANCE
: Files transitioned to write once, read many (WORM) on a Compliance volume can't be
* deleted until their retention periods expire. This retention mode is used to address government or
* industry-specific mandates or to protect against ransomware attacks. For more information, see SnapLock Compliance.
*
*
* -
*
* ENTERPRISE
: Files transitioned to WORM on an Enterprise volume can be deleted by authorized users
* before their retention periods expire using privileged delete. This retention mode is used to advance an
* organization's data integrity and internal compliance or to test retention settings before using SnapLock
* Compliance. For more information, see SnapLock Enterprise.
*
*
*
*
* @param snaplockType
* Specifies the retention mode of an FSx for ONTAP SnapLock volume. After it is set, it can't be changed.
* You can choose one of the following retention modes:
*
* -
*
* COMPLIANCE
: Files transitioned to write once, read many (WORM) on a Compliance volume can't
* be deleted until their retention periods expire. This retention mode is used to address government or
* industry-specific mandates or to protect against ransomware attacks. For more information, see SnapLock Compliance.
*
*
* -
*
* ENTERPRISE
: Files transitioned to WORM on an Enterprise volume can be deleted by authorized
* users before their retention periods expire using privileged delete. This retention mode is used to
* advance an organization's data integrity and internal compliance or to test retention settings before
* using SnapLock Compliance. For more information, see SnapLock Enterprise.
*
*
* @see SnaplockType
*/
public void setSnaplockType(String snaplockType) {
this.snaplockType = snaplockType;
}
/**
*
* Specifies the retention mode of an FSx for ONTAP SnapLock volume. After it is set, it can't be changed. You can
* choose one of the following retention modes:
*
*
* -
*
* COMPLIANCE
: Files transitioned to write once, read many (WORM) on a Compliance volume can't be
* deleted until their retention periods expire. This retention mode is used to address government or
* industry-specific mandates or to protect against ransomware attacks. For more information, see SnapLock Compliance.
*
*
* -
*
* ENTERPRISE
: Files transitioned to WORM on an Enterprise volume can be deleted by authorized users
* before their retention periods expire using privileged delete. This retention mode is used to advance an
* organization's data integrity and internal compliance or to test retention settings before using SnapLock
* Compliance. For more information, see SnapLock Enterprise.
*
*
*
*
* @return Specifies the retention mode of an FSx for ONTAP SnapLock volume. After it is set, it can't be changed.
* You can choose one of the following retention modes:
*
* -
*
* COMPLIANCE
: Files transitioned to write once, read many (WORM) on a Compliance volume can't
* be deleted until their retention periods expire. This retention mode is used to address government or
* industry-specific mandates or to protect against ransomware attacks. For more information, see SnapLock
* Compliance.
*
*
* -
*
* ENTERPRISE
: Files transitioned to WORM on an Enterprise volume can be deleted by authorized
* users before their retention periods expire using privileged delete. This retention mode is used to
* advance an organization's data integrity and internal compliance or to test retention settings before
* using SnapLock Compliance. For more information, see SnapLock
* Enterprise.
*
*
* @see SnaplockType
*/
public String getSnaplockType() {
return this.snaplockType;
}
/**
*
* Specifies the retention mode of an FSx for ONTAP SnapLock volume. After it is set, it can't be changed. You can
* choose one of the following retention modes:
*
*
* -
*
* COMPLIANCE
: Files transitioned to write once, read many (WORM) on a Compliance volume can't be
* deleted until their retention periods expire. This retention mode is used to address government or
* industry-specific mandates or to protect against ransomware attacks. For more information, see SnapLock Compliance.
*
*
* -
*
* ENTERPRISE
: Files transitioned to WORM on an Enterprise volume can be deleted by authorized users
* before their retention periods expire using privileged delete. This retention mode is used to advance an
* organization's data integrity and internal compliance or to test retention settings before using SnapLock
* Compliance. For more information, see SnapLock Enterprise.
*
*
*
*
* @param snaplockType
* Specifies the retention mode of an FSx for ONTAP SnapLock volume. After it is set, it can't be changed.
* You can choose one of the following retention modes:
*
* -
*
* COMPLIANCE
: Files transitioned to write once, read many (WORM) on a Compliance volume can't
* be deleted until their retention periods expire. This retention mode is used to address government or
* industry-specific mandates or to protect against ransomware attacks. For more information, see SnapLock Compliance.
*
*
* -
*
* ENTERPRISE
: Files transitioned to WORM on an Enterprise volume can be deleted by authorized
* users before their retention periods expire using privileged delete. This retention mode is used to
* advance an organization's data integrity and internal compliance or to test retention settings before
* using SnapLock Compliance. For more information, see SnapLock Enterprise.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see SnaplockType
*/
public CreateSnaplockConfiguration withSnaplockType(String snaplockType) {
setSnaplockType(snaplockType);
return this;
}
/**
*
* Specifies the retention mode of an FSx for ONTAP SnapLock volume. After it is set, it can't be changed. You can
* choose one of the following retention modes:
*
*
* -
*
* COMPLIANCE
: Files transitioned to write once, read many (WORM) on a Compliance volume can't be
* deleted until their retention periods expire. This retention mode is used to address government or
* industry-specific mandates or to protect against ransomware attacks. For more information, see SnapLock Compliance.
*
*
* -
*
* ENTERPRISE
: Files transitioned to WORM on an Enterprise volume can be deleted by authorized users
* before their retention periods expire using privileged delete. This retention mode is used to advance an
* organization's data integrity and internal compliance or to test retention settings before using SnapLock
* Compliance. For more information, see SnapLock Enterprise.
*
*
*
*
* @param snaplockType
* Specifies the retention mode of an FSx for ONTAP SnapLock volume. After it is set, it can't be changed.
* You can choose one of the following retention modes:
*
* -
*
* COMPLIANCE
: Files transitioned to write once, read many (WORM) on a Compliance volume can't
* be deleted until their retention periods expire. This retention mode is used to address government or
* industry-specific mandates or to protect against ransomware attacks. For more information, see SnapLock Compliance.
*
*
* -
*
* ENTERPRISE
: Files transitioned to WORM on an Enterprise volume can be deleted by authorized
* users before their retention periods expire using privileged delete. This retention mode is used to
* advance an organization's data integrity and internal compliance or to test retention settings before
* using SnapLock Compliance. For more information, see SnapLock Enterprise.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see SnaplockType
*/
public CreateSnaplockConfiguration withSnaplockType(SnaplockType snaplockType) {
this.snaplockType = snaplockType.toString();
return this;
}
/**
*
* Enables or disables volume-append mode on an FSx for ONTAP SnapLock volume. Volume-append mode allows you to
* create WORM-appendable files and write data to them incrementally. The default value is false
.
*
*
* For more information, see Volume-append
* mode.
*
*
* @param volumeAppendModeEnabled
* Enables or disables volume-append mode on an FSx for ONTAP SnapLock volume. Volume-append mode allows you
* to create WORM-appendable files and write data to them incrementally. The default value is
* false
.
*
* For more information, see Volume-append
* mode.
*/
public void setVolumeAppendModeEnabled(Boolean volumeAppendModeEnabled) {
this.volumeAppendModeEnabled = volumeAppendModeEnabled;
}
/**
*
* Enables or disables volume-append mode on an FSx for ONTAP SnapLock volume. Volume-append mode allows you to
* create WORM-appendable files and write data to them incrementally. The default value is false
.
*
*
* For more information, see Volume-append
* mode.
*
*
* @return Enables or disables volume-append mode on an FSx for ONTAP SnapLock volume. Volume-append mode allows you
* to create WORM-appendable files and write data to them incrementally. The default value is
* false
.
*
* For more information, see Volume-append
* mode.
*/
public Boolean getVolumeAppendModeEnabled() {
return this.volumeAppendModeEnabled;
}
/**
*
* Enables or disables volume-append mode on an FSx for ONTAP SnapLock volume. Volume-append mode allows you to
* create WORM-appendable files and write data to them incrementally. The default value is false
.
*
*
* For more information, see Volume-append
* mode.
*
*
* @param volumeAppendModeEnabled
* Enables or disables volume-append mode on an FSx for ONTAP SnapLock volume. Volume-append mode allows you
* to create WORM-appendable files and write data to them incrementally. The default value is
* false
.
*
* For more information, see Volume-append
* mode.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateSnaplockConfiguration withVolumeAppendModeEnabled(Boolean volumeAppendModeEnabled) {
setVolumeAppendModeEnabled(volumeAppendModeEnabled);
return this;
}
/**
*
* Enables or disables volume-append mode on an FSx for ONTAP SnapLock volume. Volume-append mode allows you to
* create WORM-appendable files and write data to them incrementally. The default value is false
.
*
*
* For more information, see Volume-append
* mode.
*
*
* @return Enables or disables volume-append mode on an FSx for ONTAP SnapLock volume. Volume-append mode allows you
* to create WORM-appendable files and write data to them incrementally. The default value is
* false
.
*
* For more information, see Volume-append
* mode.
*/
public Boolean isVolumeAppendModeEnabled() {
return this.volumeAppendModeEnabled;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getAuditLogVolume() != null)
sb.append("AuditLogVolume: ").append(getAuditLogVolume()).append(",");
if (getAutocommitPeriod() != null)
sb.append("AutocommitPeriod: ").append(getAutocommitPeriod()).append(",");
if (getPrivilegedDelete() != null)
sb.append("PrivilegedDelete: ").append(getPrivilegedDelete()).append(",");
if (getRetentionPeriod() != null)
sb.append("RetentionPeriod: ").append(getRetentionPeriod()).append(",");
if (getSnaplockType() != null)
sb.append("SnaplockType: ").append(getSnaplockType()).append(",");
if (getVolumeAppendModeEnabled() != null)
sb.append("VolumeAppendModeEnabled: ").append(getVolumeAppendModeEnabled());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof CreateSnaplockConfiguration == false)
return false;
CreateSnaplockConfiguration other = (CreateSnaplockConfiguration) obj;
if (other.getAuditLogVolume() == null ^ this.getAuditLogVolume() == null)
return false;
if (other.getAuditLogVolume() != null && other.getAuditLogVolume().equals(this.getAuditLogVolume()) == false)
return false;
if (other.getAutocommitPeriod() == null ^ this.getAutocommitPeriod() == null)
return false;
if (other.getAutocommitPeriod() != null && other.getAutocommitPeriod().equals(this.getAutocommitPeriod()) == false)
return false;
if (other.getPrivilegedDelete() == null ^ this.getPrivilegedDelete() == null)
return false;
if (other.getPrivilegedDelete() != null && other.getPrivilegedDelete().equals(this.getPrivilegedDelete()) == false)
return false;
if (other.getRetentionPeriod() == null ^ this.getRetentionPeriod() == null)
return false;
if (other.getRetentionPeriod() != null && other.getRetentionPeriod().equals(this.getRetentionPeriod()) == false)
return false;
if (other.getSnaplockType() == null ^ this.getSnaplockType() == null)
return false;
if (other.getSnaplockType() != null && other.getSnaplockType().equals(this.getSnaplockType()) == false)
return false;
if (other.getVolumeAppendModeEnabled() == null ^ this.getVolumeAppendModeEnabled() == null)
return false;
if (other.getVolumeAppendModeEnabled() != null && other.getVolumeAppendModeEnabled().equals(this.getVolumeAppendModeEnabled()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getAuditLogVolume() == null) ? 0 : getAuditLogVolume().hashCode());
hashCode = prime * hashCode + ((getAutocommitPeriod() == null) ? 0 : getAutocommitPeriod().hashCode());
hashCode = prime * hashCode + ((getPrivilegedDelete() == null) ? 0 : getPrivilegedDelete().hashCode());
hashCode = prime * hashCode + ((getRetentionPeriod() == null) ? 0 : getRetentionPeriod().hashCode());
hashCode = prime * hashCode + ((getSnaplockType() == null) ? 0 : getSnaplockType().hashCode());
hashCode = prime * hashCode + ((getVolumeAppendModeEnabled() == null) ? 0 : getVolumeAppendModeEnabled().hashCode());
return hashCode;
}
@Override
public CreateSnaplockConfiguration clone() {
try {
return (CreateSnaplockConfiguration) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.fsx.model.transform.CreateSnaplockConfigurationMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}