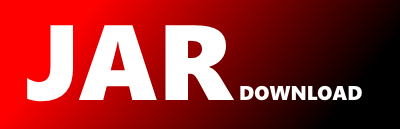
com.amazonaws.services.fsx.model.UpdateFileSystemOpenZFSConfiguration Maven / Gradle / Ivy
Show all versions of aws-java-sdk-fsx Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.fsx.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* The configuration updates for an Amazon FSx for OpenZFS file system.
*
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class UpdateFileSystemOpenZFSConfiguration implements Serializable, Cloneable, StructuredPojo {
private Integer automaticBackupRetentionDays;
/**
*
* A Boolean value indicating whether tags for the file system should be copied to backups. This value defaults to
* false
. If it's set to true
, all tags for the file system are copied to all automatic
* and user-initiated backups where the user doesn't specify tags. If this value is true
and you
* specify one or more tags, only the specified tags are copied to backups. If you specify one or more tags when
* creating a user-initiated backup, no tags are copied from the file system, regardless of this value.
*
*/
private Boolean copyTagsToBackups;
/**
*
* A Boolean value indicating whether tags for the volume should be copied to snapshots. This value defaults to
* false
. If it's set to true
, all tags for the volume are copied to snapshots where the
* user doesn't specify tags. If this value is true
and you specify one or more tags, only the
* specified tags are copied to snapshots. If you specify one or more tags when creating the snapshot, no tags are
* copied from the volume, regardless of this value.
*
*/
private Boolean copyTagsToVolumes;
private String dailyAutomaticBackupStartTime;
/**
*
* The throughput of an Amazon FSx for OpenZFS file system, measured in megabytes per second
(MB/s). Valid
* values depend on the DeploymentType you choose, as follows:
*
*
* -
*
* For MULTI_AZ_1
and SINGLE_AZ_2
, valid values are 160, 320, 640, 1280, 2560, 3840, 5120,
* 7680, or 10240 MB/s.
*
*
* -
*
* For SINGLE_AZ_1
, valid values are 64, 128, 256, 512, 1024, 2048, 3072, or 4096 MB/s.
*
*
*
*/
private Integer throughputCapacity;
private String weeklyMaintenanceStartTime;
private DiskIopsConfiguration diskIopsConfiguration;
/**
*
* (Multi-AZ only) A list of IDs of new virtual private cloud (VPC) route tables to associate (add) with your Amazon
* FSx for OpenZFS file system.
*
*/
private java.util.List addRouteTableIds;
/**
*
* (Multi-AZ only) A list of IDs of existing virtual private cloud (VPC) route tables to disassociate (remove) from
* your Amazon FSx for OpenZFS file system. You can use the API operation to retrieve the list of VPC route table
* IDs for a file system.
*
*/
private java.util.List removeRouteTableIds;
/**
* @param automaticBackupRetentionDays
*/
public void setAutomaticBackupRetentionDays(Integer automaticBackupRetentionDays) {
this.automaticBackupRetentionDays = automaticBackupRetentionDays;
}
/**
* @return
*/
public Integer getAutomaticBackupRetentionDays() {
return this.automaticBackupRetentionDays;
}
/**
* @param automaticBackupRetentionDays
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateFileSystemOpenZFSConfiguration withAutomaticBackupRetentionDays(Integer automaticBackupRetentionDays) {
setAutomaticBackupRetentionDays(automaticBackupRetentionDays);
return this;
}
/**
*
* A Boolean value indicating whether tags for the file system should be copied to backups. This value defaults to
* false
. If it's set to true
, all tags for the file system are copied to all automatic
* and user-initiated backups where the user doesn't specify tags. If this value is true
and you
* specify one or more tags, only the specified tags are copied to backups. If you specify one or more tags when
* creating a user-initiated backup, no tags are copied from the file system, regardless of this value.
*
*
* @param copyTagsToBackups
* A Boolean value indicating whether tags for the file system should be copied to backups. This value
* defaults to false
. If it's set to true
, all tags for the file system are copied
* to all automatic and user-initiated backups where the user doesn't specify tags. If this value is
* true
and you specify one or more tags, only the specified tags are copied to backups. If you
* specify one or more tags when creating a user-initiated backup, no tags are copied from the file system,
* regardless of this value.
*/
public void setCopyTagsToBackups(Boolean copyTagsToBackups) {
this.copyTagsToBackups = copyTagsToBackups;
}
/**
*
* A Boolean value indicating whether tags for the file system should be copied to backups. This value defaults to
* false
. If it's set to true
, all tags for the file system are copied to all automatic
* and user-initiated backups where the user doesn't specify tags. If this value is true
and you
* specify one or more tags, only the specified tags are copied to backups. If you specify one or more tags when
* creating a user-initiated backup, no tags are copied from the file system, regardless of this value.
*
*
* @return A Boolean value indicating whether tags for the file system should be copied to backups. This value
* defaults to false
. If it's set to true
, all tags for the file system are copied
* to all automatic and user-initiated backups where the user doesn't specify tags. If this value is
* true
and you specify one or more tags, only the specified tags are copied to backups. If you
* specify one or more tags when creating a user-initiated backup, no tags are copied from the file system,
* regardless of this value.
*/
public Boolean getCopyTagsToBackups() {
return this.copyTagsToBackups;
}
/**
*
* A Boolean value indicating whether tags for the file system should be copied to backups. This value defaults to
* false
. If it's set to true
, all tags for the file system are copied to all automatic
* and user-initiated backups where the user doesn't specify tags. If this value is true
and you
* specify one or more tags, only the specified tags are copied to backups. If you specify one or more tags when
* creating a user-initiated backup, no tags are copied from the file system, regardless of this value.
*
*
* @param copyTagsToBackups
* A Boolean value indicating whether tags for the file system should be copied to backups. This value
* defaults to false
. If it's set to true
, all tags for the file system are copied
* to all automatic and user-initiated backups where the user doesn't specify tags. If this value is
* true
and you specify one or more tags, only the specified tags are copied to backups. If you
* specify one or more tags when creating a user-initiated backup, no tags are copied from the file system,
* regardless of this value.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateFileSystemOpenZFSConfiguration withCopyTagsToBackups(Boolean copyTagsToBackups) {
setCopyTagsToBackups(copyTagsToBackups);
return this;
}
/**
*
* A Boolean value indicating whether tags for the file system should be copied to backups. This value defaults to
* false
. If it's set to true
, all tags for the file system are copied to all automatic
* and user-initiated backups where the user doesn't specify tags. If this value is true
and you
* specify one or more tags, only the specified tags are copied to backups. If you specify one or more tags when
* creating a user-initiated backup, no tags are copied from the file system, regardless of this value.
*
*
* @return A Boolean value indicating whether tags for the file system should be copied to backups. This value
* defaults to false
. If it's set to true
, all tags for the file system are copied
* to all automatic and user-initiated backups where the user doesn't specify tags. If this value is
* true
and you specify one or more tags, only the specified tags are copied to backups. If you
* specify one or more tags when creating a user-initiated backup, no tags are copied from the file system,
* regardless of this value.
*/
public Boolean isCopyTagsToBackups() {
return this.copyTagsToBackups;
}
/**
*
* A Boolean value indicating whether tags for the volume should be copied to snapshots. This value defaults to
* false
. If it's set to true
, all tags for the volume are copied to snapshots where the
* user doesn't specify tags. If this value is true
and you specify one or more tags, only the
* specified tags are copied to snapshots. If you specify one or more tags when creating the snapshot, no tags are
* copied from the volume, regardless of this value.
*
*
* @param copyTagsToVolumes
* A Boolean value indicating whether tags for the volume should be copied to snapshots. This value defaults
* to false
. If it's set to true
, all tags for the volume are copied to snapshots
* where the user doesn't specify tags. If this value is true
and you specify one or more tags,
* only the specified tags are copied to snapshots. If you specify one or more tags when creating the
* snapshot, no tags are copied from the volume, regardless of this value.
*/
public void setCopyTagsToVolumes(Boolean copyTagsToVolumes) {
this.copyTagsToVolumes = copyTagsToVolumes;
}
/**
*
* A Boolean value indicating whether tags for the volume should be copied to snapshots. This value defaults to
* false
. If it's set to true
, all tags for the volume are copied to snapshots where the
* user doesn't specify tags. If this value is true
and you specify one or more tags, only the
* specified tags are copied to snapshots. If you specify one or more tags when creating the snapshot, no tags are
* copied from the volume, regardless of this value.
*
*
* @return A Boolean value indicating whether tags for the volume should be copied to snapshots. This value defaults
* to false
. If it's set to true
, all tags for the volume are copied to snapshots
* where the user doesn't specify tags. If this value is true
and you specify one or more tags,
* only the specified tags are copied to snapshots. If you specify one or more tags when creating the
* snapshot, no tags are copied from the volume, regardless of this value.
*/
public Boolean getCopyTagsToVolumes() {
return this.copyTagsToVolumes;
}
/**
*
* A Boolean value indicating whether tags for the volume should be copied to snapshots. This value defaults to
* false
. If it's set to true
, all tags for the volume are copied to snapshots where the
* user doesn't specify tags. If this value is true
and you specify one or more tags, only the
* specified tags are copied to snapshots. If you specify one or more tags when creating the snapshot, no tags are
* copied from the volume, regardless of this value.
*
*
* @param copyTagsToVolumes
* A Boolean value indicating whether tags for the volume should be copied to snapshots. This value defaults
* to false
. If it's set to true
, all tags for the volume are copied to snapshots
* where the user doesn't specify tags. If this value is true
and you specify one or more tags,
* only the specified tags are copied to snapshots. If you specify one or more tags when creating the
* snapshot, no tags are copied from the volume, regardless of this value.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateFileSystemOpenZFSConfiguration withCopyTagsToVolumes(Boolean copyTagsToVolumes) {
setCopyTagsToVolumes(copyTagsToVolumes);
return this;
}
/**
*
* A Boolean value indicating whether tags for the volume should be copied to snapshots. This value defaults to
* false
. If it's set to true
, all tags for the volume are copied to snapshots where the
* user doesn't specify tags. If this value is true
and you specify one or more tags, only the
* specified tags are copied to snapshots. If you specify one or more tags when creating the snapshot, no tags are
* copied from the volume, regardless of this value.
*
*
* @return A Boolean value indicating whether tags for the volume should be copied to snapshots. This value defaults
* to false
. If it's set to true
, all tags for the volume are copied to snapshots
* where the user doesn't specify tags. If this value is true
and you specify one or more tags,
* only the specified tags are copied to snapshots. If you specify one or more tags when creating the
* snapshot, no tags are copied from the volume, regardless of this value.
*/
public Boolean isCopyTagsToVolumes() {
return this.copyTagsToVolumes;
}
/**
* @param dailyAutomaticBackupStartTime
*/
public void setDailyAutomaticBackupStartTime(String dailyAutomaticBackupStartTime) {
this.dailyAutomaticBackupStartTime = dailyAutomaticBackupStartTime;
}
/**
* @return
*/
public String getDailyAutomaticBackupStartTime() {
return this.dailyAutomaticBackupStartTime;
}
/**
* @param dailyAutomaticBackupStartTime
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateFileSystemOpenZFSConfiguration withDailyAutomaticBackupStartTime(String dailyAutomaticBackupStartTime) {
setDailyAutomaticBackupStartTime(dailyAutomaticBackupStartTime);
return this;
}
/**
*
* The throughput of an Amazon FSx for OpenZFS file system, measured in megabytes per second
(MB/s). Valid
* values depend on the DeploymentType you choose, as follows:
*
*
* -
*
* For MULTI_AZ_1
and SINGLE_AZ_2
, valid values are 160, 320, 640, 1280, 2560, 3840, 5120,
* 7680, or 10240 MB/s.
*
*
* -
*
* For SINGLE_AZ_1
, valid values are 64, 128, 256, 512, 1024, 2048, 3072, or 4096 MB/s.
*
*
*
*
* @param throughputCapacity
* The throughput of an Amazon FSx for OpenZFS file system, measured in megabytes per second
(MB/s).
* Valid values depend on the DeploymentType you choose, as follows:
*
* -
*
* For MULTI_AZ_1
and SINGLE_AZ_2
, valid values are 160, 320, 640, 1280, 2560,
* 3840, 5120, 7680, or 10240 MB/s.
*
*
* -
*
* For SINGLE_AZ_1
, valid values are 64, 128, 256, 512, 1024, 2048, 3072, or 4096 MB/s.
*
*
*/
public void setThroughputCapacity(Integer throughputCapacity) {
this.throughputCapacity = throughputCapacity;
}
/**
*
* The throughput of an Amazon FSx for OpenZFS file system, measured in megabytes per second
(MB/s). Valid
* values depend on the DeploymentType you choose, as follows:
*
*
* -
*
* For MULTI_AZ_1
and SINGLE_AZ_2
, valid values are 160, 320, 640, 1280, 2560, 3840, 5120,
* 7680, or 10240 MB/s.
*
*
* -
*
* For SINGLE_AZ_1
, valid values are 64, 128, 256, 512, 1024, 2048, 3072, or 4096 MB/s.
*
*
*
*
* @return The throughput of an Amazon FSx for OpenZFS file system, measured in megabytes per second
(MB/s).
* Valid values depend on the DeploymentType you choose, as follows:
*
* -
*
* For MULTI_AZ_1
and SINGLE_AZ_2
, valid values are 160, 320, 640, 1280, 2560,
* 3840, 5120, 7680, or 10240 MB/s.
*
*
* -
*
* For SINGLE_AZ_1
, valid values are 64, 128, 256, 512, 1024, 2048, 3072, or 4096 MB/s.
*
*
*/
public Integer getThroughputCapacity() {
return this.throughputCapacity;
}
/**
*
* The throughput of an Amazon FSx for OpenZFS file system, measured in megabytes per second
(MB/s). Valid
* values depend on the DeploymentType you choose, as follows:
*
*
* -
*
* For MULTI_AZ_1
and SINGLE_AZ_2
, valid values are 160, 320, 640, 1280, 2560, 3840, 5120,
* 7680, or 10240 MB/s.
*
*
* -
*
* For SINGLE_AZ_1
, valid values are 64, 128, 256, 512, 1024, 2048, 3072, or 4096 MB/s.
*
*
*
*
* @param throughputCapacity
* The throughput of an Amazon FSx for OpenZFS file system, measured in megabytes per second
(MB/s).
* Valid values depend on the DeploymentType you choose, as follows:
*
* -
*
* For MULTI_AZ_1
and SINGLE_AZ_2
, valid values are 160, 320, 640, 1280, 2560,
* 3840, 5120, 7680, or 10240 MB/s.
*
*
* -
*
* For SINGLE_AZ_1
, valid values are 64, 128, 256, 512, 1024, 2048, 3072, or 4096 MB/s.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateFileSystemOpenZFSConfiguration withThroughputCapacity(Integer throughputCapacity) {
setThroughputCapacity(throughputCapacity);
return this;
}
/**
* @param weeklyMaintenanceStartTime
*/
public void setWeeklyMaintenanceStartTime(String weeklyMaintenanceStartTime) {
this.weeklyMaintenanceStartTime = weeklyMaintenanceStartTime;
}
/**
* @return
*/
public String getWeeklyMaintenanceStartTime() {
return this.weeklyMaintenanceStartTime;
}
/**
* @param weeklyMaintenanceStartTime
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateFileSystemOpenZFSConfiguration withWeeklyMaintenanceStartTime(String weeklyMaintenanceStartTime) {
setWeeklyMaintenanceStartTime(weeklyMaintenanceStartTime);
return this;
}
/**
* @param diskIopsConfiguration
*/
public void setDiskIopsConfiguration(DiskIopsConfiguration diskIopsConfiguration) {
this.diskIopsConfiguration = diskIopsConfiguration;
}
/**
* @return
*/
public DiskIopsConfiguration getDiskIopsConfiguration() {
return this.diskIopsConfiguration;
}
/**
* @param diskIopsConfiguration
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateFileSystemOpenZFSConfiguration withDiskIopsConfiguration(DiskIopsConfiguration diskIopsConfiguration) {
setDiskIopsConfiguration(diskIopsConfiguration);
return this;
}
/**
*
* (Multi-AZ only) A list of IDs of new virtual private cloud (VPC) route tables to associate (add) with your Amazon
* FSx for OpenZFS file system.
*
*
* @return (Multi-AZ only) A list of IDs of new virtual private cloud (VPC) route tables to associate (add) with
* your Amazon FSx for OpenZFS file system.
*/
public java.util.List getAddRouteTableIds() {
return addRouteTableIds;
}
/**
*
* (Multi-AZ only) A list of IDs of new virtual private cloud (VPC) route tables to associate (add) with your Amazon
* FSx for OpenZFS file system.
*
*
* @param addRouteTableIds
* (Multi-AZ only) A list of IDs of new virtual private cloud (VPC) route tables to associate (add) with your
* Amazon FSx for OpenZFS file system.
*/
public void setAddRouteTableIds(java.util.Collection addRouteTableIds) {
if (addRouteTableIds == null) {
this.addRouteTableIds = null;
return;
}
this.addRouteTableIds = new java.util.ArrayList(addRouteTableIds);
}
/**
*
* (Multi-AZ only) A list of IDs of new virtual private cloud (VPC) route tables to associate (add) with your Amazon
* FSx for OpenZFS file system.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setAddRouteTableIds(java.util.Collection)} or {@link #withAddRouteTableIds(java.util.Collection)} if you
* want to override the existing values.
*
*
* @param addRouteTableIds
* (Multi-AZ only) A list of IDs of new virtual private cloud (VPC) route tables to associate (add) with your
* Amazon FSx for OpenZFS file system.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateFileSystemOpenZFSConfiguration withAddRouteTableIds(String... addRouteTableIds) {
if (this.addRouteTableIds == null) {
setAddRouteTableIds(new java.util.ArrayList(addRouteTableIds.length));
}
for (String ele : addRouteTableIds) {
this.addRouteTableIds.add(ele);
}
return this;
}
/**
*
* (Multi-AZ only) A list of IDs of new virtual private cloud (VPC) route tables to associate (add) with your Amazon
* FSx for OpenZFS file system.
*
*
* @param addRouteTableIds
* (Multi-AZ only) A list of IDs of new virtual private cloud (VPC) route tables to associate (add) with your
* Amazon FSx for OpenZFS file system.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateFileSystemOpenZFSConfiguration withAddRouteTableIds(java.util.Collection addRouteTableIds) {
setAddRouteTableIds(addRouteTableIds);
return this;
}
/**
*
* (Multi-AZ only) A list of IDs of existing virtual private cloud (VPC) route tables to disassociate (remove) from
* your Amazon FSx for OpenZFS file system. You can use the API operation to retrieve the list of VPC route table
* IDs for a file system.
*
*
* @return (Multi-AZ only) A list of IDs of existing virtual private cloud (VPC) route tables to disassociate
* (remove) from your Amazon FSx for OpenZFS file system. You can use the API operation to retrieve the list
* of VPC route table IDs for a file system.
*/
public java.util.List getRemoveRouteTableIds() {
return removeRouteTableIds;
}
/**
*
* (Multi-AZ only) A list of IDs of existing virtual private cloud (VPC) route tables to disassociate (remove) from
* your Amazon FSx for OpenZFS file system. You can use the API operation to retrieve the list of VPC route table
* IDs for a file system.
*
*
* @param removeRouteTableIds
* (Multi-AZ only) A list of IDs of existing virtual private cloud (VPC) route tables to disassociate
* (remove) from your Amazon FSx for OpenZFS file system. You can use the API operation to retrieve the list
* of VPC route table IDs for a file system.
*/
public void setRemoveRouteTableIds(java.util.Collection removeRouteTableIds) {
if (removeRouteTableIds == null) {
this.removeRouteTableIds = null;
return;
}
this.removeRouteTableIds = new java.util.ArrayList(removeRouteTableIds);
}
/**
*
* (Multi-AZ only) A list of IDs of existing virtual private cloud (VPC) route tables to disassociate (remove) from
* your Amazon FSx for OpenZFS file system. You can use the API operation to retrieve the list of VPC route table
* IDs for a file system.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setRemoveRouteTableIds(java.util.Collection)} or {@link #withRemoveRouteTableIds(java.util.Collection)}
* if you want to override the existing values.
*
*
* @param removeRouteTableIds
* (Multi-AZ only) A list of IDs of existing virtual private cloud (VPC) route tables to disassociate
* (remove) from your Amazon FSx for OpenZFS file system. You can use the API operation to retrieve the list
* of VPC route table IDs for a file system.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateFileSystemOpenZFSConfiguration withRemoveRouteTableIds(String... removeRouteTableIds) {
if (this.removeRouteTableIds == null) {
setRemoveRouteTableIds(new java.util.ArrayList(removeRouteTableIds.length));
}
for (String ele : removeRouteTableIds) {
this.removeRouteTableIds.add(ele);
}
return this;
}
/**
*
* (Multi-AZ only) A list of IDs of existing virtual private cloud (VPC) route tables to disassociate (remove) from
* your Amazon FSx for OpenZFS file system. You can use the API operation to retrieve the list of VPC route table
* IDs for a file system.
*
*
* @param removeRouteTableIds
* (Multi-AZ only) A list of IDs of existing virtual private cloud (VPC) route tables to disassociate
* (remove) from your Amazon FSx for OpenZFS file system. You can use the API operation to retrieve the list
* of VPC route table IDs for a file system.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateFileSystemOpenZFSConfiguration withRemoveRouteTableIds(java.util.Collection removeRouteTableIds) {
setRemoveRouteTableIds(removeRouteTableIds);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getAutomaticBackupRetentionDays() != null)
sb.append("AutomaticBackupRetentionDays: ").append(getAutomaticBackupRetentionDays()).append(",");
if (getCopyTagsToBackups() != null)
sb.append("CopyTagsToBackups: ").append(getCopyTagsToBackups()).append(",");
if (getCopyTagsToVolumes() != null)
sb.append("CopyTagsToVolumes: ").append(getCopyTagsToVolumes()).append(",");
if (getDailyAutomaticBackupStartTime() != null)
sb.append("DailyAutomaticBackupStartTime: ").append(getDailyAutomaticBackupStartTime()).append(",");
if (getThroughputCapacity() != null)
sb.append("ThroughputCapacity: ").append(getThroughputCapacity()).append(",");
if (getWeeklyMaintenanceStartTime() != null)
sb.append("WeeklyMaintenanceStartTime: ").append(getWeeklyMaintenanceStartTime()).append(",");
if (getDiskIopsConfiguration() != null)
sb.append("DiskIopsConfiguration: ").append(getDiskIopsConfiguration()).append(",");
if (getAddRouteTableIds() != null)
sb.append("AddRouteTableIds: ").append(getAddRouteTableIds()).append(",");
if (getRemoveRouteTableIds() != null)
sb.append("RemoveRouteTableIds: ").append(getRemoveRouteTableIds());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof UpdateFileSystemOpenZFSConfiguration == false)
return false;
UpdateFileSystemOpenZFSConfiguration other = (UpdateFileSystemOpenZFSConfiguration) obj;
if (other.getAutomaticBackupRetentionDays() == null ^ this.getAutomaticBackupRetentionDays() == null)
return false;
if (other.getAutomaticBackupRetentionDays() != null && other.getAutomaticBackupRetentionDays().equals(this.getAutomaticBackupRetentionDays()) == false)
return false;
if (other.getCopyTagsToBackups() == null ^ this.getCopyTagsToBackups() == null)
return false;
if (other.getCopyTagsToBackups() != null && other.getCopyTagsToBackups().equals(this.getCopyTagsToBackups()) == false)
return false;
if (other.getCopyTagsToVolumes() == null ^ this.getCopyTagsToVolumes() == null)
return false;
if (other.getCopyTagsToVolumes() != null && other.getCopyTagsToVolumes().equals(this.getCopyTagsToVolumes()) == false)
return false;
if (other.getDailyAutomaticBackupStartTime() == null ^ this.getDailyAutomaticBackupStartTime() == null)
return false;
if (other.getDailyAutomaticBackupStartTime() != null
&& other.getDailyAutomaticBackupStartTime().equals(this.getDailyAutomaticBackupStartTime()) == false)
return false;
if (other.getThroughputCapacity() == null ^ this.getThroughputCapacity() == null)
return false;
if (other.getThroughputCapacity() != null && other.getThroughputCapacity().equals(this.getThroughputCapacity()) == false)
return false;
if (other.getWeeklyMaintenanceStartTime() == null ^ this.getWeeklyMaintenanceStartTime() == null)
return false;
if (other.getWeeklyMaintenanceStartTime() != null && other.getWeeklyMaintenanceStartTime().equals(this.getWeeklyMaintenanceStartTime()) == false)
return false;
if (other.getDiskIopsConfiguration() == null ^ this.getDiskIopsConfiguration() == null)
return false;
if (other.getDiskIopsConfiguration() != null && other.getDiskIopsConfiguration().equals(this.getDiskIopsConfiguration()) == false)
return false;
if (other.getAddRouteTableIds() == null ^ this.getAddRouteTableIds() == null)
return false;
if (other.getAddRouteTableIds() != null && other.getAddRouteTableIds().equals(this.getAddRouteTableIds()) == false)
return false;
if (other.getRemoveRouteTableIds() == null ^ this.getRemoveRouteTableIds() == null)
return false;
if (other.getRemoveRouteTableIds() != null && other.getRemoveRouteTableIds().equals(this.getRemoveRouteTableIds()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getAutomaticBackupRetentionDays() == null) ? 0 : getAutomaticBackupRetentionDays().hashCode());
hashCode = prime * hashCode + ((getCopyTagsToBackups() == null) ? 0 : getCopyTagsToBackups().hashCode());
hashCode = prime * hashCode + ((getCopyTagsToVolumes() == null) ? 0 : getCopyTagsToVolumes().hashCode());
hashCode = prime * hashCode + ((getDailyAutomaticBackupStartTime() == null) ? 0 : getDailyAutomaticBackupStartTime().hashCode());
hashCode = prime * hashCode + ((getThroughputCapacity() == null) ? 0 : getThroughputCapacity().hashCode());
hashCode = prime * hashCode + ((getWeeklyMaintenanceStartTime() == null) ? 0 : getWeeklyMaintenanceStartTime().hashCode());
hashCode = prime * hashCode + ((getDiskIopsConfiguration() == null) ? 0 : getDiskIopsConfiguration().hashCode());
hashCode = prime * hashCode + ((getAddRouteTableIds() == null) ? 0 : getAddRouteTableIds().hashCode());
hashCode = prime * hashCode + ((getRemoveRouteTableIds() == null) ? 0 : getRemoveRouteTableIds().hashCode());
return hashCode;
}
@Override
public UpdateFileSystemOpenZFSConfiguration clone() {
try {
return (UpdateFileSystemOpenZFSConfiguration) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.fsx.model.transform.UpdateFileSystemOpenZFSConfigurationMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}